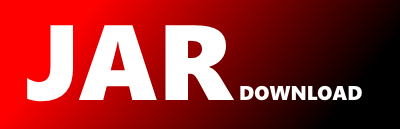
com.hwaipy.quantity.Quantity Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of Quantity Show documentation
Show all versions of Quantity Show documentation
For calculation of quantities and units.
The newest version!
package com.hwaipy.quantity;
import java.util.Objects;
/**
*
* @author Hwaipy
*/
public class Quantity {
private final double value;
private final Unit unit;
public Quantity(double value, Unit unit) {
this.value = value;
this.unit = unit == null ? Units.DIMENSIONLESS : unit;
}
public double getValue() {
return value;
}
public double getValueInSI() {
return value * unit.getFactor();
}
public Unit getUnit() {
return unit;
}
public double getValue(Unit unit) throws UnitDimensionMissmatchException {
if (this.unit.equalsDimension(unit)) {
return this.value * this.unit.getFactor() / unit.getFactor();
}
else {
throw new UnitDimensionMissmatchException(this.unit.toDimensionString() + " can not be converted to " + unit.toDimensionString());
}
}
public double getValue(String unit) throws UnitDimensionMissmatchException {
return getValue(Unit.of(unit));
}
public Quantity add(Quantity quantity) throws UnitDimensionMissmatchException {
if (unit.equalsDimension(quantity.getUnit())) {
double value2 = quantity.getValueInSI();
return new Quantity(value + value2 / unit.getFactor(), unit);
}
else {
throw new UnitDimensionMissmatchException(unit.toDimensionString() + "can not add with " + quantity.getUnit().toDimensionString());
}
}
public Quantity minus(Quantity quantity) throws UnitDimensionMissmatchException {
if (unit.equalsDimension(quantity.getUnit())) {
double value2 = quantity.getValueInSI();
return new Quantity(value - value2 / unit.getFactor(), unit);
}
else {
throw new UnitDimensionMissmatchException(unit.toDimensionString() + "can not minus with " + quantity.getUnit().toDimensionString());
}
}
public Quantity times(double d) {
return new Quantity(value * d, unit);
}
public Quantity times(Quantity quantity) {
return new Quantity(value * quantity.getValue(), unit.times(quantity.getUnit()));
}
public Quantity divide(double d) {
return new Quantity(value / d, unit);
}
public Quantity divide(Quantity quantity) {
return new Quantity(value / quantity.getValue(), unit.divide(quantity.getUnit()));
}
public Quantity power(int power) {
return new Quantity(Math.pow(value, power), Units.DIMENSIONLESS.times(unit, power));
}
public void assertDimention(String dimention) {
unit.assertDimension(dimention);
}
public boolean isDimentionless() {
return unit.isDimentionless();
}
public static Quantity of(String quantityString) throws QuantityParseException {
return new QuantityParser(quantityString).parse();
}
@Override
public String toString() {
return isDimentionless() ? "" + getValueInSI() : getValueInSI() + unit.toDimensionString();
}
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (obj == null || this.getClass() != obj.getClass()) {
return false;
}
Quantity quantity = (Quantity) obj;
return equals(quantity);
}
public boolean equals(Quantity quantity) {
return getUnit().equalsDimension(quantity.getUnit())
&& getValueInSI() == quantity.getValueInSI();
}
public boolean equals(Quantity quantity, double relative) {
return equals(quantity, quantity.times(relative));
}
public boolean equals(Quantity quantity, Quantity absolute) {
if (!getUnit().equalsDimension(quantity.getUnit()) || !getUnit().equalsDimension(absolute.getUnit())) {
return false;
}
double v = getValueInSI();
double q = quantity.getValueInSI();
double a = Math.abs(absolute.getValueInSI());
return v <= q + a && v >= q - a;
}
@Override
public int hashCode() {
int hash = 7;
hash = 23 * hash + (int) (Double.doubleToLongBits(this.value) ^ (Double.doubleToLongBits(this.value) >>> 32));
hash = 23 * hash + Objects.hashCode(this.unit);
return hash;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy