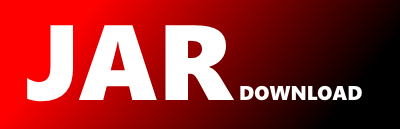
com.ibm.cloud.objectstorage.smoketest.AWSCucumberStepdefs Maven / Gradle / Ivy
/*
* Copyright 2011-2023 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at:
*
* http://aws.amazon.com/apache2.0
*
* This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES
* OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and
* limitations under the License.
*/
package com.ibm.cloud.objectstorage.smoketest;
import static org.junit.Assert.assertEquals;
import static org.junit.Assert.assertNotNull;
import static org.junit.Assert.assertTrue;
import java.util.List;
import java.lang.reflect.InvocationTargetException;
import java.lang.reflect.Method;
import java.util.Arrays;
import java.util.Map;
import com.ibm.cloud.objectstorage.AmazonServiceException;
import com.ibm.cloud.objectstorage.AmazonWebServiceClient;
import com.ibm.cloud.objectstorage.regions.RegionUtils;
import com.ibm.cloud.objectstorage.util.Classes;
import com.google.inject.Inject;
import cucumber.api.java.en.And;
import cucumber.api.java.en.Then;
import cucumber.api.java.en.When;
import cucumber.runtime.java.guice.ScenarioScoped;
/**
* Step definitions that perform actions for every matcher that is agreed.
*/
@ScenarioScoped
public class AWSCucumberStepdefs {
private AmazonWebServiceClient client;
private String packageName;
private Object result;
private AmazonServiceException exception;
@Inject
public AWSCucumberStepdefs(AmazonWebServiceClient client) {
this.client = client;
this.client.setRegion(RegionUtils.getRegion("us-east-1"));
Class> httpClientClass = Classes.childClassOf(AmazonWebServiceClient.class, this.client);
this.packageName = httpClientClass.getPackage().getName();
}
@When("^I call the \"(.*?)\" API$")
public void when_I_call_the_API(String operation)
throws IllegalAccessException, IllegalArgumentException, InvocationTargetException {
call(operation, null);
}
@When("^I call the \"(.*?)\" API with:$")
public void when_I_call_the_API(String operation, Map args)
throws IllegalAccessException, IllegalArgumentException, InvocationTargetException {
call(operation, args);
}
@When("^I attempt to call the \"(.+?)\" API with:$")
public void when_I_attempt_to_call_API(String operation, Map args)
throws IllegalAccessException, IllegalArgumentException, InvocationTargetException {
attemptCall(operation, args);
}
@Then("^the response should contain a \"([^\"]*)\"$")
public void the_response_should_contain_a(String memberName) throws Throwable {
String[] path = memberName.split("[.]");
Object member = ReflectionUtils.getByPath(result, Arrays.asList(path));
assertNotNull(member);
}
@Then("^the value at \"(.*?)\" should be a list")
public void then_the_value_at_should_be_a_list(String memberName) {
String[] path = memberName.split("[.]");
Object member = ReflectionUtils.getByPath(result, Arrays.asList(path));
assertTrue(member instanceof java.util.List);
}
@Then("^the value at \"(.*?)\" should be a map")
public void then_the_value_at_should_be_a_map(String memberName) {
String[] path = memberName.split("[.]");
Object member = ReflectionUtils.getByPath(result, Arrays.asList(path));
assertTrue(member instanceof java.util.Map);
}
@Then("^I expect the response error code to be \"(.+?)\"$")
public void then_I_expect_response_error_code(String expected) {
assertNotNull(exception);
assertTrue(exception instanceof AmazonServiceException);
String actual = exception.getErrorCode();
assertEquals("Error code doesn't match. Expected : " + expected + ". Actual :" + actual, expected, actual);
}
@And("^I expect the response error message to include:$")
public void and_I_expect_the_response_error_message_include(String expected) {
assertNotNull(exception);
assertTrue(exception instanceof AmazonServiceException);
String actual = exception.getErrorMessage().toLowerCase();
assertTrue("Error message doesn't match. Expected : " + expected + ". Actual :" + actual,
actual.contains(expected.toLowerCase()));
}
private void attemptCall(String operation, Map args)
throws IllegalAccessException, IllegalArgumentException {
try {
call(operation, args);
} catch (InvocationTargetException ite) {
exception = (AmazonServiceException) ite.getCause();
}
}
private void call(String operation, Map args)
throws IllegalAccessException, IllegalArgumentException, InvocationTargetException {
final String requestClassName = packageName + ".model." + operation + "Request";
final String operationMethodName = operation.substring(0, 1).toLowerCase() + operation.substring(1);
Class
© 2015 - 2025 Weber Informatics LLC | Privacy Policy