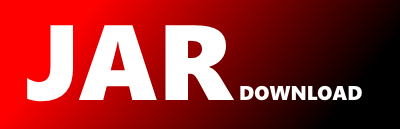
com.hubspot.slack.client.models.blocks.Input Maven / Gradle / Ivy
package com.hubspot.slack.client.models.blocks;
import com.fasterxml.jackson.annotation.JsonAutoDetect;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonTypeInfo;
import com.hubspot.immutables.validation.InvalidImmutableStateException;
import com.hubspot.slack.client.models.blocks.elements.BlockElement;
import com.hubspot.slack.client.models.blocks.objects.Text;
import edu.umd.cs.findbugs.annotations.SuppressFBWarnings;
import java.util.ArrayList;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
import javax.annotation.ParametersAreNonnullByDefault;
import javax.annotation.concurrent.Immutable;
import javax.annotation.concurrent.NotThreadSafe;
import org.immutables.value.Generated;
/**
* Immutable implementation of {@link InputIF}.
*
* Use the builder to create immutable instances:
* {@code Input.builder()}.
* Use the static factory method to create immutable instances:
* {@code Input.of()}.
*/
@Generated(from = "InputIF", generator = "Immutables")
@SuppressWarnings({"all"})
@SuppressFBWarnings
@ParametersAreNonnullByDefault
@javax.annotation.processing.Generated("org.immutables.processor.ProxyProcessor")
@Immutable
public final class Input implements InputIF {
private final @Nullable String blockId;
private transient final String type;
private final Text label;
private final BlockElement element;
private final @Nullable Text hint;
private final @Nullable Boolean optional;
private final @Nullable Boolean dispatchAction;
private Input(
Text label,
BlockElement element) {
this.label = Objects.requireNonNull(label, "label");
this.element = Objects.requireNonNull(element, "element");
this.blockId = null;
this.hint = null;
this.optional = null;
this.dispatchAction = null;
this.type = Objects.requireNonNull(InputIF.super.getType(), "type");
}
private Input(
@Nullable String blockId,
Text label,
BlockElement element,
@Nullable Text hint,
@Nullable Boolean optional,
@Nullable Boolean dispatchAction) {
this.blockId = blockId;
this.label = label;
this.element = element;
this.hint = hint;
this.optional = optional;
this.dispatchAction = dispatchAction;
this.type = Objects.requireNonNull(InputIF.super.getType(), "type");
}
/**
* @return The value of the {@code blockId} attribute
*/
@JsonProperty
@Override
public Optional getBlockId() {
return Optional.ofNullable(blockId);
}
/**
* @return The computed-at-construction value of the {@code type} attribute
*/
@JsonProperty
@Override
public String getType() {
return type;
}
/**
* @return The value of the {@code label} attribute
*/
@JsonProperty
@Override
public Text getLabel() {
return label;
}
/**
* @return The value of the {@code element} attribute
*/
@JsonProperty
@Override
public BlockElement getElement() {
return element;
}
/**
* @return The value of the {@code hint} attribute
*/
@JsonProperty
@Override
public Optional getHint() {
return Optional.ofNullable(hint);
}
/**
* @return The value of the {@code optional} attribute
*/
@JsonProperty("optional")
@Override
public Optional isOptional() {
return Optional.ofNullable(optional);
}
/**
* @return The value of the {@code dispatchAction} attribute
*/
@JsonProperty
@Override
public Optional getDispatchAction() {
return Optional.ofNullable(dispatchAction);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link InputIF#getBlockId() blockId} attribute.
* @param value The value for blockId, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final Input withBlockId(@Nullable String value) {
@Nullable String newValue = value;
if (Objects.equals(this.blockId, newValue)) return this;
return validate(new Input(newValue, this.label, this.element, this.hint, this.optional, this.dispatchAction));
}
/**
* Copy the current immutable object by setting an optional value for the {@link InputIF#getBlockId() blockId} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for blockId
* @return A modified copy of {@code this} object
*/
public final Input withBlockId(Optional optional) {
@Nullable String value = optional.orElse(null);
if (Objects.equals(this.blockId, value)) return this;
return validate(new Input(value, this.label, this.element, this.hint, this.optional, this.dispatchAction));
}
/**
* Copy the current immutable object by setting a value for the {@link InputIF#getLabel() label} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for label
* @return A modified copy of the {@code this} object
*/
public final Input withLabel(Text value) {
if (this.label == value) return this;
Text newValue = Objects.requireNonNull(value, "label");
return validate(new Input(this.blockId, newValue, this.element, this.hint, this.optional, this.dispatchAction));
}
/**
* Copy the current immutable object by setting a value for the {@link InputIF#getElement() element} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for element
* @return A modified copy of the {@code this} object
*/
public final Input withElement(BlockElement value) {
if (this.element == value) return this;
BlockElement newValue = Objects.requireNonNull(value, "element");
return validate(new Input(this.blockId, this.label, newValue, this.hint, this.optional, this.dispatchAction));
}
/**
* Copy the current immutable object by setting a present value for the optional {@link InputIF#getHint() hint} attribute.
* @param value The value for hint, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final Input withHint(@Nullable Text value) {
@Nullable Text newValue = value;
if (this.hint == newValue) return this;
return validate(new Input(this.blockId, this.label, this.element, newValue, this.optional, this.dispatchAction));
}
/**
* Copy the current immutable object by setting an optional value for the {@link InputIF#getHint() hint} attribute.
* A shallow reference equality check is used on unboxed optional value to prevent copying of the same value by returning {@code this}.
* @param optional A value for hint
* @return A modified copy of {@code this} object
*/
@SuppressWarnings("unchecked") // safe covariant cast
public final Input withHint(Optional extends Text> optional) {
@Nullable Text value = optional.orElse(null);
if (this.hint == value) return this;
return validate(new Input(this.blockId, this.label, this.element, value, this.optional, this.dispatchAction));
}
/**
* Copy the current immutable object by setting a present value for the optional {@link InputIF#isOptional() optional} attribute.
* @param value The value for optional, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final Input withOptional(@Nullable Boolean value) {
@Nullable Boolean newValue = value;
if (Objects.equals(this.optional, newValue)) return this;
return validate(new Input(this.blockId, this.label, this.element, this.hint, newValue, this.dispatchAction));
}
/**
* Copy the current immutable object by setting an optional value for the {@link InputIF#isOptional() optional} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for optional
* @return A modified copy of {@code this} object
*/
public final Input withOptional(Optional optional) {
@Nullable Boolean value = optional.orElse(null);
if (Objects.equals(this.optional, value)) return this;
return validate(new Input(this.blockId, this.label, this.element, this.hint, value, this.dispatchAction));
}
/**
* Copy the current immutable object by setting a present value for the optional {@link InputIF#getDispatchAction() dispatchAction} attribute.
* @param value The value for dispatchAction, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final Input withDispatchAction(@Nullable Boolean value) {
@Nullable Boolean newValue = value;
if (Objects.equals(this.dispatchAction, newValue)) return this;
return validate(new Input(this.blockId, this.label, this.element, this.hint, this.optional, newValue));
}
/**
* Copy the current immutable object by setting an optional value for the {@link InputIF#getDispatchAction() dispatchAction} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for dispatchAction
* @return A modified copy of {@code this} object
*/
public final Input withDispatchAction(Optional optional) {
@Nullable Boolean value = optional.orElse(null);
if (Objects.equals(this.dispatchAction, value)) return this;
return validate(new Input(this.blockId, this.label, this.element, this.hint, this.optional, value));
}
/**
* This instance is equal to all instances of {@code Input} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(@Nullable Object another) {
if (this == another) return true;
return another instanceof Input
&& equalTo(0, (Input) another);
}
private boolean equalTo(int synthetic, Input another) {
return Objects.equals(blockId, another.blockId)
&& type.equals(another.type)
&& label.equals(another.label)
&& element.equals(another.element)
&& Objects.equals(hint, another.hint)
&& Objects.equals(optional, another.optional)
&& Objects.equals(dispatchAction, another.dispatchAction);
}
/**
* Computes a hash code from attributes: {@code blockId}, {@code type}, {@code label}, {@code element}, {@code hint}, {@code optional}, {@code dispatchAction}.
* @return hashCode value
*/
@Override
public int hashCode() {
int h = 5381;
h += (h << 5) + Objects.hashCode(blockId);
h += (h << 5) + type.hashCode();
h += (h << 5) + label.hashCode();
h += (h << 5) + element.hashCode();
h += (h << 5) + Objects.hashCode(hint);
h += (h << 5) + Objects.hashCode(optional);
h += (h << 5) + Objects.hashCode(dispatchAction);
return h;
}
/**
* Prints the immutable value {@code Input} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
StringBuilder builder = new StringBuilder("Input{");
if (blockId != null) {
builder.append("blockId=").append(blockId);
}
if (builder.length() > 6) builder.append(", ");
builder.append("type=").append(type);
builder.append(", ");
builder.append("label=").append(label);
builder.append(", ");
builder.append("element=").append(element);
if (hint != null) {
builder.append(", ");
builder.append("hint=").append(hint);
}
if (optional != null) {
builder.append(", ");
builder.append("optional=").append(optional);
}
if (dispatchAction != null) {
builder.append(", ");
builder.append("dispatchAction=").append(dispatchAction);
}
return builder.append("}").toString();
}
/**
* Utility type used to correctly read immutable object from JSON representation.
* @deprecated Do not use this type directly, it exists only for the Jackson-binding infrastructure
*/
@Generated(from = "InputIF", generator = "Immutables")
@Deprecated
@JsonTypeInfo(use=JsonTypeInfo.Id.NONE)
@JsonAutoDetect(fieldVisibility = JsonAutoDetect.Visibility.NONE)
static final class Json implements InputIF {
@Nullable Optional blockId = Optional.empty();
@Nullable Text label;
@Nullable BlockElement element;
@Nullable Optional hint = Optional.empty();
@Nullable Optional optional = Optional.empty();
@Nullable Optional dispatchAction = Optional.empty();
@JsonProperty
public void setBlockId(Optional blockId) {
this.blockId = blockId;
}
@JsonProperty
public void setLabel(Text label) {
this.label = label;
}
@JsonProperty
public void setElement(BlockElement element) {
this.element = element;
}
@JsonProperty
public void setHint(Optional hint) {
this.hint = hint;
}
@JsonProperty("optional")
public void setOptional(Optional optional) {
this.optional = optional;
}
@JsonProperty
public void setDispatchAction(Optional dispatchAction) {
this.dispatchAction = dispatchAction;
}
@Override
public Optional getBlockId() { throw new UnsupportedOperationException(); }
@JsonIgnore
@Override
public String getType() { throw new UnsupportedOperationException(); }
@Override
public Text getLabel() { throw new UnsupportedOperationException(); }
@Override
public BlockElement getElement() { throw new UnsupportedOperationException(); }
@Override
public Optional getHint() { throw new UnsupportedOperationException(); }
@Override
public Optional isOptional() { throw new UnsupportedOperationException(); }
@Override
public Optional getDispatchAction() { throw new UnsupportedOperationException(); }
}
/**
* @param json A JSON-bindable data structure
* @return An immutable value type
* @deprecated Do not use this method directly, it exists only for the Jackson-binding infrastructure
*/
@Deprecated
@JsonCreator(mode = JsonCreator.Mode.DELEGATING)
static Input fromJson(Json json) {
Input.Builder builder = Input.builder();
if (json.blockId != null) {
builder.setBlockId(json.blockId);
}
if (json.label != null) {
builder.setLabel(json.label);
}
if (json.element != null) {
builder.setElement(json.element);
}
if (json.hint != null) {
builder.setHint(json.hint);
}
if (json.optional != null) {
builder.setOptional(json.optional);
}
if (json.dispatchAction != null) {
builder.setDispatchAction(json.dispatchAction);
}
return builder.build();
}
/**
* Construct a new immutable {@code Input} instance.
* @param label The value for the {@code label} attribute
* @param element The value for the {@code element} attribute
* @return An immutable Input instance
*/
public static Input of(Text label, BlockElement element) {
return validate(new Input(label, element));
}
private static Input validate(Input instance) {
instance = (Input) instance.validate();
return instance;
}
/**
* Creates an immutable copy of a {@link InputIF} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable Input instance
*/
public static Input copyOf(InputIF instance) {
if (instance instanceof Input) {
return (Input) instance;
}
return Input.builder()
.from(instance)
.build();
}
/**
* Creates a builder for {@link Input Input}.
*
* Input.builder()
* .setBlockId(String) // optional {@link InputIF#getBlockId() blockId}
* .setLabel(com.hubspot.slack.client.models.blocks.objects.Text) // required {@link InputIF#getLabel() label}
* .setElement(com.hubspot.slack.client.models.blocks.elements.BlockElement) // required {@link InputIF#getElement() element}
* .setHint(com.hubspot.slack.client.models.blocks.objects.Text) // optional {@link InputIF#getHint() hint}
* .setOptional(Boolean) // optional {@link InputIF#isOptional() optional}
* .setDispatchAction(Boolean) // optional {@link InputIF#getDispatchAction() dispatchAction}
* .build();
*
* @return A new Input builder
*/
public static Input.Builder builder() {
return new Input.Builder();
}
/**
* Builds instances of type {@link Input Input}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
@Generated(from = "InputIF", generator = "Immutables")
@NotThreadSafe
public static final class Builder {
private static final long INIT_BIT_LABEL = 0x1L;
private static final long INIT_BIT_ELEMENT = 0x2L;
private long initBits = 0x3L;
private @Nullable String blockId;
private @Nullable Text label;
private @Nullable BlockElement element;
private @Nullable Text hint;
private @Nullable Boolean optional;
private @Nullable Boolean dispatchAction;
private Builder() {
}
/**
* Fill a builder with attribute values from the provided {@code com.hubspot.slack.client.models.blocks.InputIF} instance.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(InputIF instance) {
Objects.requireNonNull(instance, "instance");
from((short) 0, (Object) instance);
return this;
}
/**
* Fill a builder with attribute values from the provided {@code com.hubspot.slack.client.models.blocks.Block} instance.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(Block instance) {
Objects.requireNonNull(instance, "instance");
from((short) 0, (Object) instance);
return this;
}
private void from(short _unused, Object object) {
long bits = 0;
if (object instanceof InputIF) {
InputIF instance = (InputIF) object;
if ((bits & 0x1L) == 0) {
Optional blockIdOptional = instance.getBlockId();
if (blockIdOptional.isPresent()) {
setBlockId(blockIdOptional);
}
bits |= 0x1L;
}
Optional optionalOptional = instance.isOptional();
if (optionalOptional.isPresent()) {
setOptional(optionalOptional);
}
Optional dispatchActionOptional = instance.getDispatchAction();
if (dispatchActionOptional.isPresent()) {
setDispatchAction(dispatchActionOptional);
}
this.setLabel(instance.getLabel());
Optional hintOptional = instance.getHint();
if (hintOptional.isPresent()) {
setHint(hintOptional);
}
this.setElement(instance.getElement());
}
if (object instanceof Block) {
Block instance = (Block) object;
if ((bits & 0x1L) == 0) {
Optional blockIdOptional = instance.getBlockId();
if (blockIdOptional.isPresent()) {
setBlockId(blockIdOptional);
}
bits |= 0x1L;
}
}
}
/**
* Initializes the optional value {@link InputIF#getBlockId() blockId} to blockId.
* @param blockId The value for blockId, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
public final Builder setBlockId(@Nullable String blockId) {
this.blockId = blockId;
return this;
}
/**
* Initializes the optional value {@link InputIF#getBlockId() blockId} to blockId.
* @param blockId The value for blockId
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setBlockId(Optional blockId) {
this.blockId = blockId.orElse(null);
return this;
}
/**
* Initializes the value for the {@link InputIF#getLabel() label} attribute.
* @param label The value for label
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setLabel(Text label) {
this.label = Objects.requireNonNull(label, "label");
initBits &= ~INIT_BIT_LABEL;
return this;
}
/**
* Initializes the value for the {@link InputIF#getElement() element} attribute.
* @param element The value for element
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setElement(BlockElement element) {
this.element = Objects.requireNonNull(element, "element");
initBits &= ~INIT_BIT_ELEMENT;
return this;
}
/**
* Initializes the optional value {@link InputIF#getHint() hint} to hint.
* @param hint The value for hint, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
public final Builder setHint(@Nullable Text hint) {
this.hint = hint;
return this;
}
/**
* Initializes the optional value {@link InputIF#getHint() hint} to hint.
* @param hint The value for hint
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setHint(Optional extends Text> hint) {
this.hint = hint.orElse(null);
return this;
}
/**
* Initializes the optional value {@link InputIF#isOptional() optional} to optional.
* @param optional The value for optional, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
public final Builder setOptional(@Nullable Boolean optional) {
this.optional = optional;
return this;
}
/**
* Initializes the optional value {@link InputIF#isOptional() optional} to optional.
* @param optional The value for optional
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setOptional(Optional optional) {
this.optional = optional.orElse(null);
return this;
}
/**
* Initializes the optional value {@link InputIF#getDispatchAction() dispatchAction} to dispatchAction.
* @param dispatchAction The value for dispatchAction, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
public final Builder setDispatchAction(@Nullable Boolean dispatchAction) {
this.dispatchAction = dispatchAction;
return this;
}
/**
* Initializes the optional value {@link InputIF#getDispatchAction() dispatchAction} to dispatchAction.
* @param dispatchAction The value for dispatchAction
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setDispatchAction(Optional dispatchAction) {
this.dispatchAction = dispatchAction.orElse(null);
return this;
}
/**
* Builds a new {@link Input Input}.
* @return An immutable instance of Input
* @throws com.hubspot.immutables.validation.InvalidImmutableStateException if any required attributes are missing
*/
public Input build() {
checkRequiredAttributes();
return Input.validate(new Input(blockId, label, element, hint, optional, dispatchAction));
}
private boolean labelIsSet() {
return (initBits & INIT_BIT_LABEL) == 0;
}
private boolean elementIsSet() {
return (initBits & INIT_BIT_ELEMENT) == 0;
}
private void checkRequiredAttributes() {
if (initBits != 0) {
throw new InvalidImmutableStateException(formatRequiredAttributesMessage());
}
}
private String formatRequiredAttributesMessage() {
List attributes = new ArrayList<>();
if (!labelIsSet()) attributes.add("label");
if (!elementIsSet()) attributes.add("element");
return "Cannot build Input, some of required attributes are not set " + attributes;
}
}
}