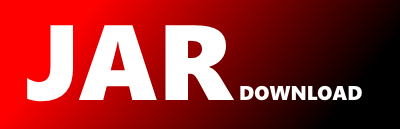
com.hubspot.slack.client.models.Message Maven / Gradle / Ivy
package com.hubspot.slack.client.models;
import com.fasterxml.jackson.annotation.JsonAutoDetect;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.hubspot.immutables.validation.InvalidImmutableStateException;
import edu.umd.cs.findbugs.annotations.SuppressFBWarnings;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
import javax.annotation.ParametersAreNonnullByDefault;
import javax.annotation.concurrent.Immutable;
import javax.annotation.concurrent.NotThreadSafe;
import org.immutables.value.Generated;
/**
* Immutable implementation of {@link MessageIF}.
*
* Use the builder to create immutable instances:
* {@code Message.builder()}.
*/
@Generated(from = "MessageIF", generator = "Immutables")
@SuppressWarnings({"all"})
@SuppressFBWarnings
@ParametersAreNonnullByDefault
@javax.annotation.processing.Generated("org.immutables.processor.ProxyProcessor")
@Immutable
public final class Message implements MessageIF {
private final String type;
private final String team;
private final SlackChannel channel;
private final @Nullable String user;
private final String username;
private final String timestamp;
private final String text;
private final @Nullable String permalink;
private final List attachments;
private final @Nullable LiteMessage previousPrevious;
private final @Nullable LiteMessage previous;
private final @Nullable LiteMessage next;
private final @Nullable LiteMessage nextNext;
private transient final String slackLink;
private Message(
String type,
String team,
SlackChannel channel,
@Nullable String user,
String username,
String timestamp,
String text,
@Nullable String permalink,
List attachments,
@Nullable LiteMessage previousPrevious,
@Nullable LiteMessage previous,
@Nullable LiteMessage next,
@Nullable LiteMessage nextNext) {
this.type = type;
this.team = team;
this.channel = channel;
this.user = user;
this.username = username;
this.timestamp = timestamp;
this.text = text;
this.permalink = permalink;
this.attachments = attachments;
this.previousPrevious = previousPrevious;
this.previous = previous;
this.next = next;
this.nextNext = nextNext;
this.slackLink = Objects.requireNonNull(MessageIF.super.getSlackLink(), "slackLink");
}
/**
* @return The value of the {@code type} attribute
*/
@JsonProperty
@Override
public String getType() {
return type;
}
/**
* @return The value of the {@code team} attribute
*/
@JsonProperty
@Override
public String getTeam() {
return team;
}
/**
* @return The value of the {@code channel} attribute
*/
@JsonProperty
@Override
public SlackChannel getChannel() {
return channel;
}
/**
* @return The value of the {@code user} attribute
*/
@JsonProperty
@Override
public Optional getUser() {
return Optional.ofNullable(user);
}
/**
* @return The value of the {@code username} attribute
*/
@JsonProperty
@Override
public String getUsername() {
return username;
}
/**
* @return The value of the {@code timestamp} attribute
*/
@JsonProperty("ts")
@Override
public String getTimestamp() {
return timestamp;
}
/**
* @return The value of the {@code text} attribute
*/
@JsonProperty
@Override
public String getText() {
return text;
}
/**
* @return The value of the {@code permalink} attribute
*/
@JsonProperty
@Override
public Optional getPermalink() {
return Optional.ofNullable(permalink);
}
/**
* @return The value of the {@code attachments} attribute
*/
@JsonProperty
@Override
public List getAttachments() {
return attachments;
}
/**
* @return The value of the {@code previousPrevious} attribute
*/
@JsonProperty("previous_2")
@Override
public Optional getPreviousPrevious() {
return Optional.ofNullable(previousPrevious);
}
/**
* @return The value of the {@code previous} attribute
*/
@JsonProperty("previous")
@Override
public Optional getPrevious() {
return Optional.ofNullable(previous);
}
/**
* @return The value of the {@code next} attribute
*/
@JsonProperty("next")
@Override
public Optional getNext() {
return Optional.ofNullable(next);
}
/**
* @return The value of the {@code nextNext} attribute
*/
@JsonProperty("next_2")
@Override
public Optional getNextNext() {
return Optional.ofNullable(nextNext);
}
/**
* @return The computed-at-construction value of the {@code slackLink} attribute
*/
@JsonProperty
@Override
public String getSlackLink() {
return slackLink;
}
/**
* Copy the current immutable object by setting a value for the {@link MessageIF#getType() type} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for type
* @return A modified copy of the {@code this} object
*/
public final Message withType(String value) {
String newValue = Objects.requireNonNull(value, "type");
if (this.type.equals(newValue)) return this;
return new Message(
newValue,
this.team,
this.channel,
this.user,
this.username,
this.timestamp,
this.text,
this.permalink,
this.attachments,
this.previousPrevious,
this.previous,
this.next,
this.nextNext);
}
/**
* Copy the current immutable object by setting a value for the {@link MessageIF#getTeam() team} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for team
* @return A modified copy of the {@code this} object
*/
public final Message withTeam(String value) {
String newValue = Objects.requireNonNull(value, "team");
if (this.team.equals(newValue)) return this;
return new Message(
this.type,
newValue,
this.channel,
this.user,
this.username,
this.timestamp,
this.text,
this.permalink,
this.attachments,
this.previousPrevious,
this.previous,
this.next,
this.nextNext);
}
/**
* Copy the current immutable object by setting a value for the {@link MessageIF#getChannel() channel} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for channel
* @return A modified copy of the {@code this} object
*/
public final Message withChannel(SlackChannel value) {
if (this.channel == value) return this;
SlackChannel newValue = Objects.requireNonNull(value, "channel");
return new Message(
this.type,
this.team,
newValue,
this.user,
this.username,
this.timestamp,
this.text,
this.permalink,
this.attachments,
this.previousPrevious,
this.previous,
this.next,
this.nextNext);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link MessageIF#getUser() user} attribute.
* @param value The value for user, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final Message withUser(@Nullable String value) {
@Nullable String newValue = value;
if (Objects.equals(this.user, newValue)) return this;
return new Message(
this.type,
this.team,
this.channel,
newValue,
this.username,
this.timestamp,
this.text,
this.permalink,
this.attachments,
this.previousPrevious,
this.previous,
this.next,
this.nextNext);
}
/**
* Copy the current immutable object by setting an optional value for the {@link MessageIF#getUser() user} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for user
* @return A modified copy of {@code this} object
*/
public final Message withUser(Optional optional) {
@Nullable String value = optional.orElse(null);
if (Objects.equals(this.user, value)) return this;
return new Message(
this.type,
this.team,
this.channel,
value,
this.username,
this.timestamp,
this.text,
this.permalink,
this.attachments,
this.previousPrevious,
this.previous,
this.next,
this.nextNext);
}
/**
* Copy the current immutable object by setting a value for the {@link MessageIF#getUsername() username} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for username
* @return A modified copy of the {@code this} object
*/
public final Message withUsername(String value) {
String newValue = Objects.requireNonNull(value, "username");
if (this.username.equals(newValue)) return this;
return new Message(
this.type,
this.team,
this.channel,
this.user,
newValue,
this.timestamp,
this.text,
this.permalink,
this.attachments,
this.previousPrevious,
this.previous,
this.next,
this.nextNext);
}
/**
* Copy the current immutable object by setting a value for the {@link MessageIF#getTimestamp() timestamp} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for timestamp
* @return A modified copy of the {@code this} object
*/
public final Message withTimestamp(String value) {
String newValue = Objects.requireNonNull(value, "timestamp");
if (this.timestamp.equals(newValue)) return this;
return new Message(
this.type,
this.team,
this.channel,
this.user,
this.username,
newValue,
this.text,
this.permalink,
this.attachments,
this.previousPrevious,
this.previous,
this.next,
this.nextNext);
}
/**
* Copy the current immutable object by setting a value for the {@link MessageIF#getText() text} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for text
* @return A modified copy of the {@code this} object
*/
public final Message withText(String value) {
String newValue = Objects.requireNonNull(value, "text");
if (this.text.equals(newValue)) return this;
return new Message(
this.type,
this.team,
this.channel,
this.user,
this.username,
this.timestamp,
newValue,
this.permalink,
this.attachments,
this.previousPrevious,
this.previous,
this.next,
this.nextNext);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link MessageIF#getPermalink() permalink} attribute.
* @param value The value for permalink, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final Message withPermalink(@Nullable String value) {
@Nullable String newValue = value;
if (Objects.equals(this.permalink, newValue)) return this;
return new Message(
this.type,
this.team,
this.channel,
this.user,
this.username,
this.timestamp,
this.text,
newValue,
this.attachments,
this.previousPrevious,
this.previous,
this.next,
this.nextNext);
}
/**
* Copy the current immutable object by setting an optional value for the {@link MessageIF#getPermalink() permalink} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for permalink
* @return A modified copy of {@code this} object
*/
public final Message withPermalink(Optional optional) {
@Nullable String value = optional.orElse(null);
if (Objects.equals(this.permalink, value)) return this;
return new Message(
this.type,
this.team,
this.channel,
this.user,
this.username,
this.timestamp,
this.text,
value,
this.attachments,
this.previousPrevious,
this.previous,
this.next,
this.nextNext);
}
/**
* Copy the current immutable object with elements that replace the content of {@link MessageIF#getAttachments() attachments}.
* @param elements The elements to set
* @return A modified copy of {@code this} object
*/
public final Message withAttachments(Attachment... elements) {
List newValue = createUnmodifiableList(false, createSafeList(Arrays.asList(elements), true, false));
return new Message(
this.type,
this.team,
this.channel,
this.user,
this.username,
this.timestamp,
this.text,
this.permalink,
newValue,
this.previousPrevious,
this.previous,
this.next,
this.nextNext);
}
/**
* Copy the current immutable object with elements that replace the content of {@link MessageIF#getAttachments() attachments}.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements An iterable of attachments elements to set
* @return A modified copy of {@code this} object
*/
public final Message withAttachments(Iterable extends Attachment> elements) {
if (this.attachments == elements) return this;
List newValue = createUnmodifiableList(false, createSafeList(elements, true, false));
return new Message(
this.type,
this.team,
this.channel,
this.user,
this.username,
this.timestamp,
this.text,
this.permalink,
newValue,
this.previousPrevious,
this.previous,
this.next,
this.nextNext);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link MessageIF#getPreviousPrevious() previousPrevious} attribute.
* @param value The value for previousPrevious, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final Message withPreviousPrevious(@Nullable LiteMessage value) {
@Nullable LiteMessage newValue = value;
if (this.previousPrevious == newValue) return this;
return new Message(
this.type,
this.team,
this.channel,
this.user,
this.username,
this.timestamp,
this.text,
this.permalink,
this.attachments,
newValue,
this.previous,
this.next,
this.nextNext);
}
/**
* Copy the current immutable object by setting an optional value for the {@link MessageIF#getPreviousPrevious() previousPrevious} attribute.
* A shallow reference equality check is used on unboxed optional value to prevent copying of the same value by returning {@code this}.
* @param optional A value for previousPrevious
* @return A modified copy of {@code this} object
*/
@SuppressWarnings("unchecked") // safe covariant cast
public final Message withPreviousPrevious(Optional extends LiteMessage> optional) {
@Nullable LiteMessage value = optional.orElse(null);
if (this.previousPrevious == value) return this;
return new Message(
this.type,
this.team,
this.channel,
this.user,
this.username,
this.timestamp,
this.text,
this.permalink,
this.attachments,
value,
this.previous,
this.next,
this.nextNext);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link MessageIF#getPrevious() previous} attribute.
* @param value The value for previous, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final Message withPrevious(@Nullable LiteMessage value) {
@Nullable LiteMessage newValue = value;
if (this.previous == newValue) return this;
return new Message(
this.type,
this.team,
this.channel,
this.user,
this.username,
this.timestamp,
this.text,
this.permalink,
this.attachments,
this.previousPrevious,
newValue,
this.next,
this.nextNext);
}
/**
* Copy the current immutable object by setting an optional value for the {@link MessageIF#getPrevious() previous} attribute.
* A shallow reference equality check is used on unboxed optional value to prevent copying of the same value by returning {@code this}.
* @param optional A value for previous
* @return A modified copy of {@code this} object
*/
@SuppressWarnings("unchecked") // safe covariant cast
public final Message withPrevious(Optional extends LiteMessage> optional) {
@Nullable LiteMessage value = optional.orElse(null);
if (this.previous == value) return this;
return new Message(
this.type,
this.team,
this.channel,
this.user,
this.username,
this.timestamp,
this.text,
this.permalink,
this.attachments,
this.previousPrevious,
value,
this.next,
this.nextNext);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link MessageIF#getNext() next} attribute.
