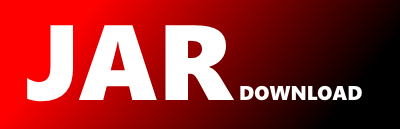
com.hubspot.jinjava.interpret.ContextConfiguration Maven / Gradle / Ivy
Show all versions of jinjava Show documentation
package com.hubspot.jinjava.interpret;
import com.fasterxml.jackson.annotation.JsonAutoDetect;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.google.common.base.MoreObjects;
import com.google.common.primitives.Booleans;
import com.hubspot.immutables.validation.InvalidImmutableStateException;
import com.hubspot.jinjava.lib.expression.ExpressionStrategy;
import edu.umd.cs.findbugs.annotations.SuppressFBWarnings;
import java.util.ArrayList;
import java.util.List;
import java.util.Objects;
import javax.annotation.Nullable;
import javax.annotation.ParametersAreNonnullByDefault;
import javax.annotation.concurrent.Immutable;
import javax.annotation.concurrent.NotThreadSafe;
import org.immutables.value.Generated;
/**
* Immutable implementation of {@link ContextConfigurationIF}.
*
* Use the builder to create immutable instances:
* {@code ContextConfiguration.builder()}.
* Use the static factory method to get the default singleton instance:
* {@code ContextConfiguration.of()}.
*/
@Generated(from = "ContextConfigurationIF", generator = "Immutables")
@SuppressWarnings({"all"})
@SuppressFBWarnings
@ParametersAreNonnullByDefault
@Immutable
public final class ContextConfiguration implements ContextConfigurationIF {
private final ExpressionStrategy expressionStrategy;
private final @Nullable DynamicVariableResolver dynamicVariableResolver;
private final boolean validationMode;
private final boolean deferredExecutionMode;
private final boolean deferLargeObjects;
private final boolean throwInterpreterErrors;
private final boolean partialMacroEvaluation;
private final boolean unwrapRawOverride;
private ContextConfiguration() {
this.dynamicVariableResolver = null;
this.expressionStrategy = initShim.getExpressionStrategy();
this.validationMode = initShim.isValidationMode();
this.deferredExecutionMode = initShim.isDeferredExecutionMode();
this.deferLargeObjects = initShim.isDeferLargeObjects();
this.throwInterpreterErrors = initShim.isThrowInterpreterErrors();
this.partialMacroEvaluation = initShim.isPartialMacroEvaluation();
this.unwrapRawOverride = initShim.isUnwrapRawOverride();
this.initShim = null;
}
private ContextConfiguration(ContextConfiguration.Builder builder) {
this.dynamicVariableResolver = builder.dynamicVariableResolver;
if (builder.expressionStrategy != null) {
initShim.setExpressionStrategy(builder.expressionStrategy);
}
if (builder.validationModeIsSet()) {
initShim.setValidationMode(builder.validationMode);
}
if (builder.deferredExecutionModeIsSet()) {
initShim.setDeferredExecutionMode(builder.deferredExecutionMode);
}
if (builder.deferLargeObjectsIsSet()) {
initShim.setDeferLargeObjects(builder.deferLargeObjects);
}
if (builder.throwInterpreterErrorsIsSet()) {
initShim.setThrowInterpreterErrors(builder.throwInterpreterErrors);
}
if (builder.partialMacroEvaluationIsSet()) {
initShim.setPartialMacroEvaluation(builder.partialMacroEvaluation);
}
if (builder.unwrapRawOverrideIsSet()) {
initShim.setUnwrapRawOverride(builder.unwrapRawOverride);
}
this.expressionStrategy = initShim.getExpressionStrategy();
this.validationMode = initShim.isValidationMode();
this.deferredExecutionMode = initShim.isDeferredExecutionMode();
this.deferLargeObjects = initShim.isDeferLargeObjects();
this.throwInterpreterErrors = initShim.isThrowInterpreterErrors();
this.partialMacroEvaluation = initShim.isPartialMacroEvaluation();
this.unwrapRawOverride = initShim.isUnwrapRawOverride();
this.initShim = null;
}
private ContextConfiguration(
ExpressionStrategy expressionStrategy,
@Nullable DynamicVariableResolver dynamicVariableResolver,
boolean validationMode,
boolean deferredExecutionMode,
boolean deferLargeObjects,
boolean throwInterpreterErrors,
boolean partialMacroEvaluation,
boolean unwrapRawOverride) {
this.expressionStrategy = expressionStrategy;
this.dynamicVariableResolver = dynamicVariableResolver;
this.validationMode = validationMode;
this.deferredExecutionMode = deferredExecutionMode;
this.deferLargeObjects = deferLargeObjects;
this.throwInterpreterErrors = throwInterpreterErrors;
this.partialMacroEvaluation = partialMacroEvaluation;
this.unwrapRawOverride = unwrapRawOverride;
this.initShim = null;
}
private static final byte STAGE_INITIALIZING = -1;
private static final byte STAGE_UNINITIALIZED = 0;
private static final byte STAGE_INITIALIZED = 1;
private transient volatile InitShim initShim = new InitShim();
@Generated(from = "ContextConfigurationIF", generator = "Immutables")
private final class InitShim {
private byte expressionStrategyBuildStage = STAGE_UNINITIALIZED;
private ExpressionStrategy expressionStrategy;
ExpressionStrategy getExpressionStrategy() {
if (expressionStrategyBuildStage == STAGE_INITIALIZING) throw new InvalidImmutableStateException(formatInitCycleMessage());
if (expressionStrategyBuildStage == STAGE_UNINITIALIZED) {
expressionStrategyBuildStage = STAGE_INITIALIZING;
this.expressionStrategy = Objects.requireNonNull(getExpressionStrategyInitialize(), "expressionStrategy");
expressionStrategyBuildStage = STAGE_INITIALIZED;
}
return this.expressionStrategy;
}
void setExpressionStrategy(ExpressionStrategy expressionStrategy) {
this.expressionStrategy = expressionStrategy;
expressionStrategyBuildStage = STAGE_INITIALIZED;
}
private byte validationModeBuildStage = STAGE_UNINITIALIZED;
private boolean validationMode;
boolean isValidationMode() {
if (validationModeBuildStage == STAGE_INITIALIZING) throw new InvalidImmutableStateException(formatInitCycleMessage());
if (validationModeBuildStage == STAGE_UNINITIALIZED) {
validationModeBuildStage = STAGE_INITIALIZING;
this.validationMode = isValidationModeInitialize();
validationModeBuildStage = STAGE_INITIALIZED;
}
return this.validationMode;
}
void setValidationMode(boolean validationMode) {
this.validationMode = validationMode;
validationModeBuildStage = STAGE_INITIALIZED;
}
private byte deferredExecutionModeBuildStage = STAGE_UNINITIALIZED;
private boolean deferredExecutionMode;
boolean isDeferredExecutionMode() {
if (deferredExecutionModeBuildStage == STAGE_INITIALIZING) throw new InvalidImmutableStateException(formatInitCycleMessage());
if (deferredExecutionModeBuildStage == STAGE_UNINITIALIZED) {
deferredExecutionModeBuildStage = STAGE_INITIALIZING;
this.deferredExecutionMode = isDeferredExecutionModeInitialize();
deferredExecutionModeBuildStage = STAGE_INITIALIZED;
}
return this.deferredExecutionMode;
}
void setDeferredExecutionMode(boolean deferredExecutionMode) {
this.deferredExecutionMode = deferredExecutionMode;
deferredExecutionModeBuildStage = STAGE_INITIALIZED;
}
private byte deferLargeObjectsBuildStage = STAGE_UNINITIALIZED;
private boolean deferLargeObjects;
boolean isDeferLargeObjects() {
if (deferLargeObjectsBuildStage == STAGE_INITIALIZING) throw new InvalidImmutableStateException(formatInitCycleMessage());
if (deferLargeObjectsBuildStage == STAGE_UNINITIALIZED) {
deferLargeObjectsBuildStage = STAGE_INITIALIZING;
this.deferLargeObjects = isDeferLargeObjectsInitialize();
deferLargeObjectsBuildStage = STAGE_INITIALIZED;
}
return this.deferLargeObjects;
}
void setDeferLargeObjects(boolean deferLargeObjects) {
this.deferLargeObjects = deferLargeObjects;
deferLargeObjectsBuildStage = STAGE_INITIALIZED;
}
private byte throwInterpreterErrorsBuildStage = STAGE_UNINITIALIZED;
private boolean throwInterpreterErrors;
boolean isThrowInterpreterErrors() {
if (throwInterpreterErrorsBuildStage == STAGE_INITIALIZING) throw new InvalidImmutableStateException(formatInitCycleMessage());
if (throwInterpreterErrorsBuildStage == STAGE_UNINITIALIZED) {
throwInterpreterErrorsBuildStage = STAGE_INITIALIZING;
this.throwInterpreterErrors = isThrowInterpreterErrorsInitialize();
throwInterpreterErrorsBuildStage = STAGE_INITIALIZED;
}
return this.throwInterpreterErrors;
}
void setThrowInterpreterErrors(boolean throwInterpreterErrors) {
this.throwInterpreterErrors = throwInterpreterErrors;
throwInterpreterErrorsBuildStage = STAGE_INITIALIZED;
}
private byte partialMacroEvaluationBuildStage = STAGE_UNINITIALIZED;
private boolean partialMacroEvaluation;
boolean isPartialMacroEvaluation() {
if (partialMacroEvaluationBuildStage == STAGE_INITIALIZING) throw new InvalidImmutableStateException(formatInitCycleMessage());
if (partialMacroEvaluationBuildStage == STAGE_UNINITIALIZED) {
partialMacroEvaluationBuildStage = STAGE_INITIALIZING;
this.partialMacroEvaluation = isPartialMacroEvaluationInitialize();
partialMacroEvaluationBuildStage = STAGE_INITIALIZED;
}
return this.partialMacroEvaluation;
}
void setPartialMacroEvaluation(boolean partialMacroEvaluation) {
this.partialMacroEvaluation = partialMacroEvaluation;
partialMacroEvaluationBuildStage = STAGE_INITIALIZED;
}
private byte unwrapRawOverrideBuildStage = STAGE_UNINITIALIZED;
private boolean unwrapRawOverride;
boolean isUnwrapRawOverride() {
if (unwrapRawOverrideBuildStage == STAGE_INITIALIZING) throw new InvalidImmutableStateException(formatInitCycleMessage());
if (unwrapRawOverrideBuildStage == STAGE_UNINITIALIZED) {
unwrapRawOverrideBuildStage = STAGE_INITIALIZING;
this.unwrapRawOverride = isUnwrapRawOverrideInitialize();
unwrapRawOverrideBuildStage = STAGE_INITIALIZED;
}
return this.unwrapRawOverride;
}
void setUnwrapRawOverride(boolean unwrapRawOverride) {
this.unwrapRawOverride = unwrapRawOverride;
unwrapRawOverrideBuildStage = STAGE_INITIALIZED;
}
private String formatInitCycleMessage() {
List attributes = new ArrayList<>();
if (expressionStrategyBuildStage == STAGE_INITIALIZING) attributes.add("expressionStrategy");
if (validationModeBuildStage == STAGE_INITIALIZING) attributes.add("validationMode");
if (deferredExecutionModeBuildStage == STAGE_INITIALIZING) attributes.add("deferredExecutionMode");
if (deferLargeObjectsBuildStage == STAGE_INITIALIZING) attributes.add("deferLargeObjects");
if (throwInterpreterErrorsBuildStage == STAGE_INITIALIZING) attributes.add("throwInterpreterErrors");
if (partialMacroEvaluationBuildStage == STAGE_INITIALIZING) attributes.add("partialMacroEvaluation");
if (unwrapRawOverrideBuildStage == STAGE_INITIALIZING) attributes.add("unwrapRawOverride");
return "Cannot build ContextConfiguration, attribute initializers form cycle " + attributes;
}
}
private ExpressionStrategy getExpressionStrategyInitialize() {
return ContextConfigurationIF.super.getExpressionStrategy();
}
private boolean isValidationModeInitialize() {
return ContextConfigurationIF.super.isValidationMode();
}
private boolean isDeferredExecutionModeInitialize() {
return ContextConfigurationIF.super.isDeferredExecutionMode();
}
private boolean isDeferLargeObjectsInitialize() {
return ContextConfigurationIF.super.isDeferLargeObjects();
}
private boolean isThrowInterpreterErrorsInitialize() {
return ContextConfigurationIF.super.isThrowInterpreterErrors();
}
private boolean isPartialMacroEvaluationInitialize() {
return ContextConfigurationIF.super.isPartialMacroEvaluation();
}
private boolean isUnwrapRawOverrideInitialize() {
return ContextConfigurationIF.super.isUnwrapRawOverride();
}
/**
* @return The value of the {@code expressionStrategy} attribute
*/
@JsonProperty
@Override
public ExpressionStrategy getExpressionStrategy() {
InitShim shim = this.initShim;
return shim != null
? shim.getExpressionStrategy()
: this.expressionStrategy;
}
/**
* @return The value of the {@code dynamicVariableResolver} attribute
*/
@JsonProperty
@Override
public @Nullable DynamicVariableResolver getDynamicVariableResolver() {
return dynamicVariableResolver;
}
/**
* @return The value of the {@code validationMode} attribute
*/
@JsonProperty
@Override
public boolean isValidationMode() {
InitShim shim = this.initShim;
return shim != null
? shim.isValidationMode()
: this.validationMode;
}
/**
* @return The value of the {@code deferredExecutionMode} attribute
*/
@JsonProperty
@Override
public boolean isDeferredExecutionMode() {
InitShim shim = this.initShim;
return shim != null
? shim.isDeferredExecutionMode()
: this.deferredExecutionMode;
}
/**
* @return The value of the {@code deferLargeObjects} attribute
*/
@JsonProperty
@Override
public boolean isDeferLargeObjects() {
InitShim shim = this.initShim;
return shim != null
? shim.isDeferLargeObjects()
: this.deferLargeObjects;
}
/**
* @return The value of the {@code throwInterpreterErrors} attribute
*/
@JsonProperty
@Override
public boolean isThrowInterpreterErrors() {
InitShim shim = this.initShim;
return shim != null
? shim.isThrowInterpreterErrors()
: this.throwInterpreterErrors;
}
/**
* @return The value of the {@code partialMacroEvaluation} attribute
*/
@JsonProperty
@Override
public boolean isPartialMacroEvaluation() {
InitShim shim = this.initShim;
return shim != null
? shim.isPartialMacroEvaluation()
: this.partialMacroEvaluation;
}
/**
* @return The value of the {@code unwrapRawOverride} attribute
*/
@JsonProperty
@Override
public boolean isUnwrapRawOverride() {
InitShim shim = this.initShim;
return shim != null
? shim.isUnwrapRawOverride()
: this.unwrapRawOverride;
}
/**
* Copy the current immutable object by setting a value for the {@link ContextConfigurationIF#getExpressionStrategy() expressionStrategy} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for expressionStrategy
* @return A modified copy of the {@code this} object
*/
public final ContextConfiguration withExpressionStrategy(ExpressionStrategy value) {
if (this.expressionStrategy == value) return this;
ExpressionStrategy newValue = Objects.requireNonNull(value, "expressionStrategy");
return validate(new ContextConfiguration(
newValue,
this.dynamicVariableResolver,
this.validationMode,
this.deferredExecutionMode,
this.deferLargeObjects,
this.throwInterpreterErrors,
this.partialMacroEvaluation,
this.unwrapRawOverride));
}
/**
* Copy the current immutable object by setting a value for the {@link ContextConfigurationIF#getDynamicVariableResolver() dynamicVariableResolver} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for dynamicVariableResolver (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ContextConfiguration withDynamicVariableResolver(@Nullable DynamicVariableResolver value) {
if (this.dynamicVariableResolver == value) return this;
return validate(new ContextConfiguration(
this.expressionStrategy,
value,
this.validationMode,
this.deferredExecutionMode,
this.deferLargeObjects,
this.throwInterpreterErrors,
this.partialMacroEvaluation,
this.unwrapRawOverride));
}
/**
* Copy the current immutable object by setting a value for the {@link ContextConfigurationIF#isValidationMode() validationMode} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for validationMode
* @return A modified copy of the {@code this} object
*/
public final ContextConfiguration withValidationMode(boolean value) {
if (this.validationMode == value) return this;
return validate(new ContextConfiguration(
this.expressionStrategy,
this.dynamicVariableResolver,
value,
this.deferredExecutionMode,
this.deferLargeObjects,
this.throwInterpreterErrors,
this.partialMacroEvaluation,
this.unwrapRawOverride));
}
/**
* Copy the current immutable object by setting a value for the {@link ContextConfigurationIF#isDeferredExecutionMode() deferredExecutionMode} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for deferredExecutionMode
* @return A modified copy of the {@code this} object
*/
public final ContextConfiguration withDeferredExecutionMode(boolean value) {
if (this.deferredExecutionMode == value) return this;
return validate(new ContextConfiguration(
this.expressionStrategy,
this.dynamicVariableResolver,
this.validationMode,
value,
this.deferLargeObjects,
this.throwInterpreterErrors,
this.partialMacroEvaluation,
this.unwrapRawOverride));
}
/**
* Copy the current immutable object by setting a value for the {@link ContextConfigurationIF#isDeferLargeObjects() deferLargeObjects} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for deferLargeObjects
* @return A modified copy of the {@code this} object
*/
public final ContextConfiguration withDeferLargeObjects(boolean value) {
if (this.deferLargeObjects == value) return this;
return validate(new ContextConfiguration(
this.expressionStrategy,
this.dynamicVariableResolver,
this.validationMode,
this.deferredExecutionMode,
value,
this.throwInterpreterErrors,
this.partialMacroEvaluation,
this.unwrapRawOverride));
}
/**
* Copy the current immutable object by setting a value for the {@link ContextConfigurationIF#isThrowInterpreterErrors() throwInterpreterErrors} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for throwInterpreterErrors
* @return A modified copy of the {@code this} object
*/
public final ContextConfiguration withThrowInterpreterErrors(boolean value) {
if (this.throwInterpreterErrors == value) return this;
return validate(new ContextConfiguration(
this.expressionStrategy,
this.dynamicVariableResolver,
this.validationMode,
this.deferredExecutionMode,
this.deferLargeObjects,
value,
this.partialMacroEvaluation,
this.unwrapRawOverride));
}
/**
* Copy the current immutable object by setting a value for the {@link ContextConfigurationIF#isPartialMacroEvaluation() partialMacroEvaluation} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for partialMacroEvaluation
* @return A modified copy of the {@code this} object
*/
public final ContextConfiguration withPartialMacroEvaluation(boolean value) {
if (this.partialMacroEvaluation == value) return this;
return validate(new ContextConfiguration(
this.expressionStrategy,
this.dynamicVariableResolver,
this.validationMode,
this.deferredExecutionMode,
this.deferLargeObjects,
this.throwInterpreterErrors,
value,
this.unwrapRawOverride));
}
/**
* Copy the current immutable object by setting a value for the {@link ContextConfigurationIF#isUnwrapRawOverride() unwrapRawOverride} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for unwrapRawOverride
* @return A modified copy of the {@code this} object
*/
public final ContextConfiguration withUnwrapRawOverride(boolean value) {
if (this.unwrapRawOverride == value) return this;
return validate(new ContextConfiguration(
this.expressionStrategy,
this.dynamicVariableResolver,
this.validationMode,
this.deferredExecutionMode,
this.deferLargeObjects,
this.throwInterpreterErrors,
this.partialMacroEvaluation,
value));
}
/**
* This instance is equal to all instances of {@code ContextConfiguration} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(@Nullable Object another) {
if (this == another) return true;
return another instanceof ContextConfiguration
&& equalTo(0, (ContextConfiguration) another);
}
private boolean equalTo(int synthetic, ContextConfiguration another) {
return expressionStrategy.equals(another.expressionStrategy)
&& Objects.equals(dynamicVariableResolver, another.dynamicVariableResolver)
&& validationMode == another.validationMode
&& deferredExecutionMode == another.deferredExecutionMode
&& deferLargeObjects == another.deferLargeObjects
&& throwInterpreterErrors == another.throwInterpreterErrors
&& partialMacroEvaluation == another.partialMacroEvaluation
&& unwrapRawOverride == another.unwrapRawOverride;
}
/**
* Computes a hash code from attributes: {@code expressionStrategy}, {@code dynamicVariableResolver}, {@code validationMode}, {@code deferredExecutionMode}, {@code deferLargeObjects}, {@code throwInterpreterErrors}, {@code partialMacroEvaluation}, {@code unwrapRawOverride}.
* @return hashCode value
*/
@Override
public int hashCode() {
int h = 5381;
h += (h << 5) + expressionStrategy.hashCode();
h += (h << 5) + Objects.hashCode(dynamicVariableResolver);
h += (h << 5) + Booleans.hashCode(validationMode);
h += (h << 5) + Booleans.hashCode(deferredExecutionMode);
h += (h << 5) + Booleans.hashCode(deferLargeObjects);
h += (h << 5) + Booleans.hashCode(throwInterpreterErrors);
h += (h << 5) + Booleans.hashCode(partialMacroEvaluation);
h += (h << 5) + Booleans.hashCode(unwrapRawOverride);
return h;
}
/**
* Prints the immutable value {@code ContextConfiguration} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
return MoreObjects.toStringHelper("ContextConfiguration")
.omitNullValues()
.add("expressionStrategy", expressionStrategy)
.add("dynamicVariableResolver", dynamicVariableResolver)
.add("validationMode", validationMode)
.add("deferredExecutionMode", deferredExecutionMode)
.add("deferLargeObjects", deferLargeObjects)
.add("throwInterpreterErrors", throwInterpreterErrors)
.add("partialMacroEvaluation", partialMacroEvaluation)
.add("unwrapRawOverride", unwrapRawOverride)
.toString();
}
/**
* Utility type used to correctly read immutable object from JSON representation.
* @deprecated Do not use this type directly, it exists only for the Jackson-binding infrastructure
*/
@Generated(from = "ContextConfigurationIF", generator = "Immutables")
@Deprecated
@JsonAutoDetect(fieldVisibility = JsonAutoDetect.Visibility.NONE)
static final class Json implements ContextConfigurationIF {
@Nullable ExpressionStrategy expressionStrategy;
@Nullable DynamicVariableResolver dynamicVariableResolver;
boolean validationMode;
boolean validationModeIsSet;
boolean deferredExecutionMode;
boolean deferredExecutionModeIsSet;
boolean deferLargeObjects;
boolean deferLargeObjectsIsSet;
boolean throwInterpreterErrors;
boolean throwInterpreterErrorsIsSet;
boolean partialMacroEvaluation;
boolean partialMacroEvaluationIsSet;
boolean unwrapRawOverride;
boolean unwrapRawOverrideIsSet;
@JsonProperty
public void setExpressionStrategy(ExpressionStrategy expressionStrategy) {
this.expressionStrategy = expressionStrategy;
}
@JsonProperty
public void setDynamicVariableResolver(@Nullable DynamicVariableResolver dynamicVariableResolver) {
this.dynamicVariableResolver = dynamicVariableResolver;
}
@JsonProperty
public void setValidationMode(boolean validationMode) {
this.validationMode = validationMode;
this.validationModeIsSet = true;
}
@JsonProperty
public void setDeferredExecutionMode(boolean deferredExecutionMode) {
this.deferredExecutionMode = deferredExecutionMode;
this.deferredExecutionModeIsSet = true;
}
@JsonProperty
public void setDeferLargeObjects(boolean deferLargeObjects) {
this.deferLargeObjects = deferLargeObjects;
this.deferLargeObjectsIsSet = true;
}
@JsonProperty
public void setThrowInterpreterErrors(boolean throwInterpreterErrors) {
this.throwInterpreterErrors = throwInterpreterErrors;
this.throwInterpreterErrorsIsSet = true;
}
@JsonProperty
public void setPartialMacroEvaluation(boolean partialMacroEvaluation) {
this.partialMacroEvaluation = partialMacroEvaluation;
this.partialMacroEvaluationIsSet = true;
}
@JsonProperty
public void setUnwrapRawOverride(boolean unwrapRawOverride) {
this.unwrapRawOverride = unwrapRawOverride;
this.unwrapRawOverrideIsSet = true;
}
@Override
public ExpressionStrategy getExpressionStrategy() { throw new UnsupportedOperationException(); }
@Override
public DynamicVariableResolver getDynamicVariableResolver() { throw new UnsupportedOperationException(); }
@Override
public boolean isValidationMode() { throw new UnsupportedOperationException(); }
@Override
public boolean isDeferredExecutionMode() { throw new UnsupportedOperationException(); }
@Override
public boolean isDeferLargeObjects() { throw new UnsupportedOperationException(); }
@Override
public boolean isThrowInterpreterErrors() { throw new UnsupportedOperationException(); }
@Override
public boolean isPartialMacroEvaluation() { throw new UnsupportedOperationException(); }
@Override
public boolean isUnwrapRawOverride() { throw new UnsupportedOperationException(); }
}
/**
* @param json A JSON-bindable data structure
* @return An immutable value type
* @deprecated Do not use this method directly, it exists only for the Jackson-binding infrastructure
*/
@Deprecated
@JsonCreator(mode = JsonCreator.Mode.DELEGATING)
static ContextConfiguration fromJson(Json json) {
ContextConfiguration.Builder builder = ContextConfiguration.builder();
if (json.expressionStrategy != null) {
builder.setExpressionStrategy(json.expressionStrategy);
}
if (json.dynamicVariableResolver != null) {
builder.setDynamicVariableResolver(json.dynamicVariableResolver);
}
if (json.validationModeIsSet) {
builder.setValidationMode(json.validationMode);
}
if (json.deferredExecutionModeIsSet) {
builder.setDeferredExecutionMode(json.deferredExecutionMode);
}
if (json.deferLargeObjectsIsSet) {
builder.setDeferLargeObjects(json.deferLargeObjects);
}
if (json.throwInterpreterErrorsIsSet) {
builder.setThrowInterpreterErrors(json.throwInterpreterErrors);
}
if (json.partialMacroEvaluationIsSet) {
builder.setPartialMacroEvaluation(json.partialMacroEvaluation);
}
if (json.unwrapRawOverrideIsSet) {
builder.setUnwrapRawOverride(json.unwrapRawOverride);
}
return builder.build();
}
private static final ContextConfiguration INSTANCE = validate(new ContextConfiguration());
/**
* Returns the default immutable singleton value of {@code ContextConfiguration}
* @return An immutable instance of ContextConfiguration
*/
public static ContextConfiguration of() {
return INSTANCE;
}
private static ContextConfiguration validate(ContextConfiguration instance) {
return INSTANCE != null && INSTANCE.equalTo(0, instance) ? INSTANCE : instance;
}
/**
* Creates an immutable copy of a {@link ContextConfigurationIF} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable ContextConfiguration instance
*/
public static ContextConfiguration copyOf(ContextConfigurationIF instance) {
if (instance instanceof ContextConfiguration) {
return (ContextConfiguration) instance;
}
return ContextConfiguration.builder()
.from(instance)
.build();
}
/**
* Creates a builder for {@link ContextConfiguration ContextConfiguration}.
*
* ContextConfiguration.builder()
* .setExpressionStrategy(com.hubspot.jinjava.lib.expression.ExpressionStrategy) // optional {@link ContextConfigurationIF#getExpressionStrategy() expressionStrategy}
* .setDynamicVariableResolver(com.hubspot.jinjava.interpret.DynamicVariableResolver | null) // nullable {@link ContextConfigurationIF#getDynamicVariableResolver() dynamicVariableResolver}
* .setValidationMode(boolean) // optional {@link ContextConfigurationIF#isValidationMode() validationMode}
* .setDeferredExecutionMode(boolean) // optional {@link ContextConfigurationIF#isDeferredExecutionMode() deferredExecutionMode}
* .setDeferLargeObjects(boolean) // optional {@link ContextConfigurationIF#isDeferLargeObjects() deferLargeObjects}
* .setThrowInterpreterErrors(boolean) // optional {@link ContextConfigurationIF#isThrowInterpreterErrors() throwInterpreterErrors}
* .setPartialMacroEvaluation(boolean) // optional {@link ContextConfigurationIF#isPartialMacroEvaluation() partialMacroEvaluation}
* .setUnwrapRawOverride(boolean) // optional {@link ContextConfigurationIF#isUnwrapRawOverride() unwrapRawOverride}
* .build();
*
* @return A new ContextConfiguration builder
*/
public static ContextConfiguration.Builder builder() {
return new ContextConfiguration.Builder();
}
/**
* Builds instances of type {@link ContextConfiguration ContextConfiguration}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
@Generated(from = "ContextConfigurationIF", generator = "Immutables")
@NotThreadSafe
public static final class Builder {
private static final long OPT_BIT_VALIDATION_MODE = 0x1L;
private static final long OPT_BIT_DEFERRED_EXECUTION_MODE = 0x2L;
private static final long OPT_BIT_DEFER_LARGE_OBJECTS = 0x4L;
private static final long OPT_BIT_THROW_INTERPRETER_ERRORS = 0x8L;
private static final long OPT_BIT_PARTIAL_MACRO_EVALUATION = 0x10L;
private static final long OPT_BIT_UNWRAP_RAW_OVERRIDE = 0x20L;
private long optBits;
private @Nullable ExpressionStrategy expressionStrategy;
private @Nullable DynamicVariableResolver dynamicVariableResolver;
private boolean validationMode;
private boolean deferredExecutionMode;
private boolean deferLargeObjects;
private boolean throwInterpreterErrors;
private boolean partialMacroEvaluation;
private boolean unwrapRawOverride;
private Builder() {
}
/**
* Fill a builder with attribute values from the provided {@code ContextConfigurationIF} instance.
* Regular attribute values will be replaced with those from the given instance.
* Absent optional values will not replace present values.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(ContextConfigurationIF instance) {
Objects.requireNonNull(instance, "instance");
this.setExpressionStrategy(instance.getExpressionStrategy());
@Nullable DynamicVariableResolver dynamicVariableResolverValue = instance.getDynamicVariableResolver();
if (dynamicVariableResolverValue != null) {
setDynamicVariableResolver(dynamicVariableResolverValue);
}
this.setValidationMode(instance.isValidationMode());
this.setDeferredExecutionMode(instance.isDeferredExecutionMode());
this.setDeferLargeObjects(instance.isDeferLargeObjects());
this.setThrowInterpreterErrors(instance.isThrowInterpreterErrors());
this.setPartialMacroEvaluation(instance.isPartialMacroEvaluation());
this.setUnwrapRawOverride(instance.isUnwrapRawOverride());
return this;
}
/**
* Initializes the value for the {@link ContextConfigurationIF#getExpressionStrategy() expressionStrategy} attribute.
*
If not set, this attribute will have a default value as returned by the initializer of {@link ContextConfigurationIF#getExpressionStrategy() expressionStrategy}.
* @param expressionStrategy The value for expressionStrategy
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setExpressionStrategy(ExpressionStrategy expressionStrategy) {
this.expressionStrategy = Objects.requireNonNull(expressionStrategy, "expressionStrategy");
return this;
}
/**
* Initializes the value for the {@link ContextConfigurationIF#getDynamicVariableResolver() dynamicVariableResolver} attribute.
