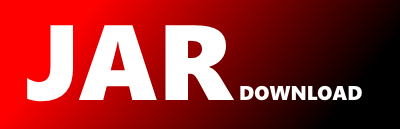
com.hubspot.chrome.devtools.base.ChromeResponse Maven / Gradle / Ivy
package com.hubspot.chrome.devtools.base;
import com.fasterxml.jackson.annotation.JsonAutoDetect;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.databind.JsonNode;
import com.sun.istack.internal.Nullable;
import java.util.Objects;
import javax.annotation.Generated;
/**
* Immutable implementation of {@link ChromeResponseIF}.
*
* Use the builder to create immutable instances:
* {@code ChromeResponse.builder()}.
*/
@SuppressWarnings({"all"})
@Generated({"Immutables.generator", "ChromeResponseIF"})
public final class ChromeResponse implements ChromeResponseIF {
private final @Nullable Integer id;
private final @Nullable JsonNode result;
private final @Nullable String method;
private final @Nullable JsonNode params;
private final @Nullable ChromeResponseErrorBody error;
private ChromeResponse(
@Nullable Integer id,
@Nullable JsonNode result,
@Nullable String method,
@Nullable JsonNode params,
@Nullable ChromeResponseErrorBody error) {
this.id = id;
this.result = result;
this.method = method;
this.params = params;
this.error = error;
}
/**
* @return The value of the {@code id} attribute
*/
@JsonProperty("id")
@Override
public @Nullable Integer getId() {
return id;
}
/**
* @return The value of the {@code result} attribute
*/
@JsonProperty("result")
@Override
public @Nullable JsonNode getResult() {
return result;
}
/**
* @return The value of the {@code method} attribute
*/
@JsonProperty("method")
@Override
public @Nullable String getMethod() {
return method;
}
/**
* @return The value of the {@code params} attribute
*/
@JsonProperty("params")
@Override
public @Nullable JsonNode getParams() {
return params;
}
/**
* @return The value of the {@code error} attribute
*/
@JsonProperty("error")
@Override
public @Nullable ChromeResponseErrorBody getError() {
return error;
}
/**
* Copy the current immutable object by setting a value for the {@link ChromeResponseIF#getId() id} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for id (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ChromeResponse withId(@Nullable Integer value) {
if (Objects.equals(this.id, value)) return this;
return new ChromeResponse(value, this.result, this.method, this.params, this.error);
}
/**
* Copy the current immutable object by setting a value for the {@link ChromeResponseIF#getResult() result} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for result (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ChromeResponse withResult(@Nullable JsonNode value) {
if (this.result == value) return this;
return new ChromeResponse(this.id, value, this.method, this.params, this.error);
}
/**
* Copy the current immutable object by setting a value for the {@link ChromeResponseIF#getMethod() method} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for method (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ChromeResponse withMethod(@Nullable String value) {
if (Objects.equals(this.method, value)) return this;
return new ChromeResponse(this.id, this.result, value, this.params, this.error);
}
/**
* Copy the current immutable object by setting a value for the {@link ChromeResponseIF#getParams() params} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for params (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ChromeResponse withParams(@Nullable JsonNode value) {
if (this.params == value) return this;
return new ChromeResponse(this.id, this.result, this.method, value, this.error);
}
/**
* Copy the current immutable object by setting a value for the {@link ChromeResponseIF#getError() error} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for error (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ChromeResponse withError(@Nullable ChromeResponseErrorBody value) {
if (this.error == value) return this;
return new ChromeResponse(this.id, this.result, this.method, this.params, value);
}
/**
* This instance is equal to all instances of {@code ChromeResponse} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(Object another) {
if (this == another) return true;
return another instanceof ChromeResponse
&& equalTo((ChromeResponse) another);
}
private boolean equalTo(ChromeResponse another) {
return Objects.equals(id, another.id)
&& Objects.equals(result, another.result)
&& Objects.equals(method, another.method)
&& Objects.equals(params, another.params)
&& Objects.equals(error, another.error);
}
/**
* Computes a hash code from attributes: {@code id}, {@code result}, {@code method}, {@code params}, {@code error}.
* @return hashCode value
*/
@Override
public int hashCode() {
int h = 5381;
h += (h << 5) + Objects.hashCode(id);
h += (h << 5) + Objects.hashCode(result);
h += (h << 5) + Objects.hashCode(method);
h += (h << 5) + Objects.hashCode(params);
h += (h << 5) + Objects.hashCode(error);
return h;
}
/**
* Prints the immutable value {@code ChromeResponse} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
return "ChromeResponse{"
+ "id=" + id
+ ", result=" + result
+ ", method=" + method
+ ", params=" + params
+ ", error=" + error
+ "}";
}
/**
* Utility type used to correctly read immutable object from JSON representation.
* @deprecated Do not use this type directly, it exists only for the Jackson-binding infrastructure
*/
@Deprecated
@JsonAutoDetect(fieldVisibility = JsonAutoDetect.Visibility.NONE)
static final class Json implements ChromeResponseIF {
Integer id;
JsonNode result;
String method;
JsonNode params;
ChromeResponseErrorBody error;
@JsonProperty("id")
public void setId(@Nullable Integer id) {
this.id = id;
}
@JsonProperty("result")
public void setResult(@Nullable JsonNode result) {
this.result = result;
}
@JsonProperty("method")
public void setMethod(@Nullable String method) {
this.method = method;
}
@JsonProperty("params")
public void setParams(@Nullable JsonNode params) {
this.params = params;
}
@JsonProperty("error")
public void setError(@Nullable ChromeResponseErrorBody error) {
this.error = error;
}
@Override
public Integer getId() { throw new UnsupportedOperationException(); }
@Override
public JsonNode getResult() { throw new UnsupportedOperationException(); }
@Override
public String getMethod() { throw new UnsupportedOperationException(); }
@Override
public JsonNode getParams() { throw new UnsupportedOperationException(); }
@Override
public ChromeResponseErrorBody getError() { throw new UnsupportedOperationException(); }
}
/**
* @param json A JSON-bindable data structure
* @return An immutable value type
* @deprecated Do not use this method directly, it exists only for the Jackson-binding infrastructure
*/
@Deprecated
@JsonCreator(mode = JsonCreator.Mode.DELEGATING)
static ChromeResponse fromJson(Json json) {
ChromeResponse.Builder builder = ChromeResponse.builder();
if (json.id != null) {
builder.setId(json.id);
}
if (json.result != null) {
builder.setResult(json.result);
}
if (json.method != null) {
builder.setMethod(json.method);
}
if (json.params != null) {
builder.setParams(json.params);
}
if (json.error != null) {
builder.setError(json.error);
}
return builder.build();
}
/**
* Creates an immutable copy of a {@link ChromeResponseIF} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable ChromeResponse instance
*/
public static ChromeResponse copyOf(ChromeResponseIF instance) {
if (instance instanceof ChromeResponse) {
return (ChromeResponse) instance;
}
return ChromeResponse.builder()
.from(instance)
.build();
}
/**
* Creates a builder for {@link ChromeResponse ChromeResponse}.
* @return A new ChromeResponse builder
*/
public static ChromeResponse.Builder builder() {
return new ChromeResponse.Builder();
}
/**
* Builds instances of type {@link ChromeResponse ChromeResponse}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
*
{@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
public static final class Builder {
private Integer id;
private JsonNode result;
private String method;
private JsonNode params;
private ChromeResponseErrorBody error;
private Builder() {
}
/**
* Fill a builder with attribute values from the provided {@code ChromeResponseIF} instance.
* Regular attribute values will be replaced with those from the given instance.
* Absent optional values will not replace present values.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(ChromeResponseIF instance) {
Objects.requireNonNull(instance, "instance");
@Nullable Integer idValue = instance.getId();
if (idValue != null) {
setId(idValue);
}
@Nullable JsonNode resultValue = instance.getResult();
if (resultValue != null) {
setResult(resultValue);
}
@Nullable String methodValue = instance.getMethod();
if (methodValue != null) {
setMethod(methodValue);
}
@Nullable JsonNode paramsValue = instance.getParams();
if (paramsValue != null) {
setParams(paramsValue);
}
@Nullable ChromeResponseErrorBody errorValue = instance.getError();
if (errorValue != null) {
setError(errorValue);
}
return this;
}
/**
* Initializes the value for the {@link ChromeResponseIF#getId() id} attribute.
* @param id The value for id (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setId(@Nullable Integer id) {
this.id = id;
return this;
}
/**
* Initializes the value for the {@link ChromeResponseIF#getResult() result} attribute.
* @param result The value for result (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setResult(@Nullable JsonNode result) {
this.result = result;
return this;
}
/**
* Initializes the value for the {@link ChromeResponseIF#getMethod() method} attribute.
* @param method The value for method (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setMethod(@Nullable String method) {
this.method = method;
return this;
}
/**
* Initializes the value for the {@link ChromeResponseIF#getParams() params} attribute.
* @param params The value for params (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setParams(@Nullable JsonNode params) {
this.params = params;
return this;
}
/**
* Initializes the value for the {@link ChromeResponseIF#getError() error} attribute.
* @param error The value for error (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setError(@Nullable ChromeResponseErrorBody error) {
this.error = error;
return this;
}
/**
* Builds a new {@link ChromeResponse ChromeResponse}.
* @return An immutable instance of ChromeResponse
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
public ChromeResponse build() {
return new ChromeResponse(id, result, method, params, error);
}
}
}