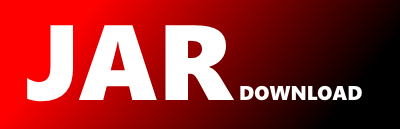
com.ibasco.agql.protocols.valve.dota2.webapi.pojos.Dota2MatchDetails Maven / Gradle / Ivy
Show all versions of agql-dota2-webapi Show documentation
/*
* Copyright (c) 2022 Asynchronous Game Query Library
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.ibasco.agql.protocols.valve.dota2.webapi.pojos;
import com.google.gson.annotations.SerializedName;
import org.apache.commons.lang3.builder.ReflectionToStringBuilder;
import org.apache.commons.lang3.builder.ToStringStyle;
import java.util.ArrayList;
import java.util.List;
/**
* Dota2MatchDetails class.
*
* @author Rafael Luis Ibasco
*/
public class Dota2MatchDetails {
@SerializedName("players")
private List players = new ArrayList<>();
@SerializedName("radiant_win")
private boolean radiantWin;
@SerializedName("duration")
private int duration;
@SerializedName("pre_game_duration")
private int preGameDuration;
@SerializedName("start_time")
private int startTime;
@SerializedName("match_id")
private long matchId;
@SerializedName("match_seq_num")
private long matchSeqNum;
@SerializedName("tower_status_radiant")
private int towerStatusRadiant;
@SerializedName("tower_status_dire")
private int towerStatusDire;
@SerializedName("barracks_status_radiant")
private int barracksStatusRadiant;
@SerializedName("barracks_status_dire")
private int barracksStatusDire;
@SerializedName("cluster")
private int cluster;
@SerializedName("first_blood_time")
private int firstBloodTime;
@SerializedName("lobby_type")
private int lobbyType;
@SerializedName("human_players")
private int humanPlayers;
@SerializedName("leagueId")
private int leagueId;
@SerializedName("positive_votes")
private int positiveVotes;
@SerializedName("negative_votes")
private int negativeVotes;
@SerializedName("game_mode")
private int gameMode;
@SerializedName("flags")
private int flags;
@SerializedName("engine")
private int engine;
@SerializedName("radiant_score")
private int radiantScore;
@SerializedName("dire_score")
private int direScore;
/**
* Getter for the field players
.
*
* @return The players
*/
public List getPlayers() {
return players;
}
/**
* Setter for the field players
.
*
* @param players
* The players
*/
public void setPlayers(List players) {
this.players = players;
}
/**
* isRadiantWin.
*
* @return The radiantWin
*/
public boolean isRadiantWin() {
return radiantWin;
}
/**
* Setter for the field radiantWin
.
*
* @param radiantWin
* The radiant_win
*/
public void setRadiantWin(boolean radiantWin) {
this.radiantWin = radiantWin;
}
/**
* Getter for the field duration
.
*
* @return The duration
*/
public int getDuration() {
return duration;
}
/**
* Setter for the field duration
.
*
* @param duration
* The duration
*/
public void setDuration(int duration) {
this.duration = duration;
}
/**
* Getter for the field preGameDuration
.
*
* @return The preGameDuration
*/
public int getPreGameDuration() {
return preGameDuration;
}
/**
* Setter for the field preGameDuration
.
*
* @param preGameDuration
* The pre_game_duration
*/
public void setPreGameDuration(int preGameDuration) {
this.preGameDuration = preGameDuration;
}
/**
* Getter for the field startTime
.
*
* @return The startTime
*/
public int getStartTime() {
return startTime;
}
/**
* Setter for the field startTime
.
*
* @param startTime
* The start_time
*/
public void setStartTime(int startTime) {
this.startTime = startTime;
}
/**
* Getter for the field matchId
.
*
* @return The matchId
*/
public long getMatchId() {
return matchId;
}
/**
* Setter for the field matchId
.
*
* @param matchId
* The match_id
*/
public void setMatchId(long matchId) {
this.matchId = matchId;
}
/**
* Getter for the field matchSeqNum
.
*
* @return The matchSeqNum
*/
public long getMatchSeqNum() {
return matchSeqNum;
}
/**
* Setter for the field matchSeqNum
.
*
* @param matchSeqNum
* The match_seq_num
*/
public void setMatchSeqNum(long matchSeqNum) {
this.matchSeqNum = matchSeqNum;
}
/**
* Getter for the field towerStatusRadiant
.
*
* @return The towerStatusRadiant
*/
public int getTowerStatusRadiant() {
return towerStatusRadiant;
}
/**
* Setter for the field towerStatusRadiant
.
*
* @param towerStatusRadiant
* The tower_status_radiant
*/
public void setTowerStatusRadiant(int towerStatusRadiant) {
this.towerStatusRadiant = towerStatusRadiant;
}
/**
* Getter for the field towerStatusDire
.
*
* @return The towerStatusDire
*/
public int getTowerStatusDire() {
return towerStatusDire;
}
/**
* Setter for the field towerStatusDire
.
