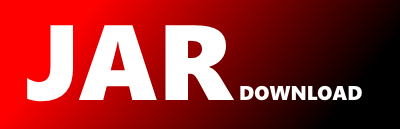
com.hubspot.slack.client.models.usergroups.SlackUsergroup Maven / Gradle / Ivy
package com.hubspot.slack.client.models.usergroups;
import com.fasterxml.jackson.annotation.JsonAutoDetect;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.hubspot.immutables.validation.InvalidImmutableStateException;
import edu.umd.cs.findbugs.annotations.SuppressFBWarnings;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
import javax.annotation.ParametersAreNonnullByDefault;
import javax.annotation.concurrent.Immutable;
import javax.annotation.concurrent.NotThreadSafe;
import org.immutables.value.Generated;
/**
* Immutable implementation of {@link SlackUsergroupIF}.
*
* Use the builder to create immutable instances:
* {@code SlackUsergroup.builder()}.
*/
@Generated(from = "SlackUsergroupIF", generator = "Immutables")
@SuppressWarnings({"all"})
@SuppressFBWarnings
@ParametersAreNonnullByDefault
@javax.annotation.processing.Generated("org.immutables.processor.ProxyProcessor")
@Immutable
public final class SlackUsergroup implements SlackUsergroupIF {
private final String id;
private final String teamId;
private final boolean usergroup;
private final String name;
private final @Nullable String description;
private final String handle;
private final boolean external;
private final int dateCreatedEpochSeconds;
private final int dateUpdatedEpochSeconds;
private final int dateDeletedEpochSeconds;
private final @Nullable String autoType;
private final @Nullable String createdBy;
private final @Nullable String updatedBy;
private final @Nullable String deletedBy;
private transient final boolean deleted;
private final UsergroupPreferences preferences;
private final List userIdsInGroup;
private final @Nullable Integer userCount;
private SlackUsergroup(
String id,
String teamId,
boolean usergroup,
String name,
@Nullable String description,
String handle,
boolean external,
int dateCreatedEpochSeconds,
int dateUpdatedEpochSeconds,
int dateDeletedEpochSeconds,
@Nullable String autoType,
@Nullable String createdBy,
@Nullable String updatedBy,
@Nullable String deletedBy,
UsergroupPreferences preferences,
List userIdsInGroup,
@Nullable Integer userCount) {
this.id = id;
this.teamId = teamId;
this.usergroup = usergroup;
this.name = name;
this.description = description;
this.handle = handle;
this.external = external;
this.dateCreatedEpochSeconds = dateCreatedEpochSeconds;
this.dateUpdatedEpochSeconds = dateUpdatedEpochSeconds;
this.dateDeletedEpochSeconds = dateDeletedEpochSeconds;
this.autoType = autoType;
this.createdBy = createdBy;
this.updatedBy = updatedBy;
this.deletedBy = deletedBy;
this.preferences = preferences;
this.userIdsInGroup = userIdsInGroup;
this.userCount = userCount;
this.deleted = SlackUsergroupIF.super.isDeleted();
}
/**
* @return The value of the {@code id} attribute
*/
@JsonProperty
@Override
public String getId() {
return id;
}
/**
* @return The value of the {@code teamId} attribute
*/
@JsonProperty
@Override
public String getTeamId() {
return teamId;
}
/**
* @return The value of the {@code usergroup} attribute
*/
@JsonProperty("is_usergroup")
@Override
public boolean isUsergroup() {
return usergroup;
}
/**
* @return The value of the {@code name} attribute
*/
@JsonProperty
@Override
public String getName() {
return name;
}
/**
* @return The value of the {@code description} attribute
*/
@JsonProperty
@Override
public Optional getDescription() {
return Optional.ofNullable(description);
}
/**
* @return The value of the {@code handle} attribute
*/
@JsonProperty
@Override
public String getHandle() {
return handle;
}
/**
* @return The value of the {@code external} attribute
*/
@JsonProperty("is_external")
@Override
public boolean isExternal() {
return external;
}
/**
* @return The value of the {@code dateCreatedEpochSeconds} attribute
*/
@JsonProperty("date_create")
@Override
public int getDateCreatedEpochSeconds() {
return dateCreatedEpochSeconds;
}
/**
* @return The value of the {@code dateUpdatedEpochSeconds} attribute
*/
@JsonProperty("date_update")
@Override
public int getDateUpdatedEpochSeconds() {
return dateUpdatedEpochSeconds;
}
/**
* @return The value of the {@code dateDeletedEpochSeconds} attribute
*/
@JsonProperty("date_delete")
@Override
public int getDateDeletedEpochSeconds() {
return dateDeletedEpochSeconds;
}
/**
* @return The value of the {@code autoType} attribute
*/
@JsonProperty
@Override
public Optional getAutoType() {
return Optional.ofNullable(autoType);
}
/**
* @return The value of the {@code createdBy} attribute
*/
@JsonProperty
@Override
public Optional getCreatedBy() {
return Optional.ofNullable(createdBy);
}
/**
* @return The value of the {@code updatedBy} attribute
*/
@JsonProperty
@Override
public Optional getUpdatedBy() {
return Optional.ofNullable(updatedBy);
}
/**
* @return The value of the {@code deletedBy} attribute
*/
@JsonProperty
@Override
public Optional getDeletedBy() {
return Optional.ofNullable(deletedBy);
}
/**
* @return The computed-at-construction value of the {@code deleted} attribute
*/
@JsonProperty
@Override
public boolean isDeleted() {
return deleted;
}
/**
* @return The value of the {@code preferences} attribute
*/
@JsonProperty("prefs")
@Override
public UsergroupPreferences getPreferences() {
return preferences;
}
/**
* @return The value of the {@code userIdsInGroup} attribute
*/
@JsonProperty("users")
@Override
public List getUserIdsInGroup() {
return userIdsInGroup;
}
/**
* @return The value of the {@code userCount} attribute
*/
@JsonProperty
@Override
public Optional getUserCount() {
return Optional.ofNullable(userCount);
}
/**
* Copy the current immutable object by setting a value for the {@link SlackUsergroupIF#getId() id} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for id
* @return A modified copy of the {@code this} object
*/
public final SlackUsergroup withId(String value) {
String newValue = Objects.requireNonNull(value, "id");
if (this.id.equals(newValue)) return this;
return new SlackUsergroup(
newValue,
this.teamId,
this.usergroup,
this.name,
this.description,
this.handle,
this.external,
this.dateCreatedEpochSeconds,
this.dateUpdatedEpochSeconds,
this.dateDeletedEpochSeconds,
this.autoType,
this.createdBy,
this.updatedBy,
this.deletedBy,
this.preferences,
this.userIdsInGroup,
this.userCount);
}
/**
* Copy the current immutable object by setting a value for the {@link SlackUsergroupIF#getTeamId() teamId} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for teamId
* @return A modified copy of the {@code this} object
*/
public final SlackUsergroup withTeamId(String value) {
String newValue = Objects.requireNonNull(value, "teamId");
if (this.teamId.equals(newValue)) return this;
return new SlackUsergroup(
this.id,
newValue,
this.usergroup,
this.name,
this.description,
this.handle,
this.external,
this.dateCreatedEpochSeconds,
this.dateUpdatedEpochSeconds,
this.dateDeletedEpochSeconds,
this.autoType,
this.createdBy,
this.updatedBy,
this.deletedBy,
this.preferences,
this.userIdsInGroup,
this.userCount);
}
/**
* Copy the current immutable object by setting a value for the {@link SlackUsergroupIF#isUsergroup() usergroup} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for usergroup
* @return A modified copy of the {@code this} object
*/
public final SlackUsergroup withUsergroup(boolean value) {
if (this.usergroup == value) return this;
return new SlackUsergroup(
this.id,
this.teamId,
value,
this.name,
this.description,
this.handle,
this.external,
this.dateCreatedEpochSeconds,
this.dateUpdatedEpochSeconds,
this.dateDeletedEpochSeconds,
this.autoType,
this.createdBy,
this.updatedBy,
this.deletedBy,
this.preferences,
this.userIdsInGroup,
this.userCount);
}
/**
* Copy the current immutable object by setting a value for the {@link SlackUsergroupIF#getName() name} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for name
* @return A modified copy of the {@code this} object
*/
public final SlackUsergroup withName(String value) {
String newValue = Objects.requireNonNull(value, "name");
if (this.name.equals(newValue)) return this;
return new SlackUsergroup(
this.id,
this.teamId,
this.usergroup,
newValue,
this.description,
this.handle,
this.external,
this.dateCreatedEpochSeconds,
this.dateUpdatedEpochSeconds,
this.dateDeletedEpochSeconds,
this.autoType,
this.createdBy,
this.updatedBy,
this.deletedBy,
this.preferences,
this.userIdsInGroup,
this.userCount);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link SlackUsergroupIF#getDescription() description} attribute.
* @param value The value for description, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final SlackUsergroup withDescription(@Nullable String value) {
@Nullable String newValue = value;
if (Objects.equals(this.description, newValue)) return this;
return new SlackUsergroup(
this.id,
this.teamId,
this.usergroup,
this.name,
newValue,
this.handle,
this.external,
this.dateCreatedEpochSeconds,
this.dateUpdatedEpochSeconds,
this.dateDeletedEpochSeconds,
this.autoType,
this.createdBy,
this.updatedBy,
this.deletedBy,
this.preferences,
this.userIdsInGroup,
this.userCount);
}
/**
* Copy the current immutable object by setting an optional value for the {@link SlackUsergroupIF#getDescription() description} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for description
* @return A modified copy of {@code this} object
*/
public final SlackUsergroup withDescription(Optional optional) {
@Nullable String value = optional.orElse(null);
if (Objects.equals(this.description, value)) return this;
return new SlackUsergroup(
this.id,
this.teamId,
this.usergroup,
this.name,
value,
this.handle,
this.external,
this.dateCreatedEpochSeconds,
this.dateUpdatedEpochSeconds,
this.dateDeletedEpochSeconds,
this.autoType,
this.createdBy,
this.updatedBy,
this.deletedBy,
this.preferences,
this.userIdsInGroup,
this.userCount);
}
/**
* Copy the current immutable object by setting a value for the {@link SlackUsergroupIF#getHandle() handle} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for handle
* @return A modified copy of the {@code this} object
*/
public final SlackUsergroup withHandle(String value) {
String newValue = Objects.requireNonNull(value, "handle");
if (this.handle.equals(newValue)) return this;
return new SlackUsergroup(
this.id,
this.teamId,
this.usergroup,
this.name,
this.description,
newValue,
this.external,
this.dateCreatedEpochSeconds,
this.dateUpdatedEpochSeconds,
this.dateDeletedEpochSeconds,
this.autoType,
this.createdBy,
this.updatedBy,
this.deletedBy,
this.preferences,
this.userIdsInGroup,
this.userCount);
}
/**
* Copy the current immutable object by setting a value for the {@link SlackUsergroupIF#isExternal() external} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for external
* @return A modified copy of the {@code this} object
*/
public final SlackUsergroup withExternal(boolean value) {
if (this.external == value) return this;
return new SlackUsergroup(
this.id,
this.teamId,
this.usergroup,
this.name,
this.description,
this.handle,
value,
this.dateCreatedEpochSeconds,
this.dateUpdatedEpochSeconds,
this.dateDeletedEpochSeconds,
this.autoType,
this.createdBy,
this.updatedBy,
this.deletedBy,
this.preferences,
this.userIdsInGroup,
this.userCount);
}
/**
* Copy the current immutable object by setting a value for the {@link SlackUsergroupIF#getDateCreatedEpochSeconds() dateCreatedEpochSeconds} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for dateCreatedEpochSeconds
* @return A modified copy of the {@code this} object
*/
public final SlackUsergroup withDateCreatedEpochSeconds(int value) {
if (this.dateCreatedEpochSeconds == value) return this;
return new SlackUsergroup(
this.id,
this.teamId,
this.usergroup,
this.name,
this.description,
this.handle,
this.external,
value,
this.dateUpdatedEpochSeconds,
this.dateDeletedEpochSeconds,
this.autoType,
this.createdBy,
this.updatedBy,
this.deletedBy,
this.preferences,
this.userIdsInGroup,
this.userCount);
}
/**
* Copy the current immutable object by setting a value for the {@link SlackUsergroupIF#getDateUpdatedEpochSeconds() dateUpdatedEpochSeconds} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for dateUpdatedEpochSeconds
* @return A modified copy of the {@code this} object
*/
public final SlackUsergroup withDateUpdatedEpochSeconds(int value) {
if (this.dateUpdatedEpochSeconds == value) return this;
return new SlackUsergroup(
this.id,
this.teamId,
this.usergroup,
this.name,
this.description,
this.handle,
this.external,
this.dateCreatedEpochSeconds,
value,
this.dateDeletedEpochSeconds,
this.autoType,
this.createdBy,
this.updatedBy,
this.deletedBy,
this.preferences,
this.userIdsInGroup,
this.userCount);
}
/**
* Copy the current immutable object by setting a value for the {@link SlackUsergroupIF#getDateDeletedEpochSeconds() dateDeletedEpochSeconds} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for dateDeletedEpochSeconds
* @return A modified copy of the {@code this} object
*/
public final SlackUsergroup withDateDeletedEpochSeconds(int value) {
if (this.dateDeletedEpochSeconds == value) return this;
return new SlackUsergroup(
this.id,
this.teamId,
this.usergroup,
this.name,
this.description,
this.handle,
this.external,
this.dateCreatedEpochSeconds,
this.dateUpdatedEpochSeconds,
value,
this.autoType,
this.createdBy,
this.updatedBy,
this.deletedBy,
this.preferences,
this.userIdsInGroup,
this.userCount);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link SlackUsergroupIF#getAutoType() autoType} attribute.
* @param value The value for autoType, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final SlackUsergroup withAutoType(@Nullable String value) {
@Nullable String newValue = value;
if (Objects.equals(this.autoType, newValue)) return this;
return new SlackUsergroup(
this.id,
this.teamId,
this.usergroup,
this.name,
this.description,
this.handle,
this.external,
this.dateCreatedEpochSeconds,
this.dateUpdatedEpochSeconds,
this.dateDeletedEpochSeconds,
newValue,
this.createdBy,
this.updatedBy,
this.deletedBy,
this.preferences,
this.userIdsInGroup,
this.userCount);
}
/**
* Copy the current immutable object by setting an optional value for the {@link SlackUsergroupIF#getAutoType() autoType} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for autoType
* @return A modified copy of {@code this} object
*/
public final SlackUsergroup withAutoType(Optional optional) {
@Nullable String value = optional.orElse(null);
if (Objects.equals(this.autoType, value)) return this;
return new SlackUsergroup(
this.id,
this.teamId,
this.usergroup,
this.name,
this.description,
this.handle,
this.external,
this.dateCreatedEpochSeconds,
this.dateUpdatedEpochSeconds,
this.dateDeletedEpochSeconds,
value,
this.createdBy,
this.updatedBy,
this.deletedBy,
this.preferences,
this.userIdsInGroup,
this.userCount);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link SlackUsergroupIF#getCreatedBy() createdBy} attribute.
* @param value The value for createdBy, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final SlackUsergroup withCreatedBy(@Nullable String value) {
@Nullable String newValue = value;
if (Objects.equals(this.createdBy, newValue)) return this;
return new SlackUsergroup(
this.id,
this.teamId,
this.usergroup,
this.name,
this.description,
this.handle,
this.external,
this.dateCreatedEpochSeconds,
this.dateUpdatedEpochSeconds,
this.dateDeletedEpochSeconds,
this.autoType,
newValue,
this.updatedBy,
this.deletedBy,
this.preferences,
this.userIdsInGroup,
this.userCount);
}
/**
* Copy the current immutable object by setting an optional value for the {@link SlackUsergroupIF#getCreatedBy() createdBy} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for createdBy
* @return A modified copy of {@code this} object
*/
public final SlackUsergroup withCreatedBy(Optional optional) {
@Nullable String value = optional.orElse(null);
if (Objects.equals(this.createdBy, value)) return this;
return new SlackUsergroup(
this.id,
this.teamId,
this.usergroup,
this.name,
this.description,
this.handle,
this.external,
this.dateCreatedEpochSeconds,
this.dateUpdatedEpochSeconds,
this.dateDeletedEpochSeconds,
this.autoType,
value,
this.updatedBy,
this.deletedBy,
this.preferences,
this.userIdsInGroup,
this.userCount);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link SlackUsergroupIF#getUpdatedBy() updatedBy} attribute.
* @param value The value for updatedBy, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final SlackUsergroup withUpdatedBy(@Nullable String value) {
@Nullable String newValue = value;
if (Objects.equals(this.updatedBy, newValue)) return this;
return new SlackUsergroup(
this.id,
this.teamId,
this.usergroup,
this.name,
this.description,
this.handle,
this.external,
this.dateCreatedEpochSeconds,
this.dateUpdatedEpochSeconds,
this.dateDeletedEpochSeconds,
this.autoType,
this.createdBy,
newValue,
this.deletedBy,
this.preferences,
this.userIdsInGroup,
this.userCount);
}
/**
* Copy the current immutable object by setting an optional value for the {@link SlackUsergroupIF#getUpdatedBy() updatedBy} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for updatedBy
* @return A modified copy of {@code this} object
*/
public final SlackUsergroup withUpdatedBy(Optional optional) {
@Nullable String value = optional.orElse(null);
if (Objects.equals(this.updatedBy, value)) return this;
return new SlackUsergroup(
this.id,
this.teamId,
this.usergroup,
this.name,
this.description,
this.handle,
this.external,
this.dateCreatedEpochSeconds,
this.dateUpdatedEpochSeconds,
this.dateDeletedEpochSeconds,
this.autoType,
this.createdBy,
value,
this.deletedBy,
this.preferences,
this.userIdsInGroup,
this.userCount);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link SlackUsergroupIF#getDeletedBy() deletedBy} attribute.
* @param value The value for deletedBy, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final SlackUsergroup withDeletedBy(@Nullable String value) {
@Nullable String newValue = value;
if (Objects.equals(this.deletedBy, newValue)) return this;
return new SlackUsergroup(
this.id,
this.teamId,
this.usergroup,
this.name,
this.description,
this.handle,
this.external,
this.dateCreatedEpochSeconds,
this.dateUpdatedEpochSeconds,
this.dateDeletedEpochSeconds,
this.autoType,
this.createdBy,
this.updatedBy,
newValue,
this.preferences,
this.userIdsInGroup,
this.userCount);
}
/**
* Copy the current immutable object by setting an optional value for the {@link SlackUsergroupIF#getDeletedBy() deletedBy} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for deletedBy
* @return A modified copy of {@code this} object
*/
public final SlackUsergroup withDeletedBy(Optional optional) {
@Nullable String value = optional.orElse(null);
if (Objects.equals(this.deletedBy, value)) return this;
return new SlackUsergroup(
this.id,
this.teamId,
this.usergroup,
this.name,
this.description,
this.handle,
this.external,
this.dateCreatedEpochSeconds,
this.dateUpdatedEpochSeconds,
this.dateDeletedEpochSeconds,
this.autoType,
this.createdBy,
this.updatedBy,
value,
this.preferences,
this.userIdsInGroup,
this.userCount);
}
/**
* Copy the current immutable object by setting a value for the {@link SlackUsergroupIF#getPreferences() preferences} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for preferences
* @return A modified copy of the {@code this} object
*/
public final SlackUsergroup withPreferences(UsergroupPreferences value) {
if (this.preferences == value) return this;
UsergroupPreferences newValue = Objects.requireNonNull(value, "preferences");
return new SlackUsergroup(
this.id,
this.teamId,
this.usergroup,
this.name,
this.description,
this.handle,
this.external,
this.dateCreatedEpochSeconds,
this.dateUpdatedEpochSeconds,
this.dateDeletedEpochSeconds,
this.autoType,
this.createdBy,
this.updatedBy,
this.deletedBy,
newValue,
this.userIdsInGroup,
this.userCount);
}
/**
* Copy the current immutable object with elements that replace the content of {@link SlackUsergroupIF#getUserIdsInGroup() userIdsInGroup}.
* @param elements The elements to set
* @return A modified copy of {@code this} object
*/
public final SlackUsergroup withUserIdsInGroup(String... elements) {
List newValue = createUnmodifiableList(false, createSafeList(Arrays.asList(elements), true, false));
return new SlackUsergroup(
this.id,
this.teamId,
this.usergroup,
this.name,
this.description,
this.handle,
this.external,
this.dateCreatedEpochSeconds,
this.dateUpdatedEpochSeconds,
this.dateDeletedEpochSeconds,
this.autoType,
this.createdBy,
this.updatedBy,
this.deletedBy,
this.preferences,
newValue,
this.userCount);
}
/**
* Copy the current immutable object with elements that replace the content of {@link SlackUsergroupIF#getUserIdsInGroup() userIdsInGroup}.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements An iterable of userIdsInGroup elements to set
* @return A modified copy of {@code this} object
*/
public final SlackUsergroup withUserIdsInGroup(Iterable elements) {
if (this.userIdsInGroup == elements) return this;
List newValue = createUnmodifiableList(false, createSafeList(elements, true, false));
return new SlackUsergroup(
this.id,
this.teamId,
this.usergroup,
this.name,
this.description,
this.handle,
this.external,
this.dateCreatedEpochSeconds,
this.dateUpdatedEpochSeconds,
this.dateDeletedEpochSeconds,
this.autoType,
this.createdBy,
this.updatedBy,
this.deletedBy,
this.preferences,
newValue,
this.userCount);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link SlackUsergroupIF#getUserCount() userCount} attribute.
* @param value The value for userCount, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final SlackUsergroup withUserCount(@Nullable Integer value) {
@Nullable Integer newValue = value;
if (Objects.equals(this.userCount, newValue)) return this;
return new SlackUsergroup(
this.id,
this.teamId,
this.usergroup,
this.name,
this.description,
this.handle,
this.external,
this.dateCreatedEpochSeconds,
this.dateUpdatedEpochSeconds,
this.dateDeletedEpochSeconds,
this.autoType,
this.createdBy,
this.updatedBy,
this.deletedBy,
this.preferences,
this.userIdsInGroup,
newValue);
}
/**
* Copy the current immutable object by setting an optional value for the {@link SlackUsergroupIF#getUserCount() userCount} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for userCount
* @return A modified copy of {@code this} object
*/
public final SlackUsergroup withUserCount(Optional optional) {
@Nullable Integer value = optional.orElse(null);
if (Objects.equals(this.userCount, value)) return this;
return new SlackUsergroup(
this.id,
this.teamId,
this.usergroup,
this.name,
this.description,
this.handle,
this.external,
this.dateCreatedEpochSeconds,
this.dateUpdatedEpochSeconds,
this.dateDeletedEpochSeconds,
this.autoType,
this.createdBy,
this.updatedBy,
this.deletedBy,
this.preferences,
this.userIdsInGroup,
value);
}
/**
* This instance is equal to all instances of {@code SlackUsergroup} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(@Nullable Object another) {
if (this == another) return true;
return another instanceof SlackUsergroup
&& equalTo(0, (SlackUsergroup) another);
}
private boolean equalTo(int synthetic, SlackUsergroup another) {
return id.equals(another.id)
&& teamId.equals(another.teamId)
&& usergroup == another.usergroup
&& name.equals(another.name)
&& Objects.equals(description, another.description)
&& handle.equals(another.handle)
&& external == another.external
&& dateCreatedEpochSeconds == another.dateCreatedEpochSeconds
&& dateUpdatedEpochSeconds == another.dateUpdatedEpochSeconds
&& dateDeletedEpochSeconds == another.dateDeletedEpochSeconds
&& Objects.equals(autoType, another.autoType)
&& Objects.equals(createdBy, another.createdBy)
&& Objects.equals(updatedBy, another.updatedBy)
&& Objects.equals(deletedBy, another.deletedBy)
&& deleted == another.deleted
&& preferences.equals(another.preferences)
&& userIdsInGroup.equals(another.userIdsInGroup)
&& Objects.equals(userCount, another.userCount);
}
/**
* Computes a hash code from attributes: {@code id}, {@code teamId}, {@code usergroup}, {@code name}, {@code description}, {@code handle}, {@code external}, {@code dateCreatedEpochSeconds}, {@code dateUpdatedEpochSeconds}, {@code dateDeletedEpochSeconds}, {@code autoType}, {@code createdBy}, {@code updatedBy}, {@code deletedBy}, {@code deleted}, {@code preferences}, {@code userIdsInGroup}, {@code userCount}.
* @return hashCode value
*/
@Override
public int hashCode() {
int h = 5381;
h += (h << 5) + id.hashCode();
h += (h << 5) + teamId.hashCode();
h += (h << 5) + Boolean.hashCode(usergroup);
h += (h << 5) + name.hashCode();
h += (h << 5) + Objects.hashCode(description);
h += (h << 5) + handle.hashCode();
h += (h << 5) + Boolean.hashCode(external);
h += (h << 5) + dateCreatedEpochSeconds;
h += (h << 5) + dateUpdatedEpochSeconds;
h += (h << 5) + dateDeletedEpochSeconds;
h += (h << 5) + Objects.hashCode(autoType);
h += (h << 5) + Objects.hashCode(createdBy);
h += (h << 5) + Objects.hashCode(updatedBy);
h += (h << 5) + Objects.hashCode(deletedBy);
h += (h << 5) + Boolean.hashCode(deleted);
h += (h << 5) + preferences.hashCode();
h += (h << 5) + userIdsInGroup.hashCode();
h += (h << 5) + Objects.hashCode(userCount);
return h;
}
/**
* Prints the immutable value {@code SlackUsergroup} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
StringBuilder builder = new StringBuilder("SlackUsergroup{");
builder.append("id=").append(id);
builder.append(", ");
builder.append("teamId=").append(teamId);
builder.append(", ");
builder.append("usergroup=").append(usergroup);
builder.append(", ");
builder.append("name=").append(name);
if (description != null) {
builder.append(", ");
builder.append("description=").append(description);
}
builder.append(", ");
builder.append("handle=").append(handle);
builder.append(", ");
builder.append("external=").append(external);
builder.append(", ");
builder.append("dateCreatedEpochSeconds=").append(dateCreatedEpochSeconds);
builder.append(", ");
builder.append("dateUpdatedEpochSeconds=").append(dateUpdatedEpochSeconds);
builder.append(", ");
builder.append("dateDeletedEpochSeconds=").append(dateDeletedEpochSeconds);
if (autoType != null) {
builder.append(", ");
builder.append("autoType=").append(autoType);
}
if (createdBy != null) {
builder.append(", ");
builder.append("createdBy=").append(createdBy);
}
if (updatedBy != null) {
builder.append(", ");
builder.append("updatedBy=").append(updatedBy);
}
if (deletedBy != null) {
builder.append(", ");
builder.append("deletedBy=").append(deletedBy);
}
builder.append(", ");
builder.append("deleted=").append(deleted);
builder.append(", ");
builder.append("preferences=").append(preferences);
builder.append(", ");
builder.append("userIdsInGroup=").append(userIdsInGroup);
if (userCount != null) {
builder.append(", ");
builder.append("userCount=").append(userCount);
}
return builder.append("}").toString();
}
/**
* Utility type used to correctly read immutable object from JSON representation.
* @deprecated Do not use this type directly, it exists only for the Jackson-binding infrastructure
*/
@Generated(from = "SlackUsergroupIF", generator = "Immutables")
@Deprecated
@JsonAutoDetect(fieldVisibility = JsonAutoDetect.Visibility.NONE)
static final class Json implements SlackUsergroupIF {
@Nullable String id;
@Nullable String teamId;
boolean usergroup;
boolean usergroupIsSet;
@Nullable String name;
@Nullable Optional description = Optional.empty();
@Nullable String handle;
boolean external;
boolean externalIsSet;
int dateCreatedEpochSeconds;
boolean dateCreatedEpochSecondsIsSet;
int dateUpdatedEpochSeconds;
boolean dateUpdatedEpochSecondsIsSet;
int dateDeletedEpochSeconds;
boolean dateDeletedEpochSecondsIsSet;
@Nullable Optional autoType = Optional.empty();
@Nullable Optional createdBy = Optional.empty();
@Nullable Optional updatedBy = Optional.empty();
@Nullable Optional deletedBy = Optional.empty();
@Nullable UsergroupPreferences preferences;
@Nullable List userIdsInGroup = Collections.emptyList();
@Nullable Optional userCount = Optional.empty();
@JsonProperty
public void setId(String id) {
this.id = id;
}
@JsonProperty
public void setTeamId(String teamId) {
this.teamId = teamId;
}
@JsonProperty("is_usergroup")
public void setUsergroup(boolean usergroup) {
this.usergroup = usergroup;
this.usergroupIsSet = true;
}
@JsonProperty
public void setName(String name) {
this.name = name;
}
@JsonProperty
public void setDescription(Optional description) {
this.description = description;
}
@JsonProperty
public void setHandle(String handle) {
this.handle = handle;
}
@JsonProperty("is_external")
public void setExternal(boolean external) {
this.external = external;
this.externalIsSet = true;
}
@JsonProperty("date_create")
public void setDateCreatedEpochSeconds(int dateCreatedEpochSeconds) {
this.dateCreatedEpochSeconds = dateCreatedEpochSeconds;
this.dateCreatedEpochSecondsIsSet = true;
}
@JsonProperty("date_update")
public void setDateUpdatedEpochSeconds(int dateUpdatedEpochSeconds) {
this.dateUpdatedEpochSeconds = dateUpdatedEpochSeconds;
this.dateUpdatedEpochSecondsIsSet = true;
}
@JsonProperty("date_delete")
public void setDateDeletedEpochSeconds(int dateDeletedEpochSeconds) {
this.dateDeletedEpochSeconds = dateDeletedEpochSeconds;
this.dateDeletedEpochSecondsIsSet = true;
}
@JsonProperty
public void setAutoType(Optional autoType) {
this.autoType = autoType;
}
@JsonProperty
public void setCreatedBy(Optional createdBy) {
this.createdBy = createdBy;
}
@JsonProperty
public void setUpdatedBy(Optional updatedBy) {
this.updatedBy = updatedBy;
}
@JsonProperty
public void setDeletedBy(Optional deletedBy) {
this.deletedBy = deletedBy;
}
@JsonProperty("prefs")
public void setPreferences(UsergroupPreferences preferences) {
this.preferences = preferences;
}
@JsonProperty("users")
public void setUserIdsInGroup(List userIdsInGroup) {
this.userIdsInGroup = userIdsInGroup;
}
@JsonProperty
public void setUserCount(Optional userCount) {
this.userCount = userCount;
}
@Override
public String getId() { throw new UnsupportedOperationException(); }
@Override
public String getTeamId() { throw new UnsupportedOperationException(); }
@Override
public boolean isUsergroup() { throw new UnsupportedOperationException(); }
@Override
public String getName() { throw new UnsupportedOperationException(); }
@Override
public Optional getDescription() { throw new UnsupportedOperationException(); }
@Override
public String getHandle() { throw new UnsupportedOperationException(); }
@Override
public boolean isExternal() { throw new UnsupportedOperationException(); }
@Override
public int getDateCreatedEpochSeconds() { throw new UnsupportedOperationException(); }
@Override
public int getDateUpdatedEpochSeconds() { throw new UnsupportedOperationException(); }
@Override
public int getDateDeletedEpochSeconds() { throw new UnsupportedOperationException(); }
@Override
public Optional getAutoType() { throw new UnsupportedOperationException(); }
@Override
public Optional getCreatedBy() { throw new UnsupportedOperationException(); }
@Override
public Optional getUpdatedBy() { throw new UnsupportedOperationException(); }
@Override
public Optional getDeletedBy() { throw new UnsupportedOperationException(); }
@JsonIgnore
@Override
public boolean isDeleted() { throw new UnsupportedOperationException(); }
@Override
public UsergroupPreferences getPreferences() { throw new UnsupportedOperationException(); }
@Override
public List getUserIdsInGroup() { throw new UnsupportedOperationException(); }
@Override
public Optional getUserCount() { throw new UnsupportedOperationException(); }
}
/**
* @param json A JSON-bindable data structure
* @return An immutable value type
* @deprecated Do not use this method directly, it exists only for the Jackson-binding infrastructure
*/
@Deprecated
@JsonCreator(mode = JsonCreator.Mode.DELEGATING)
static SlackUsergroup fromJson(Json json) {
SlackUsergroup.Builder builder = SlackUsergroup.builder();
if (json.id != null) {
builder.setId(json.id);
}
if (json.teamId != null) {
builder.setTeamId(json.teamId);
}
if (json.usergroupIsSet) {
builder.setUsergroup(json.usergroup);
}
if (json.name != null) {
builder.setName(json.name);
}
if (json.description != null) {
builder.setDescription(json.description);
}
if (json.handle != null) {
builder.setHandle(json.handle);
}
if (json.externalIsSet) {
builder.setExternal(json.external);
}
if (json.dateCreatedEpochSecondsIsSet) {
builder.setDateCreatedEpochSeconds(json.dateCreatedEpochSeconds);
}
if (json.dateUpdatedEpochSecondsIsSet) {
builder.setDateUpdatedEpochSeconds(json.dateUpdatedEpochSeconds);
}
if (json.dateDeletedEpochSecondsIsSet) {
builder.setDateDeletedEpochSeconds(json.dateDeletedEpochSeconds);
}
if (json.autoType != null) {
builder.setAutoType(json.autoType);
}
if (json.createdBy != null) {
builder.setCreatedBy(json.createdBy);
}
if (json.updatedBy != null) {
builder.setUpdatedBy(json.updatedBy);
}
if (json.deletedBy != null) {
builder.setDeletedBy(json.deletedBy);
}
if (json.preferences != null) {
builder.setPreferences(json.preferences);
}
if (json.userIdsInGroup != null) {
builder.addAllUserIdsInGroup(json.userIdsInGroup);
}
if (json.userCount != null) {
builder.setUserCount(json.userCount);
}
return builder.build();
}
/**
* Creates an immutable copy of a {@link SlackUsergroupIF} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable SlackUsergroup instance
*/
public static SlackUsergroup copyOf(SlackUsergroupIF instance) {
if (instance instanceof SlackUsergroup) {
return (SlackUsergroup) instance;
}
return SlackUsergroup.builder()
.from(instance)
.build();
}
/**
* Creates a builder for {@link SlackUsergroup SlackUsergroup}.
*
* SlackUsergroup.builder()
* .setId(String) // required {@link SlackUsergroupIF#getId() id}
* .setTeamId(String) // required {@link SlackUsergroupIF#getTeamId() teamId}
* .setUsergroup(boolean) // required {@link SlackUsergroupIF#isUsergroup() usergroup}
* .setName(String) // required {@link SlackUsergroupIF#getName() name}
* .setDescription(String) // optional {@link SlackUsergroupIF#getDescription() description}
* .setHandle(String) // required {@link SlackUsergroupIF#getHandle() handle}
* .setExternal(boolean) // required {@link SlackUsergroupIF#isExternal() external}
* .setDateCreatedEpochSeconds(int) // required {@link SlackUsergroupIF#getDateCreatedEpochSeconds() dateCreatedEpochSeconds}
* .setDateUpdatedEpochSeconds(int) // required {@link SlackUsergroupIF#getDateUpdatedEpochSeconds() dateUpdatedEpochSeconds}
* .setDateDeletedEpochSeconds(int) // required {@link SlackUsergroupIF#getDateDeletedEpochSeconds() dateDeletedEpochSeconds}
* .setAutoType(String) // optional {@link SlackUsergroupIF#getAutoType() autoType}
* .setCreatedBy(String) // optional {@link SlackUsergroupIF#getCreatedBy() createdBy}
* .setUpdatedBy(String) // optional {@link SlackUsergroupIF#getUpdatedBy() updatedBy}
* .setDeletedBy(String) // optional {@link SlackUsergroupIF#getDeletedBy() deletedBy}
* .setPreferences(com.hubspot.slack.client.models.usergroups.UsergroupPreferences) // required {@link SlackUsergroupIF#getPreferences() preferences}
* .addUserIdsInGroup|addAllUserIdsInGroup(String) // {@link SlackUsergroupIF#getUserIdsInGroup() userIdsInGroup} elements
* .setUserCount(Integer) // optional {@link SlackUsergroupIF#getUserCount() userCount}
* .build();
*
* @return A new SlackUsergroup builder
*/
public static SlackUsergroup.Builder builder() {
return new SlackUsergroup.Builder();
}
/**
* Builds instances of type {@link SlackUsergroup SlackUsergroup}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
@Generated(from = "SlackUsergroupIF", generator = "Immutables")
@NotThreadSafe
public static final class Builder {
private static final long INIT_BIT_ID = 0x1L;
private static final long INIT_BIT_TEAM_ID = 0x2L;
private static final long INIT_BIT_USERGROUP = 0x4L;
private static final long INIT_BIT_NAME = 0x8L;
private static final long INIT_BIT_HANDLE = 0x10L;
private static final long INIT_BIT_EXTERNAL = 0x20L;
private static final long INIT_BIT_DATE_CREATED_EPOCH_SECONDS = 0x40L;
private static final long INIT_BIT_DATE_UPDATED_EPOCH_SECONDS = 0x80L;
private static final long INIT_BIT_DATE_DELETED_EPOCH_SECONDS = 0x100L;
private static final long INIT_BIT_PREFERENCES = 0x200L;
private long initBits = 0x3ffL;
private @Nullable String id;
private @Nullable String teamId;
private boolean usergroup;
private @Nullable String name;
private @Nullable String description;
private @Nullable String handle;
private boolean external;
private int dateCreatedEpochSeconds;
private int dateUpdatedEpochSeconds;
private int dateDeletedEpochSeconds;
private @Nullable String autoType;
private @Nullable String createdBy;
private @Nullable String updatedBy;
private @Nullable String deletedBy;
private @Nullable UsergroupPreferences preferences;
private List userIdsInGroup = new ArrayList();
private @Nullable Integer userCount;
private Builder() {
}
/**
* Fill a builder with attribute values from the provided {@code SlackUsergroupIF} instance.
* Regular attribute values will be replaced with those from the given instance.
* Absent optional values will not replace present values.
* Collection elements and entries will be added, not replaced.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(SlackUsergroupIF instance) {
Objects.requireNonNull(instance, "instance");
this.setId(instance.getId());
this.setTeamId(instance.getTeamId());
this.setUsergroup(instance.isUsergroup());
this.setName(instance.getName());
Optional descriptionOptional = instance.getDescription();
if (descriptionOptional.isPresent()) {
setDescription(descriptionOptional);
}
this.setHandle(instance.getHandle());
this.setExternal(instance.isExternal());
this.setDateCreatedEpochSeconds(instance.getDateCreatedEpochSeconds());
this.setDateUpdatedEpochSeconds(instance.getDateUpdatedEpochSeconds());
this.setDateDeletedEpochSeconds(instance.getDateDeletedEpochSeconds());
Optional autoTypeOptional = instance.getAutoType();
if (autoTypeOptional.isPresent()) {
setAutoType(autoTypeOptional);
}
Optional createdByOptional = instance.getCreatedBy();
if (createdByOptional.isPresent()) {
setCreatedBy(createdByOptional);
}
Optional updatedByOptional = instance.getUpdatedBy();
if (updatedByOptional.isPresent()) {
setUpdatedBy(updatedByOptional);
}
Optional deletedByOptional = instance.getDeletedBy();
if (deletedByOptional.isPresent()) {
setDeletedBy(deletedByOptional);
}
this.setPreferences(instance.getPreferences());
addAllUserIdsInGroup(instance.getUserIdsInGroup());
Optional userCountOptional = instance.getUserCount();
if (userCountOptional.isPresent()) {
setUserCount(userCountOptional);
}
return this;
}
/**
* Initializes the value for the {@link SlackUsergroupIF#getId() id} attribute.
* @param id The value for id
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setId(String id) {
this.id = Objects.requireNonNull(id, "id");
initBits &= ~INIT_BIT_ID;
return this;
}
/**
* Initializes the value for the {@link SlackUsergroupIF#getTeamId() teamId} attribute.
* @param teamId The value for teamId
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setTeamId(String teamId) {
this.teamId = Objects.requireNonNull(teamId, "teamId");
initBits &= ~INIT_BIT_TEAM_ID;
return this;
}
/**
* Initializes the value for the {@link SlackUsergroupIF#isUsergroup() usergroup} attribute.
* @param usergroup The value for usergroup
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setUsergroup(boolean usergroup) {
this.usergroup = usergroup;
initBits &= ~INIT_BIT_USERGROUP;
return this;
}
/**
* Initializes the value for the {@link SlackUsergroupIF#getName() name} attribute.
* @param name The value for name
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setName(String name) {
this.name = Objects.requireNonNull(name, "name");
initBits &= ~INIT_BIT_NAME;
return this;
}
/**
* Initializes the optional value {@link SlackUsergroupIF#getDescription() description} to description.
* @param description The value for description, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
public final Builder setDescription(@Nullable String description) {
this.description = description;
return this;
}
/**
* Initializes the optional value {@link SlackUsergroupIF#getDescription() description} to description.
* @param description The value for description
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setDescription(Optional description) {
this.description = description.orElse(null);
return this;
}
/**
* Initializes the value for the {@link SlackUsergroupIF#getHandle() handle} attribute.
* @param handle The value for handle
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setHandle(String handle) {
this.handle = Objects.requireNonNull(handle, "handle");
initBits &= ~INIT_BIT_HANDLE;
return this;
}
/**
* Initializes the value for the {@link SlackUsergroupIF#isExternal() external} attribute.
* @param external The value for external
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setExternal(boolean external) {
this.external = external;
initBits &= ~INIT_BIT_EXTERNAL;
return this;
}
/**
* Initializes the value for the {@link SlackUsergroupIF#getDateCreatedEpochSeconds() dateCreatedEpochSeconds} attribute.
* @param dateCreatedEpochSeconds The value for dateCreatedEpochSeconds
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setDateCreatedEpochSeconds(int dateCreatedEpochSeconds) {
this.dateCreatedEpochSeconds = dateCreatedEpochSeconds;
initBits &= ~INIT_BIT_DATE_CREATED_EPOCH_SECONDS;
return this;
}
/**
* Initializes the value for the {@link SlackUsergroupIF#getDateUpdatedEpochSeconds() dateUpdatedEpochSeconds} attribute.
* @param dateUpdatedEpochSeconds The value for dateUpdatedEpochSeconds
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setDateUpdatedEpochSeconds(int dateUpdatedEpochSeconds) {
this.dateUpdatedEpochSeconds = dateUpdatedEpochSeconds;
initBits &= ~INIT_BIT_DATE_UPDATED_EPOCH_SECONDS;
return this;
}
/**
* Initializes the value for the {@link SlackUsergroupIF#getDateDeletedEpochSeconds() dateDeletedEpochSeconds} attribute.
* @param dateDeletedEpochSeconds The value for dateDeletedEpochSeconds
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setDateDeletedEpochSeconds(int dateDeletedEpochSeconds) {
this.dateDeletedEpochSeconds = dateDeletedEpochSeconds;
initBits &= ~INIT_BIT_DATE_DELETED_EPOCH_SECONDS;
return this;
}
/**
* Initializes the optional value {@link SlackUsergroupIF#getAutoType() autoType} to autoType.
* @param autoType The value for autoType, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
public final Builder setAutoType(@Nullable String autoType) {
this.autoType = autoType;
return this;
}
/**
* Initializes the optional value {@link SlackUsergroupIF#getAutoType() autoType} to autoType.
* @param autoType The value for autoType
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setAutoType(Optional autoType) {
this.autoType = autoType.orElse(null);
return this;
}
/**
* Initializes the optional value {@link SlackUsergroupIF#getCreatedBy() createdBy} to createdBy.
* @param createdBy The value for createdBy, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
public final Builder setCreatedBy(@Nullable String createdBy) {
this.createdBy = createdBy;
return this;
}
/**
* Initializes the optional value {@link SlackUsergroupIF#getCreatedBy() createdBy} to createdBy.
* @param createdBy The value for createdBy
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setCreatedBy(Optional createdBy) {
this.createdBy = createdBy.orElse(null);
return this;
}
/**
* Initializes the optional value {@link SlackUsergroupIF#getUpdatedBy() updatedBy} to updatedBy.
* @param updatedBy The value for updatedBy, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
public final Builder setUpdatedBy(@Nullable String updatedBy) {
this.updatedBy = updatedBy;
return this;
}
/**
* Initializes the optional value {@link SlackUsergroupIF#getUpdatedBy() updatedBy} to updatedBy.
* @param updatedBy The value for updatedBy
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setUpdatedBy(Optional updatedBy) {
this.updatedBy = updatedBy.orElse(null);
return this;
}
/**
* Initializes the optional value {@link SlackUsergroupIF#getDeletedBy() deletedBy} to deletedBy.
* @param deletedBy The value for deletedBy, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
public final Builder setDeletedBy(@Nullable String deletedBy) {
this.deletedBy = deletedBy;
return this;
}
/**
* Initializes the optional value {@link SlackUsergroupIF#getDeletedBy() deletedBy} to deletedBy.
* @param deletedBy The value for deletedBy
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setDeletedBy(Optional deletedBy) {
this.deletedBy = deletedBy.orElse(null);
return this;
}
/**
* Initializes the value for the {@link SlackUsergroupIF#getPreferences() preferences} attribute.
* @param preferences The value for preferences
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setPreferences(UsergroupPreferences preferences) {
this.preferences = Objects.requireNonNull(preferences, "preferences");
initBits &= ~INIT_BIT_PREFERENCES;
return this;
}
/**
* Adds one element to {@link SlackUsergroupIF#getUserIdsInGroup() userIdsInGroup} list.
* @param element A userIdsInGroup element
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addUserIdsInGroup(String element) {
this.userIdsInGroup.add(Objects.requireNonNull(element, "userIdsInGroup element"));
return this;
}
/**
* Adds elements to {@link SlackUsergroupIF#getUserIdsInGroup() userIdsInGroup} list.
* @param elements An array of userIdsInGroup elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addUserIdsInGroup(String... elements) {
for (String element : elements) {
this.userIdsInGroup.add(Objects.requireNonNull(element, "userIdsInGroup element"));
}
return this;
}
/**
* Sets or replaces all elements for {@link SlackUsergroupIF#getUserIdsInGroup() userIdsInGroup} list.
* @param elements An iterable of userIdsInGroup elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setUserIdsInGroup(Iterable elements) {
this.userIdsInGroup.clear();
return addAllUserIdsInGroup(elements);
}
/**
* Adds elements to {@link SlackUsergroupIF#getUserIdsInGroup() userIdsInGroup} list.
* @param elements An iterable of userIdsInGroup elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addAllUserIdsInGroup(Iterable elements) {
for (String element : elements) {
this.userIdsInGroup.add(Objects.requireNonNull(element, "userIdsInGroup element"));
}
return this;
}
/**
* Initializes the optional value {@link SlackUsergroupIF#getUserCount() userCount} to userCount.
* @param userCount The value for userCount, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
public final Builder setUserCount(@Nullable Integer userCount) {
this.userCount = userCount;
return this;
}
/**
* Initializes the optional value {@link SlackUsergroupIF#getUserCount() userCount} to userCount.
* @param userCount The value for userCount
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setUserCount(Optional userCount) {
this.userCount = userCount.orElse(null);
return this;
}
/**
* Builds a new {@link SlackUsergroup SlackUsergroup}.
* @return An immutable instance of SlackUsergroup
* @throws com.hubspot.immutables.validation.InvalidImmutableStateException if any required attributes are missing
*/
public SlackUsergroup build() {
checkRequiredAttributes();
return new SlackUsergroup(
id,
teamId,
usergroup,
name,
description,
handle,
external,
dateCreatedEpochSeconds,
dateUpdatedEpochSeconds,
dateDeletedEpochSeconds,
autoType,
createdBy,
updatedBy,
deletedBy,
preferences,
createUnmodifiableList(true, userIdsInGroup),
userCount);
}
private boolean idIsSet() {
return (initBits & INIT_BIT_ID) == 0;
}
private boolean teamIdIsSet() {
return (initBits & INIT_BIT_TEAM_ID) == 0;
}
private boolean usergroupIsSet() {
return (initBits & INIT_BIT_USERGROUP) == 0;
}
private boolean nameIsSet() {
return (initBits & INIT_BIT_NAME) == 0;
}
private boolean handleIsSet() {
return (initBits & INIT_BIT_HANDLE) == 0;
}
private boolean externalIsSet() {
return (initBits & INIT_BIT_EXTERNAL) == 0;
}
private boolean dateCreatedEpochSecondsIsSet() {
return (initBits & INIT_BIT_DATE_CREATED_EPOCH_SECONDS) == 0;
}
private boolean dateUpdatedEpochSecondsIsSet() {
return (initBits & INIT_BIT_DATE_UPDATED_EPOCH_SECONDS) == 0;
}
private boolean dateDeletedEpochSecondsIsSet() {
return (initBits & INIT_BIT_DATE_DELETED_EPOCH_SECONDS) == 0;
}
private boolean preferencesIsSet() {
return (initBits & INIT_BIT_PREFERENCES) == 0;
}
private void checkRequiredAttributes() {
if (initBits != 0) {
throw new InvalidImmutableStateException(formatRequiredAttributesMessage());
}
}
private String formatRequiredAttributesMessage() {
List attributes = new ArrayList<>();
if (!idIsSet()) attributes.add("id");
if (!teamIdIsSet()) attributes.add("teamId");
if (!usergroupIsSet()) attributes.add("usergroup");
if (!nameIsSet()) attributes.add("name");
if (!handleIsSet()) attributes.add("handle");
if (!externalIsSet()) attributes.add("external");
if (!dateCreatedEpochSecondsIsSet()) attributes.add("dateCreatedEpochSeconds");
if (!dateUpdatedEpochSecondsIsSet()) attributes.add("dateUpdatedEpochSeconds");
if (!dateDeletedEpochSecondsIsSet()) attributes.add("dateDeletedEpochSeconds");
if (!preferencesIsSet()) attributes.add("preferences");
return "Cannot build SlackUsergroup, some of required attributes are not set " + attributes;
}
}
private static List createSafeList(Iterable extends T> iterable, boolean checkNulls, boolean skipNulls) {
ArrayList list;
if (iterable instanceof Collection>) {
int size = ((Collection>) iterable).size();
if (size == 0) return Collections.emptyList();
list = new ArrayList<>(size);
} else {
list = new ArrayList<>();
}
for (T element : iterable) {
if (skipNulls && element == null) continue;
if (checkNulls) Objects.requireNonNull(element, "element");
list.add(element);
}
return list;
}
private static List createUnmodifiableList(boolean clone, List list) {
switch(list.size()) {
case 0: return Collections.emptyList();
case 1: return Collections.singletonList(list.get(0));
default:
if (clone) {
return Collections.unmodifiableList(new ArrayList<>(list));
} else {
if (list instanceof ArrayList>) {
((ArrayList>) list).trimToSize();
}
return Collections.unmodifiableList(list);
}
}
}
}