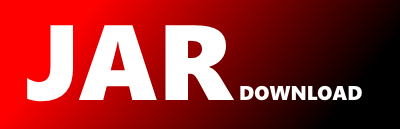
com.hubspot.slack.client.models.bookmarks.Bookmark Maven / Gradle / Ivy
package com.hubspot.slack.client.models.bookmarks;
import com.fasterxml.jackson.annotation.JsonAutoDetect;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.hubspot.immutables.validation.InvalidImmutableStateException;
import edu.umd.cs.findbugs.annotations.SuppressFBWarnings;
import java.time.Instant;
import java.util.ArrayList;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
import javax.annotation.ParametersAreNonnullByDefault;
import javax.annotation.concurrent.Immutable;
import javax.annotation.concurrent.NotThreadSafe;
import org.immutables.value.Generated;
/**
* Immutable implementation of {@link BookmarkIF}.
*
* Use the builder to create immutable instances:
* {@code Bookmark.builder()}.
*/
@Generated(from = "BookmarkIF", generator = "Immutables")
@SuppressWarnings({"all"})
@SuppressFBWarnings
@ParametersAreNonnullByDefault
@javax.annotation.processing.Generated("org.immutables.processor.ProxyProcessor")
@Immutable
public final class Bookmark implements BookmarkIF {
private final BookmarkType type;
private final String id;
private final String channelId;
private final String title;
private final @Nullable String link;
private final @Nullable String emoji;
private final @Nullable String iconUrl;
private final @Nullable String entityId;
private final long dateCreatedEpochSeconds;
private transient final Instant createdAt;
private final long dateUpdatedEpochSeconds;
private transient final Instant updatedAt;
private final @Nullable String rank;
private final @Nullable String lastUpdatedByUserId;
private final @Nullable String lastUpdatedByTeamId;
private final @Nullable String shortcutId;
private final @Nullable String appId;
private Bookmark(
BookmarkType type,
String id,
String channelId,
String title,
@Nullable String link,
@Nullable String emoji,
@Nullable String iconUrl,
@Nullable String entityId,
long dateCreatedEpochSeconds,
long dateUpdatedEpochSeconds,
@Nullable String rank,
@Nullable String lastUpdatedByUserId,
@Nullable String lastUpdatedByTeamId,
@Nullable String shortcutId,
@Nullable String appId) {
this.type = type;
this.id = id;
this.channelId = channelId;
this.title = title;
this.link = link;
this.emoji = emoji;
this.iconUrl = iconUrl;
this.entityId = entityId;
this.dateCreatedEpochSeconds = dateCreatedEpochSeconds;
this.dateUpdatedEpochSeconds = dateUpdatedEpochSeconds;
this.rank = rank;
this.lastUpdatedByUserId = lastUpdatedByUserId;
this.lastUpdatedByTeamId = lastUpdatedByTeamId;
this.shortcutId = shortcutId;
this.appId = appId;
this.createdAt = initShim.getCreatedAt();
this.updatedAt = initShim.getUpdatedAt();
this.initShim = null;
}
private static final byte STAGE_INITIALIZING = -1;
private static final byte STAGE_UNINITIALIZED = 0;
private static final byte STAGE_INITIALIZED = 1;
private transient volatile InitShim initShim = new InitShim();
@Generated(from = "BookmarkIF", generator = "Immutables")
private final class InitShim {
private byte createdAtBuildStage = STAGE_UNINITIALIZED;
private Instant createdAt;
Instant getCreatedAt() {
if (createdAtBuildStage == STAGE_INITIALIZING) throw new InvalidImmutableStateException(formatInitCycleMessage());
if (createdAtBuildStage == STAGE_UNINITIALIZED) {
createdAtBuildStage = STAGE_INITIALIZING;
this.createdAt = Objects.requireNonNull(getCreatedAtInitialize(), "createdAt");
createdAtBuildStage = STAGE_INITIALIZED;
}
return this.createdAt;
}
private byte updatedAtBuildStage = STAGE_UNINITIALIZED;
private Instant updatedAt;
Instant getUpdatedAt() {
if (updatedAtBuildStage == STAGE_INITIALIZING) throw new InvalidImmutableStateException(formatInitCycleMessage());
if (updatedAtBuildStage == STAGE_UNINITIALIZED) {
updatedAtBuildStage = STAGE_INITIALIZING;
this.updatedAt = Objects.requireNonNull(getUpdatedAtInitialize(), "updatedAt");
updatedAtBuildStage = STAGE_INITIALIZED;
}
return this.updatedAt;
}
private String formatInitCycleMessage() {
List attributes = new ArrayList<>();
if (createdAtBuildStage == STAGE_INITIALIZING) attributes.add("createdAt");
if (updatedAtBuildStage == STAGE_INITIALIZING) attributes.add("updatedAt");
return "Cannot build Bookmark, attribute initializers form cycle " + attributes;
}
}
private Instant getCreatedAtInitialize() {
return BookmarkIF.super.getCreatedAt();
}
private Instant getUpdatedAtInitialize() {
return BookmarkIF.super.getUpdatedAt();
}
/**
* @return The value of the {@code type} attribute
*/
@JsonProperty
@Override
public BookmarkType getType() {
return type;
}
/**
* @return The value of the {@code id} attribute
*/
@JsonProperty
@Override
public String getId() {
return id;
}
/**
* @return The value of the {@code channelId} attribute
*/
@JsonProperty
@Override
public String getChannelId() {
return channelId;
}
/**
* @return The value of the {@code title} attribute
*/
@JsonProperty
@Override
public String getTitle() {
return title;
}
/**
* @return The value of the {@code link} attribute
*/
@JsonProperty
@Override
public Optional getLink() {
return Optional.ofNullable(link);
}
/**
* @return The value of the {@code emoji} attribute
*/
@JsonProperty
@Override
public Optional getEmoji() {
return Optional.ofNullable(emoji);
}
/**
* @return The value of the {@code iconUrl} attribute
*/
@JsonProperty
@Override
public Optional getIconUrl() {
return Optional.ofNullable(iconUrl);
}
/**
* @return The value of the {@code entityId} attribute
*/
@JsonProperty
@Override
public Optional getEntityId() {
return Optional.ofNullable(entityId);
}
/**
* @return The value of the {@code dateCreatedEpochSeconds} attribute
*/
@JsonProperty("date_created")
@Override
public long getDateCreatedEpochSeconds() {
return dateCreatedEpochSeconds;
}
/**
* @return The computed-at-construction value of the {@code createdAt} attribute
*/
@JsonProperty
@JsonIgnore
@Override
public Instant getCreatedAt() {
InitShim shim = this.initShim;
return shim != null
? shim.getCreatedAt()
: this.createdAt;
}
/**
* @return The value of the {@code dateUpdatedEpochSeconds} attribute
*/
@JsonProperty("date_updated")
@Override
public long getDateUpdatedEpochSeconds() {
return dateUpdatedEpochSeconds;
}
/**
* @return The computed-at-construction value of the {@code updatedAt} attribute
*/
@JsonProperty
@JsonIgnore
@Override
public Instant getUpdatedAt() {
InitShim shim = this.initShim;
return shim != null
? shim.getUpdatedAt()
: this.updatedAt;
}
/**
* @return The value of the {@code rank} attribute
*/
@JsonProperty
@Override
public Optional getRank() {
return Optional.ofNullable(rank);
}
/**
* @return The value of the {@code lastUpdatedByUserId} attribute
*/
@JsonProperty
@Override
public Optional getLastUpdatedByUserId() {
return Optional.ofNullable(lastUpdatedByUserId);
}
/**
* @return The value of the {@code lastUpdatedByTeamId} attribute
*/
@JsonProperty
@Override
public Optional getLastUpdatedByTeamId() {
return Optional.ofNullable(lastUpdatedByTeamId);
}
/**
* @return The value of the {@code shortcutId} attribute
*/
@JsonProperty
@Override
public Optional getShortcutId() {
return Optional.ofNullable(shortcutId);
}
/**
* @return The value of the {@code appId} attribute
*/
@JsonProperty
@Override
public Optional getAppId() {
return Optional.ofNullable(appId);
}
/**
* Copy the current immutable object by setting a value for the {@link BookmarkIF#getType() type} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for type
* @return A modified copy of the {@code this} object
*/
public final Bookmark withType(BookmarkType value) {
BookmarkType newValue = Objects.requireNonNull(value, "type");
if (this.type == newValue) return this;
return new Bookmark(
newValue,
this.id,
this.channelId,
this.title,
this.link,
this.emoji,
this.iconUrl,
this.entityId,
this.dateCreatedEpochSeconds,
this.dateUpdatedEpochSeconds,
this.rank,
this.lastUpdatedByUserId,
this.lastUpdatedByTeamId,
this.shortcutId,
this.appId);
}
/**
* Copy the current immutable object by setting a value for the {@link BookmarkIF#getId() id} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for id
* @return A modified copy of the {@code this} object
*/
public final Bookmark withId(String value) {
String newValue = Objects.requireNonNull(value, "id");
if (this.id.equals(newValue)) return this;
return new Bookmark(
this.type,
newValue,
this.channelId,
this.title,
this.link,
this.emoji,
this.iconUrl,
this.entityId,
this.dateCreatedEpochSeconds,
this.dateUpdatedEpochSeconds,
this.rank,
this.lastUpdatedByUserId,
this.lastUpdatedByTeamId,
this.shortcutId,
this.appId);
}
/**
* Copy the current immutable object by setting a value for the {@link BookmarkIF#getChannelId() channelId} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for channelId
* @return A modified copy of the {@code this} object
*/
public final Bookmark withChannelId(String value) {
String newValue = Objects.requireNonNull(value, "channelId");
if (this.channelId.equals(newValue)) return this;
return new Bookmark(
this.type,
this.id,
newValue,
this.title,
this.link,
this.emoji,
this.iconUrl,
this.entityId,
this.dateCreatedEpochSeconds,
this.dateUpdatedEpochSeconds,
this.rank,
this.lastUpdatedByUserId,
this.lastUpdatedByTeamId,
this.shortcutId,
this.appId);
}
/**
* Copy the current immutable object by setting a value for the {@link BookmarkIF#getTitle() title} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for title
* @return A modified copy of the {@code this} object
*/
public final Bookmark withTitle(String value) {
String newValue = Objects.requireNonNull(value, "title");
if (this.title.equals(newValue)) return this;
return new Bookmark(
this.type,
this.id,
this.channelId,
newValue,
this.link,
this.emoji,
this.iconUrl,
this.entityId,
this.dateCreatedEpochSeconds,
this.dateUpdatedEpochSeconds,
this.rank,
this.lastUpdatedByUserId,
this.lastUpdatedByTeamId,
this.shortcutId,
this.appId);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link BookmarkIF#getLink() link} attribute.
* @param value The value for link, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final Bookmark withLink(@Nullable String value) {
@Nullable String newValue = value;
if (Objects.equals(this.link, newValue)) return this;
return new Bookmark(
this.type,
this.id,
this.channelId,
this.title,
newValue,
this.emoji,
this.iconUrl,
this.entityId,
this.dateCreatedEpochSeconds,
this.dateUpdatedEpochSeconds,
this.rank,
this.lastUpdatedByUserId,
this.lastUpdatedByTeamId,
this.shortcutId,
this.appId);
}
/**
* Copy the current immutable object by setting an optional value for the {@link BookmarkIF#getLink() link} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for link
* @return A modified copy of {@code this} object
*/
public final Bookmark withLink(Optional optional) {
@Nullable String value = optional.orElse(null);
if (Objects.equals(this.link, value)) return this;
return new Bookmark(
this.type,
this.id,
this.channelId,
this.title,
value,
this.emoji,
this.iconUrl,
this.entityId,
this.dateCreatedEpochSeconds,
this.dateUpdatedEpochSeconds,
this.rank,
this.lastUpdatedByUserId,
this.lastUpdatedByTeamId,
this.shortcutId,
this.appId);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link BookmarkIF#getEmoji() emoji} attribute.
* @param value The value for emoji, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final Bookmark withEmoji(@Nullable String value) {
@Nullable String newValue = value;
if (Objects.equals(this.emoji, newValue)) return this;
return new Bookmark(
this.type,
this.id,
this.channelId,
this.title,
this.link,
newValue,
this.iconUrl,
this.entityId,
this.dateCreatedEpochSeconds,
this.dateUpdatedEpochSeconds,
this.rank,
this.lastUpdatedByUserId,
this.lastUpdatedByTeamId,
this.shortcutId,
this.appId);
}
/**
* Copy the current immutable object by setting an optional value for the {@link BookmarkIF#getEmoji() emoji} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for emoji
* @return A modified copy of {@code this} object
*/
public final Bookmark withEmoji(Optional optional) {
@Nullable String value = optional.orElse(null);
if (Objects.equals(this.emoji, value)) return this;
return new Bookmark(
this.type,
this.id,
this.channelId,
this.title,
this.link,
value,
this.iconUrl,
this.entityId,
this.dateCreatedEpochSeconds,
this.dateUpdatedEpochSeconds,
this.rank,
this.lastUpdatedByUserId,
this.lastUpdatedByTeamId,
this.shortcutId,
this.appId);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link BookmarkIF#getIconUrl() iconUrl} attribute.
* @param value The value for iconUrl, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final Bookmark withIconUrl(@Nullable String value) {
@Nullable String newValue = value;
if (Objects.equals(this.iconUrl, newValue)) return this;
return new Bookmark(
this.type,
this.id,
this.channelId,
this.title,
this.link,
this.emoji,
newValue,
this.entityId,
this.dateCreatedEpochSeconds,
this.dateUpdatedEpochSeconds,
this.rank,
this.lastUpdatedByUserId,
this.lastUpdatedByTeamId,
this.shortcutId,
this.appId);
}
/**
* Copy the current immutable object by setting an optional value for the {@link BookmarkIF#getIconUrl() iconUrl} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for iconUrl
* @return A modified copy of {@code this} object
*/
public final Bookmark withIconUrl(Optional optional) {
@Nullable String value = optional.orElse(null);
if (Objects.equals(this.iconUrl, value)) return this;
return new Bookmark(
this.type,
this.id,
this.channelId,
this.title,
this.link,
this.emoji,
value,
this.entityId,
this.dateCreatedEpochSeconds,
this.dateUpdatedEpochSeconds,
this.rank,
this.lastUpdatedByUserId,
this.lastUpdatedByTeamId,
this.shortcutId,
this.appId);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link BookmarkIF#getEntityId() entityId} attribute.
* @param value The value for entityId, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final Bookmark withEntityId(@Nullable String value) {
@Nullable String newValue = value;
if (Objects.equals(this.entityId, newValue)) return this;
return new Bookmark(
this.type,
this.id,
this.channelId,
this.title,
this.link,
this.emoji,
this.iconUrl,
newValue,
this.dateCreatedEpochSeconds,
this.dateUpdatedEpochSeconds,
this.rank,
this.lastUpdatedByUserId,
this.lastUpdatedByTeamId,
this.shortcutId,
this.appId);
}
/**
* Copy the current immutable object by setting an optional value for the {@link BookmarkIF#getEntityId() entityId} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for entityId
* @return A modified copy of {@code this} object
*/
public final Bookmark withEntityId(Optional optional) {
@Nullable String value = optional.orElse(null);
if (Objects.equals(this.entityId, value)) return this;
return new Bookmark(
this.type,
this.id,
this.channelId,
this.title,
this.link,
this.emoji,
this.iconUrl,
value,
this.dateCreatedEpochSeconds,
this.dateUpdatedEpochSeconds,
this.rank,
this.lastUpdatedByUserId,
this.lastUpdatedByTeamId,
this.shortcutId,
this.appId);
}
/**
* Copy the current immutable object by setting a value for the {@link BookmarkIF#getDateCreatedEpochSeconds() dateCreatedEpochSeconds} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for dateCreatedEpochSeconds
* @return A modified copy of the {@code this} object
*/
public final Bookmark withDateCreatedEpochSeconds(long value) {
if (this.dateCreatedEpochSeconds == value) return this;
return new Bookmark(
this.type,
this.id,
this.channelId,
this.title,
this.link,
this.emoji,
this.iconUrl,
this.entityId,
value,
this.dateUpdatedEpochSeconds,
this.rank,
this.lastUpdatedByUserId,
this.lastUpdatedByTeamId,
this.shortcutId,
this.appId);
}
/**
* Copy the current immutable object by setting a value for the {@link BookmarkIF#getDateUpdatedEpochSeconds() dateUpdatedEpochSeconds} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for dateUpdatedEpochSeconds
* @return A modified copy of the {@code this} object
*/
public final Bookmark withDateUpdatedEpochSeconds(long value) {
if (this.dateUpdatedEpochSeconds == value) return this;
return new Bookmark(
this.type,
this.id,
this.channelId,
this.title,
this.link,
this.emoji,
this.iconUrl,
this.entityId,
this.dateCreatedEpochSeconds,
value,
this.rank,
this.lastUpdatedByUserId,
this.lastUpdatedByTeamId,
this.shortcutId,
this.appId);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link BookmarkIF#getRank() rank} attribute.
* @param value The value for rank, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final Bookmark withRank(@Nullable String value) {
@Nullable String newValue = value;
if (Objects.equals(this.rank, newValue)) return this;
return new Bookmark(
this.type,
this.id,
this.channelId,
this.title,
this.link,
this.emoji,
this.iconUrl,
this.entityId,
this.dateCreatedEpochSeconds,
this.dateUpdatedEpochSeconds,
newValue,
this.lastUpdatedByUserId,
this.lastUpdatedByTeamId,
this.shortcutId,
this.appId);
}
/**
* Copy the current immutable object by setting an optional value for the {@link BookmarkIF#getRank() rank} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for rank
* @return A modified copy of {@code this} object
*/
public final Bookmark withRank(Optional optional) {
@Nullable String value = optional.orElse(null);
if (Objects.equals(this.rank, value)) return this;
return new Bookmark(
this.type,
this.id,
this.channelId,
this.title,
this.link,
this.emoji,
this.iconUrl,
this.entityId,
this.dateCreatedEpochSeconds,
this.dateUpdatedEpochSeconds,
value,
this.lastUpdatedByUserId,
this.lastUpdatedByTeamId,
this.shortcutId,
this.appId);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link BookmarkIF#getLastUpdatedByUserId() lastUpdatedByUserId} attribute.
* @param value The value for lastUpdatedByUserId, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final Bookmark withLastUpdatedByUserId(@Nullable String value) {
@Nullable String newValue = value;
if (Objects.equals(this.lastUpdatedByUserId, newValue)) return this;
return new Bookmark(
this.type,
this.id,
this.channelId,
this.title,
this.link,
this.emoji,
this.iconUrl,
this.entityId,
this.dateCreatedEpochSeconds,
this.dateUpdatedEpochSeconds,
this.rank,
newValue,
this.lastUpdatedByTeamId,
this.shortcutId,
this.appId);
}
/**
* Copy the current immutable object by setting an optional value for the {@link BookmarkIF#getLastUpdatedByUserId() lastUpdatedByUserId} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for lastUpdatedByUserId
* @return A modified copy of {@code this} object
*/
public final Bookmark withLastUpdatedByUserId(Optional optional) {
@Nullable String value = optional.orElse(null);
if (Objects.equals(this.lastUpdatedByUserId, value)) return this;
return new Bookmark(
this.type,
this.id,
this.channelId,
this.title,
this.link,
this.emoji,
this.iconUrl,
this.entityId,
this.dateCreatedEpochSeconds,
this.dateUpdatedEpochSeconds,
this.rank,
value,
this.lastUpdatedByTeamId,
this.shortcutId,
this.appId);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link BookmarkIF#getLastUpdatedByTeamId() lastUpdatedByTeamId} attribute.
* @param value The value for lastUpdatedByTeamId, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final Bookmark withLastUpdatedByTeamId(@Nullable String value) {
@Nullable String newValue = value;
if (Objects.equals(this.lastUpdatedByTeamId, newValue)) return this;
return new Bookmark(
this.type,
this.id,
this.channelId,
this.title,
this.link,
this.emoji,
this.iconUrl,
this.entityId,
this.dateCreatedEpochSeconds,
this.dateUpdatedEpochSeconds,
this.rank,
this.lastUpdatedByUserId,
newValue,
this.shortcutId,
this.appId);
}
/**
* Copy the current immutable object by setting an optional value for the {@link BookmarkIF#getLastUpdatedByTeamId() lastUpdatedByTeamId} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for lastUpdatedByTeamId
* @return A modified copy of {@code this} object
*/
public final Bookmark withLastUpdatedByTeamId(Optional optional) {
@Nullable String value = optional.orElse(null);
if (Objects.equals(this.lastUpdatedByTeamId, value)) return this;
return new Bookmark(
this.type,
this.id,
this.channelId,
this.title,
this.link,
this.emoji,
this.iconUrl,
this.entityId,
this.dateCreatedEpochSeconds,
this.dateUpdatedEpochSeconds,
this.rank,
this.lastUpdatedByUserId,
value,
this.shortcutId,
this.appId);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link BookmarkIF#getShortcutId() shortcutId} attribute.
* @param value The value for shortcutId, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final Bookmark withShortcutId(@Nullable String value) {
@Nullable String newValue = value;
if (Objects.equals(this.shortcutId, newValue)) return this;
return new Bookmark(
this.type,
this.id,
this.channelId,
this.title,
this.link,
this.emoji,
this.iconUrl,
this.entityId,
this.dateCreatedEpochSeconds,
this.dateUpdatedEpochSeconds,
this.rank,
this.lastUpdatedByUserId,
this.lastUpdatedByTeamId,
newValue,
this.appId);
}
/**
* Copy the current immutable object by setting an optional value for the {@link BookmarkIF#getShortcutId() shortcutId} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for shortcutId
* @return A modified copy of {@code this} object
*/
public final Bookmark withShortcutId(Optional optional) {
@Nullable String value = optional.orElse(null);
if (Objects.equals(this.shortcutId, value)) return this;
return new Bookmark(
this.type,
this.id,
this.channelId,
this.title,
this.link,
this.emoji,
this.iconUrl,
this.entityId,
this.dateCreatedEpochSeconds,
this.dateUpdatedEpochSeconds,
this.rank,
this.lastUpdatedByUserId,
this.lastUpdatedByTeamId,
value,
this.appId);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link BookmarkIF#getAppId() appId} attribute.
* @param value The value for appId, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final Bookmark withAppId(@Nullable String value) {
@Nullable String newValue = value;
if (Objects.equals(this.appId, newValue)) return this;
return new Bookmark(
this.type,
this.id,
this.channelId,
this.title,
this.link,
this.emoji,
this.iconUrl,
this.entityId,
this.dateCreatedEpochSeconds,
this.dateUpdatedEpochSeconds,
this.rank,
this.lastUpdatedByUserId,
this.lastUpdatedByTeamId,
this.shortcutId,
newValue);
}
/**
* Copy the current immutable object by setting an optional value for the {@link BookmarkIF#getAppId() appId} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for appId
* @return A modified copy of {@code this} object
*/
public final Bookmark withAppId(Optional optional) {
@Nullable String value = optional.orElse(null);
if (Objects.equals(this.appId, value)) return this;
return new Bookmark(
this.type,
this.id,
this.channelId,
this.title,
this.link,
this.emoji,
this.iconUrl,
this.entityId,
this.dateCreatedEpochSeconds,
this.dateUpdatedEpochSeconds,
this.rank,
this.lastUpdatedByUserId,
this.lastUpdatedByTeamId,
this.shortcutId,
value);
}
/**
* This instance is equal to all instances of {@code Bookmark} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(@Nullable Object another) {
if (this == another) return true;
return another instanceof Bookmark
&& equalTo(0, (Bookmark) another);
}
private boolean equalTo(int synthetic, Bookmark another) {
return type.equals(another.type)
&& id.equals(another.id)
&& channelId.equals(another.channelId)
&& title.equals(another.title)
&& Objects.equals(link, another.link)
&& Objects.equals(emoji, another.emoji)
&& Objects.equals(iconUrl, another.iconUrl)
&& Objects.equals(entityId, another.entityId)
&& dateCreatedEpochSeconds == another.dateCreatedEpochSeconds
&& createdAt.equals(another.createdAt)
&& dateUpdatedEpochSeconds == another.dateUpdatedEpochSeconds
&& updatedAt.equals(another.updatedAt)
&& Objects.equals(rank, another.rank)
&& Objects.equals(lastUpdatedByUserId, another.lastUpdatedByUserId)
&& Objects.equals(lastUpdatedByTeamId, another.lastUpdatedByTeamId)
&& Objects.equals(shortcutId, another.shortcutId)
&& Objects.equals(appId, another.appId);
}
/**
* Computes a hash code from attributes: {@code type}, {@code id}, {@code channelId}, {@code title}, {@code link}, {@code emoji}, {@code iconUrl}, {@code entityId}, {@code dateCreatedEpochSeconds}, {@code createdAt}, {@code dateUpdatedEpochSeconds}, {@code updatedAt}, {@code rank}, {@code lastUpdatedByUserId}, {@code lastUpdatedByTeamId}, {@code shortcutId}, {@code appId}.
* @return hashCode value
*/
@Override
public int hashCode() {
int h = 5381;
h += (h << 5) + type.hashCode();
h += (h << 5) + id.hashCode();
h += (h << 5) + channelId.hashCode();
h += (h << 5) + title.hashCode();
h += (h << 5) + Objects.hashCode(link);
h += (h << 5) + Objects.hashCode(emoji);
h += (h << 5) + Objects.hashCode(iconUrl);
h += (h << 5) + Objects.hashCode(entityId);
h += (h << 5) + Long.hashCode(dateCreatedEpochSeconds);
h += (h << 5) + createdAt.hashCode();
h += (h << 5) + Long.hashCode(dateUpdatedEpochSeconds);
h += (h << 5) + updatedAt.hashCode();
h += (h << 5) + Objects.hashCode(rank);
h += (h << 5) + Objects.hashCode(lastUpdatedByUserId);
h += (h << 5) + Objects.hashCode(lastUpdatedByTeamId);
h += (h << 5) + Objects.hashCode(shortcutId);
h += (h << 5) + Objects.hashCode(appId);
return h;
}
/**
* Prints the immutable value {@code Bookmark} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
StringBuilder builder = new StringBuilder("Bookmark{");
builder.append("type=").append(type);
builder.append(", ");
builder.append("id=").append(id);
builder.append(", ");
builder.append("channelId=").append(channelId);
builder.append(", ");
builder.append("title=").append(title);
if (link != null) {
builder.append(", ");
builder.append("link=").append(link);
}
if (emoji != null) {
builder.append(", ");
builder.append("emoji=").append(emoji);
}
if (iconUrl != null) {
builder.append(", ");
builder.append("iconUrl=").append(iconUrl);
}
if (entityId != null) {
builder.append(", ");
builder.append("entityId=").append(entityId);
}
builder.append(", ");
builder.append("dateCreatedEpochSeconds=").append(dateCreatedEpochSeconds);
builder.append(", ");
builder.append("createdAt=").append(createdAt);
builder.append(", ");
builder.append("dateUpdatedEpochSeconds=").append(dateUpdatedEpochSeconds);
builder.append(", ");
builder.append("updatedAt=").append(updatedAt);
if (rank != null) {
builder.append(", ");
builder.append("rank=").append(rank);
}
if (lastUpdatedByUserId != null) {
builder.append(", ");
builder.append("lastUpdatedByUserId=").append(lastUpdatedByUserId);
}
if (lastUpdatedByTeamId != null) {
builder.append(", ");
builder.append("lastUpdatedByTeamId=").append(lastUpdatedByTeamId);
}
if (shortcutId != null) {
builder.append(", ");
builder.append("shortcutId=").append(shortcutId);
}
if (appId != null) {
builder.append(", ");
builder.append("appId=").append(appId);
}
return builder.append("}").toString();
}
/**
* Utility type used to correctly read immutable object from JSON representation.
* @deprecated Do not use this type directly, it exists only for the Jackson-binding infrastructure
*/
@Generated(from = "BookmarkIF", generator = "Immutables")
@Deprecated
@JsonAutoDetect(fieldVisibility = JsonAutoDetect.Visibility.NONE)
static final class Json implements BookmarkIF {
@Nullable BookmarkType type;
@Nullable String id;
@Nullable String channelId;
@Nullable String title;
@Nullable Optional link = Optional.empty();
@Nullable Optional emoji = Optional.empty();
@Nullable Optional iconUrl = Optional.empty();
@Nullable Optional entityId = Optional.empty();
long dateCreatedEpochSeconds;
boolean dateCreatedEpochSecondsIsSet;
long dateUpdatedEpochSeconds;
boolean dateUpdatedEpochSecondsIsSet;
@Nullable Optional rank = Optional.empty();
@Nullable Optional lastUpdatedByUserId = Optional.empty();
@Nullable Optional lastUpdatedByTeamId = Optional.empty();
@Nullable Optional shortcutId = Optional.empty();
@Nullable Optional appId = Optional.empty();
@JsonProperty
public void setType(BookmarkType type) {
this.type = type;
}
@JsonProperty
public void setId(String id) {
this.id = id;
}
@JsonProperty
public void setChannelId(String channelId) {
this.channelId = channelId;
}
@JsonProperty
public void setTitle(String title) {
this.title = title;
}
@JsonProperty
public void setLink(Optional link) {
this.link = link;
}
@JsonProperty
public void setEmoji(Optional emoji) {
this.emoji = emoji;
}
@JsonProperty
public void setIconUrl(Optional iconUrl) {
this.iconUrl = iconUrl;
}
@JsonProperty
public void setEntityId(Optional entityId) {
this.entityId = entityId;
}
@JsonProperty("date_created")
public void setDateCreatedEpochSeconds(long dateCreatedEpochSeconds) {
this.dateCreatedEpochSeconds = dateCreatedEpochSeconds;
this.dateCreatedEpochSecondsIsSet = true;
}
@JsonProperty("date_updated")
public void setDateUpdatedEpochSeconds(long dateUpdatedEpochSeconds) {
this.dateUpdatedEpochSeconds = dateUpdatedEpochSeconds;
this.dateUpdatedEpochSecondsIsSet = true;
}
@JsonProperty
public void setRank(Optional rank) {
this.rank = rank;
}
@JsonProperty
public void setLastUpdatedByUserId(Optional lastUpdatedByUserId) {
this.lastUpdatedByUserId = lastUpdatedByUserId;
}
@JsonProperty
public void setLastUpdatedByTeamId(Optional lastUpdatedByTeamId) {
this.lastUpdatedByTeamId = lastUpdatedByTeamId;
}
@JsonProperty
public void setShortcutId(Optional shortcutId) {
this.shortcutId = shortcutId;
}
@JsonProperty
public void setAppId(Optional appId) {
this.appId = appId;
}
@Override
public BookmarkType getType() { throw new UnsupportedOperationException(); }
@Override
public String getId() { throw new UnsupportedOperationException(); }
@Override
public String getChannelId() { throw new UnsupportedOperationException(); }
@Override
public String getTitle() { throw new UnsupportedOperationException(); }
@Override
public Optional getLink() { throw new UnsupportedOperationException(); }
@Override
public Optional getEmoji() { throw new UnsupportedOperationException(); }
@Override
public Optional getIconUrl() { throw new UnsupportedOperationException(); }
@Override
public Optional getEntityId() { throw new UnsupportedOperationException(); }
@Override
public long getDateCreatedEpochSeconds() { throw new UnsupportedOperationException(); }
@JsonIgnore
@Override
public Instant getCreatedAt() { throw new UnsupportedOperationException(); }
@Override
public long getDateUpdatedEpochSeconds() { throw new UnsupportedOperationException(); }
@JsonIgnore
@Override
public Instant getUpdatedAt() { throw new UnsupportedOperationException(); }
@Override
public Optional getRank() { throw new UnsupportedOperationException(); }
@Override
public Optional getLastUpdatedByUserId() { throw new UnsupportedOperationException(); }
@Override
public Optional getLastUpdatedByTeamId() { throw new UnsupportedOperationException(); }
@Override
public Optional getShortcutId() { throw new UnsupportedOperationException(); }
@Override
public Optional getAppId() { throw new UnsupportedOperationException(); }
}
/**
* @param json A JSON-bindable data structure
* @return An immutable value type
* @deprecated Do not use this method directly, it exists only for the Jackson-binding infrastructure
*/
@Deprecated
@JsonCreator(mode = JsonCreator.Mode.DELEGATING)
static Bookmark fromJson(Json json) {
Bookmark.Builder builder = Bookmark.builder();
if (json.type != null) {
builder.setType(json.type);
}
if (json.id != null) {
builder.setId(json.id);
}
if (json.channelId != null) {
builder.setChannelId(json.channelId);
}
if (json.title != null) {
builder.setTitle(json.title);
}
if (json.link != null) {
builder.setLink(json.link);
}
if (json.emoji != null) {
builder.setEmoji(json.emoji);
}
if (json.iconUrl != null) {
builder.setIconUrl(json.iconUrl);
}
if (json.entityId != null) {
builder.setEntityId(json.entityId);
}
if (json.dateCreatedEpochSecondsIsSet) {
builder.setDateCreatedEpochSeconds(json.dateCreatedEpochSeconds);
}
if (json.dateUpdatedEpochSecondsIsSet) {
builder.setDateUpdatedEpochSeconds(json.dateUpdatedEpochSeconds);
}
if (json.rank != null) {
builder.setRank(json.rank);
}
if (json.lastUpdatedByUserId != null) {
builder.setLastUpdatedByUserId(json.lastUpdatedByUserId);
}
if (json.lastUpdatedByTeamId != null) {
builder.setLastUpdatedByTeamId(json.lastUpdatedByTeamId);
}
if (json.shortcutId != null) {
builder.setShortcutId(json.shortcutId);
}
if (json.appId != null) {
builder.setAppId(json.appId);
}
return builder.build();
}
/**
* Creates an immutable copy of a {@link BookmarkIF} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable Bookmark instance
*/
public static Bookmark copyOf(BookmarkIF instance) {
if (instance instanceof Bookmark) {
return (Bookmark) instance;
}
return Bookmark.builder()
.from(instance)
.build();
}
/**
* Creates a builder for {@link Bookmark Bookmark}.
*
* Bookmark.builder()
* .setType(com.hubspot.slack.client.models.bookmarks.BookmarkType) // required {@link BookmarkIF#getType() type}
* .setId(String) // required {@link BookmarkIF#getId() id}
* .setChannelId(String) // required {@link BookmarkIF#getChannelId() channelId}
* .setTitle(String) // required {@link BookmarkIF#getTitle() title}
* .setLink(String) // optional {@link BookmarkIF#getLink() link}
* .setEmoji(String) // optional {@link BookmarkIF#getEmoji() emoji}
* .setIconUrl(String) // optional {@link BookmarkIF#getIconUrl() iconUrl}
* .setEntityId(String) // optional {@link BookmarkIF#getEntityId() entityId}
* .setDateCreatedEpochSeconds(long) // required {@link BookmarkIF#getDateCreatedEpochSeconds() dateCreatedEpochSeconds}
* .setDateUpdatedEpochSeconds(long) // required {@link BookmarkIF#getDateUpdatedEpochSeconds() dateUpdatedEpochSeconds}
* .setRank(String) // optional {@link BookmarkIF#getRank() rank}
* .setLastUpdatedByUserId(String) // optional {@link BookmarkIF#getLastUpdatedByUserId() lastUpdatedByUserId}
* .setLastUpdatedByTeamId(String) // optional {@link BookmarkIF#getLastUpdatedByTeamId() lastUpdatedByTeamId}
* .setShortcutId(String) // optional {@link BookmarkIF#getShortcutId() shortcutId}
* .setAppId(String) // optional {@link BookmarkIF#getAppId() appId}
* .build();
*
* @return A new Bookmark builder
*/
public static Bookmark.Builder builder() {
return new Bookmark.Builder();
}
/**
* Builds instances of type {@link Bookmark Bookmark}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
@Generated(from = "BookmarkIF", generator = "Immutables")
@NotThreadSafe
public static final class Builder {
private static final long INIT_BIT_TYPE = 0x1L;
private static final long INIT_BIT_ID = 0x2L;
private static final long INIT_BIT_CHANNEL_ID = 0x4L;
private static final long INIT_BIT_TITLE = 0x8L;
private static final long INIT_BIT_DATE_CREATED_EPOCH_SECONDS = 0x10L;
private static final long INIT_BIT_DATE_UPDATED_EPOCH_SECONDS = 0x20L;
private long initBits = 0x3fL;
private @Nullable BookmarkType type;
private @Nullable String id;
private @Nullable String channelId;
private @Nullable String title;
private @Nullable String link;
private @Nullable String emoji;
private @Nullable String iconUrl;
private @Nullable String entityId;
private long dateCreatedEpochSeconds;
private long dateUpdatedEpochSeconds;
private @Nullable String rank;
private @Nullable String lastUpdatedByUserId;
private @Nullable String lastUpdatedByTeamId;
private @Nullable String shortcutId;
private @Nullable String appId;
private Builder() {
}
/**
* Fill a builder with attribute values from the provided {@code BookmarkIF} instance.
* Regular attribute values will be replaced with those from the given instance.
* Absent optional values will not replace present values.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(BookmarkIF instance) {
Objects.requireNonNull(instance, "instance");
this.setType(instance.getType());
this.setId(instance.getId());
this.setChannelId(instance.getChannelId());
this.setTitle(instance.getTitle());
Optional linkOptional = instance.getLink();
if (linkOptional.isPresent()) {
setLink(linkOptional);
}
Optional emojiOptional = instance.getEmoji();
if (emojiOptional.isPresent()) {
setEmoji(emojiOptional);
}
Optional iconUrlOptional = instance.getIconUrl();
if (iconUrlOptional.isPresent()) {
setIconUrl(iconUrlOptional);
}
Optional entityIdOptional = instance.getEntityId();
if (entityIdOptional.isPresent()) {
setEntityId(entityIdOptional);
}
this.setDateCreatedEpochSeconds(instance.getDateCreatedEpochSeconds());
this.setDateUpdatedEpochSeconds(instance.getDateUpdatedEpochSeconds());
Optional rankOptional = instance.getRank();
if (rankOptional.isPresent()) {
setRank(rankOptional);
}
Optional lastUpdatedByUserIdOptional = instance.getLastUpdatedByUserId();
if (lastUpdatedByUserIdOptional.isPresent()) {
setLastUpdatedByUserId(lastUpdatedByUserIdOptional);
}
Optional lastUpdatedByTeamIdOptional = instance.getLastUpdatedByTeamId();
if (lastUpdatedByTeamIdOptional.isPresent()) {
setLastUpdatedByTeamId(lastUpdatedByTeamIdOptional);
}
Optional shortcutIdOptional = instance.getShortcutId();
if (shortcutIdOptional.isPresent()) {
setShortcutId(shortcutIdOptional);
}
Optional appIdOptional = instance.getAppId();
if (appIdOptional.isPresent()) {
setAppId(appIdOptional);
}
return this;
}
/**
* Initializes the value for the {@link BookmarkIF#getType() type} attribute.
* @param type The value for type
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setType(BookmarkType type) {
this.type = Objects.requireNonNull(type, "type");
initBits &= ~INIT_BIT_TYPE;
return this;
}
/**
* Initializes the value for the {@link BookmarkIF#getId() id} attribute.
* @param id The value for id
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setId(String id) {
this.id = Objects.requireNonNull(id, "id");
initBits &= ~INIT_BIT_ID;
return this;
}
/**
* Initializes the value for the {@link BookmarkIF#getChannelId() channelId} attribute.
* @param channelId The value for channelId
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setChannelId(String channelId) {
this.channelId = Objects.requireNonNull(channelId, "channelId");
initBits &= ~INIT_BIT_CHANNEL_ID;
return this;
}
/**
* Initializes the value for the {@link BookmarkIF#getTitle() title} attribute.
* @param title The value for title
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setTitle(String title) {
this.title = Objects.requireNonNull(title, "title");
initBits &= ~INIT_BIT_TITLE;
return this;
}
/**
* Initializes the optional value {@link BookmarkIF#getLink() link} to link.
* @param link The value for link, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
public final Builder setLink(@Nullable String link) {
this.link = link;
return this;
}
/**
* Initializes the optional value {@link BookmarkIF#getLink() link} to link.
* @param link The value for link
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setLink(Optional link) {
this.link = link.orElse(null);
return this;
}
/**
* Initializes the optional value {@link BookmarkIF#getEmoji() emoji} to emoji.
* @param emoji The value for emoji, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
public final Builder setEmoji(@Nullable String emoji) {
this.emoji = emoji;
return this;
}
/**
* Initializes the optional value {@link BookmarkIF#getEmoji() emoji} to emoji.
* @param emoji The value for emoji
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setEmoji(Optional emoji) {
this.emoji = emoji.orElse(null);
return this;
}
/**
* Initializes the optional value {@link BookmarkIF#getIconUrl() iconUrl} to iconUrl.
* @param iconUrl The value for iconUrl, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
public final Builder setIconUrl(@Nullable String iconUrl) {
this.iconUrl = iconUrl;
return this;
}
/**
* Initializes the optional value {@link BookmarkIF#getIconUrl() iconUrl} to iconUrl.
* @param iconUrl The value for iconUrl
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setIconUrl(Optional iconUrl) {
this.iconUrl = iconUrl.orElse(null);
return this;
}
/**
* Initializes the optional value {@link BookmarkIF#getEntityId() entityId} to entityId.
* @param entityId The value for entityId, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
public final Builder setEntityId(@Nullable String entityId) {
this.entityId = entityId;
return this;
}
/**
* Initializes the optional value {@link BookmarkIF#getEntityId() entityId} to entityId.
* @param entityId The value for entityId
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setEntityId(Optional entityId) {
this.entityId = entityId.orElse(null);
return this;
}
/**
* Initializes the value for the {@link BookmarkIF#getDateCreatedEpochSeconds() dateCreatedEpochSeconds} attribute.
* @param dateCreatedEpochSeconds The value for dateCreatedEpochSeconds
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setDateCreatedEpochSeconds(long dateCreatedEpochSeconds) {
this.dateCreatedEpochSeconds = dateCreatedEpochSeconds;
initBits &= ~INIT_BIT_DATE_CREATED_EPOCH_SECONDS;
return this;
}
/**
* Initializes the value for the {@link BookmarkIF#getDateUpdatedEpochSeconds() dateUpdatedEpochSeconds} attribute.
* @param dateUpdatedEpochSeconds The value for dateUpdatedEpochSeconds
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setDateUpdatedEpochSeconds(long dateUpdatedEpochSeconds) {
this.dateUpdatedEpochSeconds = dateUpdatedEpochSeconds;
initBits &= ~INIT_BIT_DATE_UPDATED_EPOCH_SECONDS;
return this;
}
/**
* Initializes the optional value {@link BookmarkIF#getRank() rank} to rank.
* @param rank The value for rank, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
public final Builder setRank(@Nullable String rank) {
this.rank = rank;
return this;
}
/**
* Initializes the optional value {@link BookmarkIF#getRank() rank} to rank.
* @param rank The value for rank
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setRank(Optional rank) {
this.rank = rank.orElse(null);
return this;
}
/**
* Initializes the optional value {@link BookmarkIF#getLastUpdatedByUserId() lastUpdatedByUserId} to lastUpdatedByUserId.
* @param lastUpdatedByUserId The value for lastUpdatedByUserId, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
public final Builder setLastUpdatedByUserId(@Nullable String lastUpdatedByUserId) {
this.lastUpdatedByUserId = lastUpdatedByUserId;
return this;
}
/**
* Initializes the optional value {@link BookmarkIF#getLastUpdatedByUserId() lastUpdatedByUserId} to lastUpdatedByUserId.
* @param lastUpdatedByUserId The value for lastUpdatedByUserId
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setLastUpdatedByUserId(Optional lastUpdatedByUserId) {
this.lastUpdatedByUserId = lastUpdatedByUserId.orElse(null);
return this;
}
/**
* Initializes the optional value {@link BookmarkIF#getLastUpdatedByTeamId() lastUpdatedByTeamId} to lastUpdatedByTeamId.
* @param lastUpdatedByTeamId The value for lastUpdatedByTeamId, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
public final Builder setLastUpdatedByTeamId(@Nullable String lastUpdatedByTeamId) {
this.lastUpdatedByTeamId = lastUpdatedByTeamId;
return this;
}
/**
* Initializes the optional value {@link BookmarkIF#getLastUpdatedByTeamId() lastUpdatedByTeamId} to lastUpdatedByTeamId.
* @param lastUpdatedByTeamId The value for lastUpdatedByTeamId
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setLastUpdatedByTeamId(Optional lastUpdatedByTeamId) {
this.lastUpdatedByTeamId = lastUpdatedByTeamId.orElse(null);
return this;
}
/**
* Initializes the optional value {@link BookmarkIF#getShortcutId() shortcutId} to shortcutId.
* @param shortcutId The value for shortcutId, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
public final Builder setShortcutId(@Nullable String shortcutId) {
this.shortcutId = shortcutId;
return this;
}
/**
* Initializes the optional value {@link BookmarkIF#getShortcutId() shortcutId} to shortcutId.
* @param shortcutId The value for shortcutId
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setShortcutId(Optional shortcutId) {
this.shortcutId = shortcutId.orElse(null);
return this;
}
/**
* Initializes the optional value {@link BookmarkIF#getAppId() appId} to appId.
* @param appId The value for appId, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
public final Builder setAppId(@Nullable String appId) {
this.appId = appId;
return this;
}
/**
* Initializes the optional value {@link BookmarkIF#getAppId() appId} to appId.
* @param appId The value for appId
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setAppId(Optional appId) {
this.appId = appId.orElse(null);
return this;
}
/**
* Builds a new {@link Bookmark Bookmark}.
* @return An immutable instance of Bookmark
* @throws com.hubspot.immutables.validation.InvalidImmutableStateException if any required attributes are missing
*/
public Bookmark build() {
checkRequiredAttributes();
return new Bookmark(
type,
id,
channelId,
title,
link,
emoji,
iconUrl,
entityId,
dateCreatedEpochSeconds,
dateUpdatedEpochSeconds,
rank,
lastUpdatedByUserId,
lastUpdatedByTeamId,
shortcutId,
appId);
}
private boolean typeIsSet() {
return (initBits & INIT_BIT_TYPE) == 0;
}
private boolean idIsSet() {
return (initBits & INIT_BIT_ID) == 0;
}
private boolean channelIdIsSet() {
return (initBits & INIT_BIT_CHANNEL_ID) == 0;
}
private boolean titleIsSet() {
return (initBits & INIT_BIT_TITLE) == 0;
}
private boolean dateCreatedEpochSecondsIsSet() {
return (initBits & INIT_BIT_DATE_CREATED_EPOCH_SECONDS) == 0;
}
private boolean dateUpdatedEpochSecondsIsSet() {
return (initBits & INIT_BIT_DATE_UPDATED_EPOCH_SECONDS) == 0;
}
private void checkRequiredAttributes() {
if (initBits != 0) {
throw new InvalidImmutableStateException(formatRequiredAttributesMessage());
}
}
private String formatRequiredAttributesMessage() {
List attributes = new ArrayList<>();
if (!typeIsSet()) attributes.add("type");
if (!idIsSet()) attributes.add("id");
if (!channelIdIsSet()) attributes.add("channelId");
if (!titleIsSet()) attributes.add("title");
if (!dateCreatedEpochSecondsIsSet()) attributes.add("dateCreatedEpochSeconds");
if (!dateUpdatedEpochSecondsIsSet()) attributes.add("dateUpdatedEpochSeconds");
return "Cannot build Bookmark, some of required attributes are not set " + attributes;
}
}
}