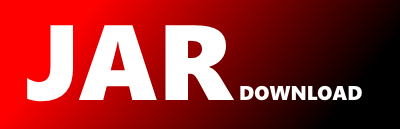
com.hubspot.slack.client.models.response.views.ModalViewResponse Maven / Gradle / Ivy
package com.hubspot.slack.client.models.response.views;
import com.fasterxml.jackson.annotation.JsonAutoDetect;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonTypeInfo;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import com.fasterxml.jackson.databind.annotation.JsonSerialize;
import com.hubspot.immutables.validation.InvalidImmutableStateException;
import com.hubspot.slack.client.models.blocks.Block;
import com.hubspot.slack.client.models.blocks.objects.Text;
import com.hubspot.slack.client.models.response.views.json.StateBlockDeserializer;
import com.hubspot.slack.client.models.response.views.json.StateBlockSerializer;
import com.hubspot.slack.client.models.views.ModalViewPayloadBase;
import com.hubspot.slack.client.models.views.ViewPayloadBase;
import edu.umd.cs.findbugs.annotations.SuppressFBWarnings;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
import javax.annotation.ParametersAreNonnullByDefault;
import javax.annotation.concurrent.Immutable;
import javax.annotation.concurrent.NotThreadSafe;
import org.immutables.value.Generated;
/**
* Immutable implementation of {@link ModalViewResponseIF}.
*
* Use the builder to create immutable instances:
* {@code ModalViewResponse.builder()}.
*/
@Generated(from = "ModalViewResponseIF", generator = "Immutables")
@SuppressWarnings({"all"})
@SuppressFBWarnings
@ParametersAreNonnullByDefault
@javax.annotation.processing.Generated("org.immutables.processor.ProxyProcessor")
@Immutable
public final class ModalViewResponse
implements ModalViewResponseIF {
private final List blocks;
private final @Nullable String privateMetadata;
private final @Nullable String callbackId;
private final @Nullable String externalId;
private transient final String type;
private final Text title;
private final @Nullable Text closeButtonText;
private final @Nullable Text submitButtonText;
private final @Nullable Boolean clearOnClose;
private final @Nullable Boolean notifyOnClose;
private final String appId;
private final String botId;
private final String currentViewId;
private final String rootViewId;
private final String teamId;
private final StateBlock stateValues;
private final String hash;
private final @Nullable String previousViewId;
private ModalViewResponse(
List blocks,
@Nullable String privateMetadata,
@Nullable String callbackId,
@Nullable String externalId,
Text title,
@Nullable Text closeButtonText,
@Nullable Text submitButtonText,
@Nullable Boolean clearOnClose,
@Nullable Boolean notifyOnClose,
String appId,
String botId,
String currentViewId,
String rootViewId,
String teamId,
StateBlock stateValues,
String hash,
@Nullable String previousViewId) {
this.blocks = blocks;
this.privateMetadata = privateMetadata;
this.callbackId = callbackId;
this.externalId = externalId;
this.title = title;
this.closeButtonText = closeButtonText;
this.submitButtonText = submitButtonText;
this.clearOnClose = clearOnClose;
this.notifyOnClose = notifyOnClose;
this.appId = appId;
this.botId = botId;
this.currentViewId = currentViewId;
this.rootViewId = rootViewId;
this.teamId = teamId;
this.stateValues = stateValues;
this.hash = hash;
this.previousViewId = previousViewId;
this.type = Objects.requireNonNull(ModalViewResponseIF.super.getType(), "type");
}
/**
* @return The value of the {@code blocks} attribute
*/
@JsonProperty
@Override
public List getBlocks() {
return blocks;
}
/**
* @return The value of the {@code privateMetadata} attribute
*/
@JsonProperty
@Override
public Optional getPrivateMetadata() {
return Optional.ofNullable(privateMetadata);
}
/**
* @return The value of the {@code callbackId} attribute
*/
@JsonProperty
@Override
public Optional getCallbackId() {
return Optional.ofNullable(callbackId);
}
/**
* @return The value of the {@code externalId} attribute
*/
@JsonProperty
@Override
public Optional getExternalId() {
return Optional.ofNullable(externalId);
}
/**
* @return The computed-at-construction value of the {@code type} attribute
*/
@JsonProperty
@Override
public String getType() {
return type;
}
/**
* @return The value of the {@code title} attribute
*/
@JsonProperty
@Override
public Text getTitle() {
return title;
}
/**
* @return The value of the {@code closeButtonText} attribute
*/
@JsonProperty("close")
@Override
public Optional getCloseButtonText() {
return Optional.ofNullable(closeButtonText);
}
/**
* @return The value of the {@code submitButtonText} attribute
*/
@JsonProperty("submit")
@Override
public Optional getSubmitButtonText() {
return Optional.ofNullable(submitButtonText);
}
/**
* @return The value of the {@code clearOnClose} attribute
*/
@JsonProperty
@Override
public Optional getClearOnClose() {
return Optional.ofNullable(clearOnClose);
}
/**
* @return The value of the {@code notifyOnClose} attribute
*/
@JsonProperty
@Override
public Optional getNotifyOnClose() {
return Optional.ofNullable(notifyOnClose);
}
/**
* @return The value of the {@code appId} attribute
*/
@JsonProperty
@Override
public String getAppId() {
return appId;
}
/**
* @return The value of the {@code botId} attribute
*/
@JsonProperty
@Override
public String getBotId() {
return botId;
}
/**
* @return The value of the {@code currentViewId} attribute
*/
@JsonProperty("id")
@Override
public String getCurrentViewId() {
return currentViewId;
}
/**
* @return The value of the {@code rootViewId} attribute
*/
@JsonProperty
@Override
public String getRootViewId() {
return rootViewId;
}
/**
* @return The value of the {@code teamId} attribute
*/
@JsonProperty
@Override
public String getTeamId() {
return teamId;
}
/**
* @return The value of the {@code stateValues} attribute
*/
@JsonProperty("state")
@JsonDeserialize(using = StateBlockDeserializer.class)
@JsonSerialize(using = StateBlockSerializer.class)
@Override
public StateBlock getStateValues() {
return stateValues;
}
/**
* @return The value of the {@code hash} attribute
*/
@JsonProperty
@Override
public String getHash() {
return hash;
}
/**
* @return The value of the {@code previousViewId} attribute
*/
@JsonProperty
@Override
public Optional getPreviousViewId() {
return Optional.ofNullable(previousViewId);
}
/**
* Copy the current immutable object with elements that replace the content of {@link ModalViewResponseIF#getBlocks() blocks}.
* @param elements The elements to set
* @return A modified copy of {@code this} object
*/
public final ModalViewResponse withBlocks(Block... elements) {
List newValue = createUnmodifiableList(false, createSafeList(Arrays.asList(elements), true, false));
return new ModalViewResponse(
newValue,
this.privateMetadata,
this.callbackId,
this.externalId,
this.title,
this.closeButtonText,
this.submitButtonText,
this.clearOnClose,
this.notifyOnClose,
this.appId,
this.botId,
this.currentViewId,
this.rootViewId,
this.teamId,
this.stateValues,
this.hash,
this.previousViewId);
}
/**
* Copy the current immutable object with elements that replace the content of {@link ModalViewResponseIF#getBlocks() blocks}.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements An iterable of blocks elements to set
* @return A modified copy of {@code this} object
*/
public final ModalViewResponse withBlocks(Iterable extends Block> elements) {
if (this.blocks == elements) return this;
List newValue = createUnmodifiableList(false, createSafeList(elements, true, false));
return new ModalViewResponse(
newValue,
this.privateMetadata,
this.callbackId,
this.externalId,
this.title,
this.closeButtonText,
this.submitButtonText,
this.clearOnClose,
this.notifyOnClose,
this.appId,
this.botId,
this.currentViewId,
this.rootViewId,
this.teamId,
this.stateValues,
this.hash,
this.previousViewId);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link ModalViewResponseIF#getPrivateMetadata() privateMetadata} attribute.
* @param value The value for privateMetadata, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final ModalViewResponse withPrivateMetadata(@Nullable String value) {
@Nullable String newValue = value;
if (Objects.equals(this.privateMetadata, newValue)) return this;
return new ModalViewResponse(
this.blocks,
newValue,
this.callbackId,
this.externalId,
this.title,
this.closeButtonText,
this.submitButtonText,
this.clearOnClose,
this.notifyOnClose,
this.appId,
this.botId,
this.currentViewId,
this.rootViewId,
this.teamId,
this.stateValues,
this.hash,
this.previousViewId);
}
/**
* Copy the current immutable object by setting an optional value for the {@link ModalViewResponseIF#getPrivateMetadata() privateMetadata} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for privateMetadata
* @return A modified copy of {@code this} object
*/
public final ModalViewResponse withPrivateMetadata(Optional optional) {
@Nullable String value = optional.orElse(null);
if (Objects.equals(this.privateMetadata, value)) return this;
return new ModalViewResponse(
this.blocks,
value,
this.callbackId,
this.externalId,
this.title,
this.closeButtonText,
this.submitButtonText,
this.clearOnClose,
this.notifyOnClose,
this.appId,
this.botId,
this.currentViewId,
this.rootViewId,
this.teamId,
this.stateValues,
this.hash,
this.previousViewId);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link ModalViewResponseIF#getCallbackId() callbackId} attribute.
* @param value The value for callbackId, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final ModalViewResponse withCallbackId(@Nullable String value) {
@Nullable String newValue = value;
if (Objects.equals(this.callbackId, newValue)) return this;
return new ModalViewResponse(
this.blocks,
this.privateMetadata,
newValue,
this.externalId,
this.title,
this.closeButtonText,
this.submitButtonText,
this.clearOnClose,
this.notifyOnClose,
this.appId,
this.botId,
this.currentViewId,
this.rootViewId,
this.teamId,
this.stateValues,
this.hash,
this.previousViewId);
}
/**
* Copy the current immutable object by setting an optional value for the {@link ModalViewResponseIF#getCallbackId() callbackId} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for callbackId
* @return A modified copy of {@code this} object
*/
public final ModalViewResponse withCallbackId(Optional optional) {
@Nullable String value = optional.orElse(null);
if (Objects.equals(this.callbackId, value)) return this;
return new ModalViewResponse(
this.blocks,
this.privateMetadata,
value,
this.externalId,
this.title,
this.closeButtonText,
this.submitButtonText,
this.clearOnClose,
this.notifyOnClose,
this.appId,
this.botId,
this.currentViewId,
this.rootViewId,
this.teamId,
this.stateValues,
this.hash,
this.previousViewId);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link ModalViewResponseIF#getExternalId() externalId} attribute.
* @param value The value for externalId, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final ModalViewResponse withExternalId(@Nullable String value) {
@Nullable String newValue = value;
if (Objects.equals(this.externalId, newValue)) return this;
return new ModalViewResponse(
this.blocks,
this.privateMetadata,
this.callbackId,
newValue,
this.title,
this.closeButtonText,
this.submitButtonText,
this.clearOnClose,
this.notifyOnClose,
this.appId,
this.botId,
this.currentViewId,
this.rootViewId,
this.teamId,
this.stateValues,
this.hash,
this.previousViewId);
}
/**
* Copy the current immutable object by setting an optional value for the {@link ModalViewResponseIF#getExternalId() externalId} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for externalId
* @return A modified copy of {@code this} object
*/
public final ModalViewResponse withExternalId(Optional optional) {
@Nullable String value = optional.orElse(null);
if (Objects.equals(this.externalId, value)) return this;
return new ModalViewResponse(
this.blocks,
this.privateMetadata,
this.callbackId,
value,
this.title,
this.closeButtonText,
this.submitButtonText,
this.clearOnClose,
this.notifyOnClose,
this.appId,
this.botId,
this.currentViewId,
this.rootViewId,
this.teamId,
this.stateValues,
this.hash,
this.previousViewId);
}
/**
* Copy the current immutable object by setting a value for the {@link ModalViewResponseIF#getTitle() title} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for title
* @return A modified copy of the {@code this} object
*/
public final ModalViewResponse withTitle(Text value) {
if (this.title == value) return this;
Text newValue = Objects.requireNonNull(value, "title");
return new ModalViewResponse(
this.blocks,
this.privateMetadata,
this.callbackId,
this.externalId,
newValue,
this.closeButtonText,
this.submitButtonText,
this.clearOnClose,
this.notifyOnClose,
this.appId,
this.botId,
this.currentViewId,
this.rootViewId,
this.teamId,
this.stateValues,
this.hash,
this.previousViewId);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link ModalViewResponseIF#getCloseButtonText() closeButtonText} attribute.
* @param value The value for closeButtonText, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final ModalViewResponse withCloseButtonText(@Nullable Text value) {
@Nullable Text newValue = value;
if (this.closeButtonText == newValue) return this;
return new ModalViewResponse(
this.blocks,
this.privateMetadata,
this.callbackId,
this.externalId,
this.title,
newValue,
this.submitButtonText,
this.clearOnClose,
this.notifyOnClose,
this.appId,
this.botId,
this.currentViewId,
this.rootViewId,
this.teamId,
this.stateValues,
this.hash,
this.previousViewId);
}
/**
* Copy the current immutable object by setting an optional value for the {@link ModalViewResponseIF#getCloseButtonText() closeButtonText} attribute.
* A shallow reference equality check is used on unboxed optional value to prevent copying of the same value by returning {@code this}.
* @param optional A value for closeButtonText
* @return A modified copy of {@code this} object
*/
@SuppressWarnings("unchecked") // safe covariant cast
public final ModalViewResponse withCloseButtonText(Optional extends Text> optional) {
@Nullable Text value = optional.orElse(null);
if (this.closeButtonText == value) return this;
return new ModalViewResponse(
this.blocks,
this.privateMetadata,
this.callbackId,
this.externalId,
this.title,
value,
this.submitButtonText,
this.clearOnClose,
this.notifyOnClose,
this.appId,
this.botId,
this.currentViewId,
this.rootViewId,
this.teamId,
this.stateValues,
this.hash,
this.previousViewId);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link ModalViewResponseIF#getSubmitButtonText() submitButtonText} attribute.
* @param value The value for submitButtonText, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final ModalViewResponse withSubmitButtonText(@Nullable Text value) {
@Nullable Text newValue = value;
if (this.submitButtonText == newValue) return this;
return new ModalViewResponse(
this.blocks,
this.privateMetadata,
this.callbackId,
this.externalId,
this.title,
this.closeButtonText,
newValue,
this.clearOnClose,
this.notifyOnClose,
this.appId,
this.botId,
this.currentViewId,
this.rootViewId,
this.teamId,
this.stateValues,
this.hash,
this.previousViewId);
}
/**
* Copy the current immutable object by setting an optional value for the {@link ModalViewResponseIF#getSubmitButtonText() submitButtonText} attribute.
* A shallow reference equality check is used on unboxed optional value to prevent copying of the same value by returning {@code this}.
* @param optional A value for submitButtonText
* @return A modified copy of {@code this} object
*/
@SuppressWarnings("unchecked") // safe covariant cast
public final ModalViewResponse withSubmitButtonText(Optional extends Text> optional) {
@Nullable Text value = optional.orElse(null);
if (this.submitButtonText == value) return this;
return new ModalViewResponse(
this.blocks,
this.privateMetadata,
this.callbackId,
this.externalId,
this.title,
this.closeButtonText,
value,
this.clearOnClose,
this.notifyOnClose,
this.appId,
this.botId,
this.currentViewId,
this.rootViewId,
this.teamId,
this.stateValues,
this.hash,
this.previousViewId);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link ModalViewResponseIF#getClearOnClose() clearOnClose} attribute.
* @param value The value for clearOnClose, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final ModalViewResponse withClearOnClose(@Nullable Boolean value) {
@Nullable Boolean newValue = value;
if (Objects.equals(this.clearOnClose, newValue)) return this;
return new ModalViewResponse(
this.blocks,
this.privateMetadata,
this.callbackId,
this.externalId,
this.title,
this.closeButtonText,
this.submitButtonText,
newValue,
this.notifyOnClose,
this.appId,
this.botId,
this.currentViewId,
this.rootViewId,
this.teamId,
this.stateValues,
this.hash,
this.previousViewId);
}
/**
* Copy the current immutable object by setting an optional value for the {@link ModalViewResponseIF#getClearOnClose() clearOnClose} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for clearOnClose
* @return A modified copy of {@code this} object
*/
public final ModalViewResponse withClearOnClose(Optional optional) {
@Nullable Boolean value = optional.orElse(null);
if (Objects.equals(this.clearOnClose, value)) return this;
return new ModalViewResponse(
this.blocks,
this.privateMetadata,
this.callbackId,
this.externalId,
this.title,
this.closeButtonText,
this.submitButtonText,
value,
this.notifyOnClose,
this.appId,
this.botId,
this.currentViewId,
this.rootViewId,
this.teamId,
this.stateValues,
this.hash,
this.previousViewId);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link ModalViewResponseIF#getNotifyOnClose() notifyOnClose} attribute.
* @param value The value for notifyOnClose, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final ModalViewResponse withNotifyOnClose(@Nullable Boolean value) {
@Nullable Boolean newValue = value;
if (Objects.equals(this.notifyOnClose, newValue)) return this;
return new ModalViewResponse(
this.blocks,
this.privateMetadata,
this.callbackId,
this.externalId,
this.title,
this.closeButtonText,
this.submitButtonText,
this.clearOnClose,
newValue,
this.appId,
this.botId,
this.currentViewId,
this.rootViewId,
this.teamId,
this.stateValues,
this.hash,
this.previousViewId);
}
/**
* Copy the current immutable object by setting an optional value for the {@link ModalViewResponseIF#getNotifyOnClose() notifyOnClose} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for notifyOnClose
* @return A modified copy of {@code this} object
*/
public final ModalViewResponse withNotifyOnClose(Optional optional) {
@Nullable Boolean value = optional.orElse(null);
if (Objects.equals(this.notifyOnClose, value)) return this;
return new ModalViewResponse(
this.blocks,
this.privateMetadata,
this.callbackId,
this.externalId,
this.title,
this.closeButtonText,
this.submitButtonText,
this.clearOnClose,
value,
this.appId,
this.botId,
this.currentViewId,
this.rootViewId,
this.teamId,
this.stateValues,
this.hash,
this.previousViewId);
}
/**
* Copy the current immutable object by setting a value for the {@link ModalViewResponseIF#getAppId() appId} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for appId
* @return A modified copy of the {@code this} object
*/
public final ModalViewResponse withAppId(String value) {
String newValue = Objects.requireNonNull(value, "appId");
if (this.appId.equals(newValue)) return this;
return new ModalViewResponse(
this.blocks,
this.privateMetadata,
this.callbackId,
this.externalId,
this.title,
this.closeButtonText,
this.submitButtonText,
this.clearOnClose,
this.notifyOnClose,
newValue,
this.botId,
this.currentViewId,
this.rootViewId,
this.teamId,
this.stateValues,
this.hash,
this.previousViewId);
}
/**
* Copy the current immutable object by setting a value for the {@link ModalViewResponseIF#getBotId() botId} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for botId
* @return A modified copy of the {@code this} object
*/
public final ModalViewResponse withBotId(String value) {
String newValue = Objects.requireNonNull(value, "botId");
if (this.botId.equals(newValue)) return this;
return new ModalViewResponse(
this.blocks,
this.privateMetadata,
this.callbackId,
this.externalId,
this.title,
this.closeButtonText,
this.submitButtonText,
this.clearOnClose,
this.notifyOnClose,
this.appId,
newValue,
this.currentViewId,
this.rootViewId,
this.teamId,
this.stateValues,
this.hash,
this.previousViewId);
}
/**
* Copy the current immutable object by setting a value for the {@link ModalViewResponseIF#getCurrentViewId() currentViewId} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for currentViewId
* @return A modified copy of the {@code this} object
*/
public final ModalViewResponse withCurrentViewId(String value) {
String newValue = Objects.requireNonNull(value, "currentViewId");
if (this.currentViewId.equals(newValue)) return this;
return new ModalViewResponse(
this.blocks,
this.privateMetadata,
this.callbackId,
this.externalId,
this.title,
this.closeButtonText,
this.submitButtonText,
this.clearOnClose,
this.notifyOnClose,
this.appId,
this.botId,
newValue,
this.rootViewId,
this.teamId,
this.stateValues,
this.hash,
this.previousViewId);
}
/**
* Copy the current immutable object by setting a value for the {@link ModalViewResponseIF#getRootViewId() rootViewId} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for rootViewId
* @return A modified copy of the {@code this} object
*/
public final ModalViewResponse withRootViewId(String value) {
String newValue = Objects.requireNonNull(value, "rootViewId");
if (this.rootViewId.equals(newValue)) return this;
return new ModalViewResponse(
this.blocks,
this.privateMetadata,
this.callbackId,
this.externalId,
this.title,
this.closeButtonText,
this.submitButtonText,
this.clearOnClose,
this.notifyOnClose,
this.appId,
this.botId,
this.currentViewId,
newValue,
this.teamId,
this.stateValues,
this.hash,
this.previousViewId);
}
/**
* Copy the current immutable object by setting a value for the {@link ModalViewResponseIF#getTeamId() teamId} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for teamId
* @return A modified copy of the {@code this} object
*/
public final ModalViewResponse withTeamId(String value) {
String newValue = Objects.requireNonNull(value, "teamId");
if (this.teamId.equals(newValue)) return this;
return new ModalViewResponse(
this.blocks,
this.privateMetadata,
this.callbackId,
this.externalId,
this.title,
this.closeButtonText,
this.submitButtonText,
this.clearOnClose,
this.notifyOnClose,
this.appId,
this.botId,
this.currentViewId,
this.rootViewId,
newValue,
this.stateValues,
this.hash,
this.previousViewId);
}
/**
* Copy the current immutable object by setting a value for the {@link ModalViewResponseIF#getStateValues() stateValues} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for stateValues
* @return A modified copy of the {@code this} object
*/
public final ModalViewResponse withStateValues(StateBlock value) {
if (this.stateValues == value) return this;
StateBlock newValue = Objects.requireNonNull(value, "stateValues");
return new ModalViewResponse(
this.blocks,
this.privateMetadata,
this.callbackId,
this.externalId,
this.title,
this.closeButtonText,
this.submitButtonText,
this.clearOnClose,
this.notifyOnClose,
this.appId,
this.botId,
this.currentViewId,
this.rootViewId,
this.teamId,
newValue,
this.hash,
this.previousViewId);
}
/**
* Copy the current immutable object by setting a value for the {@link ModalViewResponseIF#getHash() hash} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for hash
* @return A modified copy of the {@code this} object
*/
public final ModalViewResponse withHash(String value) {
String newValue = Objects.requireNonNull(value, "hash");
if (this.hash.equals(newValue)) return this;
return new ModalViewResponse(
this.blocks,
this.privateMetadata,
this.callbackId,
this.externalId,
this.title,
this.closeButtonText,
this.submitButtonText,
this.clearOnClose,
this.notifyOnClose,
this.appId,
this.botId,
this.currentViewId,
this.rootViewId,
this.teamId,
this.stateValues,
newValue,
this.previousViewId);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link ModalViewResponseIF#getPreviousViewId() previousViewId} attribute.
* @param value The value for previousViewId, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final ModalViewResponse withPreviousViewId(@Nullable String value) {
@Nullable String newValue = value;
if (Objects.equals(this.previousViewId, newValue)) return this;
return new ModalViewResponse(
this.blocks,
this.privateMetadata,
this.callbackId,
this.externalId,
this.title,
this.closeButtonText,
this.submitButtonText,
this.clearOnClose,
this.notifyOnClose,
this.appId,
this.botId,
this.currentViewId,
this.rootViewId,
this.teamId,
this.stateValues,
this.hash,
newValue);
}
/**
* Copy the current immutable object by setting an optional value for the {@link ModalViewResponseIF#getPreviousViewId() previousViewId} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for previousViewId
* @return A modified copy of {@code this} object
*/
public final ModalViewResponse withPreviousViewId(Optional optional) {
@Nullable String value = optional.orElse(null);
if (Objects.equals(this.previousViewId, value)) return this;
return new ModalViewResponse(
this.blocks,
this.privateMetadata,
this.callbackId,
this.externalId,
this.title,
this.closeButtonText,
this.submitButtonText,
this.clearOnClose,
this.notifyOnClose,
this.appId,
this.botId,
this.currentViewId,
this.rootViewId,
this.teamId,
this.stateValues,
this.hash,
value);
}
/**
* This instance is equal to all instances of {@code ModalViewResponse} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(@Nullable Object another) {
if (this == another) return true;
return another instanceof ModalViewResponse
&& equalTo(0, (ModalViewResponse) another);
}
private boolean equalTo(int synthetic, ModalViewResponse another) {
return blocks.equals(another.blocks)
&& Objects.equals(privateMetadata, another.privateMetadata)
&& Objects.equals(callbackId, another.callbackId)
&& Objects.equals(externalId, another.externalId)
&& type.equals(another.type)
&& title.equals(another.title)
&& Objects.equals(closeButtonText, another.closeButtonText)
&& Objects.equals(submitButtonText, another.submitButtonText)
&& Objects.equals(clearOnClose, another.clearOnClose)
&& Objects.equals(notifyOnClose, another.notifyOnClose)
&& appId.equals(another.appId)
&& botId.equals(another.botId)
&& currentViewId.equals(another.currentViewId)
&& rootViewId.equals(another.rootViewId)
&& teamId.equals(another.teamId)
&& stateValues.equals(another.stateValues)
&& hash.equals(another.hash)
&& Objects.equals(previousViewId, another.previousViewId);
}
/**
* Computes a hash code from attributes: {@code blocks}, {@code privateMetadata}, {@code callbackId}, {@code externalId}, {@code type}, {@code title}, {@code closeButtonText}, {@code submitButtonText}, {@code clearOnClose}, {@code notifyOnClose}, {@code appId}, {@code botId}, {@code currentViewId}, {@code rootViewId}, {@code teamId}, {@code stateValues}, {@code hash}, {@code previousViewId}.
* @return hashCode value
*/
@Override
public int hashCode() {
int h = 5381;
h += (h << 5) + blocks.hashCode();
h += (h << 5) + Objects.hashCode(privateMetadata);
h += (h << 5) + Objects.hashCode(callbackId);
h += (h << 5) + Objects.hashCode(externalId);
h += (h << 5) + type.hashCode();
h += (h << 5) + title.hashCode();
h += (h << 5) + Objects.hashCode(closeButtonText);
h += (h << 5) + Objects.hashCode(submitButtonText);
h += (h << 5) + Objects.hashCode(clearOnClose);
h += (h << 5) + Objects.hashCode(notifyOnClose);
h += (h << 5) + appId.hashCode();
h += (h << 5) + botId.hashCode();
h += (h << 5) + currentViewId.hashCode();
h += (h << 5) + rootViewId.hashCode();
h += (h << 5) + teamId.hashCode();
h += (h << 5) + stateValues.hashCode();
h += (h << 5) + hash.hashCode();
h += (h << 5) + Objects.hashCode(previousViewId);
return h;
}
/**
* Prints the immutable value {@code ModalViewResponse} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
StringBuilder builder = new StringBuilder("ModalViewResponse{");
builder.append("blocks=").append(blocks);
if (privateMetadata != null) {
builder.append(", ");
builder.append("privateMetadata=").append(privateMetadata);
}
if (callbackId != null) {
builder.append(", ");
builder.append("callbackId=").append(callbackId);
}
if (externalId != null) {
builder.append(", ");
builder.append("externalId=").append(externalId);
}
builder.append(", ");
builder.append("type=").append(type);
builder.append(", ");
builder.append("title=").append(title);
if (closeButtonText != null) {
builder.append(", ");
builder.append("closeButtonText=").append(closeButtonText);
}
if (submitButtonText != null) {
builder.append(", ");
builder.append("submitButtonText=").append(submitButtonText);
}
if (clearOnClose != null) {
builder.append(", ");
builder.append("clearOnClose=").append(clearOnClose);
}
if (notifyOnClose != null) {
builder.append(", ");
builder.append("notifyOnClose=").append(notifyOnClose);
}
builder.append(", ");
builder.append("appId=").append(appId);
builder.append(", ");
builder.append("botId=").append(botId);
builder.append(", ");
builder.append("currentViewId=").append(currentViewId);
builder.append(", ");
builder.append("rootViewId=").append(rootViewId);
builder.append(", ");
builder.append("teamId=").append(teamId);
builder.append(", ");
builder.append("stateValues=").append(stateValues);
builder.append(", ");
builder.append("hash=").append(hash);
if (previousViewId != null) {
builder.append(", ");
builder.append("previousViewId=").append(previousViewId);
}
return builder.append("}").toString();
}
/**
* Utility type used to correctly read immutable object from JSON representation.
* @deprecated Do not use this type directly, it exists only for the Jackson-binding infrastructure
*/
@Generated(from = "ModalViewResponseIF", generator = "Immutables")
@Deprecated
@JsonTypeInfo(use=JsonTypeInfo.Id.NONE)
@JsonAutoDetect(fieldVisibility = JsonAutoDetect.Visibility.NONE)
static final class Json implements ModalViewResponseIF {
@Nullable List blocks = Collections.emptyList();
@Nullable Optional privateMetadata = Optional.empty();
@Nullable Optional callbackId = Optional.empty();
@Nullable Optional externalId = Optional.empty();
@Nullable Text title;
@Nullable Optional closeButtonText = Optional.empty();
@Nullable Optional submitButtonText = Optional.empty();
@Nullable Optional clearOnClose = Optional.empty();
@Nullable Optional notifyOnClose = Optional.empty();
@Nullable String appId;
@Nullable String botId;
@Nullable String currentViewId;
@Nullable String rootViewId;
@Nullable String teamId;
@Nullable StateBlock stateValues;
@Nullable String hash;
@Nullable Optional previousViewId = Optional.empty();
@JsonProperty
public void setBlocks(List blocks) {
this.blocks = blocks;
}
@JsonProperty
public void setPrivateMetadata(Optional privateMetadata) {
this.privateMetadata = privateMetadata;
}
@JsonProperty
public void setCallbackId(Optional callbackId) {
this.callbackId = callbackId;
}
@JsonProperty
public void setExternalId(Optional externalId) {
this.externalId = externalId;
}
@JsonProperty
public void setTitle(Text title) {
this.title = title;
}
@JsonProperty("close")
public void setCloseButtonText(Optional closeButtonText) {
this.closeButtonText = closeButtonText;
}
@JsonProperty("submit")
public void setSubmitButtonText(Optional submitButtonText) {
this.submitButtonText = submitButtonText;
}
@JsonProperty
public void setClearOnClose(Optional clearOnClose) {
this.clearOnClose = clearOnClose;
}
@JsonProperty
public void setNotifyOnClose(Optional notifyOnClose) {
this.notifyOnClose = notifyOnClose;
}
@JsonProperty
public void setAppId(String appId) {
this.appId = appId;
}
@JsonProperty
public void setBotId(String botId) {
this.botId = botId;
}
@JsonProperty("id")
public void setCurrentViewId(String currentViewId) {
this.currentViewId = currentViewId;
}
@JsonProperty
public void setRootViewId(String rootViewId) {
this.rootViewId = rootViewId;
}
@JsonProperty
public void setTeamId(String teamId) {
this.teamId = teamId;
}
@JsonProperty("state")
@JsonDeserialize(using = StateBlockDeserializer.class)
@JsonSerialize(using = StateBlockSerializer.class)
public void setStateValues(StateBlock stateValues) {
this.stateValues = stateValues;
}
@JsonProperty
public void setHash(String hash) {
this.hash = hash;
}
@JsonProperty
public void setPreviousViewId(Optional previousViewId) {
this.previousViewId = previousViewId;
}
@Override
public List getBlocks() { throw new UnsupportedOperationException(); }
@Override
public Optional getPrivateMetadata() { throw new UnsupportedOperationException(); }
@Override
public Optional getCallbackId() { throw new UnsupportedOperationException(); }
@Override
public Optional getExternalId() { throw new UnsupportedOperationException(); }
@JsonIgnore
@Override
public String getType() { throw new UnsupportedOperationException(); }
@Override
public Text getTitle() { throw new UnsupportedOperationException(); }
@Override
public Optional getCloseButtonText() { throw new UnsupportedOperationException(); }
@Override
public Optional getSubmitButtonText() { throw new UnsupportedOperationException(); }
@Override
public Optional getClearOnClose() { throw new UnsupportedOperationException(); }
@Override
public Optional getNotifyOnClose() { throw new UnsupportedOperationException(); }
@Override
public String getAppId() { throw new UnsupportedOperationException(); }
@Override
public String getBotId() { throw new UnsupportedOperationException(); }
@Override
public String getCurrentViewId() { throw new UnsupportedOperationException(); }
@Override
public String getRootViewId() { throw new UnsupportedOperationException(); }
@Override
public String getTeamId() { throw new UnsupportedOperationException(); }
@Override
public StateBlock getStateValues() { throw new UnsupportedOperationException(); }
@Override
public String getHash() { throw new UnsupportedOperationException(); }
@Override
public Optional getPreviousViewId() { throw new UnsupportedOperationException(); }
}
/**
* @param json A JSON-bindable data structure
* @return An immutable value type
* @deprecated Do not use this method directly, it exists only for the Jackson-binding infrastructure
*/
@Deprecated
@JsonCreator(mode = JsonCreator.Mode.DELEGATING)
static ModalViewResponse fromJson(Json json) {
ModalViewResponse.Builder builder = ModalViewResponse.builder();
if (json.blocks != null) {
builder.addAllBlocks(json.blocks);
}
if (json.privateMetadata != null) {
builder.setPrivateMetadata(json.privateMetadata);
}
if (json.callbackId != null) {
builder.setCallbackId(json.callbackId);
}
if (json.externalId != null) {
builder.setExternalId(json.externalId);
}
if (json.title != null) {
builder.setTitle(json.title);
}
if (json.closeButtonText != null) {
builder.setCloseButtonText(json.closeButtonText);
}
if (json.submitButtonText != null) {
builder.setSubmitButtonText(json.submitButtonText);
}
if (json.clearOnClose != null) {
builder.setClearOnClose(json.clearOnClose);
}
if (json.notifyOnClose != null) {
builder.setNotifyOnClose(json.notifyOnClose);
}
if (json.appId != null) {
builder.setAppId(json.appId);
}
if (json.botId != null) {
builder.setBotId(json.botId);
}
if (json.currentViewId != null) {
builder.setCurrentViewId(json.currentViewId);
}
if (json.rootViewId != null) {
builder.setRootViewId(json.rootViewId);
}
if (json.teamId != null) {
builder.setTeamId(json.teamId);
}
if (json.stateValues != null) {
builder.setStateValues(json.stateValues);
}
if (json.hash != null) {
builder.setHash(json.hash);
}
if (json.previousViewId != null) {
builder.setPreviousViewId(json.previousViewId);
}
return builder.build();
}
/**
* Creates an immutable copy of a {@link ModalViewResponseIF} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable ModalViewResponse instance
*/
public static ModalViewResponse copyOf(ModalViewResponseIF instance) {
if (instance instanceof ModalViewResponse) {
return (ModalViewResponse) instance;
}
return ModalViewResponse.builder()
.from(instance)
.build();
}
/**
* Creates a builder for {@link ModalViewResponse ModalViewResponse}.
*
* ModalViewResponse.builder()
* .addBlocks|addAllBlocks(com.hubspot.slack.client.models.blocks.Block) // {@link ModalViewResponseIF#getBlocks() blocks} elements
* .setPrivateMetadata(String) // optional {@link ModalViewResponseIF#getPrivateMetadata() privateMetadata}
* .setCallbackId(String) // optional {@link ModalViewResponseIF#getCallbackId() callbackId}
* .setExternalId(String) // optional {@link ModalViewResponseIF#getExternalId() externalId}
* .setTitle(com.hubspot.slack.client.models.blocks.objects.Text) // required {@link ModalViewResponseIF#getTitle() title}
* .setCloseButtonText(com.hubspot.slack.client.models.blocks.objects.Text) // optional {@link ModalViewResponseIF#getCloseButtonText() closeButtonText}
* .setSubmitButtonText(com.hubspot.slack.client.models.blocks.objects.Text) // optional {@link ModalViewResponseIF#getSubmitButtonText() submitButtonText}
* .setClearOnClose(Boolean) // optional {@link ModalViewResponseIF#getClearOnClose() clearOnClose}
* .setNotifyOnClose(Boolean) // optional {@link ModalViewResponseIF#getNotifyOnClose() notifyOnClose}
* .setAppId(String) // required {@link ModalViewResponseIF#getAppId() appId}
* .setBotId(String) // required {@link ModalViewResponseIF#getBotId() botId}
* .setCurrentViewId(String) // required {@link ModalViewResponseIF#getCurrentViewId() currentViewId}
* .setRootViewId(String) // required {@link ModalViewResponseIF#getRootViewId() rootViewId}
* .setTeamId(String) // required {@link ModalViewResponseIF#getTeamId() teamId}
* .setStateValues(com.hubspot.slack.client.models.response.views.StateBlock) // required {@link ModalViewResponseIF#getStateValues() stateValues}
* .setHash(String) // required {@link ModalViewResponseIF#getHash() hash}
* .setPreviousViewId(String) // optional {@link ModalViewResponseIF#getPreviousViewId() previousViewId}
* .build();
*
* @return A new ModalViewResponse builder
*/
public static ModalViewResponse.Builder builder() {
return new ModalViewResponse.Builder();
}
/**
* Builds instances of type {@link ModalViewResponse ModalViewResponse}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
@Generated(from = "ModalViewResponseIF", generator = "Immutables")
@NotThreadSafe
public static final class Builder {
private static final long INIT_BIT_TITLE = 0x1L;
private static final long INIT_BIT_APP_ID = 0x2L;
private static final long INIT_BIT_BOT_ID = 0x4L;
private static final long INIT_BIT_CURRENT_VIEW_ID = 0x8L;
private static final long INIT_BIT_ROOT_VIEW_ID = 0x10L;
private static final long INIT_BIT_TEAM_ID = 0x20L;
private static final long INIT_BIT_STATE_VALUES = 0x40L;
private static final long INIT_BIT_HASH = 0x80L;
private long initBits = 0xffL;
private List blocks = new ArrayList();
private @Nullable String privateMetadata;
private @Nullable String callbackId;
private @Nullable String externalId;
private @Nullable Text title;
private @Nullable Text closeButtonText;
private @Nullable Text submitButtonText;
private @Nullable Boolean clearOnClose;
private @Nullable Boolean notifyOnClose;
private @Nullable String appId;
private @Nullable String botId;
private @Nullable String currentViewId;
private @Nullable String rootViewId;
private @Nullable String teamId;
private @Nullable StateBlock stateValues;
private @Nullable String hash;
private @Nullable String previousViewId;
private Builder() {
}
/**
* Fill a builder with attribute values from the provided {@code com.hubspot.slack.client.models.views.ModalViewPayloadBase} instance.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(ModalViewPayloadBase instance) {
Objects.requireNonNull(instance, "instance");
from((short) 0, (Object) instance);
return this;
}
/**
* Fill a builder with attribute values from the provided {@code com.hubspot.slack.client.models.views.ViewPayloadBase} instance.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(ViewPayloadBase instance) {
Objects.requireNonNull(instance, "instance");
from((short) 0, (Object) instance);
return this;
}
/**
* Fill a builder with attribute values from the provided {@code com.hubspot.slack.client.models.response.views.ModalViewResponseIF} instance.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(ModalViewResponseIF instance) {
Objects.requireNonNull(instance, "instance");
from((short) 0, (Object) instance);
return this;
}
/**
* Fill a builder with attribute values from the provided {@code com.hubspot.slack.client.models.response.views.ViewResponseBase} instance.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(ViewResponseBase instance) {
Objects.requireNonNull(instance, "instance");
from((short) 0, (Object) instance);
return this;
}
private void from(short _unused, Object object) {
long bits = 0;
if (object instanceof ModalViewPayloadBase) {
ModalViewPayloadBase instance = (ModalViewPayloadBase) object;
if ((bits & 0x1L) == 0) {
Optional closeButtonTextOptional = instance.getCloseButtonText();
if (closeButtonTextOptional.isPresent()) {
setCloseButtonText(closeButtonTextOptional);
}
bits |= 0x1L;
}
if ((bits & 0x400L) == 0) {
Optional callbackIdOptional = instance.getCallbackId();
if (callbackIdOptional.isPresent()) {
setCallbackId(callbackIdOptional);
}
bits |= 0x400L;
}
if ((bits & 0x2L) == 0) {
addAllBlocks(instance.getBlocks());
bits |= 0x2L;
}
if ((bits & 0x4L) == 0) {
Optional externalIdOptional = instance.getExternalId();
if (externalIdOptional.isPresent()) {
setExternalId(externalIdOptional);
}
bits |= 0x4L;
}
if ((bits & 0x8L) == 0) {
Optional privateMetadataOptional = instance.getPrivateMetadata();
if (privateMetadataOptional.isPresent()) {
setPrivateMetadata(privateMetadataOptional);
}
bits |= 0x8L;
}
if ((bits & 0x2000L) == 0) {
Optional submitButtonTextOptional = instance.getSubmitButtonText();
if (submitButtonTextOptional.isPresent()) {
setSubmitButtonText(submitButtonTextOptional);
}
bits |= 0x2000L;
}
if ((bits & 0x4000L) == 0) {
Optional notifyOnCloseOptional = instance.getNotifyOnClose();
if (notifyOnCloseOptional.isPresent()) {
setNotifyOnClose(notifyOnCloseOptional);
}
bits |= 0x4000L;
}
if ((bits & 0x20L) == 0) {
this.setTitle(instance.getTitle());
bits |= 0x20L;
}
if ((bits & 0x40L) == 0) {
Optional clearOnCloseOptional = instance.getClearOnClose();
if (clearOnCloseOptional.isPresent()) {
setClearOnClose(clearOnCloseOptional);
}
bits |= 0x40L;
}
}
if (object instanceof ViewPayloadBase) {
ViewPayloadBase instance = (ViewPayloadBase) object;
if ((bits & 0x4L) == 0) {
Optional externalIdOptional = instance.getExternalId();
if (externalIdOptional.isPresent()) {
setExternalId(externalIdOptional);
}
bits |= 0x4L;
}
if ((bits & 0x8L) == 0) {
Optional privateMetadataOptional = instance.getPrivateMetadata();
if (privateMetadataOptional.isPresent()) {
setPrivateMetadata(privateMetadataOptional);
}
bits |= 0x8L;
}
if ((bits & 0x400L) == 0) {
Optional callbackIdOptional = instance.getCallbackId();
if (callbackIdOptional.isPresent()) {
setCallbackId(callbackIdOptional);
}
bits |= 0x400L;
}
if ((bits & 0x2L) == 0) {
addAllBlocks(instance.getBlocks());
bits |= 0x2L;
}
}
if (object instanceof ModalViewResponseIF) {
ModalViewResponseIF instance = (ModalViewResponseIF) object;
if ((bits & 0x1L) == 0) {
Optional closeButtonTextOptional = instance.getCloseButtonText();
if (closeButtonTextOptional.isPresent()) {
setCloseButtonText(closeButtonTextOptional);
}
bits |= 0x1L;
}
if ((bits & 0x2L) == 0) {
addAllBlocks(instance.getBlocks());
bits |= 0x2L;
}
if ((bits & 0x4L) == 0) {
Optional externalIdOptional = instance.getExternalId();
if (externalIdOptional.isPresent()) {
setExternalId(externalIdOptional);
}
bits |= 0x4L;
}
if ((bits & 0x8L) == 0) {
Optional privateMetadataOptional = instance.getPrivateMetadata();
if (privateMetadataOptional.isPresent()) {
setPrivateMetadata(privateMetadataOptional);
}
bits |= 0x8L;
}
if ((bits & 0x10L) == 0) {
this.setStateValues(instance.getStateValues());
bits |= 0x10L;
}
if ((bits & 0x20L) == 0) {
this.setTitle(instance.getTitle());
bits |= 0x20L;
}
if ((bits & 0x40L) == 0) {
Optional clearOnCloseOptional = instance.getClearOnClose();
if (clearOnCloseOptional.isPresent()) {
setClearOnClose(clearOnCloseOptional);
}
bits |= 0x40L;
}
if ((bits & 0x80L) == 0) {
Optional previousViewIdOptional = instance.getPreviousViewId();
if (previousViewIdOptional.isPresent()) {
setPreviousViewId(previousViewIdOptional);
}
bits |= 0x80L;
}
if ((bits & 0x100L) == 0) {
this.setCurrentViewId(instance.getCurrentViewId());
bits |= 0x100L;
}
if ((bits & 0x200L) == 0) {
this.setRootViewId(instance.getRootViewId());
bits |= 0x200L;
}
if ((bits & 0x400L) == 0) {
Optional callbackIdOptional = instance.getCallbackId();
if (callbackIdOptional.isPresent()) {
setCallbackId(callbackIdOptional);
}
bits |= 0x400L;
}
if ((bits & 0x800L) == 0) {
this.setAppId(instance.getAppId());
bits |= 0x800L;
}
if ((bits & 0x1000L) == 0) {
this.setTeamId(instance.getTeamId());
bits |= 0x1000L;
}
if ((bits & 0x2000L) == 0) {
Optional submitButtonTextOptional = instance.getSubmitButtonText();
if (submitButtonTextOptional.isPresent()) {
setSubmitButtonText(submitButtonTextOptional);
}
bits |= 0x2000L;
}
if ((bits & 0x4000L) == 0) {
Optional notifyOnCloseOptional = instance.getNotifyOnClose();
if (notifyOnCloseOptional.isPresent()) {
setNotifyOnClose(notifyOnCloseOptional);
}
bits |= 0x4000L;
}
if ((bits & 0x8000L) == 0) {
this.setBotId(instance.getBotId());
bits |= 0x8000L;
}
if ((bits & 0x10000L) == 0) {
this.setHash(instance.getHash());
bits |= 0x10000L;
}
}
if (object instanceof ViewResponseBase) {
ViewResponseBase instance = (ViewResponseBase) object;
if ((bits & 0x400L) == 0) {
Optional callbackIdOptional = instance.getCallbackId();
if (callbackIdOptional.isPresent()) {
setCallbackId(callbackIdOptional);
}
bits |= 0x400L;
}
if ((bits & 0x2L) == 0) {
addAllBlocks(instance.getBlocks());
bits |= 0x2L;
}
if ((bits & 0x800L) == 0) {
this.setAppId(instance.getAppId());
bits |= 0x800L;
}
if ((bits & 0x1000L) == 0) {
this.setTeamId(instance.getTeamId());
bits |= 0x1000L;
}
if ((bits & 0x4L) == 0) {
Optional externalIdOptional = instance.getExternalId();
if (externalIdOptional.isPresent()) {
setExternalId(externalIdOptional);
}
bits |= 0x4L;
}
if ((bits & 0x8L) == 0) {
Optional privateMetadataOptional = instance.getPrivateMetadata();
if (privateMetadataOptional.isPresent()) {
setPrivateMetadata(privateMetadataOptional);
}
bits |= 0x8L;
}
if ((bits & 0x10L) == 0) {
this.setStateValues(instance.getStateValues());
bits |= 0x10L;
}
if ((bits & 0x8000L) == 0) {
this.setBotId(instance.getBotId());
bits |= 0x8000L;
}
if ((bits & 0x80L) == 0) {
Optional previousViewIdOptional = instance.getPreviousViewId();
if (previousViewIdOptional.isPresent()) {
setPreviousViewId(previousViewIdOptional);
}
bits |= 0x80L;
}
if ((bits & 0x100L) == 0) {
this.setCurrentViewId(instance.getCurrentViewId());
bits |= 0x100L;
}
if ((bits & 0x10000L) == 0) {
this.setHash(instance.getHash());
bits |= 0x10000L;
}
if ((bits & 0x200L) == 0) {
this.setRootViewId(instance.getRootViewId());
bits |= 0x200L;
}
}
}
/**
* Adds one element to {@link ModalViewResponseIF#getBlocks() blocks} list.
* @param element A blocks element
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addBlocks(Block element) {
this.blocks.add(Objects.requireNonNull(element, "blocks element"));
return this;
}
/**
* Adds elements to {@link ModalViewResponseIF#getBlocks() blocks} list.
* @param elements An array of blocks elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addBlocks(Block... elements) {
for (Block element : elements) {
this.blocks.add(Objects.requireNonNull(element, "blocks element"));
}
return this;
}
/**
* Sets or replaces all elements for {@link ModalViewResponseIF#getBlocks() blocks} list.
* @param elements An iterable of blocks elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setBlocks(Iterable extends Block> elements) {
this.blocks.clear();
return addAllBlocks(elements);
}
/**
* Adds elements to {@link ModalViewResponseIF#getBlocks() blocks} list.
* @param elements An iterable of blocks elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addAllBlocks(Iterable extends Block> elements) {
for (Block element : elements) {
this.blocks.add(Objects.requireNonNull(element, "blocks element"));
}
return this;
}
/**
* Initializes the optional value {@link ModalViewResponseIF#getPrivateMetadata() privateMetadata} to privateMetadata.
* @param privateMetadata The value for privateMetadata, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
public final Builder setPrivateMetadata(@Nullable String privateMetadata) {
this.privateMetadata = privateMetadata;
return this;
}
/**
* Initializes the optional value {@link ModalViewResponseIF#getPrivateMetadata() privateMetadata} to privateMetadata.
* @param privateMetadata The value for privateMetadata
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setPrivateMetadata(Optional privateMetadata) {
this.privateMetadata = privateMetadata.orElse(null);
return this;
}
/**
* Initializes the optional value {@link ModalViewResponseIF#getCallbackId() callbackId} to callbackId.
* @param callbackId The value for callbackId, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
public final Builder setCallbackId(@Nullable String callbackId) {
this.callbackId = callbackId;
return this;
}
/**
* Initializes the optional value {@link ModalViewResponseIF#getCallbackId() callbackId} to callbackId.
* @param callbackId The value for callbackId
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setCallbackId(Optional callbackId) {
this.callbackId = callbackId.orElse(null);
return this;
}
/**
* Initializes the optional value {@link ModalViewResponseIF#getExternalId() externalId} to externalId.
* @param externalId The value for externalId, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
public final Builder setExternalId(@Nullable String externalId) {
this.externalId = externalId;
return this;
}
/**
* Initializes the optional value {@link ModalViewResponseIF#getExternalId() externalId} to externalId.
* @param externalId The value for externalId
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setExternalId(Optional externalId) {
this.externalId = externalId.orElse(null);
return this;
}
/**
* Initializes the value for the {@link ModalViewResponseIF#getTitle() title} attribute.
* @param title The value for title
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setTitle(Text title) {
this.title = Objects.requireNonNull(title, "title");
initBits &= ~INIT_BIT_TITLE;
return this;
}
/**
* Initializes the optional value {@link ModalViewResponseIF#getCloseButtonText() closeButtonText} to closeButtonText.
* @param closeButtonText The value for closeButtonText, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
public final Builder setCloseButtonText(@Nullable Text closeButtonText) {
this.closeButtonText = closeButtonText;
return this;
}
/**
* Initializes the optional value {@link ModalViewResponseIF#getCloseButtonText() closeButtonText} to closeButtonText.
* @param closeButtonText The value for closeButtonText
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setCloseButtonText(Optional extends Text> closeButtonText) {
this.closeButtonText = closeButtonText.orElse(null);
return this;
}
/**
* Initializes the optional value {@link ModalViewResponseIF#getSubmitButtonText() submitButtonText} to submitButtonText.
* @param submitButtonText The value for submitButtonText, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
public final Builder setSubmitButtonText(@Nullable Text submitButtonText) {
this.submitButtonText = submitButtonText;
return this;
}
/**
* Initializes the optional value {@link ModalViewResponseIF#getSubmitButtonText() submitButtonText} to submitButtonText.
* @param submitButtonText The value for submitButtonText
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setSubmitButtonText(Optional extends Text> submitButtonText) {
this.submitButtonText = submitButtonText.orElse(null);
return this;
}
/**
* Initializes the optional value {@link ModalViewResponseIF#getClearOnClose() clearOnClose} to clearOnClose.
* @param clearOnClose The value for clearOnClose, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
public final Builder setClearOnClose(@Nullable Boolean clearOnClose) {
this.clearOnClose = clearOnClose;
return this;
}
/**
* Initializes the optional value {@link ModalViewResponseIF#getClearOnClose() clearOnClose} to clearOnClose.
* @param clearOnClose The value for clearOnClose
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setClearOnClose(Optional clearOnClose) {
this.clearOnClose = clearOnClose.orElse(null);
return this;
}
/**
* Initializes the optional value {@link ModalViewResponseIF#getNotifyOnClose() notifyOnClose} to notifyOnClose.
* @param notifyOnClose The value for notifyOnClose, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
public final Builder setNotifyOnClose(@Nullable Boolean notifyOnClose) {
this.notifyOnClose = notifyOnClose;
return this;
}
/**
* Initializes the optional value {@link ModalViewResponseIF#getNotifyOnClose() notifyOnClose} to notifyOnClose.
* @param notifyOnClose The value for notifyOnClose
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setNotifyOnClose(Optional notifyOnClose) {
this.notifyOnClose = notifyOnClose.orElse(null);
return this;
}
/**
* Initializes the value for the {@link ModalViewResponseIF#getAppId() appId} attribute.
* @param appId The value for appId
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setAppId(String appId) {
this.appId = Objects.requireNonNull(appId, "appId");
initBits &= ~INIT_BIT_APP_ID;
return this;
}
/**
* Initializes the value for the {@link ModalViewResponseIF#getBotId() botId} attribute.
* @param botId The value for botId
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setBotId(String botId) {
this.botId = Objects.requireNonNull(botId, "botId");
initBits &= ~INIT_BIT_BOT_ID;
return this;
}
/**
* Initializes the value for the {@link ModalViewResponseIF#getCurrentViewId() currentViewId} attribute.
* @param currentViewId The value for currentViewId
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setCurrentViewId(String currentViewId) {
this.currentViewId = Objects.requireNonNull(currentViewId, "currentViewId");
initBits &= ~INIT_BIT_CURRENT_VIEW_ID;
return this;
}
/**
* Initializes the value for the {@link ModalViewResponseIF#getRootViewId() rootViewId} attribute.
* @param rootViewId The value for rootViewId
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setRootViewId(String rootViewId) {
this.rootViewId = Objects.requireNonNull(rootViewId, "rootViewId");
initBits &= ~INIT_BIT_ROOT_VIEW_ID;
return this;
}
/**
* Initializes the value for the {@link ModalViewResponseIF#getTeamId() teamId} attribute.
* @param teamId The value for teamId
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setTeamId(String teamId) {
this.teamId = Objects.requireNonNull(teamId, "teamId");
initBits &= ~INIT_BIT_TEAM_ID;
return this;
}
/**
* Initializes the value for the {@link ModalViewResponseIF#getStateValues() stateValues} attribute.
* @param stateValues The value for stateValues
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setStateValues(StateBlock stateValues) {
this.stateValues = Objects.requireNonNull(stateValues, "stateValues");
initBits &= ~INIT_BIT_STATE_VALUES;
return this;
}
/**
* Initializes the value for the {@link ModalViewResponseIF#getHash() hash} attribute.
* @param hash The value for hash
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setHash(String hash) {
this.hash = Objects.requireNonNull(hash, "hash");
initBits &= ~INIT_BIT_HASH;
return this;
}
/**
* Initializes the optional value {@link ModalViewResponseIF#getPreviousViewId() previousViewId} to previousViewId.
* @param previousViewId The value for previousViewId, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
public final Builder setPreviousViewId(@Nullable String previousViewId) {
this.previousViewId = previousViewId;
return this;
}
/**
* Initializes the optional value {@link ModalViewResponseIF#getPreviousViewId() previousViewId} to previousViewId.
* @param previousViewId The value for previousViewId
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setPreviousViewId(Optional previousViewId) {
this.previousViewId = previousViewId.orElse(null);
return this;
}
/**
* Builds a new {@link ModalViewResponse ModalViewResponse}.
* @return An immutable instance of ModalViewResponse
* @throws com.hubspot.immutables.validation.InvalidImmutableStateException if any required attributes are missing
*/
public ModalViewResponse build() {
checkRequiredAttributes();
return new ModalViewResponse(
createUnmodifiableList(true, blocks),
privateMetadata,
callbackId,
externalId,
title,
closeButtonText,
submitButtonText,
clearOnClose,
notifyOnClose,
appId,
botId,
currentViewId,
rootViewId,
teamId,
stateValues,
hash,
previousViewId);
}
private boolean titleIsSet() {
return (initBits & INIT_BIT_TITLE) == 0;
}
private boolean appIdIsSet() {
return (initBits & INIT_BIT_APP_ID) == 0;
}
private boolean botIdIsSet() {
return (initBits & INIT_BIT_BOT_ID) == 0;
}
private boolean currentViewIdIsSet() {
return (initBits & INIT_BIT_CURRENT_VIEW_ID) == 0;
}
private boolean rootViewIdIsSet() {
return (initBits & INIT_BIT_ROOT_VIEW_ID) == 0;
}
private boolean teamIdIsSet() {
return (initBits & INIT_BIT_TEAM_ID) == 0;
}
private boolean stateValuesIsSet() {
return (initBits & INIT_BIT_STATE_VALUES) == 0;
}
private boolean hashIsSet() {
return (initBits & INIT_BIT_HASH) == 0;
}
private void checkRequiredAttributes() {
if (initBits != 0) {
throw new InvalidImmutableStateException(formatRequiredAttributesMessage());
}
}
private String formatRequiredAttributesMessage() {
List attributes = new ArrayList<>();
if (!titleIsSet()) attributes.add("title");
if (!appIdIsSet()) attributes.add("appId");
if (!botIdIsSet()) attributes.add("botId");
if (!currentViewIdIsSet()) attributes.add("currentViewId");
if (!rootViewIdIsSet()) attributes.add("rootViewId");
if (!teamIdIsSet()) attributes.add("teamId");
if (!stateValuesIsSet()) attributes.add("stateValues");
if (!hashIsSet()) attributes.add("hash");
return "Cannot build ModalViewResponse, some of required attributes are not set " + attributes;
}
}
private static List createSafeList(Iterable extends T> iterable, boolean checkNulls, boolean skipNulls) {
ArrayList list;
if (iterable instanceof Collection>) {
int size = ((Collection>) iterable).size();
if (size == 0) return Collections.emptyList();
list = new ArrayList<>(size);
} else {
list = new ArrayList<>();
}
for (T element : iterable) {
if (skipNulls && element == null) continue;
if (checkNulls) Objects.requireNonNull(element, "element");
list.add(element);
}
return list;
}
private static List createUnmodifiableList(boolean clone, List list) {
switch(list.size()) {
case 0: return Collections.emptyList();
case 1: return Collections.singletonList(list.get(0));
default:
if (clone) {
return Collections.unmodifiableList(new ArrayList<>(list));
} else {
if (list instanceof ArrayList>) {
((ArrayList>) list).trimToSize();
}
return Collections.unmodifiableList(list);
}
}
}
}