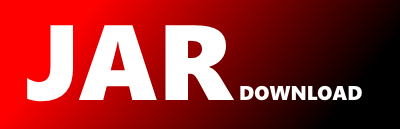
com.hubspot.singularity.runner.base.config.SingularityRunnerBaseLogging Maven / Gradle / Ivy
package com.hubspot.singularity.runner.base.config;
import java.util.Map.Entry;
import java.util.Properties;
import org.slf4j.LoggerFactory;
import ch.qos.logback.classic.Logger;
import ch.qos.logback.classic.LoggerContext;
import ch.qos.logback.classic.encoder.PatternLayoutEncoder;
import ch.qos.logback.classic.spi.ILoggingEvent;
import ch.qos.logback.core.FileAppender;
import com.google.inject.Inject;
import com.google.inject.name.Named;
public class SingularityRunnerBaseLogging {
private final String rootLogPath;
private final String loggingPattern;
private final Properties properties;
@Inject
public SingularityRunnerBaseLogging(@Named(SingularityRunnerBaseConfigurationLoader.ROOT_LOG_PATH) String rootLogPath, @Named(SingularityRunnerBaseConfigurationLoader.LOGGING_PATTERN) String loggingPattern, Properties properties) {
this.rootLogPath = rootLogPath;
this.loggingPattern = loggingPattern;
this.properties = properties;
Logger rootLogger = configureRootLogger();
printProperties(rootLogger);
}
public void printProperties(Logger rootLogger) {
rootLogger.debug("Loaded {} properties", properties.size());
for (Entry
© 2015 - 2025 Weber Informatics LLC | Privacy Policy