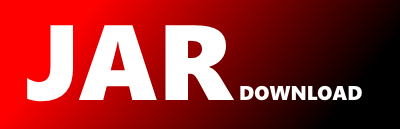
com.hubspot.slack.client.models.blocks.elements.UserSelectMenu Maven / Gradle / Ivy
package com.hubspot.slack.client.models.blocks.elements;
import com.fasterxml.jackson.annotation.JsonAutoDetect;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonTypeInfo;
import com.hubspot.immutables.validation.InvalidImmutableStateException;
import com.hubspot.slack.client.models.blocks.objects.ConfirmationDialog;
import com.hubspot.slack.client.models.blocks.objects.Text;
import edu.umd.cs.findbugs.annotations.SuppressFBWarnings;
import java.util.ArrayList;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
import javax.annotation.ParametersAreNonnullByDefault;
import javax.annotation.concurrent.Immutable;
import javax.annotation.concurrent.NotThreadSafe;
import org.immutables.value.Generated;
/**
* Immutable implementation of {@link UserSelectMenuIF}.
*
* Use the builder to create immutable instances:
* {@code UserSelectMenu.builder()}.
* Use the static factory method to create immutable instances:
* {@code UserSelectMenu.of()}.
*/
@Generated(from = "UserSelectMenuIF", generator = "Immutables")
@SuppressWarnings({"all"})
@SuppressFBWarnings
@ParametersAreNonnullByDefault
@javax.annotation.processing.Generated("org.immutables.processor.ProxyProcessor")
@Immutable
public final class UserSelectMenu implements UserSelectMenuIF {
private transient final String type;
private final Text placeholder;
private final String actionId;
private final @Nullable String initialUserId;
private final @Nullable ConfirmationDialog confirmationDialog;
private UserSelectMenu(Text placeholder, String actionId) {
this.placeholder = Objects.requireNonNull(placeholder, "placeholder");
this.actionId = Objects.requireNonNull(actionId, "actionId");
this.initialUserId = null;
this.confirmationDialog = null;
this.type = Objects.requireNonNull(UserSelectMenuIF.super.getType(), "type");
}
private UserSelectMenu(
Text placeholder,
String actionId,
@Nullable String initialUserId,
@Nullable ConfirmationDialog confirmationDialog) {
this.placeholder = placeholder;
this.actionId = actionId;
this.initialUserId = initialUserId;
this.confirmationDialog = confirmationDialog;
this.type = Objects.requireNonNull(UserSelectMenuIF.super.getType(), "type");
}
/**
* @return The computed-at-construction value of the {@code type} attribute
*/
@JsonProperty
@Override
public String getType() {
return type;
}
/**
* @return The value of the {@code placeholder} attribute
*/
@JsonProperty
@Override
public Text getPlaceholder() {
return placeholder;
}
/**
* @return The value of the {@code actionId} attribute
*/
@JsonProperty
@Override
public String getActionId() {
return actionId;
}
/**
* @return The value of the {@code initialUserId} attribute
*/
@JsonProperty("initial_user")
@Override
public Optional getInitialUserId() {
return Optional.ofNullable(initialUserId);
}
/**
* @return The value of the {@code confirmationDialog} attribute
*/
@JsonProperty("confirm")
@Override
public Optional getConfirmationDialog() {
return Optional.ofNullable(confirmationDialog);
}
/**
* Copy the current immutable object by setting a value for the {@link UserSelectMenuIF#getPlaceholder() placeholder} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for placeholder
* @return A modified copy of the {@code this} object
*/
public final UserSelectMenu withPlaceholder(Text value) {
if (this.placeholder == value) return this;
Text newValue = Objects.requireNonNull(value, "placeholder");
return new UserSelectMenu(newValue, this.actionId, this.initialUserId, this.confirmationDialog);
}
/**
* Copy the current immutable object by setting a value for the {@link UserSelectMenuIF#getActionId() actionId} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for actionId
* @return A modified copy of the {@code this} object
*/
public final UserSelectMenu withActionId(String value) {
String newValue = Objects.requireNonNull(value, "actionId");
if (this.actionId.equals(newValue)) return this;
return new UserSelectMenu(this.placeholder, newValue, this.initialUserId, this.confirmationDialog);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link UserSelectMenuIF#getInitialUserId() initialUserId} attribute.
* @param value The value for initialUserId, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final UserSelectMenu withInitialUserId(@Nullable String value) {
@Nullable String newValue = value;
if (Objects.equals(this.initialUserId, newValue)) return this;
return new UserSelectMenu(this.placeholder, this.actionId, newValue, this.confirmationDialog);
}
/**
* Copy the current immutable object by setting an optional value for the {@link UserSelectMenuIF#getInitialUserId() initialUserId} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for initialUserId
* @return A modified copy of {@code this} object
*/
public final UserSelectMenu withInitialUserId(Optional optional) {
@Nullable String value = optional.orElse(null);
if (Objects.equals(this.initialUserId, value)) return this;
return new UserSelectMenu(this.placeholder, this.actionId, value, this.confirmationDialog);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link UserSelectMenuIF#getConfirmationDialog() confirmationDialog} attribute.
* @param value The value for confirmationDialog, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final UserSelectMenu withConfirmationDialog(@Nullable ConfirmationDialog value) {
@Nullable ConfirmationDialog newValue = value;
if (this.confirmationDialog == newValue) return this;
return new UserSelectMenu(this.placeholder, this.actionId, this.initialUserId, newValue);
}
/**
* Copy the current immutable object by setting an optional value for the {@link UserSelectMenuIF#getConfirmationDialog() confirmationDialog} attribute.
* A shallow reference equality check is used on unboxed optional value to prevent copying of the same value by returning {@code this}.
* @param optional A value for confirmationDialog
* @return A modified copy of {@code this} object
*/
@SuppressWarnings("unchecked") // safe covariant cast
public final UserSelectMenu withConfirmationDialog(Optional optional) {
@Nullable ConfirmationDialog value = optional.orElse(null);
if (this.confirmationDialog == value) return this;
return new UserSelectMenu(this.placeholder, this.actionId, this.initialUserId, value);
}
/**
* This instance is equal to all instances of {@code UserSelectMenu} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(@Nullable Object another) {
if (this == another) return true;
return another instanceof UserSelectMenu
&& equalTo(0, (UserSelectMenu) another);
}
private boolean equalTo(int synthetic, UserSelectMenu another) {
return type.equals(another.type)
&& placeholder.equals(another.placeholder)
&& actionId.equals(another.actionId)
&& Objects.equals(initialUserId, another.initialUserId)
&& Objects.equals(confirmationDialog, another.confirmationDialog);
}
/**
* Computes a hash code from attributes: {@code type}, {@code placeholder}, {@code actionId}, {@code initialUserId}, {@code confirmationDialog}.
* @return hashCode value
*/
@Override
public int hashCode() {
int h = 5381;
h += (h << 5) + type.hashCode();
h += (h << 5) + placeholder.hashCode();
h += (h << 5) + actionId.hashCode();
h += (h << 5) + Objects.hashCode(initialUserId);
h += (h << 5) + Objects.hashCode(confirmationDialog);
return h;
}
/**
* Prints the immutable value {@code UserSelectMenu} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
StringBuilder builder = new StringBuilder("UserSelectMenu{");
builder.append("type=").append(type);
builder.append(", ");
builder.append("placeholder=").append(placeholder);
builder.append(", ");
builder.append("actionId=").append(actionId);
if (initialUserId != null) {
builder.append(", ");
builder.append("initialUserId=").append(initialUserId);
}
if (confirmationDialog != null) {
builder.append(", ");
builder.append("confirmationDialog=").append(confirmationDialog);
}
return builder.append("}").toString();
}
/**
* Utility type used to correctly read immutable object from JSON representation.
* @deprecated Do not use this type directly, it exists only for the Jackson-binding infrastructure
*/
@Generated(from = "UserSelectMenuIF", generator = "Immutables")
@Deprecated
@JsonTypeInfo(use=JsonTypeInfo.Id.NONE)
@JsonAutoDetect(fieldVisibility = JsonAutoDetect.Visibility.NONE)
static final class Json implements UserSelectMenuIF {
@Nullable Text placeholder;
@Nullable String actionId;
@Nullable Optional initialUserId = Optional.empty();
@Nullable Optional confirmationDialog = Optional.empty();
@JsonProperty
public void setPlaceholder(Text placeholder) {
this.placeholder = placeholder;
}
@JsonProperty
public void setActionId(String actionId) {
this.actionId = actionId;
}
@JsonProperty("initial_user")
public void setInitialUserId(Optional initialUserId) {
this.initialUserId = initialUserId;
}
@JsonProperty("confirm")
public void setConfirmationDialog(Optional confirmationDialog) {
this.confirmationDialog = confirmationDialog;
}
@JsonIgnore
@Override
public String getType() { throw new UnsupportedOperationException(); }
@Override
public Text getPlaceholder() { throw new UnsupportedOperationException(); }
@Override
public String getActionId() { throw new UnsupportedOperationException(); }
@Override
public Optional getInitialUserId() { throw new UnsupportedOperationException(); }
@Override
public Optional getConfirmationDialog() { throw new UnsupportedOperationException(); }
}
/**
* @param json A JSON-bindable data structure
* @return An immutable value type
* @deprecated Do not use this method directly, it exists only for the Jackson-binding infrastructure
*/
@Deprecated
@JsonCreator(mode = JsonCreator.Mode.DELEGATING)
static UserSelectMenu fromJson(Json json) {
UserSelectMenu.Builder builder = UserSelectMenu.builder();
if (json.placeholder != null) {
builder.setPlaceholder(json.placeholder);
}
if (json.actionId != null) {
builder.setActionId(json.actionId);
}
if (json.initialUserId != null) {
builder.setInitialUserId(json.initialUserId);
}
if (json.confirmationDialog != null) {
builder.setConfirmationDialog(json.confirmationDialog);
}
return builder.build();
}
/**
* Construct a new immutable {@code UserSelectMenu} instance.
* @param placeholder The value for the {@code placeholder} attribute
* @param actionId The value for the {@code actionId} attribute
* @return An immutable UserSelectMenu instance
*/
public static UserSelectMenu of(Text placeholder, String actionId) {
return new UserSelectMenu(placeholder, actionId);
}
/**
* Creates an immutable copy of a {@link UserSelectMenuIF} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable UserSelectMenu instance
*/
public static UserSelectMenu copyOf(UserSelectMenuIF instance) {
if (instance instanceof UserSelectMenu) {
return (UserSelectMenu) instance;
}
return UserSelectMenu.builder()
.from(instance)
.build();
}
/**
* Creates a builder for {@link UserSelectMenu UserSelectMenu}.
*
* UserSelectMenu.builder()
* .setPlaceholder(com.hubspot.slack.client.models.blocks.objects.Text) // required {@link UserSelectMenuIF#getPlaceholder() placeholder}
* .setActionId(String) // required {@link UserSelectMenuIF#getActionId() actionId}
* .setInitialUserId(String) // optional {@link UserSelectMenuIF#getInitialUserId() initialUserId}
* .setConfirmationDialog(com.hubspot.slack.client.models.blocks.objects.ConfirmationDialog) // optional {@link UserSelectMenuIF#getConfirmationDialog() confirmationDialog}
* .build();
*
* @return A new UserSelectMenu builder
*/
public static UserSelectMenu.Builder builder() {
return new UserSelectMenu.Builder();
}
/**
* Builds instances of type {@link UserSelectMenu UserSelectMenu}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
*
{@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
@Generated(from = "UserSelectMenuIF", generator = "Immutables")
@NotThreadSafe
public static final class Builder {
private static final long INIT_BIT_PLACEHOLDER = 0x1L;
private static final long INIT_BIT_ACTION_ID = 0x2L;
private long initBits = 0x3L;
private @Nullable Text placeholder;
private @Nullable String actionId;
private @Nullable String initialUserId;
private @Nullable ConfirmationDialog confirmationDialog;
private Builder() {
}
/**
* Fill a builder with attribute values from the provided {@code com.hubspot.slack.client.models.blocks.elements.HasActionId} instance.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(HasActionId instance) {
Objects.requireNonNull(instance, "instance");
from((short) 0, (Object) instance);
return this;
}
/**
* Fill a builder with attribute values from the provided {@code com.hubspot.slack.client.models.blocks.elements.UserSelectMenuIF} instance.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(UserSelectMenuIF instance) {
Objects.requireNonNull(instance, "instance");
from((short) 0, (Object) instance);
return this;
}
private void from(short _unused, Object object) {
long bits = 0;
if (object instanceof HasActionId) {
HasActionId instance = (HasActionId) object;
if ((bits & 0x1L) == 0) {
this.setActionId(instance.getActionId());
bits |= 0x1L;
}
}
if (object instanceof UserSelectMenuIF) {
UserSelectMenuIF instance = (UserSelectMenuIF) object;
Optional initialUserIdOptional = instance.getInitialUserId();
if (initialUserIdOptional.isPresent()) {
setInitialUserId(initialUserIdOptional);
}
if ((bits & 0x1L) == 0) {
this.setActionId(instance.getActionId());
bits |= 0x1L;
}
Optional confirmationDialogOptional = instance.getConfirmationDialog();
if (confirmationDialogOptional.isPresent()) {
setConfirmationDialog(confirmationDialogOptional);
}
this.setPlaceholder(instance.getPlaceholder());
}
}
/**
* Initializes the value for the {@link UserSelectMenuIF#getPlaceholder() placeholder} attribute.
* @param placeholder The value for placeholder
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setPlaceholder(Text placeholder) {
this.placeholder = Objects.requireNonNull(placeholder, "placeholder");
initBits &= ~INIT_BIT_PLACEHOLDER;
return this;
}
/**
* Initializes the value for the {@link UserSelectMenuIF#getActionId() actionId} attribute.
* @param actionId The value for actionId
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setActionId(String actionId) {
this.actionId = Objects.requireNonNull(actionId, "actionId");
initBits &= ~INIT_BIT_ACTION_ID;
return this;
}
/**
* Initializes the optional value {@link UserSelectMenuIF#getInitialUserId() initialUserId} to initialUserId.
* @param initialUserId The value for initialUserId, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
public final Builder setInitialUserId(@Nullable String initialUserId) {
this.initialUserId = initialUserId;
return this;
}
/**
* Initializes the optional value {@link UserSelectMenuIF#getInitialUserId() initialUserId} to initialUserId.
* @param initialUserId The value for initialUserId
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setInitialUserId(Optional initialUserId) {
this.initialUserId = initialUserId.orElse(null);
return this;
}
/**
* Initializes the optional value {@link UserSelectMenuIF#getConfirmationDialog() confirmationDialog} to confirmationDialog.
* @param confirmationDialog The value for confirmationDialog, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
public final Builder setConfirmationDialog(@Nullable ConfirmationDialog confirmationDialog) {
this.confirmationDialog = confirmationDialog;
return this;
}
/**
* Initializes the optional value {@link UserSelectMenuIF#getConfirmationDialog() confirmationDialog} to confirmationDialog.
* @param confirmationDialog The value for confirmationDialog
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setConfirmationDialog(Optional confirmationDialog) {
this.confirmationDialog = confirmationDialog.orElse(null);
return this;
}
/**
* Builds a new {@link UserSelectMenu UserSelectMenu}.
* @return An immutable instance of UserSelectMenu
* @throws com.hubspot.immutables.validation.InvalidImmutableStateException if any required attributes are missing
*/
public UserSelectMenu build() {
checkRequiredAttributes();
return new UserSelectMenu(placeholder, actionId, initialUserId, confirmationDialog);
}
private boolean placeholderIsSet() {
return (initBits & INIT_BIT_PLACEHOLDER) == 0;
}
private boolean actionIdIsSet() {
return (initBits & INIT_BIT_ACTION_ID) == 0;
}
private void checkRequiredAttributes() {
if (initBits != 0) {
throw new InvalidImmutableStateException(formatRequiredAttributesMessage());
}
}
private String formatRequiredAttributesMessage() {
List attributes = new ArrayList<>();
if (!placeholderIsSet()) attributes.add("placeholder");
if (!actionIdIsSet()) attributes.add("actionId");
return "Cannot build UserSelectMenu, some of required attributes are not set " + attributes;
}
}
}