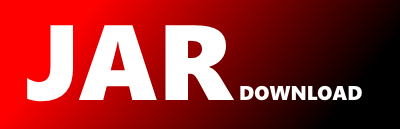
com.hubspot.slack.client.models.users.SlackUser Maven / Gradle / Ivy
package com.hubspot.slack.client.models.users;
import com.fasterxml.jackson.annotation.JsonAutoDetect;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonSetter;
import com.hubspot.immutables.validation.InvalidImmutableStateException;
import edu.umd.cs.findbugs.annotations.SuppressFBWarnings;
import java.util.ArrayList;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
import javax.annotation.ParametersAreNonnullByDefault;
import javax.annotation.concurrent.Immutable;
import javax.annotation.concurrent.NotThreadSafe;
import org.immutables.value.Generated;
/**
* Immutable implementation of {@link SlackUserIF}.
*
* Use the builder to create immutable instances:
* {@code SlackUser.builder()}.
*/
@Generated(from = "SlackUserIF", generator = "Immutables")
@SuppressWarnings({"all"})
@SuppressFBWarnings
@ParametersAreNonnullByDefault
@javax.annotation.processing.Generated("org.immutables.processor.ProxyProcessor")
@Immutable
public final class SlackUser implements SlackUserIF {
private final String id;
private final @Nullable String username;
private final @Nullable UserProfile profile;
private final @Nullable Boolean deleted;
private final @Nullable String color;
private final @Nullable String admin;
private final @Nullable String owner;
private final @Nullable String teamId;
private final @Nullable String realName;
private final @Nullable Boolean primaryOwner;
private final @Nullable Boolean restricted;
private final @Nullable Boolean ultraRestricted;
private final @Nullable Boolean bot;
private final @Nullable Boolean appUser;
private final @Nullable String timezone;
private final @Nullable String timezoneLabel;
private final @Nullable String timezoneOffset;
private final @Nullable Integer rawUpdated;
private final @Nullable String locale;
private transient final Optional updatedAt;
private SlackUser(
String id,
@Nullable String username,
@Nullable UserProfile profile,
@Nullable Boolean deleted,
@Nullable String color,
@Nullable String admin,
@Nullable String owner,
@Nullable String teamId,
@Nullable String realName,
@Nullable Boolean primaryOwner,
@Nullable Boolean restricted,
@Nullable Boolean ultraRestricted,
@Nullable Boolean bot,
@Nullable Boolean appUser,
@Nullable String timezone,
@Nullable String timezoneLabel,
@Nullable String timezoneOffset,
@Nullable Integer rawUpdated,
@Nullable String locale) {
this.id = id;
this.username = username;
this.profile = profile;
this.deleted = deleted;
this.color = color;
this.admin = admin;
this.owner = owner;
this.teamId = teamId;
this.realName = realName;
this.primaryOwner = primaryOwner;
this.restricted = restricted;
this.ultraRestricted = ultraRestricted;
this.bot = bot;
this.appUser = appUser;
this.timezone = timezone;
this.timezoneLabel = timezoneLabel;
this.timezoneOffset = timezoneOffset;
this.rawUpdated = rawUpdated;
this.locale = locale;
this.updatedAt = Objects.requireNonNull(SlackUserIF.super.getUpdatedAt(), "updatedAt");
}
/**
* @return The value of the {@code id} attribute
*/
@JsonProperty
@Override
public String getId() {
return id;
}
/**
* @return The value of the {@code username} attribute
*/
@JsonProperty("name")
@Override
public Optional getUsername() {
return Optional.ofNullable(username);
}
/**
* @return The value of the {@code profile} attribute
*/
@JsonProperty
@Override
public Optional getProfile() {
return Optional.ofNullable(profile);
}
/**
* @return The value of the {@code deleted} attribute
*/
@JsonProperty("deleted")
@Override
public Optional isDeleted() {
return Optional.ofNullable(deleted);
}
/**
* @return The value of the {@code color} attribute
*/
@JsonProperty
@Override
public Optional getColor() {
return Optional.ofNullable(color);
}
/**
* @return The value of the {@code admin} attribute
*/
@JsonProperty
@Override
public Optional isAdmin() {
return Optional.ofNullable(admin);
}
/**
* @return The value of the {@code owner} attribute
*/
@JsonProperty
@Override
public Optional isOwner() {
return Optional.ofNullable(owner);
}
/**
* @return The value of the {@code teamId} attribute
*/
@JsonProperty
@Override
public Optional getTeamId() {
return Optional.ofNullable(teamId);
}
/**
* @return The value of the {@code realName} attribute
*/
@JsonProperty
@Override
public Optional getRealName() {
return Optional.ofNullable(realName);
}
/**
* @return The value of the {@code primaryOwner} attribute
*/
@JsonSetter("primaryOwner")
@JsonProperty("is_primary_owner")
@Override
public Optional isPrimaryOwner() {
return Optional.ofNullable(primaryOwner);
}
/**
* @return The value of the {@code restricted} attribute
*/
@JsonSetter("restricted")
@JsonProperty("is_restricted")
@Override
public Optional isRestricted() {
return Optional.ofNullable(restricted);
}
/**
* @return The value of the {@code ultraRestricted} attribute
*/
@JsonSetter("ultra_restricted")
@JsonProperty("is_ultra_restricted")
@Override
public Optional isUltraRestricted() {
return Optional.ofNullable(ultraRestricted);
}
/**
* @return The value of the {@code bot} attribute
*/
@JsonProperty("is_bot")
@Override
public Optional isBot() {
return Optional.ofNullable(bot);
}
/**
* @return The value of the {@code appUser} attribute
*/
@JsonSetter("app_user")
@JsonProperty("is_app_user")
@Override
public Optional isAppUser() {
return Optional.ofNullable(appUser);
}
/**
* @return The value of the {@code timezone} attribute
*/
@JsonProperty("tz")
@Override
public Optional getTimezone() {
return Optional.ofNullable(timezone);
}
/**
* @return The value of the {@code timezoneLabel} attribute
*/
@JsonProperty("tz_label")
@Override
public Optional getTimezoneLabel() {
return Optional.ofNullable(timezoneLabel);
}
/**
* @return The value of the {@code timezoneOffset} attribute
*/
@JsonProperty("tz_offset")
@Override
public Optional getTimezoneOffset() {
return Optional.ofNullable(timezoneOffset);
}
/**
* @return The value of the {@code rawUpdated} attribute
*/
@JsonProperty("updated")
@Override
public Optional getRawUpdated() {
return Optional.ofNullable(rawUpdated);
}
/**
* @return The value of the {@code locale} attribute
*/
@JsonProperty
@Override
public Optional getLocale() {
return Optional.ofNullable(locale);
}
/**
* @return The computed-at-construction value of the {@code updatedAt} attribute
*/
@JsonProperty
@Override
public Optional getUpdatedAt() {
return updatedAt;
}
/**
* Copy the current immutable object by setting a value for the {@link SlackUserIF#getId() id} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for id
* @return A modified copy of the {@code this} object
*/
public final SlackUser withId(String value) {
String newValue = Objects.requireNonNull(value, "id");
if (this.id.equals(newValue)) return this;
return new SlackUser(
newValue,
this.username,
this.profile,
this.deleted,
this.color,
this.admin,
this.owner,
this.teamId,
this.realName,
this.primaryOwner,
this.restricted,
this.ultraRestricted,
this.bot,
this.appUser,
this.timezone,
this.timezoneLabel,
this.timezoneOffset,
this.rawUpdated,
this.locale);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link SlackUserIF#getUsername() username} attribute.
* @param value The value for username, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final SlackUser withUsername(@Nullable String value) {
@Nullable String newValue = value;
if (Objects.equals(this.username, newValue)) return this;
return new SlackUser(
this.id,
newValue,
this.profile,
this.deleted,
this.color,
this.admin,
this.owner,
this.teamId,
this.realName,
this.primaryOwner,
this.restricted,
this.ultraRestricted,
this.bot,
this.appUser,
this.timezone,
this.timezoneLabel,
this.timezoneOffset,
this.rawUpdated,
this.locale);
}
/**
* Copy the current immutable object by setting an optional value for the {@link SlackUserIF#getUsername() username} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for username
* @return A modified copy of {@code this} object
*/
public final SlackUser withUsername(Optional optional) {
@Nullable String value = optional.orElse(null);
if (Objects.equals(this.username, value)) return this;
return new SlackUser(
this.id,
value,
this.profile,
this.deleted,
this.color,
this.admin,
this.owner,
this.teamId,
this.realName,
this.primaryOwner,
this.restricted,
this.ultraRestricted,
this.bot,
this.appUser,
this.timezone,
this.timezoneLabel,
this.timezoneOffset,
this.rawUpdated,
this.locale);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link SlackUserIF#getProfile() profile} attribute.
* @param value The value for profile, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final SlackUser withProfile(@Nullable UserProfile value) {
@Nullable UserProfile newValue = value;
if (this.profile == newValue) return this;
return new SlackUser(
this.id,
this.username,
newValue,
this.deleted,
this.color,
this.admin,
this.owner,
this.teamId,
this.realName,
this.primaryOwner,
this.restricted,
this.ultraRestricted,
this.bot,
this.appUser,
this.timezone,
this.timezoneLabel,
this.timezoneOffset,
this.rawUpdated,
this.locale);
}
/**
* Copy the current immutable object by setting an optional value for the {@link SlackUserIF#getProfile() profile} attribute.
* A shallow reference equality check is used on unboxed optional value to prevent copying of the same value by returning {@code this}.
* @param optional A value for profile
* @return A modified copy of {@code this} object
*/
@SuppressWarnings("unchecked") // safe covariant cast
public final SlackUser withProfile(Optional extends UserProfile> optional) {
@Nullable UserProfile value = optional.orElse(null);
if (this.profile == value) return this;
return new SlackUser(
this.id,
this.username,
value,
this.deleted,
this.color,
this.admin,
this.owner,
this.teamId,
this.realName,
this.primaryOwner,
this.restricted,
this.ultraRestricted,
this.bot,
this.appUser,
this.timezone,
this.timezoneLabel,
this.timezoneOffset,
this.rawUpdated,
this.locale);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link SlackUserIF#isDeleted() deleted} attribute.
* @param value The value for deleted, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final SlackUser withDeleted(@Nullable Boolean value) {
@Nullable Boolean newValue = value;
if (Objects.equals(this.deleted, newValue)) return this;
return new SlackUser(
this.id,
this.username,
this.profile,
newValue,
this.color,
this.admin,
this.owner,
this.teamId,
this.realName,
this.primaryOwner,
this.restricted,
this.ultraRestricted,
this.bot,
this.appUser,
this.timezone,
this.timezoneLabel,
this.timezoneOffset,
this.rawUpdated,
this.locale);
}
/**
* Copy the current immutable object by setting an optional value for the {@link SlackUserIF#isDeleted() deleted} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for deleted
* @return A modified copy of {@code this} object
*/
public final SlackUser withDeleted(Optional optional) {
@Nullable Boolean value = optional.orElse(null);
if (Objects.equals(this.deleted, value)) return this;
return new SlackUser(
this.id,
this.username,
this.profile,
value,
this.color,
this.admin,
this.owner,
this.teamId,
this.realName,
this.primaryOwner,
this.restricted,
this.ultraRestricted,
this.bot,
this.appUser,
this.timezone,
this.timezoneLabel,
this.timezoneOffset,
this.rawUpdated,
this.locale);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link SlackUserIF#getColor() color} attribute.
* @param value The value for color, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final SlackUser withColor(@Nullable String value) {
@Nullable String newValue = value;
if (Objects.equals(this.color, newValue)) return this;
return new SlackUser(
this.id,
this.username,
this.profile,
this.deleted,
newValue,
this.admin,
this.owner,
this.teamId,
this.realName,
this.primaryOwner,
this.restricted,
this.ultraRestricted,
this.bot,
this.appUser,
this.timezone,
this.timezoneLabel,
this.timezoneOffset,
this.rawUpdated,
this.locale);
}
/**
* Copy the current immutable object by setting an optional value for the {@link SlackUserIF#getColor() color} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for color
* @return A modified copy of {@code this} object
*/
public final SlackUser withColor(Optional optional) {
@Nullable String value = optional.orElse(null);
if (Objects.equals(this.color, value)) return this;
return new SlackUser(
this.id,
this.username,
this.profile,
this.deleted,
value,
this.admin,
this.owner,
this.teamId,
this.realName,
this.primaryOwner,
this.restricted,
this.ultraRestricted,
this.bot,
this.appUser,
this.timezone,
this.timezoneLabel,
this.timezoneOffset,
this.rawUpdated,
this.locale);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link SlackUserIF#isAdmin() admin} attribute.
* @param value The value for admin, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final SlackUser withAdmin(@Nullable String value) {
@Nullable String newValue = value;
if (Objects.equals(this.admin, newValue)) return this;
return new SlackUser(
this.id,
this.username,
this.profile,
this.deleted,
this.color,
newValue,
this.owner,
this.teamId,
this.realName,
this.primaryOwner,
this.restricted,
this.ultraRestricted,
this.bot,
this.appUser,
this.timezone,
this.timezoneLabel,
this.timezoneOffset,
this.rawUpdated,
this.locale);
}
/**
* Copy the current immutable object by setting an optional value for the {@link SlackUserIF#isAdmin() admin} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for admin
* @return A modified copy of {@code this} object
*/
public final SlackUser withAdmin(Optional optional) {
@Nullable String value = optional.orElse(null);
if (Objects.equals(this.admin, value)) return this;
return new SlackUser(
this.id,
this.username,
this.profile,
this.deleted,
this.color,
value,
this.owner,
this.teamId,
this.realName,
this.primaryOwner,
this.restricted,
this.ultraRestricted,
this.bot,
this.appUser,
this.timezone,
this.timezoneLabel,
this.timezoneOffset,
this.rawUpdated,
this.locale);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link SlackUserIF#isOwner() owner} attribute.
* @param value The value for owner, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final SlackUser withOwner(@Nullable String value) {
@Nullable String newValue = value;
if (Objects.equals(this.owner, newValue)) return this;
return new SlackUser(
this.id,
this.username,
this.profile,
this.deleted,
this.color,
this.admin,
newValue,
this.teamId,
this.realName,
this.primaryOwner,
this.restricted,
this.ultraRestricted,
this.bot,
this.appUser,
this.timezone,
this.timezoneLabel,
this.timezoneOffset,
this.rawUpdated,
this.locale);
}
/**
* Copy the current immutable object by setting an optional value for the {@link SlackUserIF#isOwner() owner} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for owner
* @return A modified copy of {@code this} object
*/
public final SlackUser withOwner(Optional optional) {
@Nullable String value = optional.orElse(null);
if (Objects.equals(this.owner, value)) return this;
return new SlackUser(
this.id,
this.username,
this.profile,
this.deleted,
this.color,
this.admin,
value,
this.teamId,
this.realName,
this.primaryOwner,
this.restricted,
this.ultraRestricted,
this.bot,
this.appUser,
this.timezone,
this.timezoneLabel,
this.timezoneOffset,
this.rawUpdated,
this.locale);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link SlackUserIF#getTeamId() teamId} attribute.
* @param value The value for teamId, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final SlackUser withTeamId(@Nullable String value) {
@Nullable String newValue = value;
if (Objects.equals(this.teamId, newValue)) return this;
return new SlackUser(
this.id,
this.username,
this.profile,
this.deleted,
this.color,
this.admin,
this.owner,
newValue,
this.realName,
this.primaryOwner,
this.restricted,
this.ultraRestricted,
this.bot,
this.appUser,
this.timezone,
this.timezoneLabel,
this.timezoneOffset,
this.rawUpdated,
this.locale);
}
/**
* Copy the current immutable object by setting an optional value for the {@link SlackUserIF#getTeamId() teamId} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for teamId
* @return A modified copy of {@code this} object
*/
public final SlackUser withTeamId(Optional optional) {
@Nullable String value = optional.orElse(null);
if (Objects.equals(this.teamId, value)) return this;
return new SlackUser(
this.id,
this.username,
this.profile,
this.deleted,
this.color,
this.admin,
this.owner,
value,
this.realName,
this.primaryOwner,
this.restricted,
this.ultraRestricted,
this.bot,
this.appUser,
this.timezone,
this.timezoneLabel,
this.timezoneOffset,
this.rawUpdated,
this.locale);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link SlackUserIF#getRealName() realName} attribute.
* @param value The value for realName, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final SlackUser withRealName(@Nullable String value) {
@Nullable String newValue = value;
if (Objects.equals(this.realName, newValue)) return this;
return new SlackUser(
this.id,
this.username,
this.profile,
this.deleted,
this.color,
this.admin,
this.owner,
this.teamId,
newValue,
this.primaryOwner,
this.restricted,
this.ultraRestricted,
this.bot,
this.appUser,
this.timezone,
this.timezoneLabel,
this.timezoneOffset,
this.rawUpdated,
this.locale);
}
/**
* Copy the current immutable object by setting an optional value for the {@link SlackUserIF#getRealName() realName} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for realName
* @return A modified copy of {@code this} object
*/
public final SlackUser withRealName(Optional optional) {
@Nullable String value = optional.orElse(null);
if (Objects.equals(this.realName, value)) return this;
return new SlackUser(
this.id,
this.username,
this.profile,
this.deleted,
this.color,
this.admin,
this.owner,
this.teamId,
value,
this.primaryOwner,
this.restricted,
this.ultraRestricted,
this.bot,
this.appUser,
this.timezone,
this.timezoneLabel,
this.timezoneOffset,
this.rawUpdated,
this.locale);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link SlackUserIF#isPrimaryOwner() primaryOwner} attribute.
* @param value The value for primaryOwner, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final SlackUser withPrimaryOwner(@Nullable Boolean value) {
@Nullable Boolean newValue = value;
if (Objects.equals(this.primaryOwner, newValue)) return this;
return new SlackUser(
this.id,
this.username,
this.profile,
this.deleted,
this.color,
this.admin,
this.owner,
this.teamId,
this.realName,
newValue,
this.restricted,
this.ultraRestricted,
this.bot,
this.appUser,
this.timezone,
this.timezoneLabel,
this.timezoneOffset,
this.rawUpdated,
this.locale);
}
/**
* Copy the current immutable object by setting an optional value for the {@link SlackUserIF#isPrimaryOwner() primaryOwner} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for primaryOwner
* @return A modified copy of {@code this} object
*/
public final SlackUser withPrimaryOwner(Optional optional) {
@Nullable Boolean value = optional.orElse(null);
if (Objects.equals(this.primaryOwner, value)) return this;
return new SlackUser(
this.id,
this.username,
this.profile,
this.deleted,
this.color,
this.admin,
this.owner,
this.teamId,
this.realName,
value,
this.restricted,
this.ultraRestricted,
this.bot,
this.appUser,
this.timezone,
this.timezoneLabel,
this.timezoneOffset,
this.rawUpdated,
this.locale);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link SlackUserIF#isRestricted() restricted} attribute.
* @param value The value for restricted, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final SlackUser withRestricted(@Nullable Boolean value) {
@Nullable Boolean newValue = value;
if (Objects.equals(this.restricted, newValue)) return this;
return new SlackUser(
this.id,
this.username,
this.profile,
this.deleted,
this.color,
this.admin,
this.owner,
this.teamId,
this.realName,
this.primaryOwner,
newValue,
this.ultraRestricted,
this.bot,
this.appUser,
this.timezone,
this.timezoneLabel,
this.timezoneOffset,
this.rawUpdated,
this.locale);
}
/**
* Copy the current immutable object by setting an optional value for the {@link SlackUserIF#isRestricted() restricted} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for restricted
* @return A modified copy of {@code this} object
*/
public final SlackUser withRestricted(Optional optional) {
@Nullable Boolean value = optional.orElse(null);
if (Objects.equals(this.restricted, value)) return this;
return new SlackUser(
this.id,
this.username,
this.profile,
this.deleted,
this.color,
this.admin,
this.owner,
this.teamId,
this.realName,
this.primaryOwner,
value,
this.ultraRestricted,
this.bot,
this.appUser,
this.timezone,
this.timezoneLabel,
this.timezoneOffset,
this.rawUpdated,
this.locale);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link SlackUserIF#isUltraRestricted() ultraRestricted} attribute.
* @param value The value for ultraRestricted, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final SlackUser withUltraRestricted(@Nullable Boolean value) {
@Nullable Boolean newValue = value;
if (Objects.equals(this.ultraRestricted, newValue)) return this;
return new SlackUser(
this.id,
this.username,
this.profile,
this.deleted,
this.color,
this.admin,
this.owner,
this.teamId,
this.realName,
this.primaryOwner,
this.restricted,
newValue,
this.bot,
this.appUser,
this.timezone,
this.timezoneLabel,
this.timezoneOffset,
this.rawUpdated,
this.locale);
}
/**
* Copy the current immutable object by setting an optional value for the {@link SlackUserIF#isUltraRestricted() ultraRestricted} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for ultraRestricted
* @return A modified copy of {@code this} object
*/
public final SlackUser withUltraRestricted(Optional optional) {
@Nullable Boolean value = optional.orElse(null);
if (Objects.equals(this.ultraRestricted, value)) return this;
return new SlackUser(
this.id,
this.username,
this.profile,
this.deleted,
this.color,
this.admin,
this.owner,
this.teamId,
this.realName,
this.primaryOwner,
this.restricted,
value,
this.bot,
this.appUser,
this.timezone,
this.timezoneLabel,
this.timezoneOffset,
this.rawUpdated,
this.locale);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link SlackUserIF#isBot() bot} attribute.
* @param value The value for bot, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final SlackUser withBot(@Nullable Boolean value) {
@Nullable Boolean newValue = value;
if (Objects.equals(this.bot, newValue)) return this;
return new SlackUser(
this.id,
this.username,
this.profile,
this.deleted,
this.color,
this.admin,
this.owner,
this.teamId,
this.realName,
this.primaryOwner,
this.restricted,
this.ultraRestricted,
newValue,
this.appUser,
this.timezone,
this.timezoneLabel,
this.timezoneOffset,
this.rawUpdated,
this.locale);
}
/**
* Copy the current immutable object by setting an optional value for the {@link SlackUserIF#isBot() bot} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for bot
* @return A modified copy of {@code this} object
*/
public final SlackUser withBot(Optional optional) {
@Nullable Boolean value = optional.orElse(null);
if (Objects.equals(this.bot, value)) return this;
return new SlackUser(
this.id,
this.username,
this.profile,
this.deleted,
this.color,
this.admin,
this.owner,
this.teamId,
this.realName,
this.primaryOwner,
this.restricted,
this.ultraRestricted,
value,
this.appUser,
this.timezone,
this.timezoneLabel,
this.timezoneOffset,
this.rawUpdated,
this.locale);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link SlackUserIF#isAppUser() appUser} attribute.
* @param value The value for appUser, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final SlackUser withAppUser(@Nullable Boolean value) {
@Nullable Boolean newValue = value;
if (Objects.equals(this.appUser, newValue)) return this;
return new SlackUser(
this.id,
this.username,
this.profile,
this.deleted,
this.color,
this.admin,
this.owner,
this.teamId,
this.realName,
this.primaryOwner,
this.restricted,
this.ultraRestricted,
this.bot,
newValue,
this.timezone,
this.timezoneLabel,
this.timezoneOffset,
this.rawUpdated,
this.locale);
}
/**
* Copy the current immutable object by setting an optional value for the {@link SlackUserIF#isAppUser() appUser} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for appUser
* @return A modified copy of {@code this} object
*/
public final SlackUser withAppUser(Optional optional) {
@Nullable Boolean value = optional.orElse(null);
if (Objects.equals(this.appUser, value)) return this;
return new SlackUser(
this.id,
this.username,
this.profile,
this.deleted,
this.color,
this.admin,
this.owner,
this.teamId,
this.realName,
this.primaryOwner,
this.restricted,
this.ultraRestricted,
this.bot,
value,
this.timezone,
this.timezoneLabel,
this.timezoneOffset,
this.rawUpdated,
this.locale);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link SlackUserIF#getTimezone() timezone} attribute.
* @param value The value for timezone, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final SlackUser withTimezone(@Nullable String value) {
@Nullable String newValue = value;
if (Objects.equals(this.timezone, newValue)) return this;
return new SlackUser(
this.id,
this.username,
this.profile,
this.deleted,
this.color,
this.admin,
this.owner,
this.teamId,
this.realName,
this.primaryOwner,
this.restricted,
this.ultraRestricted,
this.bot,
this.appUser,
newValue,
this.timezoneLabel,
this.timezoneOffset,
this.rawUpdated,
this.locale);
}
/**
* Copy the current immutable object by setting an optional value for the {@link SlackUserIF#getTimezone() timezone} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for timezone
* @return A modified copy of {@code this} object
*/
public final SlackUser withTimezone(Optional optional) {
@Nullable String value = optional.orElse(null);
if (Objects.equals(this.timezone, value)) return this;
return new SlackUser(
this.id,
this.username,
this.profile,
this.deleted,
this.color,
this.admin,
this.owner,
this.teamId,
this.realName,
this.primaryOwner,
this.restricted,
this.ultraRestricted,
this.bot,
this.appUser,
value,
this.timezoneLabel,
this.timezoneOffset,
this.rawUpdated,
this.locale);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link SlackUserIF#getTimezoneLabel() timezoneLabel} attribute.
* @param value The value for timezoneLabel, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final SlackUser withTimezoneLabel(@Nullable String value) {
@Nullable String newValue = value;
if (Objects.equals(this.timezoneLabel, newValue)) return this;
return new SlackUser(
this.id,
this.username,
this.profile,
this.deleted,
this.color,
this.admin,
this.owner,
this.teamId,
this.realName,
this.primaryOwner,
this.restricted,
this.ultraRestricted,
this.bot,
this.appUser,
this.timezone,
newValue,
this.timezoneOffset,
this.rawUpdated,
this.locale);
}
/**
* Copy the current immutable object by setting an optional value for the {@link SlackUserIF#getTimezoneLabel() timezoneLabel} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for timezoneLabel
* @return A modified copy of {@code this} object
*/
public final SlackUser withTimezoneLabel(Optional optional) {
@Nullable String value = optional.orElse(null);
if (Objects.equals(this.timezoneLabel, value)) return this;
return new SlackUser(
this.id,
this.username,
this.profile,
this.deleted,
this.color,
this.admin,
this.owner,
this.teamId,
this.realName,
this.primaryOwner,
this.restricted,
this.ultraRestricted,
this.bot,
this.appUser,
this.timezone,
value,
this.timezoneOffset,
this.rawUpdated,
this.locale);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link SlackUserIF#getTimezoneOffset() timezoneOffset} attribute.
* @param value The value for timezoneOffset, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final SlackUser withTimezoneOffset(@Nullable String value) {
@Nullable String newValue = value;
if (Objects.equals(this.timezoneOffset, newValue)) return this;
return new SlackUser(
this.id,
this.username,
this.profile,
this.deleted,
this.color,
this.admin,
this.owner,
this.teamId,
this.realName,
this.primaryOwner,
this.restricted,
this.ultraRestricted,
this.bot,
this.appUser,
this.timezone,
this.timezoneLabel,
newValue,
this.rawUpdated,
this.locale);
}
/**
* Copy the current immutable object by setting an optional value for the {@link SlackUserIF#getTimezoneOffset() timezoneOffset} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for timezoneOffset
* @return A modified copy of {@code this} object
*/
public final SlackUser withTimezoneOffset(Optional optional) {
@Nullable String value = optional.orElse(null);
if (Objects.equals(this.timezoneOffset, value)) return this;
return new SlackUser(
this.id,
this.username,
this.profile,
this.deleted,
this.color,
this.admin,
this.owner,
this.teamId,
this.realName,
this.primaryOwner,
this.restricted,
this.ultraRestricted,
this.bot,
this.appUser,
this.timezone,
this.timezoneLabel,
value,
this.rawUpdated,
this.locale);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link SlackUserIF#getRawUpdated() rawUpdated} attribute.
* @param value The value for rawUpdated, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final SlackUser withRawUpdated(@Nullable Integer value) {
@Nullable Integer newValue = value;
if (Objects.equals(this.rawUpdated, newValue)) return this;
return new SlackUser(
this.id,
this.username,
this.profile,
this.deleted,
this.color,
this.admin,
this.owner,
this.teamId,
this.realName,
this.primaryOwner,
this.restricted,
this.ultraRestricted,
this.bot,
this.appUser,
this.timezone,
this.timezoneLabel,
this.timezoneOffset,
newValue,
this.locale);
}
/**
* Copy the current immutable object by setting an optional value for the {@link SlackUserIF#getRawUpdated() rawUpdated} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for rawUpdated
* @return A modified copy of {@code this} object
*/
public final SlackUser withRawUpdated(Optional optional) {
@Nullable Integer value = optional.orElse(null);
if (Objects.equals(this.rawUpdated, value)) return this;
return new SlackUser(
this.id,
this.username,
this.profile,
this.deleted,
this.color,
this.admin,
this.owner,
this.teamId,
this.realName,
this.primaryOwner,
this.restricted,
this.ultraRestricted,
this.bot,
this.appUser,
this.timezone,
this.timezoneLabel,
this.timezoneOffset,
value,
this.locale);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link SlackUserIF#getLocale() locale} attribute.
* @param value The value for locale, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return A modified copy of {@code this} object
*/
public final SlackUser withLocale(@Nullable String value) {
@Nullable String newValue = value;
if (Objects.equals(this.locale, newValue)) return this;
return new SlackUser(
this.id,
this.username,
this.profile,
this.deleted,
this.color,
this.admin,
this.owner,
this.teamId,
this.realName,
this.primaryOwner,
this.restricted,
this.ultraRestricted,
this.bot,
this.appUser,
this.timezone,
this.timezoneLabel,
this.timezoneOffset,
this.rawUpdated,
newValue);
}
/**
* Copy the current immutable object by setting an optional value for the {@link SlackUserIF#getLocale() locale} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for locale
* @return A modified copy of {@code this} object
*/
public final SlackUser withLocale(Optional optional) {
@Nullable String value = optional.orElse(null);
if (Objects.equals(this.locale, value)) return this;
return new SlackUser(
this.id,
this.username,
this.profile,
this.deleted,
this.color,
this.admin,
this.owner,
this.teamId,
this.realName,
this.primaryOwner,
this.restricted,
this.ultraRestricted,
this.bot,
this.appUser,
this.timezone,
this.timezoneLabel,
this.timezoneOffset,
this.rawUpdated,
value);
}
/**
* This instance is equal to all instances of {@code SlackUser} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(@Nullable Object another) {
if (this == another) return true;
return another instanceof SlackUser
&& equalTo(0, (SlackUser) another);
}
private boolean equalTo(int synthetic, SlackUser another) {
return id.equals(another.id)
&& Objects.equals(username, another.username)
&& Objects.equals(profile, another.profile)
&& Objects.equals(deleted, another.deleted)
&& Objects.equals(color, another.color)
&& Objects.equals(admin, another.admin)
&& Objects.equals(owner, another.owner)
&& Objects.equals(teamId, another.teamId)
&& Objects.equals(realName, another.realName)
&& Objects.equals(primaryOwner, another.primaryOwner)
&& Objects.equals(restricted, another.restricted)
&& Objects.equals(ultraRestricted, another.ultraRestricted)
&& Objects.equals(bot, another.bot)
&& Objects.equals(appUser, another.appUser)
&& Objects.equals(timezone, another.timezone)
&& Objects.equals(timezoneLabel, another.timezoneLabel)
&& Objects.equals(timezoneOffset, another.timezoneOffset)
&& Objects.equals(rawUpdated, another.rawUpdated)
&& Objects.equals(locale, another.locale)
&& updatedAt.equals(another.updatedAt);
}
/**
* Computes a hash code from attributes: {@code id}, {@code username}, {@code profile}, {@code deleted}, {@code color}, {@code admin}, {@code owner}, {@code teamId}, {@code realName}, {@code primaryOwner}, {@code restricted}, {@code ultraRestricted}, {@code bot}, {@code appUser}, {@code timezone}, {@code timezoneLabel}, {@code timezoneOffset}, {@code rawUpdated}, {@code locale}, {@code updatedAt}.
* @return hashCode value
*/
@Override
public int hashCode() {
int h = 5381;
h += (h << 5) + id.hashCode();
h += (h << 5) + Objects.hashCode(username);
h += (h << 5) + Objects.hashCode(profile);
h += (h << 5) + Objects.hashCode(deleted);
h += (h << 5) + Objects.hashCode(color);
h += (h << 5) + Objects.hashCode(admin);
h += (h << 5) + Objects.hashCode(owner);
h += (h << 5) + Objects.hashCode(teamId);
h += (h << 5) + Objects.hashCode(realName);
h += (h << 5) + Objects.hashCode(primaryOwner);
h += (h << 5) + Objects.hashCode(restricted);
h += (h << 5) + Objects.hashCode(ultraRestricted);
h += (h << 5) + Objects.hashCode(bot);
h += (h << 5) + Objects.hashCode(appUser);
h += (h << 5) + Objects.hashCode(timezone);
h += (h << 5) + Objects.hashCode(timezoneLabel);
h += (h << 5) + Objects.hashCode(timezoneOffset);
h += (h << 5) + Objects.hashCode(rawUpdated);
h += (h << 5) + Objects.hashCode(locale);
h += (h << 5) + updatedAt.hashCode();
return h;
}
/**
* Prints the immutable value {@code SlackUser} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
StringBuilder builder = new StringBuilder("SlackUser{");
builder.append("id=").append(id);
if (username != null) {
builder.append(", ");
builder.append("username=").append(username);
}
if (profile != null) {
builder.append(", ");
builder.append("profile=").append(profile);
}
if (deleted != null) {
builder.append(", ");
builder.append("deleted=").append(deleted);
}
if (color != null) {
builder.append(", ");
builder.append("color=").append(color);
}
if (admin != null) {
builder.append(", ");
builder.append("admin=").append(admin);
}
if (owner != null) {
builder.append(", ");
builder.append("owner=").append(owner);
}
if (teamId != null) {
builder.append(", ");
builder.append("teamId=").append(teamId);
}
if (realName != null) {
builder.append(", ");
builder.append("realName=").append(realName);
}
if (primaryOwner != null) {
builder.append(", ");
builder.append("primaryOwner=").append(primaryOwner);
}
if (restricted != null) {
builder.append(", ");
builder.append("restricted=").append(restricted);
}
if (ultraRestricted != null) {
builder.append(", ");
builder.append("ultraRestricted=").append(ultraRestricted);
}
if (bot != null) {
builder.append(", ");
builder.append("bot=").append(bot);
}
if (appUser != null) {
builder.append(", ");
builder.append("appUser=").append(appUser);
}
if (timezone != null) {
builder.append(", ");
builder.append("timezone=").append(timezone);
}
if (timezoneLabel != null) {
builder.append(", ");
builder.append("timezoneLabel=").append(timezoneLabel);
}
if (timezoneOffset != null) {
builder.append(", ");
builder.append("timezoneOffset=").append(timezoneOffset);
}
if (rawUpdated != null) {
builder.append(", ");
builder.append("rawUpdated=").append(rawUpdated);
}
if (locale != null) {
builder.append(", ");
builder.append("locale=").append(locale);
}
builder.append(", ");
builder.append("updatedAt=").append(updatedAt);
return builder.append("}").toString();
}
/**
* Utility type used to correctly read immutable object from JSON representation.
* @deprecated Do not use this type directly, it exists only for the Jackson-binding infrastructure
*/
@Generated(from = "SlackUserIF", generator = "Immutables")
@Deprecated
@JsonAutoDetect(fieldVisibility = JsonAutoDetect.Visibility.NONE)
static final class Json implements SlackUserIF {
@Nullable String id;
@Nullable Optional username = Optional.empty();
@Nullable Optional profile = Optional.empty();
@Nullable Optional deleted = Optional.empty();
@Nullable Optional color = Optional.empty();
@Nullable Optional admin = Optional.empty();
@Nullable Optional owner = Optional.empty();
@Nullable Optional teamId = Optional.empty();
@Nullable Optional realName = Optional.empty();
@Nullable Optional primaryOwner = Optional.empty();
@Nullable Optional restricted = Optional.empty();
@Nullable Optional ultraRestricted = Optional.empty();
@Nullable Optional bot = Optional.empty();
@Nullable Optional appUser = Optional.empty();
@Nullable Optional timezone = Optional.empty();
@Nullable Optional timezoneLabel = Optional.empty();
@Nullable Optional timezoneOffset = Optional.empty();
@Nullable Optional rawUpdated = Optional.empty();
@Nullable Optional locale = Optional.empty();
@JsonProperty
public void setId(String id) {
this.id = id;
}
@JsonProperty("name")
public void setUsername(Optional username) {
this.username = username;
}
@JsonProperty
public void setProfile(Optional profile) {
this.profile = profile;
}
@JsonProperty("deleted")
public void setDeleted(Optional deleted) {
this.deleted = deleted;
}
@JsonProperty
public void setColor(Optional color) {
this.color = color;
}
@JsonProperty
public void setAdmin(Optional admin) {
this.admin = admin;
}
@JsonProperty
public void setOwner(Optional owner) {
this.owner = owner;
}
@JsonProperty
public void setTeamId(Optional teamId) {
this.teamId = teamId;
}
@JsonProperty
public void setRealName(Optional realName) {
this.realName = realName;
}
@JsonSetter("primaryOwner")
@JsonProperty("is_primary_owner")
public void setPrimaryOwner(Optional primaryOwner) {
this.primaryOwner = primaryOwner;
}
@JsonSetter("restricted")
@JsonProperty("is_restricted")
public void setRestricted(Optional restricted) {
this.restricted = restricted;
}
@JsonSetter("ultra_restricted")
@JsonProperty("is_ultra_restricted")
public void setUltraRestricted(Optional ultraRestricted) {
this.ultraRestricted = ultraRestricted;
}
@JsonProperty("is_bot")
public void setBot(Optional bot) {
this.bot = bot;
}
@JsonSetter("app_user")
@JsonProperty("is_app_user")
public void setAppUser(Optional appUser) {
this.appUser = appUser;
}
@JsonProperty("tz")
public void setTimezone(Optional timezone) {
this.timezone = timezone;
}
@JsonProperty("tz_label")
public void setTimezoneLabel(Optional timezoneLabel) {
this.timezoneLabel = timezoneLabel;
}
@JsonProperty("tz_offset")
public void setTimezoneOffset(Optional timezoneOffset) {
this.timezoneOffset = timezoneOffset;
}
@JsonProperty("updated")
public void setRawUpdated(Optional rawUpdated) {
this.rawUpdated = rawUpdated;
}
@JsonProperty
public void setLocale(Optional locale) {
this.locale = locale;
}
@Override
public String getId() { throw new UnsupportedOperationException(); }
@Override
public Optional getUsername() { throw new UnsupportedOperationException(); }
@Override
public Optional getProfile() { throw new UnsupportedOperationException(); }
@Override
public Optional isDeleted() { throw new UnsupportedOperationException(); }
@Override
public Optional getColor() { throw new UnsupportedOperationException(); }
@Override
public Optional isAdmin() { throw new UnsupportedOperationException(); }
@Override
public Optional isOwner() { throw new UnsupportedOperationException(); }
@Override
public Optional getTeamId() { throw new UnsupportedOperationException(); }
@Override
public Optional getRealName() { throw new UnsupportedOperationException(); }
@Override
public Optional isPrimaryOwner() { throw new UnsupportedOperationException(); }
@Override
public Optional isRestricted() { throw new UnsupportedOperationException(); }
@Override
public Optional isUltraRestricted() { throw new UnsupportedOperationException(); }
@Override
public Optional isBot() { throw new UnsupportedOperationException(); }
@Override
public Optional isAppUser() { throw new UnsupportedOperationException(); }
@Override
public Optional getTimezone() { throw new UnsupportedOperationException(); }
@Override
public Optional getTimezoneLabel() { throw new UnsupportedOperationException(); }
@Override
public Optional getTimezoneOffset() { throw new UnsupportedOperationException(); }
@Override
public Optional getRawUpdated() { throw new UnsupportedOperationException(); }
@Override
public Optional getLocale() { throw new UnsupportedOperationException(); }
@JsonIgnore
@Override
public Optional getUpdatedAt() { throw new UnsupportedOperationException(); }
}
/**
* @param json A JSON-bindable data structure
* @return An immutable value type
* @deprecated Do not use this method directly, it exists only for the Jackson-binding infrastructure
*/
@Deprecated
@JsonCreator(mode = JsonCreator.Mode.DELEGATING)
static SlackUser fromJson(Json json) {
SlackUser.Builder builder = SlackUser.builder();
if (json.id != null) {
builder.setId(json.id);
}
if (json.username != null) {
builder.setUsername(json.username);
}
if (json.profile != null) {
builder.setProfile(json.profile);
}
if (json.deleted != null) {
builder.setDeleted(json.deleted);
}
if (json.color != null) {
builder.setColor(json.color);
}
if (json.admin != null) {
builder.setAdmin(json.admin);
}
if (json.owner != null) {
builder.setOwner(json.owner);
}
if (json.teamId != null) {
builder.setTeamId(json.teamId);
}
if (json.realName != null) {
builder.setRealName(json.realName);
}
if (json.primaryOwner != null) {
builder.setPrimaryOwner(json.primaryOwner);
}
if (json.restricted != null) {
builder.setRestricted(json.restricted);
}
if (json.ultraRestricted != null) {
builder.setUltraRestricted(json.ultraRestricted);
}
if (json.bot != null) {
builder.setBot(json.bot);
}
if (json.appUser != null) {
builder.setAppUser(json.appUser);
}
if (json.timezone != null) {
builder.setTimezone(json.timezone);
}
if (json.timezoneLabel != null) {
builder.setTimezoneLabel(json.timezoneLabel);
}
if (json.timezoneOffset != null) {
builder.setTimezoneOffset(json.timezoneOffset);
}
if (json.rawUpdated != null) {
builder.setRawUpdated(json.rawUpdated);
}
if (json.locale != null) {
builder.setLocale(json.locale);
}
return builder.build();
}
/**
* Creates an immutable copy of a {@link SlackUserIF} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable SlackUser instance
*/
public static SlackUser copyOf(SlackUserIF instance) {
if (instance instanceof SlackUser) {
return (SlackUser) instance;
}
return SlackUser.builder()
.from(instance)
.build();
}
/**
* Creates a builder for {@link SlackUser SlackUser}.
*
* SlackUser.builder()
* .setId(String) // required {@link SlackUserIF#getId() id}
* .setUsername(String) // optional {@link SlackUserIF#getUsername() username}
* .setProfile(UserProfile) // optional {@link SlackUserIF#getProfile() profile}
* .setDeleted(Boolean) // optional {@link SlackUserIF#isDeleted() deleted}
* .setColor(String) // optional {@link SlackUserIF#getColor() color}
* .setAdmin(String) // optional {@link SlackUserIF#isAdmin() admin}
* .setOwner(String) // optional {@link SlackUserIF#isOwner() owner}
* .setTeamId(String) // optional {@link SlackUserIF#getTeamId() teamId}
* .setRealName(String) // optional {@link SlackUserIF#getRealName() realName}
* .setPrimaryOwner(Boolean) // optional {@link SlackUserIF#isPrimaryOwner() primaryOwner}
* .setRestricted(Boolean) // optional {@link SlackUserIF#isRestricted() restricted}
* .setUltraRestricted(Boolean) // optional {@link SlackUserIF#isUltraRestricted() ultraRestricted}
* .setBot(Boolean) // optional {@link SlackUserIF#isBot() bot}
* .setAppUser(Boolean) // optional {@link SlackUserIF#isAppUser() appUser}
* .setTimezone(String) // optional {@link SlackUserIF#getTimezone() timezone}
* .setTimezoneLabel(String) // optional {@link SlackUserIF#getTimezoneLabel() timezoneLabel}
* .setTimezoneOffset(String) // optional {@link SlackUserIF#getTimezoneOffset() timezoneOffset}
* .setRawUpdated(Integer) // optional {@link SlackUserIF#getRawUpdated() rawUpdated}
* .setLocale(String) // optional {@link SlackUserIF#getLocale() locale}
* .build();
*
* @return A new SlackUser builder
*/
public static SlackUser.Builder builder() {
return new SlackUser.Builder();
}
/**
* Builds instances of type {@link SlackUser SlackUser}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
@Generated(from = "SlackUserIF", generator = "Immutables")
@NotThreadSafe
public static final class Builder {
private static final long INIT_BIT_ID = 0x1L;
private long initBits = 0x1L;
private @Nullable String id;
private @Nullable String username;
private @Nullable UserProfile profile;
private @Nullable Boolean deleted;
private @Nullable String color;
private @Nullable String admin;
private @Nullable String owner;
private @Nullable String teamId;
private @Nullable String realName;
private @Nullable Boolean primaryOwner;
private @Nullable Boolean restricted;
private @Nullable Boolean ultraRestricted;
private @Nullable Boolean bot;
private @Nullable Boolean appUser;
private @Nullable String timezone;
private @Nullable String timezoneLabel;
private @Nullable String timezoneOffset;
private @Nullable Integer rawUpdated;
private @Nullable String locale;
private Builder() {
}
/**
* Fill a builder with attribute values from the provided {@code com.hubspot.slack.client.models.users.SlackUserIF} instance.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(SlackUserIF instance) {
Objects.requireNonNull(instance, "instance");
from((short) 0, (Object) instance);
return this;
}
/**
* Fill a builder with attribute values from the provided {@code com.hubspot.slack.client.models.users.SlackUserCore} instance.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(SlackUserCore instance) {
Objects.requireNonNull(instance, "instance");
from((short) 0, (Object) instance);
return this;
}
private void from(short _unused, Object object) {
long bits = 0;
if (object instanceof SlackUserIF) {
SlackUserIF instance = (SlackUserIF) object;
Optional ownerOptional = instance.isOwner();
if (ownerOptional.isPresent()) {
setOwner(ownerOptional);
}
Optional appUserOptional = instance.isAppUser();
if (appUserOptional.isPresent()) {
setAppUser(appUserOptional);
}
Optional timezoneLabelOptional = instance.getTimezoneLabel();
if (timezoneLabelOptional.isPresent()) {
setTimezoneLabel(timezoneLabelOptional);
}
Optional colorOptional = instance.getColor();
if (colorOptional.isPresent()) {
setColor(colorOptional);
}
Optional botOptional = instance.isBot();
if (botOptional.isPresent()) {
setBot(botOptional);
}
Optional timezoneOptional = instance.getTimezone();
if (timezoneOptional.isPresent()) {
setTimezone(timezoneOptional);
}
Optional profileOptional = instance.getProfile();
if (profileOptional.isPresent()) {
setProfile(profileOptional);
}
Optional primaryOwnerOptional = instance.isPrimaryOwner();
if (primaryOwnerOptional.isPresent()) {
setPrimaryOwner(primaryOwnerOptional);
}
Optional adminOptional = instance.isAdmin();
if (adminOptional.isPresent()) {
setAdmin(adminOptional);
}
Optional localeOptional = instance.getLocale();
if (localeOptional.isPresent()) {
setLocale(localeOptional);
}
Optional realNameOptional = instance.getRealName();
if (realNameOptional.isPresent()) {
setRealName(realNameOptional);
}
Optional deletedOptional = instance.isDeleted();
if (deletedOptional.isPresent()) {
setDeleted(deletedOptional);
}
Optional timezoneOffsetOptional = instance.getTimezoneOffset();
if (timezoneOffsetOptional.isPresent()) {
setTimezoneOffset(timezoneOffsetOptional);
}
Optional restrictedOptional = instance.isRestricted();
if (restrictedOptional.isPresent()) {
setRestricted(restrictedOptional);
}
Optional teamIdOptional = instance.getTeamId();
if (teamIdOptional.isPresent()) {
setTeamId(teamIdOptional);
}
if ((bits & 0x1L) == 0) {
this.setId(instance.getId());
bits |= 0x1L;
}
Optional ultraRestrictedOptional = instance.isUltraRestricted();
if (ultraRestrictedOptional.isPresent()) {
setUltraRestricted(ultraRestrictedOptional);
}
Optional rawUpdatedOptional = instance.getRawUpdated();
if (rawUpdatedOptional.isPresent()) {
setRawUpdated(rawUpdatedOptional);
}
if ((bits & 0x2L) == 0) {
Optional usernameOptional = instance.getUsername();
if (usernameOptional.isPresent()) {
setUsername(usernameOptional);
}
bits |= 0x2L;
}
}
if (object instanceof SlackUserCore) {
SlackUserCore instance = (SlackUserCore) object;
if ((bits & 0x1L) == 0) {
this.setId(instance.getId());
bits |= 0x1L;
}
if ((bits & 0x2L) == 0) {
Optional usernameOptional = instance.getUsername();
if (usernameOptional.isPresent()) {
setUsername(usernameOptional);
}
bits |= 0x2L;
}
}
}
/**
* Initializes the value for the {@link SlackUserIF#getId() id} attribute.
* @param id The value for id
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setId(String id) {
this.id = Objects.requireNonNull(id, "id");
initBits &= ~INIT_BIT_ID;
return this;
}
/**
* Initializes the optional value {@link SlackUserIF#getUsername() username} to username.
* @param username The value for username, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
public final Builder setUsername(@Nullable String username) {
this.username = username;
return this;
}
/**
* Initializes the optional value {@link SlackUserIF#getUsername() username} to username.
* @param username The value for username
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setUsername(Optional username) {
this.username = username.orElse(null);
return this;
}
/**
* Initializes the optional value {@link SlackUserIF#getProfile() profile} to profile.
* @param profile The value for profile, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
public final Builder setProfile(@Nullable UserProfile profile) {
this.profile = profile;
return this;
}
/**
* Initializes the optional value {@link SlackUserIF#getProfile() profile} to profile.
* @param profile The value for profile
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setProfile(Optional extends UserProfile> profile) {
this.profile = profile.orElse(null);
return this;
}
/**
* Initializes the optional value {@link SlackUserIF#isDeleted() deleted} to deleted.
* @param deleted The value for deleted, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
public final Builder setDeleted(@Nullable Boolean deleted) {
this.deleted = deleted;
return this;
}
/**
* Initializes the optional value {@link SlackUserIF#isDeleted() deleted} to deleted.
* @param deleted The value for deleted
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setDeleted(Optional deleted) {
this.deleted = deleted.orElse(null);
return this;
}
/**
* Initializes the optional value {@link SlackUserIF#getColor() color} to color.
* @param color The value for color, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
public final Builder setColor(@Nullable String color) {
this.color = color;
return this;
}
/**
* Initializes the optional value {@link SlackUserIF#getColor() color} to color.
* @param color The value for color
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setColor(Optional color) {
this.color = color.orElse(null);
return this;
}
/**
* Initializes the optional value {@link SlackUserIF#isAdmin() admin} to admin.
* @param admin The value for admin, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
public final Builder setAdmin(@Nullable String admin) {
this.admin = admin;
return this;
}
/**
* Initializes the optional value {@link SlackUserIF#isAdmin() admin} to admin.
* @param admin The value for admin
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setAdmin(Optional admin) {
this.admin = admin.orElse(null);
return this;
}
/**
* Initializes the optional value {@link SlackUserIF#isOwner() owner} to owner.
* @param owner The value for owner, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
public final Builder setOwner(@Nullable String owner) {
this.owner = owner;
return this;
}
/**
* Initializes the optional value {@link SlackUserIF#isOwner() owner} to owner.
* @param owner The value for owner
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setOwner(Optional owner) {
this.owner = owner.orElse(null);
return this;
}
/**
* Initializes the optional value {@link SlackUserIF#getTeamId() teamId} to teamId.
* @param teamId The value for teamId, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
public final Builder setTeamId(@Nullable String teamId) {
this.teamId = teamId;
return this;
}
/**
* Initializes the optional value {@link SlackUserIF#getTeamId() teamId} to teamId.
* @param teamId The value for teamId
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setTeamId(Optional teamId) {
this.teamId = teamId.orElse(null);
return this;
}
/**
* Initializes the optional value {@link SlackUserIF#getRealName() realName} to realName.
* @param realName The value for realName, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
public final Builder setRealName(@Nullable String realName) {
this.realName = realName;
return this;
}
/**
* Initializes the optional value {@link SlackUserIF#getRealName() realName} to realName.
* @param realName The value for realName
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setRealName(Optional realName) {
this.realName = realName.orElse(null);
return this;
}
/**
* Initializes the optional value {@link SlackUserIF#isPrimaryOwner() primaryOwner} to primaryOwner.
* @param primaryOwner The value for primaryOwner, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
public final Builder setPrimaryOwner(@Nullable Boolean primaryOwner) {
this.primaryOwner = primaryOwner;
return this;
}
/**
* Initializes the optional value {@link SlackUserIF#isPrimaryOwner() primaryOwner} to primaryOwner.
* @param primaryOwner The value for primaryOwner
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setPrimaryOwner(Optional primaryOwner) {
this.primaryOwner = primaryOwner.orElse(null);
return this;
}
/**
* Initializes the optional value {@link SlackUserIF#isRestricted() restricted} to restricted.
* @param restricted The value for restricted, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
public final Builder setRestricted(@Nullable Boolean restricted) {
this.restricted = restricted;
return this;
}
/**
* Initializes the optional value {@link SlackUserIF#isRestricted() restricted} to restricted.
* @param restricted The value for restricted
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setRestricted(Optional restricted) {
this.restricted = restricted.orElse(null);
return this;
}
/**
* Initializes the optional value {@link SlackUserIF#isUltraRestricted() ultraRestricted} to ultraRestricted.
* @param ultraRestricted The value for ultraRestricted, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
public final Builder setUltraRestricted(@Nullable Boolean ultraRestricted) {
this.ultraRestricted = ultraRestricted;
return this;
}
/**
* Initializes the optional value {@link SlackUserIF#isUltraRestricted() ultraRestricted} to ultraRestricted.
* @param ultraRestricted The value for ultraRestricted
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setUltraRestricted(Optional ultraRestricted) {
this.ultraRestricted = ultraRestricted.orElse(null);
return this;
}
/**
* Initializes the optional value {@link SlackUserIF#isBot() bot} to bot.
* @param bot The value for bot, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
public final Builder setBot(@Nullable Boolean bot) {
this.bot = bot;
return this;
}
/**
* Initializes the optional value {@link SlackUserIF#isBot() bot} to bot.
* @param bot The value for bot
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setBot(Optional bot) {
this.bot = bot.orElse(null);
return this;
}
/**
* Initializes the optional value {@link SlackUserIF#isAppUser() appUser} to appUser.
* @param appUser The value for appUser, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
public final Builder setAppUser(@Nullable Boolean appUser) {
this.appUser = appUser;
return this;
}
/**
* Initializes the optional value {@link SlackUserIF#isAppUser() appUser} to appUser.
* @param appUser The value for appUser
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setAppUser(Optional appUser) {
this.appUser = appUser.orElse(null);
return this;
}
/**
* Initializes the optional value {@link SlackUserIF#getTimezone() timezone} to timezone.
* @param timezone The value for timezone, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
public final Builder setTimezone(@Nullable String timezone) {
this.timezone = timezone;
return this;
}
/**
* Initializes the optional value {@link SlackUserIF#getTimezone() timezone} to timezone.
* @param timezone The value for timezone
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setTimezone(Optional timezone) {
this.timezone = timezone.orElse(null);
return this;
}
/**
* Initializes the optional value {@link SlackUserIF#getTimezoneLabel() timezoneLabel} to timezoneLabel.
* @param timezoneLabel The value for timezoneLabel, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
public final Builder setTimezoneLabel(@Nullable String timezoneLabel) {
this.timezoneLabel = timezoneLabel;
return this;
}
/**
* Initializes the optional value {@link SlackUserIF#getTimezoneLabel() timezoneLabel} to timezoneLabel.
* @param timezoneLabel The value for timezoneLabel
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setTimezoneLabel(Optional timezoneLabel) {
this.timezoneLabel = timezoneLabel.orElse(null);
return this;
}
/**
* Initializes the optional value {@link SlackUserIF#getTimezoneOffset() timezoneOffset} to timezoneOffset.
* @param timezoneOffset The value for timezoneOffset, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
public final Builder setTimezoneOffset(@Nullable String timezoneOffset) {
this.timezoneOffset = timezoneOffset;
return this;
}
/**
* Initializes the optional value {@link SlackUserIF#getTimezoneOffset() timezoneOffset} to timezoneOffset.
* @param timezoneOffset The value for timezoneOffset
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setTimezoneOffset(Optional timezoneOffset) {
this.timezoneOffset = timezoneOffset.orElse(null);
return this;
}
/**
* Initializes the optional value {@link SlackUserIF#getRawUpdated() rawUpdated} to rawUpdated.
* @param rawUpdated The value for rawUpdated, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
public final Builder setRawUpdated(@Nullable Integer rawUpdated) {
this.rawUpdated = rawUpdated;
return this;
}
/**
* Initializes the optional value {@link SlackUserIF#getRawUpdated() rawUpdated} to rawUpdated.
* @param rawUpdated The value for rawUpdated
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setRawUpdated(Optional rawUpdated) {
this.rawUpdated = rawUpdated.orElse(null);
return this;
}
/**
* Initializes the optional value {@link SlackUserIF#getLocale() locale} to locale.
* @param locale The value for locale, {@code null} is accepted as {@code java.util.Optional.empty()}
* @return {@code this} builder for chained invocation
*/
public final Builder setLocale(@Nullable String locale) {
this.locale = locale;
return this;
}
/**
* Initializes the optional value {@link SlackUserIF#getLocale() locale} to locale.
* @param locale The value for locale
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setLocale(Optional locale) {
this.locale = locale.orElse(null);
return this;
}
/**
* Builds a new {@link SlackUser SlackUser}.
* @return An immutable instance of SlackUser
* @throws com.hubspot.immutables.validation.InvalidImmutableStateException if any required attributes are missing
*/
public SlackUser build() {
checkRequiredAttributes();
return new SlackUser(
id,
username,
profile,
deleted,
color,
admin,
owner,
teamId,
realName,
primaryOwner,
restricted,
ultraRestricted,
bot,
appUser,
timezone,
timezoneLabel,
timezoneOffset,
rawUpdated,
locale);
}
private boolean idIsSet() {
return (initBits & INIT_BIT_ID) == 0;
}
private void checkRequiredAttributes() {
if (initBits != 0) {
throw new InvalidImmutableStateException(formatRequiredAttributesMessage());
}
}
private String formatRequiredAttributesMessage() {
List attributes = new ArrayList<>();
if (!idIsSet()) attributes.add("id");
return "Cannot build SlackUser, some of required attributes are not set " + attributes;
}
}
}