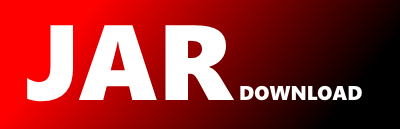
com.ibasco.agql.protocols.valve.steam.webapi.pojos.SteamEconPlayerItem Maven / Gradle / Ivy
Show all versions of agql-steam-webapi Show documentation
/*
* Copyright (c) 2022 Asynchronous Game Query Library
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.ibasco.agql.protocols.valve.steam.webapi.pojos;
import com.google.gson.annotations.SerializedName;
import org.apache.commons.lang3.builder.ReflectionToStringBuilder;
import org.apache.commons.lang3.builder.ToStringStyle;
import java.util.ArrayList;
import java.util.List;
/**
* SteamEconPlayerItem class.
*
* @author Rafael Luis Ibasco
*/
public class SteamEconPlayerItem {
private int id;
@SerializedName("original_id")
private int originalId;
@SerializedName("defindex")
private int defIndex;
private int level;
private int quality;
private int inventory;
private int quantity;
private int rarity;
@SerializedName("flag_cannot_trade")
private boolean cannotTrade;
@SerializedName("attributes")
private List itemAttributes = new ArrayList<>();
private List equipInfos = new ArrayList<>();
@SerializedName("flag_cannot_craft")
private boolean cannotCraft; //tf2 specific
@SerializedName("custom_name")
private String customName;
@SerializedName("custom_desc")
private String customDescription;
private int style;
/**
* Getter for the field id
.
*
* @return a int
*/
public int getId() {
return id;
}
/**
* Setter for the field id
.
*
* @param id
* a int
*/
public void setId(int id) {
this.id = id;
}
/**
* Getter for the field originalId
.
*
* @return a int
*/
public int getOriginalId() {
return originalId;
}
/**
* Setter for the field originalId
.
*
* @param originalId
* a int
*/
public void setOriginalId(int originalId) {
this.originalId = originalId;
}
/**
* Getter for the field defIndex
.
*
* @return a int
*/
public int getDefIndex() {
return defIndex;
}
/**
* Setter for the field defIndex
.
*
* @param defIndex
* a int
*/
public void setDefIndex(int defIndex) {
this.defIndex = defIndex;
}
/**
* Getter for the field level
.
*
* @return a int
*/
public int getLevel() {
return level;
}
/**
* Setter for the field level
.
*
* @param level
* a int
*/
public void setLevel(int level) {
this.level = level;
}
/**
* Getter for the field quality
.
*
* @return a int
*/
public int getQuality() {
return quality;
}
/**
* Setter for the field quality
.
*
* @param quality
* a int
*/
public void setQuality(int quality) {
this.quality = quality;
}
/**
* Getter for the field inventory
.
*
* @return a int
*/
public int getInventory() {
return inventory;
}
/**
* Setter for the field inventory
.
*
* @param inventory
* a int
*/
public void setInventory(int inventory) {
this.inventory = inventory;
}
/**
* Getter for the field quantity
.
*
* @return a int
*/
public int getQuantity() {
return quantity;
}
/**
* Setter for the field quantity
.
*
* @param quantity
* a int
*/
public void setQuantity(int quantity) {
this.quantity = quantity;
}
/**
* Getter for the field rarity
.
*
* @return a int
*/
public int getRarity() {
return rarity;
}
/**
* Setter for the field rarity
.
*
* @param rarity
* a int
*/
public void setRarity(int rarity) {
this.rarity = rarity;
}
/**
* isCannotTrade.
*
* @return a boolean
*/
public boolean isCannotTrade() {
return cannotTrade;
}
/**
* Setter for the field cannotTrade
.
*
* @param cannotTrade
* a boolean
*/
public void setCannotTrade(boolean cannotTrade) {
this.cannotTrade = cannotTrade;
}
/**
* Getter for the field itemAttributes
.
*
* @return a {@link java.util.List} object
*/
public List getItemAttributes() {
return itemAttributes;
}
/**
* Setter for the field itemAttributes
.
*
* @param itemAttributes
* a {@link java.util.List} object
*/
public void setItemAttributes(List itemAttributes) {
this.itemAttributes = itemAttributes;
}
/**
* Getter for the field equipInfos
.
*
* @return a {@link java.util.List} object
*/
public List getEquipInfos() {
return equipInfos;
}
/**
* Setter for the field equipInfos
.
*
* @param equipInfos
* a {@link java.util.List} object
*/
public void setEquipInfos(List equipInfos) {
this.equipInfos = equipInfos;
}
/**
* isCannotCraft.
*
* @return a boolean
*/
public boolean isCannotCraft() {
return cannotCraft;
}
/**
* Setter for the field cannotCraft
.
*
* @param cannotCraft
* a boolean
*/
public void setCannotCraft(boolean cannotCraft) {
this.cannotCraft = cannotCraft;
}
/**
* Getter for the field customName
.
*
* @return a {@link java.lang.String} object
*/
public String getCustomName() {
return customName;
}
/**
* Setter for the field customName
.
*
* @param customName
* a {@link java.lang.String} object
*/
public void setCustomName(String customName) {
this.customName = customName;
}
/**
* Getter for the field customDescription
.
*
* @return a {@link java.lang.String} object
*/
public String getCustomDescription() {
return customDescription;
}
/**
* Setter for the field customDescription
.
*
* @param customDescription
* a {@link java.lang.String} object
*/
public void setCustomDescription(String customDescription) {
this.customDescription = customDescription;
}
/**
* Getter for the field style
.
*
* @return a int
*/
public int getStyle() {
return style;
}
/**
* Setter for the field style
.
*
* @param style
* a int
*/
public void setStyle(int style) {
this.style = style;
}
/** {@inheritDoc} */
@Override
public String toString() {
return ReflectionToStringBuilder.toString(this, ToStringStyle.NO_CLASS_NAME_STYLE);
}
}