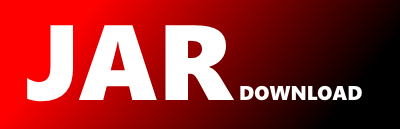
com.ibasco.agql.protocols.valve.steam.webapi.pojos.CheatData Maven / Gradle / Ivy
Show all versions of agql-steam-webapi Show documentation
/*
* Copyright (c) 2022 Asynchronous Game Query Library
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.ibasco.agql.protocols.valve.steam.webapi.pojos;
/**
* CheatData class.
*
* @author Rafael Luis Ibasco
*/
public class CheatData {
private long steamId;
private int appId;
private String filePath;
private String webCheatUrl;
private long timeNow;
private long timeStarted;
private long timeStopped;
private String cheatName;
private int gameProcessId;
private int cheatProcessId;
private long cheatParam1;
private long cheatParam2;
/**
* Getter for the field steamId
.
*
* @return Steamid of the user running and reporting the cheat.
*/
public long getSteamId() {
return steamId;
}
/**
* Setter for the field steamId
.
*
* @param steamId
* a long
*/
public void setSteamId(long steamId) {
this.steamId = steamId;
}
/**
* Getter for the field appId
.
*
* @return The Steam APP Id
*/
public int getAppId() {
return appId;
}
/**
* Setter for the field appId
.
*
* @param appId
* a int
*/
public void setAppId(int appId) {
this.appId = appId;
}
/**
* Getter for the field filePath
.
*
* @return Path and file name of the cheat executable.
*/
public String getFilePath() {
return filePath;
}
/**
* Setter for the field filePath
.
*
* @param filePath
* a {@link java.lang.String} object
*/
public void setFilePath(String filePath) {
this.filePath = filePath;
}
/**
* Getter for the field webCheatUrl
.
*
* @return The web url where the cheat was found and downloaded.
*/
public String getWebCheatUrl() {
return webCheatUrl;
}
/**
* Setter for the field webCheatUrl
.
*
* @param webCheatUrl
* a {@link java.lang.String} object
*/
public void setWebCheatUrl(String webCheatUrl) {
this.webCheatUrl = webCheatUrl;
}
/**
* Getter for the field timeNow
.
*
* @return Local system time now.
*/
public long getTimeNow() {
return timeNow;
}
/**
* Setter for the field timeNow
.
*
* @param timeNow
* a long
*/
public void setTimeNow(long timeNow) {
this.timeNow = timeNow;
}
/**
* Getter for the field timeStarted
.
*
* @return Local system time when cheat process started. ( 0 if not yet run )
*/
public long getTimeStarted() {
return timeStarted;
}
/**
* Setter for the field timeStarted
.
*
* @param timeStarted
* a long
*/
public void setTimeStarted(long timeStarted) {
this.timeStarted = timeStarted;
}
/**
* Getter for the field timeStopped
.
*
* @return Local system time when cheat process stopped. ( 0 if still running )
*/
public long getTimeStopped() {
return timeStopped;
}
/**
* Setter for the field timeStopped
.
*
* @param timeStopped
* a long
*/
public void setTimeStopped(long timeStopped) {
this.timeStopped = timeStopped;
}
/**
* Getter for the field cheatName
.
*
* @return The descriptive name for the cheat.
*/
public String getCheatName() {
return cheatName;
}
/**
* Setter for the field cheatName
.
*
* @param cheatName
* a {@link java.lang.String} object
*/
public void setCheatName(String cheatName) {
this.cheatName = cheatName;
}
/**
* Getter for the field gameProcessId
.
*
* @return The process ID of the running game.
*/
public int getGameProcessId() {
return gameProcessId;
}
/**
* Setter for the field gameProcessId
.
*
* @param gameProcessId
* a int
*/
public void setGameProcessId(int gameProcessId) {
this.gameProcessId = gameProcessId;
}
/**
* Getter for the field cheatProcessId
.
*
* @return The process ID of the cheat process that ran
*/
public int getCheatProcessId() {
return cheatProcessId;
}
/**
* Setter for the field cheatProcessId
.
*
* @param cheatProcessId
* a int
*/
public void setCheatProcessId(int cheatProcessId) {
this.cheatProcessId = cheatProcessId;
}
/**
* Getter for the field cheatParam1
.
*
* @return Cheat param 1
*/
public long getCheatParam1() {
return cheatParam1;
}
/**
* Setter for the field cheatParam1
.
*
* @param cheatParam1
* a long
*/
public void setCheatParam1(long cheatParam1) {
this.cheatParam1 = cheatParam1;
}
/**
* Getter for the field cheatParam2
.
*
* @return Cheat param 2
*/
public long getCheatParam2() {
return cheatParam2;
}
/**
* Setter for the field cheatParam2
.
*
* @param cheatParam2
* a long
*/
public void setCheatParam2(long cheatParam2) {
this.cheatParam2 = cheatParam2;
}
}