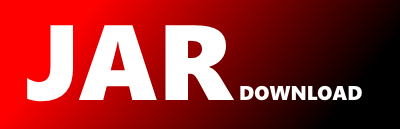
com.hubspot.slack.client.methods.params.chat.SlashCommandResponseParams Maven / Gradle / Ivy
package com.hubspot.slack.client.methods.params.chat;
import com.fasterxml.jackson.annotation.JsonAutoDetect;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.hubspot.immutables.validation.InvalidImmutableStateException;
import com.hubspot.slack.client.methods.interceptor.HasChannel;
import com.hubspot.slack.client.models.Attachment;
import com.hubspot.slack.client.models.TopLevelMessageResponseType;
import com.hubspot.slack.client.models.blocks.Block;
import edu.umd.cs.findbugs.annotations.SuppressFBWarnings;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
import javax.annotation.ParametersAreNonnullByDefault;
import javax.annotation.concurrent.Immutable;
import javax.annotation.concurrent.NotThreadSafe;
import org.immutables.value.Generated;
/**
* Immutable implementation of {@link AbstractSlashCommandResponseParams}.
*
* Use the builder to create immutable instances:
* {@code SlashCommandResponseParams.builder()}.
*/
@Generated(from = "AbstractSlashCommandResponseParams", generator = "Immutables")
@SuppressWarnings({"all"})
@SuppressFBWarnings
@ParametersAreNonnullByDefault
@javax.annotation.processing.Generated("org.immutables.processor.ProxyProcessor")
@Immutable
public final class SlashCommandResponseParams
extends AbstractSlashCommandResponseParams {
private final List attachments;
private final List blocks;
private final String channelId;
private final @Nullable String text;
private final @Nullable String threadTs;
private final @Nullable String username;
private final @Nullable Boolean asUser;
private final @Nullable String iconEmoji;
private final @Nullable String iconUrl;
private final @Nullable Boolean linkNames;
private final @Nullable Boolean unfurlLinks;
private final @Nullable Boolean unfurlMedia;
private final @Nullable Boolean replyBroadcast;
private final TopLevelMessageResponseType responseType;
private SlashCommandResponseParams(SlashCommandResponseParams.Builder builder) {
this.attachments = createUnmodifiableList(true, builder.attachments);
this.channelId = builder.channelId;
this.text = builder.text;
this.threadTs = builder.threadTs;
this.username = builder.username;
this.asUser = builder.asUser;
this.iconEmoji = builder.iconEmoji;
this.iconUrl = builder.iconUrl;
this.linkNames = builder.linkNames;
this.unfurlLinks = builder.unfurlLinks;
this.unfurlMedia = builder.unfurlMedia;
this.replyBroadcast = builder.replyBroadcast;
if (builder.blocksIsSet()) {
initShim.setBlocks(createUnmodifiableList(true, builder.blocks));
}
if (builder.responseType != null) {
initShim.setResponseType(builder.responseType);
}
this.blocks = initShim.getBlocks();
this.responseType = initShim.getResponseType();
this.initShim = null;
}
private SlashCommandResponseParams(
List attachments,
List blocks,
String channelId,
@Nullable String text,
@Nullable String threadTs,
@Nullable String username,
@Nullable Boolean asUser,
@Nullable String iconEmoji,
@Nullable String iconUrl,
@Nullable Boolean linkNames,
@Nullable Boolean unfurlLinks,
@Nullable Boolean unfurlMedia,
@Nullable Boolean replyBroadcast,
TopLevelMessageResponseType responseType) {
this.attachments = attachments;
this.blocks = blocks;
this.channelId = channelId;
this.text = text;
this.threadTs = threadTs;
this.username = username;
this.asUser = asUser;
this.iconEmoji = iconEmoji;
this.iconUrl = iconUrl;
this.linkNames = linkNames;
this.unfurlLinks = unfurlLinks;
this.unfurlMedia = unfurlMedia;
this.replyBroadcast = replyBroadcast;
this.responseType = responseType;
this.initShim = null;
}
private static final byte STAGE_INITIALIZING = -1;
private static final byte STAGE_UNINITIALIZED = 0;
private static final byte STAGE_INITIALIZED = 1;
private transient volatile InitShim initShim = new InitShim();
@Generated(from = "AbstractSlashCommandResponseParams", generator = "Immutables")
private final class InitShim {
private byte blocksBuildStage = STAGE_UNINITIALIZED;
private List blocks;
List getBlocks() {
if (blocksBuildStage == STAGE_INITIALIZING) throw new InvalidImmutableStateException(formatInitCycleMessage());
if (blocksBuildStage == STAGE_UNINITIALIZED) {
blocksBuildStage = STAGE_INITIALIZING;
this.blocks = createUnmodifiableList(false, createSafeList(SlashCommandResponseParams.super.getBlocks(), true, false));
blocksBuildStage = STAGE_INITIALIZED;
}
return this.blocks;
}
void setBlocks(List blocks) {
this.blocks = blocks;
blocksBuildStage = STAGE_INITIALIZED;
}
private byte responseTypeBuildStage = STAGE_UNINITIALIZED;
private TopLevelMessageResponseType responseType;
TopLevelMessageResponseType getResponseType() {
if (responseTypeBuildStage == STAGE_INITIALIZING) throw new InvalidImmutableStateException(formatInitCycleMessage());
if (responseTypeBuildStage == STAGE_UNINITIALIZED) {
responseTypeBuildStage = STAGE_INITIALIZING;
this.responseType = Objects.requireNonNull(SlashCommandResponseParams.super.getResponseType(), "responseType");
responseTypeBuildStage = STAGE_INITIALIZED;
}
return this.responseType;
}
void setResponseType(TopLevelMessageResponseType responseType) {
this.responseType = responseType;
responseTypeBuildStage = STAGE_INITIALIZED;
}
private String formatInitCycleMessage() {
List attributes = new ArrayList<>();
if (blocksBuildStage == STAGE_INITIALIZING) attributes.add("blocks");
if (responseTypeBuildStage == STAGE_INITIALIZING) attributes.add("responseType");
return "Cannot build SlashCommandResponseParams, attribute initializers form cycle " + attributes;
}
}
/**
* @return The value of the {@code attachments} attribute
*/
@JsonProperty
@Override
public List getAttachments() {
return attachments;
}
/**
* @return The value of the {@code blocks} attribute
*/
@JsonProperty
@Override
public List getBlocks() {
InitShim shim = this.initShim;
return shim != null
? shim.getBlocks()
: this.blocks;
}
/**
* @return The value of the {@code channelId} attribute
*/
@JsonProperty("channel")
@Override
public String getChannelId() {
return channelId;
}
/**
* @return The value of the {@code text} attribute
*/
@JsonProperty
@Override
public Optional getText() {
return Optional.ofNullable(text);
}
/**
* @return The value of the {@code threadTs} attribute
*/
@JsonProperty
@Override
public Optional getThreadTs() {
return Optional.ofNullable(threadTs);
}
/**
* @return The value of the {@code username} attribute
*/
@JsonProperty
@Override
public Optional getUsername() {
return Optional.ofNullable(username);
}
/**
* @return The value of the {@code asUser} attribute
*/
@JsonProperty
@JsonInclude(JsonInclude.Include.NON_ABSENT)
@Override
public Optional getAsUser() {
return Optional.ofNullable(asUser);
}
/**
* @return The value of the {@code iconEmoji} attribute
*/
@JsonProperty
@Override
public Optional getIconEmoji() {
return Optional.ofNullable(iconEmoji);
}
/**
* @return The value of the {@code iconUrl} attribute
*/
@JsonProperty
@Override
public Optional getIconUrl() {
return Optional.ofNullable(iconUrl);
}
/**
* @return The value of the {@code linkNames} attribute
*/
@JsonProperty
@Override
public Optional getLinkNames() {
return Optional.ofNullable(linkNames);
}
/**
* @return The value of the {@code unfurlLinks} attribute
*/
@JsonProperty
@Override
public Optional getUnfurlLinks() {
return Optional.ofNullable(unfurlLinks);
}
/**
* @return The value of the {@code unfurlMedia} attribute
*/
@JsonProperty
@Override
public Optional getUnfurlMedia() {
return Optional.ofNullable(unfurlMedia);
}
/**
* @return The value of the {@code replyBroadcast} attribute
*/
@JsonProperty
@Override
public Optional getReplyBroadcast() {
return Optional.ofNullable(replyBroadcast);
}
/**
* @return The value of the {@code responseType} attribute
*/
@JsonProperty
@Override
public TopLevelMessageResponseType getResponseType() {
InitShim shim = this.initShim;
return shim != null
? shim.getResponseType()
: this.responseType;
}
/**
* Copy the current immutable object with elements that replace the content of {@link AbstractSlashCommandResponseParams#getAttachments() attachments}.
* @param elements The elements to set
* @return A modified copy of {@code this} object
*/
public final SlashCommandResponseParams withAttachments(Attachment... elements) {
List newValue = createUnmodifiableList(false, createSafeList(Arrays.asList(elements), true, false));
return validate(new SlashCommandResponseParams(
newValue,
this.blocks,
this.channelId,
this.text,
this.threadTs,
this.username,
this.asUser,
this.iconEmoji,
this.iconUrl,
this.linkNames,
this.unfurlLinks,
this.unfurlMedia,
this.replyBroadcast,
this.responseType));
}
/**
* Copy the current immutable object with elements that replace the content of {@link AbstractSlashCommandResponseParams#getAttachments() attachments}.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements An iterable of attachments elements to set
* @return A modified copy of {@code this} object
*/
public final SlashCommandResponseParams withAttachments(Iterable elements) {
if (this.attachments == elements) return this;
List newValue = createUnmodifiableList(false, createSafeList(elements, true, false));
return validate(new SlashCommandResponseParams(
newValue,
this.blocks,
this.channelId,
this.text,
this.threadTs,
this.username,
this.asUser,
this.iconEmoji,
this.iconUrl,
this.linkNames,
this.unfurlLinks,
this.unfurlMedia,
this.replyBroadcast,
this.responseType));
}
/**
* Copy the current immutable object with elements that replace the content of {@link AbstractSlashCommandResponseParams#getBlocks() blocks}.
* @param elements The elements to set
* @return A modified copy of {@code this} object
*/
public final SlashCommandResponseParams withBlocks(Block... elements) {
List newValue = createUnmodifiableList(false, createSafeList(Arrays.asList(elements), true, false));
return validate(new SlashCommandResponseParams(
this.attachments,
newValue,
this.channelId,
this.text,
this.threadTs,
this.username,
this.asUser,
this.iconEmoji,
this.iconUrl,
this.linkNames,
this.unfurlLinks,
this.unfurlMedia,
this.replyBroadcast,
this.responseType));
}
/**
* Copy the current immutable object with elements that replace the content of {@link AbstractSlashCommandResponseParams#getBlocks() blocks}.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements An iterable of blocks elements to set
* @return A modified copy of {@code this} object
*/
public final SlashCommandResponseParams withBlocks(Iterable elements) {
if (this.blocks == elements) return this;
List newValue = createUnmodifiableList(false, createSafeList(elements, true, false));
return validate(new SlashCommandResponseParams(
this.attachments,
newValue,
this.channelId,
this.text,
this.threadTs,
this.username,
this.asUser,
this.iconEmoji,
this.iconUrl,
this.linkNames,
this.unfurlLinks,
this.unfurlMedia,
this.replyBroadcast,
this.responseType));
}
/**
* Copy the current immutable object by setting a value for the {@link AbstractSlashCommandResponseParams#getChannelId() channelId} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for channelId
* @return A modified copy of the {@code this} object
*/
public final SlashCommandResponseParams withChannelId(String value) {
String newValue = Objects.requireNonNull(value, "channelId");
if (this.channelId.equals(newValue)) return this;
return validate(new SlashCommandResponseParams(
this.attachments,
this.blocks,
newValue,
this.text,
this.threadTs,
this.username,
this.asUser,
this.iconEmoji,
this.iconUrl,
this.linkNames,
this.unfurlLinks,
this.unfurlMedia,
this.replyBroadcast,
this.responseType));
}
/**
* Copy the current immutable object by setting a present value for the optional {@link AbstractSlashCommandResponseParams#getText() text} attribute.
* @param value The value for text, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final SlashCommandResponseParams withText(@Nullable String value) {
@Nullable String newValue = value;
if (Objects.equals(this.text, newValue)) return this;
return validate(new SlashCommandResponseParams(
this.attachments,
this.blocks,
this.channelId,
newValue,
this.threadTs,
this.username,
this.asUser,
this.iconEmoji,
this.iconUrl,
this.linkNames,
this.unfurlLinks,
this.unfurlMedia,
this.replyBroadcast,
this.responseType));
}
/**
* Copy the current immutable object by setting an optional value for the {@link AbstractSlashCommandResponseParams#getText() text} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for text
* @return A modified copy of {@code this} object
*/
public final SlashCommandResponseParams withText(Optional optional) {
@Nullable String value = optional.orElse(null);
if (Objects.equals(this.text, value)) return this;
return validate(new SlashCommandResponseParams(
this.attachments,
this.blocks,
this.channelId,
value,
this.threadTs,
this.username,
this.asUser,
this.iconEmoji,
this.iconUrl,
this.linkNames,
this.unfurlLinks,
this.unfurlMedia,
this.replyBroadcast,
this.responseType));
}
/**
* Copy the current immutable object by setting a present value for the optional {@link AbstractSlashCommandResponseParams#getThreadTs() threadTs} attribute.
* @param value The value for threadTs, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final SlashCommandResponseParams withThreadTs(@Nullable String value) {
@Nullable String newValue = value;
if (Objects.equals(this.threadTs, newValue)) return this;
return validate(new SlashCommandResponseParams(
this.attachments,
this.blocks,
this.channelId,
this.text,
newValue,
this.username,
this.asUser,
this.iconEmoji,
this.iconUrl,
this.linkNames,
this.unfurlLinks,
this.unfurlMedia,
this.replyBroadcast,
this.responseType));
}
/**
* Copy the current immutable object by setting an optional value for the {@link AbstractSlashCommandResponseParams#getThreadTs() threadTs} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for threadTs
* @return A modified copy of {@code this} object
*/
public final SlashCommandResponseParams withThreadTs(Optional optional) {
@Nullable String value = optional.orElse(null);
if (Objects.equals(this.threadTs, value)) return this;
return validate(new SlashCommandResponseParams(
this.attachments,
this.blocks,
this.channelId,
this.text,
value,
this.username,
this.asUser,
this.iconEmoji,
this.iconUrl,
this.linkNames,
this.unfurlLinks,
this.unfurlMedia,
this.replyBroadcast,
this.responseType));
}
/**
* Copy the current immutable object by setting a present value for the optional {@link AbstractSlashCommandResponseParams#getUsername() username} attribute.
* @param value The value for username, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final SlashCommandResponseParams withUsername(@Nullable String value) {
@Nullable String newValue = value;
if (Objects.equals(this.username, newValue)) return this;
return validate(new SlashCommandResponseParams(
this.attachments,
this.blocks,
this.channelId,
this.text,
this.threadTs,
newValue,
this.asUser,
this.iconEmoji,
this.iconUrl,
this.linkNames,
this.unfurlLinks,
this.unfurlMedia,
this.replyBroadcast,
this.responseType));
}
/**
* Copy the current immutable object by setting an optional value for the {@link AbstractSlashCommandResponseParams#getUsername() username} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for username
* @return A modified copy of {@code this} object
*/
public final SlashCommandResponseParams withUsername(Optional optional) {
@Nullable String value = optional.orElse(null);
if (Objects.equals(this.username, value)) return this;
return validate(new SlashCommandResponseParams(
this.attachments,
this.blocks,
this.channelId,
this.text,
this.threadTs,
value,
this.asUser,
this.iconEmoji,
this.iconUrl,
this.linkNames,
this.unfurlLinks,
this.unfurlMedia,
this.replyBroadcast,
this.responseType));
}
/**
* Copy the current immutable object by setting a present value for the optional {@link AbstractSlashCommandResponseParams#getAsUser() asUser} attribute.
* @param value The value for asUser, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final SlashCommandResponseParams withAsUser(@Nullable Boolean value) {
@Nullable Boolean newValue = value;
if (Objects.equals(this.asUser, newValue)) return this;
return validate(new SlashCommandResponseParams(
this.attachments,
this.blocks,
this.channelId,
this.text,
this.threadTs,
this.username,
newValue,
this.iconEmoji,
this.iconUrl,
this.linkNames,
this.unfurlLinks,
this.unfurlMedia,
this.replyBroadcast,
this.responseType));
}
/**
* Copy the current immutable object by setting an optional value for the {@link AbstractSlashCommandResponseParams#getAsUser() asUser} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for asUser
* @return A modified copy of {@code this} object
*/
public final SlashCommandResponseParams withAsUser(Optional optional) {
@Nullable Boolean value = optional.orElse(null);
if (Objects.equals(this.asUser, value)) return this;
return validate(new SlashCommandResponseParams(
this.attachments,
this.blocks,
this.channelId,
this.text,
this.threadTs,
this.username,
value,
this.iconEmoji,
this.iconUrl,
this.linkNames,
this.unfurlLinks,
this.unfurlMedia,
this.replyBroadcast,
this.responseType));
}
/**
* Copy the current immutable object by setting a present value for the optional {@link AbstractSlashCommandResponseParams#getIconEmoji() iconEmoji} attribute.
* @param value The value for iconEmoji, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final SlashCommandResponseParams withIconEmoji(@Nullable String value) {
@Nullable String newValue = value;
if (Objects.equals(this.iconEmoji, newValue)) return this;
return validate(new SlashCommandResponseParams(
this.attachments,
this.blocks,
this.channelId,
this.text,
this.threadTs,
this.username,
this.asUser,
newValue,
this.iconUrl,
this.linkNames,
this.unfurlLinks,
this.unfurlMedia,
this.replyBroadcast,
this.responseType));
}
/**
* Copy the current immutable object by setting an optional value for the {@link AbstractSlashCommandResponseParams#getIconEmoji() iconEmoji} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for iconEmoji
* @return A modified copy of {@code this} object
*/
public final SlashCommandResponseParams withIconEmoji(Optional optional) {
@Nullable String value = optional.orElse(null);
if (Objects.equals(this.iconEmoji, value)) return this;
return validate(new SlashCommandResponseParams(
this.attachments,
this.blocks,
this.channelId,
this.text,
this.threadTs,
this.username,
this.asUser,
value,
this.iconUrl,
this.linkNames,
this.unfurlLinks,
this.unfurlMedia,
this.replyBroadcast,
this.responseType));
}
/**
* Copy the current immutable object by setting a present value for the optional {@link AbstractSlashCommandResponseParams#getIconUrl() iconUrl} attribute.
* @param value The value for iconUrl, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final SlashCommandResponseParams withIconUrl(@Nullable String value) {
@Nullable String newValue = value;
if (Objects.equals(this.iconUrl, newValue)) return this;
return validate(new SlashCommandResponseParams(
this.attachments,
this.blocks,
this.channelId,
this.text,
this.threadTs,
this.username,
this.asUser,
this.iconEmoji,
newValue,
this.linkNames,
this.unfurlLinks,
this.unfurlMedia,
this.replyBroadcast,
this.responseType));
}
/**
* Copy the current immutable object by setting an optional value for the {@link AbstractSlashCommandResponseParams#getIconUrl() iconUrl} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for iconUrl
* @return A modified copy of {@code this} object
*/
public final SlashCommandResponseParams withIconUrl(Optional optional) {
@Nullable String value = optional.orElse(null);
if (Objects.equals(this.iconUrl, value)) return this;
return validate(new SlashCommandResponseParams(
this.attachments,
this.blocks,
this.channelId,
this.text,
this.threadTs,
this.username,
this.asUser,
this.iconEmoji,
value,
this.linkNames,
this.unfurlLinks,
this.unfurlMedia,
this.replyBroadcast,
this.responseType));
}
/**
* Copy the current immutable object by setting a present value for the optional {@link AbstractSlashCommandResponseParams#getLinkNames() linkNames} attribute.
* @param value The value for linkNames, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final SlashCommandResponseParams withLinkNames(@Nullable Boolean value) {
@Nullable Boolean newValue = value;
if (Objects.equals(this.linkNames, newValue)) return this;
return validate(new SlashCommandResponseParams(
this.attachments,
this.blocks,
this.channelId,
this.text,
this.threadTs,
this.username,
this.asUser,
this.iconEmoji,
this.iconUrl,
newValue,
this.unfurlLinks,
this.unfurlMedia,
this.replyBroadcast,
this.responseType));
}
/**
* Copy the current immutable object by setting an optional value for the {@link AbstractSlashCommandResponseParams#getLinkNames() linkNames} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for linkNames
* @return A modified copy of {@code this} object
*/
public final SlashCommandResponseParams withLinkNames(Optional optional) {
@Nullable Boolean value = optional.orElse(null);
if (Objects.equals(this.linkNames, value)) return this;
return validate(new SlashCommandResponseParams(
this.attachments,
this.blocks,
this.channelId,
this.text,
this.threadTs,
this.username,
this.asUser,
this.iconEmoji,
this.iconUrl,
value,
this.unfurlLinks,
this.unfurlMedia,
this.replyBroadcast,
this.responseType));
}
/**
* Copy the current immutable object by setting a present value for the optional {@link AbstractSlashCommandResponseParams#getUnfurlLinks() unfurlLinks} attribute.
* @param value The value for unfurlLinks, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final SlashCommandResponseParams withUnfurlLinks(@Nullable Boolean value) {
@Nullable Boolean newValue = value;
if (Objects.equals(this.unfurlLinks, newValue)) return this;
return validate(new SlashCommandResponseParams(
this.attachments,
this.blocks,
this.channelId,
this.text,
this.threadTs,
this.username,
this.asUser,
this.iconEmoji,
this.iconUrl,
this.linkNames,
newValue,
this.unfurlMedia,
this.replyBroadcast,
this.responseType));
}
/**
* Copy the current immutable object by setting an optional value for the {@link AbstractSlashCommandResponseParams#getUnfurlLinks() unfurlLinks} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for unfurlLinks
* @return A modified copy of {@code this} object
*/
public final SlashCommandResponseParams withUnfurlLinks(Optional optional) {
@Nullable Boolean value = optional.orElse(null);
if (Objects.equals(this.unfurlLinks, value)) return this;
return validate(new SlashCommandResponseParams(
this.attachments,
this.blocks,
this.channelId,
this.text,
this.threadTs,
this.username,
this.asUser,
this.iconEmoji,
this.iconUrl,
this.linkNames,
value,
this.unfurlMedia,
this.replyBroadcast,
this.responseType));
}
/**
* Copy the current immutable object by setting a present value for the optional {@link AbstractSlashCommandResponseParams#getUnfurlMedia() unfurlMedia} attribute.
* @param value The value for unfurlMedia, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final SlashCommandResponseParams withUnfurlMedia(@Nullable Boolean value) {
@Nullable Boolean newValue = value;
if (Objects.equals(this.unfurlMedia, newValue)) return this;
return validate(new SlashCommandResponseParams(
this.attachments,
this.blocks,
this.channelId,
this.text,
this.threadTs,
this.username,
this.asUser,
this.iconEmoji,
this.iconUrl,
this.linkNames,
this.unfurlLinks,
newValue,
this.replyBroadcast,
this.responseType));
}
/**
* Copy the current immutable object by setting an optional value for the {@link AbstractSlashCommandResponseParams#getUnfurlMedia() unfurlMedia} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for unfurlMedia
* @return A modified copy of {@code this} object
*/
public final SlashCommandResponseParams withUnfurlMedia(Optional optional) {
@Nullable Boolean value = optional.orElse(null);
if (Objects.equals(this.unfurlMedia, value)) return this;
return validate(new SlashCommandResponseParams(
this.attachments,
this.blocks,
this.channelId,
this.text,
this.threadTs,
this.username,
this.asUser,
this.iconEmoji,
this.iconUrl,
this.linkNames,
this.unfurlLinks,
value,
this.replyBroadcast,
this.responseType));
}
/**
* Copy the current immutable object by setting a present value for the optional {@link AbstractSlashCommandResponseParams#getReplyBroadcast() replyBroadcast} attribute.
* @param value The value for replyBroadcast, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final SlashCommandResponseParams withReplyBroadcast(@Nullable Boolean value) {
@Nullable Boolean newValue = value;
if (Objects.equals(this.replyBroadcast, newValue)) return this;
return validate(new SlashCommandResponseParams(
this.attachments,
this.blocks,
this.channelId,
this.text,
this.threadTs,
this.username,
this.asUser,
this.iconEmoji,
this.iconUrl,
this.linkNames,
this.unfurlLinks,
this.unfurlMedia,
newValue,
this.responseType));
}
/**
* Copy the current immutable object by setting an optional value for the {@link AbstractSlashCommandResponseParams#getReplyBroadcast() replyBroadcast} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for replyBroadcast
* @return A modified copy of {@code this} object
*/
public final SlashCommandResponseParams withReplyBroadcast(Optional optional) {
@Nullable Boolean value = optional.orElse(null);
if (Objects.equals(this.replyBroadcast, value)) return this;
return validate(new SlashCommandResponseParams(
this.attachments,
this.blocks,
this.channelId,
this.text,
this.threadTs,
this.username,
this.asUser,
this.iconEmoji,
this.iconUrl,
this.linkNames,
this.unfurlLinks,
this.unfurlMedia,
value,
this.responseType));
}
/**
* Copy the current immutable object by setting a value for the {@link AbstractSlashCommandResponseParams#getResponseType() responseType} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for responseType
* @return A modified copy of the {@code this} object
*/
public final SlashCommandResponseParams withResponseType(TopLevelMessageResponseType value) {
TopLevelMessageResponseType newValue = Objects.requireNonNull(value, "responseType");
if (this.responseType == newValue) return this;
return validate(new SlashCommandResponseParams(
this.attachments,
this.blocks,
this.channelId,
this.text,
this.threadTs,
this.username,
this.asUser,
this.iconEmoji,
this.iconUrl,
this.linkNames,
this.unfurlLinks,
this.unfurlMedia,
this.replyBroadcast,
newValue));
}
/**
* This instance is equal to all instances of {@code SlashCommandResponseParams} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(@Nullable Object another) {
if (this == another) return true;
return another instanceof SlashCommandResponseParams
&& equalTo(0, (SlashCommandResponseParams) another);
}
private boolean equalTo(int synthetic, SlashCommandResponseParams another) {
return attachments.equals(another.attachments)
&& blocks.equals(another.blocks)
&& channelId.equals(another.channelId)
&& Objects.equals(text, another.text)
&& Objects.equals(threadTs, another.threadTs)
&& Objects.equals(username, another.username)
&& Objects.equals(asUser, another.asUser)
&& Objects.equals(iconEmoji, another.iconEmoji)
&& Objects.equals(iconUrl, another.iconUrl)
&& Objects.equals(linkNames, another.linkNames)
&& Objects.equals(unfurlLinks, another.unfurlLinks)
&& Objects.equals(unfurlMedia, another.unfurlMedia)
&& Objects.equals(replyBroadcast, another.replyBroadcast)
&& responseType.equals(another.responseType);
}
/**
* Computes a hash code from attributes: {@code attachments}, {@code blocks}, {@code channelId}, {@code text}, {@code threadTs}, {@code username}, {@code asUser}, {@code iconEmoji}, {@code iconUrl}, {@code linkNames}, {@code unfurlLinks}, {@code unfurlMedia}, {@code replyBroadcast}, {@code responseType}.
* @return hashCode value
*/
@Override
public int hashCode() {
int h = 5381;
h += (h << 5) + attachments.hashCode();
h += (h << 5) + blocks.hashCode();
h += (h << 5) + channelId.hashCode();
h += (h << 5) + Objects.hashCode(text);
h += (h << 5) + Objects.hashCode(threadTs);
h += (h << 5) + Objects.hashCode(username);
h += (h << 5) + Objects.hashCode(asUser);
h += (h << 5) + Objects.hashCode(iconEmoji);
h += (h << 5) + Objects.hashCode(iconUrl);
h += (h << 5) + Objects.hashCode(linkNames);
h += (h << 5) + Objects.hashCode(unfurlLinks);
h += (h << 5) + Objects.hashCode(unfurlMedia);
h += (h << 5) + Objects.hashCode(replyBroadcast);
h += (h << 5) + responseType.hashCode();
return h;
}
/**
* Prints the immutable value {@code SlashCommandResponseParams} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
StringBuilder builder = new StringBuilder("SlashCommandResponseParams{");
builder.append("attachments=").append(attachments);
builder.append(", ");
builder.append("blocks=").append(blocks);
builder.append(", ");
builder.append("channelId=").append(channelId);
if (text != null) {
builder.append(", ");
builder.append("text=").append(text);
}
if (threadTs != null) {
builder.append(", ");
builder.append("threadTs=").append(threadTs);
}
if (username != null) {
builder.append(", ");
builder.append("username=").append(username);
}
if (asUser != null) {
builder.append(", ");
builder.append("asUser=").append(asUser);
}
if (iconEmoji != null) {
builder.append(", ");
builder.append("iconEmoji=").append(iconEmoji);
}
if (iconUrl != null) {
builder.append(", ");
builder.append("iconUrl=").append(iconUrl);
}
if (linkNames != null) {
builder.append(", ");
builder.append("linkNames=").append(linkNames);
}
if (unfurlLinks != null) {
builder.append(", ");
builder.append("unfurlLinks=").append(unfurlLinks);
}
if (unfurlMedia != null) {
builder.append(", ");
builder.append("unfurlMedia=").append(unfurlMedia);
}
if (replyBroadcast != null) {
builder.append(", ");
builder.append("replyBroadcast=").append(replyBroadcast);
}
builder.append(", ");
builder.append("responseType=").append(responseType);
return builder.append("}").toString();
}
/**
* Utility type used to correctly read immutable object from JSON representation.
* @deprecated Do not use this type directly, it exists only for the Jackson-binding infrastructure
*/
@Generated(from = "AbstractSlashCommandResponseParams", generator = "Immutables")
@Deprecated
@JsonAutoDetect(fieldVisibility = JsonAutoDetect.Visibility.NONE)
static final class Json
extends AbstractSlashCommandResponseParams {
@Nullable List attachments = Collections.emptyList();
@Nullable List blocks = Collections.emptyList();
boolean blocksIsSet;
@Nullable String channelId;
@Nullable Optional text = Optional.empty();
@Nullable Optional threadTs = Optional.empty();
@Nullable Optional username = Optional.empty();
@Nullable Optional asUser = Optional.empty();
@Nullable Optional iconEmoji = Optional.empty();
@Nullable Optional iconUrl = Optional.empty();
@Nullable Optional linkNames = Optional.empty();
@Nullable Optional unfurlLinks = Optional.empty();
@Nullable Optional unfurlMedia = Optional.empty();
@Nullable Optional replyBroadcast = Optional.empty();
@Nullable TopLevelMessageResponseType responseType;
@JsonProperty
public void setAttachments(List attachments) {
this.attachments = attachments;
}
@JsonProperty
public void setBlocks(List blocks) {
this.blocks = blocks;
this.blocksIsSet = null != blocks;
}
@JsonProperty("channel")
public void setChannelId(String channelId) {
this.channelId = channelId;
}
@JsonProperty
public void setText(Optional text) {
this.text = text;
}
@JsonProperty
public void setThreadTs(Optional threadTs) {
this.threadTs = threadTs;
}
@JsonProperty
public void setUsername(Optional username) {
this.username = username;
}
@JsonProperty
@JsonInclude(JsonInclude.Include.NON_ABSENT)
public void setAsUser(Optional asUser) {
this.asUser = asUser;
}
@JsonProperty
public void setIconEmoji(Optional iconEmoji) {
this.iconEmoji = iconEmoji;
}
@JsonProperty
public void setIconUrl(Optional iconUrl) {
this.iconUrl = iconUrl;
}
@JsonProperty
public void setLinkNames(Optional linkNames) {
this.linkNames = linkNames;
}
@JsonProperty
public void setUnfurlLinks(Optional unfurlLinks) {
this.unfurlLinks = unfurlLinks;
}
@JsonProperty
public void setUnfurlMedia(Optional unfurlMedia) {
this.unfurlMedia = unfurlMedia;
}
@JsonProperty
public void setReplyBroadcast(Optional replyBroadcast) {
this.replyBroadcast = replyBroadcast;
}
@JsonProperty
public void setResponseType(TopLevelMessageResponseType responseType) {
this.responseType = responseType;
}
@Override
public List getAttachments() { throw new UnsupportedOperationException(); }
@Override
public List getBlocks() { throw new UnsupportedOperationException(); }
@Override
public String getChannelId() { throw new UnsupportedOperationException(); }
@Override
public Optional getText() { throw new UnsupportedOperationException(); }
@Override
public Optional getThreadTs() { throw new UnsupportedOperationException(); }
@Override
public Optional getUsername() { throw new UnsupportedOperationException(); }
@Override
public Optional getAsUser() { throw new UnsupportedOperationException(); }
@Override
public Optional getIconEmoji() { throw new UnsupportedOperationException(); }
@Override
public Optional getIconUrl() { throw new UnsupportedOperationException(); }
@Override
public Optional getLinkNames() { throw new UnsupportedOperationException(); }
@Override
public Optional getUnfurlLinks() { throw new UnsupportedOperationException(); }
@Override
public Optional getUnfurlMedia() { throw new UnsupportedOperationException(); }
@Override
public Optional getReplyBroadcast() { throw new UnsupportedOperationException(); }
@Override
public TopLevelMessageResponseType getResponseType() { throw new UnsupportedOperationException(); }
}
/**
* @param json A JSON-bindable data structure
* @return An immutable value type
* @deprecated Do not use this method directly, it exists only for the Jackson-binding infrastructure
*/
@Deprecated
@JsonCreator(mode = JsonCreator.Mode.DELEGATING)
static SlashCommandResponseParams fromJson(Json json) {
SlashCommandResponseParams.Builder builder = SlashCommandResponseParams.builder();
if (json.attachments != null) {
builder.addAllAttachments(json.attachments);
}
if (json.blocksIsSet) {
builder.addAllBlocks(json.blocks);
}
if (json.channelId != null) {
builder.setChannelId(json.channelId);
}
if (json.text != null) {
builder.setText(json.text);
}
if (json.threadTs != null) {
builder.setThreadTs(json.threadTs);
}
if (json.username != null) {
builder.setUsername(json.username);
}
if (json.asUser != null) {
builder.setAsUser(json.asUser);
}
if (json.iconEmoji != null) {
builder.setIconEmoji(json.iconEmoji);
}
if (json.iconUrl != null) {
builder.setIconUrl(json.iconUrl);
}
if (json.linkNames != null) {
builder.setLinkNames(json.linkNames);
}
if (json.unfurlLinks != null) {
builder.setUnfurlLinks(json.unfurlLinks);
}
if (json.unfurlMedia != null) {
builder.setUnfurlMedia(json.unfurlMedia);
}
if (json.replyBroadcast != null) {
builder.setReplyBroadcast(json.replyBroadcast);
}
if (json.responseType != null) {
builder.setResponseType(json.responseType);
}
return builder.build();
}
private static SlashCommandResponseParams validate(SlashCommandResponseParams instance) {
instance.check();
return instance;
}
/**
* Creates an immutable copy of a {@link AbstractSlashCommandResponseParams} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable SlashCommandResponseParams instance
*/
public static SlashCommandResponseParams copyOf(AbstractSlashCommandResponseParams instance) {
if (instance instanceof SlashCommandResponseParams) {
return (SlashCommandResponseParams) instance;
}
return SlashCommandResponseParams.builder()
.from(instance)
.build();
}
/**
* Creates a builder for {@link SlashCommandResponseParams SlashCommandResponseParams}.
*
* SlashCommandResponseParams.builder()
* .addAttachments|addAllAttachments(com.hubspot.slack.client.models.Attachment) // {@link AbstractSlashCommandResponseParams#getAttachments() attachments} elements
* .addBlocks|addAllBlocks(com.hubspot.slack.client.models.blocks.Block) // {@link AbstractSlashCommandResponseParams#getBlocks() blocks} elements
* .setChannelId(String) // required {@link AbstractSlashCommandResponseParams#getChannelId() channelId}
* .setText(String) // optional {@link AbstractSlashCommandResponseParams#getText() text}
* .setThreadTs(String) // optional {@link AbstractSlashCommandResponseParams#getThreadTs() threadTs}
* .setUsername(String) // optional {@link AbstractSlashCommandResponseParams#getUsername() username}
* .setAsUser(Boolean) // optional {@link AbstractSlashCommandResponseParams#getAsUser() asUser}
* .setIconEmoji(String) // optional {@link AbstractSlashCommandResponseParams#getIconEmoji() iconEmoji}
* .setIconUrl(String) // optional {@link AbstractSlashCommandResponseParams#getIconUrl() iconUrl}
* .setLinkNames(Boolean) // optional {@link AbstractSlashCommandResponseParams#getLinkNames() linkNames}
* .setUnfurlLinks(Boolean) // optional {@link AbstractSlashCommandResponseParams#getUnfurlLinks() unfurlLinks}
* .setUnfurlMedia(Boolean) // optional {@link AbstractSlashCommandResponseParams#getUnfurlMedia() unfurlMedia}
* .setReplyBroadcast(Boolean) // optional {@link AbstractSlashCommandResponseParams#getReplyBroadcast() replyBroadcast}
* .setResponseType(com.hubspot.slack.client.models.TopLevelMessageResponseType) // optional {@link AbstractSlashCommandResponseParams#getResponseType() responseType}
* .build();
*
* @return A new SlashCommandResponseParams builder
*/
public static SlashCommandResponseParams.Builder builder() {
return new SlashCommandResponseParams.Builder();
}
/**
* Builds instances of type {@link SlashCommandResponseParams SlashCommandResponseParams}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
@Generated(from = "AbstractSlashCommandResponseParams", generator = "Immutables")
@NotThreadSafe
public static final class Builder {
private static final long INIT_BIT_CHANNEL_ID = 0x1L;
private static final long OPT_BIT_BLOCKS = 0x1L;
private long initBits = 0x1L;
private long optBits;
private List attachments = new ArrayList();
private List blocks = new ArrayList();
private @Nullable String channelId;
private @Nullable String text;
private @Nullable String threadTs;
private @Nullable String username;
private @Nullable Boolean asUser;
private @Nullable String iconEmoji;
private @Nullable String iconUrl;
private @Nullable Boolean linkNames;
private @Nullable Boolean unfurlLinks;
private @Nullable Boolean unfurlMedia;
private @Nullable Boolean replyBroadcast;
private @Nullable TopLevelMessageResponseType responseType;
private Builder() {
}
/**
* Fill a builder with attribute values from the provided {@code com.hubspot.slack.client.methods.params.chat.AbstractSlashCommandResponseParams} instance.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(AbstractSlashCommandResponseParams instance) {
Objects.requireNonNull(instance, "instance");
from((short) 0, (Object) instance);
return this;
}
/**
* Fill a builder with attribute values from the provided {@code com.hubspot.slack.client.methods.interceptor.HasChannel} instance.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(HasChannel instance) {
Objects.requireNonNull(instance, "instance");
from((short) 0, (Object) instance);
return this;
}
/**
* Fill a builder with attribute values from the provided {@code com.hubspot.slack.client.methods.params.chat.MessageParams} instance.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(MessageParams instance) {
Objects.requireNonNull(instance, "instance");
from((short) 0, (Object) instance);
return this;
}
/**
* Fill a builder with attribute values from the provided {@code com.hubspot.slack.client.methods.params.chat.AbstractChatMessageParams} instance.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(AbstractChatMessageParams instance) {
Objects.requireNonNull(instance, "instance");
from((short) 0, (Object) instance);
return this;
}
private void from(short _unused, Object object) {
long bits = 0;
if (object instanceof AbstractSlashCommandResponseParams) {
AbstractSlashCommandResponseParams instance = (AbstractSlashCommandResponseParams) object;
if ((bits & 0x1L) == 0) {
Optional unfurlMediaOptional = instance.getUnfurlMedia();
if (unfurlMediaOptional.isPresent()) {
setUnfurlMedia(unfurlMediaOptional);
}
bits |= 0x1L;
}
if ((bits & 0x2L) == 0) {
addAllAttachments(instance.getAttachments());
bits |= 0x2L;
}
if ((bits & 0x4L) == 0) {
Optional iconEmojiOptional = instance.getIconEmoji();
if (iconEmojiOptional.isPresent()) {
setIconEmoji(iconEmojiOptional);
}
bits |= 0x4L;
}
if ((bits & 0x8L) == 0) {
Optional unfurlLinksOptional = instance.getUnfurlLinks();
if (unfurlLinksOptional.isPresent()) {
setUnfurlLinks(unfurlLinksOptional);
}
bits |= 0x8L;
}
if ((bits & 0x10L) == 0) {
Optional replyBroadcastOptional = instance.getReplyBroadcast();
if (replyBroadcastOptional.isPresent()) {
setReplyBroadcast(replyBroadcastOptional);
}
bits |= 0x10L;
}
if ((bits & 0x20L) == 0) {
addAllBlocks(instance.getBlocks());
bits |= 0x20L;
}
this.setResponseType(instance.getResponseType());
if ((bits & 0x40L) == 0) {
Optional linkNamesOptional = instance.getLinkNames();
if (linkNamesOptional.isPresent()) {
setLinkNames(linkNamesOptional);
}
bits |= 0x40L;
}
if ((bits & 0x80L) == 0) {
Optional textOptional = instance.getText();
if (textOptional.isPresent()) {
setText(textOptional);
}
bits |= 0x80L;
}
if ((bits & 0x100L) == 0) {
Optional iconUrlOptional = instance.getIconUrl();
if (iconUrlOptional.isPresent()) {
setIconUrl(iconUrlOptional);
}
bits |= 0x100L;
}
if ((bits & 0x200L) == 0) {
Optional threadTsOptional = instance.getThreadTs();
if (threadTsOptional.isPresent()) {
setThreadTs(threadTsOptional);
}
bits |= 0x200L;
}
if ((bits & 0x800L) == 0) {
Optional asUserOptional = instance.getAsUser();
if (asUserOptional.isPresent()) {
setAsUser(asUserOptional);
}
bits |= 0x800L;
}
if ((bits & 0x1000L) == 0) {
Optional usernameOptional = instance.getUsername();
if (usernameOptional.isPresent()) {
setUsername(usernameOptional);
}
bits |= 0x1000L;
}
}
if (object instanceof HasChannel) {
HasChannel instance = (HasChannel) object;
if ((bits & 0x400L) == 0) {
this.setChannelId(instance.getChannelId());
bits |= 0x400L;
}
}
if (object instanceof MessageParams) {
MessageParams instance = (MessageParams) object;