*
* @param towerStatusDire
* The tower_status_dire
*/
public void setTowerStatusDire(int towerStatusDire) {
this.towerStatusDire = towerStatusDire;
}
/**
* Getter for the field barracksStatusRadiant
.
*
* @return The barracksStatusRadiant
*/
public int getBarracksStatusRadiant() {
return barracksStatusRadiant;
}
/**
* Setter for the field barracksStatusRadiant
.
*
* @param barracksStatusRadiant
* The barracks_status_radiant
*/
public void setBarracksStatusRadiant(int barracksStatusRadiant) {
this.barracksStatusRadiant = barracksStatusRadiant;
}
/**
* Getter for the field barracksStatusDire
.
*
* @return The barracksStatusDire
*/
public int getBarracksStatusDire() {
return barracksStatusDire;
}
/**
* Setter for the field barracksStatusDire
.
*
* @param barracksStatusDire
* The barracks_status_dire
*/
public void setBarracksStatusDire(int barracksStatusDire) {
this.barracksStatusDire = barracksStatusDire;
}
/**
* Getter for the field cluster
.
*
* @return The cluster
*/
public int getCluster() {
return cluster;
}
/**
* Setter for the field cluster
.
*
* @param cluster
* The cluster
*/
public void setCluster(int cluster) {
this.cluster = cluster;
}
/**
* Getter for the field firstBloodTime
.
*
* @return The firstBloodTime
*/
public int getFirstBloodTime() {
return firstBloodTime;
}
/**
* Setter for the field firstBloodTime
.
*
* @param firstBloodTime
* The first_blood_time
*/
public void setFirstBloodTime(int firstBloodTime) {
this.firstBloodTime = firstBloodTime;
}
/**
* Getter for the field lobbyType
.
*
* @return The lobbyType
*/
public int getLobbyType() {
return lobbyType;
}
/**
* Setter for the field lobbyType
.
*
* @param lobbyType
* The lobby_type
*/
public void setLobbyType(int lobbyType) {
this.lobbyType = lobbyType;
}
/**
* Getter for the field humanPlayers
.
*
* @return The humanPlayers
*/
public int getHumanPlayers() {
return humanPlayers;
}
/**
* Setter for the field humanPlayers
.
*
* @param humanPlayers
* The human_players
*/
public void setHumanPlayers(int humanPlayers) {
this.humanPlayers = humanPlayers;
}
/**
* Getter for the field leagueId
.
*
* @return The leagueId
*/
public int getLeagueId() {
return leagueId;
}
/**
* Setter for the field leagueId
.
*
* @param leagueId
* The leagueId
*/
public void setLeagueId(int leagueId) {
this.leagueId = leagueId;
}
/**
* Getter for the field positiveVotes
.
*
* @return The positiveVotes
*/
public int getPositiveVotes() {
return positiveVotes;
}
/**
* Setter for the field positiveVotes
.
*
* @param positiveVotes
* The positive_votes
*/
public void setPositiveVotes(int positiveVotes) {
this.positiveVotes = positiveVotes;
}
/**
* Getter for the field negativeVotes
.
*
* @return The negativeVotes
*/
public int getNegativeVotes() {
return negativeVotes;
}
/**
* Setter for the field negativeVotes
.
*
* @param negativeVotes
* The negative_votes
*/
public void setNegativeVotes(int negativeVotes) {
this.negativeVotes = negativeVotes;
}
/**
* Getter for the field gameMode
.
*
* @return The gameMode
*/
public int getGameMode() {
return gameMode;
}
/**
* Setter for the field gameMode
.
*
* @param gameMode
* The game_mode
*/
public void setGameMode(int gameMode) {
this.gameMode = gameMode;
}
/**
* Getter for the field flags
.
*
* @return The flags
*/
public int getFlags() {
return flags;
}
/**
* Setter for the field flags
.
*
* @param flags
* The flags
*/
public void setFlags(int flags) {
this.flags = flags;
}
/**
* Getter for the field engine
.
*
* @return The engine
*/
public int getEngine() {
return engine;
}
/**
* Setter for the field engine
.
*
* @param engine
* The engine
*/
public void setEngine(int engine) {
this.engine = engine;
}
/**
* Getter for the field radiantScore
.
*
* @return The radiantScore
*/
public int getRadiantScore() {
return radiantScore;
}
/**
* Setter for the field radiantScore
.
*
* @param radiantScore
* The radiant_score
*/
public void setRadiantScore(int radiantScore) {
this.radiantScore = radiantScore;
}
/**
* Getter for the field direScore
.
*
* @return The direScore
*/
public int getDireScore() {
return direScore;
}
/**
* Setter for the field direScore
.
*
* @param direScore
* The dire_score
*/
public void setDireScore(int direScore) {
this.direScore = direScore;
}
/** {@inheritDoc} */
@Override
public String toString() {
return ReflectionToStringBuilder.toString(this, ToStringStyle.NO_CLASS_NAME_STYLE);
}
}