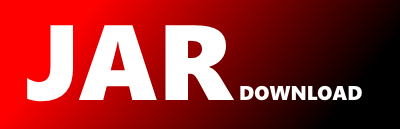
com.hubspot.maven.plugins.dependency.scope.TraversalContext Maven / Gradle / Ivy
package com.hubspot.maven.plugins.dependency.scope;
import java.util.ArrayList;
import java.util.Collections;
import java.util.HashSet;
import java.util.List;
import java.util.Set;
import org.apache.maven.artifact.Artifact;
import org.apache.maven.model.Dependency;
import org.apache.maven.model.Exclusion;
import org.apache.maven.project.MavenProject;
import org.apache.maven.shared.dependency.graph.DependencyNode;
public class TraversalContext {
private final DependencyNode node;
private final List path;
private final Set testScopedArtifacts;
private final Set exclusions;
private TraversalContext(DependencyNode node,
List path,
Set testScopedArtifacts,
Set exclusions) {
this.node = node;
this.path = Collections.unmodifiableList(path);
this.testScopedArtifacts = Collections.unmodifiableSet(testScopedArtifacts);
this.exclusions = Collections.unmodifiableSet(exclusions);
}
public static TraversalContext newContextFor(DependencyNode node) {
List path = Collections.singletonList(node.getArtifact());
Set testScopedArtifacts = new HashSet<>();
for (DependencyNode dependency : node.getChildren()) {
if (Artifact.SCOPE_TEST.equals(dependency.getArtifact().getScope())) {
testScopedArtifacts.add(dependency.getArtifact().getDependencyConflictId());
}
}
return new TraversalContext(node, path, testScopedArtifacts, Collections.emptySet());
}
public TraversalContext stepInto(MavenProject project, DependencyNode node) {
String artifactKey = node.getArtifact().getDependencyConflictId();
List path = new ArrayList<>(this.path);
path.add(node.getArtifact());
Set exclusions = new HashSet<>(this.exclusions);
for (Dependency dependency : project.getDependencies()) {
if (artifactKey.equals(dependency.getManagementKey())) {
for (Exclusion exclusion : dependency.getExclusions()) {
exclusions.add(exclusion.getGroupId() + ":" + exclusion.getArtifactId());
}
}
}
return new TraversalContext(node, path, testScopedArtifacts, exclusions);
}
public boolean isOverriddenToTestScope(Dependency dependency) {
return !excluded(dependency) && testScopedArtifacts.contains(dependency.getManagementKey());
}
public Artifact currentArtifact() {
return node.getArtifact();
}
public List path() {
return path;
}
private boolean excluded(Dependency dependency) {
return exclusions.contains(dependency.getGroupId() + ":" + dependency.getArtifactId());
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy