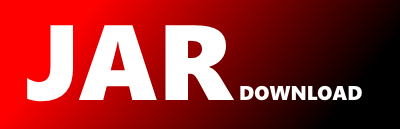
com.huaweicloud.dws.client.worker.BackgroundExecutor Maven / Gradle / Ivy
package com.huaweicloud.dws.client.worker;
import com.huaweicloud.dws.client.DwsClient;
import com.huaweicloud.dws.client.DwsConfig;
import com.huaweicloud.dws.client.collector.ActionCollector;
import com.huaweicloud.dws.client.exception.DwsClientException;
import com.huaweicloud.dws.client.exception.DwsClientRecordException;
import com.huaweicloud.dws.client.function.DwsClientExceptionFunction;
import com.huaweicloud.dws.client.util.LogUtil;
import lombok.extern.slf4j.Slf4j;
import java.io.Closeable;
import java.io.IOException;
import java.util.concurrent.atomic.AtomicBoolean;
import java.util.concurrent.atomic.AtomicReference;
/**
* @ProjectName: dws-connector
* @ClassName: BackgroundExecutor
* @Description: 后台任务执行
* @Date: 2023/1/9 19:39
* @Version: 1.0
*/
@Slf4j
public class BackgroundExecutor implements Runnable, Closeable {
private final DwsConfig config;
private final DwsClient client;
private final AtomicBoolean started;
private final ActionCollector collector;
private DwsClientException lastException;
private final AtomicBoolean running = new AtomicBoolean(true);
private final AtomicReference lastRecordException = new AtomicReference<>(null);
public BackgroundExecutor(DwsConfig config, AtomicBoolean started, ActionCollector collector, DwsClient client) {
this.config = config;
this.started = started;
this.collector = collector;
this.client = client;
}
@Override
public void run() {
while (started.get() && running.get()) {
LogUtil.withLogSwitch(config, () -> log.info("background task try to flush buffer."));
try {
tryFlush();
} catch (DwsClientRecordException recordException) {
lastRecordException.accumulateAndGet(recordException, (last, n) -> {
if (last == null) {
return n;
}
return last.merge(n);
});
} catch (DwsClientException e) {
log.error("background task flush error.", e);
lastException = e;
} catch (Throwable unknown) {
log.error("background task running error.", unknown);
}
try {
Thread.sleep(1L);
} catch (InterruptedException e) {
}
}
}
private synchronized void tryFlush() throws DwsClientException {
try {
collector.tryFlush();
} catch (DwsClientException e) {
DwsClientExceptionFunction function = config.getErrorFunction();
if (function == null) {
LogUtil.withLogSwitch(config, () -> log.info("try flush error, no exception handler "));
throw e;
}
LogUtil.withLogSwitch(config, () -> log.info("try flush error, will apply exception handler."));
function.apply(e, client);
}
}
/**
* 检查后台任务异常
*/
public void tryException() throws DwsClientException {
DwsClientException t = lastRecordException.getAndSet(null);
if (t != null) {
throw t;
}
t = lastException;
if (t != null) {
lastException = null;
throw t;
}
}
@Override
public synchronized void close() throws IOException {
running.compareAndSet(true, false);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy