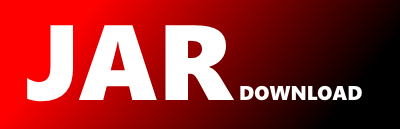
com.hubspot.slack.client.models.auth.OAuthCredentials Maven / Gradle / Ivy
package com.hubspot.slack.client.models.auth;
import com.fasterxml.jackson.annotation.JsonAutoDetect;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.hubspot.immutables.validation.InvalidImmutableStateException;
import edu.umd.cs.findbugs.annotations.SuppressFBWarnings;
import java.util.ArrayList;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
import javax.annotation.ParametersAreNonnullByDefault;
import javax.annotation.concurrent.Immutable;
import javax.annotation.concurrent.NotThreadSafe;
import org.immutables.value.Generated;
/**
* Immutable implementation of {@link OAuthCredentialsIF}.
*
* Use the builder to create immutable instances:
* {@code OAuthCredentials.builder()}.
*/
@Generated(from = "OAuthCredentialsIF", generator = "Immutables")
@SuppressWarnings({"all"})
@SuppressFBWarnings
@ParametersAreNonnullByDefault
@javax.annotation.processing.Generated("org.immutables.processor.ProxyProcessor")
@Immutable
public final class OAuthCredentials implements OAuthCredentialsIF {
private final String accessToken;
private final String teamName;
private final String teamId;
private final @Nullable String scope;
private final @Nullable String userId;
private final @Nullable BotCredentials bot;
private final @Nullable IncomingWebhook incomingWebhook;
private OAuthCredentials(
String accessToken,
String teamName,
String teamId,
@Nullable String scope,
@Nullable String userId,
@Nullable BotCredentials bot,
@Nullable IncomingWebhook incomingWebhook) {
this.accessToken = accessToken;
this.teamName = teamName;
this.teamId = teamId;
this.scope = scope;
this.userId = userId;
this.bot = bot;
this.incomingWebhook = incomingWebhook;
}
/**
* @return The value of the {@code accessToken} attribute
*/
@JsonProperty
@Override
public String getAccessToken() {
return accessToken;
}
/**
* @return The value of the {@code teamName} attribute
*/
@JsonProperty
@Override
public String getTeamName() {
return teamName;
}
/**
* @return The value of the {@code teamId} attribute
*/
@JsonProperty
@Override
public String getTeamId() {
return teamId;
}
/**
* @return The value of the {@code scope} attribute
*/
@JsonProperty
@Override
public Optional getScope() {
return Optional.ofNullable(scope);
}
/**
* @return The value of the {@code userId} attribute
*/
@JsonProperty
@Override
public Optional getUserId() {
return Optional.ofNullable(userId);
}
/**
* @return The value of the {@code bot} attribute
*/
@JsonProperty
@Override
public Optional getBot() {
return Optional.ofNullable(bot);
}
/**
* @return The value of the {@code incomingWebhook} attribute
*/
@JsonProperty
@Override
public Optional getIncomingWebhook() {
return Optional.ofNullable(incomingWebhook);
}
/**
* Copy the current immutable object by setting a value for the {@link OAuthCredentialsIF#getAccessToken() accessToken} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for accessToken
* @return A modified copy of the {@code this} object
*/
public final OAuthCredentials withAccessToken(String value) {
String newValue = Objects.requireNonNull(value, "accessToken");
if (this.accessToken.equals(newValue)) return this;
return new OAuthCredentials(newValue, this.teamName, this.teamId, this.scope, this.userId, this.bot, this.incomingWebhook);
}
/**
* Copy the current immutable object by setting a value for the {@link OAuthCredentialsIF#getTeamName() teamName} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for teamName
* @return A modified copy of the {@code this} object
*/
public final OAuthCredentials withTeamName(String value) {
String newValue = Objects.requireNonNull(value, "teamName");
if (this.teamName.equals(newValue)) return this;
return new OAuthCredentials(
this.accessToken,
newValue,
this.teamId,
this.scope,
this.userId,
this.bot,
this.incomingWebhook);
}
/**
* Copy the current immutable object by setting a value for the {@link OAuthCredentialsIF#getTeamId() teamId} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for teamId
* @return A modified copy of the {@code this} object
*/
public final OAuthCredentials withTeamId(String value) {
String newValue = Objects.requireNonNull(value, "teamId");
if (this.teamId.equals(newValue)) return this;
return new OAuthCredentials(
this.accessToken,
this.teamName,
newValue,
this.scope,
this.userId,
this.bot,
this.incomingWebhook);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link OAuthCredentialsIF#getScope() scope} attribute.
* @param value The value for scope, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final OAuthCredentials withScope(@Nullable String value) {
@Nullable String newValue = value;
if (Objects.equals(this.scope, newValue)) return this;
return new OAuthCredentials(
this.accessToken,
this.teamName,
this.teamId,
newValue,
this.userId,
this.bot,
this.incomingWebhook);
}
/**
* Copy the current immutable object by setting an optional value for the {@link OAuthCredentialsIF#getScope() scope} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for scope
* @return A modified copy of {@code this} object
*/
public final OAuthCredentials withScope(Optional optional) {
@Nullable String value = optional.orElse(null);
if (Objects.equals(this.scope, value)) return this;
return new OAuthCredentials(
this.accessToken,
this.teamName,
this.teamId,
value,
this.userId,
this.bot,
this.incomingWebhook);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link OAuthCredentialsIF#getUserId() userId} attribute.
* @param value The value for userId, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final OAuthCredentials withUserId(@Nullable String value) {
@Nullable String newValue = value;
if (Objects.equals(this.userId, newValue)) return this;
return new OAuthCredentials(
this.accessToken,
this.teamName,
this.teamId,
this.scope,
newValue,
this.bot,
this.incomingWebhook);
}
/**
* Copy the current immutable object by setting an optional value for the {@link OAuthCredentialsIF#getUserId() userId} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for userId
* @return A modified copy of {@code this} object
*/
public final OAuthCredentials withUserId(Optional optional) {
@Nullable String value = optional.orElse(null);
if (Objects.equals(this.userId, value)) return this;
return new OAuthCredentials(this.accessToken, this.teamName, this.teamId, this.scope, value, this.bot, this.incomingWebhook);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link OAuthCredentialsIF#getBot() bot} attribute.
* @param value The value for bot, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final OAuthCredentials withBot(@Nullable BotCredentials value) {
@Nullable BotCredentials newValue = value;
if (this.bot == newValue) return this;
return new OAuthCredentials(
this.accessToken,
this.teamName,
this.teamId,
this.scope,
this.userId,
newValue,
this.incomingWebhook);
}
/**
* Copy the current immutable object by setting an optional value for the {@link OAuthCredentialsIF#getBot() bot} attribute.
* A shallow reference equality check is used on unboxed optional value to prevent copying of the same value by returning {@code this}.
* @param optional A value for bot
* @return A modified copy of {@code this} object
*/
@SuppressWarnings("unchecked") // safe covariant cast
public final OAuthCredentials withBot(Optional extends BotCredentials> optional) {
@Nullable BotCredentials value = optional.orElse(null);
if (this.bot == value) return this;
return new OAuthCredentials(
this.accessToken,
this.teamName,
this.teamId,
this.scope,
this.userId,
value,
this.incomingWebhook);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link OAuthCredentialsIF#getIncomingWebhook() incomingWebhook} attribute.
* @param value The value for incomingWebhook, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final OAuthCredentials withIncomingWebhook(@Nullable IncomingWebhook value) {
@Nullable IncomingWebhook newValue = value;
if (this.incomingWebhook == newValue) return this;
return new OAuthCredentials(this.accessToken, this.teamName, this.teamId, this.scope, this.userId, this.bot, newValue);
}
/**
* Copy the current immutable object by setting an optional value for the {@link OAuthCredentialsIF#getIncomingWebhook() incomingWebhook} attribute.
* A shallow reference equality check is used on unboxed optional value to prevent copying of the same value by returning {@code this}.
* @param optional A value for incomingWebhook
* @return A modified copy of {@code this} object
*/
@SuppressWarnings("unchecked") // safe covariant cast
public final OAuthCredentials withIncomingWebhook(Optional extends IncomingWebhook> optional) {
@Nullable IncomingWebhook value = optional.orElse(null);
if (this.incomingWebhook == value) return this;
return new OAuthCredentials(this.accessToken, this.teamName, this.teamId, this.scope, this.userId, this.bot, value);
}
/**
* This instance is equal to all instances of {@code OAuthCredentials} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(@Nullable Object another) {
if (this == another) return true;
return another instanceof OAuthCredentials
&& equalTo(0, (OAuthCredentials) another);
}
private boolean equalTo(int synthetic, OAuthCredentials another) {
return accessToken.equals(another.accessToken)
&& teamName.equals(another.teamName)
&& teamId.equals(another.teamId)
&& Objects.equals(scope, another.scope)
&& Objects.equals(userId, another.userId)
&& Objects.equals(bot, another.bot)
&& Objects.equals(incomingWebhook, another.incomingWebhook);
}
/**
* Computes a hash code from attributes: {@code accessToken}, {@code teamName}, {@code teamId}, {@code scope}, {@code userId}, {@code bot}, {@code incomingWebhook}.
* @return hashCode value
*/
@Override
public int hashCode() {
int h = 5381;
h += (h << 5) + accessToken.hashCode();
h += (h << 5) + teamName.hashCode();
h += (h << 5) + teamId.hashCode();
h += (h << 5) + Objects.hashCode(scope);
h += (h << 5) + Objects.hashCode(userId);
h += (h << 5) + Objects.hashCode(bot);
h += (h << 5) + Objects.hashCode(incomingWebhook);
return h;
}
/**
* Prints the immutable value {@code OAuthCredentials} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
StringBuilder builder = new StringBuilder("OAuthCredentials{");
builder.append("accessToken=").append(accessToken);
builder.append(", ");
builder.append("teamName=").append(teamName);
builder.append(", ");
builder.append("teamId=").append(teamId);
if (scope != null) {
builder.append(", ");
builder.append("scope=").append(scope);
}
if (userId != null) {
builder.append(", ");
builder.append("userId=").append(userId);
}
if (bot != null) {
builder.append(", ");
builder.append("bot=").append(bot);
}
if (incomingWebhook != null) {
builder.append(", ");
builder.append("incomingWebhook=").append(incomingWebhook);
}
return builder.append("}").toString();
}
/**
* Utility type used to correctly read immutable object from JSON representation.
* @deprecated Do not use this type directly, it exists only for the Jackson-binding infrastructure
*/
@Generated(from = "OAuthCredentialsIF", generator = "Immutables")
@Deprecated
@JsonAutoDetect(fieldVisibility = JsonAutoDetect.Visibility.NONE)
static final class Json implements OAuthCredentialsIF {
@Nullable String accessToken;
@Nullable String teamName;
@Nullable String teamId;
@Nullable Optional scope = Optional.empty();
@Nullable Optional userId = Optional.empty();
@Nullable Optional bot = Optional.empty();
@Nullable Optional incomingWebhook = Optional.empty();
@JsonProperty
public void setAccessToken(String accessToken) {
this.accessToken = accessToken;
}
@JsonProperty
public void setTeamName(String teamName) {
this.teamName = teamName;
}
@JsonProperty
public void setTeamId(String teamId) {
this.teamId = teamId;
}
@JsonProperty
public void setScope(Optional scope) {
this.scope = scope;
}
@JsonProperty
public void setUserId(Optional userId) {
this.userId = userId;
}
@JsonProperty
public void setBot(Optional bot) {
this.bot = bot;
}
@JsonProperty
public void setIncomingWebhook(Optional incomingWebhook) {
this.incomingWebhook = incomingWebhook;
}
@Override
public String getAccessToken() { throw new UnsupportedOperationException(); }
@Override
public String getTeamName() { throw new UnsupportedOperationException(); }
@Override
public String getTeamId() { throw new UnsupportedOperationException(); }
@Override
public Optional getScope() { throw new UnsupportedOperationException(); }
@Override
public Optional getUserId() { throw new UnsupportedOperationException(); }
@Override
public Optional getBot() { throw new UnsupportedOperationException(); }
@Override
public Optional getIncomingWebhook() { throw new UnsupportedOperationException(); }
}
/**
* @param json A JSON-bindable data structure
* @return An immutable value type
* @deprecated Do not use this method directly, it exists only for the Jackson-binding infrastructure
*/
@Deprecated
@JsonCreator(mode = JsonCreator.Mode.DELEGATING)
static OAuthCredentials fromJson(Json json) {
OAuthCredentials.Builder builder = OAuthCredentials.builder();
if (json.accessToken != null) {
builder.setAccessToken(json.accessToken);
}
if (json.teamName != null) {
builder.setTeamName(json.teamName);
}
if (json.teamId != null) {
builder.setTeamId(json.teamId);
}
if (json.scope != null) {
builder.setScope(json.scope);
}
if (json.userId != null) {
builder.setUserId(json.userId);
}
if (json.bot != null) {
builder.setBot(json.bot);
}
if (json.incomingWebhook != null) {
builder.setIncomingWebhook(json.incomingWebhook);
}
return builder.build();
}
/**
* Creates an immutable copy of a {@link OAuthCredentialsIF} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable OAuthCredentials instance
*/
public static OAuthCredentials copyOf(OAuthCredentialsIF instance) {
if (instance instanceof OAuthCredentials) {
return (OAuthCredentials) instance;
}
return OAuthCredentials.builder()
.from(instance)
.build();
}
/**
* Creates a builder for {@link OAuthCredentials OAuthCredentials}.
*
* OAuthCredentials.builder()
* .setAccessToken(String) // required {@link OAuthCredentialsIF#getAccessToken() accessToken}
* .setTeamName(String) // required {@link OAuthCredentialsIF#getTeamName() teamName}
* .setTeamId(String) // required {@link OAuthCredentialsIF#getTeamId() teamId}
* .setScope(String) // optional {@link OAuthCredentialsIF#getScope() scope}
* .setUserId(String) // optional {@link OAuthCredentialsIF#getUserId() userId}
* .setBot(BotCredentials) // optional {@link OAuthCredentialsIF#getBot() bot}
* .setIncomingWebhook(IncomingWebhook) // optional {@link OAuthCredentialsIF#getIncomingWebhook() incomingWebhook}
* .build();
*
* @return A new OAuthCredentials builder
*/
public static OAuthCredentials.Builder builder() {
return new OAuthCredentials.Builder();
}
/**
* Builds instances of type {@link OAuthCredentials OAuthCredentials}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
@Generated(from = "OAuthCredentialsIF", generator = "Immutables")
@NotThreadSafe
public static final class Builder {
private static final long INIT_BIT_ACCESS_TOKEN = 0x1L;
private static final long INIT_BIT_TEAM_NAME = 0x2L;
private static final long INIT_BIT_TEAM_ID = 0x4L;
private long initBits = 0x7L;
private @Nullable String accessToken;
private @Nullable String teamName;
private @Nullable String teamId;
private @Nullable String scope;
private @Nullable String userId;
private @Nullable BotCredentials bot;
private @Nullable IncomingWebhook incomingWebhook;
private Builder() {
}
/**
* Fill a builder with attribute values from the provided {@code OAuthCredentialsIF} instance.
* Regular attribute values will be replaced with those from the given instance.
* Absent optional values will not replace present values.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(OAuthCredentialsIF instance) {
Objects.requireNonNull(instance, "instance");
this.setAccessToken(instance.getAccessToken());
this.setTeamName(instance.getTeamName());
this.setTeamId(instance.getTeamId());
Optional scopeOptional = instance.getScope();
if (scopeOptional.isPresent()) {
setScope(scopeOptional);
}
Optional userIdOptional = instance.getUserId();
if (userIdOptional.isPresent()) {
setUserId(userIdOptional);
}
Optional botOptional = instance.getBot();
if (botOptional.isPresent()) {
setBot(botOptional);
}
Optional incomingWebhookOptional = instance.getIncomingWebhook();
if (incomingWebhookOptional.isPresent()) {
setIncomingWebhook(incomingWebhookOptional);
}
return this;
}
/**
* Initializes the value for the {@link OAuthCredentialsIF#getAccessToken() accessToken} attribute.
* @param accessToken The value for accessToken
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setAccessToken(String accessToken) {
this.accessToken = Objects.requireNonNull(accessToken, "accessToken");
initBits &= ~INIT_BIT_ACCESS_TOKEN;
return this;
}
/**
* Initializes the value for the {@link OAuthCredentialsIF#getTeamName() teamName} attribute.
* @param teamName The value for teamName
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setTeamName(String teamName) {
this.teamName = Objects.requireNonNull(teamName, "teamName");
initBits &= ~INIT_BIT_TEAM_NAME;
return this;
}
/**
* Initializes the value for the {@link OAuthCredentialsIF#getTeamId() teamId} attribute.
* @param teamId The value for teamId
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setTeamId(String teamId) {
this.teamId = Objects.requireNonNull(teamId, "teamId");
initBits &= ~INIT_BIT_TEAM_ID;
return this;
}
/**
* Initializes the optional value {@link OAuthCredentialsIF#getScope() scope} to scope.
* @param scope The value for scope, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
public final Builder setScope(@Nullable String scope) {
this.scope = scope;
return this;
}
/**
* Initializes the optional value {@link OAuthCredentialsIF#getScope() scope} to scope.
* @param scope The value for scope
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setScope(Optional scope) {
this.scope = scope.orElse(null);
return this;
}
/**
* Initializes the optional value {@link OAuthCredentialsIF#getUserId() userId} to userId.
* @param userId The value for userId, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
public final Builder setUserId(@Nullable String userId) {
this.userId = userId;
return this;
}
/**
* Initializes the optional value {@link OAuthCredentialsIF#getUserId() userId} to userId.
* @param userId The value for userId
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setUserId(Optional userId) {
this.userId = userId.orElse(null);
return this;
}
/**
* Initializes the optional value {@link OAuthCredentialsIF#getBot() bot} to bot.
* @param bot The value for bot, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
public final Builder setBot(@Nullable BotCredentials bot) {
this.bot = bot;
return this;
}
/**
* Initializes the optional value {@link OAuthCredentialsIF#getBot() bot} to bot.
* @param bot The value for bot
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setBot(Optional extends BotCredentials> bot) {
this.bot = bot.orElse(null);
return this;
}
/**
* Initializes the optional value {@link OAuthCredentialsIF#getIncomingWebhook() incomingWebhook} to incomingWebhook.
* @param incomingWebhook The value for incomingWebhook, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
public final Builder setIncomingWebhook(@Nullable IncomingWebhook incomingWebhook) {
this.incomingWebhook = incomingWebhook;
return this;
}
/**
* Initializes the optional value {@link OAuthCredentialsIF#getIncomingWebhook() incomingWebhook} to incomingWebhook.
* @param incomingWebhook The value for incomingWebhook
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setIncomingWebhook(Optional extends IncomingWebhook> incomingWebhook) {
this.incomingWebhook = incomingWebhook.orElse(null);
return this;
}
/**
* Builds a new {@link OAuthCredentials OAuthCredentials}.
* @return An immutable instance of OAuthCredentials
* @throws com.hubspot.immutables.validation.InvalidImmutableStateException if any required attributes are missing
*/
public OAuthCredentials build() {
checkRequiredAttributes();
return new OAuthCredentials(accessToken, teamName, teamId, scope, userId, bot, incomingWebhook);
}
private boolean accessTokenIsSet() {
return (initBits & INIT_BIT_ACCESS_TOKEN) == 0;
}
private boolean teamNameIsSet() {
return (initBits & INIT_BIT_TEAM_NAME) == 0;
}
private boolean teamIdIsSet() {
return (initBits & INIT_BIT_TEAM_ID) == 0;
}
private void checkRequiredAttributes() {
if (initBits != 0) {
throw new InvalidImmutableStateException(formatRequiredAttributesMessage());
}
}
private String formatRequiredAttributesMessage() {
List attributes = new ArrayList<>();
if (!accessTokenIsSet()) attributes.add("accessToken");
if (!teamNameIsSet()) attributes.add("teamName");
if (!teamIdIsSet()) attributes.add("teamId");
return "Cannot build OAuthCredentials, some of required attributes are not set " + attributes;
}
}
}