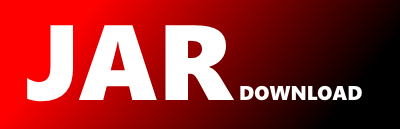
com.ibasco.agql.protocols.valve.steam.webapi.pojos.SteamEconSchemaCapabilities Maven / Gradle / Ivy
/*
* Copyright (c) 2022 Asynchronous Game Query Library
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.ibasco.agql.protocols.valve.steam.webapi.pojos;
import com.google.gson.annotations.SerializedName;
import org.apache.commons.lang3.builder.ReflectionToStringBuilder;
import org.apache.commons.lang3.builder.ToStringStyle;
/**
* SteamEconSchemaCapabilities class.
*
* @author Rafael Luis Ibasco
*/
public class SteamEconSchemaCapabilities {
private Boolean nameable;
@SerializedName("can_gift_wrap")
private Boolean canGiftWrap;
@SerializedName("can_craft_mark")
private Boolean canCraftMark;
@SerializedName("can_be_restored ")
private Boolean canBeRestored;
@SerializedName("strange_parts")
private Boolean strangeParts;
@SerializedName("can_card_upgrade")
private Boolean canCardUpgrade;
@SerializedName("can_strangify")
private Boolean canStrangify;
@SerializedName("can_killstreakify")
private Boolean canKillStreakify;
@SerializedName("can_consume")
private Boolean canConsume;
private Boolean decodable;
@SerializedName("usable_gc")
private Boolean usableGc;
@SerializedName("usable_out_of_game")
private Boolean usableOutOfGame;
@SerializedName("can_sticker")
private Boolean canSticker; //for version 2
@SerializedName("can_stattrack_swap")
private Boolean canStattrackSwap; //for version 2
/**
* Getter for the field nameable
.
*
* @return a {@link java.lang.Boolean} object
*/
public Boolean getNameable() {
return nameable;
}
/**
* Setter for the field nameable
.
*
* @param nameable
* a {@link java.lang.Boolean} object
*/
public void setNameable(Boolean nameable) {
this.nameable = nameable;
}
/**
* Getter for the field canGiftWrap
.
*
* @return a {@link java.lang.Boolean} object
*/
public Boolean getCanGiftWrap() {
return canGiftWrap;
}
/**
* Setter for the field canGiftWrap
.
*
* @param canGiftWrap
* a {@link java.lang.Boolean} object
*/
public void setCanGiftWrap(Boolean canGiftWrap) {
this.canGiftWrap = canGiftWrap;
}
/**
* Getter for the field canCraftMark
.
*
* @return a {@link java.lang.Boolean} object
*/
public Boolean getCanCraftMark() {
return canCraftMark;
}
/**
* Setter for the field canCraftMark
.
*
* @param canCraftMark
* a {@link java.lang.Boolean} object
*/
public void setCanCraftMark(Boolean canCraftMark) {
this.canCraftMark = canCraftMark;
}
/**
* Getter for the field canBeRestored
.
*
* @return a {@link java.lang.Boolean} object
*/
public Boolean getCanBeRestored() {
return canBeRestored;
}
/**
* Setter for the field canBeRestored
.
*
* @param canBeRestored
* a {@link java.lang.Boolean} object
*/
public void setCanBeRestored(Boolean canBeRestored) {
this.canBeRestored = canBeRestored;
}
/**
* Getter for the field strangeParts
.
*
* @return a {@link java.lang.Boolean} object
*/
public Boolean getStrangeParts() {
return strangeParts;
}
/**
* Setter for the field strangeParts
.
*
* @param strangeParts
* a {@link java.lang.Boolean} object
*/
public void setStrangeParts(Boolean strangeParts) {
this.strangeParts = strangeParts;
}
/**
* Getter for the field canCardUpgrade
.
*
* @return a {@link java.lang.Boolean} object
*/
public Boolean getCanCardUpgrade() {
return canCardUpgrade;
}
/**
* Setter for the field canCardUpgrade
.
*
* @param canCardUpgrade
* a {@link java.lang.Boolean} object
*/
public void setCanCardUpgrade(Boolean canCardUpgrade) {
this.canCardUpgrade = canCardUpgrade;
}
/**
* Getter for the field canStrangify
.
*
* @return a {@link java.lang.Boolean} object
*/
public Boolean getCanStrangify() {
return canStrangify;
}
/**
* Setter for the field canStrangify
.
*
* @param canStrangify
* a {@link java.lang.Boolean} object
*/
public void setCanStrangify(Boolean canStrangify) {
this.canStrangify = canStrangify;
}
/**
* Getter for the field canKillStreakify
.
*
* @return a {@link java.lang.Boolean} object
*/
public Boolean getCanKillStreakify() {
return canKillStreakify;
}
/**
* Setter for the field canKillStreakify
.
*
* @param canKillStreakify
* a {@link java.lang.Boolean} object
*/
public void setCanKillStreakify(Boolean canKillStreakify) {
this.canKillStreakify = canKillStreakify;
}
/**
* Getter for the field canConsume
.
*
* @return a {@link java.lang.Boolean} object
*/
public Boolean getCanConsume() {
return canConsume;
}
/**
* Setter for the field canConsume
.
*
* @param canConsume
* a {@link java.lang.Boolean} object
*/
public void setCanConsume(Boolean canConsume) {
this.canConsume = canConsume;
}
/**
* Getter for the field decodable
.
*
* @return a {@link java.lang.Boolean} object
*/
public Boolean getDecodable() {
return decodable;
}
/**
* Setter for the field decodable
.
*
* @param decodable
* a {@link java.lang.Boolean} object
*/
public void setDecodable(Boolean decodable) {
this.decodable = decodable;
}
/**
* Getter for the field usableGc
.
*
* @return a {@link java.lang.Boolean} object
*/
public Boolean getUsableGc() {
return usableGc;
}
/**
* Setter for the field usableGc
.
*
* @param usableGc
* a {@link java.lang.Boolean} object
*/
public void setUsableGc(Boolean usableGc) {
this.usableGc = usableGc;
}
/**
* Getter for the field usableOutOfGame
.
*
* @return a {@link java.lang.Boolean} object
*/
public Boolean getUsableOutOfGame() {
return usableOutOfGame;
}
/**
* Setter for the field usableOutOfGame
.
*
* @param usableOutOfGame
* a {@link java.lang.Boolean} object
*/
public void setUsableOutOfGame(Boolean usableOutOfGame) {
this.usableOutOfGame = usableOutOfGame;
}
/**
* Getter for the field canSticker
.
*
* @return a {@link java.lang.Boolean} object
*/
public Boolean getCanSticker() {
return canSticker;
}
/**
* Setter for the field canSticker
.
*
* @param canSticker
* a {@link java.lang.Boolean} object
*/
public void setCanSticker(Boolean canSticker) {
this.canSticker = canSticker;
}
/**
* Getter for the field canStattrackSwap
.
*
* @return a {@link java.lang.Boolean} object
*/
public Boolean getCanStattrackSwap() {
return canStattrackSwap;
}
/**
* Setter for the field canStattrackSwap
.
*
* @param canStattrackSwap
* a {@link java.lang.Boolean} object
*/
public void setCanStattrackSwap(Boolean canStattrackSwap) {
this.canStattrackSwap = canStattrackSwap;
}
/** {@inheritDoc} */
@Override
public String toString() {
return ReflectionToStringBuilder.toString(this, ToStringStyle.NO_CLASS_NAME_STYLE);
}
}