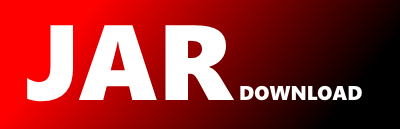
com.hubspot.slack.client.models.ChannelMetadata Maven / Gradle / Ivy
package com.hubspot.slack.client.models;
import com.fasterxml.jackson.annotation.JsonAutoDetect;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.hubspot.immutables.validation.InvalidImmutableStateException;
import edu.umd.cs.findbugs.annotations.SuppressFBWarnings;
import java.util.ArrayList;
import java.util.List;
import java.util.Objects;
import javax.annotation.Nullable;
import javax.annotation.ParametersAreNonnullByDefault;
import javax.annotation.concurrent.Immutable;
import javax.annotation.concurrent.NotThreadSafe;
import org.immutables.value.Generated;
/**
* Immutable implementation of {@link ChannelMetadataIF}.
*
* Use the builder to create immutable instances:
* {@code ChannelMetadata.builder()}.
*/
@Generated(from = "ChannelMetadataIF", generator = "Immutables")
@SuppressWarnings({"all"})
@SuppressFBWarnings
@ParametersAreNonnullByDefault
@javax.annotation.processing.Generated("org.immutables.processor.ProxyProcessor")
@Immutable
public final class ChannelMetadata implements ChannelMetadataIF {
private final String value;
private final String creatorId;
private final long lastSetEpochSeconds;
private ChannelMetadata(String value, String creatorId, long lastSetEpochSeconds) {
this.value = value;
this.creatorId = creatorId;
this.lastSetEpochSeconds = lastSetEpochSeconds;
}
/**
* @return The value of the {@code value} attribute
*/
@JsonProperty
@Override
public String getValue() {
return value;
}
/**
* @return The value of the {@code creatorId} attribute
*/
@JsonProperty("creator")
@Override
public String getCreatorId() {
return creatorId;
}
/**
* @return The value of the {@code lastSetEpochSeconds} attribute
*/
@JsonProperty("last_set")
@Override
public long getLastSetEpochSeconds() {
return lastSetEpochSeconds;
}
/**
* Copy the current immutable object by setting a value for the {@link ChannelMetadataIF#getValue() value} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for value
* @return A modified copy of the {@code this} object
*/
public final ChannelMetadata withValue(String value) {
String newValue = Objects.requireNonNull(value, "value");
if (this.value.equals(newValue)) return this;
return new ChannelMetadata(newValue, this.creatorId, this.lastSetEpochSeconds);
}
/**
* Copy the current immutable object by setting a value for the {@link ChannelMetadataIF#getCreatorId() creatorId} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for creatorId
* @return A modified copy of the {@code this} object
*/
public final ChannelMetadata withCreatorId(String value) {
String newValue = Objects.requireNonNull(value, "creatorId");
if (this.creatorId.equals(newValue)) return this;
return new ChannelMetadata(this.value, newValue, this.lastSetEpochSeconds);
}
/**
* Copy the current immutable object by setting a value for the {@link ChannelMetadataIF#getLastSetEpochSeconds() lastSetEpochSeconds} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for lastSetEpochSeconds
* @return A modified copy of the {@code this} object
*/
public final ChannelMetadata withLastSetEpochSeconds(long value) {
if (this.lastSetEpochSeconds == value) return this;
return new ChannelMetadata(this.value, this.creatorId, value);
}
/**
* This instance is equal to all instances of {@code ChannelMetadata} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(@Nullable Object another) {
if (this == another) return true;
return another instanceof ChannelMetadata
&& equalTo(0, (ChannelMetadata) another);
}
private boolean equalTo(int synthetic, ChannelMetadata another) {
return value.equals(another.value)
&& creatorId.equals(another.creatorId)
&& lastSetEpochSeconds == another.lastSetEpochSeconds;
}
/**
* Computes a hash code from attributes: {@code value}, {@code creatorId}, {@code lastSetEpochSeconds}.
* @return hashCode value
*/
@Override
public int hashCode() {
int h = 5381;
h += (h << 5) + value.hashCode();
h += (h << 5) + creatorId.hashCode();
h += (h << 5) + Long.hashCode(lastSetEpochSeconds);
return h;
}
/**
* Prints the immutable value {@code ChannelMetadata} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
return "ChannelMetadata{"
+ "value=" + value
+ ", creatorId=" + creatorId
+ ", lastSetEpochSeconds=" + lastSetEpochSeconds
+ "}";
}
/**
* Utility type used to correctly read immutable object from JSON representation.
* @deprecated Do not use this type directly, it exists only for the Jackson-binding infrastructure
*/
@Generated(from = "ChannelMetadataIF", generator = "Immutables")
@Deprecated
@JsonAutoDetect(fieldVisibility = JsonAutoDetect.Visibility.NONE)
static final class Json implements ChannelMetadataIF {
@Nullable String value;
@Nullable String creatorId;
long lastSetEpochSeconds;
boolean lastSetEpochSecondsIsSet;
@JsonProperty
public void setValue(String value) {
this.value = value;
}
@JsonProperty("creator")
public void setCreatorId(String creatorId) {
this.creatorId = creatorId;
}
@JsonProperty("last_set")
public void setLastSetEpochSeconds(long lastSetEpochSeconds) {
this.lastSetEpochSeconds = lastSetEpochSeconds;
this.lastSetEpochSecondsIsSet = true;
}
@Override
public String getValue() { throw new UnsupportedOperationException(); }
@Override
public String getCreatorId() { throw new UnsupportedOperationException(); }
@Override
public long getLastSetEpochSeconds() { throw new UnsupportedOperationException(); }
}
/**
* @param json A JSON-bindable data structure
* @return An immutable value type
* @deprecated Do not use this method directly, it exists only for the Jackson-binding infrastructure
*/
@Deprecated
@JsonCreator(mode = JsonCreator.Mode.DELEGATING)
static ChannelMetadata fromJson(Json json) {
ChannelMetadata.Builder builder = ChannelMetadata.builder();
if (json.value != null) {
builder.setValue(json.value);
}
if (json.creatorId != null) {
builder.setCreatorId(json.creatorId);
}
if (json.lastSetEpochSecondsIsSet) {
builder.setLastSetEpochSeconds(json.lastSetEpochSeconds);
}
return builder.build();
}
/**
* Creates an immutable copy of a {@link ChannelMetadataIF} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable ChannelMetadata instance
*/
public static ChannelMetadata copyOf(ChannelMetadataIF instance) {
if (instance instanceof ChannelMetadata) {
return (ChannelMetadata) instance;
}
return ChannelMetadata.builder()
.from(instance)
.build();
}
/**
* Creates a builder for {@link ChannelMetadata ChannelMetadata}.
*
* ChannelMetadata.builder()
* .setValue(String) // required {@link ChannelMetadataIF#getValue() value}
* .setCreatorId(String) // required {@link ChannelMetadataIF#getCreatorId() creatorId}
* .setLastSetEpochSeconds(long) // required {@link ChannelMetadataIF#getLastSetEpochSeconds() lastSetEpochSeconds}
* .build();
*
* @return A new ChannelMetadata builder
*/
public static ChannelMetadata.Builder builder() {
return new ChannelMetadata.Builder();
}
/**
* Builds instances of type {@link ChannelMetadata ChannelMetadata}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
@Generated(from = "ChannelMetadataIF", generator = "Immutables")
@NotThreadSafe
public static final class Builder {
private static final long INIT_BIT_VALUE = 0x1L;
private static final long INIT_BIT_CREATOR_ID = 0x2L;
private static final long INIT_BIT_LAST_SET_EPOCH_SECONDS = 0x4L;
private long initBits = 0x7L;
private @Nullable String value;
private @Nullable String creatorId;
private long lastSetEpochSeconds;
private Builder() {
}
/**
* Fill a builder with attribute values from the provided {@code ChannelMetadataIF} instance.
* Regular attribute values will be replaced with those from the given instance.
* Absent optional values will not replace present values.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(ChannelMetadataIF instance) {
Objects.requireNonNull(instance, "instance");
this.setValue(instance.getValue());
this.setCreatorId(instance.getCreatorId());
this.setLastSetEpochSeconds(instance.getLastSetEpochSeconds());
return this;
}
/**
* Initializes the value for the {@link ChannelMetadataIF#getValue() value} attribute.
* @param value The value for value
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setValue(String value) {
this.value = Objects.requireNonNull(value, "value");
initBits &= ~INIT_BIT_VALUE;
return this;
}
/**
* Initializes the value for the {@link ChannelMetadataIF#getCreatorId() creatorId} attribute.
* @param creatorId The value for creatorId
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setCreatorId(String creatorId) {
this.creatorId = Objects.requireNonNull(creatorId, "creatorId");
initBits &= ~INIT_BIT_CREATOR_ID;
return this;
}
/**
* Initializes the value for the {@link ChannelMetadataIF#getLastSetEpochSeconds() lastSetEpochSeconds} attribute.
* @param lastSetEpochSeconds The value for lastSetEpochSeconds
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setLastSetEpochSeconds(long lastSetEpochSeconds) {
this.lastSetEpochSeconds = lastSetEpochSeconds;
initBits &= ~INIT_BIT_LAST_SET_EPOCH_SECONDS;
return this;
}
/**
* Builds a new {@link ChannelMetadata ChannelMetadata}.
* @return An immutable instance of ChannelMetadata
* @throws com.hubspot.immutables.validation.InvalidImmutableStateException if any required attributes are missing
*/
public ChannelMetadata build() {
checkRequiredAttributes();
return new ChannelMetadata(value, creatorId, lastSetEpochSeconds);
}
private boolean valueIsSet() {
return (initBits & INIT_BIT_VALUE) == 0;
}
private boolean creatorIdIsSet() {
return (initBits & INIT_BIT_CREATOR_ID) == 0;
}
private boolean lastSetEpochSecondsIsSet() {
return (initBits & INIT_BIT_LAST_SET_EPOCH_SECONDS) == 0;
}
private void checkRequiredAttributes() {
if (initBits != 0) {
throw new InvalidImmutableStateException(formatRequiredAttributesMessage());
}
}
private String formatRequiredAttributesMessage() {
List attributes = new ArrayList<>();
if (!valueIsSet()) attributes.add("value");
if (!creatorIdIsSet()) attributes.add("creatorId");
if (!lastSetEpochSecondsIsSet()) attributes.add("lastSetEpochSeconds");
return "Cannot build ChannelMetadata, some of required attributes are not set " + attributes;
}
}
}