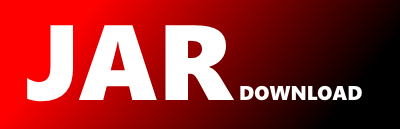
com.huaweicloud.dws.client.model.TableSchema Maven / Gradle / Ivy
package com.huaweicloud.dws.client.model;
import com.huaweicloud.dws.client.exception.InvalidException;
import java.io.Serializable;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.stream.Collectors;
/**
* @ProjectName: dws-connector
* @ClassName: TableSchema
* @Description: 表结构定义
* @Date: 2022/12/22 11:49
* @Version: 1.0
*/
public class TableSchema implements Serializable {
/**
* 表名
*/
private final TableName tableName;
/**
* 所有列
*/
private final List columns;
public TableSchema(TableName tableName, List columns) {
this.tableName = tableName;
this.columns = columns;
}
private Map columnIndex;
private Map columnNameMap;
public List getColumns() {
return columns;
}
public TableName getTableName() {
return tableName;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
TableSchema that = (TableSchema) o;
return tableName.equals(that.tableName)
&& columns.equals(that.columns);
}
@Override
public int hashCode() {
return Objects.hash(tableName, columns);
}
/**
* 主键列
*/
public List getPrimaryKeys() {
if (columns == null || columns.isEmpty()) {
return Collections.emptyList();
}
return columns.stream().filter(Column::getPrimaryKey).collect(Collectors.toList());
}
public List getPrimaryKeyNames() {
if (columns == null || columns.isEmpty()) {
return Collections.emptyList();
}
return columns.stream().filter(Column::getPrimaryKey).map(Column::getName).collect(Collectors.toList());
}
public boolean isPrimaryKey(String name) {
return Optional.ofNullable(getColumn(name)).orElseThrow(() -> new InvalidException("can not find column " + name)).getPrimaryKey();
}
public List getColumnNames() {
if (columns == null || columns.isEmpty()) {
return Collections.emptyList();
}
return columns.stream().map(Column::getName).collect(Collectors.toList());
}
public Column getColumn(String name) {
if (columnNameMap == null) {
synchronized (this) {
if (columnNameMap == null) {
Map tmp = new HashMap<>(columns.size());
for (Column column : columns) {
tmp.put(column.getName(), column);
}
columnNameMap = tmp;
}
}
}
if (!columnNameMap.containsKey(name)) {
throw new InvalidException(name);
}
return columnNameMap.get(name);
}
public Integer getColumnIndex(String name) {
if (columnIndex == null) {
synchronized (this) {
if (columnIndex == null) {
Map tmp = new HashMap<>(columns.size());
for (int i = 0; i < columns.size(); i++) {
tmp.put(columns.get(i).getName(), i);
}
columnIndex = tmp;
}
}
}
if (!columnIndex.containsKey(name)) {
throw new InvalidException(name);
}
return columnIndex.get(name);
}
@Override
public String toString() {
return "TableSchema{" +
"tableName=" + tableName +
", columns=" + columns +
'}';
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy