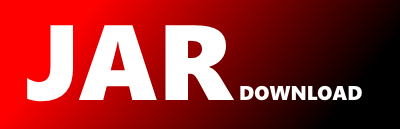
com.huaweicloud.dws.client.op.Write Maven / Gradle / Ivy
package com.huaweicloud.dws.client.op;
import com.huaweicloud.dws.client.TableConfig;
import com.huaweicloud.dws.client.collector.ITableCollector;
import com.huaweicloud.dws.client.exception.DwsClientException;
import com.huaweicloud.dws.client.exception.InvalidException;
import com.huaweicloud.dws.client.model.ConflictStrategy;
import com.huaweicloud.dws.client.model.OperationType;
import com.huaweicloud.dws.client.model.TableSchema;
import com.huaweicloud.dws.client.model.WriteMode;
/**
* @ProjectName: dws-connector
* @ClassName: Upsert
* @Description:
* @Date: 2023/1/14 20:03
* @Version: 1.0
*/
public class Write extends Operate {
protected final TableSchema schema;
protected TableConfig tableConfig;
public Write(TableSchema schema, ITableCollector collector) {
this(schema, collector, collector.getTableConfig());
}
public Write(TableSchema schema, ITableCollector collector, TableConfig config) {
super(schema, collector, OperationType.WRITE);
this.tableConfig = config;
this.schema = schema;
}
public Write(TableSchema schema, ITableCollector collector, TableConfig config, Object[] values, IConvert convert) {
super(schema, collector, OperationType.WRITE, values, convert);
this.tableConfig = config;
this.schema = schema;
}
/**
* 有主键 && 替换模式下,设置用户未设置的字段为null, 便于后续流程执行
*/
protected void fillRecord() {
if (tableConfig.getConflictStrategy() == ConflictStrategy.INSERT_OR_REPLACE
&& !schema.getPrimaryKeys().isEmpty()) {
for (int i = 0; i < schema.getColumns().size(); i++) {
if (record.isSet(i)) {
continue;
}
record.setValue(i, null, false);
}
}
}
protected void checkRecord() {
// 安全模式下不需要检查,直接信任
if (tableConfig.isSecurityMode()) {
return;
}
// update模式下必须主键全填充
WriteMode writeMode = tableConfig.getWriteMode();
if (WriteMode.updateWrites().contains(writeMode)) {
// update 需要检查主键是否全覆盖
for (Integer key : schema.getKeyIndexList()) {
if (record.isSet(key)) {
continue;
}
throw new InvalidException("update must set primary key " + schema.getColumn(key).getName());
}
}
}
@Override
public void commit() throws DwsClientException {
checkRecord();
fillRecord();
super.commit();
}
/**
* 同步提交
*/
@Override
public void syncCommit() throws DwsClientException {
checkRecord();
fillRecord();
super.syncCommit();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy