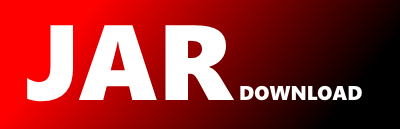
org.apache.http.message.BasicLineParser Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of httpcore Show documentation
Show all versions of httpcore Show documentation
Apache HttpComponents Core (blocking I/O) for jdk 1.5
/*
* ====================================================================
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
* ====================================================================
*
* This software consists of voluntary contributions made by many
* individuals on behalf of the Apache Software Foundation. For more
* information on the Apache Software Foundation, please see
* .
*
*/
package org.apache.http.message;
import org.apache.http.Header;
import org.apache.http.HttpVersion;
import org.apache.http.ParseException;
import org.apache.http.ProtocolVersion;
import org.apache.http.RequestLine;
import org.apache.http.StatusLine;
import org.apache.http.annotation.Immutable;
import org.apache.http.protocol.HTTP;
import org.apache.http.util.Args;
import org.apache.http.util.CharArrayBuffer;
/**
* Basic parser for lines in the head section of an HTTP message.
* There are individual methods for parsing a request line, a
* status line, or a header line.
* The lines to parse are passed in memory, the parser does not depend
* on any specific IO mechanism.
* Instances of this class are stateless and thread-safe.
* Derived classes MUST maintain these properties.
*
*
* Note: This class was created by refactoring parsing code located in
* various other classes. The author tags from those other classes have
* been replicated here, although the association with the parsing code
* taken from there has not been traced.
*
*
* @since 4.0
*/
@Immutable
public class BasicLineParser implements LineParser {
/**
* A default instance of this class, for use as default or fallback.
* Note that {@link BasicLineParser} is not a singleton, there can
* be many instances of the class itself and of derived classes.
* The instance here provides non-customized, default behavior.
*
* @deprecated (4.3) use {@link #INSTANCE}
*/
@Deprecated
public final static BasicLineParser DEFAULT = new BasicLineParser();
public final static BasicLineParser INSTANCE = new BasicLineParser();
/**
* A version of the protocol to parse.
* The version is typically not relevant, but the protocol name.
*/
protected final ProtocolVersion protocol;
/**
* Creates a new line parser for the given HTTP-like protocol.
*
* @param proto a version of the protocol to parse, or
* {@code null} for HTTP. The actual version
* is not relevant, only the protocol name.
*/
public BasicLineParser(final ProtocolVersion proto) {
this.protocol = proto != null? proto : HttpVersion.HTTP_1_1;
}
/**
* Creates a new line parser for HTTP.
*/
public BasicLineParser() {
this(null);
}
public static
ProtocolVersion parseProtocolVersion(final String value,
final LineParser parser) throws ParseException {
Args.notNull(value, "Value");
final CharArrayBuffer buffer = new CharArrayBuffer(value.length());
buffer.append(value);
final ParserCursor cursor = new ParserCursor(0, value.length());
return (parser != null ? parser : BasicLineParser.INSTANCE)
.parseProtocolVersion(buffer, cursor);
}
// non-javadoc, see interface LineParser
@Override
public ProtocolVersion parseProtocolVersion(final CharArrayBuffer buffer,
final ParserCursor cursor) throws ParseException {
Args.notNull(buffer, "Char array buffer");
Args.notNull(cursor, "Parser cursor");
final String protoname = this.protocol.getProtocol();
final int protolength = protoname.length();
final int indexFrom = cursor.getPos();
final int indexTo = cursor.getUpperBound();
skipWhitespace(buffer, cursor);
int i = cursor.getPos();
// long enough for "HTTP/1.1"?
if (i + protolength + 4 > indexTo) {
throw new ParseException
("Not a valid protocol version: " +
buffer.substring(indexFrom, indexTo));
}
// check the protocol name and slash
boolean ok = true;
for (int j=0; ok && (j buffer.length()) {
return false;
}
// just check protocol name and slash, no need to analyse the version
boolean ok = true;
for (int j=0; ok && (j
© 2015 - 2024 Weber Informatics LLC | Privacy Policy