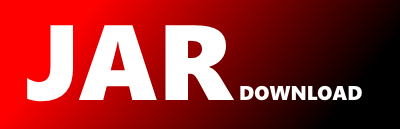
com.ibm.cp4waiops.connectors.sdk.ServiceBindingLoader Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of connectors-sdk Show documentation
Show all versions of connectors-sdk Show documentation
A developer SDK for creating connectors for CP4WAIOps.
package com.ibm.cp4waiops.connectors.sdk;
import java.io.IOException;
import java.nio.charset.StandardCharsets;
import java.nio.file.Files;
import java.nio.file.NoSuchFileException;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.util.Optional;
import java.util.logging.Level;
import java.util.logging.Logger;
/**
* A utility class for loading service bindings
*/
public class ServiceBindingLoader {
private static final Logger logger = Logger.getLogger(ServiceBindingLoader.class.getName());
String _bindingDirectory;
/**
* Constructs a ServiceBindingLoader using the standard binding directory
*/
public ServiceBindingLoader() {
_bindingDirectory = System.getenv(Constant.SERVICE_BINDING_DIR_ENV_VAR);
if (_bindingDirectory == null) {
_bindingDirectory = Constant.DEFAULT_BINDING_DIRECTORY;
}
}
/**
* Constructs a ServiceBindingLoader using the provided binding directory
*
* @param directory
*/
public ServiceBindingLoader(String directory) {
_bindingDirectory = directory;
}
/**
* Retrieves the root service binding directory
*
* @return the directory
*/
public String getRootBindingDirectory() {
return _bindingDirectory;
}
/**
* Retrieves the service binding value as a String. Throws if the value does not exists.
*
* @param bindingName
* The directory of the binding
* @param key
* The filename that will be read
*
* @return The binding value
*/
public String getString(String bindingName, String key) {
Path fileName = Paths.get(_bindingDirectory, bindingName, key);
try {
return Files.readString(fileName, StandardCharsets.UTF_8).trim();
} catch (IOException error) {
String message = "failed to read service binding: filename=" + fileName.toString();
if (!(error instanceof NoSuchFileException)) {
logger.log(Level.WARNING, message, error);
}
throw new PropertyReadException(message, error);
}
}
/**
* Retrieves the service binding value as a String if it exists.
*
* @param bindingName
* The directory of the binding
* @param key
* The filename that will be read
*
* @return The binding value
*/
public Optional getOptionalString(String bindingName, String key) {
try {
String value = getString(bindingName, key);
return Optional.of(value);
} catch (Exception error) {
return Optional.empty();
}
}
/**
* Retrieves the service binding value as an Integer. Throws if the value does not exists or cannot be parsed.
*
* @param bindingName
* The directory of the binding
* @param key
* The filename that will be read
*
* @return The binding value
*/
public Integer getInteger(String bindingName, String key) {
try {
String rawValue = getString(bindingName, key);
return Integer.valueOf(rawValue);
} catch (PropertyReadException error) {
throw error;
} catch (Exception error) {
String message = "failed to parse service binding as int: bindingName=" + bindingName + ",key=" + key;
logger.log(Level.WARNING, message, error);
throw new PropertyReadException(message, error);
}
}
/**
* Retrieves the service binding value as an Integer if it exists and can be parsed.
*
* @param bindingName
* The directory of the binding
* @param key
* The filename that will be read
*
* @return The binding value
*/
public Optional getOptionalInteger(String bindingName, String key) {
try {
Integer value = getInteger(bindingName, key);
return Optional.of(value);
} catch (Exception error) {
return Optional.empty();
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy