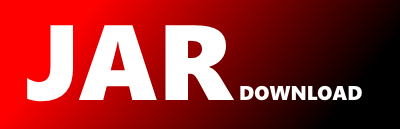
com.ibm.cp4waiops.connectors.sdk.EventLifeCycleEvent Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of connectors-sdk Show documentation
Show all versions of connectors-sdk Show documentation
A developer SDK for creating connectors for CP4WAIOps.
package com.ibm.cp4waiops.connectors.sdk;
import java.text.DateFormat;
import java.text.SimpleDateFormat;
import java.util.Arrays;
import java.util.Date;
import java.util.Map;
import java.util.Objects;
import java.util.TimeZone;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.core.JsonProcessingException;
import com.fasterxml.jackson.databind.ObjectMapper;
@JsonInclude(Include.NON_NULL)
public class EventLifeCycleEvent {
public static final String RESOURCE_TYPE_FIELD = "type";
public static final String RESOURCE_NAME_FIELD = "name";
public static final String RESOURCE_SOURCE_ID_FIELD = "sourceId";
public static final String RESOURCE_HOSTNAME_FIELD = "hostname";
public static final String RESOURCE_IP_ADDRESS_FIELD = "ipAddress";
public static final String RESOURCE_SERVICE_FIELD = "service";
public static final String RESOURCE_PORT_FIELD = "port";
public static final String RESOURCE_INTERFACE_FIELD = "interface";
public static final String RESOURCE_APPLICATION_FIELD = "application";
public static final String RESOURCE_CONTROLLER_FIELD = "controller";
public static final String RESOURCE_COMPONENT_FIELD = "component";
public static final String RESOURCE_CLUSTER_FIELD = "cluster";
public static final String RESOURCE_LOCATION_FIELD = "location";
public static final String RESOURCE_ACCESS_SCOPE_FIELD = "accessScope";
public static final String EVENT_TYPE_PROBLEM = "problem";
public static final String EVENT_TYPE_RESOLUTION = "resolution";
public static final ObjectMapper mapper = new ObjectMapper();
static {
DateFormat df = new SimpleDateFormat("yyyy-MM-dd'T'HH:mm:ssXXX");
df.setTimeZone(TimeZone.getTimeZone("UTC"));
mapper.setDateFormat(df);
}
public static class Type {
private String eventType;
private String classification;
private String condition;
public Type() {
}
@Override
public boolean equals(Object obj) {
if (this == null || obj == null)
return this == obj;
if (!(obj instanceof Type))
return false;
Type other = (Type) obj;
return Objects.equals(this.eventType, other.eventType)
&& Objects.equals(this.classification, other.classification)
&& Objects.equals(this.condition, other.condition);
}
@Override
public int hashCode() {
return Objects.hash(eventType, classification, condition);
}
public String getCondition() {
return condition;
}
public void setCondition(String condition) {
this.condition = condition;
}
public String getClassification() {
return classification;
}
public void setClassification(String classification) {
this.classification = classification;
}
public String getEventType() {
return eventType;
}
public void setEventType(String eventType) {
this.eventType = eventType;
}
}
public static class Link {
private String linkType;
private String name;
private String description;
private String url;
public Link() {
}
@Override
public boolean equals(Object obj) {
if (this == null || obj == null)
return this == obj;
if (!(obj instanceof Link))
return false;
Link other = (Link) obj;
return Objects.equals(this.linkType, other.linkType) && Objects.equals(this.name, other.name)
&& Objects.equals(this.description, other.description) && Objects.equals(this.url, other.url);
}
@Override
public int hashCode() {
return Objects.hash(linkType, name, description, url);
}
public String getUrl() {
return url;
}
public void setUrl(String url) {
this.url = url;
}
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getLinkType() {
return linkType;
}
public void setLinkType(String linkType) {
this.linkType = linkType;
}
}
private String id;
private Date occurrenceTime;
private String summary;
private Integer severity;
private Type type;
private Map sender;
private Map resource;
private Integer expirySeconds;
private Link[] links;
private Map details;
public EventLifeCycleEvent() {
}
@Override
public boolean equals(Object obj) {
if (this == null || obj == null)
return this == obj;
if (!(obj instanceof EventLifeCycleEvent))
return false;
EventLifeCycleEvent other = (EventLifeCycleEvent) obj;
return Objects.equals(this.id, other.id) && datesEqual(this.occurrenceTime, other.occurrenceTime)
&& Objects.equals(this.summary, other.summary) && Objects.equals(this.severity, other.severity)
&& Objects.equals(this.type, other.type) && Objects.equals(this.sender, other.sender)
&& Objects.equals(this.resource, other.resource)
&& Objects.equals(this.expirySeconds, other.expirySeconds) && Arrays.equals(this.links, other.links)
&& Objects.equals(this.details, other.details);
}
protected boolean datesEqual(Date a, Date b) {
if (a == null || b == null)
return a == b;
// tolerance for up to a second difference (JSON conversion)
return Math.abs(a.getTime() - b.getTime()) < 1000;
}
@Override
public int hashCode() {
return Objects.hash(this.id, this.occurrenceTime, this.summary, this.severity, this.type, this.sender,
this.resource, this.expirySeconds, Objects.hash((Object[]) this.links), this.details);
}
public String toJSON() throws JsonProcessingException {
return mapper.writeValueAsString(this);
}
public static EventLifeCycleEvent fromJSON(String value) throws JsonProcessingException {
return mapper.readValue(value, EventLifeCycleEvent.class);
}
public Map getDetails() {
return details;
}
public void setDetails(Map details) {
this.details = details;
}
public Link[] getLinks() {
return links;
}
public void setLinks(Link[] links) {
this.links = links;
}
public Integer getExpirySeconds() {
return expirySeconds;
}
public void setExpirySeconds(Integer expirySeconds) {
this.expirySeconds = expirySeconds;
}
public Map getResource() {
return resource;
}
public void setResource(Map resource) {
this.resource = resource;
}
public Map getSender() {
return sender;
}
public void setSender(Map sender) {
this.sender = sender;
}
public Integer getSeverity() {
return severity;
}
public void setSeverity(Integer severity) {
if (severity > 6) {
this.severity = 6;
} else if (severity < 1) {
this.severity = 1;
} else {
this.severity = severity;
}
}
public Type getType() {
return type;
}
public void setType(Type type) {
this.type = type;
}
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public String getSummary() {
return summary;
}
public void setSummary(String summary) {
this.summary = summary;
}
public Date getOccurrenceTime() {
return occurrenceTime;
}
public void setOccurrenceTime(Date occurrenceTime) {
this.occurrenceTime = occurrenceTime;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy