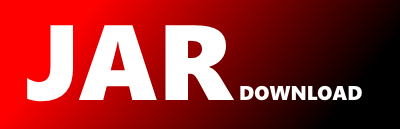
com.hzlinks.swagger.rpc.http.ReferenceManager Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of swaggerpc-springboot-starter Show documentation
Show all versions of swaggerpc-springboot-starter Show documentation
swagger for dubbo rpc with spring boot
package com.hzlinks.swagger.rpc.http;
import java.util.Collection;
import java.util.HashSet;
import java.util.Map;
import java.util.Map.Entry;
import java.util.Set;
import java.util.concurrent.ConcurrentHashMap;
import org.apache.dubbo.config.ApplicationConfig;
import org.apache.dubbo.config.ReferenceConfig;
import org.apache.dubbo.config.spring.ServiceBean;
import org.apache.dubbo.config.spring.extension.SpringExtensionFactory;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.context.ApplicationContext;
public class ReferenceManager {
private static final Logger logger = LoggerFactory.getLogger(ReferenceManager.class);
@SuppressWarnings("rawtypes")
private static Collection services;
private static final Map, Object> interfaceMapProxy = new ConcurrentHashMap, Object>();
private static final Map, Object> interfaceMapRef = new ConcurrentHashMap, Object>();
private static ReferenceManager instance;
private static ApplicationConfig application;
private ReferenceManager() {
}
@SuppressWarnings({"rawtypes", "unchecked"})
public synchronized static ReferenceManager getInstance() {
if (null != instance) {
return instance;
}
instance = new ReferenceManager();
services = new HashSet();
try {
Set contexts = SpringExtensionFactory.getContexts();
for (ApplicationContext context : contexts) {
services.addAll(context.getBeansOfType(ServiceBean.class).values());
}
} catch (Exception e) {
logger.error("Get All Dubbo Service Error", e);
return instance;
}
for (ServiceBean> bean : services) {
interfaceMapRef.putIfAbsent(bean.getInterfaceClass(), bean.getRef());
}
//
if (!services.isEmpty()) {
ServiceBean> bean = services.toArray(new ServiceBean[]{})[0];
application = bean.getApplication();
}
return instance;
}
public Object getProxy(String interfaceClass) {
Set, Object>> entrySet = interfaceMapProxy.entrySet();
for (Entry, Object> entry : entrySet) {
if (entry.getKey().getName().equals(interfaceClass)) {
return entry.getValue();
}
}
for (ServiceBean> service : services) {
if (interfaceClass.equals(service.getInterfaceClass().getName())) {
ReferenceConfig
© 2015 - 2025 Weber Informatics LLC | Privacy Policy