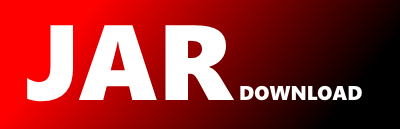
com.ibasco.agql.protocols.valve.dota2.webapi.pojos.Dota2MatchTeamInfo Maven / Gradle / Ivy
Show all versions of agql-dota2-webapi Show documentation
/*
* Copyright (c) 2022 Asynchronous Game Query Library
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.ibasco.agql.protocols.valve.dota2.webapi.pojos;
import com.google.gson.annotations.SerializedName;
import org.apache.commons.lang3.builder.ToStringBuilder;
import org.apache.commons.lang3.builder.ToStringStyle;
import java.util.ArrayList;
import java.util.List;
/**
* Dota2MatchTeamInfo class.
*
* @author Rafael Luis Ibasco
*/
public class Dota2MatchTeamInfo {
@SerializedName("name")
private String name;
@SerializedName("tag")
private String tag;
@SerializedName("time_created")
private long timeCreated;
@SerializedName("calibration_games_remaining")
private int calibrationGamesRemaining;
@SerializedName("logo")
private long logo;
@SerializedName("logo_sponsor")
private long logoSponsor;
@SerializedName("country_code")
private String countryCode;
@SerializedName("url")
private String url;
@SerializedName("games_played")
private int gamesPlayed;
@SerializedName("admin_account_id")
private long adminAccountId;
private List leagueIds = new ArrayList<>();
private List playerAccountIds = new ArrayList<>();
/**
* Getter for the field leagueIds
.
*
* @return a {@link java.util.List} object
*/
public List getLeagueIds() {
return leagueIds;
}
/**
* Setter for the field leagueIds
.
*
* @param leagueIds
* a {@link java.util.List} object
*/
public void setLeagueIds(List leagueIds) {
this.leagueIds = leagueIds;
}
/**
* Getter for the field playerAccountIds
.
*
* @return a {@link java.util.List} object
*/
public List getPlayerAccountIds() {
return playerAccountIds;
}
/**
* Setter for the field playerAccountIds
.
*
* @param playerAccountIds
* a {@link java.util.List} object
*/
public void setPlayerAccountIds(List playerAccountIds) {
this.playerAccountIds = playerAccountIds;
}
/**
* Getter for the field name
.
*
* @return The name
*/
public String getName() {
return name;
}
/**
* Setter for the field name
.
*
* @param name
* The name
*/
public void setName(String name) {
this.name = name;
}
/**
* Getter for the field tag
.
*
* @return The tag
*/
public String getTag() {
return tag;
}
/**
* Setter for the field tag
.
*
* @param tag
* The tag
*/
public void setTag(String tag) {
this.tag = tag;
}
/**
* Getter for the field timeCreated
.
*
* @return The timeCreated
*/
public long getTimeCreated() {
return timeCreated;
}
/**
* Setter for the field timeCreated
.
*
* @param timeCreated
* The time_created
*/
public void setTimeCreated(long timeCreated) {
this.timeCreated = timeCreated;
}
/**
* Getter for the field calibrationGamesRemaining
.
*
* @return The calibrationGamesRemaining
*/
public int getCalibrationGamesRemaining() {
return calibrationGamesRemaining;
}
/**
* Setter for the field calibrationGamesRemaining
.
*
* @param calibrationGamesRemaining
* The calibration_games_remaining
*/
public void setCalibrationGamesRemaining(int calibrationGamesRemaining) {
this.calibrationGamesRemaining = calibrationGamesRemaining;
}
/**
* Getter for the field logo
.
*
* @return The logo
*/
public long getLogo() {
return logo;
}
/**
* Setter for the field logo
.
*
* @param logo
* The logo
*/
public void setLogo(long logo) {
this.logo = logo;
}
/**
* Getter for the field logoSponsor
.
*
* @return The logoSponsor
*/
public long getLogoSponsor() {
return logoSponsor;
}
/**
* Setter for the field logoSponsor
.
*
* @param logoSponsor
* The logo_sponsor
*/
public void setLogoSponsor(long logoSponsor) {
this.logoSponsor = logoSponsor;
}
/**
* Getter for the field countryCode
.
*
* @return The countryCode
*/
public String getCountryCode() {
return countryCode;
}
/**
* Setter for the field countryCode
.
*
* @param countryCode
* The country_code
*/
public void setCountryCode(String countryCode) {
this.countryCode = countryCode;
}
/**
* Getter for the field url
.
*
* @return The url
*/
public String getUrl() {
return url;
}
/**
* Setter for the field url
.
*
* @param url
* The url
*/
public void setUrl(String url) {
this.url = url;
}
/**
* Getter for the field gamesPlayed
.
*
* @return The gamesPlayed
*/
public int getGamesPlayed() {
return gamesPlayed;
}
/**
* Setter for the field gamesPlayed
.
*
* @param gamesPlayed
* The games_played
*/
public void setGamesPlayed(int gamesPlayed) {
this.gamesPlayed = gamesPlayed;
}
/**
* Getter for the field adminAccountId
.
*
* @return The adminAccountId
*/
public long getAdminAccountId() {
return adminAccountId;
}
/**
* Setter for the field adminAccountId
.
*
* @param adminAccountId
* The admin_account_id
*/
public void setAdminAccountId(long adminAccountId) {
this.adminAccountId = adminAccountId;
}
/** {@inheritDoc} */
@Override
public String toString() {
return ToStringBuilder.reflectionToString(this, ToStringStyle.NO_CLASS_NAME_STYLE);
}
}