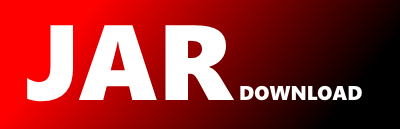
com.hzlinks.swagger.rpc.http.HttpMatch Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of swaggerpc-springboot-starter Show documentation
Show all versions of swaggerpc-springboot-starter Show documentation
swagger for dubbo rpc with spring boot
package com.hzlinks.swagger.rpc.http;
import com.hzlinks.swagger.rpc.reader.NameDiscover;
import io.swagger.annotations.ApiOperation;
import io.swagger.models.HttpMethod;
import io.swagger.util.ReflectionUtils;
import java.lang.reflect.Method;
import java.util.ArrayList;
import java.util.Collections;
import java.util.Comparator;
import java.util.List;
import java.util.Set;
import org.apache.commons.lang3.StringUtils;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class HttpMatch {
private static final Logger logger = LoggerFactory.getLogger(HttpMatch.class);
private final Class> interfaceClass;
private final Class> refClass;
public HttpMatch(Class> interfaceClass, Class> refClass) {
this.interfaceClass = interfaceClass;
this.refClass = refClass;
}
public Method[] findInterfaceMethods(String methodName) {
Method[] methods = interfaceClass.getMethods();
List ret = new ArrayList();
for (Method method : methods) {
if (method.getName().equals(methodName)) {
ret.add(method);
}
}
return ret.toArray(new Method[]{});
}
public Method[] findRefMethods(Method[] interfaceMethods, String operationId,
String requestMethod) {
List ret = new ArrayList();
for (Method method : interfaceMethods) {
Method m;
try {
m = refClass.getMethod(method.getName(), method.getParameterTypes());
final ApiOperation apiOperation = ReflectionUtils.getAnnotation(m,
ApiOperation.class);
String nickname = null == apiOperation ? null : apiOperation.nickname();
if (operationId != null) {
if (!operationId.equals(nickname)) {
continue;
}
} else {
if (StringUtils.isNotBlank(nickname)) {
continue;
}
}
if (requestMethod != null) {
String httpMethod = null == apiOperation ? null : apiOperation.httpMethod();
if (StringUtils.isNotBlank(httpMethod) && !requestMethod.equals(httpMethod)) {
continue;
}
if (StringUtils.isBlank(httpMethod)
&& !requestMethod.equalsIgnoreCase(HttpMethod.POST.name())) {
continue;
}
}
ret.add(m);
} catch (NoSuchMethodException e) {
logger.error("NoSuchMethodException", e);
} catch (SecurityException e) {
logger.error("SecurityException", e);
}
}
return ret.toArray(new Method[]{});
}
public Method matchRefMethod(Method[] refMethods, String methodName, Set keySet) {
if (refMethods.length == 0) {
return null;
}
if (refMethods.length == 1) {
return refMethods[0];
}
List rateMethods = new ArrayList();
for (Method method : refMethods) {
String[] parameterNames = NameDiscover.parameterNameDiscover
.getParameterNames(method);
if (parameterNames == null) {
return method;
}
float correctRate = 0.0f;
int hit = 0;
int error = 0;
for (String paramName : parameterNames) {
if (keySet.contains(paramName)) {
hit++;
} else {
error++;
}
}
correctRate = error / (float) hit;
rateMethods.add(new RateMethod(method, (int) correctRate * 100));
}
if (rateMethods.isEmpty()) {
return null;
}
Collections.sort(rateMethods, new Comparator() {
@Override
public int compare(RateMethod o1, RateMethod o2) {
return o2.getRate() - o1.getRate();
}
});
return rateMethods.get(0).getMethod();
}
class RateMethod {
private Method method;
private int rate;
public RateMethod(Method method, int rate) {
this.method = method;
this.rate = rate;
}
public Method getMethod() {
return method;
}
public void setMethod(Method method) {
this.method = method;
}
public int getRate() {
return rate;
}
public void setRate(int rate) {
this.rate = rate;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy