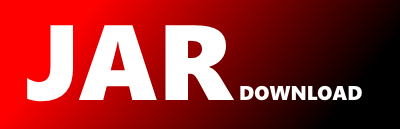
com.hubspot.singularity.executor.models.RunnerContext Maven / Gradle / Ivy
package com.hubspot.singularity.executor.models;
import com.google.common.base.Optional;
/**
* Handlebars context for generating the runner.sh file.
*/
public class RunnerContext {
private final String cmd;
private final String taskAppDirectory;
private final String logDir;
private final String user;
private final String logFile;
private final String taskId;
private final Optional maxTaskThreads;
private final boolean shouldChangeUser;
private final Integer maxOpenFiles;
private final String switchUserCommand;
public RunnerContext(String cmd, String taskAppDirectory, String logDir, String user, String logFile, String taskId, Optional maxTaskThreads, boolean shouldChangeUser, Integer maxOpenFiles, String switchUserCommand) {
this.cmd = cmd;
this.taskAppDirectory = taskAppDirectory;
this.logDir = logDir;
this.user = user;
this.logFile = logFile;
this.taskId = taskId;
this.maxTaskThreads = maxTaskThreads;
this.shouldChangeUser = shouldChangeUser;
this.maxOpenFiles = maxOpenFiles;
this.switchUserCommand = switchUserCommand;
}
public String getCmd() {
return cmd;
}
public String getTaskAppDirectory() {
return taskAppDirectory;
}
public String getLogDir() {
return logDir;
}
public String getUser() {
return user;
}
public String getLogFile() {
return logFile;
}
public String getTaskId() {
return taskId;
}
public Optional getMaxTaskThreads() {
return maxTaskThreads;
}
public boolean isShouldChangeUser() {
return shouldChangeUser;
}
public Integer getMaxOpenFiles() {
return maxOpenFiles;
}
public String getSwitchUserCommand() {
return switchUserCommand;
}
@Override
public String toString() {
return "RunnerContext[" +
"cmd='" + cmd + '\'' +
", taskAppDirectory='" + taskAppDirectory + '\'' +
", logDir='" + logDir + '\'' +
", user='" + user + '\'' +
", logFile='" + logFile + '\'' +
", taskId='" + taskId + '\'' +
", maxTaskThreads=" + maxTaskThreads +
", shouldChangeUser=" + shouldChangeUser +
", switchUserCommand=" + switchUserCommand +
']';
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy