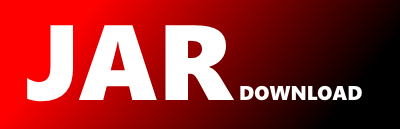
com.hubspot.slack.client.methods.params.views.UpdateViewParams Maven / Gradle / Ivy
package com.hubspot.slack.client.methods.params.views;
import com.fasterxml.jackson.annotation.JsonAutoDetect;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.hubspot.immutables.validation.InvalidImmutableStateException;
import com.hubspot.slack.client.models.views.ModalViewPayload;
import edu.umd.cs.findbugs.annotations.SuppressFBWarnings;
import java.util.ArrayList;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
import javax.annotation.ParametersAreNonnullByDefault;
import javax.annotation.concurrent.Immutable;
import javax.annotation.concurrent.NotThreadSafe;
import org.immutables.value.Generated;
/**
* Immutable implementation of {@link UpdateViewParamsIF}.
*
* Use the builder to create immutable instances:
* {@code UpdateViewParams.builder()}.
* Use the static factory method to create immutable instances:
* {@code UpdateViewParams.of()}.
*/
@Generated(from = "UpdateViewParamsIF", generator = "Immutables")
@SuppressWarnings({"all"})
@SuppressFBWarnings
@ParametersAreNonnullByDefault
@javax.annotation.processing.Generated("org.immutables.processor.ProxyProcessor")
@Immutable
public final class UpdateViewParams
implements UpdateViewParamsIF {
private final ModalViewPayload view;
private final @Nullable String externalId;
private final @Nullable String hash;
private final @Nullable String viewId;
private UpdateViewParams(ModalViewPayload view) {
this.view = Objects.requireNonNull(view, "view");
this.externalId = null;
this.hash = null;
this.viewId = null;
}
private UpdateViewParams(
ModalViewPayload view,
@Nullable String externalId,
@Nullable String hash,
@Nullable String viewId) {
this.view = view;
this.externalId = externalId;
this.hash = hash;
this.viewId = viewId;
}
/**
* @return The value of the {@code view} attribute
*/
@JsonProperty
@Override
public ModalViewPayload getView() {
return view;
}
/**
* @return The value of the {@code externalId} attribute
*/
@JsonProperty
@Override
public Optional getExternalId() {
return Optional.ofNullable(externalId);
}
/**
* @return The value of the {@code hash} attribute
*/
@JsonProperty
@Override
public Optional getHash() {
return Optional.ofNullable(hash);
}
/**
* @return The value of the {@code viewId} attribute
*/
@JsonProperty
@Override
public Optional getViewId() {
return Optional.ofNullable(viewId);
}
/**
* Copy the current immutable object by setting a value for the {@link UpdateViewParamsIF#getView() view} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for view
* @return A modified copy of the {@code this} object
*/
public final UpdateViewParams withView(ModalViewPayload value) {
if (this.view == value) return this;
ModalViewPayload newValue = Objects.requireNonNull(value, "view");
return validate(new UpdateViewParams(newValue, this.externalId, this.hash, this.viewId));
}
/**
* Copy the current immutable object by setting a present value for the optional {@link UpdateViewParamsIF#getExternalId() externalId} attribute.
* @param value The value for externalId, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final UpdateViewParams withExternalId(@Nullable String value) {
@Nullable String newValue = value;
if (Objects.equals(this.externalId, newValue)) return this;
return validate(new UpdateViewParams(this.view, newValue, this.hash, this.viewId));
}
/**
* Copy the current immutable object by setting an optional value for the {@link UpdateViewParamsIF#getExternalId() externalId} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for externalId
* @return A modified copy of {@code this} object
*/
public final UpdateViewParams withExternalId(Optional optional) {
@Nullable String value = optional.orElse(null);
if (Objects.equals(this.externalId, value)) return this;
return validate(new UpdateViewParams(this.view, value, this.hash, this.viewId));
}
/**
* Copy the current immutable object by setting a present value for the optional {@link UpdateViewParamsIF#getHash() hash} attribute.
* @param value The value for hash, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final UpdateViewParams withHash(@Nullable String value) {
@Nullable String newValue = value;
if (Objects.equals(this.hash, newValue)) return this;
return validate(new UpdateViewParams(this.view, this.externalId, newValue, this.viewId));
}
/**
* Copy the current immutable object by setting an optional value for the {@link UpdateViewParamsIF#getHash() hash} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for hash
* @return A modified copy of {@code this} object
*/
public final UpdateViewParams withHash(Optional optional) {
@Nullable String value = optional.orElse(null);
if (Objects.equals(this.hash, value)) return this;
return validate(new UpdateViewParams(this.view, this.externalId, value, this.viewId));
}
/**
* Copy the current immutable object by setting a present value for the optional {@link UpdateViewParamsIF#getViewId() viewId} attribute.
* @param value The value for viewId, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final UpdateViewParams withViewId(@Nullable String value) {
@Nullable String newValue = value;
if (Objects.equals(this.viewId, newValue)) return this;
return validate(new UpdateViewParams(this.view, this.externalId, this.hash, newValue));
}
/**
* Copy the current immutable object by setting an optional value for the {@link UpdateViewParamsIF#getViewId() viewId} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for viewId
* @return A modified copy of {@code this} object
*/
public final UpdateViewParams withViewId(Optional optional) {
@Nullable String value = optional.orElse(null);
if (Objects.equals(this.viewId, value)) return this;
return validate(new UpdateViewParams(this.view, this.externalId, this.hash, value));
}
/**
* This instance is equal to all instances of {@code UpdateViewParams} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(@Nullable Object another) {
if (this == another) return true;
return another instanceof UpdateViewParams
&& equalTo(0, (UpdateViewParams) another);
}
private boolean equalTo(int synthetic, UpdateViewParams another) {
return view.equals(another.view)
&& Objects.equals(externalId, another.externalId)
&& Objects.equals(hash, another.hash)
&& Objects.equals(viewId, another.viewId);
}
/**
* Computes a hash code from attributes: {@code view}, {@code externalId}, {@code hash}, {@code viewId}.
* @return hashCode value
*/
@Override
public int hashCode() {
int h = 5381;
h += (h << 5) + view.hashCode();
h += (h << 5) + Objects.hashCode(externalId);
h += (h << 5) + Objects.hashCode(hash);
h += (h << 5) + Objects.hashCode(viewId);
return h;
}
/**
* Prints the immutable value {@code UpdateViewParams} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
StringBuilder builder = new StringBuilder("UpdateViewParams{");
builder.append("view=").append(view);
if (externalId != null) {
builder.append(", ");
builder.append("externalId=").append(externalId);
}
if (hash != null) {
builder.append(", ");
builder.append("hash=").append(hash);
}
if (viewId != null) {
builder.append(", ");
builder.append("viewId=").append(viewId);
}
return builder.append("}").toString();
}
/**
* Utility type used to correctly read immutable object from JSON representation.
* @deprecated Do not use this type directly, it exists only for the Jackson-binding infrastructure
*/
@Generated(from = "UpdateViewParamsIF", generator = "Immutables")
@Deprecated
@JsonAutoDetect(fieldVisibility = JsonAutoDetect.Visibility.NONE)
static final class Json implements UpdateViewParamsIF {
@Nullable ModalViewPayload view;
@Nullable Optional externalId = Optional.empty();
@Nullable Optional hash = Optional.empty();
@Nullable Optional viewId = Optional.empty();
@JsonProperty
public void setView(ModalViewPayload view) {
this.view = view;
}
@JsonProperty
public void setExternalId(Optional externalId) {
this.externalId = externalId;
}
@JsonProperty
public void setHash(Optional hash) {
this.hash = hash;
}
@JsonProperty
public void setViewId(Optional viewId) {
this.viewId = viewId;
}
@Override
public ModalViewPayload getView() { throw new UnsupportedOperationException(); }
@Override
public Optional getExternalId() { throw new UnsupportedOperationException(); }
@Override
public Optional getHash() { throw new UnsupportedOperationException(); }
@Override
public Optional getViewId() { throw new UnsupportedOperationException(); }
}
/**
* @param json A JSON-bindable data structure
* @return An immutable value type
* @deprecated Do not use this method directly, it exists only for the Jackson-binding infrastructure
*/
@Deprecated
@JsonCreator(mode = JsonCreator.Mode.DELEGATING)
static UpdateViewParams fromJson(Json json) {
UpdateViewParams.Builder builder = UpdateViewParams.builder();
if (json.view != null) {
builder.setView(json.view);
}
if (json.externalId != null) {
builder.setExternalId(json.externalId);
}
if (json.hash != null) {
builder.setHash(json.hash);
}
if (json.viewId != null) {
builder.setViewId(json.viewId);
}
return builder.build();
}
/**
* Construct a new immutable {@code UpdateViewParams} instance.
* @param view The value for the {@code view} attribute
* @return An immutable UpdateViewParams instance
*/
public static UpdateViewParams of(ModalViewPayload view) {
return validate(new UpdateViewParams(view));
}
private static UpdateViewParams validate(UpdateViewParams instance) {
instance.check();
return instance;
}
/**
* Creates an immutable copy of a {@link UpdateViewParamsIF} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable UpdateViewParams instance
*/
public static UpdateViewParams copyOf(UpdateViewParamsIF instance) {
if (instance instanceof UpdateViewParams) {
return (UpdateViewParams) instance;
}
return UpdateViewParams.builder()
.from(instance)
.build();
}
/**
* Creates a builder for {@link UpdateViewParams UpdateViewParams}.
*
* UpdateViewParams.builder()
* .setView(com.hubspot.slack.client.models.views.ModalViewPayload) // required {@link UpdateViewParamsIF#getView() view}
* .setExternalId(String) // optional {@link UpdateViewParamsIF#getExternalId() externalId}
* .setHash(String) // optional {@link UpdateViewParamsIF#getHash() hash}
* .setViewId(String) // optional {@link UpdateViewParamsIF#getViewId() viewId}
* .build();
*
* @return A new UpdateViewParams builder
*/
public static UpdateViewParams.Builder builder() {
return new UpdateViewParams.Builder();
}
/**
* Builds instances of type {@link UpdateViewParams UpdateViewParams}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
@Generated(from = "UpdateViewParamsIF", generator = "Immutables")
@NotThreadSafe
public static final class Builder {
private static final long INIT_BIT_VIEW = 0x1L;
private long initBits = 0x1L;
private @Nullable ModalViewPayload view;
private @Nullable String externalId;
private @Nullable String hash;
private @Nullable String viewId;
private Builder() {
}
/**
* Fill a builder with attribute values from the provided {@code UpdateViewParamsIF} instance.
* Regular attribute values will be replaced with those from the given instance.
* Absent optional values will not replace present values.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(UpdateViewParamsIF instance) {
Objects.requireNonNull(instance, "instance");
this.setView(instance.getView());
Optional externalIdOptional = instance.getExternalId();
if (externalIdOptional.isPresent()) {
setExternalId(externalIdOptional);
}
Optional hashOptional = instance.getHash();
if (hashOptional.isPresent()) {
setHash(hashOptional);
}
Optional viewIdOptional = instance.getViewId();
if (viewIdOptional.isPresent()) {
setViewId(viewIdOptional);
}
return this;
}
/**
* Initializes the value for the {@link UpdateViewParamsIF#getView() view} attribute.
* @param view The value for view
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setView(ModalViewPayload view) {
this.view = Objects.requireNonNull(view, "view");
initBits &= ~INIT_BIT_VIEW;
return this;
}
/**
* Initializes the optional value {@link UpdateViewParamsIF#getExternalId() externalId} to externalId.
* @param externalId The value for externalId, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
public final Builder setExternalId(@Nullable String externalId) {
this.externalId = externalId;
return this;
}
/**
* Initializes the optional value {@link UpdateViewParamsIF#getExternalId() externalId} to externalId.
* @param externalId The value for externalId
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setExternalId(Optional externalId) {
this.externalId = externalId.orElse(null);
return this;
}
/**
* Initializes the optional value {@link UpdateViewParamsIF#getHash() hash} to hash.
* @param hash The value for hash, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
public final Builder setHash(@Nullable String hash) {
this.hash = hash;
return this;
}
/**
* Initializes the optional value {@link UpdateViewParamsIF#getHash() hash} to hash.
* @param hash The value for hash
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setHash(Optional hash) {
this.hash = hash.orElse(null);
return this;
}
/**
* Initializes the optional value {@link UpdateViewParamsIF#getViewId() viewId} to viewId.
* @param viewId The value for viewId, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
public final Builder setViewId(@Nullable String viewId) {
this.viewId = viewId;
return this;
}
/**
* Initializes the optional value {@link UpdateViewParamsIF#getViewId() viewId} to viewId.
* @param viewId The value for viewId
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setViewId(Optional viewId) {
this.viewId = viewId.orElse(null);
return this;
}
/**
* Builds a new {@link UpdateViewParams UpdateViewParams}.
* @return An immutable instance of UpdateViewParams
* @throws com.hubspot.immutables.validation.InvalidImmutableStateException if any required attributes are missing
*/
public UpdateViewParams build() {
checkRequiredAttributes();
return UpdateViewParams.validate(new UpdateViewParams(view, externalId, hash, viewId));
}
private boolean viewIsSet() {
return (initBits & INIT_BIT_VIEW) == 0;
}
private void checkRequiredAttributes() {
if (initBits != 0) {
throw new InvalidImmutableStateException(formatRequiredAttributesMessage());
}
}
private String formatRequiredAttributesMessage() {
List attributes = new ArrayList<>();
if (!viewIsSet()) attributes.add("view");
return "Cannot build UpdateViewParams, some of required attributes are not set " + attributes;
}
}
}