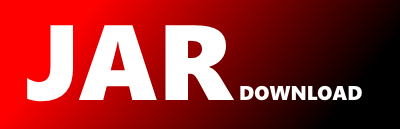
com.hubspot.slack.client.models.response.files.GetUploadUrlExternalResponse Maven / Gradle / Ivy
package com.hubspot.slack.client.models.response.files;
import com.fasterxml.jackson.annotation.JsonAutoDetect;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.hubspot.immutables.validation.InvalidImmutableStateException;
import com.hubspot.slack.client.models.response.*;
import com.hubspot.slack.client.models.response.SlackResponse;
import edu.umd.cs.findbugs.annotations.SuppressFBWarnings;
import java.util.ArrayList;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
import javax.annotation.ParametersAreNonnullByDefault;
import javax.annotation.concurrent.Immutable;
import javax.annotation.concurrent.NotThreadSafe;
import org.immutables.value.Generated;
/**
* Immutable implementation of {@link GetUploadUrlExternalResponseIF}.
*
* Use the builder to create immutable instances:
* {@code GetUploadUrlExternalResponse.builder()}.
*/
@Generated(from = "GetUploadUrlExternalResponseIF", generator = "Immutables")
@SuppressWarnings({"all"})
@SuppressFBWarnings
@ParametersAreNonnullByDefault
@javax.annotation.processing.Generated("org.immutables.processor.ProxyProcessor")
@Immutable
public final class GetUploadUrlExternalResponse
implements GetUploadUrlExternalResponseIF {
private final boolean ok;
private final @Nullable ResponseMetadata responseMetadata;
private final String uploadUrl;
private final String fileId;
private GetUploadUrlExternalResponse(
boolean ok,
@Nullable ResponseMetadata responseMetadata,
String uploadUrl,
String fileId) {
this.ok = ok;
this.responseMetadata = responseMetadata;
this.uploadUrl = uploadUrl;
this.fileId = fileId;
}
/**
* @return The value of the {@code ok} attribute
*/
@JsonProperty
@Override
public boolean isOk() {
return ok;
}
/**
* @return The value of the {@code responseMetadata} attribute
*/
@JsonProperty("response_metadata")
@Override
public Optional getResponseMetadata() {
return Optional.ofNullable(responseMetadata);
}
/**
* @return The value of the {@code uploadUrl} attribute
*/
@JsonProperty
@Override
public String getUploadUrl() {
return uploadUrl;
}
/**
* @return The value of the {@code fileId} attribute
*/
@JsonProperty
@Override
public String getFileId() {
return fileId;
}
/**
* Copy the current immutable object by setting a value for the {@link GetUploadUrlExternalResponseIF#isOk() ok} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for ok
* @return A modified copy of the {@code this} object
*/
public final GetUploadUrlExternalResponse withOk(boolean value) {
if (this.ok == value) return this;
return new GetUploadUrlExternalResponse(value, this.responseMetadata, this.uploadUrl, this.fileId);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link GetUploadUrlExternalResponseIF#getResponseMetadata() responseMetadata} attribute.
* @param value The value for responseMetadata, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final GetUploadUrlExternalResponse withResponseMetadata(@Nullable ResponseMetadata value) {
@Nullable ResponseMetadata newValue = value;
if (this.responseMetadata == newValue) return this;
return new GetUploadUrlExternalResponse(this.ok, newValue, this.uploadUrl, this.fileId);
}
/**
* Copy the current immutable object by setting an optional value for the {@link GetUploadUrlExternalResponseIF#getResponseMetadata() responseMetadata} attribute.
* A shallow reference equality check is used on unboxed optional value to prevent copying of the same value by returning {@code this}.
* @param optional A value for responseMetadata
* @return A modified copy of {@code this} object
*/
@SuppressWarnings("unchecked") // safe covariant cast
public final GetUploadUrlExternalResponse withResponseMetadata(Optional optional) {
@Nullable ResponseMetadata value = optional.orElse(null);
if (this.responseMetadata == value) return this;
return new GetUploadUrlExternalResponse(this.ok, value, this.uploadUrl, this.fileId);
}
/**
* Copy the current immutable object by setting a value for the {@link GetUploadUrlExternalResponseIF#getUploadUrl() uploadUrl} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for uploadUrl
* @return A modified copy of the {@code this} object
*/
public final GetUploadUrlExternalResponse withUploadUrl(String value) {
String newValue = Objects.requireNonNull(value, "uploadUrl");
if (this.uploadUrl.equals(newValue)) return this;
return new GetUploadUrlExternalResponse(this.ok, this.responseMetadata, newValue, this.fileId);
}
/**
* Copy the current immutable object by setting a value for the {@link GetUploadUrlExternalResponseIF#getFileId() fileId} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for fileId
* @return A modified copy of the {@code this} object
*/
public final GetUploadUrlExternalResponse withFileId(String value) {
String newValue = Objects.requireNonNull(value, "fileId");
if (this.fileId.equals(newValue)) return this;
return new GetUploadUrlExternalResponse(this.ok, this.responseMetadata, this.uploadUrl, newValue);
}
/**
* This instance is equal to all instances of {@code GetUploadUrlExternalResponse} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(@Nullable Object another) {
if (this == another) return true;
return another instanceof GetUploadUrlExternalResponse
&& equalTo(0, (GetUploadUrlExternalResponse) another);
}
private boolean equalTo(int synthetic, GetUploadUrlExternalResponse another) {
return ok == another.ok
&& Objects.equals(responseMetadata, another.responseMetadata)
&& uploadUrl.equals(another.uploadUrl)
&& fileId.equals(another.fileId);
}
/**
* Computes a hash code from attributes: {@code ok}, {@code responseMetadata}, {@code uploadUrl}, {@code fileId}.
* @return hashCode value
*/
@Override
public int hashCode() {
int h = 5381;
h += (h << 5) + Boolean.hashCode(ok);
h += (h << 5) + Objects.hashCode(responseMetadata);
h += (h << 5) + uploadUrl.hashCode();
h += (h << 5) + fileId.hashCode();
return h;
}
/**
* Prints the immutable value {@code GetUploadUrlExternalResponse} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
StringBuilder builder = new StringBuilder("GetUploadUrlExternalResponse{");
builder.append("ok=").append(ok);
if (responseMetadata != null) {
builder.append(", ");
builder.append("responseMetadata=").append(responseMetadata);
}
builder.append(", ");
builder.append("uploadUrl=").append(uploadUrl);
builder.append(", ");
builder.append("fileId=").append(fileId);
return builder.append("}").toString();
}
/**
* Utility type used to correctly read immutable object from JSON representation.
* @deprecated Do not use this type directly, it exists only for the Jackson-binding infrastructure
*/
@Generated(from = "GetUploadUrlExternalResponseIF", generator = "Immutables")
@Deprecated
@JsonAutoDetect(fieldVisibility = JsonAutoDetect.Visibility.NONE)
static final class Json
implements GetUploadUrlExternalResponseIF {
boolean ok;
boolean okIsSet;
@Nullable Optional responseMetadata = Optional.empty();
@Nullable String uploadUrl;
@Nullable String fileId;
@JsonProperty
public void setOk(boolean ok) {
this.ok = ok;
this.okIsSet = true;
}
@JsonProperty("response_metadata")
public void setResponseMetadata(Optional responseMetadata) {
this.responseMetadata = responseMetadata;
}
@JsonProperty
public void setUploadUrl(String uploadUrl) {
this.uploadUrl = uploadUrl;
}
@JsonProperty
public void setFileId(String fileId) {
this.fileId = fileId;
}
@Override
public boolean isOk() { throw new UnsupportedOperationException(); }
@Override
public Optional getResponseMetadata() { throw new UnsupportedOperationException(); }
@Override
public String getUploadUrl() { throw new UnsupportedOperationException(); }
@Override
public String getFileId() { throw new UnsupportedOperationException(); }
}
/**
* @param json A JSON-bindable data structure
* @return An immutable value type
* @deprecated Do not use this method directly, it exists only for the Jackson-binding infrastructure
*/
@Deprecated
@JsonCreator(mode = JsonCreator.Mode.DELEGATING)
static GetUploadUrlExternalResponse fromJson(Json json) {
GetUploadUrlExternalResponse.Builder builder = GetUploadUrlExternalResponse.builder();
if (json.okIsSet) {
builder.setOk(json.ok);
}
if (json.responseMetadata != null) {
builder.setResponseMetadata(json.responseMetadata);
}
if (json.uploadUrl != null) {
builder.setUploadUrl(json.uploadUrl);
}
if (json.fileId != null) {
builder.setFileId(json.fileId);
}
return builder.build();
}
/**
* Creates an immutable copy of a {@link GetUploadUrlExternalResponseIF} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable GetUploadUrlExternalResponse instance
*/
public static GetUploadUrlExternalResponse copyOf(GetUploadUrlExternalResponseIF instance) {
if (instance instanceof GetUploadUrlExternalResponse) {
return (GetUploadUrlExternalResponse) instance;
}
return GetUploadUrlExternalResponse.builder()
.from(instance)
.build();
}
/**
* Creates a builder for {@link GetUploadUrlExternalResponse GetUploadUrlExternalResponse}.
*
* GetUploadUrlExternalResponse.builder()
* .setOk(boolean) // required {@link GetUploadUrlExternalResponseIF#isOk() ok}
* .setResponseMetadata(ResponseMetadata) // optional {@link GetUploadUrlExternalResponseIF#getResponseMetadata() responseMetadata}
* .setUploadUrl(String) // required {@link GetUploadUrlExternalResponseIF#getUploadUrl() uploadUrl}
* .setFileId(String) // required {@link GetUploadUrlExternalResponseIF#getFileId() fileId}
* .build();
*
* @return A new GetUploadUrlExternalResponse builder
*/
public static GetUploadUrlExternalResponse.Builder builder() {
return new GetUploadUrlExternalResponse.Builder();
}
/**
* Builds instances of type {@link GetUploadUrlExternalResponse GetUploadUrlExternalResponse}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
*
{@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
@Generated(from = "GetUploadUrlExternalResponseIF", generator = "Immutables")
@NotThreadSafe
public static final class Builder {
private static final long INIT_BIT_OK = 0x1L;
private static final long INIT_BIT_UPLOAD_URL = 0x2L;
private static final long INIT_BIT_FILE_ID = 0x4L;
private long initBits = 0x7L;
private boolean ok;
private @Nullable ResponseMetadata responseMetadata;
private @Nullable String uploadUrl;
private @Nullable String fileId;
private Builder() {
}
/**
* Fill a builder with attribute values from the provided {@code com.hubspot.slack.client.models.response.SlackResponse} instance.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(SlackResponse instance) {
Objects.requireNonNull(instance, "instance");
from((short) 0, (Object) instance);
return this;
}
/**
* Fill a builder with attribute values from the provided {@code com.hubspot.slack.client.models.response.files.GetUploadUrlExternalResponseIF} instance.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(GetUploadUrlExternalResponseIF instance) {
Objects.requireNonNull(instance, "instance");
from((short) 0, (Object) instance);
return this;
}
private void from(short _unused, Object object) {
long bits = 0;
if (object instanceof SlackResponse) {
SlackResponse instance = (SlackResponse) object;
if ((bits & 0x2L) == 0) {
this.setOk(instance.isOk());
bits |= 0x2L;
}
if ((bits & 0x1L) == 0) {
Optional responseMetadataOptional = instance.getResponseMetadata();
if (responseMetadataOptional.isPresent()) {
setResponseMetadata(responseMetadataOptional);
}
bits |= 0x1L;
}
}
if (object instanceof GetUploadUrlExternalResponseIF) {
GetUploadUrlExternalResponseIF instance = (GetUploadUrlExternalResponseIF) object;
if ((bits & 0x1L) == 0) {
Optional responseMetadataOptional = instance.getResponseMetadata();
if (responseMetadataOptional.isPresent()) {
setResponseMetadata(responseMetadataOptional);
}
bits |= 0x1L;
}
this.setUploadUrl(instance.getUploadUrl());
if ((bits & 0x2L) == 0) {
this.setOk(instance.isOk());
bits |= 0x2L;
}
this.setFileId(instance.getFileId());
}
}
/**
* Initializes the value for the {@link GetUploadUrlExternalResponseIF#isOk() ok} attribute.
* @param ok The value for ok
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setOk(boolean ok) {
this.ok = ok;
initBits &= ~INIT_BIT_OK;
return this;
}
/**
* Initializes the optional value {@link GetUploadUrlExternalResponseIF#getResponseMetadata() responseMetadata} to responseMetadata.
* @param responseMetadata The value for responseMetadata, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
public final Builder setResponseMetadata(@Nullable ResponseMetadata responseMetadata) {
this.responseMetadata = responseMetadata;
return this;
}
/**
* Initializes the optional value {@link GetUploadUrlExternalResponseIF#getResponseMetadata() responseMetadata} to responseMetadata.
* @param responseMetadata The value for responseMetadata
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setResponseMetadata(Optional responseMetadata) {
this.responseMetadata = responseMetadata.orElse(null);
return this;
}
/**
* Initializes the value for the {@link GetUploadUrlExternalResponseIF#getUploadUrl() uploadUrl} attribute.
* @param uploadUrl The value for uploadUrl
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setUploadUrl(String uploadUrl) {
this.uploadUrl = Objects.requireNonNull(uploadUrl, "uploadUrl");
initBits &= ~INIT_BIT_UPLOAD_URL;
return this;
}
/**
* Initializes the value for the {@link GetUploadUrlExternalResponseIF#getFileId() fileId} attribute.
* @param fileId The value for fileId
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setFileId(String fileId) {
this.fileId = Objects.requireNonNull(fileId, "fileId");
initBits &= ~INIT_BIT_FILE_ID;
return this;
}
/**
* Builds a new {@link GetUploadUrlExternalResponse GetUploadUrlExternalResponse}.
* @return An immutable instance of GetUploadUrlExternalResponse
* @throws com.hubspot.immutables.validation.InvalidImmutableStateException if any required attributes are missing
*/
public GetUploadUrlExternalResponse build() {
checkRequiredAttributes();
return new GetUploadUrlExternalResponse(ok, responseMetadata, uploadUrl, fileId);
}
private boolean okIsSet() {
return (initBits & INIT_BIT_OK) == 0;
}
private boolean uploadUrlIsSet() {
return (initBits & INIT_BIT_UPLOAD_URL) == 0;
}
private boolean fileIdIsSet() {
return (initBits & INIT_BIT_FILE_ID) == 0;
}
private void checkRequiredAttributes() {
if (initBits != 0) {
throw new InvalidImmutableStateException(formatRequiredAttributesMessage());
}
}
private String formatRequiredAttributesMessage() {
List attributes = new ArrayList<>();
if (!okIsSet()) attributes.add("ok");
if (!uploadUrlIsSet()) attributes.add("uploadUrl");
if (!fileIdIsSet()) attributes.add("fileId");
return "Cannot build GetUploadUrlExternalResponse, some of required attributes are not set " + attributes;
}
}
}