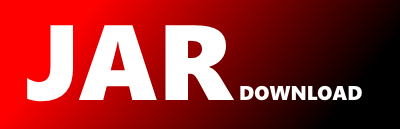
com.huaweicloud.dws.client.executor.MergeExecutor Maven / Gradle / Ivy
package com.huaweicloud.dws.client.executor;
import com.huaweicloud.dws.client.DwsConfig;
import com.huaweicloud.dws.client.exception.DwsClientException;
import com.huaweicloud.dws.client.model.Record;
import com.huaweicloud.dws.client.model.TableSchema;
import com.huaweicloud.dws.client.util.JdbcUtil;
import com.huaweicloud.dws.client.util.LocalUtil;
import com.huaweicloud.dws.client.util.LogUtil;
import lombok.extern.slf4j.Slf4j;
import java.sql.Connection;
import java.util.List;
import java.util.stream.Collectors;
/**
* @ProjectName: dws-connector
* @ClassName: MergeExecutor
* @Description: merge执行器
* @Date: 2023/1/17 17:00
* @Version: 1.0
*/
@Slf4j
public class MergeExecutor {
public void execute(List records, Connection connection, DwsConfig config, String table, String fromTable) throws DwsClientException {
long start = System.currentTimeMillis();
Record first = records.get(0);
TableSchema schema = first.getTableSchema();
List keys = schema.getPrimaryKeyNames();
List insertColumns = schema.getColumnNames().stream().filter(first::isSet).collect(Collectors.toList());
// 更新时去除主键字段 还有用户忽略的字段
boolean isUpdateAll = "upsert from ".equals(getType()) && LocalUtil.getTableConfig().isUpdateAll();
List updateColumns = insertColumns.stream().filter(key -> !(schema.isPrimaryKey(key) && !isUpdateAll) && !first.isIgnoreUpdate(key)).collect(Collectors.toList());
String mergeIntoSql = getExecuteSql(config, table, fromTable, keys, insertColumns, updateColumns);
LogUtil.withLogSwitch(config, () -> log.info("merge into sql {}", mergeIntoSql));
try {
if (config.getTableConfig(schema.getTableName()).isEnableHstoreUpsertAutocommit()) {
JdbcUtil.executeSql(connection, "set enable_hstore_upsert_autocommit=on;");
}
try {
connection.prepareStatement(mergeIntoSql).execute();
} finally {
if (config.getTableConfig(schema.getTableName()).isEnableHstoreUpsertAutocommit()) {
JdbcUtil.executeSql(connection, "set enable_hstore_upsert_autocommit=off;");
}
}
log.info("{} successful. table = {}, use time = {}, data size = {}", getType(),
schema.getTableName().getFullName(), System.currentTimeMillis() - start, records.size());
} catch (Exception e) {
log.error("{} fail. sql = {}", getType(), mergeIntoSql, e);
throw DwsClientException.fromException(e);
}
}
protected String getExecuteSql(DwsConfig config, String table, String fromTable, List keys, List insertColumns, List updateColumns) {
return JdbcUtil.getMergeIntoSql(table, fromTable, keys, updateColumns, insertColumns, config.getConflictStrategy());
}
protected String getType() {
return "merge";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy