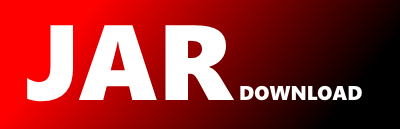
com.ibasco.agql.protocols.valve.dota2.webapi.pojos.Dota2TopLiveGame Maven / Gradle / Ivy
Show all versions of agql-dota2-webapi Show documentation
/*
* Copyright (c) 2022 Asynchronous Game Query Library
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.ibasco.agql.protocols.valve.dota2.webapi.pojos;
import com.google.gson.annotations.SerializedName;
import org.apache.commons.lang3.builder.ToStringBuilder;
import org.apache.commons.lang3.builder.ToStringStyle;
import java.util.ArrayList;
import java.util.List;
/**
* Dota2TopLiveGame class.
*
* @author Rafael Luis Ibasco
*/
public class Dota2TopLiveGame {
@SerializedName("activate_time")
private int activateTime;
@SerializedName("deactivate_time")
private int deactivateTime;
@SerializedName("server_steam_id")
private long serverSteamId;
@SerializedName("lobby_id")
private long lobbyId;
@SerializedName("league_id")
private int leagueId;
@SerializedName("lobby_type")
private int lobbyType;
@SerializedName("game_time")
private int gameTime;
@SerializedName("delay")
private int delay;
@SerializedName("spectators")
private int spectators;
@SerializedName("game_mode")
private int gameMode;
@SerializedName("average_mmr")
private int averageMmr;
@SerializedName("sort_score")
private int sortScore;
@SerializedName("last_update_time")
private int lastUpdateTime;
@SerializedName("radiant_lead")
private int radiantLead;
@SerializedName("radiant_score")
private int radiantScore;
@SerializedName("dire_score")
private int direScore;
@SerializedName("players")
private List players = new ArrayList<>();
@SerializedName("building_state")
private int buildingState;
/**
* Getter for the field activateTime
.
*
* @return The activateTime
*/
public int getActivateTime() {
return activateTime;
}
/**
* Setter for the field activateTime
.
*
* @param activateTime
* The activate_time
*/
public void setActivateTime(int activateTime) {
this.activateTime = activateTime;
}
/**
* Getter for the field deactivateTime
.
*
* @return The deactivateTime
*/
public int getDeactivateTime() {
return deactivateTime;
}
/**
* Setter for the field deactivateTime
.
*
* @param deactivateTime
* The deactivate_time
*/
public void setDeactivateTime(int deactivateTime) {
this.deactivateTime = deactivateTime;
}
/**
* Getter for the field serverSteamId
.
*
* @return The serverSteamId
*/
public long getServerSteamId() {
return serverSteamId;
}
/**
* Setter for the field serverSteamId
.
*
* @param serverSteamId
* The server_steam_id
*/
public void setServerSteamId(long serverSteamId) {
this.serverSteamId = serverSteamId;
}
/**
* Getter for the field lobbyId
.
*
* @return The lobbyId
*/
public long getLobbyId() {
return lobbyId;
}
/**
* Setter for the field lobbyId
.
*
* @param lobbyId
* The lobby_id
*/
public void setLobbyId(long lobbyId) {
this.lobbyId = lobbyId;
}
/**
* Getter for the field leagueId
.
*
* @return The leagueId
*/
public int getLeagueId() {
return leagueId;
}
/**
* Setter for the field leagueId
.
*
* @param leagueId
* The league_id
*/
public void setLeagueId(int leagueId) {
this.leagueId = leagueId;
}
/**
* Getter for the field lobbyType
.
*
* @return The lobbyType
*/
public int getLobbyType() {
return lobbyType;
}
/**
* Setter for the field lobbyType
.
*
* @param lobbyType
* The lobby_type
*/
public void setLobbyType(int lobbyType) {
this.lobbyType = lobbyType;
}
/**
* Getter for the field gameTime
.
*
* @return The gameTime
*/
public int getGameTime() {
return gameTime;
}
/**
* Setter for the field gameTime
.
*
* @param gameTime
* The game_time
*/
public void setGameTime(int gameTime) {
this.gameTime = gameTime;
}
/**
* Getter for the field delay
.
*
* @return The delay
*/
public int getDelay() {
return delay;
}
/**
* Setter for the field delay
.
*
* @param delay
* The delay
*/
public void setDelay(int delay) {
this.delay = delay;
}
/**
* Getter for the field spectators
.
*
* @return The spectators
*/
public int getSpectators() {
return spectators;
}
/**
* Setter for the field spectators
.
*
* @param spectators
* The spectators
*/
public void setSpectators(int spectators) {
this.spectators = spectators;
}
/**
* Getter for the field gameMode
.
*
* @return The gameMode
*/
public int getGameMode() {
return gameMode;
}
/**
* Setter for the field gameMode
.
*
* @param gameMode
* The game_mode
*/
public void setGameMode(int gameMode) {
this.gameMode = gameMode;
}
/**
* Getter for the field averageMmr
.
*
* @return The averageMmr
*/
public int getAverageMmr() {
return averageMmr;
}
/**
* Setter for the field averageMmr
.
*
* @param averageMmr
* The average_mmr
*/
public void setAverageMmr(int averageMmr) {
this.averageMmr = averageMmr;
}
/**
* Getter for the field sortScore
.
*
* @return The sortScore
*/
public int getSortScore() {
return sortScore;
}
/**
* Setter for the field sortScore
.
*
* @param sortScore
* The sort_score
*/
public void setSortScore(int sortScore) {
this.sortScore = sortScore;
}
/**
* Getter for the field lastUpdateTime
.
*
* @return The lastUpdateTime
*/
public int getLastUpdateTime() {
return lastUpdateTime;
}
/**
* Setter for the field lastUpdateTime
.
*
* @param lastUpdateTime
* The last_update_time
*/
public void setLastUpdateTime(int lastUpdateTime) {
this.lastUpdateTime = lastUpdateTime;
}
/**
* Getter for the field radiantLead
.
*
* @return The radiantLead
*/
public int getRadiantLead() {
return radiantLead;
}
/**
* Setter for the field radiantLead
.
*
* @param radiantLead
* The radiant_lead
*/
public void setRadiantLead(int radiantLead) {
this.radiantLead = radiantLead;
}
/**
* Getter for the field radiantScore
.
*
* @return The radiantScore
*/
public int getRadiantScore() {
return radiantScore;
}
/**
* Setter for the field radiantScore
.
*
* @param radiantScore
* The radiant_score
*/
public void setRadiantScore(int radiantScore) {
this.radiantScore = radiantScore;
}
/**
* Getter for the field direScore
.
*
* @return The direScore
*/
public int getDireScore() {
return direScore;
}
/**
* Setter for the field direScore
.
*
* @param direScore
* The dire_score
*/
public void setDireScore(int direScore) {
this.direScore = direScore;
}
/**
* Getter for the field players
.
*
* @return The players
*/
public List getPlayers() {
return players;
}
/**
* Setter for the field players
.
*
* @param players
* The players
*/
public void setPlayers(List players) {
this.players = players;
}
/**
* Getter for the field buildingState
.
*
* @return The buildingState
*/
public int getBuildingState() {
return buildingState;
}
/**
* Setter for the field buildingState
.
*
* @param buildingState
* The building_state
*/
public void setBuildingState(int buildingState) {
this.buildingState = buildingState;
}
/** {@inheritDoc} */
@Override
public String toString() {
return ToStringBuilder.reflectionToString(this, ToStringStyle.NO_CLASS_NAME_STYLE);
}
}