* @param value The value for next, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final Message withNext(@Nullable LiteMessage value) {
@Nullable LiteMessage newValue = value;
if (this.next == newValue) return this;
return new Message(
this.type,
this.team,
this.channel,
this.user,
this.username,
this.timestamp,
this.text,
this.permalink,
this.attachments,
this.previousPrevious,
this.previous,
newValue,
this.nextNext);
}
/**
* Copy the current immutable object by setting an optional value for the {@link MessageIF#getNext() next} attribute.
* A shallow reference equality check is used on unboxed optional value to prevent copying of the same value by returning {@code this}.
* @param optional A value for next
* @return A modified copy of {@code this} object
*/
@SuppressWarnings("unchecked") // safe covariant cast
public final Message withNext(Optional extends LiteMessage> optional) {
@Nullable LiteMessage value = optional.orElse(null);
if (this.next == value) return this;
return new Message(
this.type,
this.team,
this.channel,
this.user,
this.username,
this.timestamp,
this.text,
this.permalink,
this.attachments,
this.previousPrevious,
this.previous,
value,
this.nextNext);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link MessageIF#getNextNext() nextNext} attribute.
* @param value The value for nextNext, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final Message withNextNext(@Nullable LiteMessage value) {
@Nullable LiteMessage newValue = value;
if (this.nextNext == newValue) return this;
return new Message(
this.type,
this.team,
this.channel,
this.user,
this.username,
this.timestamp,
this.text,
this.permalink,
this.attachments,
this.previousPrevious,
this.previous,
this.next,
newValue);
}
/**
* Copy the current immutable object by setting an optional value for the {@link MessageIF#getNextNext() nextNext} attribute.
* A shallow reference equality check is used on unboxed optional value to prevent copying of the same value by returning {@code this}.
* @param optional A value for nextNext
* @return A modified copy of {@code this} object
*/
@SuppressWarnings("unchecked") // safe covariant cast
public final Message withNextNext(Optional extends LiteMessage> optional) {
@Nullable LiteMessage value = optional.orElse(null);
if (this.nextNext == value) return this;
return new Message(
this.type,
this.team,
this.channel,
this.user,
this.username,
this.timestamp,
this.text,
this.permalink,
this.attachments,
this.previousPrevious,
this.previous,
this.next,
value);
}
/**
* This instance is equal to all instances of {@code Message} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(@Nullable Object another) {
if (this == another) return true;
return another instanceof Message
&& equalTo(0, (Message) another);
}
private boolean equalTo(int synthetic, Message another) {
return type.equals(another.type)
&& team.equals(another.team)
&& channel.equals(another.channel)
&& Objects.equals(user, another.user)
&& username.equals(another.username)
&& timestamp.equals(another.timestamp)
&& text.equals(another.text)
&& Objects.equals(permalink, another.permalink)
&& attachments.equals(another.attachments)
&& Objects.equals(previousPrevious, another.previousPrevious)
&& Objects.equals(previous, another.previous)
&& Objects.equals(next, another.next)
&& Objects.equals(nextNext, another.nextNext)
&& slackLink.equals(another.slackLink);
}
/**
* Computes a hash code from attributes: {@code type}, {@code team}, {@code channel}, {@code user}, {@code username}, {@code timestamp}, {@code text}, {@code permalink}, {@code attachments}, {@code previousPrevious}, {@code previous}, {@code next}, {@code nextNext}, {@code slackLink}.
* @return hashCode value
*/
@Override
public int hashCode() {
int h = 5381;
h += (h << 5) + type.hashCode();
h += (h << 5) + team.hashCode();
h += (h << 5) + channel.hashCode();
h += (h << 5) + Objects.hashCode(user);
h += (h << 5) + username.hashCode();
h += (h << 5) + timestamp.hashCode();
h += (h << 5) + text.hashCode();
h += (h << 5) + Objects.hashCode(permalink);
h += (h << 5) + attachments.hashCode();
h += (h << 5) + Objects.hashCode(previousPrevious);
h += (h << 5) + Objects.hashCode(previous);
h += (h << 5) + Objects.hashCode(next);
h += (h << 5) + Objects.hashCode(nextNext);
h += (h << 5) + slackLink.hashCode();
return h;
}
/**
* Prints the immutable value {@code Message} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
StringBuilder builder = new StringBuilder("Message{");
builder.append("type=").append(type);
builder.append(", ");
builder.append("team=").append(team);
builder.append(", ");
builder.append("channel=").append(channel);
if (user != null) {
builder.append(", ");
builder.append("user=").append(user);
}
builder.append(", ");
builder.append("username=").append(username);
builder.append(", ");
builder.append("timestamp=").append(timestamp);
builder.append(", ");
builder.append("text=").append(text);
if (permalink != null) {
builder.append(", ");
builder.append("permalink=").append(permalink);
}
builder.append(", ");
builder.append("attachments=").append(attachments);
if (previousPrevious != null) {
builder.append(", ");
builder.append("previousPrevious=").append(previousPrevious);
}
if (previous != null) {
builder.append(", ");
builder.append("previous=").append(previous);
}
if (next != null) {
builder.append(", ");
builder.append("next=").append(next);
}
if (nextNext != null) {
builder.append(", ");
builder.append("nextNext=").append(nextNext);
}
builder.append(", ");
builder.append("slackLink=").append(slackLink);
return builder.append("}").toString();
}
/**
* Utility type used to correctly read immutable object from JSON representation.
* @deprecated Do not use this type directly, it exists only for the Jackson-binding infrastructure
*/
@Generated(from = "MessageIF", generator = "Immutables")
@Deprecated
@JsonAutoDetect(fieldVisibility = JsonAutoDetect.Visibility.NONE)
static final class Json implements MessageIF {
@Nullable String type;
@Nullable String team;
@Nullable SlackChannel channel;
@Nullable Optional user = Optional.empty();
@Nullable String username;
@Nullable String timestamp;
@Nullable String text;
@Nullable Optional permalink = Optional.empty();
@Nullable List attachments = Collections.emptyList();
@Nullable Optional previousPrevious = Optional.empty();
@Nullable Optional previous = Optional.empty();
@Nullable Optional next = Optional.empty();
@Nullable Optional nextNext = Optional.empty();
@JsonProperty
public void setType(String type) {
this.type = type;
}
@JsonProperty
public void setTeam(String team) {
this.team = team;
}
@JsonProperty
public void setChannel(SlackChannel channel) {
this.channel = channel;
}
@JsonProperty
public void setUser(Optional user) {
this.user = user;
}
@JsonProperty
public void setUsername(String username) {
this.username = username;
}
@JsonProperty("ts")
public void setTimestamp(String timestamp) {
this.timestamp = timestamp;
}
@JsonProperty
public void setText(String text) {
this.text = text;
}
@JsonProperty
public void setPermalink(Optional permalink) {
this.permalink = permalink;
}
@JsonProperty
public void setAttachments(List attachments) {
this.attachments = attachments;
}
@JsonProperty("previous_2")
public void setPreviousPrevious(Optional previousPrevious) {
this.previousPrevious = previousPrevious;
}
@JsonProperty("previous")
public void setPrevious(Optional previous) {
this.previous = previous;
}
@JsonProperty("next")
public void setNext(Optional next) {
this.next = next;
}
@JsonProperty("next_2")
public void setNextNext(Optional nextNext) {
this.nextNext = nextNext;
}
@Override
public String getType() { throw new UnsupportedOperationException(); }
@Override
public String getTeam() { throw new UnsupportedOperationException(); }
@Override
public SlackChannel getChannel() { throw new UnsupportedOperationException(); }
@Override
public Optional getUser() { throw new UnsupportedOperationException(); }
@Override
public String getUsername() { throw new UnsupportedOperationException(); }
@Override
public String getTimestamp() { throw new UnsupportedOperationException(); }
@Override
public String getText() { throw new UnsupportedOperationException(); }
@Override
public Optional getPermalink() { throw new UnsupportedOperationException(); }
@Override
public List getAttachments() { throw new UnsupportedOperationException(); }
@Override
public Optional getPreviousPrevious() { throw new UnsupportedOperationException(); }
@Override
public Optional getPrevious() { throw new UnsupportedOperationException(); }
@Override
public Optional getNext() { throw new UnsupportedOperationException(); }
@Override
public Optional getNextNext() { throw new UnsupportedOperationException(); }
@JsonIgnore
@Override
public String getSlackLink() { throw new UnsupportedOperationException(); }
}
/**
* @param json A JSON-bindable data structure
* @return An immutable value type
* @deprecated Do not use this method directly, it exists only for the Jackson-binding infrastructure
*/
@Deprecated
@JsonCreator(mode = JsonCreator.Mode.DELEGATING)
static Message fromJson(Json json) {
Message.Builder builder = Message.builder();
if (json.type != null) {
builder.setType(json.type);
}
if (json.team != null) {
builder.setTeam(json.team);
}
if (json.channel != null) {
builder.setChannel(json.channel);
}
if (json.user != null) {
builder.setUser(json.user);
}
if (json.username != null) {
builder.setUsername(json.username);
}
if (json.timestamp != null) {
builder.setTimestamp(json.timestamp);
}
if (json.text != null) {
builder.setText(json.text);
}
if (json.permalink != null) {
builder.setPermalink(json.permalink);
}
if (json.attachments != null) {
builder.addAllAttachments(json.attachments);
}
if (json.previousPrevious != null) {
builder.setPreviousPrevious(json.previousPrevious);
}
if (json.previous != null) {
builder.setPrevious(json.previous);
}
if (json.next != null) {
builder.setNext(json.next);
}
if (json.nextNext != null) {
builder.setNextNext(json.nextNext);
}
return builder.build();
}
/**
* Creates an immutable copy of a {@link MessageIF} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable Message instance
*/
public static Message copyOf(MessageIF instance) {
if (instance instanceof Message) {
return (Message) instance;
}
return Message.builder()
.from(instance)
.build();
}
/**
* Creates a builder for {@link Message Message}.
*
* Message.builder()
* .setType(String) // required {@link MessageIF#getType() type}
* .setTeam(String) // required {@link MessageIF#getTeam() team}
* .setChannel(com.hubspot.slack.client.models.SlackChannel) // required {@link MessageIF#getChannel() channel}
* .setUser(String) // optional {@link MessageIF#getUser() user}
* .setUsername(String) // required {@link MessageIF#getUsername() username}
* .setTimestamp(String) // required {@link MessageIF#getTimestamp() timestamp}
* .setText(String) // required {@link MessageIF#getText() text}
* .setPermalink(String) // optional {@link MessageIF#getPermalink() permalink}
* .addAttachments|addAllAttachments(Attachment) // {@link MessageIF#getAttachments() attachments} elements
* .setPreviousPrevious(LiteMessage) // optional {@link MessageIF#getPreviousPrevious() previousPrevious}
* .setPrevious(LiteMessage) // optional {@link MessageIF#getPrevious() previous}
* .setNext(LiteMessage) // optional {@link MessageIF#getNext() next}
* .setNextNext(LiteMessage) // optional {@link MessageIF#getNextNext() nextNext}
* .build();
*
* @return A new Message builder
*/
public static Message.Builder builder() {
return new Message.Builder();
}
/**
* Builds instances of type {@link Message Message}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
@Generated(from = "MessageIF", generator = "Immutables")
@NotThreadSafe
public static final class Builder {
private static final long INIT_BIT_TYPE = 0x1L;
private static final long INIT_BIT_TEAM = 0x2L;
private static final long INIT_BIT_CHANNEL = 0x4L;
private static final long INIT_BIT_USERNAME = 0x8L;
private static final long INIT_BIT_TIMESTAMP = 0x10L;
private static final long INIT_BIT_TEXT = 0x20L;
private long initBits = 0x3fL;
private @Nullable String type;
private @Nullable String team;
private @Nullable SlackChannel channel;
private @Nullable String user;
private @Nullable String username;
private @Nullable String timestamp;
private @Nullable String text;
private @Nullable String permalink;
private List attachments = new ArrayList();
private @Nullable LiteMessage previousPrevious;
private @Nullable LiteMessage previous;
private @Nullable LiteMessage next;
private @Nullable LiteMessage nextNext;
private Builder() {
}
/**
* Fill a builder with attribute values from the provided {@code MessageIF} instance.
* Regular attribute values will be replaced with those from the given instance.
* Absent optional values will not replace present values.
* Collection elements and entries will be added, not replaced.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(MessageIF instance) {
Objects.requireNonNull(instance, "instance");
this.setType(instance.getType());
this.setTeam(instance.getTeam());
this.setChannel(instance.getChannel());
Optional userOptional = instance.getUser();
if (userOptional.isPresent()) {
setUser(userOptional);
}
this.setUsername(instance.getUsername());
this.setTimestamp(instance.getTimestamp());
this.setText(instance.getText());
Optional permalinkOptional = instance.getPermalink();
if (permalinkOptional.isPresent()) {
setPermalink(permalinkOptional);
}
addAllAttachments(instance.getAttachments());
Optional previousPreviousOptional = instance.getPreviousPrevious();
if (previousPreviousOptional.isPresent()) {
setPreviousPrevious(previousPreviousOptional);
}
Optional previousOptional = instance.getPrevious();
if (previousOptional.isPresent()) {
setPrevious(previousOptional);
}
Optional nextOptional = instance.getNext();
if (nextOptional.isPresent()) {
setNext(nextOptional);
}
Optional nextNextOptional = instance.getNextNext();
if (nextNextOptional.isPresent()) {
setNextNext(nextNextOptional);
}
return this;
}
/**
* Initializes the value for the {@link MessageIF#getType() type} attribute.
* @param type The value for type
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setType(String type) {
this.type = Objects.requireNonNull(type, "type");
initBits &= ~INIT_BIT_TYPE;
return this;
}
/**
* Initializes the value for the {@link MessageIF#getTeam() team} attribute.
* @param team The value for team
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setTeam(String team) {
this.team = Objects.requireNonNull(team, "team");
initBits &= ~INIT_BIT_TEAM;
return this;
}
/**
* Initializes the value for the {@link MessageIF#getChannel() channel} attribute.
* @param channel The value for channel
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setChannel(SlackChannel channel) {
this.channel = Objects.requireNonNull(channel, "channel");
initBits &= ~INIT_BIT_CHANNEL;
return this;
}
/**
* Initializes the optional value {@link MessageIF#getUser() user} to user.
* @param user The value for user, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
public final Builder setUser(@Nullable String user) {
this.user = user;
return this;
}
/**
* Initializes the optional value {@link MessageIF#getUser() user} to user.
* @param user The value for user
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setUser(Optional user) {
this.user = user.orElse(null);
return this;
}
/**
* Initializes the value for the {@link MessageIF#getUsername() username} attribute.
* @param username The value for username
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setUsername(String username) {
this.username = Objects.requireNonNull(username, "username");
initBits &= ~INIT_BIT_USERNAME;
return this;
}
/**
* Initializes the value for the {@link MessageIF#getTimestamp() timestamp} attribute.
* @param timestamp The value for timestamp
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setTimestamp(String timestamp) {
this.timestamp = Objects.requireNonNull(timestamp, "timestamp");
initBits &= ~INIT_BIT_TIMESTAMP;
return this;
}
/**
* Initializes the value for the {@link MessageIF#getText() text} attribute.
* @param text The value for text
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setText(String text) {
this.text = Objects.requireNonNull(text, "text");
initBits &= ~INIT_BIT_TEXT;
return this;
}
/**
* Initializes the optional value {@link MessageIF#getPermalink() permalink} to permalink.
* @param permalink The value for permalink, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
public final Builder setPermalink(@Nullable String permalink) {
this.permalink = permalink;
return this;
}
/**
* Initializes the optional value {@link MessageIF#getPermalink() permalink} to permalink.
* @param permalink The value for permalink
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setPermalink(Optional permalink) {
this.permalink = permalink.orElse(null);
return this;
}
/**
* Adds one element to {@link MessageIF#getAttachments() attachments} list.
* @param element A attachments element
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addAttachments(Attachment element) {
this.attachments.add(Objects.requireNonNull(element, "attachments element"));
return this;
}
/**
* Adds elements to {@link MessageIF#getAttachments() attachments} list.
* @param elements An array of attachments elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addAttachments(Attachment... elements) {
for (Attachment element : elements) {
this.attachments.add(Objects.requireNonNull(element, "attachments element"));
}
return this;
}
/**
* Sets or replaces all elements for {@link MessageIF#getAttachments() attachments} list.
* @param elements An iterable of attachments elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setAttachments(Iterable extends Attachment> elements) {
this.attachments.clear();
return addAllAttachments(elements);
}
/**
* Adds elements to {@link MessageIF#getAttachments() attachments} list.
* @param elements An iterable of attachments elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addAllAttachments(Iterable extends Attachment> elements) {
for (Attachment element : elements) {
this.attachments.add(Objects.requireNonNull(element, "attachments element"));
}
return this;
}
/**
* Initializes the optional value {@link MessageIF#getPreviousPrevious() previousPrevious} to previousPrevious.
* @param previousPrevious The value for previousPrevious, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
public final Builder setPreviousPrevious(@Nullable LiteMessage previousPrevious) {
this.previousPrevious = previousPrevious;
return this;
}
/**
* Initializes the optional value {@link MessageIF#getPreviousPrevious() previousPrevious} to previousPrevious.
* @param previousPrevious The value for previousPrevious
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setPreviousPrevious(Optional extends LiteMessage> previousPrevious) {
this.previousPrevious = previousPrevious.orElse(null);
return this;
}
/**
* Initializes the optional value {@link MessageIF#getPrevious() previous} to previous.
* @param previous The value for previous, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
public final Builder setPrevious(@Nullable LiteMessage previous) {
this.previous = previous;
return this;
}
/**
* Initializes the optional value {@link MessageIF#getPrevious() previous} to previous.
* @param previous The value for previous
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setPrevious(Optional extends LiteMessage> previous) {
this.previous = previous.orElse(null);
return this;
}
/**
* Initializes the optional value {@link MessageIF#getNext() next} to next.
* @param next The value for next, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
public final Builder setNext(@Nullable LiteMessage next) {
this.next = next;
return this;
}
/**
* Initializes the optional value {@link MessageIF#getNext() next} to next.
* @param next The value for next
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setNext(Optional extends LiteMessage> next) {
this.next = next.orElse(null);
return this;
}
/**
* Initializes the optional value {@link MessageIF#getNextNext() nextNext} to nextNext.
* @param nextNext The value for nextNext, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
public final Builder setNextNext(@Nullable LiteMessage nextNext) {
this.nextNext = nextNext;
return this;
}
/**
* Initializes the optional value {@link MessageIF#getNextNext() nextNext} to nextNext.
* @param nextNext The value for nextNext
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setNextNext(Optional extends LiteMessage> nextNext) {
this.nextNext = nextNext.orElse(null);
return this;
}
/**
* Builds a new {@link Message Message}.
* @return An immutable instance of Message
* @throws com.hubspot.immutables.validation.InvalidImmutableStateException if any required attributes are missing
*/
public Message build() {
checkRequiredAttributes();
return new Message(
type,
team,
channel,
user,
username,
timestamp,
text,
permalink,
createUnmodifiableList(true, attachments),
previousPrevious,
previous,
next,
nextNext);
}
private boolean typeIsSet() {
return (initBits & INIT_BIT_TYPE) == 0;
}
private boolean teamIsSet() {
return (initBits & INIT_BIT_TEAM) == 0;
}
private boolean channelIsSet() {
return (initBits & INIT_BIT_CHANNEL) == 0;
}
private boolean usernameIsSet() {
return (initBits & INIT_BIT_USERNAME) == 0;
}
private boolean timestampIsSet() {
return (initBits & INIT_BIT_TIMESTAMP) == 0;
}
private boolean textIsSet() {
return (initBits & INIT_BIT_TEXT) == 0;
}
private void checkRequiredAttributes() {
if (initBits != 0) {
throw new InvalidImmutableStateException(formatRequiredAttributesMessage());
}
}
private String formatRequiredAttributesMessage() {
List attributes = new ArrayList<>();
if (!typeIsSet()) attributes.add("type");
if (!teamIsSet()) attributes.add("team");
if (!channelIsSet()) attributes.add("channel");
if (!usernameIsSet()) attributes.add("username");
if (!timestampIsSet()) attributes.add("timestamp");
if (!textIsSet()) attributes.add("text");
return "Cannot build Message, some of required attributes are not set " + attributes;
}
}
private static List createSafeList(Iterable extends T> iterable, boolean checkNulls, boolean skipNulls) {
ArrayList list;
if (iterable instanceof Collection>) {
int size = ((Collection>) iterable).size();
if (size == 0) return Collections.emptyList();
list = new ArrayList<>(size);
} else {
list = new ArrayList<>();
}
for (T element : iterable) {
if (skipNulls && element == null) continue;
if (checkNulls) Objects.requireNonNull(element, "element");
list.add(element);
}
return list;
}
private static List createUnmodifiableList(boolean clone, List list) {
switch(list.size()) {
case 0: return Collections.emptyList();
case 1: return Collections.singletonList(list.get(0));
default:
if (clone) {
return Collections.unmodifiableList(new ArrayList<>(list));
} else {
if (list instanceof ArrayList>) {
((ArrayList>) list).trimToSize();
}
return Collections.unmodifiableList(list);
}
}
}
}