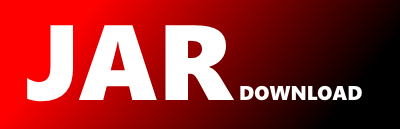
com.caseystella.analytics.util.JSONUtil Maven / Gradle / Ivy
/**
* Copyright (C) 2016 Hurence ([email protected])
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.caseystella.analytics.util;
import com.fasterxml.jackson.core.JsonProcessingException;
import com.fasterxml.jackson.core.type.TypeReference;
import com.fasterxml.jackson.databind.ObjectMapper;
import java.io.*;
import java.nio.charset.Charset;
import java.util.Map;
public enum JSONUtil {
INSTANCE;
static ThreadLocal MAPPER = new ThreadLocal() {
/**
* Returns the current thread's "initial value" for this
* thread-local variable. This method will be invoked the first
* time a thread accesses the variable with the {@link #get}
* method, unless the thread previously invoked the {@link #set}
* method, in which case the initialValue method will not
* be invoked for the thread. Normally, this method is invoked at
* most once per thread, but it may be invoked again in case of
* subsequent invocations of {@link #remove} followed by {@link #get}.
*
* This implementation simply returns null; if the
* programmer desires thread-local variables to have an initial
* value other than null, ThreadLocal must be
* subclassed, and this method overridden. Typically, an
* anonymous inner class will be used.
*
* @return the initial value for this thread-local
*/
@Override
protected ObjectMapper initialValue() {
return new ObjectMapper();
}
};
public T load(File f, Class clazz) throws IOException {
return load(new FileInputStream(f), clazz);
}
public T load(InputStream is, Class clazz) throws IOException {
T ret = MAPPER.get().readValue(is, clazz);
return ret;
}
public T load(String s, Charset c, Class clazz) throws IOException {
return load( new ByteArrayInputStream(s.getBytes(c)), clazz);
}
public T load(String s, Class clazz) throws IOException {
return load( s, Charset.defaultCharset(), clazz);
}
public T load(InputStream is, TypeReference reference) throws IOException {
T ret = MAPPER.get().readValue(is, reference);
return ret;
}
public T load(String s, Charset c, TypeReference reference) throws IOException {
return load( new ByteArrayInputStream(s.getBytes(c)), reference);
}
public T load(String s, TypeReference reference) throws IOException {
return load( s, Charset.defaultCharset(), reference);
}
public String toJSON(Object bean ) throws JsonProcessingException {
return MAPPER.get().writerWithDefaultPrettyPrinter().writeValueAsString(bean);
}
}