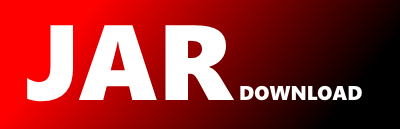
com.hubspot.slack.client.models.files.SlackAccessDeniedFile Maven / Gradle / Ivy
package com.hubspot.slack.client.models.files;
import com.fasterxml.jackson.annotation.JsonAutoDetect;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import com.hubspot.immutables.validation.InvalidImmutableStateException;
import edu.umd.cs.findbugs.annotations.SuppressFBWarnings;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
import javax.annotation.ParametersAreNonnullByDefault;
import javax.annotation.concurrent.Immutable;
import javax.annotation.concurrent.NotThreadSafe;
import org.immutables.value.Generated;
/**
* Immutable implementation of {@link SlackAccessDeniedFileIF}.
*
* Use the builder to create immutable instances:
* {@code SlackAccessDeniedFile.builder()}.
*/
@Generated(from = "SlackAccessDeniedFileIF", generator = "Immutables")
@SuppressWarnings({"all"})
@SuppressFBWarnings
@ParametersAreNonnullByDefault
@javax.annotation.processing.Generated("org.immutables.processor.ProxyProcessor")
@Immutable
public final class SlackAccessDeniedFile
implements SlackAccessDeniedFileIF {
private final String fileAccess;
private final String id;
private final long createdEpochSeconds;
private final long timestampEpochSeconds;
private final String name;
private final String title;
private final String mimetype;
private final SlackFileType filetype;
private final String prettyType;
private final String userId;
private final boolean editable;
private final long size;
private final String mode;
private final boolean external;
private final boolean isPublic;
private final boolean publicUrlShared;
private final boolean displayAsBot;
private final String username;
private final String urlPrivate;
private final @Nullable String urlPrivateDownload;
private final String permalink;
private final @Nullable String permalinkPublic;
private final int commentsCount;
private final @Nullable Boolean starred;
private final List channelIds;
private final List groupIds;
private final List imIds;
private SlackAccessDeniedFile(SlackAccessDeniedFile.Builder builder) {
this.fileAccess = builder.fileAccess;
this.urlPrivateDownload = builder.urlPrivateDownload;
this.permalinkPublic = builder.permalinkPublic;
this.starred = builder.starred;
this.channelIds = createUnmodifiableList(true, builder.channelIds);
this.groupIds = createUnmodifiableList(true, builder.groupIds);
this.imIds = createUnmodifiableList(true, builder.imIds);
if (builder.id != null) {
initShim.setId(builder.id);
}
if (builder.createdEpochSecondsIsSet()) {
initShim.setCreatedEpochSeconds(builder.createdEpochSeconds);
}
if (builder.timestampEpochSecondsIsSet()) {
initShim.setTimestampEpochSeconds(builder.timestampEpochSeconds);
}
if (builder.name != null) {
initShim.setName(builder.name);
}
if (builder.title != null) {
initShim.setTitle(builder.title);
}
if (builder.mimetype != null) {
initShim.setMimetype(builder.mimetype);
}
if (builder.filetype != null) {
initShim.setFiletype(builder.filetype);
}
if (builder.prettyType != null) {
initShim.setPrettyType(builder.prettyType);
}
if (builder.userId != null) {
initShim.setUserId(builder.userId);
}
if (builder.editableIsSet()) {
initShim.setEditable(builder.editable);
}
if (builder.sizeIsSet()) {
initShim.setSize(builder.size);
}
if (builder.mode != null) {
initShim.setMode(builder.mode);
}
if (builder.externalIsSet()) {
initShim.setExternal(builder.external);
}
if (builder.publicIsSet()) {
initShim.setPublic(builder.isPublic);
}
if (builder.publicUrlSharedIsSet()) {
initShim.setPublicUrlShared(builder.publicUrlShared);
}
if (builder.displayAsBotIsSet()) {
initShim.setDisplayAsBot(builder.displayAsBot);
}
if (builder.username != null) {
initShim.setUsername(builder.username);
}
if (builder.urlPrivate != null) {
initShim.setUrlPrivate(builder.urlPrivate);
}
if (builder.permalink != null) {
initShim.setPermalink(builder.permalink);
}
if (builder.commentsCountIsSet()) {
initShim.setCommentsCount(builder.commentsCount);
}
this.id = initShim.getId();
this.createdEpochSeconds = initShim.getCreatedEpochSeconds();
this.timestampEpochSeconds = initShim.getTimestampEpochSeconds();
this.name = initShim.getName();
this.title = initShim.getTitle();
this.mimetype = initShim.getMimetype();
this.filetype = initShim.getFiletype();
this.prettyType = initShim.getPrettyType();
this.userId = initShim.getUserId();
this.editable = initShim.isEditable();
this.size = initShim.getSize();
this.mode = initShim.getMode();
this.external = initShim.isExternal();
this.isPublic = initShim.isPublic();
this.publicUrlShared = initShim.isPublicUrlShared();
this.displayAsBot = initShim.getDisplayAsBot();
this.username = initShim.getUsername();
this.urlPrivate = initShim.getUrlPrivate();
this.permalink = initShim.getPermalink();
this.commentsCount = initShim.getCommentsCount();
this.initShim = null;
}
private SlackAccessDeniedFile(
String fileAccess,
String id,
long createdEpochSeconds,
long timestampEpochSeconds,
String name,
String title,
String mimetype,
SlackFileType filetype,
String prettyType,
String userId,
boolean editable,
long size,
String mode,
boolean external,
boolean isPublic,
boolean publicUrlShared,
boolean displayAsBot,
String username,
String urlPrivate,
@Nullable String urlPrivateDownload,
String permalink,
@Nullable String permalinkPublic,
int commentsCount,
@Nullable Boolean starred,
List channelIds,
List groupIds,
List imIds) {
this.fileAccess = fileAccess;
this.id = id;
this.createdEpochSeconds = createdEpochSeconds;
this.timestampEpochSeconds = timestampEpochSeconds;
this.name = name;
this.title = title;
this.mimetype = mimetype;
this.filetype = filetype;
this.prettyType = prettyType;
this.userId = userId;
this.editable = editable;
this.size = size;
this.mode = mode;
this.external = external;
this.isPublic = isPublic;
this.publicUrlShared = publicUrlShared;
this.displayAsBot = displayAsBot;
this.username = username;
this.urlPrivate = urlPrivate;
this.urlPrivateDownload = urlPrivateDownload;
this.permalink = permalink;
this.permalinkPublic = permalinkPublic;
this.commentsCount = commentsCount;
this.starred = starred;
this.channelIds = channelIds;
this.groupIds = groupIds;
this.imIds = imIds;
this.initShim = null;
}
private static final byte STAGE_INITIALIZING = -1;
private static final byte STAGE_UNINITIALIZED = 0;
private static final byte STAGE_INITIALIZED = 1;
private transient volatile InitShim initShim = new InitShim();
@Generated(from = "SlackAccessDeniedFileIF", generator = "Immutables")
private final class InitShim {
private byte idBuildStage = STAGE_UNINITIALIZED;
private String id;
String getId() {
if (idBuildStage == STAGE_INITIALIZING) throw new InvalidImmutableStateException(formatInitCycleMessage());
if (idBuildStage == STAGE_UNINITIALIZED) {
idBuildStage = STAGE_INITIALIZING;
this.id = Objects.requireNonNull(getIdInitialize(), "id");
idBuildStage = STAGE_INITIALIZED;
}
return this.id;
}
void setId(String id) {
this.id = id;
idBuildStage = STAGE_INITIALIZED;
}
private byte createdEpochSecondsBuildStage = STAGE_UNINITIALIZED;
private long createdEpochSeconds;
long getCreatedEpochSeconds() {
if (createdEpochSecondsBuildStage == STAGE_INITIALIZING) throw new InvalidImmutableStateException(formatInitCycleMessage());
if (createdEpochSecondsBuildStage == STAGE_UNINITIALIZED) {
createdEpochSecondsBuildStage = STAGE_INITIALIZING;
this.createdEpochSeconds = getCreatedEpochSecondsInitialize();
createdEpochSecondsBuildStage = STAGE_INITIALIZED;
}
return this.createdEpochSeconds;
}
void setCreatedEpochSeconds(long createdEpochSeconds) {
this.createdEpochSeconds = createdEpochSeconds;
createdEpochSecondsBuildStage = STAGE_INITIALIZED;
}
private byte timestampEpochSecondsBuildStage = STAGE_UNINITIALIZED;
private long timestampEpochSeconds;
long getTimestampEpochSeconds() {
if (timestampEpochSecondsBuildStage == STAGE_INITIALIZING) throw new InvalidImmutableStateException(formatInitCycleMessage());
if (timestampEpochSecondsBuildStage == STAGE_UNINITIALIZED) {
timestampEpochSecondsBuildStage = STAGE_INITIALIZING;
this.timestampEpochSeconds = getTimestampEpochSecondsInitialize();
timestampEpochSecondsBuildStage = STAGE_INITIALIZED;
}
return this.timestampEpochSeconds;
}
void setTimestampEpochSeconds(long timestampEpochSeconds) {
this.timestampEpochSeconds = timestampEpochSeconds;
timestampEpochSecondsBuildStage = STAGE_INITIALIZED;
}
private byte nameBuildStage = STAGE_UNINITIALIZED;
private String name;
String getName() {
if (nameBuildStage == STAGE_INITIALIZING) throw new InvalidImmutableStateException(formatInitCycleMessage());
if (nameBuildStage == STAGE_UNINITIALIZED) {
nameBuildStage = STAGE_INITIALIZING;
this.name = Objects.requireNonNull(getNameInitialize(), "name");
nameBuildStage = STAGE_INITIALIZED;
}
return this.name;
}
void setName(String name) {
this.name = name;
nameBuildStage = STAGE_INITIALIZED;
}
private byte titleBuildStage = STAGE_UNINITIALIZED;
private String title;
String getTitle() {
if (titleBuildStage == STAGE_INITIALIZING) throw new InvalidImmutableStateException(formatInitCycleMessage());
if (titleBuildStage == STAGE_UNINITIALIZED) {
titleBuildStage = STAGE_INITIALIZING;
this.title = Objects.requireNonNull(getTitleInitialize(), "title");
titleBuildStage = STAGE_INITIALIZED;
}
return this.title;
}
void setTitle(String title) {
this.title = title;
titleBuildStage = STAGE_INITIALIZED;
}
private byte mimetypeBuildStage = STAGE_UNINITIALIZED;
private String mimetype;
String getMimetype() {
if (mimetypeBuildStage == STAGE_INITIALIZING) throw new InvalidImmutableStateException(formatInitCycleMessage());
if (mimetypeBuildStage == STAGE_UNINITIALIZED) {
mimetypeBuildStage = STAGE_INITIALIZING;
this.mimetype = Objects.requireNonNull(getMimetypeInitialize(), "mimetype");
mimetypeBuildStage = STAGE_INITIALIZED;
}
return this.mimetype;
}
void setMimetype(String mimetype) {
this.mimetype = mimetype;
mimetypeBuildStage = STAGE_INITIALIZED;
}
private byte filetypeBuildStage = STAGE_UNINITIALIZED;
private SlackFileType filetype;
SlackFileType getFiletype() {
if (filetypeBuildStage == STAGE_INITIALIZING) throw new InvalidImmutableStateException(formatInitCycleMessage());
if (filetypeBuildStage == STAGE_UNINITIALIZED) {
filetypeBuildStage = STAGE_INITIALIZING;
this.filetype = Objects.requireNonNull(getFiletypeInitialize(), "filetype");
filetypeBuildStage = STAGE_INITIALIZED;
}
return this.filetype;
}
void setFiletype(SlackFileType filetype) {
this.filetype = filetype;
filetypeBuildStage = STAGE_INITIALIZED;
}
private byte prettyTypeBuildStage = STAGE_UNINITIALIZED;
private String prettyType;
String getPrettyType() {
if (prettyTypeBuildStage == STAGE_INITIALIZING) throw new InvalidImmutableStateException(formatInitCycleMessage());
if (prettyTypeBuildStage == STAGE_UNINITIALIZED) {
prettyTypeBuildStage = STAGE_INITIALIZING;
this.prettyType = Objects.requireNonNull(getPrettyTypeInitialize(), "prettyType");
prettyTypeBuildStage = STAGE_INITIALIZED;
}
return this.prettyType;
}
void setPrettyType(String prettyType) {
this.prettyType = prettyType;
prettyTypeBuildStage = STAGE_INITIALIZED;
}
private byte userIdBuildStage = STAGE_UNINITIALIZED;
private String userId;
String getUserId() {
if (userIdBuildStage == STAGE_INITIALIZING) throw new InvalidImmutableStateException(formatInitCycleMessage());
if (userIdBuildStage == STAGE_UNINITIALIZED) {
userIdBuildStage = STAGE_INITIALIZING;
this.userId = Objects.requireNonNull(getUserIdInitialize(), "userId");
userIdBuildStage = STAGE_INITIALIZED;
}
return this.userId;
}
void setUserId(String userId) {
this.userId = userId;
userIdBuildStage = STAGE_INITIALIZED;
}
private byte editableBuildStage = STAGE_UNINITIALIZED;
private boolean editable;
boolean isEditable() {
if (editableBuildStage == STAGE_INITIALIZING) throw new InvalidImmutableStateException(formatInitCycleMessage());
if (editableBuildStage == STAGE_UNINITIALIZED) {
editableBuildStage = STAGE_INITIALIZING;
this.editable = isEditableInitialize();
editableBuildStage = STAGE_INITIALIZED;
}
return this.editable;
}
void setEditable(boolean editable) {
this.editable = editable;
editableBuildStage = STAGE_INITIALIZED;
}
private byte sizeBuildStage = STAGE_UNINITIALIZED;
private long size;
long getSize() {
if (sizeBuildStage == STAGE_INITIALIZING) throw new InvalidImmutableStateException(formatInitCycleMessage());
if (sizeBuildStage == STAGE_UNINITIALIZED) {
sizeBuildStage = STAGE_INITIALIZING;
this.size = getSizeInitialize();
sizeBuildStage = STAGE_INITIALIZED;
}
return this.size;
}
void setSize(long size) {
this.size = size;
sizeBuildStage = STAGE_INITIALIZED;
}
private byte modeBuildStage = STAGE_UNINITIALIZED;
private String mode;
String getMode() {
if (modeBuildStage == STAGE_INITIALIZING) throw new InvalidImmutableStateException(formatInitCycleMessage());
if (modeBuildStage == STAGE_UNINITIALIZED) {
modeBuildStage = STAGE_INITIALIZING;
this.mode = Objects.requireNonNull(getModeInitialize(), "mode");
modeBuildStage = STAGE_INITIALIZED;
}
return this.mode;
}
void setMode(String mode) {
this.mode = mode;
modeBuildStage = STAGE_INITIALIZED;
}
private byte externalBuildStage = STAGE_UNINITIALIZED;
private boolean external;
boolean isExternal() {
if (externalBuildStage == STAGE_INITIALIZING) throw new InvalidImmutableStateException(formatInitCycleMessage());
if (externalBuildStage == STAGE_UNINITIALIZED) {
externalBuildStage = STAGE_INITIALIZING;
this.external = isExternalInitialize();
externalBuildStage = STAGE_INITIALIZED;
}
return this.external;
}
void setExternal(boolean external) {
this.external = external;
externalBuildStage = STAGE_INITIALIZED;
}
private byte isPublicBuildStage = STAGE_UNINITIALIZED;
private boolean isPublic;
boolean isPublic() {
if (isPublicBuildStage == STAGE_INITIALIZING) throw new InvalidImmutableStateException(formatInitCycleMessage());
if (isPublicBuildStage == STAGE_UNINITIALIZED) {
isPublicBuildStage = STAGE_INITIALIZING;
this.isPublic = isPublicInitialize();
isPublicBuildStage = STAGE_INITIALIZED;
}
return this.isPublic;
}
void setPublic(boolean isPublic) {
this.isPublic = isPublic;
isPublicBuildStage = STAGE_INITIALIZED;
}
private byte publicUrlSharedBuildStage = STAGE_UNINITIALIZED;
private boolean publicUrlShared;
boolean isPublicUrlShared() {
if (publicUrlSharedBuildStage == STAGE_INITIALIZING) throw new InvalidImmutableStateException(formatInitCycleMessage());
if (publicUrlSharedBuildStage == STAGE_UNINITIALIZED) {
publicUrlSharedBuildStage = STAGE_INITIALIZING;
this.publicUrlShared = isPublicUrlSharedInitialize();
publicUrlSharedBuildStage = STAGE_INITIALIZED;
}
return this.publicUrlShared;
}
void setPublicUrlShared(boolean publicUrlShared) {
this.publicUrlShared = publicUrlShared;
publicUrlSharedBuildStage = STAGE_INITIALIZED;
}
private byte displayAsBotBuildStage = STAGE_UNINITIALIZED;
private boolean displayAsBot;
boolean getDisplayAsBot() {
if (displayAsBotBuildStage == STAGE_INITIALIZING) throw new InvalidImmutableStateException(formatInitCycleMessage());
if (displayAsBotBuildStage == STAGE_UNINITIALIZED) {
displayAsBotBuildStage = STAGE_INITIALIZING;
this.displayAsBot = getDisplayAsBotInitialize();
displayAsBotBuildStage = STAGE_INITIALIZED;
}
return this.displayAsBot;
}
void setDisplayAsBot(boolean displayAsBot) {
this.displayAsBot = displayAsBot;
displayAsBotBuildStage = STAGE_INITIALIZED;
}
private byte usernameBuildStage = STAGE_UNINITIALIZED;
private String username;
String getUsername() {
if (usernameBuildStage == STAGE_INITIALIZING) throw new InvalidImmutableStateException(formatInitCycleMessage());
if (usernameBuildStage == STAGE_UNINITIALIZED) {
usernameBuildStage = STAGE_INITIALIZING;
this.username = Objects.requireNonNull(getUsernameInitialize(), "username");
usernameBuildStage = STAGE_INITIALIZED;
}
return this.username;
}
void setUsername(String username) {
this.username = username;
usernameBuildStage = STAGE_INITIALIZED;
}
private byte urlPrivateBuildStage = STAGE_UNINITIALIZED;
private String urlPrivate;
String getUrlPrivate() {
if (urlPrivateBuildStage == STAGE_INITIALIZING) throw new InvalidImmutableStateException(formatInitCycleMessage());
if (urlPrivateBuildStage == STAGE_UNINITIALIZED) {
urlPrivateBuildStage = STAGE_INITIALIZING;
this.urlPrivate = Objects.requireNonNull(getUrlPrivateInitialize(), "urlPrivate");
urlPrivateBuildStage = STAGE_INITIALIZED;
}
return this.urlPrivate;
}
void setUrlPrivate(String urlPrivate) {
this.urlPrivate = urlPrivate;
urlPrivateBuildStage = STAGE_INITIALIZED;
}
private byte permalinkBuildStage = STAGE_UNINITIALIZED;
private String permalink;
String getPermalink() {
if (permalinkBuildStage == STAGE_INITIALIZING) throw new InvalidImmutableStateException(formatInitCycleMessage());
if (permalinkBuildStage == STAGE_UNINITIALIZED) {
permalinkBuildStage = STAGE_INITIALIZING;
this.permalink = Objects.requireNonNull(getPermalinkInitialize(), "permalink");
permalinkBuildStage = STAGE_INITIALIZED;
}
return this.permalink;
}
void setPermalink(String permalink) {
this.permalink = permalink;
permalinkBuildStage = STAGE_INITIALIZED;
}
private byte commentsCountBuildStage = STAGE_UNINITIALIZED;
private int commentsCount;
int getCommentsCount() {
if (commentsCountBuildStage == STAGE_INITIALIZING) throw new InvalidImmutableStateException(formatInitCycleMessage());
if (commentsCountBuildStage == STAGE_UNINITIALIZED) {
commentsCountBuildStage = STAGE_INITIALIZING;
this.commentsCount = getCommentsCountInitialize();
commentsCountBuildStage = STAGE_INITIALIZED;
}
return this.commentsCount;
}
void setCommentsCount(int commentsCount) {
this.commentsCount = commentsCount;
commentsCountBuildStage = STAGE_INITIALIZED;
}
private String formatInitCycleMessage() {
List attributes = new ArrayList<>();
if (idBuildStage == STAGE_INITIALIZING) attributes.add("id");
if (createdEpochSecondsBuildStage == STAGE_INITIALIZING) attributes.add("createdEpochSeconds");
if (timestampEpochSecondsBuildStage == STAGE_INITIALIZING) attributes.add("timestampEpochSeconds");
if (nameBuildStage == STAGE_INITIALIZING) attributes.add("name");
if (titleBuildStage == STAGE_INITIALIZING) attributes.add("title");
if (mimetypeBuildStage == STAGE_INITIALIZING) attributes.add("mimetype");
if (filetypeBuildStage == STAGE_INITIALIZING) attributes.add("filetype");
if (prettyTypeBuildStage == STAGE_INITIALIZING) attributes.add("prettyType");
if (userIdBuildStage == STAGE_INITIALIZING) attributes.add("userId");
if (editableBuildStage == STAGE_INITIALIZING) attributes.add("editable");
if (sizeBuildStage == STAGE_INITIALIZING) attributes.add("size");
if (modeBuildStage == STAGE_INITIALIZING) attributes.add("mode");
if (externalBuildStage == STAGE_INITIALIZING) attributes.add("external");
if (isPublicBuildStage == STAGE_INITIALIZING) attributes.add("isPublic");
if (publicUrlSharedBuildStage == STAGE_INITIALIZING) attributes.add("publicUrlShared");
if (displayAsBotBuildStage == STAGE_INITIALIZING) attributes.add("displayAsBot");
if (usernameBuildStage == STAGE_INITIALIZING) attributes.add("username");
if (urlPrivateBuildStage == STAGE_INITIALIZING) attributes.add("urlPrivate");
if (permalinkBuildStage == STAGE_INITIALIZING) attributes.add("permalink");
if (commentsCountBuildStage == STAGE_INITIALIZING) attributes.add("commentsCount");
return "Cannot build SlackAccessDeniedFile, attribute initializers form cycle " + attributes;
}
}
private String getIdInitialize() {
return SlackAccessDeniedFileIF.super.getId();
}
private long getCreatedEpochSecondsInitialize() {
return SlackAccessDeniedFileIF.super.getCreatedEpochSeconds();
}
private long getTimestampEpochSecondsInitialize() {
return SlackAccessDeniedFileIF.super.getTimestampEpochSeconds();
}
private String getNameInitialize() {
return SlackAccessDeniedFileIF.super.getName();
}
private String getTitleInitialize() {
return SlackAccessDeniedFileIF.super.getTitle();
}
private String getMimetypeInitialize() {
return SlackAccessDeniedFileIF.super.getMimetype();
}
private SlackFileType getFiletypeInitialize() {
return SlackAccessDeniedFileIF.super.getFiletype();
}
private String getPrettyTypeInitialize() {
return SlackAccessDeniedFileIF.super.getPrettyType();
}
private String getUserIdInitialize() {
return SlackAccessDeniedFileIF.super.getUserId();
}
private boolean isEditableInitialize() {
return SlackAccessDeniedFileIF.super.isEditable();
}
private long getSizeInitialize() {
return SlackAccessDeniedFileIF.super.getSize();
}
private String getModeInitialize() {
return SlackAccessDeniedFileIF.super.getMode();
}
private boolean isExternalInitialize() {
return SlackAccessDeniedFileIF.super.isExternal();
}
private boolean isPublicInitialize() {
return SlackAccessDeniedFileIF.super.isPublic();
}
private boolean isPublicUrlSharedInitialize() {
return SlackAccessDeniedFileIF.super.isPublicUrlShared();
}
private boolean getDisplayAsBotInitialize() {
return SlackAccessDeniedFileIF.super.getDisplayAsBot();
}
private String getUsernameInitialize() {
return SlackAccessDeniedFileIF.super.getUsername();
}
private String getUrlPrivateInitialize() {
return SlackAccessDeniedFileIF.super.getUrlPrivate();
}
private String getPermalinkInitialize() {
return SlackAccessDeniedFileIF.super.getPermalink();
}
private int getCommentsCountInitialize() {
return SlackAccessDeniedFileIF.super.getCommentsCount();
}
/**
* @return The value of the {@code fileAccess} attribute
*/
@JsonProperty
@Override
public String getFileAccess() {
return fileAccess;
}
/**
* @return The value of the {@code id} attribute
*/
@JsonProperty
@Override
public String getId() {
InitShim shim = this.initShim;
return shim != null
? shim.getId()
: this.id;
}
/**
* @return The value of the {@code createdEpochSeconds} attribute
*/
@JsonProperty
@Override
public long getCreatedEpochSeconds() {
InitShim shim = this.initShim;
return shim != null
? shim.getCreatedEpochSeconds()
: this.createdEpochSeconds;
}
/**
* @return The value of the {@code timestampEpochSeconds} attribute
*/
@JsonProperty
@Override
public long getTimestampEpochSeconds() {
InitShim shim = this.initShim;
return shim != null
? shim.getTimestampEpochSeconds()
: this.timestampEpochSeconds;
}
/**
* @return The value of the {@code name} attribute
*/
@JsonProperty
@Override
public String getName() {
InitShim shim = this.initShim;
return shim != null
? shim.getName()
: this.name;
}
/**
* @return The value of the {@code title} attribute
*/
@JsonProperty
@Override
public String getTitle() {
InitShim shim = this.initShim;
return shim != null
? shim.getTitle()
: this.title;
}
/**
* @return The value of the {@code mimetype} attribute
*/
@JsonProperty
@Override
public String getMimetype() {
InitShim shim = this.initShim;
return shim != null
? shim.getMimetype()
: this.mimetype;
}
/**
* @return The value of the {@code filetype} attribute
*/
@JsonProperty
@Override
public SlackFileType getFiletype() {
InitShim shim = this.initShim;
return shim != null
? shim.getFiletype()
: this.filetype;
}
/**
* @return The value of the {@code prettyType} attribute
*/
@JsonProperty
@Override
public String getPrettyType() {
InitShim shim = this.initShim;
return shim != null
? shim.getPrettyType()
: this.prettyType;
}
/**
* @return The value of the {@code userId} attribute
*/
@JsonProperty
@Override
public String getUserId() {
InitShim shim = this.initShim;
return shim != null
? shim.getUserId()
: this.userId;
}
/**
* @return The value of the {@code editable} attribute
*/
@JsonProperty
@Override
public boolean isEditable() {
InitShim shim = this.initShim;
return shim != null
? shim.isEditable()
: this.editable;
}
/**
* @return The value of the {@code size} attribute
*/
@JsonProperty
@Override
public long getSize() {
InitShim shim = this.initShim;
return shim != null
? shim.getSize()
: this.size;
}
/**
* @return The value of the {@code mode} attribute
*/
@JsonProperty
@Override
public String getMode() {
InitShim shim = this.initShim;
return shim != null
? shim.getMode()
: this.mode;
}
/**
* @return The value of the {@code external} attribute
*/
@JsonProperty
@Override
public boolean isExternal() {
InitShim shim = this.initShim;
return shim != null
? shim.isExternal()
: this.external;
}
/**
* @return The value of the {@code isPublic} attribute
*/
@JsonProperty
@Override
public boolean isPublic() {
InitShim shim = this.initShim;
return shim != null
? shim.isPublic()
: this.isPublic;
}
/**
* @return The value of the {@code publicUrlShared} attribute
*/
@JsonProperty
@Override
public boolean isPublicUrlShared() {
InitShim shim = this.initShim;
return shim != null
? shim.isPublicUrlShared()
: this.publicUrlShared;
}
/**
* @return The value of the {@code displayAsBot} attribute
*/
@JsonProperty
@Override
public boolean getDisplayAsBot() {
InitShim shim = this.initShim;
return shim != null
? shim.getDisplayAsBot()
: this.displayAsBot;
}
/**
* @return The value of the {@code username} attribute
*/
@JsonProperty
@Override
public String getUsername() {
InitShim shim = this.initShim;
return shim != null
? shim.getUsername()
: this.username;
}
/**
* @return The value of the {@code urlPrivate} attribute
*/
@JsonProperty
@Override
public String getUrlPrivate() {
InitShim shim = this.initShim;
return shim != null
? shim.getUrlPrivate()
: this.urlPrivate;
}
/**
* @return The value of the {@code urlPrivateDownload} attribute
*/
@JsonProperty
@Override
public Optional getUrlPrivateDownload() {
return Optional.ofNullable(urlPrivateDownload);
}
/**
* @return The value of the {@code permalink} attribute
*/
@JsonProperty
@Override
public String getPermalink() {
InitShim shim = this.initShim;
return shim != null
? shim.getPermalink()
: this.permalink;
}
/**
* @return The value of the {@code permalinkPublic} attribute
*/
@JsonProperty
@Override
public Optional getPermalinkPublic() {
return Optional.ofNullable(permalinkPublic);
}
/**
* @return The value of the {@code commentsCount} attribute
*/
@JsonProperty
@Override
public int getCommentsCount() {
InitShim shim = this.initShim;
return shim != null
? shim.getCommentsCount()
: this.commentsCount;
}
/**
* @return The value of the {@code starred} attribute
*/
@JsonProperty
@Override
public Optional isStarred() {
return Optional.ofNullable(starred);
}
/**
* @return The value of the {@code channelIds} attribute
*/
@JsonProperty
@Override
public List getChannelIds() {
return channelIds;
}
/**
* @return The value of the {@code groupIds} attribute
*/
@JsonProperty
@Override
public List getGroupIds() {
return groupIds;
}
/**
* @return The value of the {@code imIds} attribute
*/
@JsonProperty
@Override
public List getImIds() {
return imIds;
}
/**
* Copy the current immutable object by setting a value for the {@link SlackAccessDeniedFileIF#getFileAccess() fileAccess} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for fileAccess
* @return A modified copy of the {@code this} object
*/
public final SlackAccessDeniedFile withFileAccess(String value) {
String newValue = Objects.requireNonNull(value, "fileAccess");
if (this.fileAccess.equals(newValue)) return this;
return new SlackAccessDeniedFile(
newValue,
this.id,
this.createdEpochSeconds,
this.timestampEpochSeconds,
this.name,
this.title,
this.mimetype,
this.filetype,
this.prettyType,
this.userId,
this.editable,
this.size,
this.mode,
this.external,
this.isPublic,
this.publicUrlShared,
this.displayAsBot,
this.username,
this.urlPrivate,
this.urlPrivateDownload,
this.permalink,
this.permalinkPublic,
this.commentsCount,
this.starred,
this.channelIds,
this.groupIds,
this.imIds);
}
/**
* Copy the current immutable object by setting a value for the {@link SlackAccessDeniedFileIF#getId() id} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for id
* @return A modified copy of the {@code this} object
*/
public final SlackAccessDeniedFile withId(String value) {
String newValue = Objects.requireNonNull(value, "id");
if (this.id.equals(newValue)) return this;
return new SlackAccessDeniedFile(
this.fileAccess,
newValue,
this.createdEpochSeconds,
this.timestampEpochSeconds,
this.name,
this.title,
this.mimetype,
this.filetype,
this.prettyType,
this.userId,
this.editable,
this.size,
this.mode,
this.external,
this.isPublic,
this.publicUrlShared,
this.displayAsBot,
this.username,
this.urlPrivate,
this.urlPrivateDownload,
this.permalink,
this.permalinkPublic,
this.commentsCount,
this.starred,
this.channelIds,
this.groupIds,
this.imIds);
}
/**
* Copy the current immutable object by setting a value for the {@link SlackAccessDeniedFileIF#getCreatedEpochSeconds() createdEpochSeconds} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for createdEpochSeconds
* @return A modified copy of the {@code this} object
*/
public final SlackAccessDeniedFile withCreatedEpochSeconds(long value) {
if (this.createdEpochSeconds == value) return this;
return new SlackAccessDeniedFile(
this.fileAccess,
this.id,
value,
this.timestampEpochSeconds,
this.name,
this.title,
this.mimetype,
this.filetype,
this.prettyType,
this.userId,
this.editable,
this.size,
this.mode,
this.external,
this.isPublic,
this.publicUrlShared,
this.displayAsBot,
this.username,
this.urlPrivate,
this.urlPrivateDownload,
this.permalink,
this.permalinkPublic,
this.commentsCount,
this.starred,
this.channelIds,
this.groupIds,
this.imIds);
}
/**
* Copy the current immutable object by setting a value for the {@link SlackAccessDeniedFileIF#getTimestampEpochSeconds() timestampEpochSeconds} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for timestampEpochSeconds
* @return A modified copy of the {@code this} object
*/
public final SlackAccessDeniedFile withTimestampEpochSeconds(long value) {
if (this.timestampEpochSeconds == value) return this;
return new SlackAccessDeniedFile(
this.fileAccess,
this.id,
this.createdEpochSeconds,
value,
this.name,
this.title,
this.mimetype,
this.filetype,
this.prettyType,
this.userId,
this.editable,
this.size,
this.mode,
this.external,
this.isPublic,
this.publicUrlShared,
this.displayAsBot,
this.username,
this.urlPrivate,
this.urlPrivateDownload,
this.permalink,
this.permalinkPublic,
this.commentsCount,
this.starred,
this.channelIds,
this.groupIds,
this.imIds);
}
/**
* Copy the current immutable object by setting a value for the {@link SlackAccessDeniedFileIF#getName() name} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for name
* @return A modified copy of the {@code this} object
*/
public final SlackAccessDeniedFile withName(String value) {
String newValue = Objects.requireNonNull(value, "name");
if (this.name.equals(newValue)) return this;
return new SlackAccessDeniedFile(
this.fileAccess,
this.id,
this.createdEpochSeconds,
this.timestampEpochSeconds,
newValue,
this.title,
this.mimetype,
this.filetype,
this.prettyType,
this.userId,
this.editable,
this.size,
this.mode,
this.external,
this.isPublic,
this.publicUrlShared,
this.displayAsBot,
this.username,
this.urlPrivate,
this.urlPrivateDownload,
this.permalink,
this.permalinkPublic,
this.commentsCount,
this.starred,
this.channelIds,
this.groupIds,
this.imIds);
}
/**
* Copy the current immutable object by setting a value for the {@link SlackAccessDeniedFileIF#getTitle() title} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for title
* @return A modified copy of the {@code this} object
*/
public final SlackAccessDeniedFile withTitle(String value) {
String newValue = Objects.requireNonNull(value, "title");
if (this.title.equals(newValue)) return this;
return new SlackAccessDeniedFile(
this.fileAccess,
this.id,
this.createdEpochSeconds,
this.timestampEpochSeconds,
this.name,
newValue,
this.mimetype,
this.filetype,
this.prettyType,
this.userId,
this.editable,
this.size,
this.mode,
this.external,
this.isPublic,
this.publicUrlShared,
this.displayAsBot,
this.username,
this.urlPrivate,
this.urlPrivateDownload,
this.permalink,
this.permalinkPublic,
this.commentsCount,
this.starred,
this.channelIds,
this.groupIds,
this.imIds);
}
/**
* Copy the current immutable object by setting a value for the {@link SlackAccessDeniedFileIF#getMimetype() mimetype} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for mimetype
* @return A modified copy of the {@code this} object
*/
public final SlackAccessDeniedFile withMimetype(String value) {
String newValue = Objects.requireNonNull(value, "mimetype");
if (this.mimetype.equals(newValue)) return this;
return new SlackAccessDeniedFile(
this.fileAccess,
this.id,
this.createdEpochSeconds,
this.timestampEpochSeconds,
this.name,
this.title,
newValue,
this.filetype,
this.prettyType,
this.userId,
this.editable,
this.size,
this.mode,
this.external,
this.isPublic,
this.publicUrlShared,
this.displayAsBot,
this.username,
this.urlPrivate,
this.urlPrivateDownload,
this.permalink,
this.permalinkPublic,
this.commentsCount,
this.starred,
this.channelIds,
this.groupIds,
this.imIds);
}
/**
* Copy the current immutable object by setting a value for the {@link SlackAccessDeniedFileIF#getFiletype() filetype} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for filetype
* @return A modified copy of the {@code this} object
*/
public final SlackAccessDeniedFile withFiletype(SlackFileType value) {
SlackFileType newValue = Objects.requireNonNull(value, "filetype");
if (this.filetype == newValue) return this;
return new SlackAccessDeniedFile(
this.fileAccess,
this.id,
this.createdEpochSeconds,
this.timestampEpochSeconds,
this.name,
this.title,
this.mimetype,
newValue,
this.prettyType,
this.userId,
this.editable,
this.size,
this.mode,
this.external,
this.isPublic,
this.publicUrlShared,
this.displayAsBot,
this.username,
this.urlPrivate,
this.urlPrivateDownload,
this.permalink,
this.permalinkPublic,
this.commentsCount,
this.starred,
this.channelIds,
this.groupIds,
this.imIds);
}
/**
* Copy the current immutable object by setting a value for the {@link SlackAccessDeniedFileIF#getPrettyType() prettyType} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for prettyType
* @return A modified copy of the {@code this} object
*/
public final SlackAccessDeniedFile withPrettyType(String value) {
String newValue = Objects.requireNonNull(value, "prettyType");
if (this.prettyType.equals(newValue)) return this;
return new SlackAccessDeniedFile(
this.fileAccess,
this.id,
this.createdEpochSeconds,
this.timestampEpochSeconds,
this.name,
this.title,
this.mimetype,
this.filetype,
newValue,
this.userId,
this.editable,
this.size,
this.mode,
this.external,
this.isPublic,
this.publicUrlShared,
this.displayAsBot,
this.username,
this.urlPrivate,
this.urlPrivateDownload,
this.permalink,
this.permalinkPublic,
this.commentsCount,
this.starred,
this.channelIds,
this.groupIds,
this.imIds);
}
/**
* Copy the current immutable object by setting a value for the {@link SlackAccessDeniedFileIF#getUserId() userId} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for userId
* @return A modified copy of the {@code this} object
*/
public final SlackAccessDeniedFile withUserId(String value) {
String newValue = Objects.requireNonNull(value, "userId");
if (this.userId.equals(newValue)) return this;
return new SlackAccessDeniedFile(
this.fileAccess,
this.id,
this.createdEpochSeconds,
this.timestampEpochSeconds,
this.name,
this.title,
this.mimetype,
this.filetype,
this.prettyType,
newValue,
this.editable,
this.size,
this.mode,
this.external,
this.isPublic,
this.publicUrlShared,
this.displayAsBot,
this.username,
this.urlPrivate,
this.urlPrivateDownload,
this.permalink,
this.permalinkPublic,
this.commentsCount,
this.starred,
this.channelIds,
this.groupIds,
this.imIds);
}
/**
* Copy the current immutable object by setting a value for the {@link SlackAccessDeniedFileIF#isEditable() editable} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for editable
* @return A modified copy of the {@code this} object
*/
public final SlackAccessDeniedFile withEditable(boolean value) {
if (this.editable == value) return this;
return new SlackAccessDeniedFile(
this.fileAccess,
this.id,
this.createdEpochSeconds,
this.timestampEpochSeconds,
this.name,
this.title,
this.mimetype,
this.filetype,
this.prettyType,
this.userId,
value,
this.size,
this.mode,
this.external,
this.isPublic,
this.publicUrlShared,
this.displayAsBot,
this.username,
this.urlPrivate,
this.urlPrivateDownload,
this.permalink,
this.permalinkPublic,
this.commentsCount,
this.starred,
this.channelIds,
this.groupIds,
this.imIds);
}
/**
* Copy the current immutable object by setting a value for the {@link SlackAccessDeniedFileIF#getSize() size} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for size
* @return A modified copy of the {@code this} object
*/
public final SlackAccessDeniedFile withSize(long value) {
if (this.size == value) return this;
return new SlackAccessDeniedFile(
this.fileAccess,
this.id,
this.createdEpochSeconds,
this.timestampEpochSeconds,
this.name,
this.title,
this.mimetype,
this.filetype,
this.prettyType,
this.userId,
this.editable,
value,
this.mode,
this.external,
this.isPublic,
this.publicUrlShared,
this.displayAsBot,
this.username,
this.urlPrivate,
this.urlPrivateDownload,
this.permalink,
this.permalinkPublic,
this.commentsCount,
this.starred,
this.channelIds,
this.groupIds,
this.imIds);
}
/**
* Copy the current immutable object by setting a value for the {@link SlackAccessDeniedFileIF#getMode() mode} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for mode
* @return A modified copy of the {@code this} object
*/
public final SlackAccessDeniedFile withMode(String value) {
String newValue = Objects.requireNonNull(value, "mode");
if (this.mode.equals(newValue)) return this;
return new SlackAccessDeniedFile(
this.fileAccess,
this.id,
this.createdEpochSeconds,
this.timestampEpochSeconds,
this.name,
this.title,
this.mimetype,
this.filetype,
this.prettyType,
this.userId,
this.editable,
this.size,
newValue,
this.external,
this.isPublic,
this.publicUrlShared,
this.displayAsBot,
this.username,
this.urlPrivate,
this.urlPrivateDownload,
this.permalink,
this.permalinkPublic,
this.commentsCount,
this.starred,
this.channelIds,
this.groupIds,
this.imIds);
}
/**
* Copy the current immutable object by setting a value for the {@link SlackAccessDeniedFileIF#isExternal() external} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for external
* @return A modified copy of the {@code this} object
*/
public final SlackAccessDeniedFile withExternal(boolean value) {
if (this.external == value) return this;
return new SlackAccessDeniedFile(
this.fileAccess,
this.id,
this.createdEpochSeconds,
this.timestampEpochSeconds,
this.name,
this.title,
this.mimetype,
this.filetype,
this.prettyType,
this.userId,
this.editable,
this.size,
this.mode,
value,
this.isPublic,
this.publicUrlShared,
this.displayAsBot,
this.username,
this.urlPrivate,
this.urlPrivateDownload,
this.permalink,
this.permalinkPublic,
this.commentsCount,
this.starred,
this.channelIds,
this.groupIds,
this.imIds);
}
/**
* Copy the current immutable object by setting a value for the {@link SlackAccessDeniedFileIF#isPublic() public} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for isPublic
* @return A modified copy of the {@code this} object
*/
public final SlackAccessDeniedFile withPublic(boolean value) {
if (this.isPublic == value) return this;
return new SlackAccessDeniedFile(
this.fileAccess,
this.id,
this.createdEpochSeconds,
this.timestampEpochSeconds,
this.name,
this.title,
this.mimetype,
this.filetype,
this.prettyType,
this.userId,
this.editable,
this.size,
this.mode,
this.external,
value,
this.publicUrlShared,
this.displayAsBot,
this.username,
this.urlPrivate,
this.urlPrivateDownload,
this.permalink,
this.permalinkPublic,
this.commentsCount,
this.starred,
this.channelIds,
this.groupIds,
this.imIds);
}
/**
* Copy the current immutable object by setting a value for the {@link SlackAccessDeniedFileIF#isPublicUrlShared() publicUrlShared} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for publicUrlShared
* @return A modified copy of the {@code this} object
*/
public final SlackAccessDeniedFile withPublicUrlShared(boolean value) {
if (this.publicUrlShared == value) return this;
return new SlackAccessDeniedFile(
this.fileAccess,
this.id,
this.createdEpochSeconds,
this.timestampEpochSeconds,
this.name,
this.title,
this.mimetype,
this.filetype,
this.prettyType,
this.userId,
this.editable,
this.size,
this.mode,
this.external,
this.isPublic,
value,
this.displayAsBot,
this.username,
this.urlPrivate,
this.urlPrivateDownload,
this.permalink,
this.permalinkPublic,
this.commentsCount,
this.starred,
this.channelIds,
this.groupIds,
this.imIds);
}
/**
* Copy the current immutable object by setting a value for the {@link SlackAccessDeniedFileIF#getDisplayAsBot() displayAsBot} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for displayAsBot
* @return A modified copy of the {@code this} object
*/
public final SlackAccessDeniedFile withDisplayAsBot(boolean value) {
if (this.displayAsBot == value) return this;
return new SlackAccessDeniedFile(
this.fileAccess,
this.id,
this.createdEpochSeconds,
this.timestampEpochSeconds,
this.name,
this.title,
this.mimetype,
this.filetype,
this.prettyType,
this.userId,
this.editable,
this.size,
this.mode,
this.external,
this.isPublic,
this.publicUrlShared,
value,
this.username,
this.urlPrivate,
this.urlPrivateDownload,
this.permalink,
this.permalinkPublic,
this.commentsCount,
this.starred,
this.channelIds,
this.groupIds,
this.imIds);
}
/**
* Copy the current immutable object by setting a value for the {@link SlackAccessDeniedFileIF#getUsername() username} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for username
* @return A modified copy of the {@code this} object
*/
public final SlackAccessDeniedFile withUsername(String value) {
String newValue = Objects.requireNonNull(value, "username");
if (this.username.equals(newValue)) return this;
return new SlackAccessDeniedFile(
this.fileAccess,
this.id,
this.createdEpochSeconds,
this.timestampEpochSeconds,
this.name,
this.title,
this.mimetype,
this.filetype,
this.prettyType,
this.userId,
this.editable,
this.size,
this.mode,
this.external,
this.isPublic,
this.publicUrlShared,
this.displayAsBot,
newValue,
this.urlPrivate,
this.urlPrivateDownload,
this.permalink,
this.permalinkPublic,
this.commentsCount,
this.starred,
this.channelIds,
this.groupIds,
this.imIds);
}
/**
* Copy the current immutable object by setting a value for the {@link SlackAccessDeniedFileIF#getUrlPrivate() urlPrivate} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for urlPrivate
* @return A modified copy of the {@code this} object
*/
public final SlackAccessDeniedFile withUrlPrivate(String value) {
String newValue = Objects.requireNonNull(value, "urlPrivate");
if (this.urlPrivate.equals(newValue)) return this;
return new SlackAccessDeniedFile(
this.fileAccess,
this.id,
this.createdEpochSeconds,
this.timestampEpochSeconds,
this.name,
this.title,
this.mimetype,
this.filetype,
this.prettyType,
this.userId,
this.editable,
this.size,
this.mode,
this.external,
this.isPublic,
this.publicUrlShared,
this.displayAsBot,
this.username,
newValue,
this.urlPrivateDownload,
this.permalink,
this.permalinkPublic,
this.commentsCount,
this.starred,
this.channelIds,
this.groupIds,
this.imIds);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link SlackAccessDeniedFileIF#getUrlPrivateDownload() urlPrivateDownload} attribute.
* @param value The value for urlPrivateDownload, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final SlackAccessDeniedFile withUrlPrivateDownload(@Nullable String value) {
@Nullable String newValue = value;
if (Objects.equals(this.urlPrivateDownload, newValue)) return this;
return new SlackAccessDeniedFile(
this.fileAccess,
this.id,
this.createdEpochSeconds,
this.timestampEpochSeconds,
this.name,
this.title,
this.mimetype,
this.filetype,
this.prettyType,
this.userId,
this.editable,
this.size,
this.mode,
this.external,
this.isPublic,
this.publicUrlShared,
this.displayAsBot,
this.username,
this.urlPrivate,
newValue,
this.permalink,
this.permalinkPublic,
this.commentsCount,
this.starred,
this.channelIds,
this.groupIds,
this.imIds);
}
/**
* Copy the current immutable object by setting an optional value for the {@link SlackAccessDeniedFileIF#getUrlPrivateDownload() urlPrivateDownload} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for urlPrivateDownload
* @return A modified copy of {@code this} object
*/
public final SlackAccessDeniedFile withUrlPrivateDownload(Optional optional) {
@Nullable String value = optional.orElse(null);
if (Objects.equals(this.urlPrivateDownload, value)) return this;
return new SlackAccessDeniedFile(
this.fileAccess,
this.id,
this.createdEpochSeconds,
this.timestampEpochSeconds,
this.name,
this.title,
this.mimetype,
this.filetype,
this.prettyType,
this.userId,
this.editable,
this.size,
this.mode,
this.external,
this.isPublic,
this.publicUrlShared,
this.displayAsBot,
this.username,
this.urlPrivate,
value,
this.permalink,
this.permalinkPublic,
this.commentsCount,
this.starred,
this.channelIds,
this.groupIds,
this.imIds);
}
/**
* Copy the current immutable object by setting a value for the {@link SlackAccessDeniedFileIF#getPermalink() permalink} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for permalink
* @return A modified copy of the {@code this} object
*/
public final SlackAccessDeniedFile withPermalink(String value) {
String newValue = Objects.requireNonNull(value, "permalink");
if (this.permalink.equals(newValue)) return this;
return new SlackAccessDeniedFile(
this.fileAccess,
this.id,
this.createdEpochSeconds,
this.timestampEpochSeconds,
this.name,
this.title,
this.mimetype,
this.filetype,
this.prettyType,
this.userId,
this.editable,
this.size,
this.mode,
this.external,
this.isPublic,
this.publicUrlShared,
this.displayAsBot,
this.username,
this.urlPrivate,
this.urlPrivateDownload,
newValue,
this.permalinkPublic,
this.commentsCount,
this.starred,
this.channelIds,
this.groupIds,
this.imIds);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link SlackAccessDeniedFileIF#getPermalinkPublic() permalinkPublic} attribute.
* @param value The value for permalinkPublic, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final SlackAccessDeniedFile withPermalinkPublic(@Nullable String value) {
@Nullable String newValue = value;
if (Objects.equals(this.permalinkPublic, newValue)) return this;
return new SlackAccessDeniedFile(
this.fileAccess,
this.id,
this.createdEpochSeconds,
this.timestampEpochSeconds,
this.name,
this.title,
this.mimetype,
this.filetype,
this.prettyType,
this.userId,
this.editable,
this.size,
this.mode,
this.external,
this.isPublic,
this.publicUrlShared,
this.displayAsBot,
this.username,
this.urlPrivate,
this.urlPrivateDownload,
this.permalink,
newValue,
this.commentsCount,
this.starred,
this.channelIds,
this.groupIds,
this.imIds);
}
/**
* Copy the current immutable object by setting an optional value for the {@link SlackAccessDeniedFileIF#getPermalinkPublic() permalinkPublic} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for permalinkPublic
* @return A modified copy of {@code this} object
*/
public final SlackAccessDeniedFile withPermalinkPublic(Optional optional) {
@Nullable String value = optional.orElse(null);
if (Objects.equals(this.permalinkPublic, value)) return this;
return new SlackAccessDeniedFile(
this.fileAccess,
this.id,
this.createdEpochSeconds,
this.timestampEpochSeconds,
this.name,
this.title,
this.mimetype,
this.filetype,
this.prettyType,
this.userId,
this.editable,
this.size,
this.mode,
this.external,
this.isPublic,
this.publicUrlShared,
this.displayAsBot,
this.username,
this.urlPrivate,
this.urlPrivateDownload,
this.permalink,
value,
this.commentsCount,
this.starred,
this.channelIds,
this.groupIds,
this.imIds);
}
/**
* Copy the current immutable object by setting a value for the {@link SlackAccessDeniedFileIF#getCommentsCount() commentsCount} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for commentsCount
* @return A modified copy of the {@code this} object
*/
public final SlackAccessDeniedFile withCommentsCount(int value) {
if (this.commentsCount == value) return this;
return new SlackAccessDeniedFile(
this.fileAccess,
this.id,
this.createdEpochSeconds,
this.timestampEpochSeconds,
this.name,
this.title,
this.mimetype,
this.filetype,
this.prettyType,
this.userId,
this.editable,
this.size,
this.mode,
this.external,
this.isPublic,
this.publicUrlShared,
this.displayAsBot,
this.username,
this.urlPrivate,
this.urlPrivateDownload,
this.permalink,
this.permalinkPublic,
value,
this.starred,
this.channelIds,
this.groupIds,
this.imIds);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link SlackAccessDeniedFileIF#isStarred() starred} attribute.
* @param value The value for starred, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final SlackAccessDeniedFile withStarred(@Nullable Boolean value) {
@Nullable Boolean newValue = value;
if (Objects.equals(this.starred, newValue)) return this;
return new SlackAccessDeniedFile(
this.fileAccess,
this.id,
this.createdEpochSeconds,
this.timestampEpochSeconds,
this.name,
this.title,
this.mimetype,
this.filetype,
this.prettyType,
this.userId,
this.editable,
this.size,
this.mode,
this.external,
this.isPublic,
this.publicUrlShared,
this.displayAsBot,
this.username,
this.urlPrivate,
this.urlPrivateDownload,
this.permalink,
this.permalinkPublic,
this.commentsCount,
newValue,
this.channelIds,
this.groupIds,
this.imIds);
}
/**
* Copy the current immutable object by setting an optional value for the {@link SlackAccessDeniedFileIF#isStarred() starred} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for starred
* @return A modified copy of {@code this} object
*/
public final SlackAccessDeniedFile withStarred(Optional optional) {
@Nullable Boolean value = optional.orElse(null);
if (Objects.equals(this.starred, value)) return this;
return new SlackAccessDeniedFile(
this.fileAccess,
this.id,
this.createdEpochSeconds,
this.timestampEpochSeconds,
this.name,
this.title,
this.mimetype,
this.filetype,
this.prettyType,
this.userId,
this.editable,
this.size,
this.mode,
this.external,
this.isPublic,
this.publicUrlShared,
this.displayAsBot,
this.username,
this.urlPrivate,
this.urlPrivateDownload,
this.permalink,
this.permalinkPublic,
this.commentsCount,
value,
this.channelIds,
this.groupIds,
this.imIds);
}
/**
* Copy the current immutable object with elements that replace the content of {@link SlackAccessDeniedFileIF#getChannelIds() channelIds}.
* @param elements The elements to set
* @return A modified copy of {@code this} object
*/
public final SlackAccessDeniedFile withChannelIds(String... elements) {
List newValue = createUnmodifiableList(false, createSafeList(Arrays.asList(elements), true, false));
return new SlackAccessDeniedFile(
this.fileAccess,
this.id,
this.createdEpochSeconds,
this.timestampEpochSeconds,
this.name,
this.title,
this.mimetype,
this.filetype,
this.prettyType,
this.userId,
this.editable,
this.size,
this.mode,
this.external,
this.isPublic,
this.publicUrlShared,
this.displayAsBot,
this.username,
this.urlPrivate,
this.urlPrivateDownload,
this.permalink,
this.permalinkPublic,
this.commentsCount,
this.starred,
newValue,
this.groupIds,
this.imIds);
}
/**
* Copy the current immutable object with elements that replace the content of {@link SlackAccessDeniedFileIF#getChannelIds() channelIds}.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements An iterable of channelIds elements to set
* @return A modified copy of {@code this} object
*/
public final SlackAccessDeniedFile withChannelIds(Iterable elements) {
if (this.channelIds == elements) return this;
List newValue = createUnmodifiableList(false, createSafeList(elements, true, false));
return new SlackAccessDeniedFile(
this.fileAccess,
this.id,
this.createdEpochSeconds,
this.timestampEpochSeconds,
this.name,
this.title,
this.mimetype,
this.filetype,
this.prettyType,
this.userId,
this.editable,
this.size,
this.mode,
this.external,
this.isPublic,
this.publicUrlShared,
this.displayAsBot,
this.username,
this.urlPrivate,
this.urlPrivateDownload,
this.permalink,
this.permalinkPublic,
this.commentsCount,
this.starred,
newValue,
this.groupIds,
this.imIds);
}
/**
* Copy the current immutable object with elements that replace the content of {@link SlackAccessDeniedFileIF#getGroupIds() groupIds}.
* @param elements The elements to set
* @return A modified copy of {@code this} object
*/
public final SlackAccessDeniedFile withGroupIds(String... elements) {
List newValue = createUnmodifiableList(false, createSafeList(Arrays.asList(elements), true, false));
return new SlackAccessDeniedFile(
this.fileAccess,
this.id,
this.createdEpochSeconds,
this.timestampEpochSeconds,
this.name,
this.title,
this.mimetype,
this.filetype,
this.prettyType,
this.userId,
this.editable,
this.size,
this.mode,
this.external,
this.isPublic,
this.publicUrlShared,
this.displayAsBot,
this.username,
this.urlPrivate,
this.urlPrivateDownload,
this.permalink,
this.permalinkPublic,
this.commentsCount,
this.starred,
this.channelIds,
newValue,
this.imIds);
}
/**
* Copy the current immutable object with elements that replace the content of {@link SlackAccessDeniedFileIF#getGroupIds() groupIds}.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements An iterable of groupIds elements to set
* @return A modified copy of {@code this} object
*/
public final SlackAccessDeniedFile withGroupIds(Iterable elements) {
if (this.groupIds == elements) return this;
List newValue = createUnmodifiableList(false, createSafeList(elements, true, false));
return new SlackAccessDeniedFile(
this.fileAccess,
this.id,
this.createdEpochSeconds,
this.timestampEpochSeconds,
this.name,
this.title,
this.mimetype,
this.filetype,
this.prettyType,
this.userId,
this.editable,
this.size,
this.mode,
this.external,
this.isPublic,
this.publicUrlShared,
this.displayAsBot,
this.username,
this.urlPrivate,
this.urlPrivateDownload,
this.permalink,
this.permalinkPublic,
this.commentsCount,
this.starred,
this.channelIds,
newValue,
this.imIds);
}
/**
* Copy the current immutable object with elements that replace the content of {@link SlackAccessDeniedFileIF#getImIds() imIds}.
* @param elements The elements to set
* @return A modified copy of {@code this} object
*/
public final SlackAccessDeniedFile withImIds(String... elements) {
List newValue = createUnmodifiableList(false, createSafeList(Arrays.asList(elements), true, false));
return new SlackAccessDeniedFile(
this.fileAccess,
this.id,
this.createdEpochSeconds,
this.timestampEpochSeconds,
this.name,
this.title,
this.mimetype,
this.filetype,
this.prettyType,
this.userId,
this.editable,
this.size,
this.mode,
this.external,
this.isPublic,
this.publicUrlShared,
this.displayAsBot,
this.username,
this.urlPrivate,
this.urlPrivateDownload,
this.permalink,
this.permalinkPublic,
this.commentsCount,
this.starred,
this.channelIds,
this.groupIds,
newValue);
}
/**
* Copy the current immutable object with elements that replace the content of {@link SlackAccessDeniedFileIF#getImIds() imIds}.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements An iterable of imIds elements to set
* @return A modified copy of {@code this} object
*/
public final SlackAccessDeniedFile withImIds(Iterable elements) {
if (this.imIds == elements) return this;
List newValue = createUnmodifiableList(false, createSafeList(elements, true, false));
return new SlackAccessDeniedFile(
this.fileAccess,
this.id,
this.createdEpochSeconds,
this.timestampEpochSeconds,
this.name,
this.title,
this.mimetype,
this.filetype,
this.prettyType,
this.userId,
this.editable,
this.size,
this.mode,
this.external,
this.isPublic,
this.publicUrlShared,
this.displayAsBot,
this.username,
this.urlPrivate,
this.urlPrivateDownload,
this.permalink,
this.permalinkPublic,
this.commentsCount,
this.starred,
this.channelIds,
this.groupIds,
newValue);
}
/**
* This instance is equal to all instances of {@code SlackAccessDeniedFile} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(@Nullable Object another) {
if (this == another) return true;
return another instanceof SlackAccessDeniedFile
&& equalTo(0, (SlackAccessDeniedFile) another);
}
private boolean equalTo(int synthetic, SlackAccessDeniedFile another) {
return fileAccess.equals(another.fileAccess)
&& id.equals(another.id)
&& createdEpochSeconds == another.createdEpochSeconds
&& timestampEpochSeconds == another.timestampEpochSeconds
&& name.equals(another.name)
&& title.equals(another.title)
&& mimetype.equals(another.mimetype)
&& filetype.equals(another.filetype)
&& prettyType.equals(another.prettyType)
&& userId.equals(another.userId)
&& editable == another.editable
&& size == another.size
&& mode.equals(another.mode)
&& external == another.external
&& isPublic == another.isPublic
&& publicUrlShared == another.publicUrlShared
&& displayAsBot == another.displayAsBot
&& username.equals(another.username)
&& urlPrivate.equals(another.urlPrivate)
&& Objects.equals(urlPrivateDownload, another.urlPrivateDownload)
&& permalink.equals(another.permalink)
&& Objects.equals(permalinkPublic, another.permalinkPublic)
&& commentsCount == another.commentsCount
&& Objects.equals(starred, another.starred)
&& channelIds.equals(another.channelIds)
&& groupIds.equals(another.groupIds)
&& imIds.equals(another.imIds);
}
/**
* Computes a hash code from attributes: {@code fileAccess}, {@code id}, {@code createdEpochSeconds}, {@code timestampEpochSeconds}, {@code name}, {@code title}, {@code mimetype}, {@code filetype}, {@code prettyType}, {@code userId}, {@code editable}, {@code size}, {@code mode}, {@code external}, {@code isPublic}, {@code publicUrlShared}, {@code displayAsBot}, {@code username}, {@code urlPrivate}, {@code urlPrivateDownload}, {@code permalink}, {@code permalinkPublic}, {@code commentsCount}, {@code starred}, {@code channelIds}, {@code groupIds}, {@code imIds}.
* @return hashCode value
*/
@Override
public int hashCode() {
int h = 5381;
h += (h << 5) + fileAccess.hashCode();
h += (h << 5) + id.hashCode();
h += (h << 5) + Long.hashCode(createdEpochSeconds);
h += (h << 5) + Long.hashCode(timestampEpochSeconds);
h += (h << 5) + name.hashCode();
h += (h << 5) + title.hashCode();
h += (h << 5) + mimetype.hashCode();
h += (h << 5) + filetype.hashCode();
h += (h << 5) + prettyType.hashCode();
h += (h << 5) + userId.hashCode();
h += (h << 5) + Boolean.hashCode(editable);
h += (h << 5) + Long.hashCode(size);
h += (h << 5) + mode.hashCode();
h += (h << 5) + Boolean.hashCode(external);
h += (h << 5) + Boolean.hashCode(isPublic);
h += (h << 5) + Boolean.hashCode(publicUrlShared);
h += (h << 5) + Boolean.hashCode(displayAsBot);
h += (h << 5) + username.hashCode();
h += (h << 5) + urlPrivate.hashCode();
h += (h << 5) + Objects.hashCode(urlPrivateDownload);
h += (h << 5) + permalink.hashCode();
h += (h << 5) + Objects.hashCode(permalinkPublic);
h += (h << 5) + commentsCount;
h += (h << 5) + Objects.hashCode(starred);
h += (h << 5) + channelIds.hashCode();
h += (h << 5) + groupIds.hashCode();
h += (h << 5) + imIds.hashCode();
return h;
}
/**
* Prints the immutable value {@code SlackAccessDeniedFile} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
StringBuilder builder = new StringBuilder("SlackAccessDeniedFile{");
builder.append("fileAccess=").append(fileAccess);
builder.append(", ");
builder.append("id=").append(id);
builder.append(", ");
builder.append("createdEpochSeconds=").append(createdEpochSeconds);
builder.append(", ");
builder.append("timestampEpochSeconds=").append(timestampEpochSeconds);
builder.append(", ");
builder.append("name=").append(name);
builder.append(", ");
builder.append("title=").append(title);
builder.append(", ");
builder.append("mimetype=").append(mimetype);
builder.append(", ");
builder.append("filetype=").append(filetype);
builder.append(", ");
builder.append("prettyType=").append(prettyType);
builder.append(", ");
builder.append("userId=").append(userId);
builder.append(", ");
builder.append("editable=").append(editable);
builder.append(", ");
builder.append("size=").append(size);
builder.append(", ");
builder.append("mode=").append(mode);
builder.append(", ");
builder.append("external=").append(external);
builder.append(", ");
builder.append("public=").append(isPublic);
builder.append(", ");
builder.append("publicUrlShared=").append(publicUrlShared);
builder.append(", ");
builder.append("displayAsBot=").append(displayAsBot);
builder.append(", ");
builder.append("username=").append(username);
builder.append(", ");
builder.append("urlPrivate=").append(urlPrivate);
if (urlPrivateDownload != null) {
builder.append(", ");
builder.append("urlPrivateDownload=").append(urlPrivateDownload);
}
builder.append(", ");
builder.append("permalink=").append(permalink);
if (permalinkPublic != null) {
builder.append(", ");
builder.append("permalinkPublic=").append(permalinkPublic);
}
builder.append(", ");
builder.append("commentsCount=").append(commentsCount);
if (starred != null) {
builder.append(", ");
builder.append("starred=").append(starred);
}
builder.append(", ");
builder.append("channelIds=").append(channelIds);
builder.append(", ");
builder.append("groupIds=").append(groupIds);
builder.append(", ");
builder.append("imIds=").append(imIds);
return builder.append("}").toString();
}
/**
* Utility type used to correctly read immutable object from JSON representation.
* @deprecated Do not use this type directly, it exists only for the Jackson-binding infrastructure
*/
@Generated(from = "SlackAccessDeniedFileIF", generator = "Immutables")
@Deprecated
@JsonDeserialize
@JsonAutoDetect(fieldVisibility = JsonAutoDetect.Visibility.NONE)
static final class Json implements SlackAccessDeniedFileIF {
@Nullable String fileAccess;
@Nullable String id;
long createdEpochSeconds;
boolean createdEpochSecondsIsSet;
long timestampEpochSeconds;
boolean timestampEpochSecondsIsSet;
@Nullable String name;
@Nullable String title;
@Nullable String mimetype;
@Nullable SlackFileType filetype;
@Nullable String prettyType;
@Nullable String userId;
boolean editable;
boolean editableIsSet;
long size;
boolean sizeIsSet;
@Nullable String mode;
boolean external;
boolean externalIsSet;
boolean isPublic;
boolean isPublicIsSet;
boolean publicUrlShared;
boolean publicUrlSharedIsSet;
boolean displayAsBot;
boolean displayAsBotIsSet;
@Nullable String username;
@Nullable String urlPrivate;
@Nullable Optional urlPrivateDownload = Optional.empty();
@Nullable String permalink;
@Nullable Optional permalinkPublic = Optional.empty();
int commentsCount;
boolean commentsCountIsSet;
@Nullable Optional starred = Optional.empty();
@Nullable List channelIds = Collections.emptyList();
@Nullable List groupIds = Collections.emptyList();
@Nullable List imIds = Collections.emptyList();
@JsonProperty
public void setFileAccess(String fileAccess) {
this.fileAccess = fileAccess;
}
@JsonProperty
public void setId(String id) {
this.id = id;
}
@JsonProperty
public void setCreatedEpochSeconds(long createdEpochSeconds) {
this.createdEpochSeconds = createdEpochSeconds;
this.createdEpochSecondsIsSet = true;
}
@JsonProperty
public void setTimestampEpochSeconds(long timestampEpochSeconds) {
this.timestampEpochSeconds = timestampEpochSeconds;
this.timestampEpochSecondsIsSet = true;
}
@JsonProperty
public void setName(String name) {
this.name = name;
}
@JsonProperty
public void setTitle(String title) {
this.title = title;
}
@JsonProperty
public void setMimetype(String mimetype) {
this.mimetype = mimetype;
}
@JsonProperty
public void setFiletype(SlackFileType filetype) {
this.filetype = filetype;
}
@JsonProperty
public void setPrettyType(String prettyType) {
this.prettyType = prettyType;
}
@JsonProperty
public void setUserId(String userId) {
this.userId = userId;
}
@JsonProperty
public void setEditable(boolean editable) {
this.editable = editable;
this.editableIsSet = true;
}
@JsonProperty
public void setSize(long size) {
this.size = size;
this.sizeIsSet = true;
}
@JsonProperty
public void setMode(String mode) {
this.mode = mode;
}
@JsonProperty
public void setExternal(boolean external) {
this.external = external;
this.externalIsSet = true;
}
@JsonProperty
public void setPublic(boolean isPublic) {
this.isPublic = isPublic;
this.isPublicIsSet = true;
}
@JsonProperty
public void setPublicUrlShared(boolean publicUrlShared) {
this.publicUrlShared = publicUrlShared;
this.publicUrlSharedIsSet = true;
}
@JsonProperty
public void setDisplayAsBot(boolean displayAsBot) {
this.displayAsBot = displayAsBot;
this.displayAsBotIsSet = true;
}
@JsonProperty
public void setUsername(String username) {
this.username = username;
}
@JsonProperty
public void setUrlPrivate(String urlPrivate) {
this.urlPrivate = urlPrivate;
}
@JsonProperty
public void setUrlPrivateDownload(Optional urlPrivateDownload) {
this.urlPrivateDownload = urlPrivateDownload;
}
@JsonProperty
public void setPermalink(String permalink) {
this.permalink = permalink;
}
@JsonProperty
public void setPermalinkPublic(Optional permalinkPublic) {
this.permalinkPublic = permalinkPublic;
}
@JsonProperty
public void setCommentsCount(int commentsCount) {
this.commentsCount = commentsCount;
this.commentsCountIsSet = true;
}
@JsonProperty
public void setStarred(Optional starred) {
this.starred = starred;
}
@JsonProperty
public void setChannelIds(List channelIds) {
this.channelIds = channelIds;
}
@JsonProperty
public void setGroupIds(List groupIds) {
this.groupIds = groupIds;
}
@JsonProperty
public void setImIds(List imIds) {
this.imIds = imIds;
}
@Override
public String getFileAccess() { throw new UnsupportedOperationException(); }
@Override
public String getId() { throw new UnsupportedOperationException(); }
@Override
public long getCreatedEpochSeconds() { throw new UnsupportedOperationException(); }
@Override
public long getTimestampEpochSeconds() { throw new UnsupportedOperationException(); }
@Override
public String getName() { throw new UnsupportedOperationException(); }
@Override
public String getTitle() { throw new UnsupportedOperationException(); }
@Override
public String getMimetype() { throw new UnsupportedOperationException(); }
@Override
public SlackFileType getFiletype() { throw new UnsupportedOperationException(); }
@Override
public String getPrettyType() { throw new UnsupportedOperationException(); }
@Override
public String getUserId() { throw new UnsupportedOperationException(); }
@Override
public boolean isEditable() { throw new UnsupportedOperationException(); }
@Override
public long getSize() { throw new UnsupportedOperationException(); }
@Override
public String getMode() { throw new UnsupportedOperationException(); }
@Override
public boolean isExternal() { throw new UnsupportedOperationException(); }
@Override
public boolean isPublic() { throw new UnsupportedOperationException(); }
@Override
public boolean isPublicUrlShared() { throw new UnsupportedOperationException(); }
@Override
public boolean getDisplayAsBot() { throw new UnsupportedOperationException(); }
@Override
public String getUsername() { throw new UnsupportedOperationException(); }
@Override
public String getUrlPrivate() { throw new UnsupportedOperationException(); }
@Override
public Optional getUrlPrivateDownload() { throw new UnsupportedOperationException(); }
@Override
public String getPermalink() { throw new UnsupportedOperationException(); }
@Override
public Optional getPermalinkPublic() { throw new UnsupportedOperationException(); }
@Override
public int getCommentsCount() { throw new UnsupportedOperationException(); }
@Override
public Optional isStarred() { throw new UnsupportedOperationException(); }
@Override
public List getChannelIds() { throw new UnsupportedOperationException(); }
@Override
public List getGroupIds() { throw new UnsupportedOperationException(); }
@Override
public List getImIds() { throw new UnsupportedOperationException(); }
}
/**
* @param json A JSON-bindable data structure
* @return An immutable value type
* @deprecated Do not use this method directly, it exists only for the Jackson-binding infrastructure
*/
@Deprecated
@JsonCreator(mode = JsonCreator.Mode.DELEGATING)
static SlackAccessDeniedFile fromJson(Json json) {
SlackAccessDeniedFile.Builder builder = SlackAccessDeniedFile.builder();
if (json.fileAccess != null) {
builder.setFileAccess(json.fileAccess);
}
if (json.id != null) {
builder.setId(json.id);
}
if (json.createdEpochSecondsIsSet) {
builder.setCreatedEpochSeconds(json.createdEpochSeconds);
}
if (json.timestampEpochSecondsIsSet) {
builder.setTimestampEpochSeconds(json.timestampEpochSeconds);
}
if (json.name != null) {
builder.setName(json.name);
}
if (json.title != null) {
builder.setTitle(json.title);
}
if (json.mimetype != null) {
builder.setMimetype(json.mimetype);
}
if (json.filetype != null) {
builder.setFiletype(json.filetype);
}
if (json.prettyType != null) {
builder.setPrettyType(json.prettyType);
}
if (json.userId != null) {
builder.setUserId(json.userId);
}
if (json.editableIsSet) {
builder.setEditable(json.editable);
}
if (json.sizeIsSet) {
builder.setSize(json.size);
}
if (json.mode != null) {
builder.setMode(json.mode);
}
if (json.externalIsSet) {
builder.setExternal(json.external);
}
if (json.isPublicIsSet) {
builder.setPublic(json.isPublic);
}
if (json.publicUrlSharedIsSet) {
builder.setPublicUrlShared(json.publicUrlShared);
}
if (json.displayAsBotIsSet) {
builder.setDisplayAsBot(json.displayAsBot);
}
if (json.username != null) {
builder.setUsername(json.username);
}
if (json.urlPrivate != null) {
builder.setUrlPrivate(json.urlPrivate);
}
if (json.urlPrivateDownload != null) {
builder.setUrlPrivateDownload(json.urlPrivateDownload);
}
if (json.permalink != null) {
builder.setPermalink(json.permalink);
}
if (json.permalinkPublic != null) {
builder.setPermalinkPublic(json.permalinkPublic);
}
if (json.commentsCountIsSet) {
builder.setCommentsCount(json.commentsCount);
}
if (json.starred != null) {
builder.setStarred(json.starred);
}
if (json.channelIds != null) {
builder.addAllChannelIds(json.channelIds);
}
if (json.groupIds != null) {
builder.addAllGroupIds(json.groupIds);
}
if (json.imIds != null) {
builder.addAllImIds(json.imIds);
}
return builder.build();
}
/**
* Creates an immutable copy of a {@link SlackAccessDeniedFileIF} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable SlackAccessDeniedFile instance
*/
public static SlackAccessDeniedFile copyOf(SlackAccessDeniedFileIF instance) {
if (instance instanceof SlackAccessDeniedFile) {
return (SlackAccessDeniedFile) instance;
}
return SlackAccessDeniedFile.builder()
.from(instance)
.build();
}
/**
* Creates a builder for {@link SlackAccessDeniedFile SlackAccessDeniedFile}.
*
* SlackAccessDeniedFile.builder()
* .setFileAccess(String) // required {@link SlackAccessDeniedFileIF#getFileAccess() fileAccess}
* .setId(String) // optional {@link SlackAccessDeniedFileIF#getId() id}
* .setCreatedEpochSeconds(long) // optional {@link SlackAccessDeniedFileIF#getCreatedEpochSeconds() createdEpochSeconds}
* .setTimestampEpochSeconds(long) // optional {@link SlackAccessDeniedFileIF#getTimestampEpochSeconds() timestampEpochSeconds}
* .setName(String) // optional {@link SlackAccessDeniedFileIF#getName() name}
* .setTitle(String) // optional {@link SlackAccessDeniedFileIF#getTitle() title}
* .setMimetype(String) // optional {@link SlackAccessDeniedFileIF#getMimetype() mimetype}
* .setFiletype(com.hubspot.slack.client.models.files.SlackFileType) // optional {@link SlackAccessDeniedFileIF#getFiletype() filetype}
* .setPrettyType(String) // optional {@link SlackAccessDeniedFileIF#getPrettyType() prettyType}
* .setUserId(String) // optional {@link SlackAccessDeniedFileIF#getUserId() userId}
* .setEditable(boolean) // optional {@link SlackAccessDeniedFileIF#isEditable() editable}
* .setSize(long) // optional {@link SlackAccessDeniedFileIF#getSize() size}
* .setMode(String) // optional {@link SlackAccessDeniedFileIF#getMode() mode}
* .setExternal(boolean) // optional {@link SlackAccessDeniedFileIF#isExternal() external}
* .setPublic(boolean) // optional {@link SlackAccessDeniedFileIF#isPublic() public}
* .setPublicUrlShared(boolean) // optional {@link SlackAccessDeniedFileIF#isPublicUrlShared() publicUrlShared}
* .setDisplayAsBot(boolean) // optional {@link SlackAccessDeniedFileIF#getDisplayAsBot() displayAsBot}
* .setUsername(String) // optional {@link SlackAccessDeniedFileIF#getUsername() username}
* .setUrlPrivate(String) // optional {@link SlackAccessDeniedFileIF#getUrlPrivate() urlPrivate}
* .setUrlPrivateDownload(String) // optional {@link SlackAccessDeniedFileIF#getUrlPrivateDownload() urlPrivateDownload}
* .setPermalink(String) // optional {@link SlackAccessDeniedFileIF#getPermalink() permalink}
* .setPermalinkPublic(String) // optional {@link SlackAccessDeniedFileIF#getPermalinkPublic() permalinkPublic}
* .setCommentsCount(int) // optional {@link SlackAccessDeniedFileIF#getCommentsCount() commentsCount}
* .setStarred(Boolean) // optional {@link SlackAccessDeniedFileIF#isStarred() starred}
* .addChannelIds|addAllChannelIds(String) // {@link SlackAccessDeniedFileIF#getChannelIds() channelIds} elements
* .addGroupIds|addAllGroupIds(String) // {@link SlackAccessDeniedFileIF#getGroupIds() groupIds} elements
* .addImIds|addAllImIds(String) // {@link SlackAccessDeniedFileIF#getImIds() imIds} elements
* .build();
*
* @return A new SlackAccessDeniedFile builder
*/
public static SlackAccessDeniedFile.Builder builder() {
return new SlackAccessDeniedFile.Builder();
}
/**
* Builds instances of type {@link SlackAccessDeniedFile SlackAccessDeniedFile}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
@Generated(from = "SlackAccessDeniedFileIF", generator = "Immutables")
@NotThreadSafe
public static final class Builder {
private static final long INIT_BIT_FILE_ACCESS = 0x1L;
private static final long OPT_BIT_CREATED_EPOCH_SECONDS = 0x1L;
private static final long OPT_BIT_TIMESTAMP_EPOCH_SECONDS = 0x2L;
private static final long OPT_BIT_EDITABLE = 0x4L;
private static final long OPT_BIT_SIZE = 0x8L;
private static final long OPT_BIT_EXTERNAL = 0x10L;
private static final long OPT_BIT_IS_PUBLIC = 0x20L;
private static final long OPT_BIT_PUBLIC_URL_SHARED = 0x40L;
private static final long OPT_BIT_DISPLAY_AS_BOT = 0x80L;
private static final long OPT_BIT_COMMENTS_COUNT = 0x100L;
private long initBits = 0x1L;
private long optBits;
private @Nullable String fileAccess;
private @Nullable String id;
private long createdEpochSeconds;
private long timestampEpochSeconds;
private @Nullable String name;
private @Nullable String title;
private @Nullable String mimetype;
private @Nullable SlackFileType filetype;
private @Nullable String prettyType;
private @Nullable String userId;
private boolean editable;
private long size;
private @Nullable String mode;
private boolean external;
private boolean isPublic;
private boolean publicUrlShared;
private boolean displayAsBot;
private @Nullable String username;
private @Nullable String urlPrivate;
private @Nullable String urlPrivateDownload;
private @Nullable String permalink;
private @Nullable String permalinkPublic;
private int commentsCount;
private @Nullable Boolean starred;
private List channelIds = new ArrayList();
private List groupIds = new ArrayList();
private List imIds = new ArrayList();
private Builder() {
}
/**
* Fill a builder with attribute values from the provided {@code com.hubspot.slack.client.models.files.SlackFileError} instance.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(SlackFileError instance) {
Objects.requireNonNull(instance, "instance");
from((short) 0, (Object) instance);
return this;
}
/**
* Fill a builder with attribute values from the provided {@code com.hubspot.slack.client.models.files.SlackAccessDeniedFileIF} instance.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(SlackAccessDeniedFileIF instance) {
Objects.requireNonNull(instance, "instance");
from((short) 0, (Object) instance);
return this;
}
/**
* Fill a builder with attribute values from the provided {@code com.hubspot.slack.client.models.files.SlackFile} instance.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(SlackFile instance) {
Objects.requireNonNull(instance, "instance");
from((short) 0, (Object) instance);
return this;
}
private void from(short _unused, Object object) {
long bits = 0;
if (object instanceof SlackFileError) {
SlackFileError instance = (SlackFileError) object;
if ((bits & 0x1L) == 0) {
this.setFiletype(instance.getFiletype());
bits |= 0x1L;
}
if ((bits & 0x2L) == 0) {
this.setTitle(instance.getTitle());
bits |= 0x2L;
}
if ((bits & 0x4L) == 0) {
this.setDisplayAsBot(instance.getDisplayAsBot());
bits |= 0x4L;
}
if ((bits & 0x8L) == 0) {
addAllImIds(instance.getImIds());
bits |= 0x8L;
}
if ((bits & 0x10L) == 0) {
Optional urlPrivateDownloadOptional = instance.getUrlPrivateDownload();
if (urlPrivateDownloadOptional.isPresent()) {
setUrlPrivateDownload(urlPrivateDownloadOptional);
}
bits |= 0x10L;
}
if ((bits & 0x20L) == 0) {
this.setMode(instance.getMode());
bits |= 0x20L;
}
if ((bits & 0x40L) == 0) {
Optional starredOptional = instance.isStarred();
if (starredOptional.isPresent()) {
setStarred(starredOptional);
}
bits |= 0x40L;
}
if ((bits & 0x80L) == 0) {
this.setUrlPrivate(instance.getUrlPrivate());
bits |= 0x80L;
}
if ((bits & 0x100L) == 0) {
this.setPrettyType(instance.getPrettyType());
bits |= 0x100L;
}
if ((bits & 0x200L) == 0) {
addAllGroupIds(instance.getGroupIds());
bits |= 0x200L;
}
if ((bits & 0x400L) == 0) {
this.setPublicUrlShared(instance.isPublicUrlShared());
bits |= 0x400L;
}
if ((bits & 0x800L) == 0) {
this.setTimestampEpochSeconds(instance.getTimestampEpochSeconds());
bits |= 0x800L;
}
if ((bits & 0x1000L) == 0) {
this.setPublic(instance.isPublic());
bits |= 0x1000L;
}
if ((bits & 0x2000L) == 0) {
this.setId(instance.getId());
bits |= 0x2000L;
}
if ((bits & 0x4000L) == 0) {
addAllChannelIds(instance.getChannelIds());
bits |= 0x4000L;
}
if ((bits & 0x8000L) == 0) {
this.setCreatedEpochSeconds(instance.getCreatedEpochSeconds());
bits |= 0x8000L;
}
if ((bits & 0x10000L) == 0) {
this.setEditable(instance.isEditable());
bits |= 0x10000L;
}
if ((bits & 0x20000L) == 0) {
this.setUserId(instance.getUserId());
bits |= 0x20000L;
}
if ((bits & 0x40000L) == 0) {
this.setExternal(instance.isExternal());
bits |= 0x40000L;
}
if ((bits & 0x80000L) == 0) {
this.setSize(instance.getSize());
bits |= 0x80000L;
}
if ((bits & 0x100000L) == 0) {
this.setFileAccess(instance.getFileAccess());
bits |= 0x100000L;
}
if ((bits & 0x200000L) == 0) {
this.setCommentsCount(instance.getCommentsCount());
bits |= 0x200000L;
}
if ((bits & 0x400000L) == 0) {
this.setName(instance.getName());
bits |= 0x400000L;
}
if ((bits & 0x800000L) == 0) {
this.setMimetype(instance.getMimetype());
bits |= 0x800000L;
}
if ((bits & 0x1000000L) == 0) {
Optional permalinkPublicOptional = instance.getPermalinkPublic();
if (permalinkPublicOptional.isPresent()) {
setPermalinkPublic(permalinkPublicOptional);
}
bits |= 0x1000000L;
}
if ((bits & 0x2000000L) == 0) {
this.setPermalink(instance.getPermalink());
bits |= 0x2000000L;
}
if ((bits & 0x4000000L) == 0) {
this.setUsername(instance.getUsername());
bits |= 0x4000000L;
}
}
if (object instanceof SlackAccessDeniedFileIF) {
SlackAccessDeniedFileIF instance = (SlackAccessDeniedFileIF) object;
if ((bits & 0x1L) == 0) {
this.setFiletype(instance.getFiletype());
bits |= 0x1L;
}
if ((bits & 0x2L) == 0) {
this.setTitle(instance.getTitle());
bits |= 0x2L;
}
if ((bits & 0x4L) == 0) {
this.setDisplayAsBot(instance.getDisplayAsBot());
bits |= 0x4L;
}
if ((bits & 0x8L) == 0) {
addAllImIds(instance.getImIds());
bits |= 0x8L;
}
if ((bits & 0x10L) == 0) {
Optional urlPrivateDownloadOptional = instance.getUrlPrivateDownload();
if (urlPrivateDownloadOptional.isPresent()) {
setUrlPrivateDownload(urlPrivateDownloadOptional);
}
bits |= 0x10L;
}
if ((bits & 0x20L) == 0) {
this.setMode(instance.getMode());
bits |= 0x20L;
}
if ((bits & 0x40L) == 0) {
Optional starredOptional = instance.isStarred();
if (starredOptional.isPresent()) {
setStarred(starredOptional);
}
bits |= 0x40L;
}
if ((bits & 0x80L) == 0) {
this.setUrlPrivate(instance.getUrlPrivate());
bits |= 0x80L;
}
if ((bits & 0x100L) == 0) {
this.setPrettyType(instance.getPrettyType());
bits |= 0x100L;
}
if ((bits & 0x200L) == 0) {
addAllGroupIds(instance.getGroupIds());
bits |= 0x200L;
}
if ((bits & 0x400L) == 0) {
this.setPublicUrlShared(instance.isPublicUrlShared());
bits |= 0x400L;
}
if ((bits & 0x800L) == 0) {
this.setTimestampEpochSeconds(instance.getTimestampEpochSeconds());
bits |= 0x800L;
}
if ((bits & 0x1000L) == 0) {
this.setPublic(instance.isPublic());
bits |= 0x1000L;
}
if ((bits & 0x2000L) == 0) {
this.setId(instance.getId());
bits |= 0x2000L;
}
if ((bits & 0x4000L) == 0) {
addAllChannelIds(instance.getChannelIds());
bits |= 0x4000L;
}
if ((bits & 0x8000L) == 0) {
this.setCreatedEpochSeconds(instance.getCreatedEpochSeconds());
bits |= 0x8000L;
}
if ((bits & 0x10000L) == 0) {
this.setEditable(instance.isEditable());
bits |= 0x10000L;
}
if ((bits & 0x20000L) == 0) {
this.setUserId(instance.getUserId());
bits |= 0x20000L;
}
if ((bits & 0x40000L) == 0) {
this.setExternal(instance.isExternal());
bits |= 0x40000L;
}
if ((bits & 0x80000L) == 0) {
this.setSize(instance.getSize());
bits |= 0x80000L;
}
if ((bits & 0x100000L) == 0) {
this.setFileAccess(instance.getFileAccess());
bits |= 0x100000L;
}
if ((bits & 0x200000L) == 0) {
this.setCommentsCount(instance.getCommentsCount());
bits |= 0x200000L;
}
if ((bits & 0x400000L) == 0) {
this.setName(instance.getName());
bits |= 0x400000L;
}
if ((bits & 0x800000L) == 0) {
this.setMimetype(instance.getMimetype());
bits |= 0x800000L;
}
if ((bits & 0x1000000L) == 0) {
Optional permalinkPublicOptional = instance.getPermalinkPublic();
if (permalinkPublicOptional.isPresent()) {
setPermalinkPublic(permalinkPublicOptional);
}
bits |= 0x1000000L;
}
if ((bits & 0x2000000L) == 0) {
this.setPermalink(instance.getPermalink());
bits |= 0x2000000L;
}
if ((bits & 0x4000000L) == 0) {
this.setUsername(instance.getUsername());
bits |= 0x4000000L;
}
}
if (object instanceof SlackFile) {
SlackFile instance = (SlackFile) object;
if ((bits & 0x1L) == 0) {
this.setFiletype(instance.getFiletype());
bits |= 0x1L;
}
if ((bits & 0x2L) == 0) {
this.setTitle(instance.getTitle());
bits |= 0x2L;
}
if ((bits & 0x4L) == 0) {
this.setDisplayAsBot(instance.getDisplayAsBot());
bits |= 0x4L;
}
if ((bits & 0x8L) == 0) {
addAllImIds(instance.getImIds());
bits |= 0x8L;
}
if ((bits & 0x10L) == 0) {
Optional urlPrivateDownloadOptional = instance.getUrlPrivateDownload();
if (urlPrivateDownloadOptional.isPresent()) {
setUrlPrivateDownload(urlPrivateDownloadOptional);
}
bits |= 0x10L;
}
if ((bits & 0x20L) == 0) {
this.setMode(instance.getMode());
bits |= 0x20L;
}
if ((bits & 0x40L) == 0) {
Optional starredOptional = instance.isStarred();
if (starredOptional.isPresent()) {
setStarred(starredOptional);
}
bits |= 0x40L;
}
if ((bits & 0x80L) == 0) {
this.setUrlPrivate(instance.getUrlPrivate());
bits |= 0x80L;
}
if ((bits & 0x100L) == 0) {
this.setPrettyType(instance.getPrettyType());
bits |= 0x100L;
}
if ((bits & 0x200L) == 0) {
addAllGroupIds(instance.getGroupIds());
bits |= 0x200L;
}
if ((bits & 0x400L) == 0) {
this.setPublicUrlShared(instance.isPublicUrlShared());
bits |= 0x400L;
}
if ((bits & 0x800L) == 0) {
this.setTimestampEpochSeconds(instance.getTimestampEpochSeconds());
bits |= 0x800L;
}
if ((bits & 0x1000L) == 0) {
this.setPublic(instance.isPublic());
bits |= 0x1000L;
}
if ((bits & 0x2000L) == 0) {
this.setId(instance.getId());
bits |= 0x2000L;
}
if ((bits & 0x4000L) == 0) {
addAllChannelIds(instance.getChannelIds());
bits |= 0x4000L;
}
if ((bits & 0x8000L) == 0) {
this.setCreatedEpochSeconds(instance.getCreatedEpochSeconds());
bits |= 0x8000L;
}
if ((bits & 0x10000L) == 0) {
this.setEditable(instance.isEditable());
bits |= 0x10000L;
}
if ((bits & 0x20000L) == 0) {
this.setUserId(instance.getUserId());
bits |= 0x20000L;
}
if ((bits & 0x40000L) == 0) {
this.setExternal(instance.isExternal());
bits |= 0x40000L;
}
if ((bits & 0x80000L) == 0) {
this.setSize(instance.getSize());
bits |= 0x80000L;
}
if ((bits & 0x200000L) == 0) {
this.setCommentsCount(instance.getCommentsCount());
bits |= 0x200000L;
}
if ((bits & 0x400000L) == 0) {
this.setName(instance.getName());
bits |= 0x400000L;
}
if ((bits & 0x800000L) == 0) {
this.setMimetype(instance.getMimetype());
bits |= 0x800000L;
}
if ((bits & 0x1000000L) == 0) {
Optional permalinkPublicOptional = instance.getPermalinkPublic();
if (permalinkPublicOptional.isPresent()) {
setPermalinkPublic(permalinkPublicOptional);
}
bits |= 0x1000000L;
}
if ((bits & 0x2000000L) == 0) {
this.setPermalink(instance.getPermalink());
bits |= 0x2000000L;
}
if ((bits & 0x4000000L) == 0) {
this.setUsername(instance.getUsername());
bits |= 0x4000000L;
}
}
}
/**
* Initializes the value for the {@link SlackAccessDeniedFileIF#getFileAccess() fileAccess} attribute.
* @param fileAccess The value for fileAccess
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty
public final Builder setFileAccess(String fileAccess) {
this.fileAccess = Objects.requireNonNull(fileAccess, "fileAccess");
initBits &= ~INIT_BIT_FILE_ACCESS;
return this;
}
/**
* Initializes the value for the {@link SlackAccessDeniedFileIF#getId() id} attribute.
*
If not set, this attribute will have a default value as returned by the initializer of {@link SlackAccessDeniedFileIF#getId() id}.
* @param id The value for id
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty
public final Builder setId(String id) {
this.id = Objects.requireNonNull(id, "id");
return this;
}
/**
* Initializes the value for the {@link SlackAccessDeniedFileIF#getCreatedEpochSeconds() createdEpochSeconds} attribute.
*
If not set, this attribute will have a default value as returned by the initializer of {@link SlackAccessDeniedFileIF#getCreatedEpochSeconds() createdEpochSeconds}.
* @param createdEpochSeconds The value for createdEpochSeconds
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty
public final Builder setCreatedEpochSeconds(long createdEpochSeconds) {
this.createdEpochSeconds = createdEpochSeconds;
optBits |= OPT_BIT_CREATED_EPOCH_SECONDS;
return this;
}
/**
* Initializes the value for the {@link SlackAccessDeniedFileIF#getTimestampEpochSeconds() timestampEpochSeconds} attribute.
*
If not set, this attribute will have a default value as returned by the initializer of {@link SlackAccessDeniedFileIF#getTimestampEpochSeconds() timestampEpochSeconds}.
* @param timestampEpochSeconds The value for timestampEpochSeconds
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty
public final Builder setTimestampEpochSeconds(long timestampEpochSeconds) {
this.timestampEpochSeconds = timestampEpochSeconds;
optBits |= OPT_BIT_TIMESTAMP_EPOCH_SECONDS;
return this;
}
/**
* Initializes the value for the {@link SlackAccessDeniedFileIF#getName() name} attribute.
*
If not set, this attribute will have a default value as returned by the initializer of {@link SlackAccessDeniedFileIF#getName() name}.
* @param name The value for name
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty
public final Builder setName(String name) {
this.name = Objects.requireNonNull(name, "name");
return this;
}
/**
* Initializes the value for the {@link SlackAccessDeniedFileIF#getTitle() title} attribute.
*
If not set, this attribute will have a default value as returned by the initializer of {@link SlackAccessDeniedFileIF#getTitle() title}.
* @param title The value for title
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty
public final Builder setTitle(String title) {
this.title = Objects.requireNonNull(title, "title");
return this;
}
/**
* Initializes the value for the {@link SlackAccessDeniedFileIF#getMimetype() mimetype} attribute.
*
If not set, this attribute will have a default value as returned by the initializer of {@link SlackAccessDeniedFileIF#getMimetype() mimetype}.
* @param mimetype The value for mimetype
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty
public final Builder setMimetype(String mimetype) {
this.mimetype = Objects.requireNonNull(mimetype, "mimetype");
return this;
}
/**
* Initializes the value for the {@link SlackAccessDeniedFileIF#getFiletype() filetype} attribute.
*
If not set, this attribute will have a default value as returned by the initializer of {@link SlackAccessDeniedFileIF#getFiletype() filetype}.
* @param filetype The value for filetype
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty
public final Builder setFiletype(SlackFileType filetype) {
this.filetype = Objects.requireNonNull(filetype, "filetype");
return this;
}
/**
* Initializes the value for the {@link SlackAccessDeniedFileIF#getPrettyType() prettyType} attribute.
*
If not set, this attribute will have a default value as returned by the initializer of {@link SlackAccessDeniedFileIF#getPrettyType() prettyType}.
* @param prettyType The value for prettyType
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty
public final Builder setPrettyType(String prettyType) {
this.prettyType = Objects.requireNonNull(prettyType, "prettyType");
return this;
}
/**
* Initializes the value for the {@link SlackAccessDeniedFileIF#getUserId() userId} attribute.
*
If not set, this attribute will have a default value as returned by the initializer of {@link SlackAccessDeniedFileIF#getUserId() userId}.
* @param userId The value for userId
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty
public final Builder setUserId(String userId) {
this.userId = Objects.requireNonNull(userId, "userId");
return this;
}
/**
* Initializes the value for the {@link SlackAccessDeniedFileIF#isEditable() editable} attribute.
*
If not set, this attribute will have a default value as returned by the initializer of {@link SlackAccessDeniedFileIF#isEditable() editable}.
* @param editable The value for editable
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty
public final Builder setEditable(boolean editable) {
this.editable = editable;
optBits |= OPT_BIT_EDITABLE;
return this;
}
/**
* Initializes the value for the {@link SlackAccessDeniedFileIF#getSize() size} attribute.
*
If not set, this attribute will have a default value as returned by the initializer of {@link SlackAccessDeniedFileIF#getSize() size}.
* @param size The value for size
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty
public final Builder setSize(long size) {
this.size = size;
optBits |= OPT_BIT_SIZE;
return this;
}
/**
* Initializes the value for the {@link SlackAccessDeniedFileIF#getMode() mode} attribute.
*
If not set, this attribute will have a default value as returned by the initializer of {@link SlackAccessDeniedFileIF#getMode() mode}.
* @param mode The value for mode
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty
public final Builder setMode(String mode) {
this.mode = Objects.requireNonNull(mode, "mode");
return this;
}
/**
* Initializes the value for the {@link SlackAccessDeniedFileIF#isExternal() external} attribute.
*
If not set, this attribute will have a default value as returned by the initializer of {@link SlackAccessDeniedFileIF#isExternal() external}.
* @param external The value for external
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty
public final Builder setExternal(boolean external) {
this.external = external;
optBits |= OPT_BIT_EXTERNAL;
return this;
}
/**
* Initializes the value for the {@link SlackAccessDeniedFileIF#isPublic() public} attribute.
*
If not set, this attribute will have a default value as returned by the initializer of {@link SlackAccessDeniedFileIF#isPublic() public}.
* @param isPublic The value for isPublic
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty
public final Builder setPublic(boolean isPublic) {
this.isPublic = isPublic;
optBits |= OPT_BIT_IS_PUBLIC;
return this;
}
/**
* Initializes the value for the {@link SlackAccessDeniedFileIF#isPublicUrlShared() publicUrlShared} attribute.
*
If not set, this attribute will have a default value as returned by the initializer of {@link SlackAccessDeniedFileIF#isPublicUrlShared() publicUrlShared}.
* @param publicUrlShared The value for publicUrlShared
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty
public final Builder setPublicUrlShared(boolean publicUrlShared) {
this.publicUrlShared = publicUrlShared;
optBits |= OPT_BIT_PUBLIC_URL_SHARED;
return this;
}
/**
* Initializes the value for the {@link SlackAccessDeniedFileIF#getDisplayAsBot() displayAsBot} attribute.
*
If not set, this attribute will have a default value as returned by the initializer of {@link SlackAccessDeniedFileIF#getDisplayAsBot() displayAsBot}.
* @param displayAsBot The value for displayAsBot
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty
public final Builder setDisplayAsBot(boolean displayAsBot) {
this.displayAsBot = displayAsBot;
optBits |= OPT_BIT_DISPLAY_AS_BOT;
return this;
}
/**
* Initializes the value for the {@link SlackAccessDeniedFileIF#getUsername() username} attribute.
*
If not set, this attribute will have a default value as returned by the initializer of {@link SlackAccessDeniedFileIF#getUsername() username}.
* @param username The value for username
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty
public final Builder setUsername(String username) {
this.username = Objects.requireNonNull(username, "username");
return this;
}
/**
* Initializes the value for the {@link SlackAccessDeniedFileIF#getUrlPrivate() urlPrivate} attribute.
*
If not set, this attribute will have a default value as returned by the initializer of {@link SlackAccessDeniedFileIF#getUrlPrivate() urlPrivate}.
* @param urlPrivate The value for urlPrivate
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty
public final Builder setUrlPrivate(String urlPrivate) {
this.urlPrivate = Objects.requireNonNull(urlPrivate, "urlPrivate");
return this;
}
/**
* Initializes the optional value {@link SlackAccessDeniedFileIF#getUrlPrivateDownload() urlPrivateDownload} to urlPrivateDownload.
* @param urlPrivateDownload The value for urlPrivateDownload, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
public final Builder setUrlPrivateDownload(@Nullable String urlPrivateDownload) {
this.urlPrivateDownload = urlPrivateDownload;
return this;
}
/**
* Initializes the optional value {@link SlackAccessDeniedFileIF#getUrlPrivateDownload() urlPrivateDownload} to urlPrivateDownload.
* @param urlPrivateDownload The value for urlPrivateDownload
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty
public final Builder setUrlPrivateDownload(Optional urlPrivateDownload) {
this.urlPrivateDownload = urlPrivateDownload.orElse(null);
return this;
}
/**
* Initializes the value for the {@link SlackAccessDeniedFileIF#getPermalink() permalink} attribute.
*
If not set, this attribute will have a default value as returned by the initializer of {@link SlackAccessDeniedFileIF#getPermalink() permalink}.
* @param permalink The value for permalink
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty
public final Builder setPermalink(String permalink) {
this.permalink = Objects.requireNonNull(permalink, "permalink");
return this;
}
/**
* Initializes the optional value {@link SlackAccessDeniedFileIF#getPermalinkPublic() permalinkPublic} to permalinkPublic.
* @param permalinkPublic The value for permalinkPublic, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
public final Builder setPermalinkPublic(@Nullable String permalinkPublic) {
this.permalinkPublic = permalinkPublic;
return this;
}
/**
* Initializes the optional value {@link SlackAccessDeniedFileIF#getPermalinkPublic() permalinkPublic} to permalinkPublic.
* @param permalinkPublic The value for permalinkPublic
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty
public final Builder setPermalinkPublic(Optional permalinkPublic) {
this.permalinkPublic = permalinkPublic.orElse(null);
return this;
}
/**
* Initializes the value for the {@link SlackAccessDeniedFileIF#getCommentsCount() commentsCount} attribute.
*
If not set, this attribute will have a default value as returned by the initializer of {@link SlackAccessDeniedFileIF#getCommentsCount() commentsCount}.
* @param commentsCount The value for commentsCount
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty
public final Builder setCommentsCount(int commentsCount) {
this.commentsCount = commentsCount;
optBits |= OPT_BIT_COMMENTS_COUNT;
return this;
}
/**
* Initializes the optional value {@link SlackAccessDeniedFileIF#isStarred() starred} to starred.
* @param starred The value for starred, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
public final Builder setStarred(@Nullable Boolean starred) {
this.starred = starred;
return this;
}
/**
* Initializes the optional value {@link SlackAccessDeniedFileIF#isStarred() starred} to starred.
* @param starred The value for starred
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty
public final Builder setStarred(Optional starred) {
this.starred = starred.orElse(null);
return this;
}
/**
* Adds one element to {@link SlackAccessDeniedFileIF#getChannelIds() channelIds} list.
* @param element A channelIds element
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addChannelIds(String element) {
this.channelIds.add(Objects.requireNonNull(element, "channelIds element"));
return this;
}
/**
* Adds elements to {@link SlackAccessDeniedFileIF#getChannelIds() channelIds} list.
* @param elements An array of channelIds elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addChannelIds(String... elements) {
for (String element : elements) {
this.channelIds.add(Objects.requireNonNull(element, "channelIds element"));
}
return this;
}
/**
* Sets or replaces all elements for {@link SlackAccessDeniedFileIF#getChannelIds() channelIds} list.
* @param elements An iterable of channelIds elements
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty
public final Builder setChannelIds(Iterable elements) {
this.channelIds.clear();
return addAllChannelIds(elements);
}
/**
* Adds elements to {@link SlackAccessDeniedFileIF#getChannelIds() channelIds} list.
* @param elements An iterable of channelIds elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addAllChannelIds(Iterable elements) {
for (String element : elements) {
this.channelIds.add(Objects.requireNonNull(element, "channelIds element"));
}
return this;
}
/**
* Adds one element to {@link SlackAccessDeniedFileIF#getGroupIds() groupIds} list.
* @param element A groupIds element
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addGroupIds(String element) {
this.groupIds.add(Objects.requireNonNull(element, "groupIds element"));
return this;
}
/**
* Adds elements to {@link SlackAccessDeniedFileIF#getGroupIds() groupIds} list.
* @param elements An array of groupIds elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addGroupIds(String... elements) {
for (String element : elements) {
this.groupIds.add(Objects.requireNonNull(element, "groupIds element"));
}
return this;
}
/**
* Sets or replaces all elements for {@link SlackAccessDeniedFileIF#getGroupIds() groupIds} list.
* @param elements An iterable of groupIds elements
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty
public final Builder setGroupIds(Iterable elements) {
this.groupIds.clear();
return addAllGroupIds(elements);
}
/**
* Adds elements to {@link SlackAccessDeniedFileIF#getGroupIds() groupIds} list.
* @param elements An iterable of groupIds elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addAllGroupIds(Iterable elements) {
for (String element : elements) {
this.groupIds.add(Objects.requireNonNull(element, "groupIds element"));
}
return this;
}
/**
* Adds one element to {@link SlackAccessDeniedFileIF#getImIds() imIds} list.
* @param element A imIds element
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addImIds(String element) {
this.imIds.add(Objects.requireNonNull(element, "imIds element"));
return this;
}
/**
* Adds elements to {@link SlackAccessDeniedFileIF#getImIds() imIds} list.
* @param elements An array of imIds elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addImIds(String... elements) {
for (String element : elements) {
this.imIds.add(Objects.requireNonNull(element, "imIds element"));
}
return this;
}
/**
* Sets or replaces all elements for {@link SlackAccessDeniedFileIF#getImIds() imIds} list.
* @param elements An iterable of imIds elements
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty
public final Builder setImIds(Iterable elements) {
this.imIds.clear();
return addAllImIds(elements);
}
/**
* Adds elements to {@link SlackAccessDeniedFileIF#getImIds() imIds} list.
* @param elements An iterable of imIds elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addAllImIds(Iterable elements) {
for (String element : elements) {
this.imIds.add(Objects.requireNonNull(element, "imIds element"));
}
return this;
}
/**
* Builds a new {@link SlackAccessDeniedFile SlackAccessDeniedFile}.
* @return An immutable instance of SlackAccessDeniedFile
* @throws com.hubspot.immutables.validation.InvalidImmutableStateException if any required attributes are missing
*/
public SlackAccessDeniedFile build() {
checkRequiredAttributes();
return new SlackAccessDeniedFile(this);
}
private boolean createdEpochSecondsIsSet() {
return (optBits & OPT_BIT_CREATED_EPOCH_SECONDS) != 0;
}
private boolean timestampEpochSecondsIsSet() {
return (optBits & OPT_BIT_TIMESTAMP_EPOCH_SECONDS) != 0;
}
private boolean editableIsSet() {
return (optBits & OPT_BIT_EDITABLE) != 0;
}
private boolean sizeIsSet() {
return (optBits & OPT_BIT_SIZE) != 0;
}
private boolean externalIsSet() {
return (optBits & OPT_BIT_EXTERNAL) != 0;
}
private boolean publicIsSet() {
return (optBits & OPT_BIT_IS_PUBLIC) != 0;
}
private boolean publicUrlSharedIsSet() {
return (optBits & OPT_BIT_PUBLIC_URL_SHARED) != 0;
}
private boolean displayAsBotIsSet() {
return (optBits & OPT_BIT_DISPLAY_AS_BOT) != 0;
}
private boolean commentsCountIsSet() {
return (optBits & OPT_BIT_COMMENTS_COUNT) != 0;
}
private boolean fileAccessIsSet() {
return (initBits & INIT_BIT_FILE_ACCESS) == 0;
}
private void checkRequiredAttributes() {
if (initBits != 0) {
throw new InvalidImmutableStateException(formatRequiredAttributesMessage());
}
}
private String formatRequiredAttributesMessage() {
List attributes = new ArrayList<>();
if (!fileAccessIsSet()) attributes.add("fileAccess");
return "Cannot build SlackAccessDeniedFile, some of required attributes are not set " + attributes;
}
}
private static List createSafeList(Iterable iterable, boolean checkNulls, boolean skipNulls) {
ArrayList list;
if (iterable instanceof Collection) {
int size = ((Collection) iterable).size();
if (size == 0) return Collections.emptyList();
list = new ArrayList<>(size);
} else {
list = new ArrayList<>();
}
for (T element : iterable) {
if (skipNulls && element == null) continue;
if (checkNulls) Objects.requireNonNull(element, "element");
list.add(element);
}
return list;
}
private static List createUnmodifiableList(boolean clone, List list) {
switch(list.size()) {
case 0: return Collections.emptyList();
case 1: return Collections.singletonList(list.get(0));
default:
if (clone) {
return Collections.unmodifiableList(new ArrayList<>(list));
} else {
if (list instanceof ArrayList) {
((ArrayList) list).trimToSize();
}
return Collections.unmodifiableList(list);
}
}
}
}