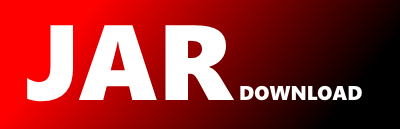
com.hubspot.slack.client.models.events.SlackEventMessageChanged Maven / Gradle / Ivy
package com.hubspot.slack.client.models.events;
import com.fasterxml.jackson.annotation.JsonAutoDetect;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import com.hubspot.immutables.validation.InvalidImmutableStateException;
import com.hubspot.slack.client.methods.interceptor.HasChannel;
import com.hubspot.slack.client.models.Attachment;
import com.hubspot.slack.client.models.LiteMessage;
import edu.umd.cs.findbugs.annotations.SuppressFBWarnings;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
import javax.annotation.ParametersAreNonnullByDefault;
import javax.annotation.concurrent.Immutable;
import javax.annotation.concurrent.NotThreadSafe;
import org.immutables.value.Generated;
/**
* Immutable implementation of {@link AbstractSlackEventMessageChanged}.
*
* Use the builder to create immutable instances:
* {@code SlackEventMessageChanged.builder()}.
*/
@Generated(from = "AbstractSlackEventMessageChanged", generator = "Immutables")
@SuppressWarnings({"all"})
@SuppressFBWarnings
@ParametersAreNonnullByDefault
@javax.annotation.processing.Generated("org.immutables.processor.ProxyProcessor")
@Immutable
public final class SlackEventMessageChanged
extends AbstractSlackEventMessageChanged {
private final SlackEventType type;
private final String ts;
private final @Nullable SlackMessageSubtype subtype;
private final List attachments;
private final String channelId;
private final boolean hidden;
private final LiteMessage message;
private final @Nullable LiteMessage previousMessage;
private final String eventTs;
private SlackEventMessageChanged(
SlackEventType type,
String ts,
@Nullable SlackMessageSubtype subtype,
List attachments,
String channelId,
boolean hidden,
LiteMessage message,
@Nullable LiteMessage previousMessage,
String eventTs) {
this.type = type;
this.ts = ts;
this.subtype = subtype;
this.attachments = attachments;
this.channelId = channelId;
this.hidden = hidden;
this.message = message;
this.previousMessage = previousMessage;
this.eventTs = eventTs;
}
/**
* @return The value of the {@code type} attribute
*/
@JsonProperty
@Override
public SlackEventType getType() {
return type;
}
/**
* @return The value of the {@code ts} attribute
*/
@JsonProperty
@Override
public String getTs() {
return ts;
}
/**
* @return The value of the {@code subtype} attribute
*/
@JsonProperty
@Override
public Optional getSubtype() {
return Optional.ofNullable(subtype);
}
/**
* @return The value of the {@code attachments} attribute
*/
@JsonProperty
@Override
public List getAttachments() {
return attachments;
}
/**
* @return The value of the {@code channelId} attribute
*/
@JsonProperty("channel")
@Override
public String getChannelId() {
return channelId;
}
/**
* @return The value of the {@code hidden} attribute
*/
@JsonProperty
@Override
public boolean isHidden() {
return hidden;
}
/**
* @return The value of the {@code message} attribute
*/
@JsonProperty
@Override
public LiteMessage getMessage() {
return message;
}
/**
* @return The value of the {@code previousMessage} attribute
*/
@JsonProperty
@Override
public Optional getPreviousMessage() {
return Optional.ofNullable(previousMessage);
}
/**
* @return The value of the {@code eventTs} attribute
*/
@JsonProperty
@Override
public String getEventTs() {
return eventTs;
}
/**
* Copy the current immutable object by setting a value for the {@link AbstractSlackEventMessageChanged#getType() type} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for type
* @return A modified copy of the {@code this} object
*/
public final SlackEventMessageChanged withType(SlackEventType value) {
SlackEventType newValue = Objects.requireNonNull(value, "type");
if (this.type == newValue) return this;
return new SlackEventMessageChanged(
newValue,
this.ts,
this.subtype,
this.attachments,
this.channelId,
this.hidden,
this.message,
this.previousMessage,
this.eventTs);
}
/**
* Copy the current immutable object by setting a value for the {@link AbstractSlackEventMessageChanged#getTs() ts} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for ts
* @return A modified copy of the {@code this} object
*/
public final SlackEventMessageChanged withTs(String value) {
String newValue = Objects.requireNonNull(value, "ts");
if (this.ts.equals(newValue)) return this;
return new SlackEventMessageChanged(
this.type,
newValue,
this.subtype,
this.attachments,
this.channelId,
this.hidden,
this.message,
this.previousMessage,
this.eventTs);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link AbstractSlackEventMessageChanged#getSubtype() subtype} attribute.
* @param value The value for subtype, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final SlackEventMessageChanged withSubtype(@Nullable SlackMessageSubtype value) {
@Nullable SlackMessageSubtype newValue = value;
if (this.subtype == newValue) return this;
return new SlackEventMessageChanged(
this.type,
this.ts,
newValue,
this.attachments,
this.channelId,
this.hidden,
this.message,
this.previousMessage,
this.eventTs);
}
/**
* Copy the current immutable object by setting an optional value for the {@link AbstractSlackEventMessageChanged#getSubtype() subtype} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for subtype
* @return A modified copy of {@code this} object
*/
@SuppressWarnings("unchecked") // safe covariant cast
public final SlackEventMessageChanged withSubtype(Optional extends SlackMessageSubtype> optional) {
@Nullable SlackMessageSubtype value = optional.orElse(null);
if (this.subtype == value) return this;
return new SlackEventMessageChanged(
this.type,
this.ts,
value,
this.attachments,
this.channelId,
this.hidden,
this.message,
this.previousMessage,
this.eventTs);
}
/**
* Copy the current immutable object with elements that replace the content of {@link AbstractSlackEventMessageChanged#getAttachments() attachments}.
* @param elements The elements to set
* @return A modified copy of {@code this} object
*/
public final SlackEventMessageChanged withAttachments(Attachment... elements) {
List newValue = createUnmodifiableList(false, createSafeList(Arrays.asList(elements), true, false));
return new SlackEventMessageChanged(
this.type,
this.ts,
this.subtype,
newValue,
this.channelId,
this.hidden,
this.message,
this.previousMessage,
this.eventTs);
}
/**
* Copy the current immutable object with elements that replace the content of {@link AbstractSlackEventMessageChanged#getAttachments() attachments}.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements An iterable of attachments elements to set
* @return A modified copy of {@code this} object
*/
public final SlackEventMessageChanged withAttachments(Iterable extends Attachment> elements) {
if (this.attachments == elements) return this;
List newValue = createUnmodifiableList(false, createSafeList(elements, true, false));
return new SlackEventMessageChanged(
this.type,
this.ts,
this.subtype,
newValue,
this.channelId,
this.hidden,
this.message,
this.previousMessage,
this.eventTs);
}
/**
* Copy the current immutable object by setting a value for the {@link AbstractSlackEventMessageChanged#getChannelId() channelId} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for channelId
* @return A modified copy of the {@code this} object
*/
public final SlackEventMessageChanged withChannelId(String value) {
String newValue = Objects.requireNonNull(value, "channelId");
if (this.channelId.equals(newValue)) return this;
return new SlackEventMessageChanged(
this.type,
this.ts,
this.subtype,
this.attachments,
newValue,
this.hidden,
this.message,
this.previousMessage,
this.eventTs);
}
/**
* Copy the current immutable object by setting a value for the {@link AbstractSlackEventMessageChanged#isHidden() hidden} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for hidden
* @return A modified copy of the {@code this} object
*/
public final SlackEventMessageChanged withHidden(boolean value) {
if (this.hidden == value) return this;
return new SlackEventMessageChanged(
this.type,
this.ts,
this.subtype,
this.attachments,
this.channelId,
value,
this.message,
this.previousMessage,
this.eventTs);
}
/**
* Copy the current immutable object by setting a value for the {@link AbstractSlackEventMessageChanged#getMessage() message} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for message
* @return A modified copy of the {@code this} object
*/
public final SlackEventMessageChanged withMessage(LiteMessage value) {
if (this.message == value) return this;
LiteMessage newValue = Objects.requireNonNull(value, "message");
return new SlackEventMessageChanged(
this.type,
this.ts,
this.subtype,
this.attachments,
this.channelId,
this.hidden,
newValue,
this.previousMessage,
this.eventTs);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link AbstractSlackEventMessageChanged#getPreviousMessage() previousMessage} attribute.
* @param value The value for previousMessage, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final SlackEventMessageChanged withPreviousMessage(@Nullable LiteMessage value) {
@Nullable LiteMessage newValue = value;
if (this.previousMessage == newValue) return this;
return new SlackEventMessageChanged(
this.type,
this.ts,
this.subtype,
this.attachments,
this.channelId,
this.hidden,
this.message,
newValue,
this.eventTs);
}
/**
* Copy the current immutable object by setting an optional value for the {@link AbstractSlackEventMessageChanged#getPreviousMessage() previousMessage} attribute.
* A shallow reference equality check is used on unboxed optional value to prevent copying of the same value by returning {@code this}.
* @param optional A value for previousMessage
* @return A modified copy of {@code this} object
*/
@SuppressWarnings("unchecked") // safe covariant cast
public final SlackEventMessageChanged withPreviousMessage(Optional extends LiteMessage> optional) {
@Nullable LiteMessage value = optional.orElse(null);
if (this.previousMessage == value) return this;
return new SlackEventMessageChanged(
this.type,
this.ts,
this.subtype,
this.attachments,
this.channelId,
this.hidden,
this.message,
value,
this.eventTs);
}
/**
* Copy the current immutable object by setting a value for the {@link AbstractSlackEventMessageChanged#getEventTs() eventTs} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for eventTs
* @return A modified copy of the {@code this} object
*/
public final SlackEventMessageChanged withEventTs(String value) {
String newValue = Objects.requireNonNull(value, "eventTs");
if (this.eventTs.equals(newValue)) return this;
return new SlackEventMessageChanged(
this.type,
this.ts,
this.subtype,
this.attachments,
this.channelId,
this.hidden,
this.message,
this.previousMessage,
newValue);
}
/**
* This instance is equal to all instances of {@code SlackEventMessageChanged} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(@Nullable Object another) {
if (this == another) return true;
return another instanceof SlackEventMessageChanged
&& equalTo(0, (SlackEventMessageChanged) another);
}
private boolean equalTo(int synthetic, SlackEventMessageChanged another) {
return type.equals(another.type)
&& ts.equals(another.ts)
&& Objects.equals(subtype, another.subtype)
&& attachments.equals(another.attachments)
&& channelId.equals(another.channelId)
&& hidden == another.hidden
&& message.equals(another.message)
&& Objects.equals(previousMessage, another.previousMessage)
&& eventTs.equals(another.eventTs);
}
/**
* Computes a hash code from attributes: {@code type}, {@code ts}, {@code subtype}, {@code attachments}, {@code channelId}, {@code hidden}, {@code message}, {@code previousMessage}, {@code eventTs}.
* @return hashCode value
*/
@Override
public int hashCode() {
int h = 5381;
h += (h << 5) + type.hashCode();
h += (h << 5) + ts.hashCode();
h += (h << 5) + Objects.hashCode(subtype);
h += (h << 5) + attachments.hashCode();
h += (h << 5) + channelId.hashCode();
h += (h << 5) + Boolean.hashCode(hidden);
h += (h << 5) + message.hashCode();
h += (h << 5) + Objects.hashCode(previousMessage);
h += (h << 5) + eventTs.hashCode();
return h;
}
/**
* Prints the immutable value {@code SlackEventMessageChanged} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
StringBuilder builder = new StringBuilder("SlackEventMessageChanged{");
builder.append("type=").append(type);
builder.append(", ");
builder.append("ts=").append(ts);
if (subtype != null) {
builder.append(", ");
builder.append("subtype=").append(subtype);
}
builder.append(", ");
builder.append("attachments=").append(attachments);
builder.append(", ");
builder.append("channelId=").append(channelId);
builder.append(", ");
builder.append("hidden=").append(hidden);
builder.append(", ");
builder.append("message=").append(message);
if (previousMessage != null) {
builder.append(", ");
builder.append("previousMessage=").append(previousMessage);
}
builder.append(", ");
builder.append("eventTs=").append(eventTs);
return builder.append("}").toString();
}
/**
* Utility type used to correctly read immutable object from JSON representation.
* @deprecated Do not use this type directly, it exists only for the Jackson-binding infrastructure
*/
@Generated(from = "AbstractSlackEventMessageChanged", generator = "Immutables")
@Deprecated
@JsonDeserialize
@JsonAutoDetect(fieldVisibility = JsonAutoDetect.Visibility.NONE)
static final class Json extends AbstractSlackEventMessageChanged {
@Nullable SlackEventType type;
@Nullable String ts;
@Nullable Optional subtype = Optional.empty();
@Nullable List attachments = Collections.emptyList();
@Nullable String channelId;
boolean hidden;
boolean hiddenIsSet;
@Nullable LiteMessage message;
@Nullable Optional previousMessage = Optional.empty();
@Nullable String eventTs;
@JsonProperty
public void setType(SlackEventType type) {
this.type = type;
}
@JsonProperty
public void setTs(String ts) {
this.ts = ts;
}
@JsonProperty
public void setSubtype(Optional subtype) {
this.subtype = subtype;
}
@JsonProperty
public void setAttachments(List attachments) {
this.attachments = attachments;
}
@JsonProperty("channel")
public void setChannelId(String channelId) {
this.channelId = channelId;
}
@JsonProperty
public void setHidden(boolean hidden) {
this.hidden = hidden;
this.hiddenIsSet = true;
}
@JsonProperty
public void setMessage(LiteMessage message) {
this.message = message;
}
@JsonProperty
public void setPreviousMessage(Optional previousMessage) {
this.previousMessage = previousMessage;
}
@JsonProperty
public void setEventTs(String eventTs) {
this.eventTs = eventTs;
}
@Override
public SlackEventType getType() { throw new UnsupportedOperationException(); }
@Override
public String getTs() { throw new UnsupportedOperationException(); }
@Override
public Optional getSubtype() { throw new UnsupportedOperationException(); }
@Override
public List getAttachments() { throw new UnsupportedOperationException(); }
@Override
public String getChannelId() { throw new UnsupportedOperationException(); }
@Override
public boolean isHidden() { throw new UnsupportedOperationException(); }
@Override
public LiteMessage getMessage() { throw new UnsupportedOperationException(); }
@Override
public Optional getPreviousMessage() { throw new UnsupportedOperationException(); }
@Override
public String getEventTs() { throw new UnsupportedOperationException(); }
}
/**
* @param json A JSON-bindable data structure
* @return An immutable value type
* @deprecated Do not use this method directly, it exists only for the Jackson-binding infrastructure
*/
@Deprecated
@JsonCreator(mode = JsonCreator.Mode.DELEGATING)
static SlackEventMessageChanged fromJson(Json json) {
SlackEventMessageChanged.Builder builder = SlackEventMessageChanged.builder();
if (json.type != null) {
builder.setType(json.type);
}
if (json.ts != null) {
builder.setTs(json.ts);
}
if (json.subtype != null) {
builder.setSubtype(json.subtype);
}
if (json.attachments != null) {
builder.addAllAttachments(json.attachments);
}
if (json.channelId != null) {
builder.setChannelId(json.channelId);
}
if (json.hiddenIsSet) {
builder.setHidden(json.hidden);
}
if (json.message != null) {
builder.setMessage(json.message);
}
if (json.previousMessage != null) {
builder.setPreviousMessage(json.previousMessage);
}
if (json.eventTs != null) {
builder.setEventTs(json.eventTs);
}
return builder.build();
}
/**
* Creates an immutable copy of a {@link AbstractSlackEventMessageChanged} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable SlackEventMessageChanged instance
*/
public static SlackEventMessageChanged copyOf(AbstractSlackEventMessageChanged instance) {
if (instance instanceof SlackEventMessageChanged) {
return (SlackEventMessageChanged) instance;
}
return SlackEventMessageChanged.builder()
.from(instance)
.build();
}
/**
* Creates a builder for {@link SlackEventMessageChanged SlackEventMessageChanged}.
*
* SlackEventMessageChanged.builder()
* .setType(com.hubspot.slack.client.models.events.SlackEventType) // required {@link AbstractSlackEventMessageChanged#getType() type}
* .setTs(String) // required {@link AbstractSlackEventMessageChanged#getTs() ts}
* .setSubtype(com.hubspot.slack.client.models.events.SlackMessageSubtype) // optional {@link AbstractSlackEventMessageChanged#getSubtype() subtype}
* .addAttachments|addAllAttachments(com.hubspot.slack.client.models.Attachment) // {@link AbstractSlackEventMessageChanged#getAttachments() attachments} elements
* .setChannelId(String) // required {@link AbstractSlackEventMessageChanged#getChannelId() channelId}
* .setHidden(boolean) // required {@link AbstractSlackEventMessageChanged#isHidden() hidden}
* .setMessage(com.hubspot.slack.client.models.LiteMessage) // required {@link AbstractSlackEventMessageChanged#getMessage() message}
* .setPreviousMessage(com.hubspot.slack.client.models.LiteMessage) // optional {@link AbstractSlackEventMessageChanged#getPreviousMessage() previousMessage}
* .setEventTs(String) // required {@link AbstractSlackEventMessageChanged#getEventTs() eventTs}
* .build();
*
* @return A new SlackEventMessageChanged builder
*/
public static SlackEventMessageChanged.Builder builder() {
return new SlackEventMessageChanged.Builder();
}
/**
* Builds instances of type {@link SlackEventMessageChanged SlackEventMessageChanged}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
@Generated(from = "AbstractSlackEventMessageChanged", generator = "Immutables")
@NotThreadSafe
public static final class Builder {
private static final long INIT_BIT_TYPE = 0x1L;
private static final long INIT_BIT_TS = 0x2L;
private static final long INIT_BIT_CHANNEL_ID = 0x4L;
private static final long INIT_BIT_HIDDEN = 0x8L;
private static final long INIT_BIT_MESSAGE = 0x10L;
private static final long INIT_BIT_EVENT_TS = 0x20L;
private long initBits = 0x3fL;
private @Nullable SlackEventType type;
private @Nullable String ts;
private @Nullable SlackMessageSubtype subtype;
private List attachments = new ArrayList();
private @Nullable String channelId;
private boolean hidden;
private @Nullable LiteMessage message;
private @Nullable LiteMessage previousMessage;
private @Nullable String eventTs;
private Builder() {
}
/**
* Fill a builder with attribute values from the provided {@code com.hubspot.slack.client.methods.interceptor.HasChannel} instance.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(HasChannel instance) {
Objects.requireNonNull(instance, "instance");
from((short) 0, (Object) instance);
return this;
}
/**
* Fill a builder with attribute values from the provided {@code com.hubspot.slack.client.models.events.SlackEvent} instance.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(SlackEvent instance) {
Objects.requireNonNull(instance, "instance");
from((short) 0, (Object) instance);
return this;
}
/**
* Fill a builder with attribute values from the provided {@code com.hubspot.slack.client.models.events.AbstractSlackEventMessageChanged} instance.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(AbstractSlackEventMessageChanged instance) {
Objects.requireNonNull(instance, "instance");
from((short) 0, (Object) instance);
return this;
}
/**
* Fill a builder with attribute values from the provided {@code com.hubspot.slack.client.models.events.SlackEventMessageBase} instance.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(SlackEventMessageBase instance) {
Objects.requireNonNull(instance, "instance");
from((short) 0, (Object) instance);
return this;
}
private void from(short _unused, Object object) {
long bits = 0;
if (object instanceof HasChannel) {
HasChannel instance = (HasChannel) object;
if ((bits & 0x8L) == 0) {
this.setChannelId(instance.getChannelId());
bits |= 0x8L;
}
}
if (object instanceof SlackEvent) {
SlackEvent instance = (SlackEvent) object;
if ((bits & 0x2L) == 0) {
this.setType(instance.getType());
bits |= 0x2L;
}
if ((bits & 0x10L) == 0) {
this.setTs(instance.getTs());
bits |= 0x10L;
}
}
if (object instanceof AbstractSlackEventMessageChanged) {
AbstractSlackEventMessageChanged instance = (AbstractSlackEventMessageChanged) object;
if ((bits & 0x1L) == 0) {
addAllAttachments(instance.getAttachments());
bits |= 0x1L;
}
this.setHidden(instance.isHidden());
if ((bits & 0x4L) == 0) {
Optional subtypeOptional = instance.getSubtype();
if (subtypeOptional.isPresent()) {
setSubtype(subtypeOptional);
}
bits |= 0x4L;
}
if ((bits & 0x2L) == 0) {
this.setType(instance.getType());
bits |= 0x2L;
}
this.setMessage(instance.getMessage());
Optional previousMessageOptional = instance.getPreviousMessage();
if (previousMessageOptional.isPresent()) {
setPreviousMessage(previousMessageOptional);
}
this.setEventTs(instance.getEventTs());
if ((bits & 0x8L) == 0) {
this.setChannelId(instance.getChannelId());
bits |= 0x8L;
}
if ((bits & 0x10L) == 0) {
this.setTs(instance.getTs());
bits |= 0x10L;
}
}
if (object instanceof SlackEventMessageBase) {
SlackEventMessageBase instance = (SlackEventMessageBase) object;
if ((bits & 0x1L) == 0) {
addAllAttachments(instance.getAttachments());
bits |= 0x1L;
}
if ((bits & 0x2L) == 0) {
this.setType(instance.getType());
bits |= 0x2L;
}
if ((bits & 0x4L) == 0) {
Optional subtypeOptional = instance.getSubtype();
if (subtypeOptional.isPresent()) {
setSubtype(subtypeOptional);
}
bits |= 0x4L;
}
if ((bits & 0x8L) == 0) {
this.setChannelId(instance.getChannelId());
bits |= 0x8L;
}
if ((bits & 0x10L) == 0) {
this.setTs(instance.getTs());
bits |= 0x10L;
}
}
}
/**
* Initializes the value for the {@link AbstractSlackEventMessageChanged#getType() type} attribute.
* @param type The value for type
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty
public final Builder setType(SlackEventType type) {
this.type = Objects.requireNonNull(type, "type");
initBits &= ~INIT_BIT_TYPE;
return this;
}
/**
* Initializes the value for the {@link AbstractSlackEventMessageChanged#getTs() ts} attribute.
* @param ts The value for ts
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty
public final Builder setTs(String ts) {
this.ts = Objects.requireNonNull(ts, "ts");
initBits &= ~INIT_BIT_TS;
return this;
}
/**
* Initializes the optional value {@link AbstractSlackEventMessageChanged#getSubtype() subtype} to subtype.
* @param subtype The value for subtype, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
public final Builder setSubtype(@Nullable SlackMessageSubtype subtype) {
this.subtype = subtype;
return this;
}
/**
* Initializes the optional value {@link AbstractSlackEventMessageChanged#getSubtype() subtype} to subtype.
* @param subtype The value for subtype
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty
public final Builder setSubtype(Optional extends SlackMessageSubtype> subtype) {
this.subtype = subtype.orElse(null);
return this;
}
/**
* Adds one element to {@link AbstractSlackEventMessageChanged#getAttachments() attachments} list.
* @param element A attachments element
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addAttachments(Attachment element) {
this.attachments.add(Objects.requireNonNull(element, "attachments element"));
return this;
}
/**
* Adds elements to {@link AbstractSlackEventMessageChanged#getAttachments() attachments} list.
* @param elements An array of attachments elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addAttachments(Attachment... elements) {
for (Attachment element : elements) {
this.attachments.add(Objects.requireNonNull(element, "attachments element"));
}
return this;
}
/**
* Sets or replaces all elements for {@link AbstractSlackEventMessageChanged#getAttachments() attachments} list.
* @param elements An iterable of attachments elements
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty
public final Builder setAttachments(Iterable extends Attachment> elements) {
this.attachments.clear();
return addAllAttachments(elements);
}
/**
* Adds elements to {@link AbstractSlackEventMessageChanged#getAttachments() attachments} list.
* @param elements An iterable of attachments elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addAllAttachments(Iterable extends Attachment> elements) {
for (Attachment element : elements) {
this.attachments.add(Objects.requireNonNull(element, "attachments element"));
}
return this;
}
/**
* Initializes the value for the {@link AbstractSlackEventMessageChanged#getChannelId() channelId} attribute.
* @param channelId The value for channelId
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("channel")
public final Builder setChannelId(String channelId) {
this.channelId = Objects.requireNonNull(channelId, "channelId");
initBits &= ~INIT_BIT_CHANNEL_ID;
return this;
}
/**
* Initializes the value for the {@link AbstractSlackEventMessageChanged#isHidden() hidden} attribute.
* @param hidden The value for hidden
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty
public final Builder setHidden(boolean hidden) {
this.hidden = hidden;
initBits &= ~INIT_BIT_HIDDEN;
return this;
}
/**
* Initializes the value for the {@link AbstractSlackEventMessageChanged#getMessage() message} attribute.
* @param message The value for message
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty
public final Builder setMessage(LiteMessage message) {
this.message = Objects.requireNonNull(message, "message");
initBits &= ~INIT_BIT_MESSAGE;
return this;
}
/**
* Initializes the optional value {@link AbstractSlackEventMessageChanged#getPreviousMessage() previousMessage} to previousMessage.
* @param previousMessage The value for previousMessage, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
public final Builder setPreviousMessage(@Nullable LiteMessage previousMessage) {
this.previousMessage = previousMessage;
return this;
}
/**
* Initializes the optional value {@link AbstractSlackEventMessageChanged#getPreviousMessage() previousMessage} to previousMessage.
* @param previousMessage The value for previousMessage
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty
public final Builder setPreviousMessage(Optional extends LiteMessage> previousMessage) {
this.previousMessage = previousMessage.orElse(null);
return this;
}
/**
* Initializes the value for the {@link AbstractSlackEventMessageChanged#getEventTs() eventTs} attribute.
* @param eventTs The value for eventTs
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty
public final Builder setEventTs(String eventTs) {
this.eventTs = Objects.requireNonNull(eventTs, "eventTs");
initBits &= ~INIT_BIT_EVENT_TS;
return this;
}
/**
* Builds a new {@link SlackEventMessageChanged SlackEventMessageChanged}.
* @return An immutable instance of SlackEventMessageChanged
* @throws com.hubspot.immutables.validation.InvalidImmutableStateException if any required attributes are missing
*/
public SlackEventMessageChanged build() {
checkRequiredAttributes();
return new SlackEventMessageChanged(
type,
ts,
subtype,
createUnmodifiableList(true, attachments),
channelId,
hidden,
message,
previousMessage,
eventTs);
}
private boolean typeIsSet() {
return (initBits & INIT_BIT_TYPE) == 0;
}
private boolean tsIsSet() {
return (initBits & INIT_BIT_TS) == 0;
}
private boolean channelIdIsSet() {
return (initBits & INIT_BIT_CHANNEL_ID) == 0;
}
private boolean hiddenIsSet() {
return (initBits & INIT_BIT_HIDDEN) == 0;
}
private boolean messageIsSet() {
return (initBits & INIT_BIT_MESSAGE) == 0;
}
private boolean eventTsIsSet() {
return (initBits & INIT_BIT_EVENT_TS) == 0;
}
private void checkRequiredAttributes() {
if (initBits != 0) {
throw new InvalidImmutableStateException(formatRequiredAttributesMessage());
}
}
private String formatRequiredAttributesMessage() {
List attributes = new ArrayList<>();
if (!typeIsSet()) attributes.add("type");
if (!tsIsSet()) attributes.add("ts");
if (!channelIdIsSet()) attributes.add("channelId");
if (!hiddenIsSet()) attributes.add("hidden");
if (!messageIsSet()) attributes.add("message");
if (!eventTsIsSet()) attributes.add("eventTs");
return "Cannot build SlackEventMessageChanged, some of required attributes are not set " + attributes;
}
}
private static List createSafeList(Iterable extends T> iterable, boolean checkNulls, boolean skipNulls) {
ArrayList list;
if (iterable instanceof Collection>) {
int size = ((Collection>) iterable).size();
if (size == 0) return Collections.emptyList();
list = new ArrayList<>(size);
} else {
list = new ArrayList<>();
}
for (T element : iterable) {
if (skipNulls && element == null) continue;
if (checkNulls) Objects.requireNonNull(element, "element");
list.add(element);
}
return list;
}
private static List createUnmodifiableList(boolean clone, List list) {
switch(list.size()) {
case 0: return Collections.emptyList();
case 1: return Collections.singletonList(list.get(0));
default:
if (clone) {
return Collections.unmodifiableList(new ArrayList<>(list));
} else {
if (list instanceof ArrayList>) {
((ArrayList>) list).trimToSize();
}
return Collections.unmodifiableList(list);
}
}
}
}