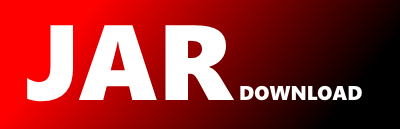
com.huaweicloud.dws.client.op.Get Maven / Gradle / Ivy
package com.huaweicloud.dws.client.op;
import com.huaweicloud.dws.client.action.GetAction;
import com.huaweicloud.dws.client.collector.ActionCollector;
import com.huaweicloud.dws.client.exception.DwsClientException;
import com.huaweicloud.dws.client.exception.ExceptionCode;
import com.huaweicloud.dws.client.exception.InvalidException;
import com.huaweicloud.dws.client.model.Column;
import com.huaweicloud.dws.client.model.OperationType;
import com.huaweicloud.dws.client.model.Record;
import com.huaweicloud.dws.client.model.TableSchema;
import com.huaweicloud.dws.client.util.AssertUtil;
import com.huaweicloud.dws.client.util.LogUtil;
import com.huaweicloud.dws.client.worker.ExecutionPool;
import lombok.extern.slf4j.Slf4j;
import java.security.InvalidParameterException;
import java.util.Collections;
import java.util.List;
import java.util.concurrent.CompletableFuture;
/**
* @ProjectName: dws-client
* @Description: Get operation
* schema 主键必须与 lookup keys 一一对应
* like:
* primaryKeys = [id, name]
* lookupKeys = [id, name]
* @Date: 2023/09/27
*/
@Slf4j
public class Get extends Operate {
CompletableFuture future = new CompletableFuture<>();
// get操作提交到dws的时间
long startTime = System.nanoTime();
boolean isFullColumn;
public Get(TableSchema schema, ActionCollector collector, boolean isFullColumn) {
super(schema, collector, OperationType.READ);
this.isFullColumn = isFullColumn;
}
public Get(Record record, ActionCollector collector, boolean isFullColumn) {
super(record, collector);
this.isFullColumn = isFullColumn;
}
public boolean isFullColumn() {
return isFullColumn;
}
public long getStartTime() {
return startTime;
}
public CompletableFuture getFuture() {
return future;
}
public static Builder newBuilder(TableSchema schema, ActionCollector collector) {
return new Builder(schema, collector);
}
public static void check(Get get) throws DwsClientException {
if (get == null) {
throw new DwsClientException(ExceptionCode.CONSTRAINT_VIOLATION, "Get cannot be null");
}
if (get.getRecord().getTableSchema().getPrimaryKeys().size() == 0) {
throw new DwsClientException(ExceptionCode.CONSTRAINT_VIOLATION, "Get table must have primary key:" + get.getRecord().getTableSchema().getTableName().getFullName());
}
for (int index : get.getRecord().getTableSchema().getKeyIndexList()) {
if (get.getRecord().getValue(index) == null || !get.getRecord().isSet(index)) {
throw new DwsClientException(ExceptionCode.CONSTRAINT_VIOLATION, "Get primary key cannot be null:" + get.getRecord().getTableSchema().getColumns().get(index).getName());
}
}
}
@Override
public void commit() throws DwsClientException {
AssertUtil.isTrue(record.getColumnBit().length() != 0, new InvalidException("must set column"));
((ActionCollector) collector).appendGet(this);
collector.getPool().tryException();
}
/**
* 同步提交
*/
@Override
public void syncCommit() throws DwsClientException {
List getList = Collections.singletonList(this);
GetAction action = new GetAction(getList, collector.getConfig());
ExecutionPool pool = collector.getPool();
pool.tryException();
while (!collector.getPool().submit(action)) {
LogUtil.withLogSwitch(collector.getConfig(), () -> log.warn("try submit."));
}
// 等待结果响应后返回
action.getResult();
}
/**
* Builder of Get request.
*/
public static class Builder {
private final TableSchema schema;
private final Record record;
private final ActionCollector collector;
private boolean isFullColumn = true;
public Builder(TableSchema schema, ActionCollector collector) {
this.schema = schema;
this.collector = collector;
this.record = new Record(schema, OperationType.READ);
}
public Builder setPrimaryKeys(List columns, List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy