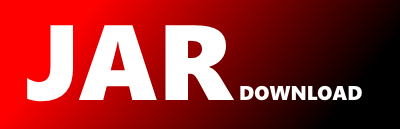
com.hubspot.slack.client.models.SlackChannel Maven / Gradle / Ivy
package com.hubspot.slack.client.models;
import com.fasterxml.jackson.annotation.JsonAutoDetect;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.hubspot.immutables.validation.InvalidImmutableStateException;
import edu.umd.cs.findbugs.annotations.SuppressFBWarnings;
import java.util.ArrayList;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
import javax.annotation.ParametersAreNonnullByDefault;
import javax.annotation.concurrent.Immutable;
import javax.annotation.concurrent.NotThreadSafe;
import org.immutables.value.Generated;
/**
* Immutable implementation of {@link SlackChannelIF}.
*
* Use the builder to create immutable instances:
* {@code SlackChannel.builder()}.
*/
@Generated(from = "SlackChannelIF", generator = "Immutables")
@SuppressWarnings({"all"})
@SuppressFBWarnings
@ParametersAreNonnullByDefault
@javax.annotation.processing.Generated("org.immutables.processor.ProxyProcessor")
@Immutable
public final class SlackChannel implements SlackChannelIF {
private final String id;
private final String name;
private final @Nullable Boolean isArchived;
private final @Nullable Boolean isGeneral;
private final @Nullable Boolean isPrivate;
private final @Nullable Boolean isMember;
private final @Nullable Boolean isShared;
private transient final ChannelType channelType;
private SlackChannel(
String id,
String name,
@Nullable Boolean isArchived,
@Nullable Boolean isGeneral,
@Nullable Boolean isPrivate,
@Nullable Boolean isMember,
@Nullable Boolean isShared) {
this.id = id;
this.name = name;
this.isArchived = isArchived;
this.isGeneral = isGeneral;
this.isPrivate = isPrivate;
this.isMember = isMember;
this.isShared = isShared;
this.channelType = Objects.requireNonNull(SlackChannelIF.super.getChannelType(), "channelType");
}
/**
* @return The value of the {@code id} attribute
*/
@JsonProperty
@Override
public String getId() {
return id;
}
/**
* @return The value of the {@code name} attribute
*/
@JsonProperty
@Override
public String getName() {
return name;
}
/**
* @return The value of the {@code isArchived} attribute
*/
@JsonProperty
@Override
public Optional getIsArchived() {
return Optional.ofNullable(isArchived);
}
/**
* @return The value of the {@code isGeneral} attribute
*/
@JsonProperty
@Override
public Optional getIsGeneral() {
return Optional.ofNullable(isGeneral);
}
/**
* @return The value of the {@code isPrivate} attribute
*/
@JsonProperty
@Override
public Optional getIsPrivate() {
return Optional.ofNullable(isPrivate);
}
/**
* @return The value of the {@code isMember} attribute
*/
@JsonProperty
@Override
public Optional getIsMember() {
return Optional.ofNullable(isMember);
}
/**
* @return The value of the {@code isShared} attribute
*/
@JsonProperty
@Override
public Optional getIsShared() {
return Optional.ofNullable(isShared);
}
/**
* @return The computed-at-construction value of the {@code channelType} attribute
*/
@JsonProperty
@JsonIgnore
@Override
public ChannelType getChannelType() {
return channelType;
}
/**
* Copy the current immutable object by setting a value for the {@link SlackChannelIF#getId() id} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for id
* @return A modified copy of the {@code this} object
*/
public final SlackChannel withId(String value) {
String newValue = Objects.requireNonNull(value, "id");
if (this.id.equals(newValue)) return this;
return new SlackChannel(
newValue,
this.name,
this.isArchived,
this.isGeneral,
this.isPrivate,
this.isMember,
this.isShared);
}
/**
* Copy the current immutable object by setting a value for the {@link SlackChannelIF#getName() name} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for name
* @return A modified copy of the {@code this} object
*/
public final SlackChannel withName(String value) {
String newValue = Objects.requireNonNull(value, "name");
if (this.name.equals(newValue)) return this;
return new SlackChannel(
this.id,
newValue,
this.isArchived,
this.isGeneral,
this.isPrivate,
this.isMember,
this.isShared);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link SlackChannelIF#getIsArchived() isArchived} attribute.
* @param value The value for isArchived, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final SlackChannel withIsArchived(@Nullable Boolean value) {
@Nullable Boolean newValue = value;
if (Objects.equals(this.isArchived, newValue)) return this;
return new SlackChannel(this.id, this.name, newValue, this.isGeneral, this.isPrivate, this.isMember, this.isShared);
}
/**
* Copy the current immutable object by setting an optional value for the {@link SlackChannelIF#getIsArchived() isArchived} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for isArchived
* @return A modified copy of {@code this} object
*/
public final SlackChannel withIsArchived(Optional optional) {
@Nullable Boolean value = optional.orElse(null);
if (Objects.equals(this.isArchived, value)) return this;
return new SlackChannel(this.id, this.name, value, this.isGeneral, this.isPrivate, this.isMember, this.isShared);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link SlackChannelIF#getIsGeneral() isGeneral} attribute.
* @param value The value for isGeneral, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final SlackChannel withIsGeneral(@Nullable Boolean value) {
@Nullable Boolean newValue = value;
if (Objects.equals(this.isGeneral, newValue)) return this;
return new SlackChannel(this.id, this.name, this.isArchived, newValue, this.isPrivate, this.isMember, this.isShared);
}
/**
* Copy the current immutable object by setting an optional value for the {@link SlackChannelIF#getIsGeneral() isGeneral} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for isGeneral
* @return A modified copy of {@code this} object
*/
public final SlackChannel withIsGeneral(Optional optional) {
@Nullable Boolean value = optional.orElse(null);
if (Objects.equals(this.isGeneral, value)) return this;
return new SlackChannel(this.id, this.name, this.isArchived, value, this.isPrivate, this.isMember, this.isShared);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link SlackChannelIF#getIsPrivate() isPrivate} attribute.
* @param value The value for isPrivate, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final SlackChannel withIsPrivate(@Nullable Boolean value) {
@Nullable Boolean newValue = value;
if (Objects.equals(this.isPrivate, newValue)) return this;
return new SlackChannel(this.id, this.name, this.isArchived, this.isGeneral, newValue, this.isMember, this.isShared);
}
/**
* Copy the current immutable object by setting an optional value for the {@link SlackChannelIF#getIsPrivate() isPrivate} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for isPrivate
* @return A modified copy of {@code this} object
*/
public final SlackChannel withIsPrivate(Optional optional) {
@Nullable Boolean value = optional.orElse(null);
if (Objects.equals(this.isPrivate, value)) return this;
return new SlackChannel(this.id, this.name, this.isArchived, this.isGeneral, value, this.isMember, this.isShared);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link SlackChannelIF#getIsMember() isMember} attribute.
* @param value The value for isMember, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final SlackChannel withIsMember(@Nullable Boolean value) {
@Nullable Boolean newValue = value;
if (Objects.equals(this.isMember, newValue)) return this;
return new SlackChannel(this.id, this.name, this.isArchived, this.isGeneral, this.isPrivate, newValue, this.isShared);
}
/**
* Copy the current immutable object by setting an optional value for the {@link SlackChannelIF#getIsMember() isMember} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for isMember
* @return A modified copy of {@code this} object
*/
public final SlackChannel withIsMember(Optional optional) {
@Nullable Boolean value = optional.orElse(null);
if (Objects.equals(this.isMember, value)) return this;
return new SlackChannel(this.id, this.name, this.isArchived, this.isGeneral, this.isPrivate, value, this.isShared);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link SlackChannelIF#getIsShared() isShared} attribute.
* @param value The value for isShared, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final SlackChannel withIsShared(@Nullable Boolean value) {
@Nullable Boolean newValue = value;
if (Objects.equals(this.isShared, newValue)) return this;
return new SlackChannel(this.id, this.name, this.isArchived, this.isGeneral, this.isPrivate, this.isMember, newValue);
}
/**
* Copy the current immutable object by setting an optional value for the {@link SlackChannelIF#getIsShared() isShared} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for isShared
* @return A modified copy of {@code this} object
*/
public final SlackChannel withIsShared(Optional optional) {
@Nullable Boolean value = optional.orElse(null);
if (Objects.equals(this.isShared, value)) return this;
return new SlackChannel(this.id, this.name, this.isArchived, this.isGeneral, this.isPrivate, this.isMember, value);
}
/**
* This instance is equal to all instances of {@code SlackChannel} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(@Nullable Object another) {
if (this == another) return true;
return another instanceof SlackChannel
&& equalTo(0, (SlackChannel) another);
}
private boolean equalTo(int synthetic, SlackChannel another) {
return id.equals(another.id)
&& name.equals(another.name)
&& Objects.equals(isArchived, another.isArchived)
&& Objects.equals(isGeneral, another.isGeneral)
&& Objects.equals(isPrivate, another.isPrivate)
&& Objects.equals(isMember, another.isMember)
&& Objects.equals(isShared, another.isShared)
&& channelType.equals(another.channelType);
}
/**
* Computes a hash code from attributes: {@code id}, {@code name}, {@code isArchived}, {@code isGeneral}, {@code isPrivate}, {@code isMember}, {@code isShared}, {@code channelType}.
* @return hashCode value
*/
@Override
public int hashCode() {
int h = 5381;
h += (h << 5) + id.hashCode();
h += (h << 5) + name.hashCode();
h += (h << 5) + Objects.hashCode(isArchived);
h += (h << 5) + Objects.hashCode(isGeneral);
h += (h << 5) + Objects.hashCode(isPrivate);
h += (h << 5) + Objects.hashCode(isMember);
h += (h << 5) + Objects.hashCode(isShared);
h += (h << 5) + channelType.hashCode();
return h;
}
/**
* Prints the immutable value {@code SlackChannel} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
StringBuilder builder = new StringBuilder("SlackChannel{");
builder.append("id=").append(id);
builder.append(", ");
builder.append("name=").append(name);
if (isArchived != null) {
builder.append(", ");
builder.append("isArchived=").append(isArchived);
}
if (isGeneral != null) {
builder.append(", ");
builder.append("isGeneral=").append(isGeneral);
}
if (isPrivate != null) {
builder.append(", ");
builder.append("isPrivate=").append(isPrivate);
}
if (isMember != null) {
builder.append(", ");
builder.append("isMember=").append(isMember);
}
if (isShared != null) {
builder.append(", ");
builder.append("isShared=").append(isShared);
}
builder.append(", ");
builder.append("channelType=").append(channelType);
return builder.append("}").toString();
}
/**
* Utility type used to correctly read immutable object from JSON representation.
* @deprecated Do not use this type directly, it exists only for the Jackson-binding infrastructure
*/
@Generated(from = "SlackChannelIF", generator = "Immutables")
@Deprecated
@JsonAutoDetect(fieldVisibility = JsonAutoDetect.Visibility.NONE)
static final class Json implements SlackChannelIF {
@Nullable String id;
@Nullable String name;
@Nullable Optional isArchived = Optional.empty();
@Nullable Optional isGeneral = Optional.empty();
@Nullable Optional isPrivate = Optional.empty();
@Nullable Optional isMember = Optional.empty();
@Nullable Optional isShared = Optional.empty();
@JsonProperty
public void setId(String id) {
this.id = id;
}
@JsonProperty
public void setName(String name) {
this.name = name;
}
@JsonProperty
public void setIsArchived(Optional isArchived) {
this.isArchived = isArchived;
}
@JsonProperty
public void setIsGeneral(Optional isGeneral) {
this.isGeneral = isGeneral;
}
@JsonProperty
public void setIsPrivate(Optional isPrivate) {
this.isPrivate = isPrivate;
}
@JsonProperty
public void setIsMember(Optional isMember) {
this.isMember = isMember;
}
@JsonProperty
public void setIsShared(Optional isShared) {
this.isShared = isShared;
}
@Override
public String getId() { throw new UnsupportedOperationException(); }
@Override
public String getName() { throw new UnsupportedOperationException(); }
@Override
public Optional getIsArchived() { throw new UnsupportedOperationException(); }
@Override
public Optional getIsGeneral() { throw new UnsupportedOperationException(); }
@Override
public Optional getIsPrivate() { throw new UnsupportedOperationException(); }
@Override
public Optional getIsMember() { throw new UnsupportedOperationException(); }
@Override
public Optional getIsShared() { throw new UnsupportedOperationException(); }
@JsonIgnore
@Override
public ChannelType getChannelType() { throw new UnsupportedOperationException(); }
}
/**
* @param json A JSON-bindable data structure
* @return An immutable value type
* @deprecated Do not use this method directly, it exists only for the Jackson-binding infrastructure
*/
@Deprecated
@JsonCreator(mode = JsonCreator.Mode.DELEGATING)
static SlackChannel fromJson(Json json) {
SlackChannel.Builder builder = SlackChannel.builder();
if (json.id != null) {
builder.setId(json.id);
}
if (json.name != null) {
builder.setName(json.name);
}
if (json.isArchived != null) {
builder.setIsArchived(json.isArchived);
}
if (json.isGeneral != null) {
builder.setIsGeneral(json.isGeneral);
}
if (json.isPrivate != null) {
builder.setIsPrivate(json.isPrivate);
}
if (json.isMember != null) {
builder.setIsMember(json.isMember);
}
if (json.isShared != null) {
builder.setIsShared(json.isShared);
}
return builder.build();
}
/**
* Creates an immutable copy of a {@link SlackChannelIF} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable SlackChannel instance
*/
public static SlackChannel copyOf(SlackChannelIF instance) {
if (instance instanceof SlackChannel) {
return (SlackChannel) instance;
}
return SlackChannel.builder()
.from(instance)
.build();
}
/**
* Creates a builder for {@link SlackChannel SlackChannel}.
*
* SlackChannel.builder()
* .setId(String) // required {@link SlackChannelIF#getId() id}
* .setName(String) // required {@link SlackChannelIF#getName() name}
* .setIsArchived(Boolean) // optional {@link SlackChannelIF#getIsArchived() isArchived}
* .setIsGeneral(Boolean) // optional {@link SlackChannelIF#getIsGeneral() isGeneral}
* .setIsPrivate(Boolean) // optional {@link SlackChannelIF#getIsPrivate() isPrivate}
* .setIsMember(Boolean) // optional {@link SlackChannelIF#getIsMember() isMember}
* .setIsShared(Boolean) // optional {@link SlackChannelIF#getIsShared() isShared}
* .build();
*
* @return A new SlackChannel builder
*/
public static SlackChannel.Builder builder() {
return new SlackChannel.Builder();
}
/**
* Builds instances of type {@link SlackChannel SlackChannel}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
@Generated(from = "SlackChannelIF", generator = "Immutables")
@NotThreadSafe
public static final class Builder {
private static final long INIT_BIT_ID = 0x1L;
private static final long INIT_BIT_NAME = 0x2L;
private long initBits = 0x3L;
private @Nullable String id;
private @Nullable String name;
private @Nullable Boolean isArchived;
private @Nullable Boolean isGeneral;
private @Nullable Boolean isPrivate;
private @Nullable Boolean isMember;
private @Nullable Boolean isShared;
private Builder() {
}
/**
* Fill a builder with attribute values from the provided {@code SlackChannelIF} instance.
* Regular attribute values will be replaced with those from the given instance.
* Absent optional values will not replace present values.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(SlackChannelIF instance) {
Objects.requireNonNull(instance, "instance");
this.setId(instance.getId());
this.setName(instance.getName());
Optional isArchivedOptional = instance.getIsArchived();
if (isArchivedOptional.isPresent()) {
setIsArchived(isArchivedOptional);
}
Optional isGeneralOptional = instance.getIsGeneral();
if (isGeneralOptional.isPresent()) {
setIsGeneral(isGeneralOptional);
}
Optional isPrivateOptional = instance.getIsPrivate();
if (isPrivateOptional.isPresent()) {
setIsPrivate(isPrivateOptional);
}
Optional isMemberOptional = instance.getIsMember();
if (isMemberOptional.isPresent()) {
setIsMember(isMemberOptional);
}
Optional isSharedOptional = instance.getIsShared();
if (isSharedOptional.isPresent()) {
setIsShared(isSharedOptional);
}
return this;
}
/**
* Initializes the value for the {@link SlackChannelIF#getId() id} attribute.
* @param id The value for id
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setId(String id) {
this.id = Objects.requireNonNull(id, "id");
initBits &= ~INIT_BIT_ID;
return this;
}
/**
* Initializes the value for the {@link SlackChannelIF#getName() name} attribute.
* @param name The value for name
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setName(String name) {
this.name = Objects.requireNonNull(name, "name");
initBits &= ~INIT_BIT_NAME;
return this;
}
/**
* Initializes the optional value {@link SlackChannelIF#getIsArchived() isArchived} to isArchived.
* @param isArchived The value for isArchived, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
public final Builder setIsArchived(@Nullable Boolean isArchived) {
this.isArchived = isArchived;
return this;
}
/**
* Initializes the optional value {@link SlackChannelIF#getIsArchived() isArchived} to isArchived.
* @param isArchived The value for isArchived
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setIsArchived(Optional isArchived) {
this.isArchived = isArchived.orElse(null);
return this;
}
/**
* Initializes the optional value {@link SlackChannelIF#getIsGeneral() isGeneral} to isGeneral.
* @param isGeneral The value for isGeneral, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
public final Builder setIsGeneral(@Nullable Boolean isGeneral) {
this.isGeneral = isGeneral;
return this;
}
/**
* Initializes the optional value {@link SlackChannelIF#getIsGeneral() isGeneral} to isGeneral.
* @param isGeneral The value for isGeneral
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setIsGeneral(Optional isGeneral) {
this.isGeneral = isGeneral.orElse(null);
return this;
}
/**
* Initializes the optional value {@link SlackChannelIF#getIsPrivate() isPrivate} to isPrivate.
* @param isPrivate The value for isPrivate, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
public final Builder setIsPrivate(@Nullable Boolean isPrivate) {
this.isPrivate = isPrivate;
return this;
}
/**
* Initializes the optional value {@link SlackChannelIF#getIsPrivate() isPrivate} to isPrivate.
* @param isPrivate The value for isPrivate
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setIsPrivate(Optional isPrivate) {
this.isPrivate = isPrivate.orElse(null);
return this;
}
/**
* Initializes the optional value {@link SlackChannelIF#getIsMember() isMember} to isMember.
* @param isMember The value for isMember, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
public final Builder setIsMember(@Nullable Boolean isMember) {
this.isMember = isMember;
return this;
}
/**
* Initializes the optional value {@link SlackChannelIF#getIsMember() isMember} to isMember.
* @param isMember The value for isMember
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setIsMember(Optional isMember) {
this.isMember = isMember.orElse(null);
return this;
}
/**
* Initializes the optional value {@link SlackChannelIF#getIsShared() isShared} to isShared.
* @param isShared The value for isShared, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
public final Builder setIsShared(@Nullable Boolean isShared) {
this.isShared = isShared;
return this;
}
/**
* Initializes the optional value {@link SlackChannelIF#getIsShared() isShared} to isShared.
* @param isShared The value for isShared
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setIsShared(Optional isShared) {
this.isShared = isShared.orElse(null);
return this;
}
/**
* Builds a new {@link SlackChannel SlackChannel}.
* @return An immutable instance of SlackChannel
* @throws com.hubspot.immutables.validation.InvalidImmutableStateException if any required attributes are missing
*/
public SlackChannel build() {
checkRequiredAttributes();
return new SlackChannel(id, name, isArchived, isGeneral, isPrivate, isMember, isShared);
}
private boolean idIsSet() {
return (initBits & INIT_BIT_ID) == 0;
}
private boolean nameIsSet() {
return (initBits & INIT_BIT_NAME) == 0;
}
private void checkRequiredAttributes() {
if (initBits != 0) {
throw new InvalidImmutableStateException(formatRequiredAttributesMessage());
}
}
private String formatRequiredAttributesMessage() {
List attributes = new ArrayList<>();
if (!idIsSet()) attributes.add("id");
if (!nameIsSet()) attributes.add("name");
return "Cannot build SlackChannel, some of required attributes are not set " + attributes;
}
}
}