if ((bits & 0x2L) == 0) {
addAllAttachments(instance.getAttachments());
bits |= 0x2L;
}
if ((bits & 0x80L) == 0) {
Optional textOptional = instance.getText();
if (textOptional.isPresent()) {
setText(textOptional);
}
bits |= 0x80L;
}
if ((bits & 0x20L) == 0) {
addAllBlocks(instance.getBlocks());
bits |= 0x20L;
}
if ((bits & 0x400L) == 0) {
this.setChannelId(instance.getChannelId());
bits |= 0x400L;
}
}
if (object instanceof AbstractChatMessageParams) {
AbstractChatMessageParams instance = (AbstractChatMessageParams) object;
if ((bits & 0x1L) == 0) {
Optional unfurlMediaOptional = instance.getUnfurlMedia();
if (unfurlMediaOptional.isPresent()) {
setUnfurlMedia(unfurlMediaOptional);
}
bits |= 0x1L;
}
if ((bits & 0x2L) == 0) {
addAllAttachments(instance.getAttachments());
bits |= 0x2L;
}
if ((bits & 0x4L) == 0) {
Optional iconEmojiOptional = instance.getIconEmoji();
if (iconEmojiOptional.isPresent()) {
setIconEmoji(iconEmojiOptional);
}
bits |= 0x4L;
}
if ((bits & 0x8L) == 0) {
Optional unfurlLinksOptional = instance.getUnfurlLinks();
if (unfurlLinksOptional.isPresent()) {
setUnfurlLinks(unfurlLinksOptional);
}
bits |= 0x8L;
}
if ((bits & 0x10L) == 0) {
Optional replyBroadcastOptional = instance.getReplyBroadcast();
if (replyBroadcastOptional.isPresent()) {
setReplyBroadcast(replyBroadcastOptional);
}
bits |= 0x10L;
}
if ((bits & 0x20L) == 0) {
addAllBlocks(instance.getBlocks());
bits |= 0x20L;
}
if ((bits & 0x40L) == 0) {
Optional linkNamesOptional = instance.getLinkNames();
if (linkNamesOptional.isPresent()) {
setLinkNames(linkNamesOptional);
}
bits |= 0x40L;
}
if ((bits & 0x80L) == 0) {
Optional textOptional = instance.getText();
if (textOptional.isPresent()) {
setText(textOptional);
}
bits |= 0x80L;
}
if ((bits & 0x100L) == 0) {
Optional iconUrlOptional = instance.getIconUrl();
if (iconUrlOptional.isPresent()) {
setIconUrl(iconUrlOptional);
}
bits |= 0x100L;
}
if ((bits & 0x200L) == 0) {
Optional threadTsOptional = instance.getThreadTs();
if (threadTsOptional.isPresent()) {
setThreadTs(threadTsOptional);
}
bits |= 0x200L;
}
if ((bits & 0x400L) == 0) {
this.setChannelId(instance.getChannelId());
bits |= 0x400L;
}
if ((bits & 0x800L) == 0) {
Optional asUserOptional = instance.getAsUser();
if (asUserOptional.isPresent()) {
setAsUser(asUserOptional);
}
bits |= 0x800L;
}
if ((bits & 0x1000L) == 0) {
Optional usernameOptional = instance.getUsername();
if (usernameOptional.isPresent()) {
setUsername(usernameOptional);
}
bits |= 0x1000L;
}
}
}
/**
* Adds one element to {@link AbstractSlashCommandResponseParams#getAttachments() attachments} list.
* @param element A attachments element
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addAttachments(Attachment element) {
this.attachments.add(Objects.requireNonNull(element, "attachments element"));
return this;
}
/**
* Adds elements to {@link AbstractSlashCommandResponseParams#getAttachments() attachments} list.
* @param elements An array of attachments elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addAttachments(Attachment... elements) {
for (Attachment element : elements) {
this.attachments.add(Objects.requireNonNull(element, "attachments element"));
}
return this;
}
/**
* Sets or replaces all elements for {@link AbstractSlashCommandResponseParams#getAttachments() attachments} list.
* @param elements An iterable of attachments elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setAttachments(Iterable elements) {
this.attachments.clear();
return addAllAttachments(elements);
}
/**
* Adds elements to {@link AbstractSlashCommandResponseParams#getAttachments() attachments} list.
* @param elements An iterable of attachments elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addAllAttachments(Iterable elements) {
for (Attachment element : elements) {
this.attachments.add(Objects.requireNonNull(element, "attachments element"));
}
return this;
}
/**
* Adds one element to {@link AbstractSlashCommandResponseParams#getBlocks() blocks} list.
* @param element A blocks element
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addBlocks(Block element) {
this.blocks.add(Objects.requireNonNull(element, "blocks element"));
optBits |= OPT_BIT_BLOCKS;
return this;
}
/**
* Adds elements to {@link AbstractSlashCommandResponseParams#getBlocks() blocks} list.
* @param elements An array of blocks elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addBlocks(Block... elements) {
for (Block element : elements) {
this.blocks.add(Objects.requireNonNull(element, "blocks element"));
}
optBits |= OPT_BIT_BLOCKS;
return this;
}
/**
* Sets or replaces all elements for {@link AbstractSlashCommandResponseParams#getBlocks() blocks} list.
* @param elements An iterable of blocks elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setBlocks(Iterable elements) {
this.blocks.clear();
return addAllBlocks(elements);
}
/**
* Adds elements to {@link AbstractSlashCommandResponseParams#getBlocks() blocks} list.
* @param elements An iterable of blocks elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addAllBlocks(Iterable elements) {
for (Block element : elements) {
this.blocks.add(Objects.requireNonNull(element, "blocks element"));
}
optBits |= OPT_BIT_BLOCKS;
return this;
}
/**
* Initializes the value for the {@link AbstractSlashCommandResponseParams#getChannelId() channelId} attribute.
* @param channelId The value for channelId
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setChannelId(String channelId) {
this.channelId = Objects.requireNonNull(channelId, "channelId");
initBits &= ~INIT_BIT_CHANNEL_ID;
return this;
}
/**
* Initializes the optional value {@link AbstractSlashCommandResponseParams#getText() text} to text.
* @param text The value for text, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
public final Builder setText(@Nullable String text) {
this.text = text;
return this;
}
/**
* Initializes the optional value {@link AbstractSlashCommandResponseParams#getText() text} to text.
* @param text The value for text
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setText(Optional text) {
this.text = text.orElse(null);
return this;
}
/**
* Initializes the optional value {@link AbstractSlashCommandResponseParams#getThreadTs() threadTs} to threadTs.
* @param threadTs The value for threadTs, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
public final Builder setThreadTs(@Nullable String threadTs) {
this.threadTs = threadTs;
return this;
}
/**
* Initializes the optional value {@link AbstractSlashCommandResponseParams#getThreadTs() threadTs} to threadTs.
* @param threadTs The value for threadTs
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setThreadTs(Optional threadTs) {
this.threadTs = threadTs.orElse(null);
return this;
}
/**
* Initializes the optional value {@link AbstractSlashCommandResponseParams#getUsername() username} to username.
* @param username The value for username, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
public final Builder setUsername(@Nullable String username) {
this.username = username;
return this;
}
/**
* Initializes the optional value {@link AbstractSlashCommandResponseParams#getUsername() username} to username.
* @param username The value for username
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setUsername(Optional username) {
this.username = username.orElse(null);
return this;
}
/**
* Initializes the optional value {@link AbstractSlashCommandResponseParams#getAsUser() asUser} to asUser.
* @param asUser The value for asUser, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
public final Builder setAsUser(@Nullable Boolean asUser) {
this.asUser = asUser;
return this;
}
/**
* Initializes the optional value {@link AbstractSlashCommandResponseParams#getAsUser() asUser} to asUser.
* @param asUser The value for asUser
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setAsUser(Optional asUser) {
this.asUser = asUser.orElse(null);
return this;
}
/**
* Initializes the optional value {@link AbstractSlashCommandResponseParams#getIconEmoji() iconEmoji} to iconEmoji.
* @param iconEmoji The value for iconEmoji, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
public final Builder setIconEmoji(@Nullable String iconEmoji) {
this.iconEmoji = iconEmoji;
return this;
}
/**
* Initializes the optional value {@link AbstractSlashCommandResponseParams#getIconEmoji() iconEmoji} to iconEmoji.
* @param iconEmoji The value for iconEmoji
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setIconEmoji(Optional iconEmoji) {
this.iconEmoji = iconEmoji.orElse(null);
return this;
}
/**
* Initializes the optional value {@link AbstractSlashCommandResponseParams#getIconUrl() iconUrl} to iconUrl.
* @param iconUrl The value for iconUrl, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
public final Builder setIconUrl(@Nullable String iconUrl) {
this.iconUrl = iconUrl;
return this;
}
/**
* Initializes the optional value {@link AbstractSlashCommandResponseParams#getIconUrl() iconUrl} to iconUrl.
* @param iconUrl The value for iconUrl
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setIconUrl(Optional iconUrl) {
this.iconUrl = iconUrl.orElse(null);
return this;
}
/**
* Initializes the optional value {@link AbstractSlashCommandResponseParams#getLinkNames() linkNames} to linkNames.
* @param linkNames The value for linkNames, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
public final Builder setLinkNames(@Nullable Boolean linkNames) {
this.linkNames = linkNames;
return this;
}
/**
* Initializes the optional value {@link AbstractSlashCommandResponseParams#getLinkNames() linkNames} to linkNames.
* @param linkNames The value for linkNames
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setLinkNames(Optional linkNames) {
this.linkNames = linkNames.orElse(null);
return this;
}
/**
* Initializes the optional value {@link AbstractSlashCommandResponseParams#getUnfurlLinks() unfurlLinks} to unfurlLinks.
* @param unfurlLinks The value for unfurlLinks, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
public final Builder setUnfurlLinks(@Nullable Boolean unfurlLinks) {
this.unfurlLinks = unfurlLinks;
return this;
}
/**
* Initializes the optional value {@link AbstractSlashCommandResponseParams#getUnfurlLinks() unfurlLinks} to unfurlLinks.
* @param unfurlLinks The value for unfurlLinks
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setUnfurlLinks(Optional unfurlLinks) {
this.unfurlLinks = unfurlLinks.orElse(null);
return this;
}
/**
* Initializes the optional value {@link AbstractSlashCommandResponseParams#getUnfurlMedia() unfurlMedia} to unfurlMedia.
* @param unfurlMedia The value for unfurlMedia, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
public final Builder setUnfurlMedia(@Nullable Boolean unfurlMedia) {
this.unfurlMedia = unfurlMedia;
return this;
}
/**
* Initializes the optional value {@link AbstractSlashCommandResponseParams#getUnfurlMedia() unfurlMedia} to unfurlMedia.
* @param unfurlMedia The value for unfurlMedia
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setUnfurlMedia(Optional unfurlMedia) {
this.unfurlMedia = unfurlMedia.orElse(null);
return this;
}
/**
* Initializes the optional value {@link AbstractSlashCommandResponseParams#getReplyBroadcast() replyBroadcast} to replyBroadcast.
* @param replyBroadcast The value for replyBroadcast, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
public final Builder setReplyBroadcast(@Nullable Boolean replyBroadcast) {
this.replyBroadcast = replyBroadcast;
return this;
}
/**
* Initializes the optional value {@link AbstractSlashCommandResponseParams#getReplyBroadcast() replyBroadcast} to replyBroadcast.
* @param replyBroadcast The value for replyBroadcast
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setReplyBroadcast(Optional replyBroadcast) {
this.replyBroadcast = replyBroadcast.orElse(null);
return this;
}
/**
* Initializes the value for the {@link AbstractSlashCommandResponseParams#getResponseType() responseType} attribute.
*
If not set, this attribute will have a default value as returned by the initializer of {@link AbstractSlashCommandResponseParams#getResponseType() responseType}.
* @param responseType The value for responseType
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setResponseType(TopLevelMessageResponseType responseType) {
this.responseType = Objects.requireNonNull(responseType, "responseType");
return this;
}
/**
* Builds a new {@link SlashCommandResponseParams SlashCommandResponseParams}.
* @return An immutable instance of SlashCommandResponseParams
* @throws com.hubspot.immutables.validation.InvalidImmutableStateException if any required attributes are missing
*/
public SlashCommandResponseParams build() {
checkRequiredAttributes();
return SlashCommandResponseParams.validate(new SlashCommandResponseParams(this));
}
private boolean blocksIsSet() {
return (optBits & OPT_BIT_BLOCKS) != 0;
}
private boolean channelIdIsSet() {
return (initBits & INIT_BIT_CHANNEL_ID) == 0;
}
private void checkRequiredAttributes() {
if (initBits != 0) {
throw new InvalidImmutableStateException(formatRequiredAttributesMessage());
}
}
private String formatRequiredAttributesMessage() {
List attributes = new ArrayList<>();
if (!channelIdIsSet()) attributes.add("channelId");
return "Cannot build SlashCommandResponseParams, some of required attributes are not set " + attributes;
}
}
private static List createSafeList(Iterable iterable, boolean checkNulls, boolean skipNulls) {
ArrayList list;
if (iterable instanceof Collection) {
int size = ((Collection) iterable).size();
if (size == 0) return Collections.emptyList();
list = new ArrayList<>(size);
} else {
list = new ArrayList<>();
}
for (T element : iterable) {
if (skipNulls && element == null) continue;
if (checkNulls) Objects.requireNonNull(element, "element");
list.add(element);
}
return list;
}
private static List createUnmodifiableList(boolean clone, List list) {
switch(list.size()) {
case 0: return Collections.emptyList();
case 1: return Collections.singletonList(list.get(0));
default:
if (clone) {
return Collections.unmodifiableList(new ArrayList<>(list));
} else {
if (list instanceof ArrayList) {
((ArrayList) list).trimToSize();
}
return Collections.unmodifiableList(list);
}
}
}
}