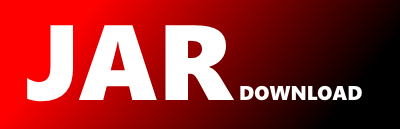
com.ibasco.agql.protocols.valve.source.query.info.SourceServer Maven / Gradle / Ivy
/*
* Copyright (c) 2022 Asynchronous Game Query Library
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.ibasco.agql.protocols.valve.source.query.info;
import org.jetbrains.annotations.ApiStatus;
import java.net.InetSocketAddress;
/**
* SourceServer class.
*
* @author Rafael Luis Ibasco
*/
public class SourceServer {
private String name;
private byte networkVersion;
private String mapName;
private String gameDirectory;
private String gameDescription;
private int appId;
private int numOfPlayers;
private int maxPlayers;
private int numOfBots;
private boolean dedicated;
private String operatingSystem;
private boolean secure;
private String gameVersion;
private long serverId;
private int tvPort;
private String tvName;
private String serverTags;
private long gameId;
private InetSocketAddress address;
private boolean privateServer;
private int gamePort;
private boolean sourceTvProxy;
/**
* Specifies if the server is a private server and password protected
*
* @return {@code true} if the server is a private server and requires a password. otherwise {@code false} if the server is public.
*/
public boolean isPrivateServer() {
return privateServer;
}
/**
* Setter for the field privateServer
.
*
* @param privateServer
* a boolean
*/
public void setPrivateServer(boolean privateServer) {
this.privateServer = privateServer;
}
/**
* Protocol version used by the server
*
* @return A byte representing the protocol version of the server.
*/
public byte getNetworkVersion() {
return networkVersion;
}
/**
* Setter for the field networkVersion
.
*
* @param networkVersion
* a byte
*/
public void setNetworkVersion(byte networkVersion) {
this.networkVersion = networkVersion;
}
/**
* The name of the map currently loaded by the server
*
* @return The map name string
*/
public String getMapName() {
return mapName;
}
/**
* Setter for the field mapName
.
*
* @param mapName
* a {@link java.lang.String} object
*/
public void setMapName(String mapName) {
this.mapName = mapName;
}
/**
* The name of the folder containing the game files
*
* @return a {@link java.lang.String} object
*/
public String getGameDirectory() {
return gameDirectory;
}
/**
* Setter for the field gameDirectory
.
*
* @param gameDirectory
* a {@link java.lang.String} object
*/
public void setGameDirectory(String gameDirectory) {
this.gameDirectory = gameDirectory;
}
/**
* Full name of the game
*
* @return A String representing the full name of the game
*/
public String getGameDescription() {
return gameDescription;
}
/**
* Setter for the field gameDescription
.
*
* @param gameDescription
* a {@link java.lang.String} object
*/
public void setGameDescription(String gameDescription) {
this.gameDescription = gameDescription;
}
/**
* The App ID of the game
*
* @return An integer representing the app id of the game
*/
public int getAppId() {
return appId;
}
/**
* Setter for the field appId
.
*
* @param appId
* a int
*/
public void setAppId(int appId) {
this.appId = appId;
}
/**
* The number of players on the server
*
* @return The number of players currently on the server
*/
public int getNumOfPlayers() {
return numOfPlayers;
}
/**
* Setter for the field numOfPlayers
.
*
* @param numOfPlayers
* a int
*/
public void setNumOfPlayers(int numOfPlayers) {
this.numOfPlayers = numOfPlayers;
}
/**
* The maximum number of players supported by the server
*
* @return The maximum number of players supported by the server
*/
public int getMaxPlayers() {
return maxPlayers;
}
/**
* Setter for the field maxPlayers
.
*
* @param maxPlayers
* a int
*/
public void setMaxPlayers(int maxPlayers) {
this.maxPlayers = maxPlayers;
}
/**
* The number of bots on the server
*
* @return The number of bots on the server
*/
public int getNumOfBots() {
return numOfBots;
}
/**
* Setter for the field numOfBots
.
*
* @param numOfBots
* a int
*/
public void setNumOfBots(int numOfBots) {
this.numOfBots = numOfBots;
}
/**
* Specified whether the server is dedicated or not
*
* @return {@code true} if the server is dedicated, otherwise {@code false} if it is non-dedicated OR a source tv proxy
*
* @see #isSourceTvProxy()
*/
public boolean isDedicated() {
return dedicated;
}
/**
* Setter for the field dedicated
.
*
* @param dedicated
* a boolean
*/
public void setDedicated(boolean dedicated) {
this.dedicated = dedicated;
}
/**
* The operating system of the server (l = linux, w = windows, m = mac)
*
* @return The code representing the operating system of the server
*/
public String getOperatingSystem() {
return operatingSystem;
}
/**
* Setter for the field operatingSystem
.
*
* @param operatingSystem
* a {@link java.lang.String} object
*/
public void setOperatingSystem(String operatingSystem) {
this.operatingSystem = operatingSystem;
}
/**
* Specifies if the server is password
*
* @return a boolean
*
* @deprecated Use {@link #isPrivateServer()}
*/
@Deprecated
@ApiStatus.ScheduledForRemoval
public boolean isPasswordProtected() {
return isPrivateServer();
}
/**
* Setter for the field passwordProtected
.
*
* @param passwordProtected
* a boolean
*
* @deprecated Use {@link #setPrivateServer(boolean)}
*/
@Deprecated
@ApiStatus.ScheduledForRemoval
public void setPasswordProtected(boolean passwordProtected) {
setPrivateServer(passwordProtected);
}
/**
* Specifies whether the server uses VAC
*
* @return {@code true} if the server has VAC enabled.
*/
public boolean isSecure() {
return secure;
}
/**
* Setter for the field secure
.
*
* @param secure
* a boolean
*/
public void setSecure(boolean secure) {
this.secure = secure;
}
/**
* The version of the game installed on the server
*
* @return a {@link java.lang.String} object
*/
public String getGameVersion() {
return gameVersion;
}
/**
* Setter for the field gameVersion
.
*
* @param gameVersion
* a {@link java.lang.String} object
*/
public void setGameVersion(String gameVersion) {
this.gameVersion = gameVersion;
}
/**
* The server's 64-bit steam id
*
* @return The server's 64-bit steam id
*/
public long getServerId() {
return serverId;
}
/**
* Setter for the field serverId
.
*
* @param serverId
* a long
*/
public void setServerId(long serverId) {
this.serverId = serverId;
}
/**
* Source TV Port
*
* @return The Source TV port number
*/
public int getTvPort() {
return tvPort;
}
/**
* The Source TV port
*
* @param tvPort
* The Source TV port number of the server
*/
public void setTvPort(int tvPort) {
this.tvPort = tvPort;
}
/**
* Source TV name
*
* @return The Source TV name
*/
public String getTvName() {
return tvName;
}
/**
* Setter for the field tvName
.
*
* @param tvName
* a {@link java.lang.String} object
*/
public void setTvName(String tvName) {
this.tvName = tvName;
}
/**
* Tags that describe the game according to the server
*
* @return A string of comma-delimited tags
*/
public String getServerTags() {
return serverTags;
}
/**
* Setter for the field serverTags
.
*
* @param serverTags
* a {@link java.lang.String} object
*/
public void setServerTags(String serverTags) {
this.serverTags = serverTags;
}
/**
* The server's 64-bit game id
*
* @return The server's 64-bit game id
*/
public long getGameId() {
return gameId;
}
/**
* Setter for the field gameId
.
*
* @param gameId
* a long
*/
public void setGameId(long gameId) {
this.gameId = gameId;
}
/**
* The name of the server
*
* @return a {@link java.lang.String} object
*/
public String getName() {
return name;
}
/**
* Setter for the field name
.
*
* @param name
* a {@link java.lang.String} object
*/
public void setName(String name) {
this.name = name;
}
/**
* The address of the connection to the server
*
* @return An {@link InetSocketAddress} containing the host and query port of the server
*/
public InetSocketAddress getAddress() {
return address;
}
/**
* Setter for the field address
.
*
* @param address
* a {@link java.net.InetSocketAddress} object
*/
public void setAddress(InetSocketAddress address) {
this.address = address;
}
/**
* The IP address of the server. This is similar to calling {@code getAddress().getHostAddress()}
*
* @return a {@link java.lang.String} representing the host address of the server
*/
public String getHostAddress() {
return address.getAddress().getHostAddress();
}
/**
* The query port of the server. This is similar to calling {@code getAddress().getPort()}.
*
* Note: For most servers, the query and game ports are usually the same. If this is not the case and you need to get the actual game port number of the server, use {@link #getGamePort()}
*
* @return The query port number of the server.
*
* @see #getGamePort()
*/
public int getPort() {
return address.getPort();
}
/**
* The game port of the server
*
* @return The game port number of the server.
*/
public int getGamePort() {
return gamePort;
}
public void setGamePort(int gamePort) {
this.gamePort = gamePort;
}
/**
* Specifies whether the server is a Source TV proxy/relay
*
* @return {@code true} if the server is a Source TV proxy
*/
public boolean isSourceTvProxy() {
return sourceTvProxy;
}
public void setSourceTvProxy(boolean sourceTvProxy) {
this.sourceTvProxy = sourceTvProxy;
}
}