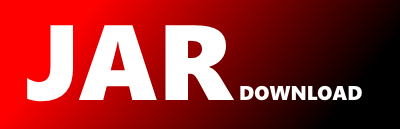
com.ibasco.agql.protocols.valve.steam.webapi.pojos.SteamEconSchema Maven / Gradle / Ivy
/*
* Copyright (c) 2022 Asynchronous Game Query Library
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.ibasco.agql.protocols.valve.steam.webapi.pojos;
import com.google.gson.annotations.SerializedName;
import org.apache.commons.lang3.builder.ReflectionToStringBuilder;
import org.apache.commons.lang3.builder.ToStringStyle;
import java.util.ArrayList;
import java.util.List;
/**
* Contains Information about the items in a supporting game.
*
* @author Rafael Luis Ibasco
*/
public class SteamEconSchema {
/**
* The status of the request, should always be 1.
*/
private int status;
/**
* A string containing the URL to the full item schema as used by the game.
*/
@SerializedName("items_game_url")
private String itemsGameUrl;
/**
* An object containing the numeric values corresponding to each "quality" an item can have:
*/
private SteamEconSchemaItemQuality qualities;
/**
* A list of objects describing an item's origin.
*/
private List originNames = new ArrayList<>();
/**
* A list of item objects.
*/
private List items = new ArrayList<>();
/**
* An object containing an array of Schema Attributes
*/
private List attributes = new ArrayList<>();
/**
* A list of objects containing item set definitions.
*/
@SerializedName("item_sets")
private List itemSets = new ArrayList<>();
/**
* An object containing a list of objects that describe the defined particle effects.
*/
@SerializedName("attribute_controlled_attached_particles")
private List attributeControlledAttachedParticles = new ArrayList<>();
/**
* A list of objects that describe ranks for kill eater items.
*/
@SerializedName("item_levels")
private List itemLevels = new ArrayList<>();
/**
* An object containing a list of objects describing suffixes
* to use after a kill eater value in an attribute display line.
*/
@SerializedName("kill_eater_score_types")
private List killEaterScoreTypes = new ArrayList<>();
@SerializedName("kill_eater_ranks")
private List killEaterRanks = new ArrayList<>();
/**
* Getter for the field status
.
*
* @return a int
*/
public int getStatus() {
return status;
}
/**
* Setter for the field status
.
*
* @param status
* a int
*/
public void setStatus(int status) {
this.status = status;
}
/**
* Getter for the field itemsGameUrl
.
*
* @return a {@link java.lang.String} object
*/
public String getItemsGameUrl() {
return itemsGameUrl;
}
/**
* Setter for the field itemsGameUrl
.
*
* @param itemsGameUrl
* a {@link java.lang.String} object
*/
public void setItemsGameUrl(String itemsGameUrl) {
this.itemsGameUrl = itemsGameUrl;
}
/**
* Getter for the field qualities
.
*
* @return a {@link com.ibasco.agql.protocols.valve.steam.webapi.pojos.SteamEconSchemaItemQuality} object
*/
public SteamEconSchemaItemQuality getQualities() {
return qualities;
}
/**
* Setter for the field qualities
.
*
* @param qualities
* a {@link com.ibasco.agql.protocols.valve.steam.webapi.pojos.SteamEconSchemaItemQuality} object
*/
public void setQualities(SteamEconSchemaItemQuality qualities) {
this.qualities = qualities;
}
/**
* Getter for the field originNames
.
*
* @return a {@link java.util.List} object
*/
public List getOriginNames() {
return originNames;
}
/**
* Setter for the field originNames
.
*
* @param originNames
* a {@link java.util.List} object
*/
public void setOriginNames(List originNames) {
this.originNames = originNames;
}
/**
* Getter for the field items
.
*
* @return a {@link java.util.List} object
*/
public List getItems() {
return items;
}
/**
* Setter for the field items
.
*
* @param items
* a {@link java.util.List} object
*/
public void setItems(List items) {
this.items = items;
}
/**
* Getter for the field attributes
.
*
* @return a {@link java.util.List} object
*/
public List getAttributes() {
return attributes;
}
/**
* Setter for the field attributes
.
*
* @param attributes
* a {@link java.util.List} object
*/
public void setAttributes(List attributes) {
this.attributes = attributes;
}
/**
* Getter for the field itemSets
.
*
* @return a {@link java.util.List} object
*/
public List getItemSets() {
return itemSets;
}
/**
* Setter for the field itemSets
.
*
* @param itemSets
* a {@link java.util.List} object
*/
public void setItemSets(List itemSets) {
this.itemSets = itemSets;
}
/**
* Getter for the field attributeControlledAttachedParticles
.
*
* @return a {@link java.util.List} object
*/
public List getAttributeControlledAttachedParticles() {
return attributeControlledAttachedParticles;
}
/**
* Setter for the field attributeControlledAttachedParticles
.
*
* @param attributeControlledAttachedParticles
* a {@link java.util.List} object
*/
public void setAttributeControlledAttachedParticles(List attributeControlledAttachedParticles) {
this.attributeControlledAttachedParticles = attributeControlledAttachedParticles;
}
/**
* Getter for the field itemLevels
.
*
* @return a {@link java.util.List} object
*/
public List getItemLevels() {
return itemLevels;
}
/**
* Setter for the field itemLevels
.
*
* @param itemLevels
* a {@link java.util.List} object
*/
public void setItemLevels(List itemLevels) {
this.itemLevels = itemLevels;
}
/**
* Getter for the field killEaterScoreTypes
.
*
* @return a {@link java.util.List} object
*/
public List getKillEaterScoreTypes() {
return killEaterScoreTypes;
}
/**
* Setter for the field killEaterScoreTypes
.
*
* @param killEaterScoreTypes
* a {@link java.util.List} object
*/
public void setKillEaterScoreTypes(List killEaterScoreTypes) {
this.killEaterScoreTypes = killEaterScoreTypes;
}
/**
* Getter for the field killEaterRanks
.
*
* @return a {@link java.util.List} object
*/
public List getKillEaterRanks() {
return killEaterRanks;
}
/**
* Setter for the field killEaterRanks
.
*
* @param killEaterRanks
* a {@link java.util.List} object
*/
public void setKillEaterRanks(List killEaterRanks) {
this.killEaterRanks = killEaterRanks;
}
/** {@inheritDoc} */
@Override
public String toString() {
return ReflectionToStringBuilder.toString(this, ToStringStyle.NO_CLASS_NAME_STYLE);
}
}