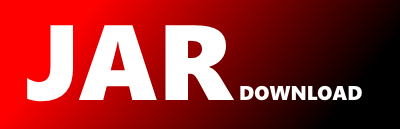
com.hubspot.slack.client.models.blocks.objects.ConfirmationDialog Maven / Gradle / Ivy
package com.hubspot.slack.client.models.blocks.objects;
import com.fasterxml.jackson.annotation.JsonAutoDetect;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.hubspot.immutables.validation.InvalidImmutableStateException;
import com.hubspot.slack.client.models.blocks.Style;
import edu.umd.cs.findbugs.annotations.SuppressFBWarnings;
import java.util.ArrayList;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
import javax.annotation.ParametersAreNonnullByDefault;
import javax.annotation.concurrent.Immutable;
import javax.annotation.concurrent.NotThreadSafe;
import org.immutables.value.Generated;
/**
* Immutable implementation of {@link ConfirmationDialogIF}.
*
* Use the builder to create immutable instances:
* {@code ConfirmationDialog.builder()}.
* Use the static factory method to create immutable instances:
* {@code ConfirmationDialog.of()}.
*/
@Generated(from = "ConfirmationDialogIF", generator = "Immutables")
@SuppressWarnings({"all"})
@SuppressFBWarnings
@ParametersAreNonnullByDefault
@javax.annotation.processing.Generated("org.immutables.processor.ProxyProcessor")
@Immutable
public final class ConfirmationDialog
implements ConfirmationDialogIF {
private final Text title;
private final Text text;
private final Text confirmButtonText;
private final Text denyButtonText;
private final @Nullable Style style;
private ConfirmationDialog(
Text title,
Text text,
Text confirmButtonText,
Text denyButtonText) {
this.title = Objects.requireNonNull(title, "title");
this.text = Objects.requireNonNull(text, "text");
this.confirmButtonText = Objects.requireNonNull(confirmButtonText, "confirmButtonText");
this.denyButtonText = Objects.requireNonNull(denyButtonText, "denyButtonText");
this.style = null;
}
private ConfirmationDialog(
Text title,
Text text,
Text confirmButtonText,
Text denyButtonText,
@Nullable Style style) {
this.title = title;
this.text = text;
this.confirmButtonText = confirmButtonText;
this.denyButtonText = denyButtonText;
this.style = style;
}
/**
* @return The value of the {@code title} attribute
*/
@JsonProperty
@Override
public Text getTitle() {
return title;
}
/**
* @return The value of the {@code text} attribute
*/
@JsonProperty
@Override
public Text getText() {
return text;
}
/**
* @return The value of the {@code confirmButtonText} attribute
*/
@JsonProperty("confirm")
@Override
public Text getConfirmButtonText() {
return confirmButtonText;
}
/**
* @return The value of the {@code denyButtonText} attribute
*/
@JsonProperty("deny")
@Override
public Text getDenyButtonText() {
return denyButtonText;
}
/**
* @return The value of the {@code style} attribute
*/
@JsonProperty
@Override
public Optional