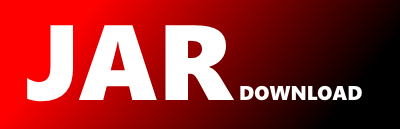
com.huaweicloud.dws.client.op.Scan Maven / Gradle / Ivy
package com.huaweicloud.dws.client.op;
import static com.huaweicloud.dws.client.model.OperationType.READ;
import com.huaweicloud.dws.client.model.Record;
import com.huaweicloud.dws.client.model.TableSchema;
import java.security.InvalidParameterException;
import java.util.BitSet;
import java.util.List;
/**
* @ProjectName: dws-client
* @Description: Scan operation
* 相比 Scan operation,SCAN 兼容了 schema 主键与 lookup keys 不一致的情况
* like:
* primaryKeys = [id]
* lookupKeys = [id, name] or []
* @Date: 2023/09/27
*/
public class Scan extends Operate {
private final BitSet selectedColumns;
private final int fetchSize;
private final int timeout;
protected Scan(TableSchema schema, BitSet selectedColumns, int fetchSize, int timeout) {
super(schema, null, READ);
this.selectedColumns = selectedColumns;
this.fetchSize = fetchSize;
this.timeout = timeout;
}
protected Scan(Record record, BitSet selectedColumns, int fetchSize, int timeout) {
super(record, null);
this.selectedColumns = selectedColumns;
this.fetchSize = fetchSize;
this.timeout = timeout;
}
public BitSet getSelectedColumns() {
return selectedColumns;
}
public int getTimeout() {
return timeout;
}
public int getFetchSize() {
return fetchSize;
}
public static Builder newBuilder(TableSchema schema) {
return new Builder(schema);
}
public static class Builder {
private final TableSchema schema;
private final Record record;
private BitSet selectedColumns = null;
private int fetchSize = -1;
private int timeout = -1;
public Builder(TableSchema schema) {
this.schema = schema;
this.record = new Record(schema, READ);
}
public Builder setLookUpKeys(List columns, List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy