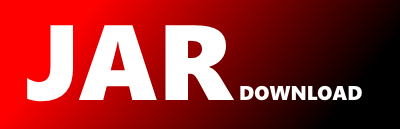
com.hubspot.slack.client.models.interaction.InteractiveAction Maven / Gradle / Ivy
package com.hubspot.slack.client.models.interaction;
import com.fasterxml.jackson.annotation.JsonAutoDetect;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonTypeInfo;
import com.hubspot.immutables.validation.InvalidImmutableStateException;
import com.hubspot.slack.client.models.LiteMessage;
import com.hubspot.slack.client.models.SlackChannel;
import com.hubspot.slack.client.models.actions.Action;
import com.hubspot.slack.client.models.teams.SlackTeam;
import com.hubspot.slack.client.models.users.SlackUserLite;
import edu.umd.cs.findbugs.annotations.SuppressFBWarnings;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
import javax.annotation.ParametersAreNonnullByDefault;
import javax.annotation.concurrent.Immutable;
import javax.annotation.concurrent.NotThreadSafe;
import org.immutables.value.Generated;
/**
* Immutable implementation of {@link InteractiveActionIF}.
*
* Use the builder to create immutable instances:
* {@code InteractiveAction.builder()}.
*/
@Generated(from = "InteractiveActionIF", generator = "Immutables")
@SuppressWarnings({"all"})
@SuppressFBWarnings
@ParametersAreNonnullByDefault
@javax.annotation.processing.Generated("org.immutables.processor.ProxyProcessor")
@Immutable
public final class InteractiveAction
implements InteractiveActionIF {
private final InteractiveCallbackType type;
private final String callbackId;
private final String actionTs;
private final String token;
private final SlackTeam team;
private final SlackUserLite user;
private final SlackChannel channel;
private final List actions;
private final String messageTs;
private final int attachmentId;
private final boolean appUnfurl;
private final @Nullable LiteMessage originalMessage;
private final String responseUrl;
private final String triggerId;
private InteractiveAction(
InteractiveCallbackType type,
String callbackId,
String actionTs,
String token,
SlackTeam team,
SlackUserLite user,
SlackChannel channel,
List actions,
String messageTs,
int attachmentId,
boolean appUnfurl,
@Nullable LiteMessage originalMessage,
String responseUrl,
String triggerId) {
this.type = type;
this.callbackId = callbackId;
this.actionTs = actionTs;
this.token = token;
this.team = team;
this.user = user;
this.channel = channel;
this.actions = actions;
this.messageTs = messageTs;
this.attachmentId = attachmentId;
this.appUnfurl = appUnfurl;
this.originalMessage = originalMessage;
this.responseUrl = responseUrl;
this.triggerId = triggerId;
}
/**
* @return The value of the {@code type} attribute
*/
@JsonProperty
@Override
public InteractiveCallbackType getType() {
return type;
}
/**
* @return The value of the {@code callbackId} attribute
*/
@JsonProperty
@Override
public String getCallbackId() {
return callbackId;
}
/**
* @return The value of the {@code actionTs} attribute
*/
@JsonProperty
@Override
public String getActionTs() {
return actionTs;
}
/**
* @return The value of the {@code token} attribute
*/
@JsonProperty
@Override
public String getToken() {
return token;
}
/**
* @return The value of the {@code team} attribute
*/
@JsonProperty
@Override
public SlackTeam getTeam() {
return team;
}
/**
* @return The value of the {@code user} attribute
*/
@JsonProperty
@Override
public SlackUserLite getUser() {
return user;
}
/**
* @return The value of the {@code channel} attribute
*/
@JsonProperty
@Override
public SlackChannel getChannel() {
return channel;
}
/**
* @return The value of the {@code actions} attribute
*/
@JsonProperty
@Override
public List getActions() {
return actions;
}
/**
* @return The value of the {@code messageTs} attribute
*/
@JsonProperty
@Override
public String getMessageTs() {
return messageTs;
}
/**
* @return The value of the {@code attachmentId} attribute
*/
@JsonProperty
@Override
public int getAttachmentId() {
return attachmentId;
}
/**
* @return The value of the {@code appUnfurl} attribute
*/
@JsonProperty("is_app_unfurl")
@Override
public boolean isAppUnfurl() {
return appUnfurl;
}
/**
* @return The value of the {@code originalMessage} attribute
*/
@JsonProperty
@Override
public Optional getOriginalMessage() {
return Optional.ofNullable(originalMessage);
}
/**
* @return The value of the {@code responseUrl} attribute
*/
@JsonProperty
@Override
public String getResponseUrl() {
return responseUrl;
}
/**
* @return The value of the {@code triggerId} attribute
*/
@JsonProperty
@Override
public String getTriggerId() {
return triggerId;
}
/**
* Copy the current immutable object by setting a value for the {@link InteractiveActionIF#getType() type} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for type
* @return A modified copy of the {@code this} object
*/
public final InteractiveAction withType(InteractiveCallbackType value) {
InteractiveCallbackType newValue = Objects.requireNonNull(value, "type");
if (this.type == newValue) return this;
return new InteractiveAction(
newValue,
this.callbackId,
this.actionTs,
this.token,
this.team,
this.user,
this.channel,
this.actions,
this.messageTs,
this.attachmentId,
this.appUnfurl,
this.originalMessage,
this.responseUrl,
this.triggerId);
}
/**
* Copy the current immutable object by setting a value for the {@link InteractiveActionIF#getCallbackId() callbackId} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for callbackId
* @return A modified copy of the {@code this} object
*/
public final InteractiveAction withCallbackId(String value) {
String newValue = Objects.requireNonNull(value, "callbackId");
if (this.callbackId.equals(newValue)) return this;
return new InteractiveAction(
this.type,
newValue,
this.actionTs,
this.token,
this.team,
this.user,
this.channel,
this.actions,
this.messageTs,
this.attachmentId,
this.appUnfurl,
this.originalMessage,
this.responseUrl,
this.triggerId);
}
/**
* Copy the current immutable object by setting a value for the {@link InteractiveActionIF#getActionTs() actionTs} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for actionTs
* @return A modified copy of the {@code this} object
*/
public final InteractiveAction withActionTs(String value) {
String newValue = Objects.requireNonNull(value, "actionTs");
if (this.actionTs.equals(newValue)) return this;
return new InteractiveAction(
this.type,
this.callbackId,
newValue,
this.token,
this.team,
this.user,
this.channel,
this.actions,
this.messageTs,
this.attachmentId,
this.appUnfurl,
this.originalMessage,
this.responseUrl,
this.triggerId);
}
/**
* Copy the current immutable object by setting a value for the {@link InteractiveActionIF#getToken() token} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for token
* @return A modified copy of the {@code this} object
*/
public final InteractiveAction withToken(String value) {
String newValue = Objects.requireNonNull(value, "token");
if (this.token.equals(newValue)) return this;
return new InteractiveAction(
this.type,
this.callbackId,
this.actionTs,
newValue,
this.team,
this.user,
this.channel,
this.actions,
this.messageTs,
this.attachmentId,
this.appUnfurl,
this.originalMessage,
this.responseUrl,
this.triggerId);
}
/**
* Copy the current immutable object by setting a value for the {@link InteractiveActionIF#getTeam() team} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for team
* @return A modified copy of the {@code this} object
*/
public final InteractiveAction withTeam(SlackTeam value) {
if (this.team == value) return this;
SlackTeam newValue = Objects.requireNonNull(value, "team");
return new InteractiveAction(
this.type,
this.callbackId,
this.actionTs,
this.token,
newValue,
this.user,
this.channel,
this.actions,
this.messageTs,
this.attachmentId,
this.appUnfurl,
this.originalMessage,
this.responseUrl,
this.triggerId);
}
/**
* Copy the current immutable object by setting a value for the {@link InteractiveActionIF#getUser() user} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for user
* @return A modified copy of the {@code this} object
*/
public final InteractiveAction withUser(SlackUserLite value) {
if (this.user == value) return this;
SlackUserLite newValue = Objects.requireNonNull(value, "user");
return new InteractiveAction(
this.type,
this.callbackId,
this.actionTs,
this.token,
this.team,
newValue,
this.channel,
this.actions,
this.messageTs,
this.attachmentId,
this.appUnfurl,
this.originalMessage,
this.responseUrl,
this.triggerId);
}
/**
* Copy the current immutable object by setting a value for the {@link InteractiveActionIF#getChannel() channel} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for channel
* @return A modified copy of the {@code this} object
*/
public final InteractiveAction withChannel(SlackChannel value) {
if (this.channel == value) return this;
SlackChannel newValue = Objects.requireNonNull(value, "channel");
return new InteractiveAction(
this.type,
this.callbackId,
this.actionTs,
this.token,
this.team,
this.user,
newValue,
this.actions,
this.messageTs,
this.attachmentId,
this.appUnfurl,
this.originalMessage,
this.responseUrl,
this.triggerId);
}
/**
* Copy the current immutable object with elements that replace the content of {@link InteractiveActionIF#getActions() actions}.
* @param elements The elements to set
* @return A modified copy of {@code this} object
*/
public final InteractiveAction withActions(Action... elements) {
List newValue = createUnmodifiableList(false, createSafeList(Arrays.asList(elements), true, false));
return new InteractiveAction(
this.type,
this.callbackId,
this.actionTs,
this.token,
this.team,
this.user,
this.channel,
newValue,
this.messageTs,
this.attachmentId,
this.appUnfurl,
this.originalMessage,
this.responseUrl,
this.triggerId);
}
/**
* Copy the current immutable object with elements that replace the content of {@link InteractiveActionIF#getActions() actions}.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements An iterable of actions elements to set
* @return A modified copy of {@code this} object
*/
public final InteractiveAction withActions(Iterable elements) {
if (this.actions == elements) return this;
List newValue = createUnmodifiableList(false, createSafeList(elements, true, false));
return new InteractiveAction(
this.type,
this.callbackId,
this.actionTs,
this.token,
this.team,
this.user,
this.channel,
newValue,
this.messageTs,
this.attachmentId,
this.appUnfurl,
this.originalMessage,
this.responseUrl,
this.triggerId);
}
/**
* Copy the current immutable object by setting a value for the {@link InteractiveActionIF#getMessageTs() messageTs} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for messageTs
* @return A modified copy of the {@code this} object
*/
public final InteractiveAction withMessageTs(String value) {
String newValue = Objects.requireNonNull(value, "messageTs");
if (this.messageTs.equals(newValue)) return this;
return new InteractiveAction(
this.type,
this.callbackId,
this.actionTs,
this.token,
this.team,
this.user,
this.channel,
this.actions,
newValue,
this.attachmentId,
this.appUnfurl,
this.originalMessage,
this.responseUrl,
this.triggerId);
}
/**
* Copy the current immutable object by setting a value for the {@link InteractiveActionIF#getAttachmentId() attachmentId} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for attachmentId
* @return A modified copy of the {@code this} object
*/
public final InteractiveAction withAttachmentId(int value) {
if (this.attachmentId == value) return this;
return new InteractiveAction(
this.type,
this.callbackId,
this.actionTs,
this.token,
this.team,
this.user,
this.channel,
this.actions,
this.messageTs,
value,
this.appUnfurl,
this.originalMessage,
this.responseUrl,
this.triggerId);
}
/**
* Copy the current immutable object by setting a value for the {@link InteractiveActionIF#isAppUnfurl() appUnfurl} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for appUnfurl
* @return A modified copy of the {@code this} object
*/
public final InteractiveAction withAppUnfurl(boolean value) {
if (this.appUnfurl == value) return this;
return new InteractiveAction(
this.type,
this.callbackId,
this.actionTs,
this.token,
this.team,
this.user,
this.channel,
this.actions,
this.messageTs,
this.attachmentId,
value,
this.originalMessage,
this.responseUrl,
this.triggerId);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link InteractiveActionIF#getOriginalMessage() originalMessage} attribute.
* @param value The value for originalMessage, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final InteractiveAction withOriginalMessage(@Nullable LiteMessage value) {
@Nullable LiteMessage newValue = value;
if (this.originalMessage == newValue) return this;
return new InteractiveAction(
this.type,
this.callbackId,
this.actionTs,
this.token,
this.team,
this.user,
this.channel,
this.actions,
this.messageTs,
this.attachmentId,
this.appUnfurl,
newValue,
this.responseUrl,
this.triggerId);
}
/**
* Copy the current immutable object by setting an optional value for the {@link InteractiveActionIF#getOriginalMessage() originalMessage} attribute.
* A shallow reference equality check is used on unboxed optional value to prevent copying of the same value by returning {@code this}.
* @param optional A value for originalMessage
* @return A modified copy of {@code this} object
*/
@SuppressWarnings("unchecked") // safe covariant cast
public final InteractiveAction withOriginalMessage(Optional optional) {
@Nullable LiteMessage value = optional.orElse(null);
if (this.originalMessage == value) return this;
return new InteractiveAction(
this.type,
this.callbackId,
this.actionTs,
this.token,
this.team,
this.user,
this.channel,
this.actions,
this.messageTs,
this.attachmentId,
this.appUnfurl,
value,
this.responseUrl,
this.triggerId);
}
/**
* Copy the current immutable object by setting a value for the {@link InteractiveActionIF#getResponseUrl() responseUrl} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for responseUrl
* @return A modified copy of the {@code this} object
*/
public final InteractiveAction withResponseUrl(String value) {
String newValue = Objects.requireNonNull(value, "responseUrl");
if (this.responseUrl.equals(newValue)) return this;
return new InteractiveAction(
this.type,
this.callbackId,
this.actionTs,
this.token,
this.team,
this.user,
this.channel,
this.actions,
this.messageTs,
this.attachmentId,
this.appUnfurl,
this.originalMessage,
newValue,
this.triggerId);
}
/**
* Copy the current immutable object by setting a value for the {@link InteractiveActionIF#getTriggerId() triggerId} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for triggerId
* @return A modified copy of the {@code this} object
*/
public final InteractiveAction withTriggerId(String value) {
String newValue = Objects.requireNonNull(value, "triggerId");
if (this.triggerId.equals(newValue)) return this;
return new InteractiveAction(
this.type,
this.callbackId,
this.actionTs,
this.token,
this.team,
this.user,
this.channel,
this.actions,
this.messageTs,
this.attachmentId,
this.appUnfurl,
this.originalMessage,
this.responseUrl,
newValue);
}
/**
* This instance is equal to all instances of {@code InteractiveAction} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(@Nullable Object another) {
if (this == another) return true;
return another instanceof InteractiveAction
&& equalTo(0, (InteractiveAction) another);
}
private boolean equalTo(int synthetic, InteractiveAction another) {
return type.equals(another.type)
&& callbackId.equals(another.callbackId)
&& actionTs.equals(another.actionTs)
&& token.equals(another.token)
&& team.equals(another.team)
&& user.equals(another.user)
&& channel.equals(another.channel)
&& actions.equals(another.actions)
&& messageTs.equals(another.messageTs)
&& attachmentId == another.attachmentId
&& appUnfurl == another.appUnfurl
&& Objects.equals(originalMessage, another.originalMessage)
&& responseUrl.equals(another.responseUrl)
&& triggerId.equals(another.triggerId);
}
/**
* Computes a hash code from attributes: {@code type}, {@code callbackId}, {@code actionTs}, {@code token}, {@code team}, {@code user}, {@code channel}, {@code actions}, {@code messageTs}, {@code attachmentId}, {@code appUnfurl}, {@code originalMessage}, {@code responseUrl}, {@code triggerId}.
* @return hashCode value
*/
@Override
public int hashCode() {
int h = 5381;
h += (h << 5) + type.hashCode();
h += (h << 5) + callbackId.hashCode();
h += (h << 5) + actionTs.hashCode();
h += (h << 5) + token.hashCode();
h += (h << 5) + team.hashCode();
h += (h << 5) + user.hashCode();
h += (h << 5) + channel.hashCode();
h += (h << 5) + actions.hashCode();
h += (h << 5) + messageTs.hashCode();
h += (h << 5) + attachmentId;
h += (h << 5) + Boolean.hashCode(appUnfurl);
h += (h << 5) + Objects.hashCode(originalMessage);
h += (h << 5) + responseUrl.hashCode();
h += (h << 5) + triggerId.hashCode();
return h;
}
/**
* Prints the immutable value {@code InteractiveAction} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
StringBuilder builder = new StringBuilder("InteractiveAction{");
builder.append("type=").append(type);
builder.append(", ");
builder.append("callbackId=").append(callbackId);
builder.append(", ");
builder.append("actionTs=").append(actionTs);
builder.append(", ");
builder.append("token=").append(token);
builder.append(", ");
builder.append("team=").append(team);
builder.append(", ");
builder.append("user=").append(user);
builder.append(", ");
builder.append("channel=").append(channel);
builder.append(", ");
builder.append("actions=").append(actions);
builder.append(", ");
builder.append("messageTs=").append(messageTs);
builder.append(", ");
builder.append("attachmentId=").append(attachmentId);
builder.append(", ");
builder.append("appUnfurl=").append(appUnfurl);
if (originalMessage != null) {
builder.append(", ");
builder.append("originalMessage=").append(originalMessage);
}
builder.append(", ");
builder.append("responseUrl=").append(responseUrl);
builder.append(", ");
builder.append("triggerId=").append(triggerId);
return builder.append("}").toString();
}
/**
* Utility type used to correctly read immutable object from JSON representation.
* @deprecated Do not use this type directly, it exists only for the Jackson-binding infrastructure
*/
@Generated(from = "InteractiveActionIF", generator = "Immutables")
@Deprecated
@JsonTypeInfo(use=JsonTypeInfo.Id.NONE)
@JsonAutoDetect(fieldVisibility = JsonAutoDetect.Visibility.NONE)
static final class Json implements InteractiveActionIF {
@Nullable InteractiveCallbackType type;
@Nullable String callbackId;
@Nullable String actionTs;
@Nullable String token;
@Nullable SlackTeam team;
@Nullable SlackUserLite user;
@Nullable SlackChannel channel;
@Nullable List actions = Collections.emptyList();
@Nullable String messageTs;
int attachmentId;
boolean attachmentIdIsSet;
boolean appUnfurl;
boolean appUnfurlIsSet;
@Nullable Optional originalMessage = Optional.empty();
@Nullable String responseUrl;
@Nullable String triggerId;
@JsonProperty
public void setType(InteractiveCallbackType type) {
this.type = type;
}
@JsonProperty
public void setCallbackId(String callbackId) {
this.callbackId = callbackId;
}
@JsonProperty
public void setActionTs(String actionTs) {
this.actionTs = actionTs;
}
@JsonProperty
public void setToken(String token) {
this.token = token;
}
@JsonProperty
public void setTeam(SlackTeam team) {
this.team = team;
}
@JsonProperty
public void setUser(SlackUserLite user) {
this.user = user;
}
@JsonProperty
public void setChannel(SlackChannel channel) {
this.channel = channel;
}
@JsonProperty
public void setActions(List actions) {
this.actions = actions;
}
@JsonProperty
public void setMessageTs(String messageTs) {
this.messageTs = messageTs;
}
@JsonProperty
public void setAttachmentId(int attachmentId) {
this.attachmentId = attachmentId;
this.attachmentIdIsSet = true;
}
@JsonProperty("is_app_unfurl")
public void setAppUnfurl(boolean appUnfurl) {
this.appUnfurl = appUnfurl;
this.appUnfurlIsSet = true;
}
@JsonProperty
public void setOriginalMessage(Optional originalMessage) {
this.originalMessage = originalMessage;
}
@JsonProperty
public void setResponseUrl(String responseUrl) {
this.responseUrl = responseUrl;
}
@JsonProperty
public void setTriggerId(String triggerId) {
this.triggerId = triggerId;
}
@Override
public InteractiveCallbackType getType() { throw new UnsupportedOperationException(); }
@Override
public String getCallbackId() { throw new UnsupportedOperationException(); }
@Override
public String getActionTs() { throw new UnsupportedOperationException(); }
@Override
public String getToken() { throw new UnsupportedOperationException(); }
@Override
public SlackTeam getTeam() { throw new UnsupportedOperationException(); }
@Override
public SlackUserLite getUser() { throw new UnsupportedOperationException(); }
@Override
public SlackChannel getChannel() { throw new UnsupportedOperationException(); }
@Override
public List getActions() { throw new UnsupportedOperationException(); }
@Override
public String getMessageTs() { throw new UnsupportedOperationException(); }
@Override
public int getAttachmentId() { throw new UnsupportedOperationException(); }
@Override
public boolean isAppUnfurl() { throw new UnsupportedOperationException(); }
@Override
public Optional getOriginalMessage() { throw new UnsupportedOperationException(); }
@Override
public String getResponseUrl() { throw new UnsupportedOperationException(); }
@Override
public String getTriggerId() { throw new UnsupportedOperationException(); }
}
/**
* @param json A JSON-bindable data structure
* @return An immutable value type
* @deprecated Do not use this method directly, it exists only for the Jackson-binding infrastructure
*/
@Deprecated
@JsonCreator(mode = JsonCreator.Mode.DELEGATING)
static InteractiveAction fromJson(Json json) {
InteractiveAction.Builder builder = InteractiveAction.builder();
if (json.type != null) {
builder.setType(json.type);
}
if (json.callbackId != null) {
builder.setCallbackId(json.callbackId);
}
if (json.actionTs != null) {
builder.setActionTs(json.actionTs);
}
if (json.token != null) {
builder.setToken(json.token);
}
if (json.team != null) {
builder.setTeam(json.team);
}
if (json.user != null) {
builder.setUser(json.user);
}
if (json.channel != null) {
builder.setChannel(json.channel);
}
if (json.actions != null) {
builder.addAllActions(json.actions);
}
if (json.messageTs != null) {
builder.setMessageTs(json.messageTs);
}
if (json.attachmentIdIsSet) {
builder.setAttachmentId(json.attachmentId);
}
if (json.appUnfurlIsSet) {
builder.setAppUnfurl(json.appUnfurl);
}
if (json.originalMessage != null) {
builder.setOriginalMessage(json.originalMessage);
}
if (json.responseUrl != null) {
builder.setResponseUrl(json.responseUrl);
}
if (json.triggerId != null) {
builder.setTriggerId(json.triggerId);
}
return builder.build();
}
/**
* Creates an immutable copy of a {@link InteractiveActionIF} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable InteractiveAction instance
*/
public static InteractiveAction copyOf(InteractiveActionIF instance) {
if (instance instanceof InteractiveAction) {
return (InteractiveAction) instance;
}
return InteractiveAction.builder()
.from(instance)
.build();
}
/**
* Creates a builder for {@link InteractiveAction InteractiveAction}.
*
* InteractiveAction.builder()
* .setType(com.hubspot.slack.client.models.interaction.InteractiveCallbackType) // required {@link InteractiveActionIF#getType() type}
* .setCallbackId(String) // required {@link InteractiveActionIF#getCallbackId() callbackId}
* .setActionTs(String) // required {@link InteractiveActionIF#getActionTs() actionTs}
* .setToken(String) // required {@link InteractiveActionIF#getToken() token}
* .setTeam(com.hubspot.slack.client.models.teams.SlackTeam) // required {@link InteractiveActionIF#getTeam() team}
* .setUser(com.hubspot.slack.client.models.users.SlackUserLite) // required {@link InteractiveActionIF#getUser() user}
* .setChannel(com.hubspot.slack.client.models.SlackChannel) // required {@link InteractiveActionIF#getChannel() channel}
* .addActions|addAllActions(com.hubspot.slack.client.models.actions.Action) // {@link InteractiveActionIF#getActions() actions} elements
* .setMessageTs(String) // required {@link InteractiveActionIF#getMessageTs() messageTs}
* .setAttachmentId(int) // required {@link InteractiveActionIF#getAttachmentId() attachmentId}
* .setAppUnfurl(boolean) // required {@link InteractiveActionIF#isAppUnfurl() appUnfurl}
* .setOriginalMessage(com.hubspot.slack.client.models.LiteMessage) // optional {@link InteractiveActionIF#getOriginalMessage() originalMessage}
* .setResponseUrl(String) // required {@link InteractiveActionIF#getResponseUrl() responseUrl}
* .setTriggerId(String) // required {@link InteractiveActionIF#getTriggerId() triggerId}
* .build();
*
* @return A new InteractiveAction builder
*/
public static InteractiveAction.Builder builder() {
return new InteractiveAction.Builder();
}
/**
* Builds instances of type {@link InteractiveAction InteractiveAction}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
*
{@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
@Generated(from = "InteractiveActionIF", generator = "Immutables")
@NotThreadSafe
public static final class Builder {
private static final long INIT_BIT_TYPE = 0x1L;
private static final long INIT_BIT_CALLBACK_ID = 0x2L;
private static final long INIT_BIT_ACTION_TS = 0x4L;
private static final long INIT_BIT_TOKEN = 0x8L;
private static final long INIT_BIT_TEAM = 0x10L;
private static final long INIT_BIT_USER = 0x20L;
private static final long INIT_BIT_CHANNEL = 0x40L;
private static final long INIT_BIT_MESSAGE_TS = 0x80L;
private static final long INIT_BIT_ATTACHMENT_ID = 0x100L;
private static final long INIT_BIT_APP_UNFURL = 0x200L;
private static final long INIT_BIT_RESPONSE_URL = 0x400L;
private static final long INIT_BIT_TRIGGER_ID = 0x800L;
private long initBits = 0xfffL;
private @Nullable InteractiveCallbackType type;
private @Nullable String callbackId;
private @Nullable String actionTs;
private @Nullable String token;
private @Nullable SlackTeam team;
private @Nullable SlackUserLite user;
private @Nullable SlackChannel channel;
private List actions = new ArrayList();
private @Nullable String messageTs;
private int attachmentId;
private boolean appUnfurl;
private @Nullable LiteMessage originalMessage;
private @Nullable String responseUrl;
private @Nullable String triggerId;
private Builder() {
}
/**
* Fill a builder with attribute values from the provided {@code com.hubspot.slack.client.models.interaction.InteractiveActionIF} instance.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(InteractiveActionIF instance) {
Objects.requireNonNull(instance, "instance");
from((short) 0, (Object) instance);
return this;
}
/**
* Fill a builder with attribute values from the provided {@code com.hubspot.slack.client.models.interaction.SlackInteractiveCallback} instance.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(SlackInteractiveCallback instance) {
Objects.requireNonNull(instance, "instance");
from((short) 0, (Object) instance);
return this;
}
private void from(short _unused, Object object) {
long bits = 0;
if (object instanceof InteractiveActionIF) {
InteractiveActionIF instance = (InteractiveActionIF) object;
this.setTriggerId(instance.getTriggerId());
if ((bits & 0x1L) == 0) {
this.setChannel(instance.getChannel());
bits |= 0x1L;
}
if ((bits & 0x2L) == 0) {
this.setTeam(instance.getTeam());
bits |= 0x2L;
}
if ((bits & 0x4L) == 0) {
this.setType(instance.getType());
bits |= 0x4L;
}
this.setMessageTs(instance.getMessageTs());
if ((bits & 0x8L) == 0) {
this.setToken(instance.getToken());
bits |= 0x8L;
}
this.setResponseUrl(instance.getResponseUrl());
if ((bits & 0x10L) == 0) {
this.setCallbackId(instance.getCallbackId());
bits |= 0x10L;
}
this.setAppUnfurl(instance.isAppUnfurl());
this.setAttachmentId(instance.getAttachmentId());
Optional originalMessageOptional = instance.getOriginalMessage();
if (originalMessageOptional.isPresent()) {
setOriginalMessage(originalMessageOptional);
}
if ((bits & 0x20L) == 0) {
this.setActionTs(instance.getActionTs());
bits |= 0x20L;
}
if ((bits & 0x40L) == 0) {
this.setUser(instance.getUser());
bits |= 0x40L;
}
addAllActions(instance.getActions());
}
if (object instanceof SlackInteractiveCallback) {
SlackInteractiveCallback instance = (SlackInteractiveCallback) object;
if ((bits & 0x10L) == 0) {
this.setCallbackId(instance.getCallbackId());
bits |= 0x10L;
}
if ((bits & 0x1L) == 0) {
this.setChannel(instance.getChannel());
bits |= 0x1L;
}
if ((bits & 0x2L) == 0) {
this.setTeam(instance.getTeam());
bits |= 0x2L;
}
if ((bits & 0x4L) == 0) {
this.setType(instance.getType());
bits |= 0x4L;
}
if ((bits & 0x20L) == 0) {
this.setActionTs(instance.getActionTs());
bits |= 0x20L;
}
if ((bits & 0x40L) == 0) {
this.setUser(instance.getUser());
bits |= 0x40L;
}
if ((bits & 0x8L) == 0) {
this.setToken(instance.getToken());
bits |= 0x8L;
}
}
}
/**
* Initializes the value for the {@link InteractiveActionIF#getType() type} attribute.
* @param type The value for type
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setType(InteractiveCallbackType type) {
this.type = Objects.requireNonNull(type, "type");
initBits &= ~INIT_BIT_TYPE;
return this;
}
/**
* Initializes the value for the {@link InteractiveActionIF#getCallbackId() callbackId} attribute.
* @param callbackId The value for callbackId
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setCallbackId(String callbackId) {
this.callbackId = Objects.requireNonNull(callbackId, "callbackId");
initBits &= ~INIT_BIT_CALLBACK_ID;
return this;
}
/**
* Initializes the value for the {@link InteractiveActionIF#getActionTs() actionTs} attribute.
* @param actionTs The value for actionTs
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setActionTs(String actionTs) {
this.actionTs = Objects.requireNonNull(actionTs, "actionTs");
initBits &= ~INIT_BIT_ACTION_TS;
return this;
}
/**
* Initializes the value for the {@link InteractiveActionIF#getToken() token} attribute.
* @param token The value for token
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setToken(String token) {
this.token = Objects.requireNonNull(token, "token");
initBits &= ~INIT_BIT_TOKEN;
return this;
}
/**
* Initializes the value for the {@link InteractiveActionIF#getTeam() team} attribute.
* @param team The value for team
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setTeam(SlackTeam team) {
this.team = Objects.requireNonNull(team, "team");
initBits &= ~INIT_BIT_TEAM;
return this;
}
/**
* Initializes the value for the {@link InteractiveActionIF#getUser() user} attribute.
* @param user The value for user
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setUser(SlackUserLite user) {
this.user = Objects.requireNonNull(user, "user");
initBits &= ~INIT_BIT_USER;
return this;
}
/**
* Initializes the value for the {@link InteractiveActionIF#getChannel() channel} attribute.
* @param channel The value for channel
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setChannel(SlackChannel channel) {
this.channel = Objects.requireNonNull(channel, "channel");
initBits &= ~INIT_BIT_CHANNEL;
return this;
}
/**
* Adds one element to {@link InteractiveActionIF#getActions() actions} list.
* @param element A actions element
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addActions(Action element) {
this.actions.add(Objects.requireNonNull(element, "actions element"));
return this;
}
/**
* Adds elements to {@link InteractiveActionIF#getActions() actions} list.
* @param elements An array of actions elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addActions(Action... elements) {
for (Action element : elements) {
this.actions.add(Objects.requireNonNull(element, "actions element"));
}
return this;
}
/**
* Sets or replaces all elements for {@link InteractiveActionIF#getActions() actions} list.
* @param elements An iterable of actions elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setActions(Iterable elements) {
this.actions.clear();
return addAllActions(elements);
}
/**
* Adds elements to {@link InteractiveActionIF#getActions() actions} list.
* @param elements An iterable of actions elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addAllActions(Iterable elements) {
for (Action element : elements) {
this.actions.add(Objects.requireNonNull(element, "actions element"));
}
return this;
}
/**
* Initializes the value for the {@link InteractiveActionIF#getMessageTs() messageTs} attribute.
* @param messageTs The value for messageTs
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setMessageTs(String messageTs) {
this.messageTs = Objects.requireNonNull(messageTs, "messageTs");
initBits &= ~INIT_BIT_MESSAGE_TS;
return this;
}
/**
* Initializes the value for the {@link InteractiveActionIF#getAttachmentId() attachmentId} attribute.
* @param attachmentId The value for attachmentId
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setAttachmentId(int attachmentId) {
this.attachmentId = attachmentId;
initBits &= ~INIT_BIT_ATTACHMENT_ID;
return this;
}
/**
* Initializes the value for the {@link InteractiveActionIF#isAppUnfurl() appUnfurl} attribute.
* @param appUnfurl The value for appUnfurl
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setAppUnfurl(boolean appUnfurl) {
this.appUnfurl = appUnfurl;
initBits &= ~INIT_BIT_APP_UNFURL;
return this;
}
/**
* Initializes the optional value {@link InteractiveActionIF#getOriginalMessage() originalMessage} to originalMessage.
* @param originalMessage The value for originalMessage, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
public final Builder setOriginalMessage(@Nullable LiteMessage originalMessage) {
this.originalMessage = originalMessage;
return this;
}
/**
* Initializes the optional value {@link InteractiveActionIF#getOriginalMessage() originalMessage} to originalMessage.
* @param originalMessage The value for originalMessage
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setOriginalMessage(Optional originalMessage) {
this.originalMessage = originalMessage.orElse(null);
return this;
}
/**
* Initializes the value for the {@link InteractiveActionIF#getResponseUrl() responseUrl} attribute.
* @param responseUrl The value for responseUrl
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setResponseUrl(String responseUrl) {
this.responseUrl = Objects.requireNonNull(responseUrl, "responseUrl");
initBits &= ~INIT_BIT_RESPONSE_URL;
return this;
}
/**
* Initializes the value for the {@link InteractiveActionIF#getTriggerId() triggerId} attribute.
* @param triggerId The value for triggerId
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setTriggerId(String triggerId) {
this.triggerId = Objects.requireNonNull(triggerId, "triggerId");
initBits &= ~INIT_BIT_TRIGGER_ID;
return this;
}
/**
* Builds a new {@link InteractiveAction InteractiveAction}.
* @return An immutable instance of InteractiveAction
* @throws com.hubspot.immutables.validation.InvalidImmutableStateException if any required attributes are missing
*/
public InteractiveAction build() {
checkRequiredAttributes();
return new InteractiveAction(
type,
callbackId,
actionTs,
token,
team,
user,
channel,
createUnmodifiableList(true, actions),
messageTs,
attachmentId,
appUnfurl,
originalMessage,
responseUrl,
triggerId);
}
private boolean typeIsSet() {
return (initBits & INIT_BIT_TYPE) == 0;
}
private boolean callbackIdIsSet() {
return (initBits & INIT_BIT_CALLBACK_ID) == 0;
}
private boolean actionTsIsSet() {
return (initBits & INIT_BIT_ACTION_TS) == 0;
}
private boolean tokenIsSet() {
return (initBits & INIT_BIT_TOKEN) == 0;
}
private boolean teamIsSet() {
return (initBits & INIT_BIT_TEAM) == 0;
}
private boolean userIsSet() {
return (initBits & INIT_BIT_USER) == 0;
}
private boolean channelIsSet() {
return (initBits & INIT_BIT_CHANNEL) == 0;
}
private boolean messageTsIsSet() {
return (initBits & INIT_BIT_MESSAGE_TS) == 0;
}
private boolean attachmentIdIsSet() {
return (initBits & INIT_BIT_ATTACHMENT_ID) == 0;
}
private boolean appUnfurlIsSet() {
return (initBits & INIT_BIT_APP_UNFURL) == 0;
}
private boolean responseUrlIsSet() {
return (initBits & INIT_BIT_RESPONSE_URL) == 0;
}
private boolean triggerIdIsSet() {
return (initBits & INIT_BIT_TRIGGER_ID) == 0;
}
private void checkRequiredAttributes() {
if (initBits != 0) {
throw new InvalidImmutableStateException(formatRequiredAttributesMessage());
}
}
private String formatRequiredAttributesMessage() {
List attributes = new ArrayList<>();
if (!typeIsSet()) attributes.add("type");
if (!callbackIdIsSet()) attributes.add("callbackId");
if (!actionTsIsSet()) attributes.add("actionTs");
if (!tokenIsSet()) attributes.add("token");
if (!teamIsSet()) attributes.add("team");
if (!userIsSet()) attributes.add("user");
if (!channelIsSet()) attributes.add("channel");
if (!messageTsIsSet()) attributes.add("messageTs");
if (!attachmentIdIsSet()) attributes.add("attachmentId");
if (!appUnfurlIsSet()) attributes.add("appUnfurl");
if (!responseUrlIsSet()) attributes.add("responseUrl");
if (!triggerIdIsSet()) attributes.add("triggerId");
return "Cannot build InteractiveAction, some of required attributes are not set " + attributes;
}
}
private static List createSafeList(Iterable iterable, boolean checkNulls, boolean skipNulls) {
ArrayList list;
if (iterable instanceof Collection) {
int size = ((Collection) iterable).size();
if (size == 0) return Collections.emptyList();
list = new ArrayList<>(size);
} else {
list = new ArrayList<>();
}
for (T element : iterable) {
if (skipNulls && element == null) continue;
if (checkNulls) Objects.requireNonNull(element, "element");
list.add(element);
}
return list;
}
private static List createUnmodifiableList(boolean clone, List list) {
switch(list.size()) {
case 0: return Collections.emptyList();
case 1: return Collections.singletonList(list.get(0));
default:
if (clone) {
return Collections.unmodifiableList(new ArrayList<>(list));
} else {
if (list instanceof ArrayList) {
((ArrayList) list).trimToSize();
}
return Collections.unmodifiableList(list);
}
}
}
}