* @param dynamicVariableResolver The value for dynamicVariableResolver (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setDynamicVariableResolver(@Nullable DynamicVariableResolver dynamicVariableResolver) {
this.dynamicVariableResolver = dynamicVariableResolver;
return this;
}
/**
* Initializes the value for the {@link ContextConfigurationIF#isValidationMode() validationMode} attribute.
*
If not set, this attribute will have a default value as returned by the initializer of {@link ContextConfigurationIF#isValidationMode() validationMode}.
* @param validationMode The value for validationMode
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setValidationMode(boolean validationMode) {
this.validationMode = validationMode;
optBits |= OPT_BIT_VALIDATION_MODE;
return this;
}
/**
* Initializes the value for the {@link ContextConfigurationIF#isDeferredExecutionMode() deferredExecutionMode} attribute.
*
If not set, this attribute will have a default value as returned by the initializer of {@link ContextConfigurationIF#isDeferredExecutionMode() deferredExecutionMode}.
* @param deferredExecutionMode The value for deferredExecutionMode
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setDeferredExecutionMode(boolean deferredExecutionMode) {
this.deferredExecutionMode = deferredExecutionMode;
optBits |= OPT_BIT_DEFERRED_EXECUTION_MODE;
return this;
}
/**
* Initializes the value for the {@link ContextConfigurationIF#isDeferLargeObjects() deferLargeObjects} attribute.
*
If not set, this attribute will have a default value as returned by the initializer of {@link ContextConfigurationIF#isDeferLargeObjects() deferLargeObjects}.
* @param deferLargeObjects The value for deferLargeObjects
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setDeferLargeObjects(boolean deferLargeObjects) {
this.deferLargeObjects = deferLargeObjects;
optBits |= OPT_BIT_DEFER_LARGE_OBJECTS;
return this;
}
/**
* Initializes the value for the {@link ContextConfigurationIF#isThrowInterpreterErrors() throwInterpreterErrors} attribute.
*
If not set, this attribute will have a default value as returned by the initializer of {@link ContextConfigurationIF#isThrowInterpreterErrors() throwInterpreterErrors}.
* @param throwInterpreterErrors The value for throwInterpreterErrors
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setThrowInterpreterErrors(boolean throwInterpreterErrors) {
this.throwInterpreterErrors = throwInterpreterErrors;
optBits |= OPT_BIT_THROW_INTERPRETER_ERRORS;
return this;
}
/**
* Initializes the value for the {@link ContextConfigurationIF#isPartialMacroEvaluation() partialMacroEvaluation} attribute.
*
If not set, this attribute will have a default value as returned by the initializer of {@link ContextConfigurationIF#isPartialMacroEvaluation() partialMacroEvaluation}.
* @param partialMacroEvaluation The value for partialMacroEvaluation
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setPartialMacroEvaluation(boolean partialMacroEvaluation) {
this.partialMacroEvaluation = partialMacroEvaluation;
optBits |= OPT_BIT_PARTIAL_MACRO_EVALUATION;
return this;
}
/**
* Initializes the value for the {@link ContextConfigurationIF#isUnwrapRawOverride() unwrapRawOverride} attribute.
*
If not set, this attribute will have a default value as returned by the initializer of {@link ContextConfigurationIF#isUnwrapRawOverride() unwrapRawOverride}.
* @param unwrapRawOverride The value for unwrapRawOverride
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setUnwrapRawOverride(boolean unwrapRawOverride) {
this.unwrapRawOverride = unwrapRawOverride;
optBits |= OPT_BIT_UNWRAP_RAW_OVERRIDE;
return this;
}
/**
* Builds a new {@link ContextConfiguration ContextConfiguration}.
* @return An immutable instance of ContextConfiguration
* @throws com.hubspot.immutables.validation.InvalidImmutableStateException if any required attributes are missing
*/
public ContextConfiguration build() {
return ContextConfiguration.validate(new ContextConfiguration(this));
}
private boolean validationModeIsSet() {
return (optBits & OPT_BIT_VALIDATION_MODE) != 0;
}
private boolean deferredExecutionModeIsSet() {
return (optBits & OPT_BIT_DEFERRED_EXECUTION_MODE) != 0;
}
private boolean deferLargeObjectsIsSet() {
return (optBits & OPT_BIT_DEFER_LARGE_OBJECTS) != 0;
}
private boolean throwInterpreterErrorsIsSet() {
return (optBits & OPT_BIT_THROW_INTERPRETER_ERRORS) != 0;
}
private boolean partialMacroEvaluationIsSet() {
return (optBits & OPT_BIT_PARTIAL_MACRO_EVALUATION) != 0;
}
private boolean unwrapRawOverrideIsSet() {
return (optBits & OPT_BIT_UNWRAP_RAW_OVERRIDE) != 0;
}
}
}