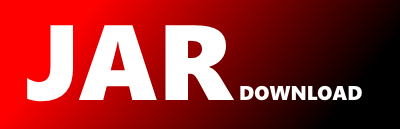
com.ibasco.agql.protocols.valve.steam.webapi.pojos.SteamAssetClassInfo Maven / Gradle / Ivy
Show all versions of agql-steam-webapi Show documentation
/*
* Copyright (c) 2022 Asynchronous Game Query Library
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.ibasco.agql.protocols.valve.steam.webapi.pojos;
import com.google.gson.annotations.SerializedName;
import org.apache.commons.lang3.builder.ToStringBuilder;
import org.apache.commons.lang3.builder.ToStringStyle;
import java.util.Map;
/**
* Created by raffy on 10/27/2016.
*
* @author Rafael Luis Ibasco
*/
public class SteamAssetClassInfo {
/**
* An encoded URL to be passed to {@code http://cdn.steamcommunity.com/economy/image/}
*/
@SerializedName("icon_url")
private String encodedIconUrl;
@SerializedName("icon_url_large")
private String encodedIconUrlLarge;
@SerializedName("icon_drag_url")
private String encodedIconDragUrl;
@SerializedName("name")
private String name;
@SerializedName("market_hash_name")
private String marketHashName;
@SerializedName("market_name")
private String marketName;
@SerializedName("name_color")
private String nameColor;
@SerializedName("background_color")
private String backgroundColor;
@SerializedName("type")
private String type;
//TODO: Use TypeAdapters as provided by GSON then convert this into a boolean type
@SerializedName("tradable")
private String tradable;
@SerializedName("marketable")
private String marketable;
@SerializedName("commodity")
private String commodity;
@SerializedName("market_tradable_restriction")
private String marketTradableRestriction;
@SerializedName("fraudwarnings")
private String fraudWarnings;
@SerializedName("descriptions")
private Map descriptions;
@SerializedName("owner_descriptions")
private String ownerDescriptions;
@SerializedName("tags")
private Map tags;
@SerializedName("classid")
private String classId;
/**
* Getter for the field encodedIconUrl
.
*
* @return a {@link java.lang.String} object
*/
public String getEncodedIconUrl() {
return encodedIconUrl;
}
/**
* Setter for the field encodedIconUrl
.
*
* @param encodedIconUrl
* a {@link java.lang.String} object
*/
public void setEncodedIconUrl(String encodedIconUrl) {
this.encodedIconUrl = encodedIconUrl;
}
/**
* Getter for the field encodedIconUrlLarge
.
*
* @return a {@link java.lang.String} object
*/
public String getEncodedIconUrlLarge() {
return encodedIconUrlLarge;
}
/**
* Setter for the field encodedIconUrlLarge
.
*
* @param encodedIconUrlLarge
* a {@link java.lang.String} object
*/
public void setEncodedIconUrlLarge(String encodedIconUrlLarge) {
this.encodedIconUrlLarge = encodedIconUrlLarge;
}
/**
* Getter for the field encodedIconDragUrl
.
*
* @return a {@link java.lang.String} object
*/
public String getEncodedIconDragUrl() {
return encodedIconDragUrl;
}
/**
* Setter for the field encodedIconDragUrl
.
*
* @param encodedIconDragUrl
* a {@link java.lang.String} object
*/
public void setEncodedIconDragUrl(String encodedIconDragUrl) {
this.encodedIconDragUrl = encodedIconDragUrl;
}
/**
* Getter for the field marketHashName
.
*
* @return a {@link java.lang.String} object
*/
public String getMarketHashName() {
return marketHashName;
}
/**
* Setter for the field marketHashName
.
*
* @param marketHashName
* a {@link java.lang.String} object
*/
public void setMarketHashName(String marketHashName) {
this.marketHashName = marketHashName;
}
/**
* Getter for the field nameColor
.
*
* @return a {@link java.lang.String} object
*/
public String getNameColor() {
return nameColor;
}
/**
* Setter for the field nameColor
.
*
* @param nameColor
* a {@link java.lang.String} object
*/
public void setNameColor(String nameColor) {
this.nameColor = nameColor;
}
/**
* Getter for the field backgroundColor
.
*
* @return a {@link java.lang.String} object
*/
public String getBackgroundColor() {
return backgroundColor;
}
/**
* Setter for the field backgroundColor
.
*
* @param backgroundColor
* a {@link java.lang.String} object
*/
public void setBackgroundColor(String backgroundColor) {
this.backgroundColor = backgroundColor;
}
/**
* Getter for the field type
.
*
* @return a {@link java.lang.String} object
*/
public String getType() {
return type;
}
/**
* Setter for the field type
.
*
* @param type
* a {@link java.lang.String} object
*/
public void setType(String type) {
this.type = type;
}
/**
* Getter for the field tradable
.
*
* @return a {@link java.lang.String} object
*/
public String getTradable() {
return tradable;
}
/**
* Setter for the field tradable
.
*
* @param tradable
* a {@link java.lang.String} object
*/
public void setTradable(String tradable) {
this.tradable = tradable;
}
/**
* Getter for the field marketable
.
*
* @return a {@link java.lang.String} object
*/
public String getMarketable() {
return marketable;
}
/**
* Setter for the field marketable
.
*
* @param marketable
* a {@link java.lang.String} object
*/
public void setMarketable(String marketable) {
this.marketable = marketable;
}
/**
* Getter for the field commodity
.
*
* @return a {@link java.lang.String} object
*/
public String getCommodity() {
return commodity;
}
/**
* Setter for the field commodity
.
*
* @param commodity
* a {@link java.lang.String} object
*/
public void setCommodity(String commodity) {
this.commodity = commodity;
}
/**
* Getter for the field marketTradableRestriction
.
*
* @return a {@link java.lang.String} object
*/
public String getMarketTradableRestriction() {
return marketTradableRestriction;
}
/**
* Setter for the field marketTradableRestriction
.
*
* @param marketTradableRestriction
* a {@link java.lang.String} object
*/
public void setMarketTradableRestriction(String marketTradableRestriction) {
this.marketTradableRestriction = marketTradableRestriction;
}
/**
* Getter for the field fraudWarnings
.
*
* @return a {@link java.lang.String} object
*/
public String getFraudWarnings() {
return fraudWarnings;
}
/**
* Setter for the field fraudWarnings
.
*
* @param fraudWarnings
* a {@link java.lang.String} object
*/
public void setFraudWarnings(String fraudWarnings) {
this.fraudWarnings = fraudWarnings;
}
/**
* Getter for the field ownerDescriptions
.
*
* @return a {@link java.lang.String} object
*/
public String getOwnerDescriptions() {
return ownerDescriptions;
}
/**
* Setter for the field ownerDescriptions
.
*
* @param ownerDescriptions
* a {@link java.lang.String} object
*/
public void setOwnerDescriptions(String ownerDescriptions) {
this.ownerDescriptions = ownerDescriptions;
}
/** {@inheritDoc} */
@Override
public String toString() {
return new ToStringBuilder(this, ToStringStyle.NO_CLASS_NAME_STYLE)
.append("classId", getClassId())
.append("name", getName())
.append("marketName", getMarketName())
.append("tags", getTags())
.append("descriptions", getDescriptions())
.toString();
}
/**
* Getter for the field classId
.
*
* @return a {@link java.lang.String} object
*/
public String getClassId() {
return classId;
}
/**
* Getter for the field name
.
*
* @return a {@link java.lang.String} object
*/
public String getName() {
return name;
}
/**
* Setter for the field name
.
*
* @param name
* a {@link java.lang.String} object
*/
public void setName(String name) {
this.name = name;
}
/**
* Getter for the field marketName
.
*
* @return a {@link java.lang.String} object
*/
public String getMarketName() {
return marketName;
}
/**
* Setter for the field marketName
.
*
* @param marketName
* a {@link java.lang.String} object
*/
public void setMarketName(String marketName) {
this.marketName = marketName;
}
/**
* Getter for the field tags
.
*
* @return a {@link java.util.Map} object
*/
public Map getTags() {
return tags;
}
/**
* Getter for the field descriptions
.
*
* @return a {@link java.util.Map} object
*/
public Map getDescriptions() {
return descriptions;
}
/**
* Setter for the field descriptions
.
*
* @param descriptions
* a {@link java.util.Map} object
*/
public void setDescriptions(Map descriptions) {
this.descriptions = descriptions;
}
/**
* Setter for the field tags
.
*
* @param tags
* a {@link java.util.Map} object
*/
public void setTags(Map tags) {
this.tags = tags;
}
/**
* Setter for the field classId
.
*
* @param classId
* a {@link java.lang.String} object
*/
public void setClassId(String classId) {
this.classId = classId;
}
}