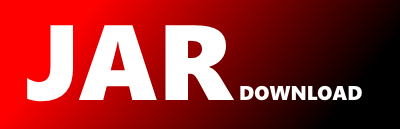
com.ibasco.agql.protocols.valve.steam.webapi.pojos.SteamPlayerBadgeInfo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of agql-steam-webapi Show documentation
Show all versions of agql-steam-webapi Show documentation
An implementation for the Steam Web API Interfaces
/*
* Copyright (c) 2022 Asynchronous Game Query Library
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.ibasco.agql.protocols.valve.steam.webapi.pojos;
import com.google.gson.annotations.SerializedName;
import java.util.ArrayList;
import java.util.List;
/**
* Created by raffy on 10/27/2016.
*
* @author Rafael Luis Ibasco
*/
public class SteamPlayerBadgeInfo {
@SerializedName("badges")
private List playerBadges = new ArrayList<>();
@SerializedName("player_xp")
private int playerXp;
@SerializedName("player_level")
private int playerLevel;
@SerializedName("player_xp_needed_to_level_up")
private int xpNeededToLevelUp;
@SerializedName("player_xp_needed_current_level")
private int xpNeededCurrentLevel;
/**
* Getter for the field playerBadges
.
*
* @return a {@link java.util.List} object
*/
public List getPlayerBadges() {
return playerBadges;
}
/**
* Setter for the field playerBadges
.
*
* @param playerBadges
* a {@link java.util.List} object
*/
public void setPlayerBadges(List playerBadges) {
this.playerBadges = playerBadges;
}
/**
* Getter for the field playerXp
.
*
* @return a int
*/
public int getPlayerXp() {
return playerXp;
}
/**
* Setter for the field playerXp
.
*
* @param playerXp
* a int
*/
public void setPlayerXp(int playerXp) {
this.playerXp = playerXp;
}
/**
* Getter for the field playerLevel
.
*
* @return a int
*/
public int getPlayerLevel() {
return playerLevel;
}
/**
* Setter for the field playerLevel
.
*
* @param playerLevel
* a int
*/
public void setPlayerLevel(int playerLevel) {
this.playerLevel = playerLevel;
}
/**
* Getter for the field xpNeededToLevelUp
.
*
* @return a int
*/
public int getXpNeededToLevelUp() {
return xpNeededToLevelUp;
}
/**
* Setter for the field xpNeededToLevelUp
.
*
* @param xpNeededToLevelUp
* a int
*/
public void setXpNeededToLevelUp(int xpNeededToLevelUp) {
this.xpNeededToLevelUp = xpNeededToLevelUp;
}
/**
* Getter for the field xpNeededCurrentLevel
.
*
* @return a int
*/
public int getXpNeededCurrentLevel() {
return xpNeededCurrentLevel;
}
/**
* Setter for the field xpNeededCurrentLevel
.
*
* @param xpNeededCurrentLevel
* a int
*/
public void setXpNeededCurrentLevel(int xpNeededCurrentLevel) {
this.xpNeededCurrentLevel = xpNeededCurrentLevel;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy