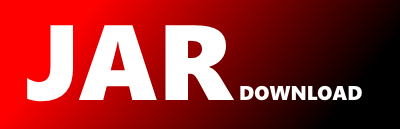
com.huaweicloud.dws.client.model.TableSchema Maven / Gradle / Ivy
package com.huaweicloud.dws.client.model;
import com.huaweicloud.dws.client.exception.InvalidException;
import com.huaweicloud.dws.client.util.IdentifierUtil;
import java.io.Serializable;
import java.util.BitSet;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.stream.Collectors;
/**
* @ProjectName: dws-connector
* @ClassName: TableSchema
* @Description: 表结构定义
* @Date: 2022/12/22 11:49
* @Version: 1.0
*/
public class TableSchema implements Serializable {
/**
* 表名
*/
private final TableName tableName;
/**
* 所有列
*/
private final List columns;
private boolean caseSensitive;
private BitSet allColumnSet;
private BitSet allNotColumnSet;
public boolean isCaseSensitive() {
return caseSensitive;
}
public TableSchema(TableName tableName, List columns) {
this(tableName, columns, false);
}
public TableSchema(TableName tableName, List columns, boolean caseSensitive) {
this.tableName = tableName;
this.columns = columns;
this.caseSensitive = caseSensitive;
init();
}
private void init() {
allColumnSet = new BitSet(columns.size());
allNotColumnSet = new BitSet(columns.size());
for (int i = 0; i < columns.size(); i++) {
allColumnSet.set(i);
}
keyColumns = columns.stream().filter(Column::getPrimaryKey).collect(Collectors.toList());
keyNames = columns.stream().filter(Column::getPrimaryKey).map(Column::getName).collect(Collectors.toList());
columnNames = columns.stream().map(Column::getName).collect(Collectors.toList());
columnNameMap = new HashMap<>(columns.size());
for (Column column : columns) {
columnNameMap.put(IdentifierUtil.toLowerIfNoSensitive(column.getName(), caseSensitive), column);
}
columnIndex = new HashMap<>(columns.size());
for (int i = 0; i < columns.size(); i++) {
columnIndex.put(IdentifierUtil.toLowerIfNoSensitive(columns.get(i).getName(), caseSensitive), i);
}
keyIndexList = keyNames.stream().map(item -> columnIndex.get(IdentifierUtil.toLowerIfNoSensitive(item, caseSensitive))).collect(Collectors.toList());
}
private Map columnIndex;
private Map columnNameMap;
private List keyIndexList;
private List keyColumns;
private List keyNames;
private List columnNames;
public List getColumns() {
return columns;
}
public TableName getTableName() {
return tableName;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
TableSchema that = (TableSchema) o;
return tableName.equals(that.tableName)
&& columns.equals(that.columns);
}
@Override
public int hashCode() {
return Objects.hash(tableName, columns);
}
/**
* 主键列
*/
public List getPrimaryKeys() {
return keyColumns;
}
public List getPrimaryKeyNames() {
return keyNames;
}
public boolean isPrimaryKey(String name) {
return Optional.ofNullable(getColumn(name)).orElseThrow(() -> new InvalidException("can not find column " + name)).getPrimaryKey();
}
public List getKeyIndexList() {
return keyIndexList;
}
public List getColumnNames() {
return columnNames;
}
public Column getColumn(String name) {
name = IdentifierUtil.toLowerIfNoSensitive(name, caseSensitive);
if (!columnNameMap.containsKey(name)) {
throw new InvalidException(String.format("column not exist in table %s: %s", tableName.getFullName(), name));
}
return columnNameMap.get(name);
}
public Column getColumn(Integer index) {
if (index < 0) {
throw new InvalidException(String.format("column index %s cannot be less than 0.", index));
}
if (index >= columns.size()) {
throw new InvalidException(String.format("column index not exist in table %s: %s",
tableName.getFullName(), index));
}
return columns.get(index);
}
public Integer getColumnIndex(String name) {
name = IdentifierUtil.toLowerIfNoSensitive(name, caseSensitive);
Integer idx = columnIndex.get(name);
if (idx == null) {
throw new InvalidException(String.format("column not exist in table %s: %s", tableName.getFullName(), name));
}
return idx;
}
public Map getColumnIndexMap() {
return columnIndex;
}
public BitSet getAllColumnSet() {
return allColumnSet;
}
public BitSet getAllNotColumnSet() {
return allNotColumnSet;
}
@Override
public String toString() {
return "TableSchema{" +
"tableName=" + tableName +
", columns=" + columns +
'}';
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy