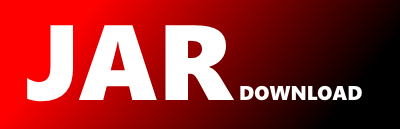
com.huaweicloud.dws.client.executor.UpsertExecutor Maven / Gradle / Ivy
package com.huaweicloud.dws.client.executor;
import com.huaweicloud.dws.client.DwsConfig;
import com.huaweicloud.dws.client.exception.DwsClientException;
import com.huaweicloud.dws.client.model.ConflictStrategy;
import com.huaweicloud.dws.client.model.Record;
import com.huaweicloud.dws.client.model.TableSchema;
import com.huaweicloud.dws.client.util.JdbcUtil;
import lombok.extern.slf4j.Slf4j;
import java.sql.Connection;
import java.util.BitSet;
import java.util.List;
import java.util.stream.Collectors;
/**
* @ProjectName: dws-connector
* @ClassName: UpsertExecutor
* @Description: 原生upsert执行
* @Date: 2023/1/16 17:37
* @Version: 1.0
*/
@Slf4j
public class UpsertExecutor {
/**
* 执行upsert
*/
public void execute(List records, Connection connection, DwsConfig config) throws DwsClientException {
if (records == null || records.isEmpty()) {
return;
}
TableSchema schema = records.get(0).getTableSchema();
Record first = records.get(0);
BitSet columnBit = first.getColumnBit();
BitSet ignoreUpdate = first.getIgnoreUpdate();
ConflictStrategy strategy = config.getConflictStrategy();
List insertFieldNames = schema.getColumnNames().stream().filter(column -> columnBit.get(schema.getColumnIndex(column))).collect(Collectors.toList());
List updateFields = insertFieldNames.stream().filter(name -> !ignoreUpdate.get(schema.getColumnIndex(name))).collect(Collectors.toList());
String upsertSql = JdbcUtil.getUpsertStatement(schema.getTableName().getFullName(), insertFieldNames, updateFields, schema.getPrimaryKeyNames(), strategy);
long startTime = System.currentTimeMillis();
try {
JdbcUtil.executeBatchRecordSql(records, connection, schema, insertFieldNames, upsertSql, config);
log.info("upsert execute end.time = {}, data size = {}, table = {}", System.currentTimeMillis() - startTime,
records.size(), schema.getTableName().getFullName());
} catch (Exception e) {
log.error("upsert executor execute fail. sql = {}, err = {}", upsertSql, e.getMessage());
throw DwsClientException.fromException(e);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy