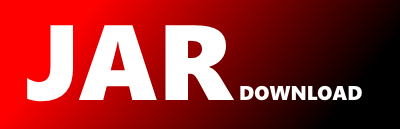
com.hubspot.slack.client.models.Attachment Maven / Gradle / Ivy
package com.hubspot.slack.client.models;
import com.fasterxml.jackson.annotation.JsonAutoDetect;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.hubspot.slack.client.models.actions.Action;
import com.hubspot.slack.client.models.blocks.Block;
import edu.umd.cs.findbugs.annotations.SuppressFBWarnings;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.LinkedHashSet;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.Set;
import javax.annotation.Nullable;
import javax.annotation.ParametersAreNonnullByDefault;
import javax.annotation.concurrent.Immutable;
import javax.annotation.concurrent.NotThreadSafe;
import org.immutables.value.Generated;
/**
* Immutable implementation of {@link AttachmentIF}.
*
* Use the builder to create immutable instances:
* {@code Attachment.builder()}.
*/
@Generated(from = "AttachmentIF", generator = "Immutables")
@SuppressWarnings({"all"})
@SuppressFBWarnings
@ParametersAreNonnullByDefault
@javax.annotation.processing.Generated("org.immutables.processor.ProxyProcessor")
@Immutable
public final class Attachment implements AttachmentIF {
private final @Nullable String fallback;
private final @Nullable String color;
private final @Nullable String pretext;
private final @Nullable String authorName;
private final @Nullable String authorLink;
private final @Nullable String authorIcon;
private final @Nullable String title;
private final @Nullable String titleLink;
private final @Nullable String text;
private final @Nullable String imageUrl;
private final @Nullable String attachmentType;
private final List fields;
private final @Nullable String footer;
private final @Nullable String footerIcon;
private final @Nullable String thumbUrl;
private final @Nullable String epochSeconds;
private final @Nullable String callbackId;
private final List actions;
private final Set mrkdwnIn;
private final List blocks;
private Attachment(
@Nullable String fallback,
@Nullable String color,
@Nullable String pretext,
@Nullable String authorName,
@Nullable String authorLink,
@Nullable String authorIcon,
@Nullable String title,
@Nullable String titleLink,
@Nullable String text,
@Nullable String imageUrl,
@Nullable String attachmentType,
List fields,
@Nullable String footer,
@Nullable String footerIcon,
@Nullable String thumbUrl,
@Nullable String epochSeconds,
@Nullable String callbackId,
List actions,
Set mrkdwnIn,
List blocks) {
this.fallback = fallback;
this.color = color;
this.pretext = pretext;
this.authorName = authorName;
this.authorLink = authorLink;
this.authorIcon = authorIcon;
this.title = title;
this.titleLink = titleLink;
this.text = text;
this.imageUrl = imageUrl;
this.attachmentType = attachmentType;
this.fields = fields;
this.footer = footer;
this.footerIcon = footerIcon;
this.thumbUrl = thumbUrl;
this.epochSeconds = epochSeconds;
this.callbackId = callbackId;
this.actions = actions;
this.mrkdwnIn = mrkdwnIn;
this.blocks = blocks;
}
/**
* @return The value of the {@code fallback} attribute
*/
@JsonProperty
@Override
public Optional getFallback() {
return Optional.ofNullable(fallback);
}
/**
* @return The value of the {@code color} attribute
*/
@JsonProperty
@Override
public Optional getColor() {
return Optional.ofNullable(color);
}
/**
* @return The value of the {@code pretext} attribute
*/
@JsonProperty
@Override
public Optional getPretext() {
return Optional.ofNullable(pretext);
}
/**
* @return The value of the {@code authorName} attribute
*/
@JsonProperty
@Override
public Optional getAuthorName() {
return Optional.ofNullable(authorName);
}
/**
* @return The value of the {@code authorLink} attribute
*/
@JsonProperty
@Override
public Optional getAuthorLink() {
return Optional.ofNullable(authorLink);
}
/**
* @return The value of the {@code authorIcon} attribute
*/
@JsonProperty
@Override
public Optional getAuthorIcon() {
return Optional.ofNullable(authorIcon);
}
/**
* @return The value of the {@code title} attribute
*/
@JsonProperty
@Override
public Optional getTitle() {
return Optional.ofNullable(title);
}
/**
* @return The value of the {@code titleLink} attribute
*/
@JsonProperty
@Override
public Optional getTitleLink() {
return Optional.ofNullable(titleLink);
}
/**
* @return The value of the {@code text} attribute
*/
@JsonProperty
@Override
public Optional getText() {
return Optional.ofNullable(text);
}
/**
* @return The value of the {@code imageUrl} attribute
*/
@JsonProperty
@Override
public Optional getImageUrl() {
return Optional.ofNullable(imageUrl);
}
/**
* @return The value of the {@code attachmentType} attribute
*/
@JsonProperty
@Override
public Optional getAttachmentType() {
return Optional.ofNullable(attachmentType);
}
/**
* @return The value of the {@code fields} attribute
*/
@JsonProperty
@Override
public List getFields() {
return fields;
}
/**
* @return The value of the {@code footer} attribute
*/
@JsonProperty
@Override
public Optional getFooter() {
return Optional.ofNullable(footer);
}
/**
* @return The value of the {@code footerIcon} attribute
*/
@JsonProperty
@Override
public Optional getFooterIcon() {
return Optional.ofNullable(footerIcon);
}
/**
* @return The value of the {@code thumbUrl} attribute
*/
@JsonProperty
@Override
public Optional getThumbUrl() {
return Optional.ofNullable(thumbUrl);
}
/**
* @return The value of the {@code epochSeconds} attribute
*/
@JsonProperty("ts")
@Override
public Optional getEpochSeconds() {
return Optional.ofNullable(epochSeconds);
}
/**
* @return The value of the {@code callbackId} attribute
*/
@JsonProperty
@Override
public Optional getCallbackId() {
return Optional.ofNullable(callbackId);
}
/**
* @return The value of the {@code actions} attribute
*/
@JsonProperty
@Override
public List getActions() {
return actions;
}
/**
* Slack will only markdown in fields whose names are included in this set. See {@link MarkdownSupportedFields}
*/
@JsonProperty
@Override
public Set getMrkdwnIn() {
return mrkdwnIn;
}
/**
* @return The value of the {@code blocks} attribute
*/
@JsonProperty
@JsonInclude(JsonInclude.Include.NON_EMPTY)
@Override
public List getBlocks() {
return blocks;
}
/**
* Copy the current immutable object by setting a present value for the optional {@link AttachmentIF#getFallback() fallback} attribute.
* @param value The value for fallback, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final Attachment withFallback(@Nullable String value) {
@Nullable String newValue = value;
if (Objects.equals(this.fallback, newValue)) return this;
return new Attachment(
newValue,
this.color,
this.pretext,
this.authorName,
this.authorLink,
this.authorIcon,
this.title,
this.titleLink,
this.text,
this.imageUrl,
this.attachmentType,
this.fields,
this.footer,
this.footerIcon,
this.thumbUrl,
this.epochSeconds,
this.callbackId,
this.actions,
this.mrkdwnIn,
this.blocks);
}
/**
* Copy the current immutable object by setting an optional value for the {@link AttachmentIF#getFallback() fallback} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for fallback
* @return A modified copy of {@code this} object
*/
public final Attachment withFallback(Optional optional) {
@Nullable String value = optional.orElse(null);
if (Objects.equals(this.fallback, value)) return this;
return new Attachment(
value,
this.color,
this.pretext,
this.authorName,
this.authorLink,
this.authorIcon,
this.title,
this.titleLink,
this.text,
this.imageUrl,
this.attachmentType,
this.fields,
this.footer,
this.footerIcon,
this.thumbUrl,
this.epochSeconds,
this.callbackId,
this.actions,
this.mrkdwnIn,
this.blocks);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link AttachmentIF#getColor() color} attribute.
* @param value The value for color, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final Attachment withColor(@Nullable String value) {
@Nullable String newValue = value;
if (Objects.equals(this.color, newValue)) return this;
return new Attachment(
this.fallback,
newValue,
this.pretext,
this.authorName,
this.authorLink,
this.authorIcon,
this.title,
this.titleLink,
this.text,
this.imageUrl,
this.attachmentType,
this.fields,
this.footer,
this.footerIcon,
this.thumbUrl,
this.epochSeconds,
this.callbackId,
this.actions,
this.mrkdwnIn,
this.blocks);
}
/**
* Copy the current immutable object by setting an optional value for the {@link AttachmentIF#getColor() color} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for color
* @return A modified copy of {@code this} object
*/
public final Attachment withColor(Optional optional) {
@Nullable String value = optional.orElse(null);
if (Objects.equals(this.color, value)) return this;
return new Attachment(
this.fallback,
value,
this.pretext,
this.authorName,
this.authorLink,
this.authorIcon,
this.title,
this.titleLink,
this.text,
this.imageUrl,
this.attachmentType,
this.fields,
this.footer,
this.footerIcon,
this.thumbUrl,
this.epochSeconds,
this.callbackId,
this.actions,
this.mrkdwnIn,
this.blocks);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link AttachmentIF#getPretext() pretext} attribute.
* @param value The value for pretext, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final Attachment withPretext(@Nullable String value) {
@Nullable String newValue = value;
if (Objects.equals(this.pretext, newValue)) return this;
return new Attachment(
this.fallback,
this.color,
newValue,
this.authorName,
this.authorLink,
this.authorIcon,
this.title,
this.titleLink,
this.text,
this.imageUrl,
this.attachmentType,
this.fields,
this.footer,
this.footerIcon,
this.thumbUrl,
this.epochSeconds,
this.callbackId,
this.actions,
this.mrkdwnIn,
this.blocks);
}
/**
* Copy the current immutable object by setting an optional value for the {@link AttachmentIF#getPretext() pretext} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for pretext
* @return A modified copy of {@code this} object
*/
public final Attachment withPretext(Optional optional) {
@Nullable String value = optional.orElse(null);
if (Objects.equals(this.pretext, value)) return this;
return new Attachment(
this.fallback,
this.color,
value,
this.authorName,
this.authorLink,
this.authorIcon,
this.title,
this.titleLink,
this.text,
this.imageUrl,
this.attachmentType,
this.fields,
this.footer,
this.footerIcon,
this.thumbUrl,
this.epochSeconds,
this.callbackId,
this.actions,
this.mrkdwnIn,
this.blocks);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link AttachmentIF#getAuthorName() authorName} attribute.
* @param value The value for authorName, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final Attachment withAuthorName(@Nullable String value) {
@Nullable String newValue = value;
if (Objects.equals(this.authorName, newValue)) return this;
return new Attachment(
this.fallback,
this.color,
this.pretext,
newValue,
this.authorLink,
this.authorIcon,
this.title,
this.titleLink,
this.text,
this.imageUrl,
this.attachmentType,
this.fields,
this.footer,
this.footerIcon,
this.thumbUrl,
this.epochSeconds,
this.callbackId,
this.actions,
this.mrkdwnIn,
this.blocks);
}
/**
* Copy the current immutable object by setting an optional value for the {@link AttachmentIF#getAuthorName() authorName} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for authorName
* @return A modified copy of {@code this} object
*/
public final Attachment withAuthorName(Optional optional) {
@Nullable String value = optional.orElse(null);
if (Objects.equals(this.authorName, value)) return this;
return new Attachment(
this.fallback,
this.color,
this.pretext,
value,
this.authorLink,
this.authorIcon,
this.title,
this.titleLink,
this.text,
this.imageUrl,
this.attachmentType,
this.fields,
this.footer,
this.footerIcon,
this.thumbUrl,
this.epochSeconds,
this.callbackId,
this.actions,
this.mrkdwnIn,
this.blocks);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link AttachmentIF#getAuthorLink() authorLink} attribute.
* @param value The value for authorLink, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final Attachment withAuthorLink(@Nullable String value) {
@Nullable String newValue = value;
if (Objects.equals(this.authorLink, newValue)) return this;
return new Attachment(
this.fallback,
this.color,
this.pretext,
this.authorName,
newValue,
this.authorIcon,
this.title,
this.titleLink,
this.text,
this.imageUrl,
this.attachmentType,
this.fields,
this.footer,
this.footerIcon,
this.thumbUrl,
this.epochSeconds,
this.callbackId,
this.actions,
this.mrkdwnIn,
this.blocks);
}
/**
* Copy the current immutable object by setting an optional value for the {@link AttachmentIF#getAuthorLink() authorLink} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for authorLink
* @return A modified copy of {@code this} object
*/
public final Attachment withAuthorLink(Optional optional) {
@Nullable String value = optional.orElse(null);
if (Objects.equals(this.authorLink, value)) return this;
return new Attachment(
this.fallback,
this.color,
this.pretext,
this.authorName,
value,
this.authorIcon,
this.title,
this.titleLink,
this.text,
this.imageUrl,
this.attachmentType,
this.fields,
this.footer,
this.footerIcon,
this.thumbUrl,
this.epochSeconds,
this.callbackId,
this.actions,
this.mrkdwnIn,
this.blocks);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link AttachmentIF#getAuthorIcon() authorIcon} attribute.
* @param value The value for authorIcon, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final Attachment withAuthorIcon(@Nullable String value) {
@Nullable String newValue = value;
if (Objects.equals(this.authorIcon, newValue)) return this;
return new Attachment(
this.fallback,
this.color,
this.pretext,
this.authorName,
this.authorLink,
newValue,
this.title,
this.titleLink,
this.text,
this.imageUrl,
this.attachmentType,
this.fields,
this.footer,
this.footerIcon,
this.thumbUrl,
this.epochSeconds,
this.callbackId,
this.actions,
this.mrkdwnIn,
this.blocks);
}
/**
* Copy the current immutable object by setting an optional value for the {@link AttachmentIF#getAuthorIcon() authorIcon} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for authorIcon
* @return A modified copy of {@code this} object
*/
public final Attachment withAuthorIcon(Optional optional) {
@Nullable String value = optional.orElse(null);
if (Objects.equals(this.authorIcon, value)) return this;
return new Attachment(
this.fallback,
this.color,
this.pretext,
this.authorName,
this.authorLink,
value,
this.title,
this.titleLink,
this.text,
this.imageUrl,
this.attachmentType,
this.fields,
this.footer,
this.footerIcon,
this.thumbUrl,
this.epochSeconds,
this.callbackId,
this.actions,
this.mrkdwnIn,
this.blocks);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link AttachmentIF#getTitle() title} attribute.
* @param value The value for title, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final Attachment withTitle(@Nullable String value) {
@Nullable String newValue = value;
if (Objects.equals(this.title, newValue)) return this;
return new Attachment(
this.fallback,
this.color,
this.pretext,
this.authorName,
this.authorLink,
this.authorIcon,
newValue,
this.titleLink,
this.text,
this.imageUrl,
this.attachmentType,
this.fields,
this.footer,
this.footerIcon,
this.thumbUrl,
this.epochSeconds,
this.callbackId,
this.actions,
this.mrkdwnIn,
this.blocks);
}
/**
* Copy the current immutable object by setting an optional value for the {@link AttachmentIF#getTitle() title} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for title
* @return A modified copy of {@code this} object
*/
public final Attachment withTitle(Optional optional) {
@Nullable String value = optional.orElse(null);
if (Objects.equals(this.title, value)) return this;
return new Attachment(
this.fallback,
this.color,
this.pretext,
this.authorName,
this.authorLink,
this.authorIcon,
value,
this.titleLink,
this.text,
this.imageUrl,
this.attachmentType,
this.fields,
this.footer,
this.footerIcon,
this.thumbUrl,
this.epochSeconds,
this.callbackId,
this.actions,
this.mrkdwnIn,
this.blocks);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link AttachmentIF#getTitleLink() titleLink} attribute.
* @param value The value for titleLink, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final Attachment withTitleLink(@Nullable String value) {
@Nullable String newValue = value;
if (Objects.equals(this.titleLink, newValue)) return this;
return new Attachment(
this.fallback,
this.color,
this.pretext,
this.authorName,
this.authorLink,
this.authorIcon,
this.title,
newValue,
this.text,
this.imageUrl,
this.attachmentType,
this.fields,
this.footer,
this.footerIcon,
this.thumbUrl,
this.epochSeconds,
this.callbackId,
this.actions,
this.mrkdwnIn,
this.blocks);
}
/**
* Copy the current immutable object by setting an optional value for the {@link AttachmentIF#getTitleLink() titleLink} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for titleLink
* @return A modified copy of {@code this} object
*/
public final Attachment withTitleLink(Optional optional) {
@Nullable String value = optional.orElse(null);
if (Objects.equals(this.titleLink, value)) return this;
return new Attachment(
this.fallback,
this.color,
this.pretext,
this.authorName,
this.authorLink,
this.authorIcon,
this.title,
value,
this.text,
this.imageUrl,
this.attachmentType,
this.fields,
this.footer,
this.footerIcon,
this.thumbUrl,
this.epochSeconds,
this.callbackId,
this.actions,
this.mrkdwnIn,
this.blocks);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link AttachmentIF#getText() text} attribute.
* @param value The value for text, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final Attachment withText(@Nullable String value) {
@Nullable String newValue = value;
if (Objects.equals(this.text, newValue)) return this;
return new Attachment(
this.fallback,
this.color,
this.pretext,
this.authorName,
this.authorLink,
this.authorIcon,
this.title,
this.titleLink,
newValue,
this.imageUrl,
this.attachmentType,
this.fields,
this.footer,
this.footerIcon,
this.thumbUrl,
this.epochSeconds,
this.callbackId,
this.actions,
this.mrkdwnIn,
this.blocks);
}
/**
* Copy the current immutable object by setting an optional value for the {@link AttachmentIF#getText() text} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for text
* @return A modified copy of {@code this} object
*/
public final Attachment withText(Optional optional) {
@Nullable String value = optional.orElse(null);
if (Objects.equals(this.text, value)) return this;
return new Attachment(
this.fallback,
this.color,
this.pretext,
this.authorName,
this.authorLink,
this.authorIcon,
this.title,
this.titleLink,
value,
this.imageUrl,
this.attachmentType,
this.fields,
this.footer,
this.footerIcon,
this.thumbUrl,
this.epochSeconds,
this.callbackId,
this.actions,
this.mrkdwnIn,
this.blocks);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link AttachmentIF#getImageUrl() imageUrl} attribute.
* @param value The value for imageUrl, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final Attachment withImageUrl(@Nullable String value) {
@Nullable String newValue = value;
if (Objects.equals(this.imageUrl, newValue)) return this;
return new Attachment(
this.fallback,
this.color,
this.pretext,
this.authorName,
this.authorLink,
this.authorIcon,
this.title,
this.titleLink,
this.text,
newValue,
this.attachmentType,
this.fields,
this.footer,
this.footerIcon,
this.thumbUrl,
this.epochSeconds,
this.callbackId,
this.actions,
this.mrkdwnIn,
this.blocks);
}
/**
* Copy the current immutable object by setting an optional value for the {@link AttachmentIF#getImageUrl() imageUrl} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for imageUrl
* @return A modified copy of {@code this} object
*/
public final Attachment withImageUrl(Optional optional) {
@Nullable String value = optional.orElse(null);
if (Objects.equals(this.imageUrl, value)) return this;
return new Attachment(
this.fallback,
this.color,
this.pretext,
this.authorName,
this.authorLink,
this.authorIcon,
this.title,
this.titleLink,
this.text,
value,
this.attachmentType,
this.fields,
this.footer,
this.footerIcon,
this.thumbUrl,
this.epochSeconds,
this.callbackId,
this.actions,
this.mrkdwnIn,
this.blocks);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link AttachmentIF#getAttachmentType() attachmentType} attribute.
* @param value The value for attachmentType, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final Attachment withAttachmentType(@Nullable String value) {
@Nullable String newValue = value;
if (Objects.equals(this.attachmentType, newValue)) return this;
return new Attachment(
this.fallback,
this.color,
this.pretext,
this.authorName,
this.authorLink,
this.authorIcon,
this.title,
this.titleLink,
this.text,
this.imageUrl,
newValue,
this.fields,
this.footer,
this.footerIcon,
this.thumbUrl,
this.epochSeconds,
this.callbackId,
this.actions,
this.mrkdwnIn,
this.blocks);
}
/**
* Copy the current immutable object by setting an optional value for the {@link AttachmentIF#getAttachmentType() attachmentType} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for attachmentType
* @return A modified copy of {@code this} object
*/
public final Attachment withAttachmentType(Optional optional) {
@Nullable String value = optional.orElse(null);
if (Objects.equals(this.attachmentType, value)) return this;
return new Attachment(
this.fallback,
this.color,
this.pretext,
this.authorName,
this.authorLink,
this.authorIcon,
this.title,
this.titleLink,
this.text,
this.imageUrl,
value,
this.fields,
this.footer,
this.footerIcon,
this.thumbUrl,
this.epochSeconds,
this.callbackId,
this.actions,
this.mrkdwnIn,
this.blocks);
}
/**
* Copy the current immutable object with elements that replace the content of {@link AttachmentIF#getFields() fields}.
* @param elements The elements to set
* @return A modified copy of {@code this} object
*/
public final Attachment withFields(Field... elements) {
List newValue = createUnmodifiableList(false, createSafeList(Arrays.asList(elements), true, false));
return new Attachment(
this.fallback,
this.color,
this.pretext,
this.authorName,
this.authorLink,
this.authorIcon,
this.title,
this.titleLink,
this.text,
this.imageUrl,
this.attachmentType,
newValue,
this.footer,
this.footerIcon,
this.thumbUrl,
this.epochSeconds,
this.callbackId,
this.actions,
this.mrkdwnIn,
this.blocks);
}
/**
* Copy the current immutable object with elements that replace the content of {@link AttachmentIF#getFields() fields}.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements An iterable of fields elements to set
* @return A modified copy of {@code this} object
*/
public final Attachment withFields(Iterable extends Field> elements) {
if (this.fields == elements) return this;
List newValue = createUnmodifiableList(false, createSafeList(elements, true, false));
return new Attachment(
this.fallback,
this.color,
this.pretext,
this.authorName,
this.authorLink,
this.authorIcon,
this.title,
this.titleLink,
this.text,
this.imageUrl,
this.attachmentType,
newValue,
this.footer,
this.footerIcon,
this.thumbUrl,
this.epochSeconds,
this.callbackId,
this.actions,
this.mrkdwnIn,
this.blocks);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link AttachmentIF#getFooter() footer} attribute.
* @param value The value for footer, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final Attachment withFooter(@Nullable String value) {
@Nullable String newValue = value;
if (Objects.equals(this.footer, newValue)) return this;
return new Attachment(
this.fallback,
this.color,
this.pretext,
this.authorName,
this.authorLink,
this.authorIcon,
this.title,
this.titleLink,
this.text,
this.imageUrl,
this.attachmentType,
this.fields,
newValue,
this.footerIcon,
this.thumbUrl,
this.epochSeconds,
this.callbackId,
this.actions,
this.mrkdwnIn,
this.blocks);
}
/**
* Copy the current immutable object by setting an optional value for the {@link AttachmentIF#getFooter() footer} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for footer
* @return A modified copy of {@code this} object
*/
public final Attachment withFooter(Optional optional) {
@Nullable String value = optional.orElse(null);
if (Objects.equals(this.footer, value)) return this;
return new Attachment(
this.fallback,
this.color,
this.pretext,
this.authorName,
this.authorLink,
this.authorIcon,
this.title,
this.titleLink,
this.text,
this.imageUrl,
this.attachmentType,
this.fields,
value,
this.footerIcon,
this.thumbUrl,
this.epochSeconds,
this.callbackId,
this.actions,
this.mrkdwnIn,
this.blocks);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link AttachmentIF#getFooterIcon() footerIcon} attribute.
* @param value The value for footerIcon, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final Attachment withFooterIcon(@Nullable String value) {
@Nullable String newValue = value;
if (Objects.equals(this.footerIcon, newValue)) return this;
return new Attachment(
this.fallback,
this.color,
this.pretext,
this.authorName,
this.authorLink,
this.authorIcon,
this.title,
this.titleLink,
this.text,
this.imageUrl,
this.attachmentType,
this.fields,
this.footer,
newValue,
this.thumbUrl,
this.epochSeconds,
this.callbackId,
this.actions,
this.mrkdwnIn,
this.blocks);
}
/**
* Copy the current immutable object by setting an optional value for the {@link AttachmentIF#getFooterIcon() footerIcon} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for footerIcon
* @return A modified copy of {@code this} object
*/
public final Attachment withFooterIcon(Optional optional) {
@Nullable String value = optional.orElse(null);
if (Objects.equals(this.footerIcon, value)) return this;
return new Attachment(
this.fallback,
this.color,
this.pretext,
this.authorName,
this.authorLink,
this.authorIcon,
this.title,
this.titleLink,
this.text,
this.imageUrl,
this.attachmentType,
this.fields,
this.footer,
value,
this.thumbUrl,
this.epochSeconds,
this.callbackId,
this.actions,
this.mrkdwnIn,
this.blocks);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link AttachmentIF#getThumbUrl() thumbUrl} attribute.
* @param value The value for thumbUrl, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final Attachment withThumbUrl(@Nullable String value) {
@Nullable String newValue = value;
if (Objects.equals(this.thumbUrl, newValue)) return this;
return new Attachment(
this.fallback,
this.color,
this.pretext,
this.authorName,
this.authorLink,
this.authorIcon,
this.title,
this.titleLink,
this.text,
this.imageUrl,
this.attachmentType,
this.fields,
this.footer,
this.footerIcon,
newValue,
this.epochSeconds,
this.callbackId,
this.actions,
this.mrkdwnIn,
this.blocks);
}
/**
* Copy the current immutable object by setting an optional value for the {@link AttachmentIF#getThumbUrl() thumbUrl} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for thumbUrl
* @return A modified copy of {@code this} object
*/
public final Attachment withThumbUrl(Optional optional) {
@Nullable String value = optional.orElse(null);
if (Objects.equals(this.thumbUrl, value)) return this;
return new Attachment(
this.fallback,
this.color,
this.pretext,
this.authorName,
this.authorLink,
this.authorIcon,
this.title,
this.titleLink,
this.text,
this.imageUrl,
this.attachmentType,
this.fields,
this.footer,
this.footerIcon,
value,
this.epochSeconds,
this.callbackId,
this.actions,
this.mrkdwnIn,
this.blocks);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link AttachmentIF#getEpochSeconds() epochSeconds} attribute.
* @param value The value for epochSeconds, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final Attachment withEpochSeconds(@Nullable String value) {
@Nullable String newValue = value;
if (Objects.equals(this.epochSeconds, newValue)) return this;
return new Attachment(
this.fallback,
this.color,
this.pretext,
this.authorName,
this.authorLink,
this.authorIcon,
this.title,
this.titleLink,
this.text,
this.imageUrl,
this.attachmentType,
this.fields,
this.footer,
this.footerIcon,
this.thumbUrl,
newValue,
this.callbackId,
this.actions,
this.mrkdwnIn,
this.blocks);
}
/**
* Copy the current immutable object by setting an optional value for the {@link AttachmentIF#getEpochSeconds() epochSeconds} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for epochSeconds
* @return A modified copy of {@code this} object
*/
public final Attachment withEpochSeconds(Optional optional) {
@Nullable String value = optional.orElse(null);
if (Objects.equals(this.epochSeconds, value)) return this;
return new Attachment(
this.fallback,
this.color,
this.pretext,
this.authorName,
this.authorLink,
this.authorIcon,
this.title,
this.titleLink,
this.text,
this.imageUrl,
this.attachmentType,
this.fields,
this.footer,
this.footerIcon,
this.thumbUrl,
value,
this.callbackId,
this.actions,
this.mrkdwnIn,
this.blocks);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link AttachmentIF#getCallbackId() callbackId} attribute.
* @param value The value for callbackId, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final Attachment withCallbackId(@Nullable String value) {
@Nullable String newValue = value;
if (Objects.equals(this.callbackId, newValue)) return this;
return new Attachment(
this.fallback,
this.color,
this.pretext,
this.authorName,
this.authorLink,
this.authorIcon,
this.title,
this.titleLink,
this.text,
this.imageUrl,
this.attachmentType,
this.fields,
this.footer,
this.footerIcon,
this.thumbUrl,
this.epochSeconds,
newValue,
this.actions,
this.mrkdwnIn,
this.blocks);
}
/**
* Copy the current immutable object by setting an optional value for the {@link AttachmentIF#getCallbackId() callbackId} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for callbackId
* @return A modified copy of {@code this} object
*/
public final Attachment withCallbackId(Optional optional) {
@Nullable String value = optional.orElse(null);
if (Objects.equals(this.callbackId, value)) return this;
return new Attachment(
this.fallback,
this.color,
this.pretext,
this.authorName,
this.authorLink,
this.authorIcon,
this.title,
this.titleLink,
this.text,
this.imageUrl,
this.attachmentType,
this.fields,
this.footer,
this.footerIcon,
this.thumbUrl,
this.epochSeconds,
value,
this.actions,
this.mrkdwnIn,
this.blocks);
}
/**
* Copy the current immutable object with elements that replace the content of {@link AttachmentIF#getActions() actions}.
* @param elements The elements to set
* @return A modified copy of {@code this} object
*/
public final Attachment withActions(Action... elements) {
List newValue = createUnmodifiableList(false, createSafeList(Arrays.asList(elements), true, false));
return new Attachment(
this.fallback,
this.color,
this.pretext,
this.authorName,
this.authorLink,
this.authorIcon,
this.title,
this.titleLink,
this.text,
this.imageUrl,
this.attachmentType,
this.fields,
this.footer,
this.footerIcon,
this.thumbUrl,
this.epochSeconds,
this.callbackId,
newValue,
this.mrkdwnIn,
this.blocks);
}
/**
* Copy the current immutable object with elements that replace the content of {@link AttachmentIF#getActions() actions}.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements An iterable of actions elements to set
* @return A modified copy of {@code this} object
*/
public final Attachment withActions(Iterable extends Action> elements) {
if (this.actions == elements) return this;
List newValue = createUnmodifiableList(false, createSafeList(elements, true, false));
return new Attachment(
this.fallback,
this.color,
this.pretext,
this.authorName,
this.authorLink,
this.authorIcon,
this.title,
this.titleLink,
this.text,
this.imageUrl,
this.attachmentType,
this.fields,
this.footer,
this.footerIcon,
this.thumbUrl,
this.epochSeconds,
this.callbackId,
newValue,
this.mrkdwnIn,
this.blocks);
}
/**
* Copy the current immutable object with elements that replace the content of {@link AttachmentIF#getMrkdwnIn() mrkdwnIn}.
* @param elements The elements to set
* @return A modified copy of {@code this} object
*/
public final Attachment withMrkdwnIn(String... elements) {
Set newValue = createUnmodifiableSet(createSafeList(Arrays.asList(elements), true, false));
return new Attachment(
this.fallback,
this.color,
this.pretext,
this.authorName,
this.authorLink,
this.authorIcon,
this.title,
this.titleLink,
this.text,
this.imageUrl,
this.attachmentType,
this.fields,
this.footer,
this.footerIcon,
this.thumbUrl,
this.epochSeconds,
this.callbackId,
this.actions,
newValue,
this.blocks);
}
/**
* Copy the current immutable object with elements that replace the content of {@link AttachmentIF#getMrkdwnIn() mrkdwnIn}.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements An iterable of mrkdwnIn elements to set
* @return A modified copy of {@code this} object
*/
public final Attachment withMrkdwnIn(Iterable elements) {
if (this.mrkdwnIn == elements) return this;
Set newValue = createUnmodifiableSet(createSafeList(elements, true, false));
return new Attachment(
this.fallback,
this.color,
this.pretext,
this.authorName,
this.authorLink,
this.authorIcon,
this.title,
this.titleLink,
this.text,
this.imageUrl,
this.attachmentType,
this.fields,
this.footer,
this.footerIcon,
this.thumbUrl,
this.epochSeconds,
this.callbackId,
this.actions,
newValue,
this.blocks);
}
/**
* Copy the current immutable object with elements that replace the content of {@link AttachmentIF#getBlocks() blocks}.
* @param elements The elements to set
* @return A modified copy of {@code this} object
*/
public final Attachment withBlocks(Block... elements) {
List newValue = createUnmodifiableList(false, createSafeList(Arrays.asList(elements), true, false));
return new Attachment(
this.fallback,
this.color,
this.pretext,
this.authorName,
this.authorLink,
this.authorIcon,
this.title,
this.titleLink,
this.text,
this.imageUrl,
this.attachmentType,
this.fields,
this.footer,
this.footerIcon,
this.thumbUrl,
this.epochSeconds,
this.callbackId,
this.actions,
this.mrkdwnIn,
newValue);
}
/**
* Copy the current immutable object with elements that replace the content of {@link AttachmentIF#getBlocks() blocks}.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements An iterable of blocks elements to set
* @return A modified copy of {@code this} object
*/
public final Attachment withBlocks(Iterable extends Block> elements) {
if (this.blocks == elements) return this;
List newValue = createUnmodifiableList(false, createSafeList(elements, true, false));
return new Attachment(
this.fallback,
this.color,
this.pretext,
this.authorName,
this.authorLink,
this.authorIcon,
this.title,
this.titleLink,
this.text,
this.imageUrl,
this.attachmentType,
this.fields,
this.footer,
this.footerIcon,
this.thumbUrl,
this.epochSeconds,
this.callbackId,
this.actions,
this.mrkdwnIn,
newValue);
}
/**
* This instance is equal to all instances of {@code Attachment} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(@Nullable Object another) {
if (this == another) return true;
return another instanceof Attachment
&& equalTo(0, (Attachment) another);
}
private boolean equalTo(int synthetic, Attachment another) {
return Objects.equals(fallback, another.fallback)
&& Objects.equals(color, another.color)
&& Objects.equals(pretext, another.pretext)
&& Objects.equals(authorName, another.authorName)
&& Objects.equals(authorLink, another.authorLink)
&& Objects.equals(authorIcon, another.authorIcon)
&& Objects.equals(title, another.title)
&& Objects.equals(titleLink, another.titleLink)
&& Objects.equals(text, another.text)
&& Objects.equals(imageUrl, another.imageUrl)
&& Objects.equals(attachmentType, another.attachmentType)
&& fields.equals(another.fields)
&& Objects.equals(footer, another.footer)
&& Objects.equals(footerIcon, another.footerIcon)
&& Objects.equals(thumbUrl, another.thumbUrl)
&& Objects.equals(epochSeconds, another.epochSeconds)
&& Objects.equals(callbackId, another.callbackId)
&& actions.equals(another.actions)
&& mrkdwnIn.equals(another.mrkdwnIn)
&& blocks.equals(another.blocks);
}
/**
* Computes a hash code from attributes: {@code fallback}, {@code color}, {@code pretext}, {@code authorName}, {@code authorLink}, {@code authorIcon}, {@code title}, {@code titleLink}, {@code text}, {@code imageUrl}, {@code attachmentType}, {@code fields}, {@code footer}, {@code footerIcon}, {@code thumbUrl}, {@code epochSeconds}, {@code callbackId}, {@code actions}, {@code mrkdwnIn}, {@code blocks}.
* @return hashCode value
*/
@Override
public int hashCode() {
int h = 5381;
h += (h << 5) + Objects.hashCode(fallback);
h += (h << 5) + Objects.hashCode(color);
h += (h << 5) + Objects.hashCode(pretext);
h += (h << 5) + Objects.hashCode(authorName);
h += (h << 5) + Objects.hashCode(authorLink);
h += (h << 5) + Objects.hashCode(authorIcon);
h += (h << 5) + Objects.hashCode(title);
h += (h << 5) + Objects.hashCode(titleLink);
h += (h << 5) + Objects.hashCode(text);
h += (h << 5) + Objects.hashCode(imageUrl);
h += (h << 5) + Objects.hashCode(attachmentType);
h += (h << 5) + fields.hashCode();
h += (h << 5) + Objects.hashCode(footer);
h += (h << 5) + Objects.hashCode(footerIcon);
h += (h << 5) + Objects.hashCode(thumbUrl);
h += (h << 5) + Objects.hashCode(epochSeconds);
h += (h << 5) + Objects.hashCode(callbackId);
h += (h << 5) + actions.hashCode();
h += (h << 5) + mrkdwnIn.hashCode();
h += (h << 5) + blocks.hashCode();
return h;
}
/**
* Prints the immutable value {@code Attachment} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
StringBuilder builder = new StringBuilder("Attachment{");
if (fallback != null) {
builder.append("fallback=").append(fallback);
}
if (color != null) {
if (builder.length() > 11) builder.append(", ");
builder.append("color=").append(color);
}
if (pretext != null) {
if (builder.length() > 11) builder.append(", ");
builder.append("pretext=").append(pretext);
}
if (authorName != null) {
if (builder.length() > 11) builder.append(", ");
builder.append("authorName=").append(authorName);
}
if (authorLink != null) {
if (builder.length() > 11) builder.append(", ");
builder.append("authorLink=").append(authorLink);
}
if (authorIcon != null) {
if (builder.length() > 11) builder.append(", ");
builder.append("authorIcon=").append(authorIcon);
}
if (title != null) {
if (builder.length() > 11) builder.append(", ");
builder.append("title=").append(title);
}
if (titleLink != null) {
if (builder.length() > 11) builder.append(", ");
builder.append("titleLink=").append(titleLink);
}
if (text != null) {
if (builder.length() > 11) builder.append(", ");
builder.append("text=").append(text);
}
if (imageUrl != null) {
if (builder.length() > 11) builder.append(", ");
builder.append("imageUrl=").append(imageUrl);
}
if (attachmentType != null) {
if (builder.length() > 11) builder.append(", ");
builder.append("attachmentType=").append(attachmentType);
}
if (builder.length() > 11) builder.append(", ");
builder.append("fields=").append(fields);
if (footer != null) {
builder.append(", ");
builder.append("footer=").append(footer);
}
if (footerIcon != null) {
builder.append(", ");
builder.append("footerIcon=").append(footerIcon);
}
if (thumbUrl != null) {
builder.append(", ");
builder.append("thumbUrl=").append(thumbUrl);
}
if (epochSeconds != null) {
builder.append(", ");
builder.append("epochSeconds=").append(epochSeconds);
}
if (callbackId != null) {
builder.append(", ");
builder.append("callbackId=").append(callbackId);
}
builder.append(", ");
builder.append("actions=").append(actions);
builder.append(", ");
builder.append("mrkdwnIn=").append(mrkdwnIn);
builder.append(", ");
builder.append("blocks=").append(blocks);
return builder.append("}").toString();
}
/**
* Utility type used to correctly read immutable object from JSON representation.
* @deprecated Do not use this type directly, it exists only for the Jackson-binding infrastructure
*/
@Generated(from = "AttachmentIF", generator = "Immutables")
@Deprecated
@JsonAutoDetect(fieldVisibility = JsonAutoDetect.Visibility.NONE)
static final class Json implements AttachmentIF {
@Nullable Optional fallback = Optional.empty();
@Nullable Optional color = Optional.empty();
@Nullable Optional pretext = Optional.empty();
@Nullable Optional authorName = Optional.empty();
@Nullable Optional authorLink = Optional.empty();
@Nullable Optional authorIcon = Optional.empty();
@Nullable Optional title = Optional.empty();
@Nullable Optional titleLink = Optional.empty();
@Nullable Optional text = Optional.empty();
@Nullable Optional imageUrl = Optional.empty();
@Nullable Optional attachmentType = Optional.empty();
@Nullable List fields = Collections.emptyList();
@Nullable Optional footer = Optional.empty();
@Nullable Optional footerIcon = Optional.empty();
@Nullable Optional thumbUrl = Optional.empty();
@Nullable Optional epochSeconds = Optional.empty();
@Nullable Optional callbackId = Optional.empty();
@Nullable List actions = Collections.emptyList();
@Nullable Set mrkdwnIn = Collections.emptySet();
@Nullable List blocks = Collections.emptyList();
@JsonProperty
public void setFallback(Optional fallback) {
this.fallback = fallback;
}
@JsonProperty
public void setColor(Optional color) {
this.color = color;
}
@JsonProperty
public void setPretext(Optional pretext) {
this.pretext = pretext;
}
@JsonProperty
public void setAuthorName(Optional authorName) {
this.authorName = authorName;
}
@JsonProperty
public void setAuthorLink(Optional authorLink) {
this.authorLink = authorLink;
}
@JsonProperty
public void setAuthorIcon(Optional authorIcon) {
this.authorIcon = authorIcon;
}
@JsonProperty
public void setTitle(Optional title) {
this.title = title;
}
@JsonProperty
public void setTitleLink(Optional titleLink) {
this.titleLink = titleLink;
}
@JsonProperty
public void setText(Optional text) {
this.text = text;
}
@JsonProperty
public void setImageUrl(Optional imageUrl) {
this.imageUrl = imageUrl;
}
@JsonProperty
public void setAttachmentType(Optional attachmentType) {
this.attachmentType = attachmentType;
}
@JsonProperty
public void setFields(List fields) {
this.fields = fields;
}
@JsonProperty
public void setFooter(Optional footer) {
this.footer = footer;
}
@JsonProperty
public void setFooterIcon(Optional footerIcon) {
this.footerIcon = footerIcon;
}
@JsonProperty
public void setThumbUrl(Optional thumbUrl) {
this.thumbUrl = thumbUrl;
}
@JsonProperty("ts")
public void setEpochSeconds(Optional epochSeconds) {
this.epochSeconds = epochSeconds;
}
@JsonProperty
public void setCallbackId(Optional callbackId) {
this.callbackId = callbackId;
}
@JsonProperty
public void setActions(List actions) {
this.actions = actions;
}
@JsonProperty
public void setMrkdwnIn(Set mrkdwnIn) {
this.mrkdwnIn = mrkdwnIn;
}
@JsonProperty
@JsonInclude(JsonInclude.Include.NON_EMPTY)
public void setBlocks(List blocks) {
this.blocks = blocks;
}
@Override
public Optional getFallback() { throw new UnsupportedOperationException(); }
@Override
public Optional getColor() { throw new UnsupportedOperationException(); }
@Override
public Optional getPretext() { throw new UnsupportedOperationException(); }
@Override
public Optional getAuthorName() { throw new UnsupportedOperationException(); }
@Override
public Optional getAuthorLink() { throw new UnsupportedOperationException(); }
@Override
public Optional getAuthorIcon() { throw new UnsupportedOperationException(); }
@Override
public Optional getTitle() { throw new UnsupportedOperationException(); }
@Override
public Optional getTitleLink() { throw new UnsupportedOperationException(); }
@Override
public Optional getText() { throw new UnsupportedOperationException(); }
@Override
public Optional getImageUrl() { throw new UnsupportedOperationException(); }
@Override
public Optional getAttachmentType() { throw new UnsupportedOperationException(); }
@Override
public List getFields() { throw new UnsupportedOperationException(); }
@Override
public Optional getFooter() { throw new UnsupportedOperationException(); }
@Override
public Optional getFooterIcon() { throw new UnsupportedOperationException(); }
@Override
public Optional getThumbUrl() { throw new UnsupportedOperationException(); }
@Override
public Optional getEpochSeconds() { throw new UnsupportedOperationException(); }
@Override
public Optional getCallbackId() { throw new UnsupportedOperationException(); }
@Override
public List getActions() { throw new UnsupportedOperationException(); }
@Override
public Set getMrkdwnIn() { throw new UnsupportedOperationException(); }
@Override
public List getBlocks() { throw new UnsupportedOperationException(); }
}
/**
* @param json A JSON-bindable data structure
* @return An immutable value type
* @deprecated Do not use this method directly, it exists only for the Jackson-binding infrastructure
*/
@Deprecated
@JsonCreator(mode = JsonCreator.Mode.DELEGATING)
static Attachment fromJson(Json json) {
Attachment.Builder builder = Attachment.builder();
if (json.fallback != null) {
builder.setFallback(json.fallback);
}
if (json.color != null) {
builder.setColor(json.color);
}
if (json.pretext != null) {
builder.setPretext(json.pretext);
}
if (json.authorName != null) {
builder.setAuthorName(json.authorName);
}
if (json.authorLink != null) {
builder.setAuthorLink(json.authorLink);
}
if (json.authorIcon != null) {
builder.setAuthorIcon(json.authorIcon);
}
if (json.title != null) {
builder.setTitle(json.title);
}
if (json.titleLink != null) {
builder.setTitleLink(json.titleLink);
}
if (json.text != null) {
builder.setText(json.text);
}
if (json.imageUrl != null) {
builder.setImageUrl(json.imageUrl);
}
if (json.attachmentType != null) {
builder.setAttachmentType(json.attachmentType);
}
if (json.fields != null) {
builder.addAllFields(json.fields);
}
if (json.footer != null) {
builder.setFooter(json.footer);
}
if (json.footerIcon != null) {
builder.setFooterIcon(json.footerIcon);
}
if (json.thumbUrl != null) {
builder.setThumbUrl(json.thumbUrl);
}
if (json.epochSeconds != null) {
builder.setEpochSeconds(json.epochSeconds);
}
if (json.callbackId != null) {
builder.setCallbackId(json.callbackId);
}
if (json.actions != null) {
builder.addAllActions(json.actions);
}
if (json.mrkdwnIn != null) {
builder.addAllMrkdwnIn(json.mrkdwnIn);
}
if (json.blocks != null) {
builder.addAllBlocks(json.blocks);
}
return builder.build();
}
/**
* Creates an immutable copy of a {@link AttachmentIF} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable Attachment instance
*/
public static Attachment copyOf(AttachmentIF instance) {
if (instance instanceof Attachment) {
return (Attachment) instance;
}
return Attachment.builder()
.from(instance)
.build();
}
/**
* Creates a builder for {@link Attachment Attachment}.
*
* Attachment.builder()
* .setFallback(String) // optional {@link AttachmentIF#getFallback() fallback}
* .setColor(String) // optional {@link AttachmentIF#getColor() color}
* .setPretext(String) // optional {@link AttachmentIF#getPretext() pretext}
* .setAuthorName(String) // optional {@link AttachmentIF#getAuthorName() authorName}
* .setAuthorLink(String) // optional {@link AttachmentIF#getAuthorLink() authorLink}
* .setAuthorIcon(String) // optional {@link AttachmentIF#getAuthorIcon() authorIcon}
* .setTitle(String) // optional {@link AttachmentIF#getTitle() title}
* .setTitleLink(String) // optional {@link AttachmentIF#getTitleLink() titleLink}
* .setText(String) // optional {@link AttachmentIF#getText() text}
* .setImageUrl(String) // optional {@link AttachmentIF#getImageUrl() imageUrl}
* .setAttachmentType(String) // optional {@link AttachmentIF#getAttachmentType() attachmentType}
* .addFields|addAllFields(Field) // {@link AttachmentIF#getFields() fields} elements
* .setFooter(String) // optional {@link AttachmentIF#getFooter() footer}
* .setFooterIcon(String) // optional {@link AttachmentIF#getFooterIcon() footerIcon}
* .setThumbUrl(String) // optional {@link AttachmentIF#getThumbUrl() thumbUrl}
* .setEpochSeconds(String) // optional {@link AttachmentIF#getEpochSeconds() epochSeconds}
* .setCallbackId(String) // optional {@link AttachmentIF#getCallbackId() callbackId}
* .addActions|addAllActions(com.hubspot.slack.client.models.actions.Action) // {@link AttachmentIF#getActions() actions} elements
* .addMrkdwnIn|addAllMrkdwnIn(String) // {@link AttachmentIF#getMrkdwnIn() mrkdwnIn} elements
* .addBlocks|addAllBlocks(com.hubspot.slack.client.models.blocks.Block) // {@link AttachmentIF#getBlocks() blocks} elements
* .build();
*
* @return A new Attachment builder
*/
public static Attachment.Builder builder() {
return new Attachment.Builder();
}
/**
* Builds instances of type {@link Attachment Attachment}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
@Generated(from = "AttachmentIF", generator = "Immutables")
@NotThreadSafe
public static final class Builder {
private @Nullable String fallback;
private @Nullable String color;
private @Nullable String pretext;
private @Nullable String authorName;
private @Nullable String authorLink;
private @Nullable String authorIcon;
private @Nullable String title;
private @Nullable String titleLink;
private @Nullable String text;
private @Nullable String imageUrl;
private @Nullable String attachmentType;
private List fields = new ArrayList();
private @Nullable String footer;
private @Nullable String footerIcon;
private @Nullable String thumbUrl;
private @Nullable String epochSeconds;
private @Nullable String callbackId;
private List actions = new ArrayList();
private List mrkdwnIn = new ArrayList();
private List blocks = new ArrayList();
private Builder() {
}
/**
* Fill a builder with attribute values from the provided {@code AttachmentIF} instance.
* Regular attribute values will be replaced with those from the given instance.
* Absent optional values will not replace present values.
* Collection elements and entries will be added, not replaced.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(AttachmentIF instance) {
Objects.requireNonNull(instance, "instance");
Optional fallbackOptional = instance.getFallback();
if (fallbackOptional.isPresent()) {
setFallback(fallbackOptional);
}
Optional colorOptional = instance.getColor();
if (colorOptional.isPresent()) {
setColor(colorOptional);
}
Optional pretextOptional = instance.getPretext();
if (pretextOptional.isPresent()) {
setPretext(pretextOptional);
}
Optional authorNameOptional = instance.getAuthorName();
if (authorNameOptional.isPresent()) {
setAuthorName(authorNameOptional);
}
Optional authorLinkOptional = instance.getAuthorLink();
if (authorLinkOptional.isPresent()) {
setAuthorLink(authorLinkOptional);
}
Optional authorIconOptional = instance.getAuthorIcon();
if (authorIconOptional.isPresent()) {
setAuthorIcon(authorIconOptional);
}
Optional titleOptional = instance.getTitle();
if (titleOptional.isPresent()) {
setTitle(titleOptional);
}
Optional titleLinkOptional = instance.getTitleLink();
if (titleLinkOptional.isPresent()) {
setTitleLink(titleLinkOptional);
}
Optional textOptional = instance.getText();
if (textOptional.isPresent()) {
setText(textOptional);
}
Optional imageUrlOptional = instance.getImageUrl();
if (imageUrlOptional.isPresent()) {
setImageUrl(imageUrlOptional);
}
Optional attachmentTypeOptional = instance.getAttachmentType();
if (attachmentTypeOptional.isPresent()) {
setAttachmentType(attachmentTypeOptional);
}
addAllFields(instance.getFields());
Optional footerOptional = instance.getFooter();
if (footerOptional.isPresent()) {
setFooter(footerOptional);
}
Optional footerIconOptional = instance.getFooterIcon();
if (footerIconOptional.isPresent()) {
setFooterIcon(footerIconOptional);
}
Optional thumbUrlOptional = instance.getThumbUrl();
if (thumbUrlOptional.isPresent()) {
setThumbUrl(thumbUrlOptional);
}
Optional epochSecondsOptional = instance.getEpochSeconds();
if (epochSecondsOptional.isPresent()) {
setEpochSeconds(epochSecondsOptional);
}
Optional callbackIdOptional = instance.getCallbackId();
if (callbackIdOptional.isPresent()) {
setCallbackId(callbackIdOptional);
}
addAllActions(instance.getActions());
addAllMrkdwnIn(instance.getMrkdwnIn());
addAllBlocks(instance.getBlocks());
return this;
}
/**
* Initializes the optional value {@link AttachmentIF#getFallback() fallback} to fallback.
* @param fallback The value for fallback, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
public final Builder setFallback(@Nullable String fallback) {
this.fallback = fallback;
return this;
}
/**
* Initializes the optional value {@link AttachmentIF#getFallback() fallback} to fallback.
* @param fallback The value for fallback
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setFallback(Optional fallback) {
this.fallback = fallback.orElse(null);
return this;
}
/**
* Initializes the optional value {@link AttachmentIF#getColor() color} to color.
* @param color The value for color, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
public final Builder setColor(@Nullable String color) {
this.color = color;
return this;
}
/**
* Initializes the optional value {@link AttachmentIF#getColor() color} to color.
* @param color The value for color
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setColor(Optional color) {
this.color = color.orElse(null);
return this;
}
/**
* Initializes the optional value {@link AttachmentIF#getPretext() pretext} to pretext.
* @param pretext The value for pretext, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
public final Builder setPretext(@Nullable String pretext) {
this.pretext = pretext;
return this;
}
/**
* Initializes the optional value {@link AttachmentIF#getPretext() pretext} to pretext.
* @param pretext The value for pretext
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setPretext(Optional pretext) {
this.pretext = pretext.orElse(null);
return this;
}
/**
* Initializes the optional value {@link AttachmentIF#getAuthorName() authorName} to authorName.
* @param authorName The value for authorName, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
public final Builder setAuthorName(@Nullable String authorName) {
this.authorName = authorName;
return this;
}
/**
* Initializes the optional value {@link AttachmentIF#getAuthorName() authorName} to authorName.
* @param authorName The value for authorName
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setAuthorName(Optional authorName) {
this.authorName = authorName.orElse(null);
return this;
}
/**
* Initializes the optional value {@link AttachmentIF#getAuthorLink() authorLink} to authorLink.
* @param authorLink The value for authorLink, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
public final Builder setAuthorLink(@Nullable String authorLink) {
this.authorLink = authorLink;
return this;
}
/**
* Initializes the optional value {@link AttachmentIF#getAuthorLink() authorLink} to authorLink.
* @param authorLink The value for authorLink
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setAuthorLink(Optional authorLink) {
this.authorLink = authorLink.orElse(null);
return this;
}
/**
* Initializes the optional value {@link AttachmentIF#getAuthorIcon() authorIcon} to authorIcon.
* @param authorIcon The value for authorIcon, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
public final Builder setAuthorIcon(@Nullable String authorIcon) {
this.authorIcon = authorIcon;
return this;
}
/**
* Initializes the optional value {@link AttachmentIF#getAuthorIcon() authorIcon} to authorIcon.
* @param authorIcon The value for authorIcon
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setAuthorIcon(Optional authorIcon) {
this.authorIcon = authorIcon.orElse(null);
return this;
}
/**
* Initializes the optional value {@link AttachmentIF#getTitle() title} to title.
* @param title The value for title, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
public final Builder setTitle(@Nullable String title) {
this.title = title;
return this;
}
/**
* Initializes the optional value {@link AttachmentIF#getTitle() title} to title.
* @param title The value for title
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setTitle(Optional title) {
this.title = title.orElse(null);
return this;
}
/**
* Initializes the optional value {@link AttachmentIF#getTitleLink() titleLink} to titleLink.
* @param titleLink The value for titleLink, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
public final Builder setTitleLink(@Nullable String titleLink) {
this.titleLink = titleLink;
return this;
}
/**
* Initializes the optional value {@link AttachmentIF#getTitleLink() titleLink} to titleLink.
* @param titleLink The value for titleLink
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setTitleLink(Optional titleLink) {
this.titleLink = titleLink.orElse(null);
return this;
}
/**
* Initializes the optional value {@link AttachmentIF#getText() text} to text.
* @param text The value for text, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
public final Builder setText(@Nullable String text) {
this.text = text;
return this;
}
/**
* Initializes the optional value {@link AttachmentIF#getText() text} to text.
* @param text The value for text
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setText(Optional text) {
this.text = text.orElse(null);
return this;
}
/**
* Initializes the optional value {@link AttachmentIF#getImageUrl() imageUrl} to imageUrl.
* @param imageUrl The value for imageUrl, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
public final Builder setImageUrl(@Nullable String imageUrl) {
this.imageUrl = imageUrl;
return this;
}
/**
* Initializes the optional value {@link AttachmentIF#getImageUrl() imageUrl} to imageUrl.
* @param imageUrl The value for imageUrl
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setImageUrl(Optional imageUrl) {
this.imageUrl = imageUrl.orElse(null);
return this;
}
/**
* Initializes the optional value {@link AttachmentIF#getAttachmentType() attachmentType} to attachmentType.
* @param attachmentType The value for attachmentType, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
public final Builder setAttachmentType(@Nullable String attachmentType) {
this.attachmentType = attachmentType;
return this;
}
/**
* Initializes the optional value {@link AttachmentIF#getAttachmentType() attachmentType} to attachmentType.
* @param attachmentType The value for attachmentType
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setAttachmentType(Optional attachmentType) {
this.attachmentType = attachmentType.orElse(null);
return this;
}
/**
* Adds one element to {@link AttachmentIF#getFields() fields} list.
* @param element A fields element
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addFields(Field element) {
this.fields.add(Objects.requireNonNull(element, "fields element"));
return this;
}
/**
* Adds elements to {@link AttachmentIF#getFields() fields} list.
* @param elements An array of fields elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addFields(Field... elements) {
for (Field element : elements) {
this.fields.add(Objects.requireNonNull(element, "fields element"));
}
return this;
}
/**
* Sets or replaces all elements for {@link AttachmentIF#getFields() fields} list.
* @param elements An iterable of fields elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setFields(Iterable extends Field> elements) {
this.fields.clear();
return addAllFields(elements);
}
/**
* Adds elements to {@link AttachmentIF#getFields() fields} list.
* @param elements An iterable of fields elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addAllFields(Iterable extends Field> elements) {
for (Field element : elements) {
this.fields.add(Objects.requireNonNull(element, "fields element"));
}
return this;
}
/**
* Initializes the optional value {@link AttachmentIF#getFooter() footer} to footer.
* @param footer The value for footer, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
public final Builder setFooter(@Nullable String footer) {
this.footer = footer;
return this;
}
/**
* Initializes the optional value {@link AttachmentIF#getFooter() footer} to footer.
* @param footer The value for footer
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setFooter(Optional footer) {
this.footer = footer.orElse(null);
return this;
}
/**
* Initializes the optional value {@link AttachmentIF#getFooterIcon() footerIcon} to footerIcon.
* @param footerIcon The value for footerIcon, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
public final Builder setFooterIcon(@Nullable String footerIcon) {
this.footerIcon = footerIcon;
return this;
}
/**
* Initializes the optional value {@link AttachmentIF#getFooterIcon() footerIcon} to footerIcon.
* @param footerIcon The value for footerIcon
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setFooterIcon(Optional footerIcon) {
this.footerIcon = footerIcon.orElse(null);
return this;
}
/**
* Initializes the optional value {@link AttachmentIF#getThumbUrl() thumbUrl} to thumbUrl.
* @param thumbUrl The value for thumbUrl, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
public final Builder setThumbUrl(@Nullable String thumbUrl) {
this.thumbUrl = thumbUrl;
return this;
}
/**
* Initializes the optional value {@link AttachmentIF#getThumbUrl() thumbUrl} to thumbUrl.
* @param thumbUrl The value for thumbUrl
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setThumbUrl(Optional thumbUrl) {
this.thumbUrl = thumbUrl.orElse(null);
return this;
}
/**
* Initializes the optional value {@link AttachmentIF#getEpochSeconds() epochSeconds} to epochSeconds.
* @param epochSeconds The value for epochSeconds, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
public final Builder setEpochSeconds(@Nullable String epochSeconds) {
this.epochSeconds = epochSeconds;
return this;
}
/**
* Initializes the optional value {@link AttachmentIF#getEpochSeconds() epochSeconds} to epochSeconds.
* @param epochSeconds The value for epochSeconds
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setEpochSeconds(Optional epochSeconds) {
this.epochSeconds = epochSeconds.orElse(null);
return this;
}
/**
* Initializes the optional value {@link AttachmentIF#getCallbackId() callbackId} to callbackId.
* @param callbackId The value for callbackId, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
public final Builder setCallbackId(@Nullable String callbackId) {
this.callbackId = callbackId;
return this;
}
/**
* Initializes the optional value {@link AttachmentIF#getCallbackId() callbackId} to callbackId.
* @param callbackId The value for callbackId
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setCallbackId(Optional callbackId) {
this.callbackId = callbackId.orElse(null);
return this;
}
/**
* Adds one element to {@link AttachmentIF#getActions() actions} list.
* @param element A actions element
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addActions(Action element) {
this.actions.add(Objects.requireNonNull(element, "actions element"));
return this;
}
/**
* Adds elements to {@link AttachmentIF#getActions() actions} list.
* @param elements An array of actions elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addActions(Action... elements) {
for (Action element : elements) {
this.actions.add(Objects.requireNonNull(element, "actions element"));
}
return this;
}
/**
* Sets or replaces all elements for {@link AttachmentIF#getActions() actions} list.
* @param elements An iterable of actions elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setActions(Iterable extends Action> elements) {
this.actions.clear();
return addAllActions(elements);
}
/**
* Adds elements to {@link AttachmentIF#getActions() actions} list.
* @param elements An iterable of actions elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addAllActions(Iterable extends Action> elements) {
for (Action element : elements) {
this.actions.add(Objects.requireNonNull(element, "actions element"));
}
return this;
}
/**
* Adds one element to {@link AttachmentIF#getMrkdwnIn() mrkdwnIn} set.
* @param element A mrkdwnIn element
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addMrkdwnIn(String element) {
this.mrkdwnIn.add(Objects.requireNonNull(element, "mrkdwnIn element"));
return this;
}
/**
* Adds elements to {@link AttachmentIF#getMrkdwnIn() mrkdwnIn} set.
* @param elements An array of mrkdwnIn elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addMrkdwnIn(String... elements) {
for (String element : elements) {
this.mrkdwnIn.add(Objects.requireNonNull(element, "mrkdwnIn element"));
}
return this;
}
/**
* Sets or replaces all elements for {@link AttachmentIF#getMrkdwnIn() mrkdwnIn} set.
* @param elements An iterable of mrkdwnIn elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setMrkdwnIn(Iterable elements) {
this.mrkdwnIn.clear();
return addAllMrkdwnIn(elements);
}
/**
* Adds elements to {@link AttachmentIF#getMrkdwnIn() mrkdwnIn} set.
* @param elements An iterable of mrkdwnIn elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addAllMrkdwnIn(Iterable elements) {
for (String element : elements) {
this.mrkdwnIn.add(Objects.requireNonNull(element, "mrkdwnIn element"));
}
return this;
}
/**
* Adds one element to {@link AttachmentIF#getBlocks() blocks} list.
* @param element A blocks element
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addBlocks(Block element) {
this.blocks.add(Objects.requireNonNull(element, "blocks element"));
return this;
}
/**
* Adds elements to {@link AttachmentIF#getBlocks() blocks} list.
* @param elements An array of blocks elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addBlocks(Block... elements) {
for (Block element : elements) {
this.blocks.add(Objects.requireNonNull(element, "blocks element"));
}
return this;
}
/**
* Sets or replaces all elements for {@link AttachmentIF#getBlocks() blocks} list.
* @param elements An iterable of blocks elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setBlocks(Iterable extends Block> elements) {
this.blocks.clear();
return addAllBlocks(elements);
}
/**
* Adds elements to {@link AttachmentIF#getBlocks() blocks} list.
* @param elements An iterable of blocks elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addAllBlocks(Iterable extends Block> elements) {
for (Block element : elements) {
this.blocks.add(Objects.requireNonNull(element, "blocks element"));
}
return this;
}
/**
* Builds a new {@link Attachment Attachment}.
* @return An immutable instance of Attachment
* @throws com.hubspot.immutables.validation.InvalidImmutableStateException if any required attributes are missing
*/
public Attachment build() {
return new Attachment(
fallback,
color,
pretext,
authorName,
authorLink,
authorIcon,
title,
titleLink,
text,
imageUrl,
attachmentType,
createUnmodifiableList(true, fields),
footer,
footerIcon,
thumbUrl,
epochSeconds,
callbackId,
createUnmodifiableList(true, actions),
createUnmodifiableSet(mrkdwnIn),
createUnmodifiableList(true, blocks));
}
}
private static List createSafeList(Iterable extends T> iterable, boolean checkNulls, boolean skipNulls) {
ArrayList list;
if (iterable instanceof Collection>) {
int size = ((Collection>) iterable).size();
if (size == 0) return Collections.emptyList();
list = new ArrayList<>(size);
} else {
list = new ArrayList<>();
}
for (T element : iterable) {
if (skipNulls && element == null) continue;
if (checkNulls) Objects.requireNonNull(element, "element");
list.add(element);
}
return list;
}
private static List createUnmodifiableList(boolean clone, List list) {
switch(list.size()) {
case 0: return Collections.emptyList();
case 1: return Collections.singletonList(list.get(0));
default:
if (clone) {
return Collections.unmodifiableList(new ArrayList<>(list));
} else {
if (list instanceof ArrayList>) {
((ArrayList>) list).trimToSize();
}
return Collections.unmodifiableList(list);
}
}
}
/** Unmodifiable set constructed from list to avoid rehashing. */
private static Set createUnmodifiableSet(List list) {
switch(list.size()) {
case 0: return Collections.emptySet();
case 1: return Collections.singleton(list.get(0));
default:
Set set = new LinkedHashSet<>(list.size() * 4 / 3 + 1);
set.addAll(list);
return Collections.unmodifiableSet(set);
}
}
}