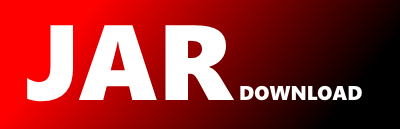
com.hubspot.slack.client.models.teams.SlackTeam Maven / Gradle / Ivy
package com.hubspot.slack.client.models.teams;
import com.fasterxml.jackson.annotation.JsonAutoDetect;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.hubspot.immutables.validation.InvalidImmutableStateException;
import edu.umd.cs.findbugs.annotations.SuppressFBWarnings;
import java.util.ArrayList;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
import javax.annotation.ParametersAreNonnullByDefault;
import javax.annotation.concurrent.Immutable;
import javax.annotation.concurrent.NotThreadSafe;
import org.immutables.value.Generated;
/**
* Immutable implementation of {@link SlackTeamIF}.
*
* Use the builder to create immutable instances:
* {@code SlackTeam.builder()}.
*/
@Generated(from = "SlackTeamIF", generator = "Immutables")
@SuppressWarnings({"all"})
@SuppressFBWarnings
@ParametersAreNonnullByDefault
@javax.annotation.processing.Generated("org.immutables.processor.ProxyProcessor")
@Immutable
public final class SlackTeam implements SlackTeamIF {
private final String id;
private final String domain;
private final @Nullable String name;
private final @Nullable String enterpriseId;
private final @Nullable String enterpriseName;
private final @Nullable SlackTeamIcon icon;
private SlackTeam(
String id,
String domain,
@Nullable String name,
@Nullable String enterpriseId,
@Nullable String enterpriseName,
@Nullable SlackTeamIcon icon) {
this.id = id;
this.domain = domain;
this.name = name;
this.enterpriseId = enterpriseId;
this.enterpriseName = enterpriseName;
this.icon = icon;
}
/**
* @return The value of the {@code id} attribute
*/
@JsonProperty
@Override
public String getId() {
return id;
}
/**
* @return The value of the {@code domain} attribute
*/
@JsonProperty
@Override
public String getDomain() {
return domain;
}
/**
* @return The value of the {@code name} attribute
*/
@JsonProperty
@Override
public Optional getName() {
return Optional.ofNullable(name);
}
/**
* @return The value of the {@code enterpriseId} attribute
*/
@JsonProperty
@Override
public Optional getEnterpriseId() {
return Optional.ofNullable(enterpriseId);
}
/**
* @return The value of the {@code enterpriseName} attribute
*/
@JsonProperty
@Override
public Optional getEnterpriseName() {
return Optional.ofNullable(enterpriseName);
}
/**
* @return The value of the {@code icon} attribute
*/
@JsonProperty
@Override
public Optional getIcon() {
return Optional.ofNullable(icon);
}
/**
* Copy the current immutable object by setting a value for the {@link SlackTeamIF#getId() id} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for id
* @return A modified copy of the {@code this} object
*/
public final SlackTeam withId(String value) {
String newValue = Objects.requireNonNull(value, "id");
if (this.id.equals(newValue)) return this;
return new SlackTeam(newValue, this.domain, this.name, this.enterpriseId, this.enterpriseName, this.icon);
}
/**
* Copy the current immutable object by setting a value for the {@link SlackTeamIF#getDomain() domain} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for domain
* @return A modified copy of the {@code this} object
*/
public final SlackTeam withDomain(String value) {
String newValue = Objects.requireNonNull(value, "domain");
if (this.domain.equals(newValue)) return this;
return new SlackTeam(this.id, newValue, this.name, this.enterpriseId, this.enterpriseName, this.icon);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link SlackTeamIF#getName() name} attribute.
* @param value The value for name, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final SlackTeam withName(@Nullable String value) {
@Nullable String newValue = value;
if (Objects.equals(this.name, newValue)) return this;
return new SlackTeam(this.id, this.domain, newValue, this.enterpriseId, this.enterpriseName, this.icon);
}
/**
* Copy the current immutable object by setting an optional value for the {@link SlackTeamIF#getName() name} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for name
* @return A modified copy of {@code this} object
*/
public final SlackTeam withName(Optional optional) {
@Nullable String value = optional.orElse(null);
if (Objects.equals(this.name, value)) return this;
return new SlackTeam(this.id, this.domain, value, this.enterpriseId, this.enterpriseName, this.icon);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link SlackTeamIF#getEnterpriseId() enterpriseId} attribute.
* @param value The value for enterpriseId, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final SlackTeam withEnterpriseId(@Nullable String value) {
@Nullable String newValue = value;
if (Objects.equals(this.enterpriseId, newValue)) return this;
return new SlackTeam(this.id, this.domain, this.name, newValue, this.enterpriseName, this.icon);
}
/**
* Copy the current immutable object by setting an optional value for the {@link SlackTeamIF#getEnterpriseId() enterpriseId} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for enterpriseId
* @return A modified copy of {@code this} object
*/
public final SlackTeam withEnterpriseId(Optional optional) {
@Nullable String value = optional.orElse(null);
if (Objects.equals(this.enterpriseId, value)) return this;
return new SlackTeam(this.id, this.domain, this.name, value, this.enterpriseName, this.icon);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link SlackTeamIF#getEnterpriseName() enterpriseName} attribute.
* @param value The value for enterpriseName, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final SlackTeam withEnterpriseName(@Nullable String value) {
@Nullable String newValue = value;
if (Objects.equals(this.enterpriseName, newValue)) return this;
return new SlackTeam(this.id, this.domain, this.name, this.enterpriseId, newValue, this.icon);
}
/**
* Copy the current immutable object by setting an optional value for the {@link SlackTeamIF#getEnterpriseName() enterpriseName} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for enterpriseName
* @return A modified copy of {@code this} object
*/
public final SlackTeam withEnterpriseName(Optional optional) {
@Nullable String value = optional.orElse(null);
if (Objects.equals(this.enterpriseName, value)) return this;
return new SlackTeam(this.id, this.domain, this.name, this.enterpriseId, value, this.icon);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link SlackTeamIF#getIcon() icon} attribute.
* @param value The value for icon, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final SlackTeam withIcon(@Nullable SlackTeamIcon value) {
@Nullable SlackTeamIcon newValue = value;
if (this.icon == newValue) return this;
return new SlackTeam(this.id, this.domain, this.name, this.enterpriseId, this.enterpriseName, newValue);
}
/**
* Copy the current immutable object by setting an optional value for the {@link SlackTeamIF#getIcon() icon} attribute.
* A shallow reference equality check is used on unboxed optional value to prevent copying of the same value by returning {@code this}.
* @param optional A value for icon
* @return A modified copy of {@code this} object
*/
@SuppressWarnings("unchecked") // safe covariant cast
public final SlackTeam withIcon(Optional extends SlackTeamIcon> optional) {
@Nullable SlackTeamIcon value = optional.orElse(null);
if (this.icon == value) return this;
return new SlackTeam(this.id, this.domain, this.name, this.enterpriseId, this.enterpriseName, value);
}
/**
* This instance is equal to all instances of {@code SlackTeam} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(@Nullable Object another) {
if (this == another) return true;
return another instanceof SlackTeam
&& equalTo(0, (SlackTeam) another);
}
private boolean equalTo(int synthetic, SlackTeam another) {
return id.equals(another.id)
&& domain.equals(another.domain)
&& Objects.equals(name, another.name)
&& Objects.equals(enterpriseId, another.enterpriseId)
&& Objects.equals(enterpriseName, another.enterpriseName)
&& Objects.equals(icon, another.icon);
}
/**
* Computes a hash code from attributes: {@code id}, {@code domain}, {@code name}, {@code enterpriseId}, {@code enterpriseName}, {@code icon}.
* @return hashCode value
*/
@Override
public int hashCode() {
int h = 5381;
h += (h << 5) + id.hashCode();
h += (h << 5) + domain.hashCode();
h += (h << 5) + Objects.hashCode(name);
h += (h << 5) + Objects.hashCode(enterpriseId);
h += (h << 5) + Objects.hashCode(enterpriseName);
h += (h << 5) + Objects.hashCode(icon);
return h;
}
/**
* Prints the immutable value {@code SlackTeam} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
StringBuilder builder = new StringBuilder("SlackTeam{");
builder.append("id=").append(id);
builder.append(", ");
builder.append("domain=").append(domain);
if (name != null) {
builder.append(", ");
builder.append("name=").append(name);
}
if (enterpriseId != null) {
builder.append(", ");
builder.append("enterpriseId=").append(enterpriseId);
}
if (enterpriseName != null) {
builder.append(", ");
builder.append("enterpriseName=").append(enterpriseName);
}
if (icon != null) {
builder.append(", ");
builder.append("icon=").append(icon);
}
return builder.append("}").toString();
}
/**
* Utility type used to correctly read immutable object from JSON representation.
* @deprecated Do not use this type directly, it exists only for the Jackson-binding infrastructure
*/
@Generated(from = "SlackTeamIF", generator = "Immutables")
@Deprecated
@JsonAutoDetect(fieldVisibility = JsonAutoDetect.Visibility.NONE)
static final class Json implements SlackTeamIF {
@Nullable String id;
@Nullable String domain;
@Nullable Optional name = Optional.empty();
@Nullable Optional enterpriseId = Optional.empty();
@Nullable Optional enterpriseName = Optional.empty();
@Nullable Optional icon = Optional.empty();
@JsonProperty
public void setId(String id) {
this.id = id;
}
@JsonProperty
public void setDomain(String domain) {
this.domain = domain;
}
@JsonProperty
public void setName(Optional name) {
this.name = name;
}
@JsonProperty
public void setEnterpriseId(Optional enterpriseId) {
this.enterpriseId = enterpriseId;
}
@JsonProperty
public void setEnterpriseName(Optional enterpriseName) {
this.enterpriseName = enterpriseName;
}
@JsonProperty
public void setIcon(Optional icon) {
this.icon = icon;
}
@Override
public String getId() { throw new UnsupportedOperationException(); }
@Override
public String getDomain() { throw new UnsupportedOperationException(); }
@Override
public Optional getName() { throw new UnsupportedOperationException(); }
@Override
public Optional getEnterpriseId() { throw new UnsupportedOperationException(); }
@Override
public Optional getEnterpriseName() { throw new UnsupportedOperationException(); }
@Override
public Optional getIcon() { throw new UnsupportedOperationException(); }
}
/**
* @param json A JSON-bindable data structure
* @return An immutable value type
* @deprecated Do not use this method directly, it exists only for the Jackson-binding infrastructure
*/
@Deprecated
@JsonCreator(mode = JsonCreator.Mode.DELEGATING)
static SlackTeam fromJson(Json json) {
SlackTeam.Builder builder = SlackTeam.builder();
if (json.id != null) {
builder.setId(json.id);
}
if (json.domain != null) {
builder.setDomain(json.domain);
}
if (json.name != null) {
builder.setName(json.name);
}
if (json.enterpriseId != null) {
builder.setEnterpriseId(json.enterpriseId);
}
if (json.enterpriseName != null) {
builder.setEnterpriseName(json.enterpriseName);
}
if (json.icon != null) {
builder.setIcon(json.icon);
}
return builder.build();
}
/**
* Creates an immutable copy of a {@link SlackTeamIF} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable SlackTeam instance
*/
public static SlackTeam copyOf(SlackTeamIF instance) {
if (instance instanceof SlackTeam) {
return (SlackTeam) instance;
}
return SlackTeam.builder()
.from(instance)
.build();
}
/**
* Creates a builder for {@link SlackTeam SlackTeam}.
*
* SlackTeam.builder()
* .setId(String) // required {@link SlackTeamIF#getId() id}
* .setDomain(String) // required {@link SlackTeamIF#getDomain() domain}
* .setName(String) // optional {@link SlackTeamIF#getName() name}
* .setEnterpriseId(String) // optional {@link SlackTeamIF#getEnterpriseId() enterpriseId}
* .setEnterpriseName(String) // optional {@link SlackTeamIF#getEnterpriseName() enterpriseName}
* .setIcon(SlackTeamIcon) // optional {@link SlackTeamIF#getIcon() icon}
* .build();
*
* @return A new SlackTeam builder
*/
public static SlackTeam.Builder builder() {
return new SlackTeam.Builder();
}
/**
* Builds instances of type {@link SlackTeam SlackTeam}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
@Generated(from = "SlackTeamIF", generator = "Immutables")
@NotThreadSafe
public static final class Builder {
private static final long INIT_BIT_ID = 0x1L;
private static final long INIT_BIT_DOMAIN = 0x2L;
private long initBits = 0x3L;
private @Nullable String id;
private @Nullable String domain;
private @Nullable String name;
private @Nullable String enterpriseId;
private @Nullable String enterpriseName;
private @Nullable SlackTeamIcon icon;
private Builder() {
}
/**
* Fill a builder with attribute values from the provided {@code SlackTeamIF} instance.
* Regular attribute values will be replaced with those from the given instance.
* Absent optional values will not replace present values.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(SlackTeamIF instance) {
Objects.requireNonNull(instance, "instance");
this.setId(instance.getId());
this.setDomain(instance.getDomain());
Optional nameOptional = instance.getName();
if (nameOptional.isPresent()) {
setName(nameOptional);
}
Optional enterpriseIdOptional = instance.getEnterpriseId();
if (enterpriseIdOptional.isPresent()) {
setEnterpriseId(enterpriseIdOptional);
}
Optional enterpriseNameOptional = instance.getEnterpriseName();
if (enterpriseNameOptional.isPresent()) {
setEnterpriseName(enterpriseNameOptional);
}
Optional iconOptional = instance.getIcon();
if (iconOptional.isPresent()) {
setIcon(iconOptional);
}
return this;
}
/**
* Initializes the value for the {@link SlackTeamIF#getId() id} attribute.
* @param id The value for id
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setId(String id) {
this.id = Objects.requireNonNull(id, "id");
initBits &= ~INIT_BIT_ID;
return this;
}
/**
* Initializes the value for the {@link SlackTeamIF#getDomain() domain} attribute.
* @param domain The value for domain
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setDomain(String domain) {
this.domain = Objects.requireNonNull(domain, "domain");
initBits &= ~INIT_BIT_DOMAIN;
return this;
}
/**
* Initializes the optional value {@link SlackTeamIF#getName() name} to name.
* @param name The value for name, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
public final Builder setName(@Nullable String name) {
this.name = name;
return this;
}
/**
* Initializes the optional value {@link SlackTeamIF#getName() name} to name.
* @param name The value for name
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setName(Optional name) {
this.name = name.orElse(null);
return this;
}
/**
* Initializes the optional value {@link SlackTeamIF#getEnterpriseId() enterpriseId} to enterpriseId.
* @param enterpriseId The value for enterpriseId, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
public final Builder setEnterpriseId(@Nullable String enterpriseId) {
this.enterpriseId = enterpriseId;
return this;
}
/**
* Initializes the optional value {@link SlackTeamIF#getEnterpriseId() enterpriseId} to enterpriseId.
* @param enterpriseId The value for enterpriseId
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setEnterpriseId(Optional enterpriseId) {
this.enterpriseId = enterpriseId.orElse(null);
return this;
}
/**
* Initializes the optional value {@link SlackTeamIF#getEnterpriseName() enterpriseName} to enterpriseName.
* @param enterpriseName The value for enterpriseName, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
public final Builder setEnterpriseName(@Nullable String enterpriseName) {
this.enterpriseName = enterpriseName;
return this;
}
/**
* Initializes the optional value {@link SlackTeamIF#getEnterpriseName() enterpriseName} to enterpriseName.
* @param enterpriseName The value for enterpriseName
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setEnterpriseName(Optional enterpriseName) {
this.enterpriseName = enterpriseName.orElse(null);
return this;
}
/**
* Initializes the optional value {@link SlackTeamIF#getIcon() icon} to icon.
* @param icon The value for icon, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
public final Builder setIcon(@Nullable SlackTeamIcon icon) {
this.icon = icon;
return this;
}
/**
* Initializes the optional value {@link SlackTeamIF#getIcon() icon} to icon.
* @param icon The value for icon
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setIcon(Optional extends SlackTeamIcon> icon) {
this.icon = icon.orElse(null);
return this;
}
/**
* Builds a new {@link SlackTeam SlackTeam}.
* @return An immutable instance of SlackTeam
* @throws com.hubspot.immutables.validation.InvalidImmutableStateException if any required attributes are missing
*/
public SlackTeam build() {
checkRequiredAttributes();
return new SlackTeam(id, domain, name, enterpriseId, enterpriseName, icon);
}
private boolean idIsSet() {
return (initBits & INIT_BIT_ID) == 0;
}
private boolean domainIsSet() {
return (initBits & INIT_BIT_DOMAIN) == 0;
}
private void checkRequiredAttributes() {
if (initBits != 0) {
throw new InvalidImmutableStateException(formatRequiredAttributesMessage());
}
}
private String formatRequiredAttributesMessage() {
List attributes = new ArrayList<>();
if (!idIsSet()) attributes.add("id");
if (!domainIsSet()) attributes.add("domain");
return "Cannot build SlackTeam, some of required attributes are not set " + attributes;
}
}
}